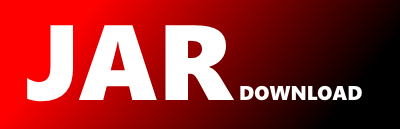
com.google.cloud.compute.v1.SavedAttachedDisk Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* DEPRECATED: Please use compute#savedDisk instead. An instance-attached disk resource.
*
*
* Protobuf type {@code google.cloud.compute.v1.SavedAttachedDisk}
*/
public final class SavedAttachedDisk extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.SavedAttachedDisk)
SavedAttachedDiskOrBuilder {
private static final long serialVersionUID = 0L;
// Use SavedAttachedDisk.newBuilder() to construct.
private SavedAttachedDisk(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SavedAttachedDisk() {
deviceName_ = "";
diskType_ = "";
guestOsFeatures_ = java.util.Collections.emptyList();
interface_ = "";
kind_ = "";
licenses_ = com.google.protobuf.LazyStringArrayList.emptyList();
mode_ = "";
source_ = "";
storageBytesStatus_ = "";
type_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new SavedAttachedDisk();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_SavedAttachedDisk_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_SavedAttachedDisk_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.SavedAttachedDisk.class,
com.google.cloud.compute.v1.SavedAttachedDisk.Builder.class);
}
/**
*
*
*
* Specifies the disk interface to use for attaching this disk, which is either SCSI or NVME.
*
*
* Protobuf enum {@code google.cloud.compute.v1.SavedAttachedDisk.Interface}
*/
public enum Interface implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_INTERFACE = 0;
*/
UNDEFINED_INTERFACE(0),
/** NVME = 2408800;
*/
NVME(2408800),
/** SCSI = 2539686;
*/
SCSI(2539686),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_INTERFACE = 0;
*/
public static final int UNDEFINED_INTERFACE_VALUE = 0;
/** NVME = 2408800;
*/
public static final int NVME_VALUE = 2408800;
/** SCSI = 2539686;
*/
public static final int SCSI_VALUE = 2539686;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Interface valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Interface forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_INTERFACE;
case 2408800:
return NVME;
case 2539686:
return SCSI;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Interface findValueByNumber(int number) {
return Interface.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.SavedAttachedDisk.getDescriptor().getEnumTypes().get(0);
}
private static final Interface[] VALUES = values();
public static Interface valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Interface(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.SavedAttachedDisk.Interface)
}
/**
*
*
*
* The mode in which this disk is attached to the source instance, either READ_WRITE or READ_ONLY.
*
*
* Protobuf enum {@code google.cloud.compute.v1.SavedAttachedDisk.Mode}
*/
public enum Mode implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_MODE = 0;
*/
UNDEFINED_MODE(0),
/**
*
*
*
* Attaches this disk in read-only mode. Multiple virtual machines can use a disk in read-only mode at a time.
*
*
* READ_ONLY = 91950261;
*/
READ_ONLY(91950261),
/**
*
*
*
* *[Default]* Attaches this disk in read-write mode. Only one virtual machine at a time can be attached to a disk in read-write mode.
*
*
* READ_WRITE = 173607894;
*/
READ_WRITE(173607894),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_MODE = 0;
*/
public static final int UNDEFINED_MODE_VALUE = 0;
/**
*
*
*
* Attaches this disk in read-only mode. Multiple virtual machines can use a disk in read-only mode at a time.
*
*
* READ_ONLY = 91950261;
*/
public static final int READ_ONLY_VALUE = 91950261;
/**
*
*
*
* *[Default]* Attaches this disk in read-write mode. Only one virtual machine at a time can be attached to a disk in read-write mode.
*
*
* READ_WRITE = 173607894;
*/
public static final int READ_WRITE_VALUE = 173607894;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Mode valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Mode forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_MODE;
case 91950261:
return READ_ONLY;
case 173607894:
return READ_WRITE;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Mode findValueByNumber(int number) {
return Mode.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.SavedAttachedDisk.getDescriptor().getEnumTypes().get(1);
}
private static final Mode[] VALUES = values();
public static Mode valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Mode(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.SavedAttachedDisk.Mode)
}
/**
*
*
*
* [Output Only] An indicator whether storageBytes is in a stable state or it is being adjusted as a result of shared storage reallocation. This status can either be UPDATING, meaning the size of the snapshot is being updated, or UP_TO_DATE, meaning the size of the snapshot is up-to-date.
*
*
* Protobuf enum {@code google.cloud.compute.v1.SavedAttachedDisk.StorageBytesStatus}
*/
public enum StorageBytesStatus implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STORAGE_BYTES_STATUS = 0;
*/
UNDEFINED_STORAGE_BYTES_STATUS(0),
/** UPDATING = 494614342;
*/
UPDATING(494614342),
/** UP_TO_DATE = 101306702;
*/
UP_TO_DATE(101306702),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STORAGE_BYTES_STATUS = 0;
*/
public static final int UNDEFINED_STORAGE_BYTES_STATUS_VALUE = 0;
/** UPDATING = 494614342;
*/
public static final int UPDATING_VALUE = 494614342;
/** UP_TO_DATE = 101306702;
*/
public static final int UP_TO_DATE_VALUE = 101306702;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static StorageBytesStatus valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static StorageBytesStatus forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_STORAGE_BYTES_STATUS;
case 494614342:
return UPDATING;
case 101306702:
return UP_TO_DATE;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public StorageBytesStatus findValueByNumber(int number) {
return StorageBytesStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.SavedAttachedDisk.getDescriptor().getEnumTypes().get(2);
}
private static final StorageBytesStatus[] VALUES = values();
public static StorageBytesStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private StorageBytesStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.SavedAttachedDisk.StorageBytesStatus)
}
/**
*
*
*
* Specifies the type of the attached disk, either SCRATCH or PERSISTENT.
*
*
* Protobuf enum {@code google.cloud.compute.v1.SavedAttachedDisk.Type}
*/
public enum Type implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_TYPE = 0;
*/
UNDEFINED_TYPE(0),
/** PERSISTENT = 460683927;
*/
PERSISTENT(460683927),
/** SCRATCH = 496778970;
*/
SCRATCH(496778970),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_TYPE = 0;
*/
public static final int UNDEFINED_TYPE_VALUE = 0;
/** PERSISTENT = 460683927;
*/
public static final int PERSISTENT_VALUE = 460683927;
/** SCRATCH = 496778970;
*/
public static final int SCRATCH_VALUE = 496778970;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Type forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_TYPE;
case 460683927:
return PERSISTENT;
case 496778970:
return SCRATCH;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.SavedAttachedDisk.getDescriptor().getEnumTypes().get(3);
}
private static final Type[] VALUES = values();
public static Type valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.SavedAttachedDisk.Type)
}
private int bitField0_;
public static final int AUTO_DELETE_FIELD_NUMBER = 464761403;
private boolean autoDelete_ = false;
/**
*
*
*
* Specifies whether the disk will be auto-deleted when the instance is deleted (but not when the disk is detached from the instance).
*
*
* optional bool auto_delete = 464761403;
*
* @return Whether the autoDelete field is set.
*/
@java.lang.Override
public boolean hasAutoDelete() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Specifies whether the disk will be auto-deleted when the instance is deleted (but not when the disk is detached from the instance).
*
*
* optional bool auto_delete = 464761403;
*
* @return The autoDelete.
*/
@java.lang.Override
public boolean getAutoDelete() {
return autoDelete_;
}
public static final int BOOT_FIELD_NUMBER = 3029746;
private boolean boot_ = false;
/**
*
*
*
* Indicates that this is a boot disk. The virtual machine will use the first partition of the disk for its root filesystem.
*
*
* optional bool boot = 3029746;
*
* @return Whether the boot field is set.
*/
@java.lang.Override
public boolean hasBoot() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Indicates that this is a boot disk. The virtual machine will use the first partition of the disk for its root filesystem.
*
*
* optional bool boot = 3029746;
*
* @return The boot.
*/
@java.lang.Override
public boolean getBoot() {
return boot_;
}
public static final int DEVICE_NAME_FIELD_NUMBER = 67541716;
@SuppressWarnings("serial")
private volatile java.lang.Object deviceName_ = "";
/**
*
*
*
* Specifies the name of the disk attached to the source instance.
*
*
* optional string device_name = 67541716;
*
* @return Whether the deviceName field is set.
*/
@java.lang.Override
public boolean hasDeviceName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Specifies the name of the disk attached to the source instance.
*
*
* optional string device_name = 67541716;
*
* @return The deviceName.
*/
@java.lang.Override
public java.lang.String getDeviceName() {
java.lang.Object ref = deviceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
deviceName_ = s;
return s;
}
}
/**
*
*
*
* Specifies the name of the disk attached to the source instance.
*
*
* optional string device_name = 67541716;
*
* @return The bytes for deviceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDeviceNameBytes() {
java.lang.Object ref = deviceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
deviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DISK_ENCRYPTION_KEY_FIELD_NUMBER = 271660677;
private com.google.cloud.compute.v1.CustomerEncryptionKey diskEncryptionKey_;
/**
*
*
*
* The encryption key for the disk.
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*
* @return Whether the diskEncryptionKey field is set.
*/
@java.lang.Override
public boolean hasDiskEncryptionKey() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The encryption key for the disk.
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*
* @return The diskEncryptionKey.
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKey getDiskEncryptionKey() {
return diskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: diskEncryptionKey_;
}
/**
*
*
*
* The encryption key for the disk.
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getDiskEncryptionKeyOrBuilder() {
return diskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: diskEncryptionKey_;
}
public static final int DISK_SIZE_GB_FIELD_NUMBER = 316263735;
private long diskSizeGb_ = 0L;
/**
*
*
*
* The size of the disk in base-2 GB.
*
*
* optional int64 disk_size_gb = 316263735;
*
* @return Whether the diskSizeGb field is set.
*/
@java.lang.Override
public boolean hasDiskSizeGb() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* The size of the disk in base-2 GB.
*
*
* optional int64 disk_size_gb = 316263735;
*
* @return The diskSizeGb.
*/
@java.lang.Override
public long getDiskSizeGb() {
return diskSizeGb_;
}
public static final int DISK_TYPE_FIELD_NUMBER = 93009052;
@SuppressWarnings("serial")
private volatile java.lang.Object diskType_ = "";
/**
*
*
*
* [Output Only] URL of the disk type resource. For example: projects/project /zones/zone/diskTypes/pd-standard or pd-ssd
*
*
* optional string disk_type = 93009052;
*
* @return Whether the diskType field is set.
*/
@java.lang.Override
public boolean hasDiskType() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* [Output Only] URL of the disk type resource. For example: projects/project /zones/zone/diskTypes/pd-standard or pd-ssd
*
*
* optional string disk_type = 93009052;
*
* @return The diskType.
*/
@java.lang.Override
public java.lang.String getDiskType() {
java.lang.Object ref = diskType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
diskType_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] URL of the disk type resource. For example: projects/project /zones/zone/diskTypes/pd-standard or pd-ssd
*
*
* optional string disk_type = 93009052;
*
* @return The bytes for diskType.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDiskTypeBytes() {
java.lang.Object ref = diskType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
diskType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GUEST_OS_FEATURES_FIELD_NUMBER = 79294545;
@SuppressWarnings("serial")
private java.util.List guestOsFeatures_;
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public java.util.List getGuestOsFeaturesList() {
return guestOsFeatures_;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
getGuestOsFeaturesOrBuilderList() {
return guestOsFeatures_;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public int getGuestOsFeaturesCount() {
return guestOsFeatures_.size();
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public com.google.cloud.compute.v1.GuestOsFeature getGuestOsFeatures(int index) {
return guestOsFeatures_.get(index);
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
@java.lang.Override
public com.google.cloud.compute.v1.GuestOsFeatureOrBuilder getGuestOsFeaturesOrBuilder(
int index) {
return guestOsFeatures_.get(index);
}
public static final int INDEX_FIELD_NUMBER = 100346066;
private int index_ = 0;
/**
*
*
*
* Specifies zero-based index of the disk that is attached to the source instance.
*
*
* optional int32 index = 100346066;
*
* @return Whether the index field is set.
*/
@java.lang.Override
public boolean hasIndex() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Specifies zero-based index of the disk that is attached to the source instance.
*
*
* optional int32 index = 100346066;
*
* @return The index.
*/
@java.lang.Override
public int getIndex() {
return index_;
}
public static final int INTERFACE_FIELD_NUMBER = 502623545;
@SuppressWarnings("serial")
private volatile java.lang.Object interface_ = "";
/**
*
*
*
* Specifies the disk interface to use for attaching this disk, which is either SCSI or NVME.
* Check the Interface enum for the list of possible values.
*
*
* optional string interface = 502623545;
*
* @return Whether the interface field is set.
*/
@java.lang.Override
public boolean hasInterface() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Specifies the disk interface to use for attaching this disk, which is either SCSI or NVME.
* Check the Interface enum for the list of possible values.
*
*
* optional string interface = 502623545;
*
* @return The interface.
*/
@java.lang.Override
public java.lang.String getInterface() {
java.lang.Object ref = interface_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
interface_ = s;
return s;
}
}
/**
*
*
*
* Specifies the disk interface to use for attaching this disk, which is either SCSI or NVME.
* Check the Interface enum for the list of possible values.
*
*
* optional string interface = 502623545;
*
* @return The bytes for interface.
*/
@java.lang.Override
public com.google.protobuf.ByteString getInterfaceBytes() {
java.lang.Object ref = interface_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
interface_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int KIND_FIELD_NUMBER = 3292052;
@SuppressWarnings("serial")
private volatile java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#attachedDisk for attached disks.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
@java.lang.Override
public boolean hasKind() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#attachedDisk for attached disks.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
@java.lang.Override
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#attachedDisk for attached disks.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LICENSES_FIELD_NUMBER = 337642578;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList licenses_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @return A list containing the licenses.
*/
public com.google.protobuf.ProtocolStringList getLicensesList() {
return licenses_;
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @return The count of licenses.
*/
public int getLicensesCount() {
return licenses_.size();
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the element to return.
* @return The licenses at the given index.
*/
public java.lang.String getLicenses(int index) {
return licenses_.get(index);
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the value to return.
* @return The bytes of the licenses at the given index.
*/
public com.google.protobuf.ByteString getLicensesBytes(int index) {
return licenses_.getByteString(index);
}
public static final int MODE_FIELD_NUMBER = 3357091;
@SuppressWarnings("serial")
private volatile java.lang.Object mode_ = "";
/**
*
*
*
* The mode in which this disk is attached to the source instance, either READ_WRITE or READ_ONLY.
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return Whether the mode field is set.
*/
@java.lang.Override
public boolean hasMode() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* The mode in which this disk is attached to the source instance, either READ_WRITE or READ_ONLY.
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return The mode.
*/
@java.lang.Override
public java.lang.String getMode() {
java.lang.Object ref = mode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mode_ = s;
return s;
}
}
/**
*
*
*
* The mode in which this disk is attached to the source instance, either READ_WRITE or READ_ONLY.
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return The bytes for mode.
*/
@java.lang.Override
public com.google.protobuf.ByteString getModeBytes() {
java.lang.Object ref = mode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
mode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_FIELD_NUMBER = 177235995;
@SuppressWarnings("serial")
private volatile java.lang.Object source_ = "";
/**
*
*
*
* Specifies a URL of the disk attached to the source instance.
*
*
* optional string source = 177235995;
*
* @return Whether the source field is set.
*/
@java.lang.Override
public boolean hasSource() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Specifies a URL of the disk attached to the source instance.
*
*
* optional string source = 177235995;
*
* @return The source.
*/
@java.lang.Override
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
source_ = s;
return s;
}
}
/**
*
*
*
* Specifies a URL of the disk attached to the source instance.
*
*
* optional string source = 177235995;
*
* @return The bytes for source.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STORAGE_BYTES_FIELD_NUMBER = 424631719;
private long storageBytes_ = 0L;
/**
*
*
*
* [Output Only] A size of the storage used by the disk's snapshot by this machine image.
*
*
* optional int64 storage_bytes = 424631719;
*
* @return Whether the storageBytes field is set.
*/
@java.lang.Override
public boolean hasStorageBytes() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* [Output Only] A size of the storage used by the disk's snapshot by this machine image.
*
*
* optional int64 storage_bytes = 424631719;
*
* @return The storageBytes.
*/
@java.lang.Override
public long getStorageBytes() {
return storageBytes_;
}
public static final int STORAGE_BYTES_STATUS_FIELD_NUMBER = 490739082;
@SuppressWarnings("serial")
private volatile java.lang.Object storageBytesStatus_ = "";
/**
*
*
*
* [Output Only] An indicator whether storageBytes is in a stable state or it is being adjusted as a result of shared storage reallocation. This status can either be UPDATING, meaning the size of the snapshot is being updated, or UP_TO_DATE, meaning the size of the snapshot is up-to-date.
* Check the StorageBytesStatus enum for the list of possible values.
*
*
* optional string storage_bytes_status = 490739082;
*
* @return Whether the storageBytesStatus field is set.
*/
@java.lang.Override
public boolean hasStorageBytesStatus() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* [Output Only] An indicator whether storageBytes is in a stable state or it is being adjusted as a result of shared storage reallocation. This status can either be UPDATING, meaning the size of the snapshot is being updated, or UP_TO_DATE, meaning the size of the snapshot is up-to-date.
* Check the StorageBytesStatus enum for the list of possible values.
*
*
* optional string storage_bytes_status = 490739082;
*
* @return The storageBytesStatus.
*/
@java.lang.Override
public java.lang.String getStorageBytesStatus() {
java.lang.Object ref = storageBytesStatus_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
storageBytesStatus_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] An indicator whether storageBytes is in a stable state or it is being adjusted as a result of shared storage reallocation. This status can either be UPDATING, meaning the size of the snapshot is being updated, or UP_TO_DATE, meaning the size of the snapshot is up-to-date.
* Check the StorageBytesStatus enum for the list of possible values.
*
*
* optional string storage_bytes_status = 490739082;
*
* @return The bytes for storageBytesStatus.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStorageBytesStatusBytes() {
java.lang.Object ref = storageBytesStatus_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
storageBytesStatus_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 3575610;
@SuppressWarnings("serial")
private volatile java.lang.Object type_ = "";
/**
*
*
*
* Specifies the type of the attached disk, either SCRATCH or PERSISTENT.
* Check the Type enum for the list of possible values.
*
*
* optional string type = 3575610;
*
* @return Whether the type field is set.
*/
@java.lang.Override
public boolean hasType() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*
*
* Specifies the type of the attached disk, either SCRATCH or PERSISTENT.
* Check the Type enum for the list of possible values.
*
*
* optional string type = 3575610;
*
* @return The type.
*/
@java.lang.Override
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
}
}
/**
*
*
*
* Specifies the type of the attached disk, either SCRATCH or PERSISTENT.
* Check the Type enum for the list of possible values.
*
*
* optional string type = 3575610;
*
* @return The bytes for type.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000002) != 0)) {
output.writeBool(3029746, boot_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3292052, kind_);
}
if (((bitField0_ & 0x00000200) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3357091, mode_);
}
if (((bitField0_ & 0x00002000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3575610, type_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 67541716, deviceName_);
}
for (int i = 0; i < guestOsFeatures_.size(); i++) {
output.writeMessage(79294545, guestOsFeatures_.get(i));
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 93009052, diskType_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeInt32(100346066, index_);
}
if (((bitField0_ & 0x00000400) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 177235995, source_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(271660677, getDiskEncryptionKey());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt64(316263735, diskSizeGb_);
}
for (int i = 0; i < licenses_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 337642578, licenses_.getRaw(i));
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeInt64(424631719, storageBytes_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBool(464761403, autoDelete_);
}
if (((bitField0_ & 0x00001000) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 490739082, storageBytesStatus_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 502623545, interface_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(3029746, boot_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3292052, kind_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3357091, mode_);
}
if (((bitField0_ & 0x00002000) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3575610, type_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(67541716, deviceName_);
}
for (int i = 0; i < guestOsFeatures_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
79294545, guestOsFeatures_.get(i));
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(93009052, diskType_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(100346066, index_);
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(177235995, source_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
271660677, getDiskEncryptionKey());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(316263735, diskSizeGb_);
}
{
int dataSize = 0;
for (int i = 0; i < licenses_.size(); i++) {
dataSize += computeStringSizeNoTag(licenses_.getRaw(i));
}
size += dataSize;
size += 5 * getLicensesList().size();
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(424631719, storageBytes_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(464761403, autoDelete_);
}
if (((bitField0_ & 0x00001000) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(490739082, storageBytesStatus_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(502623545, interface_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.SavedAttachedDisk)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.SavedAttachedDisk other =
(com.google.cloud.compute.v1.SavedAttachedDisk) obj;
if (hasAutoDelete() != other.hasAutoDelete()) return false;
if (hasAutoDelete()) {
if (getAutoDelete() != other.getAutoDelete()) return false;
}
if (hasBoot() != other.hasBoot()) return false;
if (hasBoot()) {
if (getBoot() != other.getBoot()) return false;
}
if (hasDeviceName() != other.hasDeviceName()) return false;
if (hasDeviceName()) {
if (!getDeviceName().equals(other.getDeviceName())) return false;
}
if (hasDiskEncryptionKey() != other.hasDiskEncryptionKey()) return false;
if (hasDiskEncryptionKey()) {
if (!getDiskEncryptionKey().equals(other.getDiskEncryptionKey())) return false;
}
if (hasDiskSizeGb() != other.hasDiskSizeGb()) return false;
if (hasDiskSizeGb()) {
if (getDiskSizeGb() != other.getDiskSizeGb()) return false;
}
if (hasDiskType() != other.hasDiskType()) return false;
if (hasDiskType()) {
if (!getDiskType().equals(other.getDiskType())) return false;
}
if (!getGuestOsFeaturesList().equals(other.getGuestOsFeaturesList())) return false;
if (hasIndex() != other.hasIndex()) return false;
if (hasIndex()) {
if (getIndex() != other.getIndex()) return false;
}
if (hasInterface() != other.hasInterface()) return false;
if (hasInterface()) {
if (!getInterface().equals(other.getInterface())) return false;
}
if (hasKind() != other.hasKind()) return false;
if (hasKind()) {
if (!getKind().equals(other.getKind())) return false;
}
if (!getLicensesList().equals(other.getLicensesList())) return false;
if (hasMode() != other.hasMode()) return false;
if (hasMode()) {
if (!getMode().equals(other.getMode())) return false;
}
if (hasSource() != other.hasSource()) return false;
if (hasSource()) {
if (!getSource().equals(other.getSource())) return false;
}
if (hasStorageBytes() != other.hasStorageBytes()) return false;
if (hasStorageBytes()) {
if (getStorageBytes() != other.getStorageBytes()) return false;
}
if (hasStorageBytesStatus() != other.hasStorageBytesStatus()) return false;
if (hasStorageBytesStatus()) {
if (!getStorageBytesStatus().equals(other.getStorageBytesStatus())) return false;
}
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (!getType().equals(other.getType())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAutoDelete()) {
hash = (37 * hash) + AUTO_DELETE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getAutoDelete());
}
if (hasBoot()) {
hash = (37 * hash) + BOOT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getBoot());
}
if (hasDeviceName()) {
hash = (37 * hash) + DEVICE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getDeviceName().hashCode();
}
if (hasDiskEncryptionKey()) {
hash = (37 * hash) + DISK_ENCRYPTION_KEY_FIELD_NUMBER;
hash = (53 * hash) + getDiskEncryptionKey().hashCode();
}
if (hasDiskSizeGb()) {
hash = (37 * hash) + DISK_SIZE_GB_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getDiskSizeGb());
}
if (hasDiskType()) {
hash = (37 * hash) + DISK_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getDiskType().hashCode();
}
if (getGuestOsFeaturesCount() > 0) {
hash = (37 * hash) + GUEST_OS_FEATURES_FIELD_NUMBER;
hash = (53 * hash) + getGuestOsFeaturesList().hashCode();
}
if (hasIndex()) {
hash = (37 * hash) + INDEX_FIELD_NUMBER;
hash = (53 * hash) + getIndex();
}
if (hasInterface()) {
hash = (37 * hash) + INTERFACE_FIELD_NUMBER;
hash = (53 * hash) + getInterface().hashCode();
}
if (hasKind()) {
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + getKind().hashCode();
}
if (getLicensesCount() > 0) {
hash = (37 * hash) + LICENSES_FIELD_NUMBER;
hash = (53 * hash) + getLicensesList().hashCode();
}
if (hasMode()) {
hash = (37 * hash) + MODE_FIELD_NUMBER;
hash = (53 * hash) + getMode().hashCode();
}
if (hasSource()) {
hash = (37 * hash) + SOURCE_FIELD_NUMBER;
hash = (53 * hash) + getSource().hashCode();
}
if (hasStorageBytes()) {
hash = (37 * hash) + STORAGE_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getStorageBytes());
}
if (hasStorageBytesStatus()) {
hash = (37 * hash) + STORAGE_BYTES_STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStorageBytesStatus().hashCode();
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.SavedAttachedDisk parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.SavedAttachedDisk prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* DEPRECATED: Please use compute#savedDisk instead. An instance-attached disk resource.
*
*
* Protobuf type {@code google.cloud.compute.v1.SavedAttachedDisk}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.SavedAttachedDisk)
com.google.cloud.compute.v1.SavedAttachedDiskOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_SavedAttachedDisk_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_SavedAttachedDisk_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.SavedAttachedDisk.class,
com.google.cloud.compute.v1.SavedAttachedDisk.Builder.class);
}
// Construct using com.google.cloud.compute.v1.SavedAttachedDisk.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getDiskEncryptionKeyFieldBuilder();
getGuestOsFeaturesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
autoDelete_ = false;
boot_ = false;
deviceName_ = "";
diskEncryptionKey_ = null;
if (diskEncryptionKeyBuilder_ != null) {
diskEncryptionKeyBuilder_.dispose();
diskEncryptionKeyBuilder_ = null;
}
diskSizeGb_ = 0L;
diskType_ = "";
if (guestOsFeaturesBuilder_ == null) {
guestOsFeatures_ = java.util.Collections.emptyList();
} else {
guestOsFeatures_ = null;
guestOsFeaturesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
index_ = 0;
interface_ = "";
kind_ = "";
licenses_ = com.google.protobuf.LazyStringArrayList.emptyList();
mode_ = "";
source_ = "";
storageBytes_ = 0L;
storageBytesStatus_ = "";
type_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_SavedAttachedDisk_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.SavedAttachedDisk getDefaultInstanceForType() {
return com.google.cloud.compute.v1.SavedAttachedDisk.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.SavedAttachedDisk build() {
com.google.cloud.compute.v1.SavedAttachedDisk result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.SavedAttachedDisk buildPartial() {
com.google.cloud.compute.v1.SavedAttachedDisk result =
new com.google.cloud.compute.v1.SavedAttachedDisk(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.cloud.compute.v1.SavedAttachedDisk result) {
if (guestOsFeaturesBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
guestOsFeatures_ = java.util.Collections.unmodifiableList(guestOsFeatures_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.guestOsFeatures_ = guestOsFeatures_;
} else {
result.guestOsFeatures_ = guestOsFeaturesBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.compute.v1.SavedAttachedDisk result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.autoDelete_ = autoDelete_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.boot_ = boot_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.deviceName_ = deviceName_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.diskEncryptionKey_ =
diskEncryptionKeyBuilder_ == null
? diskEncryptionKey_
: diskEncryptionKeyBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.diskSizeGb_ = diskSizeGb_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.diskType_ = diskType_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.index_ = index_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.interface_ = interface_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.kind_ = kind_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
licenses_.makeImmutable();
result.licenses_ = licenses_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.mode_ = mode_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.source_ = source_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.storageBytes_ = storageBytes_;
to_bitField0_ |= 0x00000800;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.storageBytesStatus_ = storageBytesStatus_;
to_bitField0_ |= 0x00001000;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.type_ = type_;
to_bitField0_ |= 0x00002000;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.SavedAttachedDisk) {
return mergeFrom((com.google.cloud.compute.v1.SavedAttachedDisk) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.SavedAttachedDisk other) {
if (other == com.google.cloud.compute.v1.SavedAttachedDisk.getDefaultInstance()) return this;
if (other.hasAutoDelete()) {
setAutoDelete(other.getAutoDelete());
}
if (other.hasBoot()) {
setBoot(other.getBoot());
}
if (other.hasDeviceName()) {
deviceName_ = other.deviceName_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasDiskEncryptionKey()) {
mergeDiskEncryptionKey(other.getDiskEncryptionKey());
}
if (other.hasDiskSizeGb()) {
setDiskSizeGb(other.getDiskSizeGb());
}
if (other.hasDiskType()) {
diskType_ = other.diskType_;
bitField0_ |= 0x00000020;
onChanged();
}
if (guestOsFeaturesBuilder_ == null) {
if (!other.guestOsFeatures_.isEmpty()) {
if (guestOsFeatures_.isEmpty()) {
guestOsFeatures_ = other.guestOsFeatures_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.addAll(other.guestOsFeatures_);
}
onChanged();
}
} else {
if (!other.guestOsFeatures_.isEmpty()) {
if (guestOsFeaturesBuilder_.isEmpty()) {
guestOsFeaturesBuilder_.dispose();
guestOsFeaturesBuilder_ = null;
guestOsFeatures_ = other.guestOsFeatures_;
bitField0_ = (bitField0_ & ~0x00000040);
guestOsFeaturesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getGuestOsFeaturesFieldBuilder()
: null;
} else {
guestOsFeaturesBuilder_.addAllMessages(other.guestOsFeatures_);
}
}
}
if (other.hasIndex()) {
setIndex(other.getIndex());
}
if (other.hasInterface()) {
interface_ = other.interface_;
bitField0_ |= 0x00000100;
onChanged();
}
if (other.hasKind()) {
kind_ = other.kind_;
bitField0_ |= 0x00000200;
onChanged();
}
if (!other.licenses_.isEmpty()) {
if (licenses_.isEmpty()) {
licenses_ = other.licenses_;
bitField0_ |= 0x00000400;
} else {
ensureLicensesIsMutable();
licenses_.addAll(other.licenses_);
}
onChanged();
}
if (other.hasMode()) {
mode_ = other.mode_;
bitField0_ |= 0x00000800;
onChanged();
}
if (other.hasSource()) {
source_ = other.source_;
bitField0_ |= 0x00001000;
onChanged();
}
if (other.hasStorageBytes()) {
setStorageBytes(other.getStorageBytes());
}
if (other.hasStorageBytesStatus()) {
storageBytesStatus_ = other.storageBytesStatus_;
bitField0_ |= 0x00004000;
onChanged();
}
if (other.hasType()) {
type_ = other.type_;
bitField0_ |= 0x00008000;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 24237968:
{
boot_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 24237968
case 26336418:
{
kind_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case 26336418
case 26856730:
{
mode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000800;
break;
} // case 26856730
case 28604882:
{
type_ = input.readStringRequireUtf8();
bitField0_ |= 0x00008000;
break;
} // case 28604882
case 540333730:
{
deviceName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 540333730
case 634356362:
{
com.google.cloud.compute.v1.GuestOsFeature m =
input.readMessage(
com.google.cloud.compute.v1.GuestOsFeature.parser(), extensionRegistry);
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(m);
} else {
guestOsFeaturesBuilder_.addMessage(m);
}
break;
} // case 634356362
case 744072418:
{
diskType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 744072418
case 802768528:
{
index_ = input.readInt32();
bitField0_ |= 0x00000080;
break;
} // case 802768528
case 1417887962:
{
source_ = input.readStringRequireUtf8();
bitField0_ |= 0x00001000;
break;
} // case 1417887962
case -2121681878:
{
input.readMessage(
getDiskEncryptionKeyFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case -2121681878
case -1764857416:
{
diskSizeGb_ = input.readInt64();
bitField0_ |= 0x00000010;
break;
} // case -1764857416
case -1593826670:
{
java.lang.String s = input.readStringRequireUtf8();
ensureLicensesIsMutable();
licenses_.add(s);
break;
} // case -1593826670
case -897913544:
{
storageBytes_ = input.readInt64();
bitField0_ |= 0x00002000;
break;
} // case -897913544
case -576876072:
{
autoDelete_ = input.readBool();
bitField0_ |= 0x00000001;
break;
} // case -576876072
case -369054638:
{
storageBytesStatus_ = input.readStringRequireUtf8();
bitField0_ |= 0x00004000;
break;
} // case -369054638
case -273978934:
{
interface_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case -273978934
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private boolean autoDelete_;
/**
*
*
*
* Specifies whether the disk will be auto-deleted when the instance is deleted (but not when the disk is detached from the instance).
*
*
* optional bool auto_delete = 464761403;
*
* @return Whether the autoDelete field is set.
*/
@java.lang.Override
public boolean hasAutoDelete() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Specifies whether the disk will be auto-deleted when the instance is deleted (but not when the disk is detached from the instance).
*
*
* optional bool auto_delete = 464761403;
*
* @return The autoDelete.
*/
@java.lang.Override
public boolean getAutoDelete() {
return autoDelete_;
}
/**
*
*
*
* Specifies whether the disk will be auto-deleted when the instance is deleted (but not when the disk is detached from the instance).
*
*
* optional bool auto_delete = 464761403;
*
* @param value The autoDelete to set.
* @return This builder for chaining.
*/
public Builder setAutoDelete(boolean value) {
autoDelete_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Specifies whether the disk will be auto-deleted when the instance is deleted (but not when the disk is detached from the instance).
*
*
* optional bool auto_delete = 464761403;
*
* @return This builder for chaining.
*/
public Builder clearAutoDelete() {
bitField0_ = (bitField0_ & ~0x00000001);
autoDelete_ = false;
onChanged();
return this;
}
private boolean boot_;
/**
*
*
*
* Indicates that this is a boot disk. The virtual machine will use the first partition of the disk for its root filesystem.
*
*
* optional bool boot = 3029746;
*
* @return Whether the boot field is set.
*/
@java.lang.Override
public boolean hasBoot() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Indicates that this is a boot disk. The virtual machine will use the first partition of the disk for its root filesystem.
*
*
* optional bool boot = 3029746;
*
* @return The boot.
*/
@java.lang.Override
public boolean getBoot() {
return boot_;
}
/**
*
*
*
* Indicates that this is a boot disk. The virtual machine will use the first partition of the disk for its root filesystem.
*
*
* optional bool boot = 3029746;
*
* @param value The boot to set.
* @return This builder for chaining.
*/
public Builder setBoot(boolean value) {
boot_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Indicates that this is a boot disk. The virtual machine will use the first partition of the disk for its root filesystem.
*
*
* optional bool boot = 3029746;
*
* @return This builder for chaining.
*/
public Builder clearBoot() {
bitField0_ = (bitField0_ & ~0x00000002);
boot_ = false;
onChanged();
return this;
}
private java.lang.Object deviceName_ = "";
/**
*
*
*
* Specifies the name of the disk attached to the source instance.
*
*
* optional string device_name = 67541716;
*
* @return Whether the deviceName field is set.
*/
public boolean hasDeviceName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Specifies the name of the disk attached to the source instance.
*
*
* optional string device_name = 67541716;
*
* @return The deviceName.
*/
public java.lang.String getDeviceName() {
java.lang.Object ref = deviceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
deviceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Specifies the name of the disk attached to the source instance.
*
*
* optional string device_name = 67541716;
*
* @return The bytes for deviceName.
*/
public com.google.protobuf.ByteString getDeviceNameBytes() {
java.lang.Object ref = deviceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
deviceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Specifies the name of the disk attached to the source instance.
*
*
* optional string device_name = 67541716;
*
* @param value The deviceName to set.
* @return This builder for chaining.
*/
public Builder setDeviceName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
deviceName_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Specifies the name of the disk attached to the source instance.
*
*
* optional string device_name = 67541716;
*
* @return This builder for chaining.
*/
public Builder clearDeviceName() {
deviceName_ = getDefaultInstance().getDeviceName();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* Specifies the name of the disk attached to the source instance.
*
*
* optional string device_name = 67541716;
*
* @param value The bytes for deviceName to set.
* @return This builder for chaining.
*/
public Builder setDeviceNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
deviceName_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.google.cloud.compute.v1.CustomerEncryptionKey diskEncryptionKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
diskEncryptionKeyBuilder_;
/**
*
*
*
* The encryption key for the disk.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*
* @return Whether the diskEncryptionKey field is set.
*/
public boolean hasDiskEncryptionKey() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The encryption key for the disk.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*
* @return The diskEncryptionKey.
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey getDiskEncryptionKey() {
if (diskEncryptionKeyBuilder_ == null) {
return diskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: diskEncryptionKey_;
} else {
return diskEncryptionKeyBuilder_.getMessage();
}
}
/**
*
*
*
* The encryption key for the disk.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public Builder setDiskEncryptionKey(com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (diskEncryptionKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
diskEncryptionKey_ = value;
} else {
diskEncryptionKeyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* The encryption key for the disk.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public Builder setDiskEncryptionKey(
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder builderForValue) {
if (diskEncryptionKeyBuilder_ == null) {
diskEncryptionKey_ = builderForValue.build();
} else {
diskEncryptionKeyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* The encryption key for the disk.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public Builder mergeDiskEncryptionKey(com.google.cloud.compute.v1.CustomerEncryptionKey value) {
if (diskEncryptionKeyBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& diskEncryptionKey_ != null
&& diskEncryptionKey_
!= com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()) {
getDiskEncryptionKeyBuilder().mergeFrom(value);
} else {
diskEncryptionKey_ = value;
}
} else {
diskEncryptionKeyBuilder_.mergeFrom(value);
}
if (diskEncryptionKey_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* The encryption key for the disk.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public Builder clearDiskEncryptionKey() {
bitField0_ = (bitField0_ & ~0x00000008);
diskEncryptionKey_ = null;
if (diskEncryptionKeyBuilder_ != null) {
diskEncryptionKeyBuilder_.dispose();
diskEncryptionKeyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The encryption key for the disk.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKey.Builder getDiskEncryptionKeyBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getDiskEncryptionKeyFieldBuilder().getBuilder();
}
/**
*
*
*
* The encryption key for the disk.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
public com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder
getDiskEncryptionKeyOrBuilder() {
if (diskEncryptionKeyBuilder_ != null) {
return diskEncryptionKeyBuilder_.getMessageOrBuilder();
} else {
return diskEncryptionKey_ == null
? com.google.cloud.compute.v1.CustomerEncryptionKey.getDefaultInstance()
: diskEncryptionKey_;
}
}
/**
*
*
*
* The encryption key for the disk.
*
*
*
* optional .google.cloud.compute.v1.CustomerEncryptionKey disk_encryption_key = 271660677;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>
getDiskEncryptionKeyFieldBuilder() {
if (diskEncryptionKeyBuilder_ == null) {
diskEncryptionKeyBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.CustomerEncryptionKey,
com.google.cloud.compute.v1.CustomerEncryptionKey.Builder,
com.google.cloud.compute.v1.CustomerEncryptionKeyOrBuilder>(
getDiskEncryptionKey(), getParentForChildren(), isClean());
diskEncryptionKey_ = null;
}
return diskEncryptionKeyBuilder_;
}
private long diskSizeGb_;
/**
*
*
*
* The size of the disk in base-2 GB.
*
*
* optional int64 disk_size_gb = 316263735;
*
* @return Whether the diskSizeGb field is set.
*/
@java.lang.Override
public boolean hasDiskSizeGb() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* The size of the disk in base-2 GB.
*
*
* optional int64 disk_size_gb = 316263735;
*
* @return The diskSizeGb.
*/
@java.lang.Override
public long getDiskSizeGb() {
return diskSizeGb_;
}
/**
*
*
*
* The size of the disk in base-2 GB.
*
*
* optional int64 disk_size_gb = 316263735;
*
* @param value The diskSizeGb to set.
* @return This builder for chaining.
*/
public Builder setDiskSizeGb(long value) {
diskSizeGb_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* The size of the disk in base-2 GB.
*
*
* optional int64 disk_size_gb = 316263735;
*
* @return This builder for chaining.
*/
public Builder clearDiskSizeGb() {
bitField0_ = (bitField0_ & ~0x00000010);
diskSizeGb_ = 0L;
onChanged();
return this;
}
private java.lang.Object diskType_ = "";
/**
*
*
*
* [Output Only] URL of the disk type resource. For example: projects/project /zones/zone/diskTypes/pd-standard or pd-ssd
*
*
* optional string disk_type = 93009052;
*
* @return Whether the diskType field is set.
*/
public boolean hasDiskType() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* [Output Only] URL of the disk type resource. For example: projects/project /zones/zone/diskTypes/pd-standard or pd-ssd
*
*
* optional string disk_type = 93009052;
*
* @return The diskType.
*/
public java.lang.String getDiskType() {
java.lang.Object ref = diskType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
diskType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] URL of the disk type resource. For example: projects/project /zones/zone/diskTypes/pd-standard or pd-ssd
*
*
* optional string disk_type = 93009052;
*
* @return The bytes for diskType.
*/
public com.google.protobuf.ByteString getDiskTypeBytes() {
java.lang.Object ref = diskType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
diskType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] URL of the disk type resource. For example: projects/project /zones/zone/diskTypes/pd-standard or pd-ssd
*
*
* optional string disk_type = 93009052;
*
* @param value The diskType to set.
* @return This builder for chaining.
*/
public Builder setDiskType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
diskType_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] URL of the disk type resource. For example: projects/project /zones/zone/diskTypes/pd-standard or pd-ssd
*
*
* optional string disk_type = 93009052;
*
* @return This builder for chaining.
*/
public Builder clearDiskType() {
diskType_ = getDefaultInstance().getDiskType();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] URL of the disk type resource. For example: projects/project /zones/zone/diskTypes/pd-standard or pd-ssd
*
*
* optional string disk_type = 93009052;
*
* @param value The bytes for diskType to set.
* @return This builder for chaining.
*/
public Builder setDiskTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
diskType_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.util.List guestOsFeatures_ =
java.util.Collections.emptyList();
private void ensureGuestOsFeaturesIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
guestOsFeatures_ =
new java.util.ArrayList(guestOsFeatures_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.GuestOsFeature,
com.google.cloud.compute.v1.GuestOsFeature.Builder,
com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
guestOsFeaturesBuilder_;
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public java.util.List getGuestOsFeaturesList() {
if (guestOsFeaturesBuilder_ == null) {
return java.util.Collections.unmodifiableList(guestOsFeatures_);
} else {
return guestOsFeaturesBuilder_.getMessageList();
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public int getGuestOsFeaturesCount() {
if (guestOsFeaturesBuilder_ == null) {
return guestOsFeatures_.size();
} else {
return guestOsFeaturesBuilder_.getCount();
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature getGuestOsFeatures(int index) {
if (guestOsFeaturesBuilder_ == null) {
return guestOsFeatures_.get(index);
} else {
return guestOsFeaturesBuilder_.getMessage(index);
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder setGuestOsFeatures(int index, com.google.cloud.compute.v1.GuestOsFeature value) {
if (guestOsFeaturesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.set(index, value);
onChanged();
} else {
guestOsFeaturesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder setGuestOsFeatures(
int index, com.google.cloud.compute.v1.GuestOsFeature.Builder builderForValue) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.set(index, builderForValue.build());
onChanged();
} else {
guestOsFeaturesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(com.google.cloud.compute.v1.GuestOsFeature value) {
if (guestOsFeaturesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(value);
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(int index, com.google.cloud.compute.v1.GuestOsFeature value) {
if (guestOsFeaturesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(index, value);
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(
com.google.cloud.compute.v1.GuestOsFeature.Builder builderForValue) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(builderForValue.build());
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addGuestOsFeatures(
int index, com.google.cloud.compute.v1.GuestOsFeature.Builder builderForValue) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.add(index, builderForValue.build());
onChanged();
} else {
guestOsFeaturesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder addAllGuestOsFeatures(
java.lang.Iterable extends com.google.cloud.compute.v1.GuestOsFeature> values) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, guestOsFeatures_);
onChanged();
} else {
guestOsFeaturesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder clearGuestOsFeatures() {
if (guestOsFeaturesBuilder_ == null) {
guestOsFeatures_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
guestOsFeaturesBuilder_.clear();
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public Builder removeGuestOsFeatures(int index) {
if (guestOsFeaturesBuilder_ == null) {
ensureGuestOsFeaturesIsMutable();
guestOsFeatures_.remove(index);
onChanged();
} else {
guestOsFeaturesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature.Builder getGuestOsFeaturesBuilder(int index) {
return getGuestOsFeaturesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeatureOrBuilder getGuestOsFeaturesOrBuilder(
int index) {
if (guestOsFeaturesBuilder_ == null) {
return guestOsFeatures_.get(index);
} else {
return guestOsFeaturesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public java.util.List extends com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
getGuestOsFeaturesOrBuilderList() {
if (guestOsFeaturesBuilder_ != null) {
return guestOsFeaturesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(guestOsFeatures_);
}
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature.Builder addGuestOsFeaturesBuilder() {
return getGuestOsFeaturesFieldBuilder()
.addBuilder(com.google.cloud.compute.v1.GuestOsFeature.getDefaultInstance());
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public com.google.cloud.compute.v1.GuestOsFeature.Builder addGuestOsFeaturesBuilder(int index) {
return getGuestOsFeaturesFieldBuilder()
.addBuilder(index, com.google.cloud.compute.v1.GuestOsFeature.getDefaultInstance());
}
/**
*
*
*
* A list of features to enable on the guest operating system. Applicable only for bootable images. Read Enabling guest operating system features to see a list of available options.
*
*
* repeated .google.cloud.compute.v1.GuestOsFeature guest_os_features = 79294545;
*/
public java.util.List
getGuestOsFeaturesBuilderList() {
return getGuestOsFeaturesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.GuestOsFeature,
com.google.cloud.compute.v1.GuestOsFeature.Builder,
com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>
getGuestOsFeaturesFieldBuilder() {
if (guestOsFeaturesBuilder_ == null) {
guestOsFeaturesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.GuestOsFeature,
com.google.cloud.compute.v1.GuestOsFeature.Builder,
com.google.cloud.compute.v1.GuestOsFeatureOrBuilder>(
guestOsFeatures_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
guestOsFeatures_ = null;
}
return guestOsFeaturesBuilder_;
}
private int index_;
/**
*
*
*
* Specifies zero-based index of the disk that is attached to the source instance.
*
*
* optional int32 index = 100346066;
*
* @return Whether the index field is set.
*/
@java.lang.Override
public boolean hasIndex() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Specifies zero-based index of the disk that is attached to the source instance.
*
*
* optional int32 index = 100346066;
*
* @return The index.
*/
@java.lang.Override
public int getIndex() {
return index_;
}
/**
*
*
*
* Specifies zero-based index of the disk that is attached to the source instance.
*
*
* optional int32 index = 100346066;
*
* @param value The index to set.
* @return This builder for chaining.
*/
public Builder setIndex(int value) {
index_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Specifies zero-based index of the disk that is attached to the source instance.
*
*
* optional int32 index = 100346066;
*
* @return This builder for chaining.
*/
public Builder clearIndex() {
bitField0_ = (bitField0_ & ~0x00000080);
index_ = 0;
onChanged();
return this;
}
private java.lang.Object interface_ = "";
/**
*
*
*
* Specifies the disk interface to use for attaching this disk, which is either SCSI or NVME.
* Check the Interface enum for the list of possible values.
*
*
* optional string interface = 502623545;
*
* @return Whether the interface field is set.
*/
public boolean hasInterface() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Specifies the disk interface to use for attaching this disk, which is either SCSI or NVME.
* Check the Interface enum for the list of possible values.
*
*
* optional string interface = 502623545;
*
* @return The interface.
*/
public java.lang.String getInterface() {
java.lang.Object ref = interface_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
interface_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Specifies the disk interface to use for attaching this disk, which is either SCSI or NVME.
* Check the Interface enum for the list of possible values.
*
*
* optional string interface = 502623545;
*
* @return The bytes for interface.
*/
public com.google.protobuf.ByteString getInterfaceBytes() {
java.lang.Object ref = interface_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
interface_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Specifies the disk interface to use for attaching this disk, which is either SCSI or NVME.
* Check the Interface enum for the list of possible values.
*
*
* optional string interface = 502623545;
*
* @param value The interface to set.
* @return This builder for chaining.
*/
public Builder setInterface(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
interface_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Specifies the disk interface to use for attaching this disk, which is either SCSI or NVME.
* Check the Interface enum for the list of possible values.
*
*
* optional string interface = 502623545;
*
* @return This builder for chaining.
*/
public Builder clearInterface() {
interface_ = getDefaultInstance().getInterface();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
*
*
*
* Specifies the disk interface to use for attaching this disk, which is either SCSI or NVME.
* Check the Interface enum for the list of possible values.
*
*
* optional string interface = 502623545;
*
* @param value The bytes for interface to set.
* @return This builder for chaining.
*/
public Builder setInterfaceBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
interface_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#attachedDisk for attached disks.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
public boolean hasKind() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#attachedDisk for attached disks.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#attachedDisk for attached disks.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#attachedDisk for attached disks.
*
*
* optional string kind = 3292052;
*
* @param value The kind to set.
* @return This builder for chaining.
*/
public Builder setKind(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#attachedDisk for attached disks.
*
*
* optional string kind = 3292052;
*
* @return This builder for chaining.
*/
public Builder clearKind() {
kind_ = getDefaultInstance().getKind();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#attachedDisk for attached disks.
*
*
* optional string kind = 3292052;
*
* @param value The bytes for kind to set.
* @return This builder for chaining.
*/
public Builder setKindBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
kind_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList licenses_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureLicensesIsMutable() {
if (!licenses_.isModifiable()) {
licenses_ = new com.google.protobuf.LazyStringArrayList(licenses_);
}
bitField0_ |= 0x00000400;
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @return A list containing the licenses.
*/
public com.google.protobuf.ProtocolStringList getLicensesList() {
licenses_.makeImmutable();
return licenses_;
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @return The count of licenses.
*/
public int getLicensesCount() {
return licenses_.size();
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the element to return.
* @return The licenses at the given index.
*/
public java.lang.String getLicenses(int index) {
return licenses_.get(index);
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @param index The index of the value to return.
* @return The bytes of the licenses at the given index.
*/
public com.google.protobuf.ByteString getLicensesBytes(int index) {
return licenses_.getByteString(index);
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @param index The index to set the value at.
* @param value The licenses to set.
* @return This builder for chaining.
*/
public Builder setLicenses(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLicensesIsMutable();
licenses_.set(index, value);
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @param value The licenses to add.
* @return This builder for chaining.
*/
public Builder addLicenses(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureLicensesIsMutable();
licenses_.add(value);
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @param values The licenses to add.
* @return This builder for chaining.
*/
public Builder addAllLicenses(java.lang.Iterable values) {
ensureLicensesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, licenses_);
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @return This builder for chaining.
*/
public Builder clearLicenses() {
licenses_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000400);
;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Any valid publicly visible licenses.
*
*
* repeated string licenses = 337642578;
*
* @param value The bytes of the licenses to add.
* @return This builder for chaining.
*/
public Builder addLicensesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureLicensesIsMutable();
licenses_.add(value);
bitField0_ |= 0x00000400;
onChanged();
return this;
}
private java.lang.Object mode_ = "";
/**
*
*
*
* The mode in which this disk is attached to the source instance, either READ_WRITE or READ_ONLY.
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return Whether the mode field is set.
*/
public boolean hasMode() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* The mode in which this disk is attached to the source instance, either READ_WRITE or READ_ONLY.
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return The mode.
*/
public java.lang.String getMode() {
java.lang.Object ref = mode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
mode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The mode in which this disk is attached to the source instance, either READ_WRITE or READ_ONLY.
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return The bytes for mode.
*/
public com.google.protobuf.ByteString getModeBytes() {
java.lang.Object ref = mode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
mode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The mode in which this disk is attached to the source instance, either READ_WRITE or READ_ONLY.
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @param value The mode to set.
* @return This builder for chaining.
*/
public Builder setMode(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
mode_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* The mode in which this disk is attached to the source instance, either READ_WRITE or READ_ONLY.
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @return This builder for chaining.
*/
public Builder clearMode() {
mode_ = getDefaultInstance().getMode();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
/**
*
*
*
* The mode in which this disk is attached to the source instance, either READ_WRITE or READ_ONLY.
* Check the Mode enum for the list of possible values.
*
*
* optional string mode = 3357091;
*
* @param value The bytes for mode to set.
* @return This builder for chaining.
*/
public Builder setModeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
mode_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
private java.lang.Object source_ = "";
/**
*
*
*
* Specifies a URL of the disk attached to the source instance.
*
*
* optional string source = 177235995;
*
* @return Whether the source field is set.
*/
public boolean hasSource() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* Specifies a URL of the disk attached to the source instance.
*
*
* optional string source = 177235995;
*
* @return The source.
*/
public java.lang.String getSource() {
java.lang.Object ref = source_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
source_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Specifies a URL of the disk attached to the source instance.
*
*
* optional string source = 177235995;
*
* @return The bytes for source.
*/
public com.google.protobuf.ByteString getSourceBytes() {
java.lang.Object ref = source_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
source_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Specifies a URL of the disk attached to the source instance.
*
*
* optional string source = 177235995;
*
* @param value The source to set.
* @return This builder for chaining.
*/
public Builder setSource(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
source_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* Specifies a URL of the disk attached to the source instance.
*
*
* optional string source = 177235995;
*
* @return This builder for chaining.
*/
public Builder clearSource() {
source_ = getDefaultInstance().getSource();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
return this;
}
/**
*
*
*
* Specifies a URL of the disk attached to the source instance.
*
*
* optional string source = 177235995;
*
* @param value The bytes for source to set.
* @return This builder for chaining.
*/
public Builder setSourceBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
source_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
private long storageBytes_;
/**
*
*
*
* [Output Only] A size of the storage used by the disk's snapshot by this machine image.
*
*
* optional int64 storage_bytes = 424631719;
*
* @return Whether the storageBytes field is set.
*/
@java.lang.Override
public boolean hasStorageBytes() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*
*
* [Output Only] A size of the storage used by the disk's snapshot by this machine image.
*
*
* optional int64 storage_bytes = 424631719;
*
* @return The storageBytes.
*/
@java.lang.Override
public long getStorageBytes() {
return storageBytes_;
}
/**
*
*
*
* [Output Only] A size of the storage used by the disk's snapshot by this machine image.
*
*
* optional int64 storage_bytes = 424631719;
*
* @param value The storageBytes to set.
* @return This builder for chaining.
*/
public Builder setStorageBytes(long value) {
storageBytes_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] A size of the storage used by the disk's snapshot by this machine image.
*
*
* optional int64 storage_bytes = 424631719;
*
* @return This builder for chaining.
*/
public Builder clearStorageBytes() {
bitField0_ = (bitField0_ & ~0x00002000);
storageBytes_ = 0L;
onChanged();
return this;
}
private java.lang.Object storageBytesStatus_ = "";
/**
*
*
*
* [Output Only] An indicator whether storageBytes is in a stable state or it is being adjusted as a result of shared storage reallocation. This status can either be UPDATING, meaning the size of the snapshot is being updated, or UP_TO_DATE, meaning the size of the snapshot is up-to-date.
* Check the StorageBytesStatus enum for the list of possible values.
*
*
* optional string storage_bytes_status = 490739082;
*
* @return Whether the storageBytesStatus field is set.
*/
public boolean hasStorageBytesStatus() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*
*
* [Output Only] An indicator whether storageBytes is in a stable state or it is being adjusted as a result of shared storage reallocation. This status can either be UPDATING, meaning the size of the snapshot is being updated, or UP_TO_DATE, meaning the size of the snapshot is up-to-date.
* Check the StorageBytesStatus enum for the list of possible values.
*
*
* optional string storage_bytes_status = 490739082;
*
* @return The storageBytesStatus.
*/
public java.lang.String getStorageBytesStatus() {
java.lang.Object ref = storageBytesStatus_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
storageBytesStatus_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] An indicator whether storageBytes is in a stable state or it is being adjusted as a result of shared storage reallocation. This status can either be UPDATING, meaning the size of the snapshot is being updated, or UP_TO_DATE, meaning the size of the snapshot is up-to-date.
* Check the StorageBytesStatus enum for the list of possible values.
*
*
* optional string storage_bytes_status = 490739082;
*
* @return The bytes for storageBytesStatus.
*/
public com.google.protobuf.ByteString getStorageBytesStatusBytes() {
java.lang.Object ref = storageBytesStatus_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
storageBytesStatus_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] An indicator whether storageBytes is in a stable state or it is being adjusted as a result of shared storage reallocation. This status can either be UPDATING, meaning the size of the snapshot is being updated, or UP_TO_DATE, meaning the size of the snapshot is up-to-date.
* Check the StorageBytesStatus enum for the list of possible values.
*
*
* optional string storage_bytes_status = 490739082;
*
* @param value The storageBytesStatus to set.
* @return This builder for chaining.
*/
public Builder setStorageBytesStatus(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
storageBytesStatus_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] An indicator whether storageBytes is in a stable state or it is being adjusted as a result of shared storage reallocation. This status can either be UPDATING, meaning the size of the snapshot is being updated, or UP_TO_DATE, meaning the size of the snapshot is up-to-date.
* Check the StorageBytesStatus enum for the list of possible values.
*
*
* optional string storage_bytes_status = 490739082;
*
* @return This builder for chaining.
*/
public Builder clearStorageBytesStatus() {
storageBytesStatus_ = getDefaultInstance().getStorageBytesStatus();
bitField0_ = (bitField0_ & ~0x00004000);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] An indicator whether storageBytes is in a stable state or it is being adjusted as a result of shared storage reallocation. This status can either be UPDATING, meaning the size of the snapshot is being updated, or UP_TO_DATE, meaning the size of the snapshot is up-to-date.
* Check the StorageBytesStatus enum for the list of possible values.
*
*
* optional string storage_bytes_status = 490739082;
*
* @param value The bytes for storageBytesStatus to set.
* @return This builder for chaining.
*/
public Builder setStorageBytesStatusBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
storageBytesStatus_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
*
*
*
* Specifies the type of the attached disk, either SCRATCH or PERSISTENT.
* Check the Type enum for the list of possible values.
*
*
* optional string type = 3575610;
*
* @return Whether the type field is set.
*/
public boolean hasType() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
*
*
* Specifies the type of the attached disk, either SCRATCH or PERSISTENT.
* Check the Type enum for the list of possible values.
*
*
* optional string type = 3575610;
*
* @return The type.
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Specifies the type of the attached disk, either SCRATCH or PERSISTENT.
* Check the Type enum for the list of possible values.
*
*
* optional string type = 3575610;
*
* @return The bytes for type.
*/
public com.google.protobuf.ByteString getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Specifies the type of the attached disk, either SCRATCH or PERSISTENT.
* Check the Type enum for the list of possible values.
*
*
* optional string type = 3575610;
*
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Specifies the type of the attached disk, either SCRATCH or PERSISTENT.
* Check the Type enum for the list of possible values.
*
*
* optional string type = 3575610;
*
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = getDefaultInstance().getType();
bitField0_ = (bitField0_ & ~0x00008000);
onChanged();
return this;
}
/**
*
*
*
* Specifies the type of the attached disk, either SCRATCH or PERSISTENT.
* Check the Type enum for the list of possible values.
*
*
* optional string type = 3575610;
*
* @param value The bytes for type to set.
* @return This builder for chaining.
*/
public Builder setTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
type_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.SavedAttachedDisk)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.SavedAttachedDisk)
private static final com.google.cloud.compute.v1.SavedAttachedDisk DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.SavedAttachedDisk();
}
public static com.google.cloud.compute.v1.SavedAttachedDisk getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SavedAttachedDisk parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.SavedAttachedDisk getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy