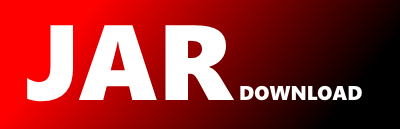
com.google.cloud.compute.v1.Scheduling Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* Sets the scheduling options for an Instance.
*
*
* Protobuf type {@code google.cloud.compute.v1.Scheduling}
*/
public final class Scheduling extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.Scheduling)
SchedulingOrBuilder {
private static final long serialVersionUID = 0L;
// Use Scheduling.newBuilder() to construct.
private Scheduling(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Scheduling() {
instanceTerminationAction_ = "";
locationHint_ = "";
nodeAffinities_ = java.util.Collections.emptyList();
onHostMaintenance_ = "";
provisioningModel_ = "";
terminationTime_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Scheduling();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Scheduling_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Scheduling_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.Scheduling.class,
com.google.cloud.compute.v1.Scheduling.Builder.class);
}
/**
*
*
*
* Specifies the termination action for the instance.
*
*
* Protobuf enum {@code google.cloud.compute.v1.Scheduling.InstanceTerminationAction}
*/
public enum InstanceTerminationAction implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_INSTANCE_TERMINATION_ACTION = 0;
*/
UNDEFINED_INSTANCE_TERMINATION_ACTION(0),
/**
*
*
*
* Delete the VM.
*
*
* DELETE = 402225579;
*/
DELETE(402225579),
/**
*
*
*
* Default value. This value is unused.
*
*
* INSTANCE_TERMINATION_ACTION_UNSPECIFIED = 92954803;
*/
INSTANCE_TERMINATION_ACTION_UNSPECIFIED(92954803),
/**
*
*
*
* Stop the VM without storing in-memory content. default action.
*
*
* STOP = 2555906;
*/
STOP(2555906),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_INSTANCE_TERMINATION_ACTION = 0;
*/
public static final int UNDEFINED_INSTANCE_TERMINATION_ACTION_VALUE = 0;
/**
*
*
*
* Delete the VM.
*
*
* DELETE = 402225579;
*/
public static final int DELETE_VALUE = 402225579;
/**
*
*
*
* Default value. This value is unused.
*
*
* INSTANCE_TERMINATION_ACTION_UNSPECIFIED = 92954803;
*/
public static final int INSTANCE_TERMINATION_ACTION_UNSPECIFIED_VALUE = 92954803;
/**
*
*
*
* Stop the VM without storing in-memory content. default action.
*
*
* STOP = 2555906;
*/
public static final int STOP_VALUE = 2555906;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static InstanceTerminationAction valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static InstanceTerminationAction forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_INSTANCE_TERMINATION_ACTION;
case 402225579:
return DELETE;
case 92954803:
return INSTANCE_TERMINATION_ACTION_UNSPECIFIED;
case 2555906:
return STOP;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public InstanceTerminationAction findValueByNumber(int number) {
return InstanceTerminationAction.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.Scheduling.getDescriptor().getEnumTypes().get(0);
}
private static final InstanceTerminationAction[] VALUES = values();
public static InstanceTerminationAction valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private InstanceTerminationAction(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.Scheduling.InstanceTerminationAction)
}
/**
*
*
*
* Defines the maintenance behavior for this instance. For standard instances, the default behavior is MIGRATE. For preemptible instances, the default and only possible behavior is TERMINATE. For more information, see Set VM host maintenance policy.
*
*
* Protobuf enum {@code google.cloud.compute.v1.Scheduling.OnHostMaintenance}
*/
public enum OnHostMaintenance implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_ON_HOST_MAINTENANCE = 0;
*/
UNDEFINED_ON_HOST_MAINTENANCE(0),
/**
*
*
*
* *[Default]* Allows Compute Engine to automatically migrate instances out of the way of maintenance events.
*
*
* MIGRATE = 165699979;
*/
MIGRATE(165699979),
/**
*
*
*
* Tells Compute Engine to terminate and (optionally) restart the instance away from the maintenance activity. If you would like your instance to be restarted, set the automaticRestart flag to true. Your instance may be restarted more than once, and it may be restarted outside the window of maintenance events.
*
*
* TERMINATE = 527617601;
*/
TERMINATE(527617601),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_ON_HOST_MAINTENANCE = 0;
*/
public static final int UNDEFINED_ON_HOST_MAINTENANCE_VALUE = 0;
/**
*
*
*
* *[Default]* Allows Compute Engine to automatically migrate instances out of the way of maintenance events.
*
*
* MIGRATE = 165699979;
*/
public static final int MIGRATE_VALUE = 165699979;
/**
*
*
*
* Tells Compute Engine to terminate and (optionally) restart the instance away from the maintenance activity. If you would like your instance to be restarted, set the automaticRestart flag to true. Your instance may be restarted more than once, and it may be restarted outside the window of maintenance events.
*
*
* TERMINATE = 527617601;
*/
public static final int TERMINATE_VALUE = 527617601;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OnHostMaintenance valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static OnHostMaintenance forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_ON_HOST_MAINTENANCE;
case 165699979:
return MIGRATE;
case 527617601:
return TERMINATE;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OnHostMaintenance findValueByNumber(int number) {
return OnHostMaintenance.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.Scheduling.getDescriptor().getEnumTypes().get(1);
}
private static final OnHostMaintenance[] VALUES = values();
public static OnHostMaintenance valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private OnHostMaintenance(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.Scheduling.OnHostMaintenance)
}
/**
*
*
*
* Specifies the provisioning model of the instance.
*
*
* Protobuf enum {@code google.cloud.compute.v1.Scheduling.ProvisioningModel}
*/
public enum ProvisioningModel implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_PROVISIONING_MODEL = 0;
*/
UNDEFINED_PROVISIONING_MODEL(0),
/**
*
*
*
* Heavily discounted, no guaranteed runtime.
*
*
* SPOT = 2552066;
*/
SPOT(2552066),
/**
*
*
*
* Standard provisioning with user controlled runtime, no discounts.
*
*
* STANDARD = 484642493;
*/
STANDARD(484642493),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_PROVISIONING_MODEL = 0;
*/
public static final int UNDEFINED_PROVISIONING_MODEL_VALUE = 0;
/**
*
*
*
* Heavily discounted, no guaranteed runtime.
*
*
* SPOT = 2552066;
*/
public static final int SPOT_VALUE = 2552066;
/**
*
*
*
* Standard provisioning with user controlled runtime, no discounts.
*
*
* STANDARD = 484642493;
*/
public static final int STANDARD_VALUE = 484642493;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ProvisioningModel valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static ProvisioningModel forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_PROVISIONING_MODEL;
case 2552066:
return SPOT;
case 484642493:
return STANDARD;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public ProvisioningModel findValueByNumber(int number) {
return ProvisioningModel.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.Scheduling.getDescriptor().getEnumTypes().get(2);
}
private static final ProvisioningModel[] VALUES = values();
public static ProvisioningModel valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private ProvisioningModel(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.Scheduling.ProvisioningModel)
}
private int bitField0_;
public static final int AUTOMATIC_RESTART_FIELD_NUMBER = 350821371;
private boolean automaticRestart_ = false;
/**
*
*
*
* Specifies whether the instance should be automatically restarted if it is terminated by Compute Engine (not terminated by a user). You can only set the automatic restart option for standard instances. Preemptible instances cannot be automatically restarted. By default, this is set to true so an instance is automatically restarted if it is terminated by Compute Engine.
*
*
* optional bool automatic_restart = 350821371;
*
* @return Whether the automaticRestart field is set.
*/
@java.lang.Override
public boolean hasAutomaticRestart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Specifies whether the instance should be automatically restarted if it is terminated by Compute Engine (not terminated by a user). You can only set the automatic restart option for standard instances. Preemptible instances cannot be automatically restarted. By default, this is set to true so an instance is automatically restarted if it is terminated by Compute Engine.
*
*
* optional bool automatic_restart = 350821371;
*
* @return The automaticRestart.
*/
@java.lang.Override
public boolean getAutomaticRestart() {
return automaticRestart_;
}
public static final int AVAILABILITY_DOMAIN_FIELD_NUMBER = 252514344;
private int availabilityDomain_ = 0;
/**
*
*
*
* Specifies the availability domain to place the instance in. The value must be a number between 1 and the number of availability domains specified in the spread placement policy attached to the instance.
*
*
* optional int32 availability_domain = 252514344;
*
* @return Whether the availabilityDomain field is set.
*/
@java.lang.Override
public boolean hasAvailabilityDomain() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Specifies the availability domain to place the instance in. The value must be a number between 1 and the number of availability domains specified in the spread placement policy attached to the instance.
*
*
* optional int32 availability_domain = 252514344;
*
* @return The availabilityDomain.
*/
@java.lang.Override
public int getAvailabilityDomain() {
return availabilityDomain_;
}
public static final int INSTANCE_TERMINATION_ACTION_FIELD_NUMBER = 107380667;
@SuppressWarnings("serial")
private volatile java.lang.Object instanceTerminationAction_ = "";
/**
*
*
*
* Specifies the termination action for the instance.
* Check the InstanceTerminationAction enum for the list of possible values.
*
*
* optional string instance_termination_action = 107380667;
*
* @return Whether the instanceTerminationAction field is set.
*/
@java.lang.Override
public boolean hasInstanceTerminationAction() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Specifies the termination action for the instance.
* Check the InstanceTerminationAction enum for the list of possible values.
*
*
* optional string instance_termination_action = 107380667;
*
* @return The instanceTerminationAction.
*/
@java.lang.Override
public java.lang.String getInstanceTerminationAction() {
java.lang.Object ref = instanceTerminationAction_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
instanceTerminationAction_ = s;
return s;
}
}
/**
*
*
*
* Specifies the termination action for the instance.
* Check the InstanceTerminationAction enum for the list of possible values.
*
*
* optional string instance_termination_action = 107380667;
*
* @return The bytes for instanceTerminationAction.
*/
@java.lang.Override
public com.google.protobuf.ByteString getInstanceTerminationActionBytes() {
java.lang.Object ref = instanceTerminationAction_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
instanceTerminationAction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOCAL_SSD_RECOVERY_TIMEOUT_FIELD_NUMBER = 268015590;
private com.google.cloud.compute.v1.Duration localSsdRecoveryTimeout_;
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*
* @return Whether the localSsdRecoveryTimeout field is set.
*/
@java.lang.Override
public boolean hasLocalSsdRecoveryTimeout() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*
* @return The localSsdRecoveryTimeout.
*/
@java.lang.Override
public com.google.cloud.compute.v1.Duration getLocalSsdRecoveryTimeout() {
return localSsdRecoveryTimeout_ == null
? com.google.cloud.compute.v1.Duration.getDefaultInstance()
: localSsdRecoveryTimeout_;
}
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*/
@java.lang.Override
public com.google.cloud.compute.v1.DurationOrBuilder getLocalSsdRecoveryTimeoutOrBuilder() {
return localSsdRecoveryTimeout_ == null
? com.google.cloud.compute.v1.Duration.getDefaultInstance()
: localSsdRecoveryTimeout_;
}
public static final int LOCATION_HINT_FIELD_NUMBER = 350519505;
@SuppressWarnings("serial")
private volatile java.lang.Object locationHint_ = "";
/**
*
*
*
* An opaque location hint used to place the instance close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return Whether the locationHint field is set.
*/
@java.lang.Override
public boolean hasLocationHint() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* An opaque location hint used to place the instance close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return The locationHint.
*/
@java.lang.Override
public java.lang.String getLocationHint() {
java.lang.Object ref = locationHint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
locationHint_ = s;
return s;
}
}
/**
*
*
*
* An opaque location hint used to place the instance close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return The bytes for locationHint.
*/
@java.lang.Override
public com.google.protobuf.ByteString getLocationHintBytes() {
java.lang.Object ref = locationHint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
locationHint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MAX_RUN_DURATION_FIELD_NUMBER = 430839747;
private com.google.cloud.compute.v1.Duration maxRunDuration_;
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*
* @return Whether the maxRunDuration field is set.
*/
@java.lang.Override
public boolean hasMaxRunDuration() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*
* @return The maxRunDuration.
*/
@java.lang.Override
public com.google.cloud.compute.v1.Duration getMaxRunDuration() {
return maxRunDuration_ == null
? com.google.cloud.compute.v1.Duration.getDefaultInstance()
: maxRunDuration_;
}
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*/
@java.lang.Override
public com.google.cloud.compute.v1.DurationOrBuilder getMaxRunDurationOrBuilder() {
return maxRunDuration_ == null
? com.google.cloud.compute.v1.Duration.getDefaultInstance()
: maxRunDuration_;
}
public static final int MIN_NODE_CPUS_FIELD_NUMBER = 317231675;
private int minNodeCpus_ = 0;
/**
*
*
*
* The minimum number of virtual CPUs this instance will consume when running on a sole-tenant node.
*
*
* optional int32 min_node_cpus = 317231675;
*
* @return Whether the minNodeCpus field is set.
*/
@java.lang.Override
public boolean hasMinNodeCpus() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* The minimum number of virtual CPUs this instance will consume when running on a sole-tenant node.
*
*
* optional int32 min_node_cpus = 317231675;
*
* @return The minNodeCpus.
*/
@java.lang.Override
public int getMinNodeCpus() {
return minNodeCpus_;
}
public static final int NODE_AFFINITIES_FIELD_NUMBER = 461799971;
@SuppressWarnings("serial")
private java.util.List nodeAffinities_;
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
@java.lang.Override
public java.util.List
getNodeAffinitiesList() {
return nodeAffinities_;
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
@java.lang.Override
public java.util.List extends com.google.cloud.compute.v1.SchedulingNodeAffinityOrBuilder>
getNodeAffinitiesOrBuilderList() {
return nodeAffinities_;
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
@java.lang.Override
public int getNodeAffinitiesCount() {
return nodeAffinities_.size();
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.SchedulingNodeAffinity getNodeAffinities(int index) {
return nodeAffinities_.get(index);
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.SchedulingNodeAffinityOrBuilder getNodeAffinitiesOrBuilder(
int index) {
return nodeAffinities_.get(index);
}
public static final int ON_HOST_MAINTENANCE_FIELD_NUMBER = 64616796;
@SuppressWarnings("serial")
private volatile java.lang.Object onHostMaintenance_ = "";
/**
*
*
*
* Defines the maintenance behavior for this instance. For standard instances, the default behavior is MIGRATE. For preemptible instances, the default and only possible behavior is TERMINATE. For more information, see Set VM host maintenance policy.
* Check the OnHostMaintenance enum for the list of possible values.
*
*
* optional string on_host_maintenance = 64616796;
*
* @return Whether the onHostMaintenance field is set.
*/
@java.lang.Override
public boolean hasOnHostMaintenance() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Defines the maintenance behavior for this instance. For standard instances, the default behavior is MIGRATE. For preemptible instances, the default and only possible behavior is TERMINATE. For more information, see Set VM host maintenance policy.
* Check the OnHostMaintenance enum for the list of possible values.
*
*
* optional string on_host_maintenance = 64616796;
*
* @return The onHostMaintenance.
*/
@java.lang.Override
public java.lang.String getOnHostMaintenance() {
java.lang.Object ref = onHostMaintenance_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
onHostMaintenance_ = s;
return s;
}
}
/**
*
*
*
* Defines the maintenance behavior for this instance. For standard instances, the default behavior is MIGRATE. For preemptible instances, the default and only possible behavior is TERMINATE. For more information, see Set VM host maintenance policy.
* Check the OnHostMaintenance enum for the list of possible values.
*
*
* optional string on_host_maintenance = 64616796;
*
* @return The bytes for onHostMaintenance.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOnHostMaintenanceBytes() {
java.lang.Object ref = onHostMaintenance_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
onHostMaintenance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ON_INSTANCE_STOP_ACTION_FIELD_NUMBER = 529876681;
private com.google.cloud.compute.v1.SchedulingOnInstanceStopAction onInstanceStopAction_;
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*
* @return Whether the onInstanceStopAction field is set.
*/
@java.lang.Override
public boolean hasOnInstanceStopAction() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*
* @return The onInstanceStopAction.
*/
@java.lang.Override
public com.google.cloud.compute.v1.SchedulingOnInstanceStopAction getOnInstanceStopAction() {
return onInstanceStopAction_ == null
? com.google.cloud.compute.v1.SchedulingOnInstanceStopAction.getDefaultInstance()
: onInstanceStopAction_;
}
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.SchedulingOnInstanceStopActionOrBuilder
getOnInstanceStopActionOrBuilder() {
return onInstanceStopAction_ == null
? com.google.cloud.compute.v1.SchedulingOnInstanceStopAction.getDefaultInstance()
: onInstanceStopAction_;
}
public static final int PREEMPTIBLE_FIELD_NUMBER = 324203169;
private boolean preemptible_ = false;
/**
*
*
*
* Defines whether the instance is preemptible. This can only be set during instance creation or while the instance is stopped and therefore, in a `TERMINATED` state. See Instance Life Cycle for more information on the possible instance states.
*
*
* optional bool preemptible = 324203169;
*
* @return Whether the preemptible field is set.
*/
@java.lang.Override
public boolean hasPreemptible() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Defines whether the instance is preemptible. This can only be set during instance creation or while the instance is stopped and therefore, in a `TERMINATED` state. See Instance Life Cycle for more information on the possible instance states.
*
*
* optional bool preemptible = 324203169;
*
* @return The preemptible.
*/
@java.lang.Override
public boolean getPreemptible() {
return preemptible_;
}
public static final int PROVISIONING_MODEL_FIELD_NUMBER = 494423;
@SuppressWarnings("serial")
private volatile java.lang.Object provisioningModel_ = "";
/**
*
*
*
* Specifies the provisioning model of the instance.
* Check the ProvisioningModel enum for the list of possible values.
*
*
* optional string provisioning_model = 494423;
*
* @return Whether the provisioningModel field is set.
*/
@java.lang.Override
public boolean hasProvisioningModel() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Specifies the provisioning model of the instance.
* Check the ProvisioningModel enum for the list of possible values.
*
*
* optional string provisioning_model = 494423;
*
* @return The provisioningModel.
*/
@java.lang.Override
public java.lang.String getProvisioningModel() {
java.lang.Object ref = provisioningModel_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
provisioningModel_ = s;
return s;
}
}
/**
*
*
*
* Specifies the provisioning model of the instance.
* Check the ProvisioningModel enum for the list of possible values.
*
*
* optional string provisioning_model = 494423;
*
* @return The bytes for provisioningModel.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProvisioningModelBytes() {
java.lang.Object ref = provisioningModel_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
provisioningModel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TERMINATION_TIME_FIELD_NUMBER = 428082984;
@SuppressWarnings("serial")
private volatile java.lang.Object terminationTime_ = "";
/**
*
*
*
* Specifies the timestamp, when the instance will be terminated, in RFC3339 text format. If specified, the instance termination action will be performed at the termination time.
*
*
* optional string termination_time = 428082984;
*
* @return Whether the terminationTime field is set.
*/
@java.lang.Override
public boolean hasTerminationTime() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* Specifies the timestamp, when the instance will be terminated, in RFC3339 text format. If specified, the instance termination action will be performed at the termination time.
*
*
* optional string termination_time = 428082984;
*
* @return The terminationTime.
*/
@java.lang.Override
public java.lang.String getTerminationTime() {
java.lang.Object ref = terminationTime_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
terminationTime_ = s;
return s;
}
}
/**
*
*
*
* Specifies the timestamp, when the instance will be terminated, in RFC3339 text format. If specified, the instance termination action will be performed at the termination time.
*
*
* optional string termination_time = 428082984;
*
* @return The bytes for terminationTime.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTerminationTimeBytes() {
java.lang.Object ref = terminationTime_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
terminationTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000400) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 494423, provisioningModel_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 64616796, onHostMaintenance_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 107380667, instanceTerminationAction_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeInt32(252514344, availabilityDomain_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(268015590, getLocalSsdRecoveryTimeout());
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeInt32(317231675, minNodeCpus_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeBool(324203169, preemptible_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 350519505, locationHint_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBool(350821371, automaticRestart_);
}
if (((bitField0_ & 0x00000800) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 428082984, terminationTime_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(430839747, getMaxRunDuration());
}
for (int i = 0; i < nodeAffinities_.size(); i++) {
output.writeMessage(461799971, nodeAffinities_.get(i));
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeMessage(529876681, getOnInstanceStopAction());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(494423, provisioningModel_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(64616796, onHostMaintenance_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(
107380667, instanceTerminationAction_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeInt32Size(252514344, availabilityDomain_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
268015590, getLocalSsdRecoveryTimeout());
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(317231675, minNodeCpus_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(324203169, preemptible_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(350519505, locationHint_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(350821371, automaticRestart_);
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(428082984, terminationTime_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(430839747, getMaxRunDuration());
}
for (int i = 0; i < nodeAffinities_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
461799971, nodeAffinities_.get(i));
}
if (((bitField0_ & 0x00000100) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
529876681, getOnInstanceStopAction());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.Scheduling)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.Scheduling other = (com.google.cloud.compute.v1.Scheduling) obj;
if (hasAutomaticRestart() != other.hasAutomaticRestart()) return false;
if (hasAutomaticRestart()) {
if (getAutomaticRestart() != other.getAutomaticRestart()) return false;
}
if (hasAvailabilityDomain() != other.hasAvailabilityDomain()) return false;
if (hasAvailabilityDomain()) {
if (getAvailabilityDomain() != other.getAvailabilityDomain()) return false;
}
if (hasInstanceTerminationAction() != other.hasInstanceTerminationAction()) return false;
if (hasInstanceTerminationAction()) {
if (!getInstanceTerminationAction().equals(other.getInstanceTerminationAction()))
return false;
}
if (hasLocalSsdRecoveryTimeout() != other.hasLocalSsdRecoveryTimeout()) return false;
if (hasLocalSsdRecoveryTimeout()) {
if (!getLocalSsdRecoveryTimeout().equals(other.getLocalSsdRecoveryTimeout())) return false;
}
if (hasLocationHint() != other.hasLocationHint()) return false;
if (hasLocationHint()) {
if (!getLocationHint().equals(other.getLocationHint())) return false;
}
if (hasMaxRunDuration() != other.hasMaxRunDuration()) return false;
if (hasMaxRunDuration()) {
if (!getMaxRunDuration().equals(other.getMaxRunDuration())) return false;
}
if (hasMinNodeCpus() != other.hasMinNodeCpus()) return false;
if (hasMinNodeCpus()) {
if (getMinNodeCpus() != other.getMinNodeCpus()) return false;
}
if (!getNodeAffinitiesList().equals(other.getNodeAffinitiesList())) return false;
if (hasOnHostMaintenance() != other.hasOnHostMaintenance()) return false;
if (hasOnHostMaintenance()) {
if (!getOnHostMaintenance().equals(other.getOnHostMaintenance())) return false;
}
if (hasOnInstanceStopAction() != other.hasOnInstanceStopAction()) return false;
if (hasOnInstanceStopAction()) {
if (!getOnInstanceStopAction().equals(other.getOnInstanceStopAction())) return false;
}
if (hasPreemptible() != other.hasPreemptible()) return false;
if (hasPreemptible()) {
if (getPreemptible() != other.getPreemptible()) return false;
}
if (hasProvisioningModel() != other.hasProvisioningModel()) return false;
if (hasProvisioningModel()) {
if (!getProvisioningModel().equals(other.getProvisioningModel())) return false;
}
if (hasTerminationTime() != other.hasTerminationTime()) return false;
if (hasTerminationTime()) {
if (!getTerminationTime().equals(other.getTerminationTime())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAutomaticRestart()) {
hash = (37 * hash) + AUTOMATIC_RESTART_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getAutomaticRestart());
}
if (hasAvailabilityDomain()) {
hash = (37 * hash) + AVAILABILITY_DOMAIN_FIELD_NUMBER;
hash = (53 * hash) + getAvailabilityDomain();
}
if (hasInstanceTerminationAction()) {
hash = (37 * hash) + INSTANCE_TERMINATION_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getInstanceTerminationAction().hashCode();
}
if (hasLocalSsdRecoveryTimeout()) {
hash = (37 * hash) + LOCAL_SSD_RECOVERY_TIMEOUT_FIELD_NUMBER;
hash = (53 * hash) + getLocalSsdRecoveryTimeout().hashCode();
}
if (hasLocationHint()) {
hash = (37 * hash) + LOCATION_HINT_FIELD_NUMBER;
hash = (53 * hash) + getLocationHint().hashCode();
}
if (hasMaxRunDuration()) {
hash = (37 * hash) + MAX_RUN_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getMaxRunDuration().hashCode();
}
if (hasMinNodeCpus()) {
hash = (37 * hash) + MIN_NODE_CPUS_FIELD_NUMBER;
hash = (53 * hash) + getMinNodeCpus();
}
if (getNodeAffinitiesCount() > 0) {
hash = (37 * hash) + NODE_AFFINITIES_FIELD_NUMBER;
hash = (53 * hash) + getNodeAffinitiesList().hashCode();
}
if (hasOnHostMaintenance()) {
hash = (37 * hash) + ON_HOST_MAINTENANCE_FIELD_NUMBER;
hash = (53 * hash) + getOnHostMaintenance().hashCode();
}
if (hasOnInstanceStopAction()) {
hash = (37 * hash) + ON_INSTANCE_STOP_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getOnInstanceStopAction().hashCode();
}
if (hasPreemptible()) {
hash = (37 * hash) + PREEMPTIBLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getPreemptible());
}
if (hasProvisioningModel()) {
hash = (37 * hash) + PROVISIONING_MODEL_FIELD_NUMBER;
hash = (53 * hash) + getProvisioningModel().hashCode();
}
if (hasTerminationTime()) {
hash = (37 * hash) + TERMINATION_TIME_FIELD_NUMBER;
hash = (53 * hash) + getTerminationTime().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.Scheduling parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Scheduling parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Scheduling parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Scheduling parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Scheduling parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.Scheduling parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.Scheduling parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Scheduling parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.Scheduling parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Scheduling parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.Scheduling parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.Scheduling parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.Scheduling prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Sets the scheduling options for an Instance.
*
*
* Protobuf type {@code google.cloud.compute.v1.Scheduling}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.Scheduling)
com.google.cloud.compute.v1.SchedulingOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Scheduling_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Scheduling_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.Scheduling.class,
com.google.cloud.compute.v1.Scheduling.Builder.class);
}
// Construct using com.google.cloud.compute.v1.Scheduling.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getLocalSsdRecoveryTimeoutFieldBuilder();
getMaxRunDurationFieldBuilder();
getNodeAffinitiesFieldBuilder();
getOnInstanceStopActionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
automaticRestart_ = false;
availabilityDomain_ = 0;
instanceTerminationAction_ = "";
localSsdRecoveryTimeout_ = null;
if (localSsdRecoveryTimeoutBuilder_ != null) {
localSsdRecoveryTimeoutBuilder_.dispose();
localSsdRecoveryTimeoutBuilder_ = null;
}
locationHint_ = "";
maxRunDuration_ = null;
if (maxRunDurationBuilder_ != null) {
maxRunDurationBuilder_.dispose();
maxRunDurationBuilder_ = null;
}
minNodeCpus_ = 0;
if (nodeAffinitiesBuilder_ == null) {
nodeAffinities_ = java.util.Collections.emptyList();
} else {
nodeAffinities_ = null;
nodeAffinitiesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
onHostMaintenance_ = "";
onInstanceStopAction_ = null;
if (onInstanceStopActionBuilder_ != null) {
onInstanceStopActionBuilder_.dispose();
onInstanceStopActionBuilder_ = null;
}
preemptible_ = false;
provisioningModel_ = "";
terminationTime_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_Scheduling_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.Scheduling getDefaultInstanceForType() {
return com.google.cloud.compute.v1.Scheduling.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.Scheduling build() {
com.google.cloud.compute.v1.Scheduling result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.Scheduling buildPartial() {
com.google.cloud.compute.v1.Scheduling result =
new com.google.cloud.compute.v1.Scheduling(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.cloud.compute.v1.Scheduling result) {
if (nodeAffinitiesBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
nodeAffinities_ = java.util.Collections.unmodifiableList(nodeAffinities_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.nodeAffinities_ = nodeAffinities_;
} else {
result.nodeAffinities_ = nodeAffinitiesBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.compute.v1.Scheduling result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.automaticRestart_ = automaticRestart_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.availabilityDomain_ = availabilityDomain_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.instanceTerminationAction_ = instanceTerminationAction_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.localSsdRecoveryTimeout_ =
localSsdRecoveryTimeoutBuilder_ == null
? localSsdRecoveryTimeout_
: localSsdRecoveryTimeoutBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.locationHint_ = locationHint_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.maxRunDuration_ =
maxRunDurationBuilder_ == null ? maxRunDuration_ : maxRunDurationBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.minNodeCpus_ = minNodeCpus_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.onHostMaintenance_ = onHostMaintenance_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.onInstanceStopAction_ =
onInstanceStopActionBuilder_ == null
? onInstanceStopAction_
: onInstanceStopActionBuilder_.build();
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.preemptible_ = preemptible_;
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.provisioningModel_ = provisioningModel_;
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.terminationTime_ = terminationTime_;
to_bitField0_ |= 0x00000800;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.Scheduling) {
return mergeFrom((com.google.cloud.compute.v1.Scheduling) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.Scheduling other) {
if (other == com.google.cloud.compute.v1.Scheduling.getDefaultInstance()) return this;
if (other.hasAutomaticRestart()) {
setAutomaticRestart(other.getAutomaticRestart());
}
if (other.hasAvailabilityDomain()) {
setAvailabilityDomain(other.getAvailabilityDomain());
}
if (other.hasInstanceTerminationAction()) {
instanceTerminationAction_ = other.instanceTerminationAction_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasLocalSsdRecoveryTimeout()) {
mergeLocalSsdRecoveryTimeout(other.getLocalSsdRecoveryTimeout());
}
if (other.hasLocationHint()) {
locationHint_ = other.locationHint_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasMaxRunDuration()) {
mergeMaxRunDuration(other.getMaxRunDuration());
}
if (other.hasMinNodeCpus()) {
setMinNodeCpus(other.getMinNodeCpus());
}
if (nodeAffinitiesBuilder_ == null) {
if (!other.nodeAffinities_.isEmpty()) {
if (nodeAffinities_.isEmpty()) {
nodeAffinities_ = other.nodeAffinities_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureNodeAffinitiesIsMutable();
nodeAffinities_.addAll(other.nodeAffinities_);
}
onChanged();
}
} else {
if (!other.nodeAffinities_.isEmpty()) {
if (nodeAffinitiesBuilder_.isEmpty()) {
nodeAffinitiesBuilder_.dispose();
nodeAffinitiesBuilder_ = null;
nodeAffinities_ = other.nodeAffinities_;
bitField0_ = (bitField0_ & ~0x00000080);
nodeAffinitiesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getNodeAffinitiesFieldBuilder()
: null;
} else {
nodeAffinitiesBuilder_.addAllMessages(other.nodeAffinities_);
}
}
}
if (other.hasOnHostMaintenance()) {
onHostMaintenance_ = other.onHostMaintenance_;
bitField0_ |= 0x00000100;
onChanged();
}
if (other.hasOnInstanceStopAction()) {
mergeOnInstanceStopAction(other.getOnInstanceStopAction());
}
if (other.hasPreemptible()) {
setPreemptible(other.getPreemptible());
}
if (other.hasProvisioningModel()) {
provisioningModel_ = other.provisioningModel_;
bitField0_ |= 0x00000800;
onChanged();
}
if (other.hasTerminationTime()) {
terminationTime_ = other.terminationTime_;
bitField0_ |= 0x00001000;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 3955386:
{
provisioningModel_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000800;
break;
} // case 3955386
case 516934370:
{
onHostMaintenance_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case 516934370
case 859045338:
{
instanceTerminationAction_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 859045338
case 2020114752:
{
availabilityDomain_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 2020114752
case 2144124722:
{
input.readMessage(
getLocalSsdRecoveryTimeoutFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 2144124722
case -1757113896:
{
minNodeCpus_ = input.readInt32();
bitField0_ |= 0x00000040;
break;
} // case -1757113896
case -1701341944:
{
preemptible_ = input.readBool();
bitField0_ |= 0x00000400;
break;
} // case -1701341944
case -1490811254:
{
locationHint_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case -1490811254
case -1488396328:
{
automaticRestart_ = input.readBool();
bitField0_ |= 0x00000001;
break;
} // case -1488396328
case -870303422:
{
terminationTime_ = input.readStringRequireUtf8();
bitField0_ |= 0x00001000;
break;
} // case -870303422
case -848249318:
{
input.readMessage(getMaxRunDurationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case -848249318
case -600567526:
{
com.google.cloud.compute.v1.SchedulingNodeAffinity m =
input.readMessage(
com.google.cloud.compute.v1.SchedulingNodeAffinity.parser(),
extensionRegistry);
if (nodeAffinitiesBuilder_ == null) {
ensureNodeAffinitiesIsMutable();
nodeAffinities_.add(m);
} else {
nodeAffinitiesBuilder_.addMessage(m);
}
break;
} // case -600567526
case -55953846:
{
input.readMessage(
getOnInstanceStopActionFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000200;
break;
} // case -55953846
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private boolean automaticRestart_;
/**
*
*
*
* Specifies whether the instance should be automatically restarted if it is terminated by Compute Engine (not terminated by a user). You can only set the automatic restart option for standard instances. Preemptible instances cannot be automatically restarted. By default, this is set to true so an instance is automatically restarted if it is terminated by Compute Engine.
*
*
* optional bool automatic_restart = 350821371;
*
* @return Whether the automaticRestart field is set.
*/
@java.lang.Override
public boolean hasAutomaticRestart() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Specifies whether the instance should be automatically restarted if it is terminated by Compute Engine (not terminated by a user). You can only set the automatic restart option for standard instances. Preemptible instances cannot be automatically restarted. By default, this is set to true so an instance is automatically restarted if it is terminated by Compute Engine.
*
*
* optional bool automatic_restart = 350821371;
*
* @return The automaticRestart.
*/
@java.lang.Override
public boolean getAutomaticRestart() {
return automaticRestart_;
}
/**
*
*
*
* Specifies whether the instance should be automatically restarted if it is terminated by Compute Engine (not terminated by a user). You can only set the automatic restart option for standard instances. Preemptible instances cannot be automatically restarted. By default, this is set to true so an instance is automatically restarted if it is terminated by Compute Engine.
*
*
* optional bool automatic_restart = 350821371;
*
* @param value The automaticRestart to set.
* @return This builder for chaining.
*/
public Builder setAutomaticRestart(boolean value) {
automaticRestart_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Specifies whether the instance should be automatically restarted if it is terminated by Compute Engine (not terminated by a user). You can only set the automatic restart option for standard instances. Preemptible instances cannot be automatically restarted. By default, this is set to true so an instance is automatically restarted if it is terminated by Compute Engine.
*
*
* optional bool automatic_restart = 350821371;
*
* @return This builder for chaining.
*/
public Builder clearAutomaticRestart() {
bitField0_ = (bitField0_ & ~0x00000001);
automaticRestart_ = false;
onChanged();
return this;
}
private int availabilityDomain_;
/**
*
*
*
* Specifies the availability domain to place the instance in. The value must be a number between 1 and the number of availability domains specified in the spread placement policy attached to the instance.
*
*
* optional int32 availability_domain = 252514344;
*
* @return Whether the availabilityDomain field is set.
*/
@java.lang.Override
public boolean hasAvailabilityDomain() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Specifies the availability domain to place the instance in. The value must be a number between 1 and the number of availability domains specified in the spread placement policy attached to the instance.
*
*
* optional int32 availability_domain = 252514344;
*
* @return The availabilityDomain.
*/
@java.lang.Override
public int getAvailabilityDomain() {
return availabilityDomain_;
}
/**
*
*
*
* Specifies the availability domain to place the instance in. The value must be a number between 1 and the number of availability domains specified in the spread placement policy attached to the instance.
*
*
* optional int32 availability_domain = 252514344;
*
* @param value The availabilityDomain to set.
* @return This builder for chaining.
*/
public Builder setAvailabilityDomain(int value) {
availabilityDomain_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Specifies the availability domain to place the instance in. The value must be a number between 1 and the number of availability domains specified in the spread placement policy attached to the instance.
*
*
* optional int32 availability_domain = 252514344;
*
* @return This builder for chaining.
*/
public Builder clearAvailabilityDomain() {
bitField0_ = (bitField0_ & ~0x00000002);
availabilityDomain_ = 0;
onChanged();
return this;
}
private java.lang.Object instanceTerminationAction_ = "";
/**
*
*
*
* Specifies the termination action for the instance.
* Check the InstanceTerminationAction enum for the list of possible values.
*
*
* optional string instance_termination_action = 107380667;
*
* @return Whether the instanceTerminationAction field is set.
*/
public boolean hasInstanceTerminationAction() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Specifies the termination action for the instance.
* Check the InstanceTerminationAction enum for the list of possible values.
*
*
* optional string instance_termination_action = 107380667;
*
* @return The instanceTerminationAction.
*/
public java.lang.String getInstanceTerminationAction() {
java.lang.Object ref = instanceTerminationAction_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
instanceTerminationAction_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Specifies the termination action for the instance.
* Check the InstanceTerminationAction enum for the list of possible values.
*
*
* optional string instance_termination_action = 107380667;
*
* @return The bytes for instanceTerminationAction.
*/
public com.google.protobuf.ByteString getInstanceTerminationActionBytes() {
java.lang.Object ref = instanceTerminationAction_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
instanceTerminationAction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Specifies the termination action for the instance.
* Check the InstanceTerminationAction enum for the list of possible values.
*
*
* optional string instance_termination_action = 107380667;
*
* @param value The instanceTerminationAction to set.
* @return This builder for chaining.
*/
public Builder setInstanceTerminationAction(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
instanceTerminationAction_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Specifies the termination action for the instance.
* Check the InstanceTerminationAction enum for the list of possible values.
*
*
* optional string instance_termination_action = 107380667;
*
* @return This builder for chaining.
*/
public Builder clearInstanceTerminationAction() {
instanceTerminationAction_ = getDefaultInstance().getInstanceTerminationAction();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* Specifies the termination action for the instance.
* Check the InstanceTerminationAction enum for the list of possible values.
*
*
* optional string instance_termination_action = 107380667;
*
* @param value The bytes for instanceTerminationAction to set.
* @return This builder for chaining.
*/
public Builder setInstanceTerminationActionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
instanceTerminationAction_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.google.cloud.compute.v1.Duration localSsdRecoveryTimeout_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.Duration,
com.google.cloud.compute.v1.Duration.Builder,
com.google.cloud.compute.v1.DurationOrBuilder>
localSsdRecoveryTimeoutBuilder_;
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*
*
* @return Whether the localSsdRecoveryTimeout field is set.
*/
public boolean hasLocalSsdRecoveryTimeout() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*
*
* @return The localSsdRecoveryTimeout.
*/
public com.google.cloud.compute.v1.Duration getLocalSsdRecoveryTimeout() {
if (localSsdRecoveryTimeoutBuilder_ == null) {
return localSsdRecoveryTimeout_ == null
? com.google.cloud.compute.v1.Duration.getDefaultInstance()
: localSsdRecoveryTimeout_;
} else {
return localSsdRecoveryTimeoutBuilder_.getMessage();
}
}
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*
*/
public Builder setLocalSsdRecoveryTimeout(com.google.cloud.compute.v1.Duration value) {
if (localSsdRecoveryTimeoutBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
localSsdRecoveryTimeout_ = value;
} else {
localSsdRecoveryTimeoutBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*
*/
public Builder setLocalSsdRecoveryTimeout(
com.google.cloud.compute.v1.Duration.Builder builderForValue) {
if (localSsdRecoveryTimeoutBuilder_ == null) {
localSsdRecoveryTimeout_ = builderForValue.build();
} else {
localSsdRecoveryTimeoutBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*
*/
public Builder mergeLocalSsdRecoveryTimeout(com.google.cloud.compute.v1.Duration value) {
if (localSsdRecoveryTimeoutBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& localSsdRecoveryTimeout_ != null
&& localSsdRecoveryTimeout_
!= com.google.cloud.compute.v1.Duration.getDefaultInstance()) {
getLocalSsdRecoveryTimeoutBuilder().mergeFrom(value);
} else {
localSsdRecoveryTimeout_ = value;
}
} else {
localSsdRecoveryTimeoutBuilder_.mergeFrom(value);
}
if (localSsdRecoveryTimeout_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*
*/
public Builder clearLocalSsdRecoveryTimeout() {
bitField0_ = (bitField0_ & ~0x00000008);
localSsdRecoveryTimeout_ = null;
if (localSsdRecoveryTimeoutBuilder_ != null) {
localSsdRecoveryTimeoutBuilder_.dispose();
localSsdRecoveryTimeoutBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*
*/
public com.google.cloud.compute.v1.Duration.Builder getLocalSsdRecoveryTimeoutBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getLocalSsdRecoveryTimeoutFieldBuilder().getBuilder();
}
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*
*/
public com.google.cloud.compute.v1.DurationOrBuilder getLocalSsdRecoveryTimeoutOrBuilder() {
if (localSsdRecoveryTimeoutBuilder_ != null) {
return localSsdRecoveryTimeoutBuilder_.getMessageOrBuilder();
} else {
return localSsdRecoveryTimeout_ == null
? com.google.cloud.compute.v1.Duration.getDefaultInstance()
: localSsdRecoveryTimeout_;
}
}
/**
*
*
*
* Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour granularity and the default value being 1 hour.
*
*
* optional .google.cloud.compute.v1.Duration local_ssd_recovery_timeout = 268015590;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.Duration,
com.google.cloud.compute.v1.Duration.Builder,
com.google.cloud.compute.v1.DurationOrBuilder>
getLocalSsdRecoveryTimeoutFieldBuilder() {
if (localSsdRecoveryTimeoutBuilder_ == null) {
localSsdRecoveryTimeoutBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.Duration,
com.google.cloud.compute.v1.Duration.Builder,
com.google.cloud.compute.v1.DurationOrBuilder>(
getLocalSsdRecoveryTimeout(), getParentForChildren(), isClean());
localSsdRecoveryTimeout_ = null;
}
return localSsdRecoveryTimeoutBuilder_;
}
private java.lang.Object locationHint_ = "";
/**
*
*
*
* An opaque location hint used to place the instance close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return Whether the locationHint field is set.
*/
public boolean hasLocationHint() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* An opaque location hint used to place the instance close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return The locationHint.
*/
public java.lang.String getLocationHint() {
java.lang.Object ref = locationHint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
locationHint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* An opaque location hint used to place the instance close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return The bytes for locationHint.
*/
public com.google.protobuf.ByteString getLocationHintBytes() {
java.lang.Object ref = locationHint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
locationHint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* An opaque location hint used to place the instance close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @param value The locationHint to set.
* @return This builder for chaining.
*/
public Builder setLocationHint(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
locationHint_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* An opaque location hint used to place the instance close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @return This builder for chaining.
*/
public Builder clearLocationHint() {
locationHint_ = getDefaultInstance().getLocationHint();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
*
*
* An opaque location hint used to place the instance close to other resources. This field is for use by internal tools that use the public API.
*
*
* optional string location_hint = 350519505;
*
* @param value The bytes for locationHint to set.
* @return This builder for chaining.
*/
public Builder setLocationHintBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
locationHint_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private com.google.cloud.compute.v1.Duration maxRunDuration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.Duration,
com.google.cloud.compute.v1.Duration.Builder,
com.google.cloud.compute.v1.DurationOrBuilder>
maxRunDurationBuilder_;
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*
* @return Whether the maxRunDuration field is set.
*/
public boolean hasMaxRunDuration() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*
* @return The maxRunDuration.
*/
public com.google.cloud.compute.v1.Duration getMaxRunDuration() {
if (maxRunDurationBuilder_ == null) {
return maxRunDuration_ == null
? com.google.cloud.compute.v1.Duration.getDefaultInstance()
: maxRunDuration_;
} else {
return maxRunDurationBuilder_.getMessage();
}
}
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*/
public Builder setMaxRunDuration(com.google.cloud.compute.v1.Duration value) {
if (maxRunDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maxRunDuration_ = value;
} else {
maxRunDurationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*/
public Builder setMaxRunDuration(com.google.cloud.compute.v1.Duration.Builder builderForValue) {
if (maxRunDurationBuilder_ == null) {
maxRunDuration_ = builderForValue.build();
} else {
maxRunDurationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*/
public Builder mergeMaxRunDuration(com.google.cloud.compute.v1.Duration value) {
if (maxRunDurationBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)
&& maxRunDuration_ != null
&& maxRunDuration_ != com.google.cloud.compute.v1.Duration.getDefaultInstance()) {
getMaxRunDurationBuilder().mergeFrom(value);
} else {
maxRunDuration_ = value;
}
} else {
maxRunDurationBuilder_.mergeFrom(value);
}
if (maxRunDuration_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*/
public Builder clearMaxRunDuration() {
bitField0_ = (bitField0_ & ~0x00000020);
maxRunDuration_ = null;
if (maxRunDurationBuilder_ != null) {
maxRunDurationBuilder_.dispose();
maxRunDurationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*/
public com.google.cloud.compute.v1.Duration.Builder getMaxRunDurationBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getMaxRunDurationFieldBuilder().getBuilder();
}
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*/
public com.google.cloud.compute.v1.DurationOrBuilder getMaxRunDurationOrBuilder() {
if (maxRunDurationBuilder_ != null) {
return maxRunDurationBuilder_.getMessageOrBuilder();
} else {
return maxRunDuration_ == null
? com.google.cloud.compute.v1.Duration.getDefaultInstance()
: maxRunDuration_;
}
}
/**
*
*
*
* Specifies the max run duration for the given instance. If specified, the instance termination action will be performed at the end of the run duration.
*
*
* optional .google.cloud.compute.v1.Duration max_run_duration = 430839747;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.Duration,
com.google.cloud.compute.v1.Duration.Builder,
com.google.cloud.compute.v1.DurationOrBuilder>
getMaxRunDurationFieldBuilder() {
if (maxRunDurationBuilder_ == null) {
maxRunDurationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.Duration,
com.google.cloud.compute.v1.Duration.Builder,
com.google.cloud.compute.v1.DurationOrBuilder>(
getMaxRunDuration(), getParentForChildren(), isClean());
maxRunDuration_ = null;
}
return maxRunDurationBuilder_;
}
private int minNodeCpus_;
/**
*
*
*
* The minimum number of virtual CPUs this instance will consume when running on a sole-tenant node.
*
*
* optional int32 min_node_cpus = 317231675;
*
* @return Whether the minNodeCpus field is set.
*/
@java.lang.Override
public boolean hasMinNodeCpus() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* The minimum number of virtual CPUs this instance will consume when running on a sole-tenant node.
*
*
* optional int32 min_node_cpus = 317231675;
*
* @return The minNodeCpus.
*/
@java.lang.Override
public int getMinNodeCpus() {
return minNodeCpus_;
}
/**
*
*
*
* The minimum number of virtual CPUs this instance will consume when running on a sole-tenant node.
*
*
* optional int32 min_node_cpus = 317231675;
*
* @param value The minNodeCpus to set.
* @return This builder for chaining.
*/
public Builder setMinNodeCpus(int value) {
minNodeCpus_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* The minimum number of virtual CPUs this instance will consume when running on a sole-tenant node.
*
*
* optional int32 min_node_cpus = 317231675;
*
* @return This builder for chaining.
*/
public Builder clearMinNodeCpus() {
bitField0_ = (bitField0_ & ~0x00000040);
minNodeCpus_ = 0;
onChanged();
return this;
}
private java.util.List nodeAffinities_ =
java.util.Collections.emptyList();
private void ensureNodeAffinitiesIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
nodeAffinities_ =
new java.util.ArrayList(
nodeAffinities_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.SchedulingNodeAffinity,
com.google.cloud.compute.v1.SchedulingNodeAffinity.Builder,
com.google.cloud.compute.v1.SchedulingNodeAffinityOrBuilder>
nodeAffinitiesBuilder_;
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public java.util.List
getNodeAffinitiesList() {
if (nodeAffinitiesBuilder_ == null) {
return java.util.Collections.unmodifiableList(nodeAffinities_);
} else {
return nodeAffinitiesBuilder_.getMessageList();
}
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public int getNodeAffinitiesCount() {
if (nodeAffinitiesBuilder_ == null) {
return nodeAffinities_.size();
} else {
return nodeAffinitiesBuilder_.getCount();
}
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public com.google.cloud.compute.v1.SchedulingNodeAffinity getNodeAffinities(int index) {
if (nodeAffinitiesBuilder_ == null) {
return nodeAffinities_.get(index);
} else {
return nodeAffinitiesBuilder_.getMessage(index);
}
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public Builder setNodeAffinities(
int index, com.google.cloud.compute.v1.SchedulingNodeAffinity value) {
if (nodeAffinitiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeAffinitiesIsMutable();
nodeAffinities_.set(index, value);
onChanged();
} else {
nodeAffinitiesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public Builder setNodeAffinities(
int index, com.google.cloud.compute.v1.SchedulingNodeAffinity.Builder builderForValue) {
if (nodeAffinitiesBuilder_ == null) {
ensureNodeAffinitiesIsMutable();
nodeAffinities_.set(index, builderForValue.build());
onChanged();
} else {
nodeAffinitiesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public Builder addNodeAffinities(com.google.cloud.compute.v1.SchedulingNodeAffinity value) {
if (nodeAffinitiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeAffinitiesIsMutable();
nodeAffinities_.add(value);
onChanged();
} else {
nodeAffinitiesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public Builder addNodeAffinities(
int index, com.google.cloud.compute.v1.SchedulingNodeAffinity value) {
if (nodeAffinitiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeAffinitiesIsMutable();
nodeAffinities_.add(index, value);
onChanged();
} else {
nodeAffinitiesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public Builder addNodeAffinities(
com.google.cloud.compute.v1.SchedulingNodeAffinity.Builder builderForValue) {
if (nodeAffinitiesBuilder_ == null) {
ensureNodeAffinitiesIsMutable();
nodeAffinities_.add(builderForValue.build());
onChanged();
} else {
nodeAffinitiesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public Builder addNodeAffinities(
int index, com.google.cloud.compute.v1.SchedulingNodeAffinity.Builder builderForValue) {
if (nodeAffinitiesBuilder_ == null) {
ensureNodeAffinitiesIsMutable();
nodeAffinities_.add(index, builderForValue.build());
onChanged();
} else {
nodeAffinitiesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public Builder addAllNodeAffinities(
java.lang.Iterable extends com.google.cloud.compute.v1.SchedulingNodeAffinity> values) {
if (nodeAffinitiesBuilder_ == null) {
ensureNodeAffinitiesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, nodeAffinities_);
onChanged();
} else {
nodeAffinitiesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public Builder clearNodeAffinities() {
if (nodeAffinitiesBuilder_ == null) {
nodeAffinities_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
nodeAffinitiesBuilder_.clear();
}
return this;
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public Builder removeNodeAffinities(int index) {
if (nodeAffinitiesBuilder_ == null) {
ensureNodeAffinitiesIsMutable();
nodeAffinities_.remove(index);
onChanged();
} else {
nodeAffinitiesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public com.google.cloud.compute.v1.SchedulingNodeAffinity.Builder getNodeAffinitiesBuilder(
int index) {
return getNodeAffinitiesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public com.google.cloud.compute.v1.SchedulingNodeAffinityOrBuilder getNodeAffinitiesOrBuilder(
int index) {
if (nodeAffinitiesBuilder_ == null) {
return nodeAffinities_.get(index);
} else {
return nodeAffinitiesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public java.util.List extends com.google.cloud.compute.v1.SchedulingNodeAffinityOrBuilder>
getNodeAffinitiesOrBuilderList() {
if (nodeAffinitiesBuilder_ != null) {
return nodeAffinitiesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(nodeAffinities_);
}
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public com.google.cloud.compute.v1.SchedulingNodeAffinity.Builder addNodeAffinitiesBuilder() {
return getNodeAffinitiesFieldBuilder()
.addBuilder(com.google.cloud.compute.v1.SchedulingNodeAffinity.getDefaultInstance());
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public com.google.cloud.compute.v1.SchedulingNodeAffinity.Builder addNodeAffinitiesBuilder(
int index) {
return getNodeAffinitiesFieldBuilder()
.addBuilder(
index, com.google.cloud.compute.v1.SchedulingNodeAffinity.getDefaultInstance());
}
/**
*
*
*
* A set of node affinity and anti-affinity configurations. Refer to Configuring node affinity for more information. Overrides reservationAffinity.
*
*
* repeated .google.cloud.compute.v1.SchedulingNodeAffinity node_affinities = 461799971;
*
*/
public java.util.List
getNodeAffinitiesBuilderList() {
return getNodeAffinitiesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.SchedulingNodeAffinity,
com.google.cloud.compute.v1.SchedulingNodeAffinity.Builder,
com.google.cloud.compute.v1.SchedulingNodeAffinityOrBuilder>
getNodeAffinitiesFieldBuilder() {
if (nodeAffinitiesBuilder_ == null) {
nodeAffinitiesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.SchedulingNodeAffinity,
com.google.cloud.compute.v1.SchedulingNodeAffinity.Builder,
com.google.cloud.compute.v1.SchedulingNodeAffinityOrBuilder>(
nodeAffinities_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
nodeAffinities_ = null;
}
return nodeAffinitiesBuilder_;
}
private java.lang.Object onHostMaintenance_ = "";
/**
*
*
*
* Defines the maintenance behavior for this instance. For standard instances, the default behavior is MIGRATE. For preemptible instances, the default and only possible behavior is TERMINATE. For more information, see Set VM host maintenance policy.
* Check the OnHostMaintenance enum for the list of possible values.
*
*
* optional string on_host_maintenance = 64616796;
*
* @return Whether the onHostMaintenance field is set.
*/
public boolean hasOnHostMaintenance() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Defines the maintenance behavior for this instance. For standard instances, the default behavior is MIGRATE. For preemptible instances, the default and only possible behavior is TERMINATE. For more information, see Set VM host maintenance policy.
* Check the OnHostMaintenance enum for the list of possible values.
*
*
* optional string on_host_maintenance = 64616796;
*
* @return The onHostMaintenance.
*/
public java.lang.String getOnHostMaintenance() {
java.lang.Object ref = onHostMaintenance_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
onHostMaintenance_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Defines the maintenance behavior for this instance. For standard instances, the default behavior is MIGRATE. For preemptible instances, the default and only possible behavior is TERMINATE. For more information, see Set VM host maintenance policy.
* Check the OnHostMaintenance enum for the list of possible values.
*
*
* optional string on_host_maintenance = 64616796;
*
* @return The bytes for onHostMaintenance.
*/
public com.google.protobuf.ByteString getOnHostMaintenanceBytes() {
java.lang.Object ref = onHostMaintenance_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
onHostMaintenance_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Defines the maintenance behavior for this instance. For standard instances, the default behavior is MIGRATE. For preemptible instances, the default and only possible behavior is TERMINATE. For more information, see Set VM host maintenance policy.
* Check the OnHostMaintenance enum for the list of possible values.
*
*
* optional string on_host_maintenance = 64616796;
*
* @param value The onHostMaintenance to set.
* @return This builder for chaining.
*/
public Builder setOnHostMaintenance(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
onHostMaintenance_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Defines the maintenance behavior for this instance. For standard instances, the default behavior is MIGRATE. For preemptible instances, the default and only possible behavior is TERMINATE. For more information, see Set VM host maintenance policy.
* Check the OnHostMaintenance enum for the list of possible values.
*
*
* optional string on_host_maintenance = 64616796;
*
* @return This builder for chaining.
*/
public Builder clearOnHostMaintenance() {
onHostMaintenance_ = getDefaultInstance().getOnHostMaintenance();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
*
*
*
* Defines the maintenance behavior for this instance. For standard instances, the default behavior is MIGRATE. For preemptible instances, the default and only possible behavior is TERMINATE. For more information, see Set VM host maintenance policy.
* Check the OnHostMaintenance enum for the list of possible values.
*
*
* optional string on_host_maintenance = 64616796;
*
* @param value The bytes for onHostMaintenance to set.
* @return This builder for chaining.
*/
public Builder setOnHostMaintenanceBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
onHostMaintenance_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private com.google.cloud.compute.v1.SchedulingOnInstanceStopAction onInstanceStopAction_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.SchedulingOnInstanceStopAction,
com.google.cloud.compute.v1.SchedulingOnInstanceStopAction.Builder,
com.google.cloud.compute.v1.SchedulingOnInstanceStopActionOrBuilder>
onInstanceStopActionBuilder_;
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*
* @return Whether the onInstanceStopAction field is set.
*/
public boolean hasOnInstanceStopAction() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*
* @return The onInstanceStopAction.
*/
public com.google.cloud.compute.v1.SchedulingOnInstanceStopAction getOnInstanceStopAction() {
if (onInstanceStopActionBuilder_ == null) {
return onInstanceStopAction_ == null
? com.google.cloud.compute.v1.SchedulingOnInstanceStopAction.getDefaultInstance()
: onInstanceStopAction_;
} else {
return onInstanceStopActionBuilder_.getMessage();
}
}
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*/
public Builder setOnInstanceStopAction(
com.google.cloud.compute.v1.SchedulingOnInstanceStopAction value) {
if (onInstanceStopActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
onInstanceStopAction_ = value;
} else {
onInstanceStopActionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*/
public Builder setOnInstanceStopAction(
com.google.cloud.compute.v1.SchedulingOnInstanceStopAction.Builder builderForValue) {
if (onInstanceStopActionBuilder_ == null) {
onInstanceStopAction_ = builderForValue.build();
} else {
onInstanceStopActionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*/
public Builder mergeOnInstanceStopAction(
com.google.cloud.compute.v1.SchedulingOnInstanceStopAction value) {
if (onInstanceStopActionBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)
&& onInstanceStopAction_ != null
&& onInstanceStopAction_
!= com.google.cloud.compute.v1.SchedulingOnInstanceStopAction
.getDefaultInstance()) {
getOnInstanceStopActionBuilder().mergeFrom(value);
} else {
onInstanceStopAction_ = value;
}
} else {
onInstanceStopActionBuilder_.mergeFrom(value);
}
if (onInstanceStopAction_ != null) {
bitField0_ |= 0x00000200;
onChanged();
}
return this;
}
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*/
public Builder clearOnInstanceStopAction() {
bitField0_ = (bitField0_ & ~0x00000200);
onInstanceStopAction_ = null;
if (onInstanceStopActionBuilder_ != null) {
onInstanceStopActionBuilder_.dispose();
onInstanceStopActionBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*/
public com.google.cloud.compute.v1.SchedulingOnInstanceStopAction.Builder
getOnInstanceStopActionBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getOnInstanceStopActionFieldBuilder().getBuilder();
}
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*/
public com.google.cloud.compute.v1.SchedulingOnInstanceStopActionOrBuilder
getOnInstanceStopActionOrBuilder() {
if (onInstanceStopActionBuilder_ != null) {
return onInstanceStopActionBuilder_.getMessageOrBuilder();
} else {
return onInstanceStopAction_ == null
? com.google.cloud.compute.v1.SchedulingOnInstanceStopAction.getDefaultInstance()
: onInstanceStopAction_;
}
}
/**
*
* optional .google.cloud.compute.v1.SchedulingOnInstanceStopAction on_instance_stop_action = 529876681;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.SchedulingOnInstanceStopAction,
com.google.cloud.compute.v1.SchedulingOnInstanceStopAction.Builder,
com.google.cloud.compute.v1.SchedulingOnInstanceStopActionOrBuilder>
getOnInstanceStopActionFieldBuilder() {
if (onInstanceStopActionBuilder_ == null) {
onInstanceStopActionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.SchedulingOnInstanceStopAction,
com.google.cloud.compute.v1.SchedulingOnInstanceStopAction.Builder,
com.google.cloud.compute.v1.SchedulingOnInstanceStopActionOrBuilder>(
getOnInstanceStopAction(), getParentForChildren(), isClean());
onInstanceStopAction_ = null;
}
return onInstanceStopActionBuilder_;
}
private boolean preemptible_;
/**
*
*
*
* Defines whether the instance is preemptible. This can only be set during instance creation or while the instance is stopped and therefore, in a `TERMINATED` state. See Instance Life Cycle for more information on the possible instance states.
*
*
* optional bool preemptible = 324203169;
*
* @return Whether the preemptible field is set.
*/
@java.lang.Override
public boolean hasPreemptible() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Defines whether the instance is preemptible. This can only be set during instance creation or while the instance is stopped and therefore, in a `TERMINATED` state. See Instance Life Cycle for more information on the possible instance states.
*
*
* optional bool preemptible = 324203169;
*
* @return The preemptible.
*/
@java.lang.Override
public boolean getPreemptible() {
return preemptible_;
}
/**
*
*
*
* Defines whether the instance is preemptible. This can only be set during instance creation or while the instance is stopped and therefore, in a `TERMINATED` state. See Instance Life Cycle for more information on the possible instance states.
*
*
* optional bool preemptible = 324203169;
*
* @param value The preemptible to set.
* @return This builder for chaining.
*/
public Builder setPreemptible(boolean value) {
preemptible_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Defines whether the instance is preemptible. This can only be set during instance creation or while the instance is stopped and therefore, in a `TERMINATED` state. See Instance Life Cycle for more information on the possible instance states.
*
*
* optional bool preemptible = 324203169;
*
* @return This builder for chaining.
*/
public Builder clearPreemptible() {
bitField0_ = (bitField0_ & ~0x00000400);
preemptible_ = false;
onChanged();
return this;
}
private java.lang.Object provisioningModel_ = "";
/**
*
*
*
* Specifies the provisioning model of the instance.
* Check the ProvisioningModel enum for the list of possible values.
*
*
* optional string provisioning_model = 494423;
*
* @return Whether the provisioningModel field is set.
*/
public boolean hasProvisioningModel() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* Specifies the provisioning model of the instance.
* Check the ProvisioningModel enum for the list of possible values.
*
*
* optional string provisioning_model = 494423;
*
* @return The provisioningModel.
*/
public java.lang.String getProvisioningModel() {
java.lang.Object ref = provisioningModel_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
provisioningModel_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Specifies the provisioning model of the instance.
* Check the ProvisioningModel enum for the list of possible values.
*
*
* optional string provisioning_model = 494423;
*
* @return The bytes for provisioningModel.
*/
public com.google.protobuf.ByteString getProvisioningModelBytes() {
java.lang.Object ref = provisioningModel_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
provisioningModel_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Specifies the provisioning model of the instance.
* Check the ProvisioningModel enum for the list of possible values.
*
*
* optional string provisioning_model = 494423;
*
* @param value The provisioningModel to set.
* @return This builder for chaining.
*/
public Builder setProvisioningModel(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
provisioningModel_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* Specifies the provisioning model of the instance.
* Check the ProvisioningModel enum for the list of possible values.
*
*
* optional string provisioning_model = 494423;
*
* @return This builder for chaining.
*/
public Builder clearProvisioningModel() {
provisioningModel_ = getDefaultInstance().getProvisioningModel();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
/**
*
*
*
* Specifies the provisioning model of the instance.
* Check the ProvisioningModel enum for the list of possible values.
*
*
* optional string provisioning_model = 494423;
*
* @param value The bytes for provisioningModel to set.
* @return This builder for chaining.
*/
public Builder setProvisioningModelBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
provisioningModel_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
private java.lang.Object terminationTime_ = "";
/**
*
*
*
* Specifies the timestamp, when the instance will be terminated, in RFC3339 text format. If specified, the instance termination action will be performed at the termination time.
*
*
* optional string termination_time = 428082984;
*
* @return Whether the terminationTime field is set.
*/
public boolean hasTerminationTime() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* Specifies the timestamp, when the instance will be terminated, in RFC3339 text format. If specified, the instance termination action will be performed at the termination time.
*
*
* optional string termination_time = 428082984;
*
* @return The terminationTime.
*/
public java.lang.String getTerminationTime() {
java.lang.Object ref = terminationTime_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
terminationTime_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Specifies the timestamp, when the instance will be terminated, in RFC3339 text format. If specified, the instance termination action will be performed at the termination time.
*
*
* optional string termination_time = 428082984;
*
* @return The bytes for terminationTime.
*/
public com.google.protobuf.ByteString getTerminationTimeBytes() {
java.lang.Object ref = terminationTime_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
terminationTime_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Specifies the timestamp, when the instance will be terminated, in RFC3339 text format. If specified, the instance termination action will be performed at the termination time.
*
*
* optional string termination_time = 428082984;
*
* @param value The terminationTime to set.
* @return This builder for chaining.
*/
public Builder setTerminationTime(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
terminationTime_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* Specifies the timestamp, when the instance will be terminated, in RFC3339 text format. If specified, the instance termination action will be performed at the termination time.
*
*
* optional string termination_time = 428082984;
*
* @return This builder for chaining.
*/
public Builder clearTerminationTime() {
terminationTime_ = getDefaultInstance().getTerminationTime();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
return this;
}
/**
*
*
*
* Specifies the timestamp, when the instance will be terminated, in RFC3339 text format. If specified, the instance termination action will be performed at the termination time.
*
*
* optional string termination_time = 428082984;
*
* @param value The bytes for terminationTime to set.
* @return This builder for chaining.
*/
public Builder setTerminationTimeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
terminationTime_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.Scheduling)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.Scheduling)
private static final com.google.cloud.compute.v1.Scheduling DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.Scheduling();
}
public static com.google.cloud.compute.v1.Scheduling getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Scheduling parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.Scheduling getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy