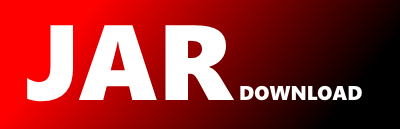
com.google.cloud.compute.v1.SecuritySettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* The authentication and authorization settings for a BackendService.
*
*
* Protobuf type {@code google.cloud.compute.v1.SecuritySettings}
*/
public final class SecuritySettings extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.SecuritySettings)
SecuritySettingsOrBuilder {
private static final long serialVersionUID = 0L;
// Use SecuritySettings.newBuilder() to construct.
private SecuritySettings(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SecuritySettings() {
clientTlsPolicy_ = "";
subjectAltNames_ = com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new SecuritySettings();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_SecuritySettings_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_SecuritySettings_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.SecuritySettings.class,
com.google.cloud.compute.v1.SecuritySettings.Builder.class);
}
private int bitField0_;
public static final int AWS_V4_AUTHENTICATION_FIELD_NUMBER = 433993111;
private com.google.cloud.compute.v1.AWSV4Signature awsV4Authentication_;
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*
* @return Whether the awsV4Authentication field is set.
*/
@java.lang.Override
public boolean hasAwsV4Authentication() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*
* @return The awsV4Authentication.
*/
@java.lang.Override
public com.google.cloud.compute.v1.AWSV4Signature getAwsV4Authentication() {
return awsV4Authentication_ == null
? com.google.cloud.compute.v1.AWSV4Signature.getDefaultInstance()
: awsV4Authentication_;
}
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.AWSV4SignatureOrBuilder getAwsV4AuthenticationOrBuilder() {
return awsV4Authentication_ == null
? com.google.cloud.compute.v1.AWSV4Signature.getDefaultInstance()
: awsV4Authentication_;
}
public static final int CLIENT_TLS_POLICY_FIELD_NUMBER = 462325226;
@SuppressWarnings("serial")
private volatile java.lang.Object clientTlsPolicy_ = "";
/**
*
*
*
* Optional. A URL referring to a networksecurity.ClientTlsPolicy resource that describes how clients should authenticate with this service's backends. clientTlsPolicy only applies to a global BackendService with the loadBalancingScheme set to INTERNAL_SELF_MANAGED. If left blank, communications are not encrypted.
*
*
* optional string client_tls_policy = 462325226;
*
* @return Whether the clientTlsPolicy field is set.
*/
@java.lang.Override
public boolean hasClientTlsPolicy() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Optional. A URL referring to a networksecurity.ClientTlsPolicy resource that describes how clients should authenticate with this service's backends. clientTlsPolicy only applies to a global BackendService with the loadBalancingScheme set to INTERNAL_SELF_MANAGED. If left blank, communications are not encrypted.
*
*
* optional string client_tls_policy = 462325226;
*
* @return The clientTlsPolicy.
*/
@java.lang.Override
public java.lang.String getClientTlsPolicy() {
java.lang.Object ref = clientTlsPolicy_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientTlsPolicy_ = s;
return s;
}
}
/**
*
*
*
* Optional. A URL referring to a networksecurity.ClientTlsPolicy resource that describes how clients should authenticate with this service's backends. clientTlsPolicy only applies to a global BackendService with the loadBalancingScheme set to INTERNAL_SELF_MANAGED. If left blank, communications are not encrypted.
*
*
* optional string client_tls_policy = 462325226;
*
* @return The bytes for clientTlsPolicy.
*/
@java.lang.Override
public com.google.protobuf.ByteString getClientTlsPolicyBytes() {
java.lang.Object ref = clientTlsPolicy_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
clientTlsPolicy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBJECT_ALT_NAMES_FIELD_NUMBER = 330029535;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList subjectAltNames_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @return A list containing the subjectAltNames.
*/
public com.google.protobuf.ProtocolStringList getSubjectAltNamesList() {
return subjectAltNames_;
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @return The count of subjectAltNames.
*/
public int getSubjectAltNamesCount() {
return subjectAltNames_.size();
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @param index The index of the element to return.
* @return The subjectAltNames at the given index.
*/
public java.lang.String getSubjectAltNames(int index) {
return subjectAltNames_.get(index);
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @param index The index of the value to return.
* @return The bytes of the subjectAltNames at the given index.
*/
public com.google.protobuf.ByteString getSubjectAltNamesBytes(int index) {
return subjectAltNames_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
for (int i = 0; i < subjectAltNames_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 330029535, subjectAltNames_.getRaw(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(433993111, getAwsV4Authentication());
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 462325226, clientTlsPolicy_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < subjectAltNames_.size(); i++) {
dataSize += computeStringSizeNoTag(subjectAltNames_.getRaw(i));
}
size += dataSize;
size += 5 * getSubjectAltNamesList().size();
}
if (((bitField0_ & 0x00000001) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
433993111, getAwsV4Authentication());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(462325226, clientTlsPolicy_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.SecuritySettings)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.SecuritySettings other =
(com.google.cloud.compute.v1.SecuritySettings) obj;
if (hasAwsV4Authentication() != other.hasAwsV4Authentication()) return false;
if (hasAwsV4Authentication()) {
if (!getAwsV4Authentication().equals(other.getAwsV4Authentication())) return false;
}
if (hasClientTlsPolicy() != other.hasClientTlsPolicy()) return false;
if (hasClientTlsPolicy()) {
if (!getClientTlsPolicy().equals(other.getClientTlsPolicy())) return false;
}
if (!getSubjectAltNamesList().equals(other.getSubjectAltNamesList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasAwsV4Authentication()) {
hash = (37 * hash) + AWS_V4_AUTHENTICATION_FIELD_NUMBER;
hash = (53 * hash) + getAwsV4Authentication().hashCode();
}
if (hasClientTlsPolicy()) {
hash = (37 * hash) + CLIENT_TLS_POLICY_FIELD_NUMBER;
hash = (53 * hash) + getClientTlsPolicy().hashCode();
}
if (getSubjectAltNamesCount() > 0) {
hash = (37 * hash) + SUBJECT_ALT_NAMES_FIELD_NUMBER;
hash = (53 * hash) + getSubjectAltNamesList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.SecuritySettings parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.SecuritySettings parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.SecuritySettings parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.SecuritySettings parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.SecuritySettings parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.SecuritySettings parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.SecuritySettings parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.SecuritySettings parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.SecuritySettings parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.SecuritySettings parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.SecuritySettings parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.SecuritySettings parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.SecuritySettings prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* The authentication and authorization settings for a BackendService.
*
*
* Protobuf type {@code google.cloud.compute.v1.SecuritySettings}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.SecuritySettings)
com.google.cloud.compute.v1.SecuritySettingsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_SecuritySettings_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_SecuritySettings_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.SecuritySettings.class,
com.google.cloud.compute.v1.SecuritySettings.Builder.class);
}
// Construct using com.google.cloud.compute.v1.SecuritySettings.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getAwsV4AuthenticationFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
awsV4Authentication_ = null;
if (awsV4AuthenticationBuilder_ != null) {
awsV4AuthenticationBuilder_.dispose();
awsV4AuthenticationBuilder_ = null;
}
clientTlsPolicy_ = "";
subjectAltNames_ = com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_SecuritySettings_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.SecuritySettings getDefaultInstanceForType() {
return com.google.cloud.compute.v1.SecuritySettings.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.SecuritySettings build() {
com.google.cloud.compute.v1.SecuritySettings result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.SecuritySettings buildPartial() {
com.google.cloud.compute.v1.SecuritySettings result =
new com.google.cloud.compute.v1.SecuritySettings(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.compute.v1.SecuritySettings result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.awsV4Authentication_ =
awsV4AuthenticationBuilder_ == null
? awsV4Authentication_
: awsV4AuthenticationBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.clientTlsPolicy_ = clientTlsPolicy_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
subjectAltNames_.makeImmutable();
result.subjectAltNames_ = subjectAltNames_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.SecuritySettings) {
return mergeFrom((com.google.cloud.compute.v1.SecuritySettings) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.SecuritySettings other) {
if (other == com.google.cloud.compute.v1.SecuritySettings.getDefaultInstance()) return this;
if (other.hasAwsV4Authentication()) {
mergeAwsV4Authentication(other.getAwsV4Authentication());
}
if (other.hasClientTlsPolicy()) {
clientTlsPolicy_ = other.clientTlsPolicy_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.subjectAltNames_.isEmpty()) {
if (subjectAltNames_.isEmpty()) {
subjectAltNames_ = other.subjectAltNames_;
bitField0_ |= 0x00000004;
} else {
ensureSubjectAltNamesIsMutable();
subjectAltNames_.addAll(other.subjectAltNames_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case -1654731014:
{
java.lang.String s = input.readStringRequireUtf8();
ensureSubjectAltNamesIsMutable();
subjectAltNames_.add(s);
break;
} // case -1654731014
case -823022406:
{
input.readMessage(
getAwsV4AuthenticationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case -823022406
case -596365486:
{
clientTlsPolicy_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case -596365486
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.cloud.compute.v1.AWSV4Signature awsV4Authentication_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AWSV4Signature,
com.google.cloud.compute.v1.AWSV4Signature.Builder,
com.google.cloud.compute.v1.AWSV4SignatureOrBuilder>
awsV4AuthenticationBuilder_;
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*
* @return Whether the awsV4Authentication field is set.
*/
public boolean hasAwsV4Authentication() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*
* @return The awsV4Authentication.
*/
public com.google.cloud.compute.v1.AWSV4Signature getAwsV4Authentication() {
if (awsV4AuthenticationBuilder_ == null) {
return awsV4Authentication_ == null
? com.google.cloud.compute.v1.AWSV4Signature.getDefaultInstance()
: awsV4Authentication_;
} else {
return awsV4AuthenticationBuilder_.getMessage();
}
}
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*/
public Builder setAwsV4Authentication(com.google.cloud.compute.v1.AWSV4Signature value) {
if (awsV4AuthenticationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
awsV4Authentication_ = value;
} else {
awsV4AuthenticationBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*/
public Builder setAwsV4Authentication(
com.google.cloud.compute.v1.AWSV4Signature.Builder builderForValue) {
if (awsV4AuthenticationBuilder_ == null) {
awsV4Authentication_ = builderForValue.build();
} else {
awsV4AuthenticationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*/
public Builder mergeAwsV4Authentication(com.google.cloud.compute.v1.AWSV4Signature value) {
if (awsV4AuthenticationBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)
&& awsV4Authentication_ != null
&& awsV4Authentication_
!= com.google.cloud.compute.v1.AWSV4Signature.getDefaultInstance()) {
getAwsV4AuthenticationBuilder().mergeFrom(value);
} else {
awsV4Authentication_ = value;
}
} else {
awsV4AuthenticationBuilder_.mergeFrom(value);
}
if (awsV4Authentication_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*/
public Builder clearAwsV4Authentication() {
bitField0_ = (bitField0_ & ~0x00000001);
awsV4Authentication_ = null;
if (awsV4AuthenticationBuilder_ != null) {
awsV4AuthenticationBuilder_.dispose();
awsV4AuthenticationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*/
public com.google.cloud.compute.v1.AWSV4Signature.Builder getAwsV4AuthenticationBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getAwsV4AuthenticationFieldBuilder().getBuilder();
}
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*/
public com.google.cloud.compute.v1.AWSV4SignatureOrBuilder getAwsV4AuthenticationOrBuilder() {
if (awsV4AuthenticationBuilder_ != null) {
return awsV4AuthenticationBuilder_.getMessageOrBuilder();
} else {
return awsV4Authentication_ == null
? com.google.cloud.compute.v1.AWSV4Signature.getDefaultInstance()
: awsV4Authentication_;
}
}
/**
*
*
*
* The configuration needed to generate a signature for access to private storage buckets that support AWS's Signature Version 4 for authentication. Allowed only for INTERNET_IP_PORT and INTERNET_FQDN_PORT NEG backends.
*
*
* optional .google.cloud.compute.v1.AWSV4Signature aws_v4_authentication = 433993111;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AWSV4Signature,
com.google.cloud.compute.v1.AWSV4Signature.Builder,
com.google.cloud.compute.v1.AWSV4SignatureOrBuilder>
getAwsV4AuthenticationFieldBuilder() {
if (awsV4AuthenticationBuilder_ == null) {
awsV4AuthenticationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.compute.v1.AWSV4Signature,
com.google.cloud.compute.v1.AWSV4Signature.Builder,
com.google.cloud.compute.v1.AWSV4SignatureOrBuilder>(
getAwsV4Authentication(), getParentForChildren(), isClean());
awsV4Authentication_ = null;
}
return awsV4AuthenticationBuilder_;
}
private java.lang.Object clientTlsPolicy_ = "";
/**
*
*
*
* Optional. A URL referring to a networksecurity.ClientTlsPolicy resource that describes how clients should authenticate with this service's backends. clientTlsPolicy only applies to a global BackendService with the loadBalancingScheme set to INTERNAL_SELF_MANAGED. If left blank, communications are not encrypted.
*
*
* optional string client_tls_policy = 462325226;
*
* @return Whether the clientTlsPolicy field is set.
*/
public boolean hasClientTlsPolicy() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Optional. A URL referring to a networksecurity.ClientTlsPolicy resource that describes how clients should authenticate with this service's backends. clientTlsPolicy only applies to a global BackendService with the loadBalancingScheme set to INTERNAL_SELF_MANAGED. If left blank, communications are not encrypted.
*
*
* optional string client_tls_policy = 462325226;
*
* @return The clientTlsPolicy.
*/
public java.lang.String getClientTlsPolicy() {
java.lang.Object ref = clientTlsPolicy_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
clientTlsPolicy_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Optional. A URL referring to a networksecurity.ClientTlsPolicy resource that describes how clients should authenticate with this service's backends. clientTlsPolicy only applies to a global BackendService with the loadBalancingScheme set to INTERNAL_SELF_MANAGED. If left blank, communications are not encrypted.
*
*
* optional string client_tls_policy = 462325226;
*
* @return The bytes for clientTlsPolicy.
*/
public com.google.protobuf.ByteString getClientTlsPolicyBytes() {
java.lang.Object ref = clientTlsPolicy_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
clientTlsPolicy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Optional. A URL referring to a networksecurity.ClientTlsPolicy resource that describes how clients should authenticate with this service's backends. clientTlsPolicy only applies to a global BackendService with the loadBalancingScheme set to INTERNAL_SELF_MANAGED. If left blank, communications are not encrypted.
*
*
* optional string client_tls_policy = 462325226;
*
* @param value The clientTlsPolicy to set.
* @return This builder for chaining.
*/
public Builder setClientTlsPolicy(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
clientTlsPolicy_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Optional. A URL referring to a networksecurity.ClientTlsPolicy resource that describes how clients should authenticate with this service's backends. clientTlsPolicy only applies to a global BackendService with the loadBalancingScheme set to INTERNAL_SELF_MANAGED. If left blank, communications are not encrypted.
*
*
* optional string client_tls_policy = 462325226;
*
* @return This builder for chaining.
*/
public Builder clearClientTlsPolicy() {
clientTlsPolicy_ = getDefaultInstance().getClientTlsPolicy();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* Optional. A URL referring to a networksecurity.ClientTlsPolicy resource that describes how clients should authenticate with this service's backends. clientTlsPolicy only applies to a global BackendService with the loadBalancingScheme set to INTERNAL_SELF_MANAGED. If left blank, communications are not encrypted.
*
*
* optional string client_tls_policy = 462325226;
*
* @param value The bytes for clientTlsPolicy to set.
* @return This builder for chaining.
*/
public Builder setClientTlsPolicyBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
clientTlsPolicy_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList subjectAltNames_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureSubjectAltNamesIsMutable() {
if (!subjectAltNames_.isModifiable()) {
subjectAltNames_ = new com.google.protobuf.LazyStringArrayList(subjectAltNames_);
}
bitField0_ |= 0x00000004;
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @return A list containing the subjectAltNames.
*/
public com.google.protobuf.ProtocolStringList getSubjectAltNamesList() {
subjectAltNames_.makeImmutable();
return subjectAltNames_;
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @return The count of subjectAltNames.
*/
public int getSubjectAltNamesCount() {
return subjectAltNames_.size();
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @param index The index of the element to return.
* @return The subjectAltNames at the given index.
*/
public java.lang.String getSubjectAltNames(int index) {
return subjectAltNames_.get(index);
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @param index The index of the value to return.
* @return The bytes of the subjectAltNames at the given index.
*/
public com.google.protobuf.ByteString getSubjectAltNamesBytes(int index) {
return subjectAltNames_.getByteString(index);
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @param index The index to set the value at.
* @param value The subjectAltNames to set.
* @return This builder for chaining.
*/
public Builder setSubjectAltNames(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSubjectAltNamesIsMutable();
subjectAltNames_.set(index, value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @param value The subjectAltNames to add.
* @return This builder for chaining.
*/
public Builder addSubjectAltNames(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSubjectAltNamesIsMutable();
subjectAltNames_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @param values The subjectAltNames to add.
* @return This builder for chaining.
*/
public Builder addAllSubjectAltNames(java.lang.Iterable values) {
ensureSubjectAltNamesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, subjectAltNames_);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @return This builder for chaining.
*/
public Builder clearSubjectAltNames() {
subjectAltNames_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
;
onChanged();
return this;
}
/**
*
*
*
* Optional. A list of Subject Alternative Names (SANs) that the client verifies during a mutual TLS handshake with an server/endpoint for this BackendService. When the server presents its X.509 certificate to the client, the client inspects the certificate's subjectAltName field. If the field contains one of the specified values, the communication continues. Otherwise, it fails. This additional check enables the client to verify that the server is authorized to run the requested service. Note that the contents of the server certificate's subjectAltName field are configured by the Public Key Infrastructure which provisions server identities. Only applies to a global BackendService with loadBalancingScheme set to INTERNAL_SELF_MANAGED. Only applies when BackendService has an attached clientTlsPolicy with clientCertificate (mTLS mode).
*
*
* repeated string subject_alt_names = 330029535;
*
* @param value The bytes of the subjectAltNames to add.
* @return This builder for chaining.
*/
public Builder addSubjectAltNamesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSubjectAltNamesIsMutable();
subjectAltNames_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.SecuritySettings)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.SecuritySettings)
private static final com.google.cloud.compute.v1.SecuritySettings DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.SecuritySettings();
}
public static com.google.cloud.compute.v1.SecuritySettings getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SecuritySettings parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.SecuritySettings getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy