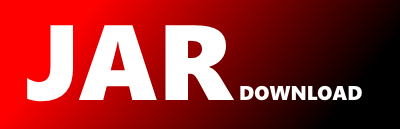
com.google.cloud.compute.v1.StoragePoolDisk Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
*
*
* Protobuf type {@code google.cloud.compute.v1.StoragePoolDisk}
*/
public final class StoragePoolDisk extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.StoragePoolDisk)
StoragePoolDiskOrBuilder {
private static final long serialVersionUID = 0L;
// Use StoragePoolDisk.newBuilder() to construct.
private StoragePoolDisk(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StoragePoolDisk() {
attachedInstances_ = com.google.protobuf.LazyStringArrayList.emptyList();
creationTimestamp_ = "";
disk_ = "";
name_ = "";
resourcePolicies_ = com.google.protobuf.LazyStringArrayList.emptyList();
status_ = "";
type_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new StoragePoolDisk();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_StoragePoolDisk_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_StoragePoolDisk_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.StoragePoolDisk.class,
com.google.cloud.compute.v1.StoragePoolDisk.Builder.class);
}
/**
*
*
*
* [Output Only] The disk status.
*
*
* Protobuf enum {@code google.cloud.compute.v1.StoragePoolDisk.Status}
*/
public enum Status implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STATUS = 0;
*/
UNDEFINED_STATUS(0),
/**
*
*
*
* Disk is provisioning
*
*
* CREATING = 455564985;
*/
CREATING(455564985),
/**
*
*
*
* Disk is deleting.
*
*
* DELETING = 528602024;
*/
DELETING(528602024),
/**
*
*
*
* Disk creation failed.
*
*
* FAILED = 455706685;
*/
FAILED(455706685),
/**
*
*
*
* Disk is ready for use.
*
*
* READY = 77848963;
*/
READY(77848963),
/**
*
*
*
* Source data is being copied into the disk.
*
*
* RESTORING = 404263851;
*/
RESTORING(404263851),
/**
*
*
*
* Disk is currently unavailable and cannot be accessed, attached or detached.
*
*
* UNAVAILABLE = 413756464;
*/
UNAVAILABLE(413756464),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STATUS = 0;
*/
public static final int UNDEFINED_STATUS_VALUE = 0;
/**
*
*
*
* Disk is provisioning
*
*
* CREATING = 455564985;
*/
public static final int CREATING_VALUE = 455564985;
/**
*
*
*
* Disk is deleting.
*
*
* DELETING = 528602024;
*/
public static final int DELETING_VALUE = 528602024;
/**
*
*
*
* Disk creation failed.
*
*
* FAILED = 455706685;
*/
public static final int FAILED_VALUE = 455706685;
/**
*
*
*
* Disk is ready for use.
*
*
* READY = 77848963;
*/
public static final int READY_VALUE = 77848963;
/**
*
*
*
* Source data is being copied into the disk.
*
*
* RESTORING = 404263851;
*/
public static final int RESTORING_VALUE = 404263851;
/**
*
*
*
* Disk is currently unavailable and cannot be accessed, attached or detached.
*
*
* UNAVAILABLE = 413756464;
*/
public static final int UNAVAILABLE_VALUE = 413756464;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Status valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Status forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_STATUS;
case 455564985:
return CREATING;
case 528602024:
return DELETING;
case 455706685:
return FAILED;
case 77848963:
return READY;
case 404263851:
return RESTORING;
case 413756464:
return UNAVAILABLE;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Status findValueByNumber(int number) {
return Status.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.StoragePoolDisk.getDescriptor().getEnumTypes().get(0);
}
private static final Status[] VALUES = values();
public static Status valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Status(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.StoragePoolDisk.Status)
}
private int bitField0_;
public static final int ATTACHED_INSTANCES_FIELD_NUMBER = 65255843;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList attachedInstances_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @return A list containing the attachedInstances.
*/
public com.google.protobuf.ProtocolStringList getAttachedInstancesList() {
return attachedInstances_;
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @return The count of attachedInstances.
*/
public int getAttachedInstancesCount() {
return attachedInstances_.size();
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @param index The index of the element to return.
* @return The attachedInstances at the given index.
*/
public java.lang.String getAttachedInstances(int index) {
return attachedInstances_.get(index);
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @param index The index of the value to return.
* @return The bytes of the attachedInstances at the given index.
*/
public com.google.protobuf.ByteString getAttachedInstancesBytes(int index) {
return attachedInstances_.getByteString(index);
}
public static final int CREATION_TIMESTAMP_FIELD_NUMBER = 30525366;
@SuppressWarnings("serial")
private volatile java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
@java.lang.Override
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
@java.lang.Override
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DISK_FIELD_NUMBER = 3083677;
@SuppressWarnings("serial")
private volatile java.lang.Object disk_ = "";
/**
*
*
*
* [Output Only] The URL of the disk.
*
*
* optional string disk = 3083677;
*
* @return Whether the disk field is set.
*/
@java.lang.Override
public boolean hasDisk() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* [Output Only] The URL of the disk.
*
*
* optional string disk = 3083677;
*
* @return The disk.
*/
@java.lang.Override
public java.lang.String getDisk() {
java.lang.Object ref = disk_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
disk_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The URL of the disk.
*
*
* optional string disk = 3083677;
*
* @return The bytes for disk.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDiskBytes() {
java.lang.Object ref = disk_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
disk_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 3373707;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* [Output Only] The name of the disk.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* [Output Only] The name of the disk.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The name of the disk.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROVISIONED_IOPS_FIELD_NUMBER = 186769108;
private long provisionedIops_ = 0L;
/**
*
*
*
* [Output Only] The number of IOPS provisioned for the disk.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @return Whether the provisionedIops field is set.
*/
@java.lang.Override
public boolean hasProvisionedIops() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* [Output Only] The number of IOPS provisioned for the disk.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @return The provisionedIops.
*/
@java.lang.Override
public long getProvisionedIops() {
return provisionedIops_;
}
public static final int PROVISIONED_THROUGHPUT_FIELD_NUMBER = 526524181;
private long provisionedThroughput_ = 0L;
/**
*
*
*
* [Output Only] The throughput provisioned for the disk.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @return Whether the provisionedThroughput field is set.
*/
@java.lang.Override
public boolean hasProvisionedThroughput() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* [Output Only] The throughput provisioned for the disk.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @return The provisionedThroughput.
*/
@java.lang.Override
public long getProvisionedThroughput() {
return provisionedThroughput_;
}
public static final int RESOURCE_POLICIES_FIELD_NUMBER = 22220385;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList resourcePolicies_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @return A list containing the resourcePolicies.
*/
public com.google.protobuf.ProtocolStringList getResourcePoliciesList() {
return resourcePolicies_;
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @return The count of resourcePolicies.
*/
public int getResourcePoliciesCount() {
return resourcePolicies_.size();
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param index The index of the element to return.
* @return The resourcePolicies at the given index.
*/
public java.lang.String getResourcePolicies(int index) {
return resourcePolicies_.get(index);
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param index The index of the value to return.
* @return The bytes of the resourcePolicies at the given index.
*/
public com.google.protobuf.ByteString getResourcePoliciesBytes(int index) {
return resourcePolicies_.getByteString(index);
}
public static final int SIZE_GB_FIELD_NUMBER = 494929369;
private long sizeGb_ = 0L;
/**
*
*
*
* [Output Only] The disk size, in GB.
*
*
* optional int64 size_gb = 494929369;
*
* @return Whether the sizeGb field is set.
*/
@java.lang.Override
public boolean hasSizeGb() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* [Output Only] The disk size, in GB.
*
*
* optional int64 size_gb = 494929369;
*
* @return The sizeGb.
*/
@java.lang.Override
public long getSizeGb() {
return sizeGb_;
}
public static final int STATUS_FIELD_NUMBER = 181260274;
@SuppressWarnings("serial")
private volatile java.lang.Object status_ = "";
/**
*
*
*
* [Output Only] The disk status.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return Whether the status field is set.
*/
@java.lang.Override
public boolean hasStatus() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* [Output Only] The disk status.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The status.
*/
@java.lang.Override
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
status_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The disk status.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The bytes for status.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TYPE_FIELD_NUMBER = 3575610;
@SuppressWarnings("serial")
private volatile java.lang.Object type_ = "";
/**
*
*
*
* [Output Only] The disk type.
*
*
* optional string type = 3575610;
*
* @return Whether the type field is set.
*/
@java.lang.Override
public boolean hasType() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* [Output Only] The disk type.
*
*
* optional string type = 3575610;
*
* @return The type.
*/
@java.lang.Override
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The disk type.
*
*
* optional string type = 3575610;
*
* @return The bytes for type.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int USED_BYTES_FIELD_NUMBER = 231640425;
private long usedBytes_ = 0L;
/**
*
*
*
* [Output Only] Amount of disk space used.
*
*
* optional int64 used_bytes = 231640425;
*
* @return Whether the usedBytes field is set.
*/
@java.lang.Override
public boolean hasUsedBytes() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* [Output Only] Amount of disk space used.
*
*
* optional int64 used_bytes = 231640425;
*
* @return The usedBytes.
*/
@java.lang.Override
public long getUsedBytes() {
return usedBytes_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3083677, disk_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3373707, name_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3575610, type_);
}
for (int i = 0; i < resourcePolicies_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 22220385, resourcePolicies_.getRaw(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30525366, creationTimestamp_);
}
for (int i = 0; i < attachedInstances_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 65255843, attachedInstances_.getRaw(i));
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 181260274, status_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeInt64(186769108, provisionedIops_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeInt64(231640425, usedBytes_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeInt64(494929369, sizeGb_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeInt64(526524181, provisionedThroughput_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3083677, disk_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3373707, name_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3575610, type_);
}
{
int dataSize = 0;
for (int i = 0; i < resourcePolicies_.size(); i++) {
dataSize += computeStringSizeNoTag(resourcePolicies_.getRaw(i));
}
size += dataSize;
size += 4 * getResourcePoliciesList().size();
}
if (((bitField0_ & 0x00000001) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(30525366, creationTimestamp_);
}
{
int dataSize = 0;
for (int i = 0; i < attachedInstances_.size(); i++) {
dataSize += computeStringSizeNoTag(attachedInstances_.getRaw(i));
}
size += dataSize;
size += 5 * getAttachedInstancesList().size();
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(181260274, status_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(186769108, provisionedIops_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(231640425, usedBytes_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(494929369, sizeGb_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeInt64Size(526524181, provisionedThroughput_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.StoragePoolDisk)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.StoragePoolDisk other =
(com.google.cloud.compute.v1.StoragePoolDisk) obj;
if (!getAttachedInstancesList().equals(other.getAttachedInstancesList())) return false;
if (hasCreationTimestamp() != other.hasCreationTimestamp()) return false;
if (hasCreationTimestamp()) {
if (!getCreationTimestamp().equals(other.getCreationTimestamp())) return false;
}
if (hasDisk() != other.hasDisk()) return false;
if (hasDisk()) {
if (!getDisk().equals(other.getDisk())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName().equals(other.getName())) return false;
}
if (hasProvisionedIops() != other.hasProvisionedIops()) return false;
if (hasProvisionedIops()) {
if (getProvisionedIops() != other.getProvisionedIops()) return false;
}
if (hasProvisionedThroughput() != other.hasProvisionedThroughput()) return false;
if (hasProvisionedThroughput()) {
if (getProvisionedThroughput() != other.getProvisionedThroughput()) return false;
}
if (!getResourcePoliciesList().equals(other.getResourcePoliciesList())) return false;
if (hasSizeGb() != other.hasSizeGb()) return false;
if (hasSizeGb()) {
if (getSizeGb() != other.getSizeGb()) return false;
}
if (hasStatus() != other.hasStatus()) return false;
if (hasStatus()) {
if (!getStatus().equals(other.getStatus())) return false;
}
if (hasType() != other.hasType()) return false;
if (hasType()) {
if (!getType().equals(other.getType())) return false;
}
if (hasUsedBytes() != other.hasUsedBytes()) return false;
if (hasUsedBytes()) {
if (getUsedBytes() != other.getUsedBytes()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getAttachedInstancesCount() > 0) {
hash = (37 * hash) + ATTACHED_INSTANCES_FIELD_NUMBER;
hash = (53 * hash) + getAttachedInstancesList().hashCode();
}
if (hasCreationTimestamp()) {
hash = (37 * hash) + CREATION_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getCreationTimestamp().hashCode();
}
if (hasDisk()) {
hash = (37 * hash) + DISK_FIELD_NUMBER;
hash = (53 * hash) + getDisk().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasProvisionedIops()) {
hash = (37 * hash) + PROVISIONED_IOPS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getProvisionedIops());
}
if (hasProvisionedThroughput()) {
hash = (37 * hash) + PROVISIONED_THROUGHPUT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getProvisionedThroughput());
}
if (getResourcePoliciesCount() > 0) {
hash = (37 * hash) + RESOURCE_POLICIES_FIELD_NUMBER;
hash = (53 * hash) + getResourcePoliciesList().hashCode();
}
if (hasSizeGb()) {
hash = (37 * hash) + SIZE_GB_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getSizeGb());
}
if (hasStatus()) {
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + getStatus().hashCode();
}
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
}
if (hasUsedBytes()) {
hash = (37 * hash) + USED_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getUsedBytes());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.StoragePoolDisk parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.StoragePoolDisk prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
*
*
* Protobuf type {@code google.cloud.compute.v1.StoragePoolDisk}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.StoragePoolDisk)
com.google.cloud.compute.v1.StoragePoolDiskOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_StoragePoolDisk_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_StoragePoolDisk_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.StoragePoolDisk.class,
com.google.cloud.compute.v1.StoragePoolDisk.Builder.class);
}
// Construct using com.google.cloud.compute.v1.StoragePoolDisk.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
attachedInstances_ = com.google.protobuf.LazyStringArrayList.emptyList();
creationTimestamp_ = "";
disk_ = "";
name_ = "";
provisionedIops_ = 0L;
provisionedThroughput_ = 0L;
resourcePolicies_ = com.google.protobuf.LazyStringArrayList.emptyList();
sizeGb_ = 0L;
status_ = "";
type_ = "";
usedBytes_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_StoragePoolDisk_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.StoragePoolDisk getDefaultInstanceForType() {
return com.google.cloud.compute.v1.StoragePoolDisk.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.StoragePoolDisk build() {
com.google.cloud.compute.v1.StoragePoolDisk result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.StoragePoolDisk buildPartial() {
com.google.cloud.compute.v1.StoragePoolDisk result =
new com.google.cloud.compute.v1.StoragePoolDisk(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.compute.v1.StoragePoolDisk result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
attachedInstances_.makeImmutable();
result.attachedInstances_ = attachedInstances_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.creationTimestamp_ = creationTimestamp_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.disk_ = disk_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.provisionedIops_ = provisionedIops_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.provisionedThroughput_ = provisionedThroughput_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
resourcePolicies_.makeImmutable();
result.resourcePolicies_ = resourcePolicies_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.sizeGb_ = sizeGb_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.status_ = status_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.type_ = type_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.usedBytes_ = usedBytes_;
to_bitField0_ |= 0x00000100;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.StoragePoolDisk) {
return mergeFrom((com.google.cloud.compute.v1.StoragePoolDisk) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.StoragePoolDisk other) {
if (other == com.google.cloud.compute.v1.StoragePoolDisk.getDefaultInstance()) return this;
if (!other.attachedInstances_.isEmpty()) {
if (attachedInstances_.isEmpty()) {
attachedInstances_ = other.attachedInstances_;
bitField0_ |= 0x00000001;
} else {
ensureAttachedInstancesIsMutable();
attachedInstances_.addAll(other.attachedInstances_);
}
onChanged();
}
if (other.hasCreationTimestamp()) {
creationTimestamp_ = other.creationTimestamp_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasDisk()) {
disk_ = other.disk_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasProvisionedIops()) {
setProvisionedIops(other.getProvisionedIops());
}
if (other.hasProvisionedThroughput()) {
setProvisionedThroughput(other.getProvisionedThroughput());
}
if (!other.resourcePolicies_.isEmpty()) {
if (resourcePolicies_.isEmpty()) {
resourcePolicies_ = other.resourcePolicies_;
bitField0_ |= 0x00000040;
} else {
ensureResourcePoliciesIsMutable();
resourcePolicies_.addAll(other.resourcePolicies_);
}
onChanged();
}
if (other.hasSizeGb()) {
setSizeGb(other.getSizeGb());
}
if (other.hasStatus()) {
status_ = other.status_;
bitField0_ |= 0x00000100;
onChanged();
}
if (other.hasType()) {
type_ = other.type_;
bitField0_ |= 0x00000200;
onChanged();
}
if (other.hasUsedBytes()) {
setUsedBytes(other.getUsedBytes());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 24669418:
{
disk_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 24669418
case 26989658:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 26989658
case 28604882:
{
type_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case 28604882
case 177763082:
{
java.lang.String s = input.readStringRequireUtf8();
ensureResourcePoliciesIsMutable();
resourcePolicies_.add(s);
break;
} // case 177763082
case 244202930:
{
creationTimestamp_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 244202930
case 522046746:
{
java.lang.String s = input.readStringRequireUtf8();
ensureAttachedInstancesIsMutable();
attachedInstances_.add(s);
break;
} // case 522046746
case 1450082194:
{
status_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case 1450082194
case 1494152864:
{
provisionedIops_ = input.readInt64();
bitField0_ |= 0x00000010;
break;
} // case 1494152864
case 1853123400:
{
usedBytes_ = input.readInt64();
bitField0_ |= 0x00000400;
break;
} // case 1853123400
case -335532344:
{
sizeGb_ = input.readInt64();
bitField0_ |= 0x00000080;
break;
} // case -335532344
case -82773848:
{
provisionedThroughput_ = input.readInt64();
bitField0_ |= 0x00000020;
break;
} // case -82773848
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList attachedInstances_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureAttachedInstancesIsMutable() {
if (!attachedInstances_.isModifiable()) {
attachedInstances_ = new com.google.protobuf.LazyStringArrayList(attachedInstances_);
}
bitField0_ |= 0x00000001;
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @return A list containing the attachedInstances.
*/
public com.google.protobuf.ProtocolStringList getAttachedInstancesList() {
attachedInstances_.makeImmutable();
return attachedInstances_;
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @return The count of attachedInstances.
*/
public int getAttachedInstancesCount() {
return attachedInstances_.size();
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @param index The index of the element to return.
* @return The attachedInstances at the given index.
*/
public java.lang.String getAttachedInstances(int index) {
return attachedInstances_.get(index);
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @param index The index of the value to return.
* @return The bytes of the attachedInstances at the given index.
*/
public com.google.protobuf.ByteString getAttachedInstancesBytes(int index) {
return attachedInstances_.getByteString(index);
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @param index The index to set the value at.
* @param value The attachedInstances to set.
* @return This builder for chaining.
*/
public Builder setAttachedInstances(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttachedInstancesIsMutable();
attachedInstances_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @param value The attachedInstances to add.
* @return This builder for chaining.
*/
public Builder addAttachedInstances(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttachedInstancesIsMutable();
attachedInstances_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @param values The attachedInstances to add.
* @return This builder for chaining.
*/
public Builder addAllAttachedInstances(java.lang.Iterable values) {
ensureAttachedInstancesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, attachedInstances_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @return This builder for chaining.
*/
public Builder clearAttachedInstances() {
attachedInstances_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Instances this disk is attached to.
*
*
* repeated string attached_instances = 65255843;
*
* @param value The bytes of the attachedInstances to add.
* @return This builder for chaining.
*/
public Builder addAttachedInstancesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureAttachedInstancesIsMutable();
attachedInstances_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestamp(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
creationTimestamp_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return This builder for chaining.
*/
public Builder clearCreationTimestamp() {
creationTimestamp_ = getDefaultInstance().getCreationTimestamp();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The bytes for creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestampBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
creationTimestamp_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object disk_ = "";
/**
*
*
*
* [Output Only] The URL of the disk.
*
*
* optional string disk = 3083677;
*
* @return Whether the disk field is set.
*/
public boolean hasDisk() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* [Output Only] The URL of the disk.
*
*
* optional string disk = 3083677;
*
* @return The disk.
*/
public java.lang.String getDisk() {
java.lang.Object ref = disk_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
disk_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The URL of the disk.
*
*
* optional string disk = 3083677;
*
* @return The bytes for disk.
*/
public com.google.protobuf.ByteString getDiskBytes() {
java.lang.Object ref = disk_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
disk_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The URL of the disk.
*
*
* optional string disk = 3083677;
*
* @param value The disk to set.
* @return This builder for chaining.
*/
public Builder setDisk(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
disk_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The URL of the disk.
*
*
* optional string disk = 3083677;
*
* @return This builder for chaining.
*/
public Builder clearDisk() {
disk_ = getDefaultInstance().getDisk();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The URL of the disk.
*
*
* optional string disk = 3083677;
*
* @param value The bytes for disk to set.
* @return This builder for chaining.
*/
public Builder setDiskBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
disk_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
*
*
* [Output Only] The name of the disk.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* [Output Only] The name of the disk.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The name of the disk.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The name of the disk.
*
*
* optional string name = 3373707;
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The name of the disk.
*
*
* optional string name = 3373707;
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The name of the disk.
*
*
* optional string name = 3373707;
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private long provisionedIops_;
/**
*
*
*
* [Output Only] The number of IOPS provisioned for the disk.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @return Whether the provisionedIops field is set.
*/
@java.lang.Override
public boolean hasProvisionedIops() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* [Output Only] The number of IOPS provisioned for the disk.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @return The provisionedIops.
*/
@java.lang.Override
public long getProvisionedIops() {
return provisionedIops_;
}
/**
*
*
*
* [Output Only] The number of IOPS provisioned for the disk.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @param value The provisionedIops to set.
* @return This builder for chaining.
*/
public Builder setProvisionedIops(long value) {
provisionedIops_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The number of IOPS provisioned for the disk.
*
*
* optional int64 provisioned_iops = 186769108;
*
* @return This builder for chaining.
*/
public Builder clearProvisionedIops() {
bitField0_ = (bitField0_ & ~0x00000010);
provisionedIops_ = 0L;
onChanged();
return this;
}
private long provisionedThroughput_;
/**
*
*
*
* [Output Only] The throughput provisioned for the disk.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @return Whether the provisionedThroughput field is set.
*/
@java.lang.Override
public boolean hasProvisionedThroughput() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* [Output Only] The throughput provisioned for the disk.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @return The provisionedThroughput.
*/
@java.lang.Override
public long getProvisionedThroughput() {
return provisionedThroughput_;
}
/**
*
*
*
* [Output Only] The throughput provisioned for the disk.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @param value The provisionedThroughput to set.
* @return This builder for chaining.
*/
public Builder setProvisionedThroughput(long value) {
provisionedThroughput_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The throughput provisioned for the disk.
*
*
* optional int64 provisioned_throughput = 526524181;
*
* @return This builder for chaining.
*/
public Builder clearProvisionedThroughput() {
bitField0_ = (bitField0_ & ~0x00000020);
provisionedThroughput_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList resourcePolicies_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureResourcePoliciesIsMutable() {
if (!resourcePolicies_.isModifiable()) {
resourcePolicies_ = new com.google.protobuf.LazyStringArrayList(resourcePolicies_);
}
bitField0_ |= 0x00000040;
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @return A list containing the resourcePolicies.
*/
public com.google.protobuf.ProtocolStringList getResourcePoliciesList() {
resourcePolicies_.makeImmutable();
return resourcePolicies_;
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @return The count of resourcePolicies.
*/
public int getResourcePoliciesCount() {
return resourcePolicies_.size();
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param index The index of the element to return.
* @return The resourcePolicies at the given index.
*/
public java.lang.String getResourcePolicies(int index) {
return resourcePolicies_.get(index);
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param index The index of the value to return.
* @return The bytes of the resourcePolicies at the given index.
*/
public com.google.protobuf.ByteString getResourcePoliciesBytes(int index) {
return resourcePolicies_.getByteString(index);
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param index The index to set the value at.
* @param value The resourcePolicies to set.
* @return This builder for chaining.
*/
public Builder setResourcePolicies(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureResourcePoliciesIsMutable();
resourcePolicies_.set(index, value);
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param value The resourcePolicies to add.
* @return This builder for chaining.
*/
public Builder addResourcePolicies(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureResourcePoliciesIsMutable();
resourcePolicies_.add(value);
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param values The resourcePolicies to add.
* @return This builder for chaining.
*/
public Builder addAllResourcePolicies(java.lang.Iterable values) {
ensureResourcePoliciesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, resourcePolicies_);
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @return This builder for chaining.
*/
public Builder clearResourcePolicies() {
resourcePolicies_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Resource policies applied to disk for automatic snapshot creations.
*
*
* repeated string resource_policies = 22220385;
*
* @param value The bytes of the resourcePolicies to add.
* @return This builder for chaining.
*/
public Builder addResourcePoliciesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureResourcePoliciesIsMutable();
resourcePolicies_.add(value);
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private long sizeGb_;
/**
*
*
*
* [Output Only] The disk size, in GB.
*
*
* optional int64 size_gb = 494929369;
*
* @return Whether the sizeGb field is set.
*/
@java.lang.Override
public boolean hasSizeGb() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* [Output Only] The disk size, in GB.
*
*
* optional int64 size_gb = 494929369;
*
* @return The sizeGb.
*/
@java.lang.Override
public long getSizeGb() {
return sizeGb_;
}
/**
*
*
*
* [Output Only] The disk size, in GB.
*
*
* optional int64 size_gb = 494929369;
*
* @param value The sizeGb to set.
* @return This builder for chaining.
*/
public Builder setSizeGb(long value) {
sizeGb_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The disk size, in GB.
*
*
* optional int64 size_gb = 494929369;
*
* @return This builder for chaining.
*/
public Builder clearSizeGb() {
bitField0_ = (bitField0_ & ~0x00000080);
sizeGb_ = 0L;
onChanged();
return this;
}
private java.lang.Object status_ = "";
/**
*
*
*
* [Output Only] The disk status.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return Whether the status field is set.
*/
public boolean hasStatus() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* [Output Only] The disk status.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The status.
*/
public java.lang.String getStatus() {
java.lang.Object ref = status_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
status_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The disk status.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return The bytes for status.
*/
public com.google.protobuf.ByteString getStatusBytes() {
java.lang.Object ref = status_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
status_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The disk status.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The disk status.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = getDefaultInstance().getStatus();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The disk status.
* Check the Status enum for the list of possible values.
*
*
* optional string status = 181260274;
*
* @param value The bytes for status to set.
* @return This builder for chaining.
*/
public Builder setStatusBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
status_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private java.lang.Object type_ = "";
/**
*
*
*
* [Output Only] The disk type.
*
*
* optional string type = 3575610;
*
* @return Whether the type field is set.
*/
public boolean hasType() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* [Output Only] The disk type.
*
*
* optional string type = 3575610;
*
* @return The type.
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The disk type.
*
*
* optional string type = 3575610;
*
* @return The bytes for type.
*/
public com.google.protobuf.ByteString getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The disk type.
*
*
* optional string type = 3575610;
*
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The disk type.
*
*
* optional string type = 3575610;
*
* @return This builder for chaining.
*/
public Builder clearType() {
type_ = getDefaultInstance().getType();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The disk type.
*
*
* optional string type = 3575610;
*
* @param value The bytes for type to set.
* @return This builder for chaining.
*/
public Builder setTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
type_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
private long usedBytes_;
/**
*
*
*
* [Output Only] Amount of disk space used.
*
*
* optional int64 used_bytes = 231640425;
*
* @return Whether the usedBytes field is set.
*/
@java.lang.Override
public boolean hasUsedBytes() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* [Output Only] Amount of disk space used.
*
*
* optional int64 used_bytes = 231640425;
*
* @return The usedBytes.
*/
@java.lang.Override
public long getUsedBytes() {
return usedBytes_;
}
/**
*
*
*
* [Output Only] Amount of disk space used.
*
*
* optional int64 used_bytes = 231640425;
*
* @param value The usedBytes to set.
* @return This builder for chaining.
*/
public Builder setUsedBytes(long value) {
usedBytes_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Amount of disk space used.
*
*
* optional int64 used_bytes = 231640425;
*
* @return This builder for chaining.
*/
public Builder clearUsedBytes() {
bitField0_ = (bitField0_ & ~0x00000400);
usedBytes_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.StoragePoolDisk)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.StoragePoolDisk)
private static final com.google.cloud.compute.v1.StoragePoolDisk DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.StoragePoolDisk();
}
public static com.google.cloud.compute.v1.StoragePoolDisk getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StoragePoolDisk parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.StoragePoolDisk getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy