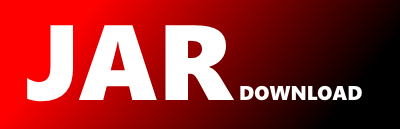
com.google.cloud.compute.v1.TargetGrpcProxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* Represents a Target gRPC Proxy resource. A target gRPC proxy is a component of load balancers intended for load balancing gRPC traffic. Only global forwarding rules with load balancing scheme INTERNAL_SELF_MANAGED can reference a target gRPC proxy. The target gRPC Proxy references a URL map that specifies how traffic is routed to gRPC backend services.
*
*
* Protobuf type {@code google.cloud.compute.v1.TargetGrpcProxy}
*/
public final class TargetGrpcProxy extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.TargetGrpcProxy)
TargetGrpcProxyOrBuilder {
private static final long serialVersionUID = 0L;
// Use TargetGrpcProxy.newBuilder() to construct.
private TargetGrpcProxy(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TargetGrpcProxy() {
creationTimestamp_ = "";
description_ = "";
fingerprint_ = "";
kind_ = "";
name_ = "";
selfLink_ = "";
selfLinkWithId_ = "";
urlMap_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new TargetGrpcProxy();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_TargetGrpcProxy_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_TargetGrpcProxy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.TargetGrpcProxy.class,
com.google.cloud.compute.v1.TargetGrpcProxy.Builder.class);
}
private int bitField0_;
public static final int CREATION_TIMESTAMP_FIELD_NUMBER = 30525366;
@SuppressWarnings("serial")
private volatile java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
@java.lang.Override
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
@java.lang.Override
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 422937596;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return Whether the description field is set.
*/
@java.lang.Override
public boolean hasDescription() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FINGERPRINT_FIELD_NUMBER = 234678500;
@SuppressWarnings("serial")
private volatile java.lang.Object fingerprint_ = "";
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. This field will be ignored when inserting a TargetGrpcProxy. An up-to-date fingerprint must be provided in order to patch/update the TargetGrpcProxy; otherwise, the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve the TargetGrpcProxy.
*
*
* optional string fingerprint = 234678500;
*
* @return Whether the fingerprint field is set.
*/
@java.lang.Override
public boolean hasFingerprint() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. This field will be ignored when inserting a TargetGrpcProxy. An up-to-date fingerprint must be provided in order to patch/update the TargetGrpcProxy; otherwise, the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve the TargetGrpcProxy.
*
*
* optional string fingerprint = 234678500;
*
* @return The fingerprint.
*/
@java.lang.Override
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fingerprint_ = s;
return s;
}
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. This field will be ignored when inserting a TargetGrpcProxy. An up-to-date fingerprint must be provided in order to patch/update the TargetGrpcProxy; otherwise, the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve the TargetGrpcProxy.
*
*
* optional string fingerprint = 234678500;
*
* @return The bytes for fingerprint.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ID_FIELD_NUMBER = 3355;
private long id_ = 0L;
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
public static final int KIND_FIELD_NUMBER = 3292052;
@SuppressWarnings("serial")
private volatile java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#targetGrpcProxy for target grpc proxies.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
@java.lang.Override
public boolean hasKind() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#targetGrpcProxy for target grpc proxies.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
@java.lang.Override
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#targetGrpcProxy for target grpc proxies.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
@java.lang.Override
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 3373707;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SELF_LINK_FIELD_NUMBER = 456214797;
@SuppressWarnings("serial")
private volatile java.lang.Object selfLink_ = "";
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return Whether the selfLink field is set.
*/
@java.lang.Override
public boolean hasSelfLink() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The selfLink.
*/
@java.lang.Override
public java.lang.String getSelfLink() {
java.lang.Object ref = selfLink_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLink_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The bytes for selfLink.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSelfLinkBytes() {
java.lang.Object ref = selfLink_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLink_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SELF_LINK_WITH_ID_FIELD_NUMBER = 44520962;
@SuppressWarnings("serial")
private volatile java.lang.Object selfLinkWithId_ = "";
/**
*
*
*
* [Output Only] Server-defined URL with id for the resource.
*
*
* optional string self_link_with_id = 44520962;
*
* @return Whether the selfLinkWithId field is set.
*/
@java.lang.Override
public boolean hasSelfLinkWithId() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* [Output Only] Server-defined URL with id for the resource.
*
*
* optional string self_link_with_id = 44520962;
*
* @return The selfLinkWithId.
*/
@java.lang.Override
public java.lang.String getSelfLinkWithId() {
java.lang.Object ref = selfLinkWithId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLinkWithId_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] Server-defined URL with id for the resource.
*
*
* optional string self_link_with_id = 44520962;
*
* @return The bytes for selfLinkWithId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSelfLinkWithIdBytes() {
java.lang.Object ref = selfLinkWithId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLinkWithId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int URL_MAP_FIELD_NUMBER = 367020684;
@SuppressWarnings("serial")
private volatile java.lang.Object urlMap_ = "";
/**
*
*
*
* URL to the UrlMap resource that defines the mapping from URL to the BackendService. The protocol field in the BackendService must be set to GRPC.
*
*
* optional string url_map = 367020684;
*
* @return Whether the urlMap field is set.
*/
@java.lang.Override
public boolean hasUrlMap() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* URL to the UrlMap resource that defines the mapping from URL to the BackendService. The protocol field in the BackendService must be set to GRPC.
*
*
* optional string url_map = 367020684;
*
* @return The urlMap.
*/
@java.lang.Override
public java.lang.String getUrlMap() {
java.lang.Object ref = urlMap_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
urlMap_ = s;
return s;
}
}
/**
*
*
*
* URL to the UrlMap resource that defines the mapping from URL to the BackendService. The protocol field in the BackendService must be set to GRPC.
*
*
* optional string url_map = 367020684;
*
* @return The bytes for urlMap.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUrlMapBytes() {
java.lang.Object ref = urlMap_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
urlMap_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALIDATE_FOR_PROXYLESS_FIELD_NUMBER = 101822888;
private boolean validateForProxyless_ = false;
/**
*
*
*
* If true, indicates that the BackendServices referenced by the urlMap may be accessed by gRPC applications without using a sidecar proxy. This will enable configuration checks on urlMap and its referenced BackendServices to not allow unsupported features. A gRPC application must use "xds:///" scheme in the target URI of the service it is connecting to. If false, indicates that the BackendServices referenced by the urlMap will be accessed by gRPC applications via a sidecar proxy. In this case, a gRPC application must not use "xds:///" scheme in the target URI of the service it is connecting to
*
*
* optional bool validate_for_proxyless = 101822888;
*
* @return Whether the validateForProxyless field is set.
*/
@java.lang.Override
public boolean hasValidateForProxyless() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* If true, indicates that the BackendServices referenced by the urlMap may be accessed by gRPC applications without using a sidecar proxy. This will enable configuration checks on urlMap and its referenced BackendServices to not allow unsupported features. A gRPC application must use "xds:///" scheme in the target URI of the service it is connecting to. If false, indicates that the BackendServices referenced by the urlMap will be accessed by gRPC applications via a sidecar proxy. In this case, a gRPC application must not use "xds:///" scheme in the target URI of the service it is connecting to
*
*
* optional bool validate_for_proxyless = 101822888;
*
* @return The validateForProxyless.
*/
@java.lang.Override
public boolean getValidateForProxyless() {
return validateForProxyless_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000008) != 0)) {
output.writeUInt64(3355, id_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3292052, kind_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3373707, name_);
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30525366, creationTimestamp_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 44520962, selfLinkWithId_);
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeBool(101822888, validateForProxyless_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 234678500, fingerprint_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 367020684, urlMap_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 422937596, description_);
}
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 456214797, selfLink_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeUInt64Size(3355, id_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3292052, kind_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3373707, name_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(30525366, creationTimestamp_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(44520962, selfLinkWithId_);
}
if (((bitField0_ & 0x00000200) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeBoolSize(101822888, validateForProxyless_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(234678500, fingerprint_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(367020684, urlMap_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(422937596, description_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(456214797, selfLink_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.TargetGrpcProxy)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.TargetGrpcProxy other =
(com.google.cloud.compute.v1.TargetGrpcProxy) obj;
if (hasCreationTimestamp() != other.hasCreationTimestamp()) return false;
if (hasCreationTimestamp()) {
if (!getCreationTimestamp().equals(other.getCreationTimestamp())) return false;
}
if (hasDescription() != other.hasDescription()) return false;
if (hasDescription()) {
if (!getDescription().equals(other.getDescription())) return false;
}
if (hasFingerprint() != other.hasFingerprint()) return false;
if (hasFingerprint()) {
if (!getFingerprint().equals(other.getFingerprint())) return false;
}
if (hasId() != other.hasId()) return false;
if (hasId()) {
if (getId() != other.getId()) return false;
}
if (hasKind() != other.hasKind()) return false;
if (hasKind()) {
if (!getKind().equals(other.getKind())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName().equals(other.getName())) return false;
}
if (hasSelfLink() != other.hasSelfLink()) return false;
if (hasSelfLink()) {
if (!getSelfLink().equals(other.getSelfLink())) return false;
}
if (hasSelfLinkWithId() != other.hasSelfLinkWithId()) return false;
if (hasSelfLinkWithId()) {
if (!getSelfLinkWithId().equals(other.getSelfLinkWithId())) return false;
}
if (hasUrlMap() != other.hasUrlMap()) return false;
if (hasUrlMap()) {
if (!getUrlMap().equals(other.getUrlMap())) return false;
}
if (hasValidateForProxyless() != other.hasValidateForProxyless()) return false;
if (hasValidateForProxyless()) {
if (getValidateForProxyless() != other.getValidateForProxyless()) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCreationTimestamp()) {
hash = (37 * hash) + CREATION_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + getCreationTimestamp().hashCode();
}
if (hasDescription()) {
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
}
if (hasFingerprint()) {
hash = (37 * hash) + FINGERPRINT_FIELD_NUMBER;
hash = (53 * hash) + getFingerprint().hashCode();
}
if (hasId()) {
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getId());
}
if (hasKind()) {
hash = (37 * hash) + KIND_FIELD_NUMBER;
hash = (53 * hash) + getKind().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasSelfLink()) {
hash = (37 * hash) + SELF_LINK_FIELD_NUMBER;
hash = (53 * hash) + getSelfLink().hashCode();
}
if (hasSelfLinkWithId()) {
hash = (37 * hash) + SELF_LINK_WITH_ID_FIELD_NUMBER;
hash = (53 * hash) + getSelfLinkWithId().hashCode();
}
if (hasUrlMap()) {
hash = (37 * hash) + URL_MAP_FIELD_NUMBER;
hash = (53 * hash) + getUrlMap().hashCode();
}
if (hasValidateForProxyless()) {
hash = (37 * hash) + VALIDATE_FOR_PROXYLESS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getValidateForProxyless());
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.TargetGrpcProxy parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.TargetGrpcProxy prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Represents a Target gRPC Proxy resource. A target gRPC proxy is a component of load balancers intended for load balancing gRPC traffic. Only global forwarding rules with load balancing scheme INTERNAL_SELF_MANAGED can reference a target gRPC proxy. The target gRPC Proxy references a URL map that specifies how traffic is routed to gRPC backend services.
*
*
* Protobuf type {@code google.cloud.compute.v1.TargetGrpcProxy}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.TargetGrpcProxy)
com.google.cloud.compute.v1.TargetGrpcProxyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_TargetGrpcProxy_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_TargetGrpcProxy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.TargetGrpcProxy.class,
com.google.cloud.compute.v1.TargetGrpcProxy.Builder.class);
}
// Construct using com.google.cloud.compute.v1.TargetGrpcProxy.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
creationTimestamp_ = "";
description_ = "";
fingerprint_ = "";
id_ = 0L;
kind_ = "";
name_ = "";
selfLink_ = "";
selfLinkWithId_ = "";
urlMap_ = "";
validateForProxyless_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_TargetGrpcProxy_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.TargetGrpcProxy getDefaultInstanceForType() {
return com.google.cloud.compute.v1.TargetGrpcProxy.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.TargetGrpcProxy build() {
com.google.cloud.compute.v1.TargetGrpcProxy result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.TargetGrpcProxy buildPartial() {
com.google.cloud.compute.v1.TargetGrpcProxy result =
new com.google.cloud.compute.v1.TargetGrpcProxy(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.compute.v1.TargetGrpcProxy result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.creationTimestamp_ = creationTimestamp_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.description_ = description_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.fingerprint_ = fingerprint_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.id_ = id_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.kind_ = kind_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.name_ = name_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.selfLink_ = selfLink_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.selfLinkWithId_ = selfLinkWithId_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.urlMap_ = urlMap_;
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.validateForProxyless_ = validateForProxyless_;
to_bitField0_ |= 0x00000200;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.TargetGrpcProxy) {
return mergeFrom((com.google.cloud.compute.v1.TargetGrpcProxy) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.TargetGrpcProxy other) {
if (other == com.google.cloud.compute.v1.TargetGrpcProxy.getDefaultInstance()) return this;
if (other.hasCreationTimestamp()) {
creationTimestamp_ = other.creationTimestamp_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasDescription()) {
description_ = other.description_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasFingerprint()) {
fingerprint_ = other.fingerprint_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasId()) {
setId(other.getId());
}
if (other.hasKind()) {
kind_ = other.kind_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasName()) {
name_ = other.name_;
bitField0_ |= 0x00000020;
onChanged();
}
if (other.hasSelfLink()) {
selfLink_ = other.selfLink_;
bitField0_ |= 0x00000040;
onChanged();
}
if (other.hasSelfLinkWithId()) {
selfLinkWithId_ = other.selfLinkWithId_;
bitField0_ |= 0x00000080;
onChanged();
}
if (other.hasUrlMap()) {
urlMap_ = other.urlMap_;
bitField0_ |= 0x00000100;
onChanged();
}
if (other.hasValidateForProxyless()) {
setValidateForProxyless(other.getValidateForProxyless());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 26840:
{
id_ = input.readUInt64();
bitField0_ |= 0x00000008;
break;
} // case 26840
case 26336418:
{
kind_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 26336418
case 26989658:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 26989658
case 244202930:
{
creationTimestamp_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 244202930
case 356167698:
{
selfLinkWithId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 356167698
case 814583104:
{
validateForProxyless_ = input.readBool();
bitField0_ |= 0x00000200;
break;
} // case 814583104
case 1877428002:
{
fingerprint_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 1877428002
case -1358801822:
{
urlMap_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case -1358801822
case -911466526:
{
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case -911466526
case -645248918:
{
selfLink_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case -645248918
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object creationTimestamp_ = "";
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return Whether the creationTimestamp field is set.
*/
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The creationTimestamp.
*/
public java.lang.String getCreationTimestamp() {
java.lang.Object ref = creationTimestamp_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
creationTimestamp_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return The bytes for creationTimestamp.
*/
public com.google.protobuf.ByteString getCreationTimestampBytes() {
java.lang.Object ref = creationTimestamp_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
creationTimestamp_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestamp(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
creationTimestamp_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @return This builder for chaining.
*/
public Builder clearCreationTimestamp() {
creationTimestamp_ = getDefaultInstance().getCreationTimestamp();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Creation timestamp in RFC3339 text format.
*
*
* optional string creation_timestamp = 30525366;
*
* @param value The bytes for creationTimestamp to set.
* @return This builder for chaining.
*/
public Builder setCreationTimestampBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
creationTimestamp_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return Whether the description field is set.
*/
public boolean hasDescription() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return The bytes for description.
*/
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* An optional description of this resource. Provide this property when you create the resource.
*
*
* optional string description = 422937596;
*
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object fingerprint_ = "";
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. This field will be ignored when inserting a TargetGrpcProxy. An up-to-date fingerprint must be provided in order to patch/update the TargetGrpcProxy; otherwise, the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve the TargetGrpcProxy.
*
*
* optional string fingerprint = 234678500;
*
* @return Whether the fingerprint field is set.
*/
public boolean hasFingerprint() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. This field will be ignored when inserting a TargetGrpcProxy. An up-to-date fingerprint must be provided in order to patch/update the TargetGrpcProxy; otherwise, the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve the TargetGrpcProxy.
*
*
* optional string fingerprint = 234678500;
*
* @return The fingerprint.
*/
public java.lang.String getFingerprint() {
java.lang.Object ref = fingerprint_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fingerprint_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. This field will be ignored when inserting a TargetGrpcProxy. An up-to-date fingerprint must be provided in order to patch/update the TargetGrpcProxy; otherwise, the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve the TargetGrpcProxy.
*
*
* optional string fingerprint = 234678500;
*
* @return The bytes for fingerprint.
*/
public com.google.protobuf.ByteString getFingerprintBytes() {
java.lang.Object ref = fingerprint_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
fingerprint_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. This field will be ignored when inserting a TargetGrpcProxy. An up-to-date fingerprint must be provided in order to patch/update the TargetGrpcProxy; otherwise, the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve the TargetGrpcProxy.
*
*
* optional string fingerprint = 234678500;
*
* @param value The fingerprint to set.
* @return This builder for chaining.
*/
public Builder setFingerprint(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
fingerprint_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. This field will be ignored when inserting a TargetGrpcProxy. An up-to-date fingerprint must be provided in order to patch/update the TargetGrpcProxy; otherwise, the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve the TargetGrpcProxy.
*
*
* optional string fingerprint = 234678500;
*
* @return This builder for chaining.
*/
public Builder clearFingerprint() {
fingerprint_ = getDefaultInstance().getFingerprint();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* Fingerprint of this resource. A hash of the contents stored in this object. This field is used in optimistic locking. This field will be ignored when inserting a TargetGrpcProxy. An up-to-date fingerprint must be provided in order to patch/update the TargetGrpcProxy; otherwise, the request will fail with error 412 conditionNotMet. To see the latest fingerprint, make a get() request to retrieve the TargetGrpcProxy.
*
*
* optional string fingerprint = 234678500;
*
* @param value The bytes for fingerprint to set.
* @return This builder for chaining.
*/
public Builder setFingerprintBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fingerprint_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private long id_;
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @return Whether the id field is set.
*/
@java.lang.Override
public boolean hasId() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @return The id.
*/
@java.lang.Override
public long getId() {
return id_;
}
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(long value) {
id_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The unique identifier for the resource type. The server generates this identifier.
*
*
* optional uint64 id = 3355;
*
* @return This builder for chaining.
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000008);
id_ = 0L;
onChanged();
return this;
}
private java.lang.Object kind_ = "";
/**
*
*
*
* [Output Only] Type of the resource. Always compute#targetGrpcProxy for target grpc proxies.
*
*
* optional string kind = 3292052;
*
* @return Whether the kind field is set.
*/
public boolean hasKind() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#targetGrpcProxy for target grpc proxies.
*
*
* optional string kind = 3292052;
*
* @return The kind.
*/
public java.lang.String getKind() {
java.lang.Object ref = kind_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
kind_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#targetGrpcProxy for target grpc proxies.
*
*
* optional string kind = 3292052;
*
* @return The bytes for kind.
*/
public com.google.protobuf.ByteString getKindBytes() {
java.lang.Object ref = kind_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
kind_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#targetGrpcProxy for target grpc proxies.
*
*
* optional string kind = 3292052;
*
* @param value The kind to set.
* @return This builder for chaining.
*/
public Builder setKind(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#targetGrpcProxy for target grpc proxies.
*
*
* optional string kind = 3292052;
*
* @return This builder for chaining.
*/
public Builder clearKind() {
kind_ = getDefaultInstance().getKind();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Type of the resource. Always compute#targetGrpcProxy for target grpc proxies.
*
*
* optional string kind = 3292052;
*
* @param value The bytes for kind to set.
* @return This builder for chaining.
*/
public Builder setKindBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
kind_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
*
*
* Name of the resource. Provided by the client when the resource is created. The name must be 1-63 characters long, and comply with RFC1035. Specifically, the name must be 1-63 characters long and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])?` which means the first character must be a lowercase letter, and all following characters must be a dash, lowercase letter, or digit, except the last character, which cannot be a dash.
*
*
* optional string name = 3373707;
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.lang.Object selfLink_ = "";
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return Whether the selfLink field is set.
*/
public boolean hasSelfLink() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The selfLink.
*/
public java.lang.String getSelfLink() {
java.lang.Object ref = selfLink_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLink_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return The bytes for selfLink.
*/
public com.google.protobuf.ByteString getSelfLinkBytes() {
java.lang.Object ref = selfLink_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLink_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @param value The selfLink to set.
* @return This builder for chaining.
*/
public Builder setSelfLink(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
selfLink_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @return This builder for chaining.
*/
public Builder clearSelfLink() {
selfLink_ = getDefaultInstance().getSelfLink();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined URL for the resource.
*
*
* optional string self_link = 456214797;
*
* @param value The bytes for selfLink to set.
* @return This builder for chaining.
*/
public Builder setSelfLinkBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
selfLink_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object selfLinkWithId_ = "";
/**
*
*
*
* [Output Only] Server-defined URL with id for the resource.
*
*
* optional string self_link_with_id = 44520962;
*
* @return Whether the selfLinkWithId field is set.
*/
public boolean hasSelfLinkWithId() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* [Output Only] Server-defined URL with id for the resource.
*
*
* optional string self_link_with_id = 44520962;
*
* @return The selfLinkWithId.
*/
public java.lang.String getSelfLinkWithId() {
java.lang.Object ref = selfLinkWithId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
selfLinkWithId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined URL with id for the resource.
*
*
* optional string self_link_with_id = 44520962;
*
* @return The bytes for selfLinkWithId.
*/
public com.google.protobuf.ByteString getSelfLinkWithIdBytes() {
java.lang.Object ref = selfLinkWithId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
selfLinkWithId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] Server-defined URL with id for the resource.
*
*
* optional string self_link_with_id = 44520962;
*
* @param value The selfLinkWithId to set.
* @return This builder for chaining.
*/
public Builder setSelfLinkWithId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
selfLinkWithId_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined URL with id for the resource.
*
*
* optional string self_link_with_id = 44520962;
*
* @return This builder for chaining.
*/
public Builder clearSelfLinkWithId() {
selfLinkWithId_ = getDefaultInstance().getSelfLinkWithId();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] Server-defined URL with id for the resource.
*
*
* optional string self_link_with_id = 44520962;
*
* @param value The bytes for selfLinkWithId to set.
* @return This builder for chaining.
*/
public Builder setSelfLinkWithIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
selfLinkWithId_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private java.lang.Object urlMap_ = "";
/**
*
*
*
* URL to the UrlMap resource that defines the mapping from URL to the BackendService. The protocol field in the BackendService must be set to GRPC.
*
*
* optional string url_map = 367020684;
*
* @return Whether the urlMap field is set.
*/
public boolean hasUrlMap() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* URL to the UrlMap resource that defines the mapping from URL to the BackendService. The protocol field in the BackendService must be set to GRPC.
*
*
* optional string url_map = 367020684;
*
* @return The urlMap.
*/
public java.lang.String getUrlMap() {
java.lang.Object ref = urlMap_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
urlMap_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* URL to the UrlMap resource that defines the mapping from URL to the BackendService. The protocol field in the BackendService must be set to GRPC.
*
*
* optional string url_map = 367020684;
*
* @return The bytes for urlMap.
*/
public com.google.protobuf.ByteString getUrlMapBytes() {
java.lang.Object ref = urlMap_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
urlMap_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* URL to the UrlMap resource that defines the mapping from URL to the BackendService. The protocol field in the BackendService must be set to GRPC.
*
*
* optional string url_map = 367020684;
*
* @param value The urlMap to set.
* @return This builder for chaining.
*/
public Builder setUrlMap(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
urlMap_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* URL to the UrlMap resource that defines the mapping from URL to the BackendService. The protocol field in the BackendService must be set to GRPC.
*
*
* optional string url_map = 367020684;
*
* @return This builder for chaining.
*/
public Builder clearUrlMap() {
urlMap_ = getDefaultInstance().getUrlMap();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
*
*
*
* URL to the UrlMap resource that defines the mapping from URL to the BackendService. The protocol field in the BackendService must be set to GRPC.
*
*
* optional string url_map = 367020684;
*
* @param value The bytes for urlMap to set.
* @return This builder for chaining.
*/
public Builder setUrlMapBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
urlMap_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private boolean validateForProxyless_;
/**
*
*
*
* If true, indicates that the BackendServices referenced by the urlMap may be accessed by gRPC applications without using a sidecar proxy. This will enable configuration checks on urlMap and its referenced BackendServices to not allow unsupported features. A gRPC application must use "xds:///" scheme in the target URI of the service it is connecting to. If false, indicates that the BackendServices referenced by the urlMap will be accessed by gRPC applications via a sidecar proxy. In this case, a gRPC application must not use "xds:///" scheme in the target URI of the service it is connecting to
*
*
* optional bool validate_for_proxyless = 101822888;
*
* @return Whether the validateForProxyless field is set.
*/
@java.lang.Override
public boolean hasValidateForProxyless() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* If true, indicates that the BackendServices referenced by the urlMap may be accessed by gRPC applications without using a sidecar proxy. This will enable configuration checks on urlMap and its referenced BackendServices to not allow unsupported features. A gRPC application must use "xds:///" scheme in the target URI of the service it is connecting to. If false, indicates that the BackendServices referenced by the urlMap will be accessed by gRPC applications via a sidecar proxy. In this case, a gRPC application must not use "xds:///" scheme in the target URI of the service it is connecting to
*
*
* optional bool validate_for_proxyless = 101822888;
*
* @return The validateForProxyless.
*/
@java.lang.Override
public boolean getValidateForProxyless() {
return validateForProxyless_;
}
/**
*
*
*
* If true, indicates that the BackendServices referenced by the urlMap may be accessed by gRPC applications without using a sidecar proxy. This will enable configuration checks on urlMap and its referenced BackendServices to not allow unsupported features. A gRPC application must use "xds:///" scheme in the target URI of the service it is connecting to. If false, indicates that the BackendServices referenced by the urlMap will be accessed by gRPC applications via a sidecar proxy. In this case, a gRPC application must not use "xds:///" scheme in the target URI of the service it is connecting to
*
*
* optional bool validate_for_proxyless = 101822888;
*
* @param value The validateForProxyless to set.
* @return This builder for chaining.
*/
public Builder setValidateForProxyless(boolean value) {
validateForProxyless_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* If true, indicates that the BackendServices referenced by the urlMap may be accessed by gRPC applications without using a sidecar proxy. This will enable configuration checks on urlMap and its referenced BackendServices to not allow unsupported features. A gRPC application must use "xds:///" scheme in the target URI of the service it is connecting to. If false, indicates that the BackendServices referenced by the urlMap will be accessed by gRPC applications via a sidecar proxy. In this case, a gRPC application must not use "xds:///" scheme in the target URI of the service it is connecting to
*
*
* optional bool validate_for_proxyless = 101822888;
*
* @return This builder for chaining.
*/
public Builder clearValidateForProxyless() {
bitField0_ = (bitField0_ & ~0x00000200);
validateForProxyless_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.TargetGrpcProxy)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.TargetGrpcProxy)
private static final com.google.cloud.compute.v1.TargetGrpcProxy DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.TargetGrpcProxy();
}
public static com.google.cloud.compute.v1.TargetGrpcProxy getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TargetGrpcProxy parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.TargetGrpcProxy getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy