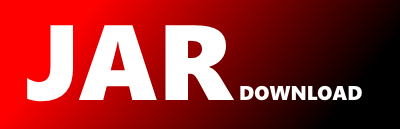
com.google.cloud.compute.v1.UsableSubnetwork Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-compute-v1 Show documentation
Show all versions of proto-google-cloud-compute-v1 Show documentation
Proto library for google-cloud-compute
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/compute/v1/compute.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.compute.v1;
/**
*
*
*
* Subnetwork which the current user has compute.subnetworks.use permission on.
*
*
* Protobuf type {@code google.cloud.compute.v1.UsableSubnetwork}
*/
public final class UsableSubnetwork extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.compute.v1.UsableSubnetwork)
UsableSubnetworkOrBuilder {
private static final long serialVersionUID = 0L;
// Use UsableSubnetwork.newBuilder() to construct.
private UsableSubnetwork(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UsableSubnetwork() {
externalIpv6Prefix_ = "";
internalIpv6Prefix_ = "";
ipCidrRange_ = "";
ipv6AccessType_ = "";
network_ = "";
purpose_ = "";
role_ = "";
secondaryIpRanges_ = java.util.Collections.emptyList();
stackType_ = "";
subnetwork_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new UsableSubnetwork();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_UsableSubnetwork_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_UsableSubnetwork_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.UsableSubnetwork.class,
com.google.cloud.compute.v1.UsableSubnetwork.Builder.class);
}
/**
*
*
*
* The access type of IPv6 address this subnet holds. It's immutable and can only be specified during creation or the first time the subnet is updated into IPV4_IPV6 dual stack.
*
*
* Protobuf enum {@code google.cloud.compute.v1.UsableSubnetwork.Ipv6AccessType}
*/
public enum Ipv6AccessType implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_IPV6_ACCESS_TYPE = 0;
*/
UNDEFINED_IPV6_ACCESS_TYPE(0),
/**
*
*
*
* VMs on this subnet will be assigned IPv6 addresses that are accessible via the Internet, as well as the VPC network.
*
*
* EXTERNAL = 35607499;
*/
EXTERNAL(35607499),
/**
*
*
*
* VMs on this subnet will be assigned IPv6 addresses that are only accessible over the VPC network.
*
*
* INTERNAL = 279295677;
*/
INTERNAL(279295677),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_IPV6_ACCESS_TYPE = 0;
*/
public static final int UNDEFINED_IPV6_ACCESS_TYPE_VALUE = 0;
/**
*
*
*
* VMs on this subnet will be assigned IPv6 addresses that are accessible via the Internet, as well as the VPC network.
*
*
* EXTERNAL = 35607499;
*/
public static final int EXTERNAL_VALUE = 35607499;
/**
*
*
*
* VMs on this subnet will be assigned IPv6 addresses that are only accessible over the VPC network.
*
*
* INTERNAL = 279295677;
*/
public static final int INTERNAL_VALUE = 279295677;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Ipv6AccessType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Ipv6AccessType forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_IPV6_ACCESS_TYPE;
case 35607499:
return EXTERNAL;
case 279295677:
return INTERNAL;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Ipv6AccessType findValueByNumber(int number) {
return Ipv6AccessType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.UsableSubnetwork.getDescriptor().getEnumTypes().get(0);
}
private static final Ipv6AccessType[] VALUES = values();
public static Ipv6AccessType valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Ipv6AccessType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.UsableSubnetwork.Ipv6AccessType)
}
/**
*
*
*
* The purpose of the resource. This field can be either PRIVATE, GLOBAL_MANAGED_PROXY, REGIONAL_MANAGED_PROXY, PEER_MIGRATION or PRIVATE_SERVICE_CONNECT. PRIVATE is the default purpose for user-created subnets or subnets that are automatically created in auto mode networks. Subnets with purpose set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY are user-created subnetworks that are reserved for Envoy-based load balancers. A subnet with purpose set to PRIVATE_SERVICE_CONNECT is used to publish services using Private Service Connect. A subnet with purpose set to PEER_MIGRATION is used for subnet migration from one peered VPC to another. If unspecified, the subnet purpose defaults to PRIVATE. The enableFlowLogs field isn't supported if the subnet purpose field is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY.
*
*
* Protobuf enum {@code google.cloud.compute.v1.UsableSubnetwork.Purpose}
*/
public enum Purpose implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_PURPOSE = 0;
*/
UNDEFINED_PURPOSE(0),
/**
*
*
*
* Subnet reserved for Global Envoy-based Load Balancing.
*
*
* GLOBAL_MANAGED_PROXY = 236463602;
*/
GLOBAL_MANAGED_PROXY(236463602),
/**
*
*
*
* Subnet reserved for Internal HTTP(S) Load Balancing. This is a legacy purpose, please use REGIONAL_MANAGED_PROXY instead.
*
*
* INTERNAL_HTTPS_LOAD_BALANCER = 248748889;
*/
INTERNAL_HTTPS_LOAD_BALANCER(248748889),
/**
*
*
*
* Subnetwork will be used for Migration from one peered VPC to another. (a transient state of subnetwork while migrating resources from one project to another).
*
*
* PEER_MIGRATION = 491902225;
*/
PEER_MIGRATION(491902225),
/**
*
*
*
* Regular user created or automatically created subnet.
*
*
* PRIVATE = 403485027;
*/
PRIVATE(403485027),
/**
*
*
*
* Subnetwork used as source range for Private NAT Gateways.
*
*
* PRIVATE_NAT = 367764517;
*/
PRIVATE_NAT(367764517),
/**
*
*
*
* Regular user created or automatically created subnet.
*
*
* PRIVATE_RFC_1918 = 254902107;
*/
PRIVATE_RFC_1918(254902107),
/**
*
*
*
* Subnetworks created for Private Service Connect in the producer network.
*
*
* PRIVATE_SERVICE_CONNECT = 48134724;
*/
PRIVATE_SERVICE_CONNECT(48134724),
/**
*
*
*
* Subnetwork used for Regional Envoy-based Load Balancing.
*
*
* REGIONAL_MANAGED_PROXY = 153049966;
*/
REGIONAL_MANAGED_PROXY(153049966),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_PURPOSE = 0;
*/
public static final int UNDEFINED_PURPOSE_VALUE = 0;
/**
*
*
*
* Subnet reserved for Global Envoy-based Load Balancing.
*
*
* GLOBAL_MANAGED_PROXY = 236463602;
*/
public static final int GLOBAL_MANAGED_PROXY_VALUE = 236463602;
/**
*
*
*
* Subnet reserved for Internal HTTP(S) Load Balancing. This is a legacy purpose, please use REGIONAL_MANAGED_PROXY instead.
*
*
* INTERNAL_HTTPS_LOAD_BALANCER = 248748889;
*/
public static final int INTERNAL_HTTPS_LOAD_BALANCER_VALUE = 248748889;
/**
*
*
*
* Subnetwork will be used for Migration from one peered VPC to another. (a transient state of subnetwork while migrating resources from one project to another).
*
*
* PEER_MIGRATION = 491902225;
*/
public static final int PEER_MIGRATION_VALUE = 491902225;
/**
*
*
*
* Regular user created or automatically created subnet.
*
*
* PRIVATE = 403485027;
*/
public static final int PRIVATE_VALUE = 403485027;
/**
*
*
*
* Subnetwork used as source range for Private NAT Gateways.
*
*
* PRIVATE_NAT = 367764517;
*/
public static final int PRIVATE_NAT_VALUE = 367764517;
/**
*
*
*
* Regular user created or automatically created subnet.
*
*
* PRIVATE_RFC_1918 = 254902107;
*/
public static final int PRIVATE_RFC_1918_VALUE = 254902107;
/**
*
*
*
* Subnetworks created for Private Service Connect in the producer network.
*
*
* PRIVATE_SERVICE_CONNECT = 48134724;
*/
public static final int PRIVATE_SERVICE_CONNECT_VALUE = 48134724;
/**
*
*
*
* Subnetwork used for Regional Envoy-based Load Balancing.
*
*
* REGIONAL_MANAGED_PROXY = 153049966;
*/
public static final int REGIONAL_MANAGED_PROXY_VALUE = 153049966;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Purpose valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Purpose forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_PURPOSE;
case 236463602:
return GLOBAL_MANAGED_PROXY;
case 248748889:
return INTERNAL_HTTPS_LOAD_BALANCER;
case 491902225:
return PEER_MIGRATION;
case 403485027:
return PRIVATE;
case 367764517:
return PRIVATE_NAT;
case 254902107:
return PRIVATE_RFC_1918;
case 48134724:
return PRIVATE_SERVICE_CONNECT;
case 153049966:
return REGIONAL_MANAGED_PROXY;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Purpose findValueByNumber(int number) {
return Purpose.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.UsableSubnetwork.getDescriptor().getEnumTypes().get(1);
}
private static final Purpose[] VALUES = values();
public static Purpose valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Purpose(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.UsableSubnetwork.Purpose)
}
/**
*
*
*
* The role of subnetwork. Currently, this field is only used when purpose is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY. The value can be set to ACTIVE or BACKUP. An ACTIVE subnetwork is one that is currently being used for Envoy-based load balancers in a region. A BACKUP subnetwork is one that is ready to be promoted to ACTIVE or is currently draining. This field can be updated with a patch request.
*
*
* Protobuf enum {@code google.cloud.compute.v1.UsableSubnetwork.Role}
*/
public enum Role implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_ROLE = 0;
*/
UNDEFINED_ROLE(0),
/**
*
*
*
* The ACTIVE subnet that is currently used.
*
*
* ACTIVE = 314733318;
*/
ACTIVE(314733318),
/**
*
*
*
* The BACKUP subnet that could be promoted to ACTIVE.
*
*
* BACKUP = 341010882;
*/
BACKUP(341010882),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_ROLE = 0;
*/
public static final int UNDEFINED_ROLE_VALUE = 0;
/**
*
*
*
* The ACTIVE subnet that is currently used.
*
*
* ACTIVE = 314733318;
*/
public static final int ACTIVE_VALUE = 314733318;
/**
*
*
*
* The BACKUP subnet that could be promoted to ACTIVE.
*
*
* BACKUP = 341010882;
*/
public static final int BACKUP_VALUE = 341010882;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Role valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Role forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_ROLE;
case 314733318:
return ACTIVE;
case 341010882:
return BACKUP;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Role findValueByNumber(int number) {
return Role.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.UsableSubnetwork.getDescriptor().getEnumTypes().get(2);
}
private static final Role[] VALUES = values();
public static Role valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Role(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.UsableSubnetwork.Role)
}
/**
*
*
*
* The stack type for the subnet. If set to IPV4_ONLY, new VMs in the subnet are assigned IPv4 addresses only. If set to IPV4_IPV6, new VMs in the subnet can be assigned both IPv4 and IPv6 addresses. If not specified, IPV4_ONLY is used. This field can be both set at resource creation time and updated using patch.
*
*
* Protobuf enum {@code google.cloud.compute.v1.UsableSubnetwork.StackType}
*/
public enum StackType implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STACK_TYPE = 0;
*/
UNDEFINED_STACK_TYPE(0),
/**
*
*
*
* New VMs in this subnet can have both IPv4 and IPv6 addresses.
*
*
* IPV4_IPV6 = 22197249;
*/
IPV4_IPV6(22197249),
/**
*
*
*
* New VMs in this subnet will only be assigned IPv4 addresses.
*
*
* IPV4_ONLY = 22373798;
*/
IPV4_ONLY(22373798),
/**
*
*
*
* New VMs in this subnet will only be assigned IPv6 addresses.
*
*
* IPV6_ONLY = 79632100;
*/
IPV6_ONLY(79632100),
UNRECOGNIZED(-1),
;
/**
*
*
*
* A value indicating that the enum field is not set.
*
*
* UNDEFINED_STACK_TYPE = 0;
*/
public static final int UNDEFINED_STACK_TYPE_VALUE = 0;
/**
*
*
*
* New VMs in this subnet can have both IPv4 and IPv6 addresses.
*
*
* IPV4_IPV6 = 22197249;
*/
public static final int IPV4_IPV6_VALUE = 22197249;
/**
*
*
*
* New VMs in this subnet will only be assigned IPv4 addresses.
*
*
* IPV4_ONLY = 22373798;
*/
public static final int IPV4_ONLY_VALUE = 22373798;
/**
*
*
*
* New VMs in this subnet will only be assigned IPv6 addresses.
*
*
* IPV6_ONLY = 79632100;
*/
public static final int IPV6_ONLY_VALUE = 79632100;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static StackType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static StackType forNumber(int value) {
switch (value) {
case 0:
return UNDEFINED_STACK_TYPE;
case 22197249:
return IPV4_IPV6;
case 22373798:
return IPV4_ONLY;
case 79632100:
return IPV6_ONLY;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public StackType findValueByNumber(int number) {
return StackType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.compute.v1.UsableSubnetwork.getDescriptor().getEnumTypes().get(3);
}
private static final StackType[] VALUES = values();
public static StackType valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private StackType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.compute.v1.UsableSubnetwork.StackType)
}
private int bitField0_;
public static final int EXTERNAL_IPV6_PREFIX_FIELD_NUMBER = 139299190;
@SuppressWarnings("serial")
private volatile java.lang.Object externalIpv6Prefix_ = "";
/**
*
*
*
* [Output Only] The external IPv6 address range that is assigned to this subnetwork.
*
*
* optional string external_ipv6_prefix = 139299190;
*
* @return Whether the externalIpv6Prefix field is set.
*/
@java.lang.Override
public boolean hasExternalIpv6Prefix() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* [Output Only] The external IPv6 address range that is assigned to this subnetwork.
*
*
* optional string external_ipv6_prefix = 139299190;
*
* @return The externalIpv6Prefix.
*/
@java.lang.Override
public java.lang.String getExternalIpv6Prefix() {
java.lang.Object ref = externalIpv6Prefix_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
externalIpv6Prefix_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The external IPv6 address range that is assigned to this subnetwork.
*
*
* optional string external_ipv6_prefix = 139299190;
*
* @return The bytes for externalIpv6Prefix.
*/
@java.lang.Override
public com.google.protobuf.ByteString getExternalIpv6PrefixBytes() {
java.lang.Object ref = externalIpv6Prefix_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
externalIpv6Prefix_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INTERNAL_IPV6_PREFIX_FIELD_NUMBER = 506270056;
@SuppressWarnings("serial")
private volatile java.lang.Object internalIpv6Prefix_ = "";
/**
*
*
*
* [Output Only] The internal IPv6 address range that is assigned to this subnetwork.
*
*
* optional string internal_ipv6_prefix = 506270056;
*
* @return Whether the internalIpv6Prefix field is set.
*/
@java.lang.Override
public boolean hasInternalIpv6Prefix() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* [Output Only] The internal IPv6 address range that is assigned to this subnetwork.
*
*
* optional string internal_ipv6_prefix = 506270056;
*
* @return The internalIpv6Prefix.
*/
@java.lang.Override
public java.lang.String getInternalIpv6Prefix() {
java.lang.Object ref = internalIpv6Prefix_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
internalIpv6Prefix_ = s;
return s;
}
}
/**
*
*
*
* [Output Only] The internal IPv6 address range that is assigned to this subnetwork.
*
*
* optional string internal_ipv6_prefix = 506270056;
*
* @return The bytes for internalIpv6Prefix.
*/
@java.lang.Override
public com.google.protobuf.ByteString getInternalIpv6PrefixBytes() {
java.lang.Object ref = internalIpv6Prefix_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
internalIpv6Prefix_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IP_CIDR_RANGE_FIELD_NUMBER = 98117322;
@SuppressWarnings("serial")
private volatile java.lang.Object ipCidrRange_ = "";
/**
*
*
*
* The range of internal addresses that are owned by this subnetwork.
*
*
* optional string ip_cidr_range = 98117322;
*
* @return Whether the ipCidrRange field is set.
*/
@java.lang.Override
public boolean hasIpCidrRange() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* The range of internal addresses that are owned by this subnetwork.
*
*
* optional string ip_cidr_range = 98117322;
*
* @return The ipCidrRange.
*/
@java.lang.Override
public java.lang.String getIpCidrRange() {
java.lang.Object ref = ipCidrRange_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ipCidrRange_ = s;
return s;
}
}
/**
*
*
*
* The range of internal addresses that are owned by this subnetwork.
*
*
* optional string ip_cidr_range = 98117322;
*
* @return The bytes for ipCidrRange.
*/
@java.lang.Override
public com.google.protobuf.ByteString getIpCidrRangeBytes() {
java.lang.Object ref = ipCidrRange_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
ipCidrRange_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int IPV6_ACCESS_TYPE_FIELD_NUMBER = 504658653;
@SuppressWarnings("serial")
private volatile java.lang.Object ipv6AccessType_ = "";
/**
*
*
*
* The access type of IPv6 address this subnet holds. It's immutable and can only be specified during creation or the first time the subnet is updated into IPV4_IPV6 dual stack.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return Whether the ipv6AccessType field is set.
*/
@java.lang.Override
public boolean hasIpv6AccessType() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The access type of IPv6 address this subnet holds. It's immutable and can only be specified during creation or the first time the subnet is updated into IPV4_IPV6 dual stack.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return The ipv6AccessType.
*/
@java.lang.Override
public java.lang.String getIpv6AccessType() {
java.lang.Object ref = ipv6AccessType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ipv6AccessType_ = s;
return s;
}
}
/**
*
*
*
* The access type of IPv6 address this subnet holds. It's immutable and can only be specified during creation or the first time the subnet is updated into IPV4_IPV6 dual stack.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return The bytes for ipv6AccessType.
*/
@java.lang.Override
public com.google.protobuf.ByteString getIpv6AccessTypeBytes() {
java.lang.Object ref = ipv6AccessType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
ipv6AccessType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NETWORK_FIELD_NUMBER = 232872494;
@SuppressWarnings("serial")
private volatile java.lang.Object network_ = "";
/**
*
*
*
* Network URL.
*
*
* optional string network = 232872494;
*
* @return Whether the network field is set.
*/
@java.lang.Override
public boolean hasNetwork() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Network URL.
*
*
* optional string network = 232872494;
*
* @return The network.
*/
@java.lang.Override
public java.lang.String getNetwork() {
java.lang.Object ref = network_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
network_ = s;
return s;
}
}
/**
*
*
*
* Network URL.
*
*
* optional string network = 232872494;
*
* @return The bytes for network.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNetworkBytes() {
java.lang.Object ref = network_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
network_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PURPOSE_FIELD_NUMBER = 316407070;
@SuppressWarnings("serial")
private volatile java.lang.Object purpose_ = "";
/**
*
*
*
* The purpose of the resource. This field can be either PRIVATE, GLOBAL_MANAGED_PROXY, REGIONAL_MANAGED_PROXY, PEER_MIGRATION or PRIVATE_SERVICE_CONNECT. PRIVATE is the default purpose for user-created subnets or subnets that are automatically created in auto mode networks. Subnets with purpose set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY are user-created subnetworks that are reserved for Envoy-based load balancers. A subnet with purpose set to PRIVATE_SERVICE_CONNECT is used to publish services using Private Service Connect. A subnet with purpose set to PEER_MIGRATION is used for subnet migration from one peered VPC to another. If unspecified, the subnet purpose defaults to PRIVATE. The enableFlowLogs field isn't supported if the subnet purpose field is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY.
* Check the Purpose enum for the list of possible values.
*
*
* optional string purpose = 316407070;
*
* @return Whether the purpose field is set.
*/
@java.lang.Override
public boolean hasPurpose() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* The purpose of the resource. This field can be either PRIVATE, GLOBAL_MANAGED_PROXY, REGIONAL_MANAGED_PROXY, PEER_MIGRATION or PRIVATE_SERVICE_CONNECT. PRIVATE is the default purpose for user-created subnets or subnets that are automatically created in auto mode networks. Subnets with purpose set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY are user-created subnetworks that are reserved for Envoy-based load balancers. A subnet with purpose set to PRIVATE_SERVICE_CONNECT is used to publish services using Private Service Connect. A subnet with purpose set to PEER_MIGRATION is used for subnet migration from one peered VPC to another. If unspecified, the subnet purpose defaults to PRIVATE. The enableFlowLogs field isn't supported if the subnet purpose field is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY.
* Check the Purpose enum for the list of possible values.
*
*
* optional string purpose = 316407070;
*
* @return The purpose.
*/
@java.lang.Override
public java.lang.String getPurpose() {
java.lang.Object ref = purpose_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
purpose_ = s;
return s;
}
}
/**
*
*
*
* The purpose of the resource. This field can be either PRIVATE, GLOBAL_MANAGED_PROXY, REGIONAL_MANAGED_PROXY, PEER_MIGRATION or PRIVATE_SERVICE_CONNECT. PRIVATE is the default purpose for user-created subnets or subnets that are automatically created in auto mode networks. Subnets with purpose set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY are user-created subnetworks that are reserved for Envoy-based load balancers. A subnet with purpose set to PRIVATE_SERVICE_CONNECT is used to publish services using Private Service Connect. A subnet with purpose set to PEER_MIGRATION is used for subnet migration from one peered VPC to another. If unspecified, the subnet purpose defaults to PRIVATE. The enableFlowLogs field isn't supported if the subnet purpose field is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY.
* Check the Purpose enum for the list of possible values.
*
*
* optional string purpose = 316407070;
*
* @return The bytes for purpose.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPurposeBytes() {
java.lang.Object ref = purpose_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
purpose_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ROLE_FIELD_NUMBER = 3506294;
@SuppressWarnings("serial")
private volatile java.lang.Object role_ = "";
/**
*
*
*
* The role of subnetwork. Currently, this field is only used when purpose is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY. The value can be set to ACTIVE or BACKUP. An ACTIVE subnetwork is one that is currently being used for Envoy-based load balancers in a region. A BACKUP subnetwork is one that is ready to be promoted to ACTIVE or is currently draining. This field can be updated with a patch request.
* Check the Role enum for the list of possible values.
*
*
* optional string role = 3506294;
*
* @return Whether the role field is set.
*/
@java.lang.Override
public boolean hasRole() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* The role of subnetwork. Currently, this field is only used when purpose is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY. The value can be set to ACTIVE or BACKUP. An ACTIVE subnetwork is one that is currently being used for Envoy-based load balancers in a region. A BACKUP subnetwork is one that is ready to be promoted to ACTIVE or is currently draining. This field can be updated with a patch request.
* Check the Role enum for the list of possible values.
*
*
* optional string role = 3506294;
*
* @return The role.
*/
@java.lang.Override
public java.lang.String getRole() {
java.lang.Object ref = role_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
role_ = s;
return s;
}
}
/**
*
*
*
* The role of subnetwork. Currently, this field is only used when purpose is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY. The value can be set to ACTIVE or BACKUP. An ACTIVE subnetwork is one that is currently being used for Envoy-based load balancers in a region. A BACKUP subnetwork is one that is ready to be promoted to ACTIVE or is currently draining. This field can be updated with a patch request.
* Check the Role enum for the list of possible values.
*
*
* optional string role = 3506294;
*
* @return The bytes for role.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRoleBytes() {
java.lang.Object ref = role_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
role_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SECONDARY_IP_RANGES_FIELD_NUMBER = 136658915;
@SuppressWarnings("serial")
private java.util.List
secondaryIpRanges_;
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
@java.lang.Override
public java.util.List
getSecondaryIpRangesList() {
return secondaryIpRanges_;
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
@java.lang.Override
public java.util.List<
? extends com.google.cloud.compute.v1.UsableSubnetworkSecondaryRangeOrBuilder>
getSecondaryIpRangesOrBuilderList() {
return secondaryIpRanges_;
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
@java.lang.Override
public int getSecondaryIpRangesCount() {
return secondaryIpRanges_.size();
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange getSecondaryIpRanges(
int index) {
return secondaryIpRanges_.get(index);
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
@java.lang.Override
public com.google.cloud.compute.v1.UsableSubnetworkSecondaryRangeOrBuilder
getSecondaryIpRangesOrBuilder(int index) {
return secondaryIpRanges_.get(index);
}
public static final int STACK_TYPE_FIELD_NUMBER = 425908881;
@SuppressWarnings("serial")
private volatile java.lang.Object stackType_ = "";
/**
*
*
*
* The stack type for the subnet. If set to IPV4_ONLY, new VMs in the subnet are assigned IPv4 addresses only. If set to IPV4_IPV6, new VMs in the subnet can be assigned both IPv4 and IPv6 addresses. If not specified, IPV4_ONLY is used. This field can be both set at resource creation time and updated using patch.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return Whether the stackType field is set.
*/
@java.lang.Override
public boolean hasStackType() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The stack type for the subnet. If set to IPV4_ONLY, new VMs in the subnet are assigned IPv4 addresses only. If set to IPV4_IPV6, new VMs in the subnet can be assigned both IPv4 and IPv6 addresses. If not specified, IPV4_ONLY is used. This field can be both set at resource creation time and updated using patch.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The stackType.
*/
@java.lang.Override
public java.lang.String getStackType() {
java.lang.Object ref = stackType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stackType_ = s;
return s;
}
}
/**
*
*
*
* The stack type for the subnet. If set to IPV4_ONLY, new VMs in the subnet are assigned IPv4 addresses only. If set to IPV4_IPV6, new VMs in the subnet can be assigned both IPv4 and IPv6 addresses. If not specified, IPV4_ONLY is used. This field can be both set at resource creation time and updated using patch.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The bytes for stackType.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStackTypeBytes() {
java.lang.Object ref = stackType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
stackType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBNETWORK_FIELD_NUMBER = 307827694;
@SuppressWarnings("serial")
private volatile java.lang.Object subnetwork_ = "";
/**
*
*
*
* Subnetwork URL.
*
*
* optional string subnetwork = 307827694;
*
* @return Whether the subnetwork field is set.
*/
@java.lang.Override
public boolean hasSubnetwork() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Subnetwork URL.
*
*
* optional string subnetwork = 307827694;
*
* @return The subnetwork.
*/
@java.lang.Override
public java.lang.String getSubnetwork() {
java.lang.Object ref = subnetwork_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subnetwork_ = s;
return s;
}
}
/**
*
*
*
* Subnetwork URL.
*
*
* optional string subnetwork = 307827694;
*
* @return The bytes for subnetwork.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSubnetworkBytes() {
java.lang.Object ref = subnetwork_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
subnetwork_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000040) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3506294, role_);
}
if (((bitField0_ & 0x00000004) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 98117322, ipCidrRange_);
}
for (int i = 0; i < secondaryIpRanges_.size(); i++) {
output.writeMessage(136658915, secondaryIpRanges_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 139299190, externalIpv6Prefix_);
}
if (((bitField0_ & 0x00000010) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 232872494, network_);
}
if (((bitField0_ & 0x00000100) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 307827694, subnetwork_);
}
if (((bitField0_ & 0x00000020) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 316407070, purpose_);
}
if (((bitField0_ & 0x00000080) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 425908881, stackType_);
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 504658653, ipv6AccessType_);
}
if (((bitField0_ & 0x00000002) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 506270056, internalIpv6Prefix_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3506294, role_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(98117322, ipCidrRange_);
}
for (int i = 0; i < secondaryIpRanges_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
136658915, secondaryIpRanges_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(139299190, externalIpv6Prefix_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(232872494, network_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(307827694, subnetwork_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(316407070, purpose_);
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(425908881, stackType_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(504658653, ipv6AccessType_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size +=
com.google.protobuf.GeneratedMessageV3.computeStringSize(506270056, internalIpv6Prefix_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.compute.v1.UsableSubnetwork)) {
return super.equals(obj);
}
com.google.cloud.compute.v1.UsableSubnetwork other =
(com.google.cloud.compute.v1.UsableSubnetwork) obj;
if (hasExternalIpv6Prefix() != other.hasExternalIpv6Prefix()) return false;
if (hasExternalIpv6Prefix()) {
if (!getExternalIpv6Prefix().equals(other.getExternalIpv6Prefix())) return false;
}
if (hasInternalIpv6Prefix() != other.hasInternalIpv6Prefix()) return false;
if (hasInternalIpv6Prefix()) {
if (!getInternalIpv6Prefix().equals(other.getInternalIpv6Prefix())) return false;
}
if (hasIpCidrRange() != other.hasIpCidrRange()) return false;
if (hasIpCidrRange()) {
if (!getIpCidrRange().equals(other.getIpCidrRange())) return false;
}
if (hasIpv6AccessType() != other.hasIpv6AccessType()) return false;
if (hasIpv6AccessType()) {
if (!getIpv6AccessType().equals(other.getIpv6AccessType())) return false;
}
if (hasNetwork() != other.hasNetwork()) return false;
if (hasNetwork()) {
if (!getNetwork().equals(other.getNetwork())) return false;
}
if (hasPurpose() != other.hasPurpose()) return false;
if (hasPurpose()) {
if (!getPurpose().equals(other.getPurpose())) return false;
}
if (hasRole() != other.hasRole()) return false;
if (hasRole()) {
if (!getRole().equals(other.getRole())) return false;
}
if (!getSecondaryIpRangesList().equals(other.getSecondaryIpRangesList())) return false;
if (hasStackType() != other.hasStackType()) return false;
if (hasStackType()) {
if (!getStackType().equals(other.getStackType())) return false;
}
if (hasSubnetwork() != other.hasSubnetwork()) return false;
if (hasSubnetwork()) {
if (!getSubnetwork().equals(other.getSubnetwork())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasExternalIpv6Prefix()) {
hash = (37 * hash) + EXTERNAL_IPV6_PREFIX_FIELD_NUMBER;
hash = (53 * hash) + getExternalIpv6Prefix().hashCode();
}
if (hasInternalIpv6Prefix()) {
hash = (37 * hash) + INTERNAL_IPV6_PREFIX_FIELD_NUMBER;
hash = (53 * hash) + getInternalIpv6Prefix().hashCode();
}
if (hasIpCidrRange()) {
hash = (37 * hash) + IP_CIDR_RANGE_FIELD_NUMBER;
hash = (53 * hash) + getIpCidrRange().hashCode();
}
if (hasIpv6AccessType()) {
hash = (37 * hash) + IPV6_ACCESS_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getIpv6AccessType().hashCode();
}
if (hasNetwork()) {
hash = (37 * hash) + NETWORK_FIELD_NUMBER;
hash = (53 * hash) + getNetwork().hashCode();
}
if (hasPurpose()) {
hash = (37 * hash) + PURPOSE_FIELD_NUMBER;
hash = (53 * hash) + getPurpose().hashCode();
}
if (hasRole()) {
hash = (37 * hash) + ROLE_FIELD_NUMBER;
hash = (53 * hash) + getRole().hashCode();
}
if (getSecondaryIpRangesCount() > 0) {
hash = (37 * hash) + SECONDARY_IP_RANGES_FIELD_NUMBER;
hash = (53 * hash) + getSecondaryIpRangesList().hashCode();
}
if (hasStackType()) {
hash = (37 * hash) + STACK_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getStackType().hashCode();
}
if (hasSubnetwork()) {
hash = (37 * hash) + SUBNETWORK_FIELD_NUMBER;
hash = (53 * hash) + getSubnetwork().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.compute.v1.UsableSubnetwork parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.compute.v1.UsableSubnetwork prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Subnetwork which the current user has compute.subnetworks.use permission on.
*
*
* Protobuf type {@code google.cloud.compute.v1.UsableSubnetwork}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.compute.v1.UsableSubnetwork)
com.google.cloud.compute.v1.UsableSubnetworkOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_UsableSubnetwork_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_UsableSubnetwork_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.compute.v1.UsableSubnetwork.class,
com.google.cloud.compute.v1.UsableSubnetwork.Builder.class);
}
// Construct using com.google.cloud.compute.v1.UsableSubnetwork.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
externalIpv6Prefix_ = "";
internalIpv6Prefix_ = "";
ipCidrRange_ = "";
ipv6AccessType_ = "";
network_ = "";
purpose_ = "";
role_ = "";
if (secondaryIpRangesBuilder_ == null) {
secondaryIpRanges_ = java.util.Collections.emptyList();
} else {
secondaryIpRanges_ = null;
secondaryIpRangesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
stackType_ = "";
subnetwork_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.compute.v1.Compute
.internal_static_google_cloud_compute_v1_UsableSubnetwork_descriptor;
}
@java.lang.Override
public com.google.cloud.compute.v1.UsableSubnetwork getDefaultInstanceForType() {
return com.google.cloud.compute.v1.UsableSubnetwork.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.compute.v1.UsableSubnetwork build() {
com.google.cloud.compute.v1.UsableSubnetwork result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.compute.v1.UsableSubnetwork buildPartial() {
com.google.cloud.compute.v1.UsableSubnetwork result =
new com.google.cloud.compute.v1.UsableSubnetwork(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.cloud.compute.v1.UsableSubnetwork result) {
if (secondaryIpRangesBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
secondaryIpRanges_ = java.util.Collections.unmodifiableList(secondaryIpRanges_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.secondaryIpRanges_ = secondaryIpRanges_;
} else {
result.secondaryIpRanges_ = secondaryIpRangesBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.compute.v1.UsableSubnetwork result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.externalIpv6Prefix_ = externalIpv6Prefix_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.internalIpv6Prefix_ = internalIpv6Prefix_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.ipCidrRange_ = ipCidrRange_;
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.ipv6AccessType_ = ipv6AccessType_;
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.network_ = network_;
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.purpose_ = purpose_;
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.role_ = role_;
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.stackType_ = stackType_;
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.subnetwork_ = subnetwork_;
to_bitField0_ |= 0x00000100;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.compute.v1.UsableSubnetwork) {
return mergeFrom((com.google.cloud.compute.v1.UsableSubnetwork) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.compute.v1.UsableSubnetwork other) {
if (other == com.google.cloud.compute.v1.UsableSubnetwork.getDefaultInstance()) return this;
if (other.hasExternalIpv6Prefix()) {
externalIpv6Prefix_ = other.externalIpv6Prefix_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.hasInternalIpv6Prefix()) {
internalIpv6Prefix_ = other.internalIpv6Prefix_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasIpCidrRange()) {
ipCidrRange_ = other.ipCidrRange_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasIpv6AccessType()) {
ipv6AccessType_ = other.ipv6AccessType_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.hasNetwork()) {
network_ = other.network_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasPurpose()) {
purpose_ = other.purpose_;
bitField0_ |= 0x00000020;
onChanged();
}
if (other.hasRole()) {
role_ = other.role_;
bitField0_ |= 0x00000040;
onChanged();
}
if (secondaryIpRangesBuilder_ == null) {
if (!other.secondaryIpRanges_.isEmpty()) {
if (secondaryIpRanges_.isEmpty()) {
secondaryIpRanges_ = other.secondaryIpRanges_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureSecondaryIpRangesIsMutable();
secondaryIpRanges_.addAll(other.secondaryIpRanges_);
}
onChanged();
}
} else {
if (!other.secondaryIpRanges_.isEmpty()) {
if (secondaryIpRangesBuilder_.isEmpty()) {
secondaryIpRangesBuilder_.dispose();
secondaryIpRangesBuilder_ = null;
secondaryIpRanges_ = other.secondaryIpRanges_;
bitField0_ = (bitField0_ & ~0x00000080);
secondaryIpRangesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getSecondaryIpRangesFieldBuilder()
: null;
} else {
secondaryIpRangesBuilder_.addAllMessages(other.secondaryIpRanges_);
}
}
}
if (other.hasStackType()) {
stackType_ = other.stackType_;
bitField0_ |= 0x00000100;
onChanged();
}
if (other.hasSubnetwork()) {
subnetwork_ = other.subnetwork_;
bitField0_ |= 0x00000200;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 28050354:
{
role_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 28050354
case 784938578:
{
ipCidrRange_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 784938578
case 1093271322:
{
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange m =
input.readMessage(
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.parser(),
extensionRegistry);
if (secondaryIpRangesBuilder_ == null) {
ensureSecondaryIpRangesIsMutable();
secondaryIpRanges_.add(m);
} else {
secondaryIpRangesBuilder_.addMessage(m);
}
break;
} // case 1093271322
case 1114393522:
{
externalIpv6Prefix_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 1114393522
case 1862979954:
{
network_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 1862979954
case -1832345742:
{
subnetwork_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case -1832345742
case -1763710734:
{
purpose_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case -1763710734
case -887696246:
{
stackType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case -887696246
case -257698070:
{
ipv6AccessType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case -257698070
case -244806846:
{
internalIpv6Prefix_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case -244806846
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object externalIpv6Prefix_ = "";
/**
*
*
*
* [Output Only] The external IPv6 address range that is assigned to this subnetwork.
*
*
* optional string external_ipv6_prefix = 139299190;
*
* @return Whether the externalIpv6Prefix field is set.
*/
public boolean hasExternalIpv6Prefix() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* [Output Only] The external IPv6 address range that is assigned to this subnetwork.
*
*
* optional string external_ipv6_prefix = 139299190;
*
* @return The externalIpv6Prefix.
*/
public java.lang.String getExternalIpv6Prefix() {
java.lang.Object ref = externalIpv6Prefix_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
externalIpv6Prefix_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The external IPv6 address range that is assigned to this subnetwork.
*
*
* optional string external_ipv6_prefix = 139299190;
*
* @return The bytes for externalIpv6Prefix.
*/
public com.google.protobuf.ByteString getExternalIpv6PrefixBytes() {
java.lang.Object ref = externalIpv6Prefix_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
externalIpv6Prefix_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The external IPv6 address range that is assigned to this subnetwork.
*
*
* optional string external_ipv6_prefix = 139299190;
*
* @param value The externalIpv6Prefix to set.
* @return This builder for chaining.
*/
public Builder setExternalIpv6Prefix(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
externalIpv6Prefix_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The external IPv6 address range that is assigned to this subnetwork.
*
*
* optional string external_ipv6_prefix = 139299190;
*
* @return This builder for chaining.
*/
public Builder clearExternalIpv6Prefix() {
externalIpv6Prefix_ = getDefaultInstance().getExternalIpv6Prefix();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The external IPv6 address range that is assigned to this subnetwork.
*
*
* optional string external_ipv6_prefix = 139299190;
*
* @param value The bytes for externalIpv6Prefix to set.
* @return This builder for chaining.
*/
public Builder setExternalIpv6PrefixBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
externalIpv6Prefix_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object internalIpv6Prefix_ = "";
/**
*
*
*
* [Output Only] The internal IPv6 address range that is assigned to this subnetwork.
*
*
* optional string internal_ipv6_prefix = 506270056;
*
* @return Whether the internalIpv6Prefix field is set.
*/
public boolean hasInternalIpv6Prefix() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* [Output Only] The internal IPv6 address range that is assigned to this subnetwork.
*
*
* optional string internal_ipv6_prefix = 506270056;
*
* @return The internalIpv6Prefix.
*/
public java.lang.String getInternalIpv6Prefix() {
java.lang.Object ref = internalIpv6Prefix_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
internalIpv6Prefix_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* [Output Only] The internal IPv6 address range that is assigned to this subnetwork.
*
*
* optional string internal_ipv6_prefix = 506270056;
*
* @return The bytes for internalIpv6Prefix.
*/
public com.google.protobuf.ByteString getInternalIpv6PrefixBytes() {
java.lang.Object ref = internalIpv6Prefix_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
internalIpv6Prefix_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* [Output Only] The internal IPv6 address range that is assigned to this subnetwork.
*
*
* optional string internal_ipv6_prefix = 506270056;
*
* @param value The internalIpv6Prefix to set.
* @return This builder for chaining.
*/
public Builder setInternalIpv6Prefix(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
internalIpv6Prefix_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The internal IPv6 address range that is assigned to this subnetwork.
*
*
* optional string internal_ipv6_prefix = 506270056;
*
* @return This builder for chaining.
*/
public Builder clearInternalIpv6Prefix() {
internalIpv6Prefix_ = getDefaultInstance().getInternalIpv6Prefix();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* [Output Only] The internal IPv6 address range that is assigned to this subnetwork.
*
*
* optional string internal_ipv6_prefix = 506270056;
*
* @param value The bytes for internalIpv6Prefix to set.
* @return This builder for chaining.
*/
public Builder setInternalIpv6PrefixBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
internalIpv6Prefix_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object ipCidrRange_ = "";
/**
*
*
*
* The range of internal addresses that are owned by this subnetwork.
*
*
* optional string ip_cidr_range = 98117322;
*
* @return Whether the ipCidrRange field is set.
*/
public boolean hasIpCidrRange() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* The range of internal addresses that are owned by this subnetwork.
*
*
* optional string ip_cidr_range = 98117322;
*
* @return The ipCidrRange.
*/
public java.lang.String getIpCidrRange() {
java.lang.Object ref = ipCidrRange_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ipCidrRange_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The range of internal addresses that are owned by this subnetwork.
*
*
* optional string ip_cidr_range = 98117322;
*
* @return The bytes for ipCidrRange.
*/
public com.google.protobuf.ByteString getIpCidrRangeBytes() {
java.lang.Object ref = ipCidrRange_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
ipCidrRange_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The range of internal addresses that are owned by this subnetwork.
*
*
* optional string ip_cidr_range = 98117322;
*
* @param value The ipCidrRange to set.
* @return This builder for chaining.
*/
public Builder setIpCidrRange(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ipCidrRange_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* The range of internal addresses that are owned by this subnetwork.
*
*
* optional string ip_cidr_range = 98117322;
*
* @return This builder for chaining.
*/
public Builder clearIpCidrRange() {
ipCidrRange_ = getDefaultInstance().getIpCidrRange();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* The range of internal addresses that are owned by this subnetwork.
*
*
* optional string ip_cidr_range = 98117322;
*
* @param value The bytes for ipCidrRange to set.
* @return This builder for chaining.
*/
public Builder setIpCidrRangeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ipCidrRange_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object ipv6AccessType_ = "";
/**
*
*
*
* The access type of IPv6 address this subnet holds. It's immutable and can only be specified during creation or the first time the subnet is updated into IPV4_IPV6 dual stack.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return Whether the ipv6AccessType field is set.
*/
public boolean hasIpv6AccessType() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* The access type of IPv6 address this subnet holds. It's immutable and can only be specified during creation or the first time the subnet is updated into IPV4_IPV6 dual stack.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return The ipv6AccessType.
*/
public java.lang.String getIpv6AccessType() {
java.lang.Object ref = ipv6AccessType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
ipv6AccessType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The access type of IPv6 address this subnet holds. It's immutable and can only be specified during creation or the first time the subnet is updated into IPV4_IPV6 dual stack.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return The bytes for ipv6AccessType.
*/
public com.google.protobuf.ByteString getIpv6AccessTypeBytes() {
java.lang.Object ref = ipv6AccessType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
ipv6AccessType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The access type of IPv6 address this subnet holds. It's immutable and can only be specified during creation or the first time the subnet is updated into IPV4_IPV6 dual stack.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @param value The ipv6AccessType to set.
* @return This builder for chaining.
*/
public Builder setIpv6AccessType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ipv6AccessType_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* The access type of IPv6 address this subnet holds. It's immutable and can only be specified during creation or the first time the subnet is updated into IPV4_IPV6 dual stack.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @return This builder for chaining.
*/
public Builder clearIpv6AccessType() {
ipv6AccessType_ = getDefaultInstance().getIpv6AccessType();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
*
*
* The access type of IPv6 address this subnet holds. It's immutable and can only be specified during creation or the first time the subnet is updated into IPV4_IPV6 dual stack.
* Check the Ipv6AccessType enum for the list of possible values.
*
*
* optional string ipv6_access_type = 504658653;
*
* @param value The bytes for ipv6AccessType to set.
* @return This builder for chaining.
*/
public Builder setIpv6AccessTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ipv6AccessType_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object network_ = "";
/**
*
*
*
* Network URL.
*
*
* optional string network = 232872494;
*
* @return Whether the network field is set.
*/
public boolean hasNetwork() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Network URL.
*
*
* optional string network = 232872494;
*
* @return The network.
*/
public java.lang.String getNetwork() {
java.lang.Object ref = network_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
network_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Network URL.
*
*
* optional string network = 232872494;
*
* @return The bytes for network.
*/
public com.google.protobuf.ByteString getNetworkBytes() {
java.lang.Object ref = network_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
network_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Network URL.
*
*
* optional string network = 232872494;
*
* @param value The network to set.
* @return This builder for chaining.
*/
public Builder setNetwork(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
network_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Network URL.
*
*
* optional string network = 232872494;
*
* @return This builder for chaining.
*/
public Builder clearNetwork() {
network_ = getDefaultInstance().getNetwork();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
*
*
* Network URL.
*
*
* optional string network = 232872494;
*
* @param value The bytes for network to set.
* @return This builder for chaining.
*/
public Builder setNetworkBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
network_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private java.lang.Object purpose_ = "";
/**
*
*
*
* The purpose of the resource. This field can be either PRIVATE, GLOBAL_MANAGED_PROXY, REGIONAL_MANAGED_PROXY, PEER_MIGRATION or PRIVATE_SERVICE_CONNECT. PRIVATE is the default purpose for user-created subnets or subnets that are automatically created in auto mode networks. Subnets with purpose set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY are user-created subnetworks that are reserved for Envoy-based load balancers. A subnet with purpose set to PRIVATE_SERVICE_CONNECT is used to publish services using Private Service Connect. A subnet with purpose set to PEER_MIGRATION is used for subnet migration from one peered VPC to another. If unspecified, the subnet purpose defaults to PRIVATE. The enableFlowLogs field isn't supported if the subnet purpose field is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY.
* Check the Purpose enum for the list of possible values.
*
*
* optional string purpose = 316407070;
*
* @return Whether the purpose field is set.
*/
public boolean hasPurpose() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* The purpose of the resource. This field can be either PRIVATE, GLOBAL_MANAGED_PROXY, REGIONAL_MANAGED_PROXY, PEER_MIGRATION or PRIVATE_SERVICE_CONNECT. PRIVATE is the default purpose for user-created subnets or subnets that are automatically created in auto mode networks. Subnets with purpose set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY are user-created subnetworks that are reserved for Envoy-based load balancers. A subnet with purpose set to PRIVATE_SERVICE_CONNECT is used to publish services using Private Service Connect. A subnet with purpose set to PEER_MIGRATION is used for subnet migration from one peered VPC to another. If unspecified, the subnet purpose defaults to PRIVATE. The enableFlowLogs field isn't supported if the subnet purpose field is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY.
* Check the Purpose enum for the list of possible values.
*
*
* optional string purpose = 316407070;
*
* @return The purpose.
*/
public java.lang.String getPurpose() {
java.lang.Object ref = purpose_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
purpose_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The purpose of the resource. This field can be either PRIVATE, GLOBAL_MANAGED_PROXY, REGIONAL_MANAGED_PROXY, PEER_MIGRATION or PRIVATE_SERVICE_CONNECT. PRIVATE is the default purpose for user-created subnets or subnets that are automatically created in auto mode networks. Subnets with purpose set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY are user-created subnetworks that are reserved for Envoy-based load balancers. A subnet with purpose set to PRIVATE_SERVICE_CONNECT is used to publish services using Private Service Connect. A subnet with purpose set to PEER_MIGRATION is used for subnet migration from one peered VPC to another. If unspecified, the subnet purpose defaults to PRIVATE. The enableFlowLogs field isn't supported if the subnet purpose field is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY.
* Check the Purpose enum for the list of possible values.
*
*
* optional string purpose = 316407070;
*
* @return The bytes for purpose.
*/
public com.google.protobuf.ByteString getPurposeBytes() {
java.lang.Object ref = purpose_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
purpose_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The purpose of the resource. This field can be either PRIVATE, GLOBAL_MANAGED_PROXY, REGIONAL_MANAGED_PROXY, PEER_MIGRATION or PRIVATE_SERVICE_CONNECT. PRIVATE is the default purpose for user-created subnets or subnets that are automatically created in auto mode networks. Subnets with purpose set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY are user-created subnetworks that are reserved for Envoy-based load balancers. A subnet with purpose set to PRIVATE_SERVICE_CONNECT is used to publish services using Private Service Connect. A subnet with purpose set to PEER_MIGRATION is used for subnet migration from one peered VPC to another. If unspecified, the subnet purpose defaults to PRIVATE. The enableFlowLogs field isn't supported if the subnet purpose field is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY.
* Check the Purpose enum for the list of possible values.
*
*
* optional string purpose = 316407070;
*
* @param value The purpose to set.
* @return This builder for chaining.
*/
public Builder setPurpose(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
purpose_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* The purpose of the resource. This field can be either PRIVATE, GLOBAL_MANAGED_PROXY, REGIONAL_MANAGED_PROXY, PEER_MIGRATION or PRIVATE_SERVICE_CONNECT. PRIVATE is the default purpose for user-created subnets or subnets that are automatically created in auto mode networks. Subnets with purpose set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY are user-created subnetworks that are reserved for Envoy-based load balancers. A subnet with purpose set to PRIVATE_SERVICE_CONNECT is used to publish services using Private Service Connect. A subnet with purpose set to PEER_MIGRATION is used for subnet migration from one peered VPC to another. If unspecified, the subnet purpose defaults to PRIVATE. The enableFlowLogs field isn't supported if the subnet purpose field is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY.
* Check the Purpose enum for the list of possible values.
*
*
* optional string purpose = 316407070;
*
* @return This builder for chaining.
*/
public Builder clearPurpose() {
purpose_ = getDefaultInstance().getPurpose();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
*
*
* The purpose of the resource. This field can be either PRIVATE, GLOBAL_MANAGED_PROXY, REGIONAL_MANAGED_PROXY, PEER_MIGRATION or PRIVATE_SERVICE_CONNECT. PRIVATE is the default purpose for user-created subnets or subnets that are automatically created in auto mode networks. Subnets with purpose set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY are user-created subnetworks that are reserved for Envoy-based load balancers. A subnet with purpose set to PRIVATE_SERVICE_CONNECT is used to publish services using Private Service Connect. A subnet with purpose set to PEER_MIGRATION is used for subnet migration from one peered VPC to another. If unspecified, the subnet purpose defaults to PRIVATE. The enableFlowLogs field isn't supported if the subnet purpose field is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY.
* Check the Purpose enum for the list of possible values.
*
*
* optional string purpose = 316407070;
*
* @param value The bytes for purpose to set.
* @return This builder for chaining.
*/
public Builder setPurposeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
purpose_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.lang.Object role_ = "";
/**
*
*
*
* The role of subnetwork. Currently, this field is only used when purpose is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY. The value can be set to ACTIVE or BACKUP. An ACTIVE subnetwork is one that is currently being used for Envoy-based load balancers in a region. A BACKUP subnetwork is one that is ready to be promoted to ACTIVE or is currently draining. This field can be updated with a patch request.
* Check the Role enum for the list of possible values.
*
*
* optional string role = 3506294;
*
* @return Whether the role field is set.
*/
public boolean hasRole() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* The role of subnetwork. Currently, this field is only used when purpose is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY. The value can be set to ACTIVE or BACKUP. An ACTIVE subnetwork is one that is currently being used for Envoy-based load balancers in a region. A BACKUP subnetwork is one that is ready to be promoted to ACTIVE or is currently draining. This field can be updated with a patch request.
* Check the Role enum for the list of possible values.
*
*
* optional string role = 3506294;
*
* @return The role.
*/
public java.lang.String getRole() {
java.lang.Object ref = role_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
role_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The role of subnetwork. Currently, this field is only used when purpose is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY. The value can be set to ACTIVE or BACKUP. An ACTIVE subnetwork is one that is currently being used for Envoy-based load balancers in a region. A BACKUP subnetwork is one that is ready to be promoted to ACTIVE or is currently draining. This field can be updated with a patch request.
* Check the Role enum for the list of possible values.
*
*
* optional string role = 3506294;
*
* @return The bytes for role.
*/
public com.google.protobuf.ByteString getRoleBytes() {
java.lang.Object ref = role_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
role_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The role of subnetwork. Currently, this field is only used when purpose is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY. The value can be set to ACTIVE or BACKUP. An ACTIVE subnetwork is one that is currently being used for Envoy-based load balancers in a region. A BACKUP subnetwork is one that is ready to be promoted to ACTIVE or is currently draining. This field can be updated with a patch request.
* Check the Role enum for the list of possible values.
*
*
* optional string role = 3506294;
*
* @param value The role to set.
* @return This builder for chaining.
*/
public Builder setRole(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
role_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* The role of subnetwork. Currently, this field is only used when purpose is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY. The value can be set to ACTIVE or BACKUP. An ACTIVE subnetwork is one that is currently being used for Envoy-based load balancers in a region. A BACKUP subnetwork is one that is ready to be promoted to ACTIVE or is currently draining. This field can be updated with a patch request.
* Check the Role enum for the list of possible values.
*
*
* optional string role = 3506294;
*
* @return This builder for chaining.
*/
public Builder clearRole() {
role_ = getDefaultInstance().getRole();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* The role of subnetwork. Currently, this field is only used when purpose is set to GLOBAL_MANAGED_PROXY or REGIONAL_MANAGED_PROXY. The value can be set to ACTIVE or BACKUP. An ACTIVE subnetwork is one that is currently being used for Envoy-based load balancers in a region. A BACKUP subnetwork is one that is ready to be promoted to ACTIVE or is currently draining. This field can be updated with a patch request.
* Check the Role enum for the list of possible values.
*
*
* optional string role = 3506294;
*
* @param value The bytes for role to set.
* @return This builder for chaining.
*/
public Builder setRoleBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
role_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private java.util.List
secondaryIpRanges_ = java.util.Collections.emptyList();
private void ensureSecondaryIpRangesIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
secondaryIpRanges_ =
new java.util.ArrayList(
secondaryIpRanges_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange,
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.Builder,
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRangeOrBuilder>
secondaryIpRangesBuilder_;
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public java.util.List
getSecondaryIpRangesList() {
if (secondaryIpRangesBuilder_ == null) {
return java.util.Collections.unmodifiableList(secondaryIpRanges_);
} else {
return secondaryIpRangesBuilder_.getMessageList();
}
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public int getSecondaryIpRangesCount() {
if (secondaryIpRangesBuilder_ == null) {
return secondaryIpRanges_.size();
} else {
return secondaryIpRangesBuilder_.getCount();
}
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange getSecondaryIpRanges(
int index) {
if (secondaryIpRangesBuilder_ == null) {
return secondaryIpRanges_.get(index);
} else {
return secondaryIpRangesBuilder_.getMessage(index);
}
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public Builder setSecondaryIpRanges(
int index, com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange value) {
if (secondaryIpRangesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSecondaryIpRangesIsMutable();
secondaryIpRanges_.set(index, value);
onChanged();
} else {
secondaryIpRangesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public Builder setSecondaryIpRanges(
int index,
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.Builder builderForValue) {
if (secondaryIpRangesBuilder_ == null) {
ensureSecondaryIpRangesIsMutable();
secondaryIpRanges_.set(index, builderForValue.build());
onChanged();
} else {
secondaryIpRangesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public Builder addSecondaryIpRanges(
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange value) {
if (secondaryIpRangesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSecondaryIpRangesIsMutable();
secondaryIpRanges_.add(value);
onChanged();
} else {
secondaryIpRangesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public Builder addSecondaryIpRanges(
int index, com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange value) {
if (secondaryIpRangesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSecondaryIpRangesIsMutable();
secondaryIpRanges_.add(index, value);
onChanged();
} else {
secondaryIpRangesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public Builder addSecondaryIpRanges(
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.Builder builderForValue) {
if (secondaryIpRangesBuilder_ == null) {
ensureSecondaryIpRangesIsMutable();
secondaryIpRanges_.add(builderForValue.build());
onChanged();
} else {
secondaryIpRangesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public Builder addSecondaryIpRanges(
int index,
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.Builder builderForValue) {
if (secondaryIpRangesBuilder_ == null) {
ensureSecondaryIpRangesIsMutable();
secondaryIpRanges_.add(index, builderForValue.build());
onChanged();
} else {
secondaryIpRangesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public Builder addAllSecondaryIpRanges(
java.lang.Iterable extends com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange>
values) {
if (secondaryIpRangesBuilder_ == null) {
ensureSecondaryIpRangesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, secondaryIpRanges_);
onChanged();
} else {
secondaryIpRangesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public Builder clearSecondaryIpRanges() {
if (secondaryIpRangesBuilder_ == null) {
secondaryIpRanges_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
secondaryIpRangesBuilder_.clear();
}
return this;
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public Builder removeSecondaryIpRanges(int index) {
if (secondaryIpRangesBuilder_ == null) {
ensureSecondaryIpRangesIsMutable();
secondaryIpRanges_.remove(index);
onChanged();
} else {
secondaryIpRangesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.Builder
getSecondaryIpRangesBuilder(int index) {
return getSecondaryIpRangesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public com.google.cloud.compute.v1.UsableSubnetworkSecondaryRangeOrBuilder
getSecondaryIpRangesOrBuilder(int index) {
if (secondaryIpRangesBuilder_ == null) {
return secondaryIpRanges_.get(index);
} else {
return secondaryIpRangesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public java.util.List<
? extends com.google.cloud.compute.v1.UsableSubnetworkSecondaryRangeOrBuilder>
getSecondaryIpRangesOrBuilderList() {
if (secondaryIpRangesBuilder_ != null) {
return secondaryIpRangesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(secondaryIpRanges_);
}
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.Builder
addSecondaryIpRangesBuilder() {
return getSecondaryIpRangesFieldBuilder()
.addBuilder(
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.getDefaultInstance());
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.Builder
addSecondaryIpRangesBuilder(int index) {
return getSecondaryIpRangesFieldBuilder()
.addBuilder(
index,
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.getDefaultInstance());
}
/**
*
*
*
* Secondary IP ranges.
*
*
*
* repeated .google.cloud.compute.v1.UsableSubnetworkSecondaryRange secondary_ip_ranges = 136658915;
*
*/
public java.util.List
getSecondaryIpRangesBuilderList() {
return getSecondaryIpRangesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange,
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.Builder,
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRangeOrBuilder>
getSecondaryIpRangesFieldBuilder() {
if (secondaryIpRangesBuilder_ == null) {
secondaryIpRangesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange,
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRange.Builder,
com.google.cloud.compute.v1.UsableSubnetworkSecondaryRangeOrBuilder>(
secondaryIpRanges_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
secondaryIpRanges_ = null;
}
return secondaryIpRangesBuilder_;
}
private java.lang.Object stackType_ = "";
/**
*
*
*
* The stack type for the subnet. If set to IPV4_ONLY, new VMs in the subnet are assigned IPv4 addresses only. If set to IPV4_IPV6, new VMs in the subnet can be assigned both IPv4 and IPv6 addresses. If not specified, IPV4_ONLY is used. This field can be both set at resource creation time and updated using patch.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return Whether the stackType field is set.
*/
public boolean hasStackType() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* The stack type for the subnet. If set to IPV4_ONLY, new VMs in the subnet are assigned IPv4 addresses only. If set to IPV4_IPV6, new VMs in the subnet can be assigned both IPv4 and IPv6 addresses. If not specified, IPV4_ONLY is used. This field can be both set at resource creation time and updated using patch.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The stackType.
*/
public java.lang.String getStackType() {
java.lang.Object ref = stackType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stackType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The stack type for the subnet. If set to IPV4_ONLY, new VMs in the subnet are assigned IPv4 addresses only. If set to IPV4_IPV6, new VMs in the subnet can be assigned both IPv4 and IPv6 addresses. If not specified, IPV4_ONLY is used. This field can be both set at resource creation time and updated using patch.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return The bytes for stackType.
*/
public com.google.protobuf.ByteString getStackTypeBytes() {
java.lang.Object ref = stackType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
stackType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The stack type for the subnet. If set to IPV4_ONLY, new VMs in the subnet are assigned IPv4 addresses only. If set to IPV4_IPV6, new VMs in the subnet can be assigned both IPv4 and IPv6 addresses. If not specified, IPV4_ONLY is used. This field can be both set at resource creation time and updated using patch.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @param value The stackType to set.
* @return This builder for chaining.
*/
public Builder setStackType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stackType_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* The stack type for the subnet. If set to IPV4_ONLY, new VMs in the subnet are assigned IPv4 addresses only. If set to IPV4_IPV6, new VMs in the subnet can be assigned both IPv4 and IPv6 addresses. If not specified, IPV4_ONLY is used. This field can be both set at resource creation time and updated using patch.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @return This builder for chaining.
*/
public Builder clearStackType() {
stackType_ = getDefaultInstance().getStackType();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
*
*
*
* The stack type for the subnet. If set to IPV4_ONLY, new VMs in the subnet are assigned IPv4 addresses only. If set to IPV4_IPV6, new VMs in the subnet can be assigned both IPv4 and IPv6 addresses. If not specified, IPV4_ONLY is used. This field can be both set at resource creation time and updated using patch.
* Check the StackType enum for the list of possible values.
*
*
* optional string stack_type = 425908881;
*
* @param value The bytes for stackType to set.
* @return This builder for chaining.
*/
public Builder setStackTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stackType_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private java.lang.Object subnetwork_ = "";
/**
*
*
*
* Subnetwork URL.
*
*
* optional string subnetwork = 307827694;
*
* @return Whether the subnetwork field is set.
*/
public boolean hasSubnetwork() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Subnetwork URL.
*
*
* optional string subnetwork = 307827694;
*
* @return The subnetwork.
*/
public java.lang.String getSubnetwork() {
java.lang.Object ref = subnetwork_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subnetwork_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Subnetwork URL.
*
*
* optional string subnetwork = 307827694;
*
* @return The bytes for subnetwork.
*/
public com.google.protobuf.ByteString getSubnetworkBytes() {
java.lang.Object ref = subnetwork_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
subnetwork_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Subnetwork URL.
*
*
* optional string subnetwork = 307827694;
*
* @param value The subnetwork to set.
* @return This builder for chaining.
*/
public Builder setSubnetwork(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
subnetwork_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Subnetwork URL.
*
*
* optional string subnetwork = 307827694;
*
* @return This builder for chaining.
*/
public Builder clearSubnetwork() {
subnetwork_ = getDefaultInstance().getSubnetwork();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
*
*
*
* Subnetwork URL.
*
*
* optional string subnetwork = 307827694;
*
* @param value The bytes for subnetwork to set.
* @return This builder for chaining.
*/
public Builder setSubnetworkBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
subnetwork_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.compute.v1.UsableSubnetwork)
}
// @@protoc_insertion_point(class_scope:google.cloud.compute.v1.UsableSubnetwork)
private static final com.google.cloud.compute.v1.UsableSubnetwork DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.compute.v1.UsableSubnetwork();
}
public static com.google.cloud.compute.v1.UsableSubnetwork getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UsableSubnetwork parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.compute.v1.UsableSubnetwork getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy