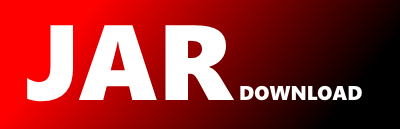
com.google.cloud.dataproc.v1.ClusterConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-dataproc-v1 Show documentation
Show all versions of proto-google-cloud-dataproc-v1 Show documentation
PROTO library for proto-google-cloud-dataproc-v1
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/dataproc/v1/clusters.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.dataproc.v1;
/**
*
*
*
* The cluster config.
*
*
* Protobuf type {@code google.cloud.dataproc.v1.ClusterConfig}
*/
public final class ClusterConfig extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.dataproc.v1.ClusterConfig)
ClusterConfigOrBuilder {
private static final long serialVersionUID = 0L;
// Use ClusterConfig.newBuilder() to construct.
private ClusterConfig(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ClusterConfig() {
configBucket_ = "";
tempBucket_ = "";
initializationActions_ = java.util.Collections.emptyList();
auxiliaryNodeGroups_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new ClusterConfig();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.dataproc.v1.ClustersProto
.internal_static_google_cloud_dataproc_v1_ClusterConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.dataproc.v1.ClustersProto
.internal_static_google_cloud_dataproc_v1_ClusterConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.dataproc.v1.ClusterConfig.class,
com.google.cloud.dataproc.v1.ClusterConfig.Builder.class);
}
private int bitField0_;
public static final int CONFIG_BUCKET_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object configBucket_ = "";
/**
*
*
*
* Optional. A Cloud Storage bucket used to stage job
* dependencies, config files, and job driver console output.
* If you do not specify a staging bucket, Cloud
* Dataproc will determine a Cloud Storage location (US,
* ASIA, or EU) for your cluster's staging bucket according to the
* Compute Engine zone where your cluster is deployed, and then create
* and manage this project-level, per-location bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string config_bucket = 1 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The configBucket.
*/
@java.lang.Override
public java.lang.String getConfigBucket() {
java.lang.Object ref = configBucket_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
configBucket_ = s;
return s;
}
}
/**
*
*
*
* Optional. A Cloud Storage bucket used to stage job
* dependencies, config files, and job driver console output.
* If you do not specify a staging bucket, Cloud
* Dataproc will determine a Cloud Storage location (US,
* ASIA, or EU) for your cluster's staging bucket according to the
* Compute Engine zone where your cluster is deployed, and then create
* and manage this project-level, per-location bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string config_bucket = 1 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for configBucket.
*/
@java.lang.Override
public com.google.protobuf.ByteString getConfigBucketBytes() {
java.lang.Object ref = configBucket_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
configBucket_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TEMP_BUCKET_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object tempBucket_ = "";
/**
*
*
*
* Optional. A Cloud Storage bucket used to store ephemeral cluster and jobs
* data, such as Spark and MapReduce history files. If you do not specify a
* temp bucket, Dataproc will determine a Cloud Storage location (US, ASIA, or
* EU) for your cluster's temp bucket according to the Compute Engine zone
* where your cluster is deployed, and then create and manage this
* project-level, per-location bucket. The default bucket has a TTL of 90
* days, but you can use any TTL (or none) if you specify a bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string temp_bucket = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The tempBucket.
*/
@java.lang.Override
public java.lang.String getTempBucket() {
java.lang.Object ref = tempBucket_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tempBucket_ = s;
return s;
}
}
/**
*
*
*
* Optional. A Cloud Storage bucket used to store ephemeral cluster and jobs
* data, such as Spark and MapReduce history files. If you do not specify a
* temp bucket, Dataproc will determine a Cloud Storage location (US, ASIA, or
* EU) for your cluster's temp bucket according to the Compute Engine zone
* where your cluster is deployed, and then create and manage this
* project-level, per-location bucket. The default bucket has a TTL of 90
* days, but you can use any TTL (or none) if you specify a bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string temp_bucket = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for tempBucket.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTempBucketBytes() {
java.lang.Object ref = tempBucket_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
tempBucket_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int GCE_CLUSTER_CONFIG_FIELD_NUMBER = 8;
private com.google.cloud.dataproc.v1.GceClusterConfig gceClusterConfig_;
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the gceClusterConfig field is set.
*/
@java.lang.Override
public boolean hasGceClusterConfig() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The gceClusterConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.GceClusterConfig getGceClusterConfig() {
return gceClusterConfig_ == null
? com.google.cloud.dataproc.v1.GceClusterConfig.getDefaultInstance()
: gceClusterConfig_;
}
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.GceClusterConfigOrBuilder getGceClusterConfigOrBuilder() {
return gceClusterConfig_ == null
? com.google.cloud.dataproc.v1.GceClusterConfig.getDefaultInstance()
: gceClusterConfig_;
}
public static final int MASTER_CONFIG_FIELD_NUMBER = 9;
private com.google.cloud.dataproc.v1.InstanceGroupConfig masterConfig_;
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the masterConfig field is set.
*/
@java.lang.Override
public boolean hasMasterConfig() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The masterConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.InstanceGroupConfig getMasterConfig() {
return masterConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: masterConfig_;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder getMasterConfigOrBuilder() {
return masterConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: masterConfig_;
}
public static final int WORKER_CONFIG_FIELD_NUMBER = 10;
private com.google.cloud.dataproc.v1.InstanceGroupConfig workerConfig_;
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the workerConfig field is set.
*/
@java.lang.Override
public boolean hasWorkerConfig() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The workerConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.InstanceGroupConfig getWorkerConfig() {
return workerConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: workerConfig_;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder getWorkerConfigOrBuilder() {
return workerConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: workerConfig_;
}
public static final int SECONDARY_WORKER_CONFIG_FIELD_NUMBER = 12;
private com.google.cloud.dataproc.v1.InstanceGroupConfig secondaryWorkerConfig_;
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the secondaryWorkerConfig field is set.
*/
@java.lang.Override
public boolean hasSecondaryWorkerConfig() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The secondaryWorkerConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.InstanceGroupConfig getSecondaryWorkerConfig() {
return secondaryWorkerConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: secondaryWorkerConfig_;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder
getSecondaryWorkerConfigOrBuilder() {
return secondaryWorkerConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: secondaryWorkerConfig_;
}
public static final int SOFTWARE_CONFIG_FIELD_NUMBER = 13;
private com.google.cloud.dataproc.v1.SoftwareConfig softwareConfig_;
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the softwareConfig field is set.
*/
@java.lang.Override
public boolean hasSoftwareConfig() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The softwareConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.SoftwareConfig getSoftwareConfig() {
return softwareConfig_ == null
? com.google.cloud.dataproc.v1.SoftwareConfig.getDefaultInstance()
: softwareConfig_;
}
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.SoftwareConfigOrBuilder getSoftwareConfigOrBuilder() {
return softwareConfig_ == null
? com.google.cloud.dataproc.v1.SoftwareConfig.getDefaultInstance()
: softwareConfig_;
}
public static final int INITIALIZATION_ACTIONS_FIELD_NUMBER = 11;
@SuppressWarnings("serial")
private java.util.List
initializationActions_;
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List
getInitializationActionsList() {
return initializationActions_;
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List extends com.google.cloud.dataproc.v1.NodeInitializationActionOrBuilder>
getInitializationActionsOrBuilderList() {
return initializationActions_;
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public int getInitializationActionsCount() {
return initializationActions_.size();
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.NodeInitializationAction getInitializationActions(int index) {
return initializationActions_.get(index);
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.NodeInitializationActionOrBuilder
getInitializationActionsOrBuilder(int index) {
return initializationActions_.get(index);
}
public static final int ENCRYPTION_CONFIG_FIELD_NUMBER = 15;
private com.google.cloud.dataproc.v1.EncryptionConfig encryptionConfig_;
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the encryptionConfig field is set.
*/
@java.lang.Override
public boolean hasEncryptionConfig() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The encryptionConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.EncryptionConfig getEncryptionConfig() {
return encryptionConfig_ == null
? com.google.cloud.dataproc.v1.EncryptionConfig.getDefaultInstance()
: encryptionConfig_;
}
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.EncryptionConfigOrBuilder getEncryptionConfigOrBuilder() {
return encryptionConfig_ == null
? com.google.cloud.dataproc.v1.EncryptionConfig.getDefaultInstance()
: encryptionConfig_;
}
public static final int AUTOSCALING_CONFIG_FIELD_NUMBER = 18;
private com.google.cloud.dataproc.v1.AutoscalingConfig autoscalingConfig_;
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the autoscalingConfig field is set.
*/
@java.lang.Override
public boolean hasAutoscalingConfig() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The autoscalingConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.AutoscalingConfig getAutoscalingConfig() {
return autoscalingConfig_ == null
? com.google.cloud.dataproc.v1.AutoscalingConfig.getDefaultInstance()
: autoscalingConfig_;
}
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.AutoscalingConfigOrBuilder getAutoscalingConfigOrBuilder() {
return autoscalingConfig_ == null
? com.google.cloud.dataproc.v1.AutoscalingConfig.getDefaultInstance()
: autoscalingConfig_;
}
public static final int SECURITY_CONFIG_FIELD_NUMBER = 16;
private com.google.cloud.dataproc.v1.SecurityConfig securityConfig_;
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the securityConfig field is set.
*/
@java.lang.Override
public boolean hasSecurityConfig() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The securityConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.SecurityConfig getSecurityConfig() {
return securityConfig_ == null
? com.google.cloud.dataproc.v1.SecurityConfig.getDefaultInstance()
: securityConfig_;
}
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.SecurityConfigOrBuilder getSecurityConfigOrBuilder() {
return securityConfig_ == null
? com.google.cloud.dataproc.v1.SecurityConfig.getDefaultInstance()
: securityConfig_;
}
public static final int LIFECYCLE_CONFIG_FIELD_NUMBER = 17;
private com.google.cloud.dataproc.v1.LifecycleConfig lifecycleConfig_;
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the lifecycleConfig field is set.
*/
@java.lang.Override
public boolean hasLifecycleConfig() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The lifecycleConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.LifecycleConfig getLifecycleConfig() {
return lifecycleConfig_ == null
? com.google.cloud.dataproc.v1.LifecycleConfig.getDefaultInstance()
: lifecycleConfig_;
}
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.LifecycleConfigOrBuilder getLifecycleConfigOrBuilder() {
return lifecycleConfig_ == null
? com.google.cloud.dataproc.v1.LifecycleConfig.getDefaultInstance()
: lifecycleConfig_;
}
public static final int ENDPOINT_CONFIG_FIELD_NUMBER = 19;
private com.google.cloud.dataproc.v1.EndpointConfig endpointConfig_;
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the endpointConfig field is set.
*/
@java.lang.Override
public boolean hasEndpointConfig() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The endpointConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.EndpointConfig getEndpointConfig() {
return endpointConfig_ == null
? com.google.cloud.dataproc.v1.EndpointConfig.getDefaultInstance()
: endpointConfig_;
}
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.EndpointConfigOrBuilder getEndpointConfigOrBuilder() {
return endpointConfig_ == null
? com.google.cloud.dataproc.v1.EndpointConfig.getDefaultInstance()
: endpointConfig_;
}
public static final int METASTORE_CONFIG_FIELD_NUMBER = 20;
private com.google.cloud.dataproc.v1.MetastoreConfig metastoreConfig_;
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the metastoreConfig field is set.
*/
@java.lang.Override
public boolean hasMetastoreConfig() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The metastoreConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.MetastoreConfig getMetastoreConfig() {
return metastoreConfig_ == null
? com.google.cloud.dataproc.v1.MetastoreConfig.getDefaultInstance()
: metastoreConfig_;
}
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.MetastoreConfigOrBuilder getMetastoreConfigOrBuilder() {
return metastoreConfig_ == null
? com.google.cloud.dataproc.v1.MetastoreConfig.getDefaultInstance()
: metastoreConfig_;
}
public static final int DATAPROC_METRIC_CONFIG_FIELD_NUMBER = 23;
private com.google.cloud.dataproc.v1.DataprocMetricConfig dataprocMetricConfig_;
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the dataprocMetricConfig field is set.
*/
@java.lang.Override
public boolean hasDataprocMetricConfig() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The dataprocMetricConfig.
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.DataprocMetricConfig getDataprocMetricConfig() {
return dataprocMetricConfig_ == null
? com.google.cloud.dataproc.v1.DataprocMetricConfig.getDefaultInstance()
: dataprocMetricConfig_;
}
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.DataprocMetricConfigOrBuilder
getDataprocMetricConfigOrBuilder() {
return dataprocMetricConfig_ == null
? com.google.cloud.dataproc.v1.DataprocMetricConfig.getDefaultInstance()
: dataprocMetricConfig_;
}
public static final int AUXILIARY_NODE_GROUPS_FIELD_NUMBER = 25;
@SuppressWarnings("serial")
private java.util.List auxiliaryNodeGroups_;
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List
getAuxiliaryNodeGroupsList() {
return auxiliaryNodeGroups_;
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public java.util.List extends com.google.cloud.dataproc.v1.AuxiliaryNodeGroupOrBuilder>
getAuxiliaryNodeGroupsOrBuilderList() {
return auxiliaryNodeGroups_;
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public int getAuxiliaryNodeGroupsCount() {
return auxiliaryNodeGroups_.size();
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.AuxiliaryNodeGroup getAuxiliaryNodeGroups(int index) {
return auxiliaryNodeGroups_.get(index);
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.cloud.dataproc.v1.AuxiliaryNodeGroupOrBuilder getAuxiliaryNodeGroupsOrBuilder(
int index) {
return auxiliaryNodeGroups_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(configBucket_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, configBucket_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tempBucket_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, tempBucket_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(8, getGceClusterConfig());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(9, getMasterConfig());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(10, getWorkerConfig());
}
for (int i = 0; i < initializationActions_.size(); i++) {
output.writeMessage(11, initializationActions_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(12, getSecondaryWorkerConfig());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(13, getSoftwareConfig());
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(15, getEncryptionConfig());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeMessage(16, getSecurityConfig());
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeMessage(17, getLifecycleConfig());
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(18, getAutoscalingConfig());
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeMessage(19, getEndpointConfig());
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeMessage(20, getMetastoreConfig());
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeMessage(23, getDataprocMetricConfig());
}
for (int i = 0; i < auxiliaryNodeGroups_.size(); i++) {
output.writeMessage(25, auxiliaryNodeGroups_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(configBucket_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, configBucket_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tempBucket_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, tempBucket_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(8, getGceClusterConfig());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(9, getMasterConfig());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(10, getWorkerConfig());
}
for (int i = 0; i < initializationActions_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
11, initializationActions_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(12, getSecondaryWorkerConfig());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(13, getSoftwareConfig());
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(15, getEncryptionConfig());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(16, getSecurityConfig());
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(17, getLifecycleConfig());
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(18, getAutoscalingConfig());
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(19, getEndpointConfig());
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(20, getMetastoreConfig());
}
if (((bitField0_ & 0x00000800) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(23, getDataprocMetricConfig());
}
for (int i = 0; i < auxiliaryNodeGroups_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(25, auxiliaryNodeGroups_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.dataproc.v1.ClusterConfig)) {
return super.equals(obj);
}
com.google.cloud.dataproc.v1.ClusterConfig other =
(com.google.cloud.dataproc.v1.ClusterConfig) obj;
if (!getConfigBucket().equals(other.getConfigBucket())) return false;
if (!getTempBucket().equals(other.getTempBucket())) return false;
if (hasGceClusterConfig() != other.hasGceClusterConfig()) return false;
if (hasGceClusterConfig()) {
if (!getGceClusterConfig().equals(other.getGceClusterConfig())) return false;
}
if (hasMasterConfig() != other.hasMasterConfig()) return false;
if (hasMasterConfig()) {
if (!getMasterConfig().equals(other.getMasterConfig())) return false;
}
if (hasWorkerConfig() != other.hasWorkerConfig()) return false;
if (hasWorkerConfig()) {
if (!getWorkerConfig().equals(other.getWorkerConfig())) return false;
}
if (hasSecondaryWorkerConfig() != other.hasSecondaryWorkerConfig()) return false;
if (hasSecondaryWorkerConfig()) {
if (!getSecondaryWorkerConfig().equals(other.getSecondaryWorkerConfig())) return false;
}
if (hasSoftwareConfig() != other.hasSoftwareConfig()) return false;
if (hasSoftwareConfig()) {
if (!getSoftwareConfig().equals(other.getSoftwareConfig())) return false;
}
if (!getInitializationActionsList().equals(other.getInitializationActionsList())) return false;
if (hasEncryptionConfig() != other.hasEncryptionConfig()) return false;
if (hasEncryptionConfig()) {
if (!getEncryptionConfig().equals(other.getEncryptionConfig())) return false;
}
if (hasAutoscalingConfig() != other.hasAutoscalingConfig()) return false;
if (hasAutoscalingConfig()) {
if (!getAutoscalingConfig().equals(other.getAutoscalingConfig())) return false;
}
if (hasSecurityConfig() != other.hasSecurityConfig()) return false;
if (hasSecurityConfig()) {
if (!getSecurityConfig().equals(other.getSecurityConfig())) return false;
}
if (hasLifecycleConfig() != other.hasLifecycleConfig()) return false;
if (hasLifecycleConfig()) {
if (!getLifecycleConfig().equals(other.getLifecycleConfig())) return false;
}
if (hasEndpointConfig() != other.hasEndpointConfig()) return false;
if (hasEndpointConfig()) {
if (!getEndpointConfig().equals(other.getEndpointConfig())) return false;
}
if (hasMetastoreConfig() != other.hasMetastoreConfig()) return false;
if (hasMetastoreConfig()) {
if (!getMetastoreConfig().equals(other.getMetastoreConfig())) return false;
}
if (hasDataprocMetricConfig() != other.hasDataprocMetricConfig()) return false;
if (hasDataprocMetricConfig()) {
if (!getDataprocMetricConfig().equals(other.getDataprocMetricConfig())) return false;
}
if (!getAuxiliaryNodeGroupsList().equals(other.getAuxiliaryNodeGroupsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONFIG_BUCKET_FIELD_NUMBER;
hash = (53 * hash) + getConfigBucket().hashCode();
hash = (37 * hash) + TEMP_BUCKET_FIELD_NUMBER;
hash = (53 * hash) + getTempBucket().hashCode();
if (hasGceClusterConfig()) {
hash = (37 * hash) + GCE_CLUSTER_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getGceClusterConfig().hashCode();
}
if (hasMasterConfig()) {
hash = (37 * hash) + MASTER_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getMasterConfig().hashCode();
}
if (hasWorkerConfig()) {
hash = (37 * hash) + WORKER_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getWorkerConfig().hashCode();
}
if (hasSecondaryWorkerConfig()) {
hash = (37 * hash) + SECONDARY_WORKER_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getSecondaryWorkerConfig().hashCode();
}
if (hasSoftwareConfig()) {
hash = (37 * hash) + SOFTWARE_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getSoftwareConfig().hashCode();
}
if (getInitializationActionsCount() > 0) {
hash = (37 * hash) + INITIALIZATION_ACTIONS_FIELD_NUMBER;
hash = (53 * hash) + getInitializationActionsList().hashCode();
}
if (hasEncryptionConfig()) {
hash = (37 * hash) + ENCRYPTION_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getEncryptionConfig().hashCode();
}
if (hasAutoscalingConfig()) {
hash = (37 * hash) + AUTOSCALING_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getAutoscalingConfig().hashCode();
}
if (hasSecurityConfig()) {
hash = (37 * hash) + SECURITY_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getSecurityConfig().hashCode();
}
if (hasLifecycleConfig()) {
hash = (37 * hash) + LIFECYCLE_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getLifecycleConfig().hashCode();
}
if (hasEndpointConfig()) {
hash = (37 * hash) + ENDPOINT_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getEndpointConfig().hashCode();
}
if (hasMetastoreConfig()) {
hash = (37 * hash) + METASTORE_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getMetastoreConfig().hashCode();
}
if (hasDataprocMetricConfig()) {
hash = (37 * hash) + DATAPROC_METRIC_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getDataprocMetricConfig().hashCode();
}
if (getAuxiliaryNodeGroupsCount() > 0) {
hash = (37 * hash) + AUXILIARY_NODE_GROUPS_FIELD_NUMBER;
hash = (53 * hash) + getAuxiliaryNodeGroupsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.dataproc.v1.ClusterConfig parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.dataproc.v1.ClusterConfig prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* The cluster config.
*
*
* Protobuf type {@code google.cloud.dataproc.v1.ClusterConfig}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.dataproc.v1.ClusterConfig)
com.google.cloud.dataproc.v1.ClusterConfigOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.dataproc.v1.ClustersProto
.internal_static_google_cloud_dataproc_v1_ClusterConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.dataproc.v1.ClustersProto
.internal_static_google_cloud_dataproc_v1_ClusterConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.dataproc.v1.ClusterConfig.class,
com.google.cloud.dataproc.v1.ClusterConfig.Builder.class);
}
// Construct using com.google.cloud.dataproc.v1.ClusterConfig.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getGceClusterConfigFieldBuilder();
getMasterConfigFieldBuilder();
getWorkerConfigFieldBuilder();
getSecondaryWorkerConfigFieldBuilder();
getSoftwareConfigFieldBuilder();
getInitializationActionsFieldBuilder();
getEncryptionConfigFieldBuilder();
getAutoscalingConfigFieldBuilder();
getSecurityConfigFieldBuilder();
getLifecycleConfigFieldBuilder();
getEndpointConfigFieldBuilder();
getMetastoreConfigFieldBuilder();
getDataprocMetricConfigFieldBuilder();
getAuxiliaryNodeGroupsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
configBucket_ = "";
tempBucket_ = "";
gceClusterConfig_ = null;
if (gceClusterConfigBuilder_ != null) {
gceClusterConfigBuilder_.dispose();
gceClusterConfigBuilder_ = null;
}
masterConfig_ = null;
if (masterConfigBuilder_ != null) {
masterConfigBuilder_.dispose();
masterConfigBuilder_ = null;
}
workerConfig_ = null;
if (workerConfigBuilder_ != null) {
workerConfigBuilder_.dispose();
workerConfigBuilder_ = null;
}
secondaryWorkerConfig_ = null;
if (secondaryWorkerConfigBuilder_ != null) {
secondaryWorkerConfigBuilder_.dispose();
secondaryWorkerConfigBuilder_ = null;
}
softwareConfig_ = null;
if (softwareConfigBuilder_ != null) {
softwareConfigBuilder_.dispose();
softwareConfigBuilder_ = null;
}
if (initializationActionsBuilder_ == null) {
initializationActions_ = java.util.Collections.emptyList();
} else {
initializationActions_ = null;
initializationActionsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
encryptionConfig_ = null;
if (encryptionConfigBuilder_ != null) {
encryptionConfigBuilder_.dispose();
encryptionConfigBuilder_ = null;
}
autoscalingConfig_ = null;
if (autoscalingConfigBuilder_ != null) {
autoscalingConfigBuilder_.dispose();
autoscalingConfigBuilder_ = null;
}
securityConfig_ = null;
if (securityConfigBuilder_ != null) {
securityConfigBuilder_.dispose();
securityConfigBuilder_ = null;
}
lifecycleConfig_ = null;
if (lifecycleConfigBuilder_ != null) {
lifecycleConfigBuilder_.dispose();
lifecycleConfigBuilder_ = null;
}
endpointConfig_ = null;
if (endpointConfigBuilder_ != null) {
endpointConfigBuilder_.dispose();
endpointConfigBuilder_ = null;
}
metastoreConfig_ = null;
if (metastoreConfigBuilder_ != null) {
metastoreConfigBuilder_.dispose();
metastoreConfigBuilder_ = null;
}
dataprocMetricConfig_ = null;
if (dataprocMetricConfigBuilder_ != null) {
dataprocMetricConfigBuilder_.dispose();
dataprocMetricConfigBuilder_ = null;
}
if (auxiliaryNodeGroupsBuilder_ == null) {
auxiliaryNodeGroups_ = java.util.Collections.emptyList();
} else {
auxiliaryNodeGroups_ = null;
auxiliaryNodeGroupsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00008000);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.dataproc.v1.ClustersProto
.internal_static_google_cloud_dataproc_v1_ClusterConfig_descriptor;
}
@java.lang.Override
public com.google.cloud.dataproc.v1.ClusterConfig getDefaultInstanceForType() {
return com.google.cloud.dataproc.v1.ClusterConfig.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.dataproc.v1.ClusterConfig build() {
com.google.cloud.dataproc.v1.ClusterConfig result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.dataproc.v1.ClusterConfig buildPartial() {
com.google.cloud.dataproc.v1.ClusterConfig result =
new com.google.cloud.dataproc.v1.ClusterConfig(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.cloud.dataproc.v1.ClusterConfig result) {
if (initializationActionsBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
initializationActions_ = java.util.Collections.unmodifiableList(initializationActions_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.initializationActions_ = initializationActions_;
} else {
result.initializationActions_ = initializationActionsBuilder_.build();
}
if (auxiliaryNodeGroupsBuilder_ == null) {
if (((bitField0_ & 0x00008000) != 0)) {
auxiliaryNodeGroups_ = java.util.Collections.unmodifiableList(auxiliaryNodeGroups_);
bitField0_ = (bitField0_ & ~0x00008000);
}
result.auxiliaryNodeGroups_ = auxiliaryNodeGroups_;
} else {
result.auxiliaryNodeGroups_ = auxiliaryNodeGroupsBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.dataproc.v1.ClusterConfig result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.configBucket_ = configBucket_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.tempBucket_ = tempBucket_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.gceClusterConfig_ =
gceClusterConfigBuilder_ == null ? gceClusterConfig_ : gceClusterConfigBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.masterConfig_ =
masterConfigBuilder_ == null ? masterConfig_ : masterConfigBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.workerConfig_ =
workerConfigBuilder_ == null ? workerConfig_ : workerConfigBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.secondaryWorkerConfig_ =
secondaryWorkerConfigBuilder_ == null
? secondaryWorkerConfig_
: secondaryWorkerConfigBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.softwareConfig_ =
softwareConfigBuilder_ == null ? softwareConfig_ : softwareConfigBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.encryptionConfig_ =
encryptionConfigBuilder_ == null ? encryptionConfig_ : encryptionConfigBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.autoscalingConfig_ =
autoscalingConfigBuilder_ == null
? autoscalingConfig_
: autoscalingConfigBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.securityConfig_ =
securityConfigBuilder_ == null ? securityConfig_ : securityConfigBuilder_.build();
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.lifecycleConfig_ =
lifecycleConfigBuilder_ == null ? lifecycleConfig_ : lifecycleConfigBuilder_.build();
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.endpointConfig_ =
endpointConfigBuilder_ == null ? endpointConfig_ : endpointConfigBuilder_.build();
to_bitField0_ |= 0x00000200;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.metastoreConfig_ =
metastoreConfigBuilder_ == null ? metastoreConfig_ : metastoreConfigBuilder_.build();
to_bitField0_ |= 0x00000400;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.dataprocMetricConfig_ =
dataprocMetricConfigBuilder_ == null
? dataprocMetricConfig_
: dataprocMetricConfigBuilder_.build();
to_bitField0_ |= 0x00000800;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.dataproc.v1.ClusterConfig) {
return mergeFrom((com.google.cloud.dataproc.v1.ClusterConfig) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.dataproc.v1.ClusterConfig other) {
if (other == com.google.cloud.dataproc.v1.ClusterConfig.getDefaultInstance()) return this;
if (!other.getConfigBucket().isEmpty()) {
configBucket_ = other.configBucket_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getTempBucket().isEmpty()) {
tempBucket_ = other.tempBucket_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasGceClusterConfig()) {
mergeGceClusterConfig(other.getGceClusterConfig());
}
if (other.hasMasterConfig()) {
mergeMasterConfig(other.getMasterConfig());
}
if (other.hasWorkerConfig()) {
mergeWorkerConfig(other.getWorkerConfig());
}
if (other.hasSecondaryWorkerConfig()) {
mergeSecondaryWorkerConfig(other.getSecondaryWorkerConfig());
}
if (other.hasSoftwareConfig()) {
mergeSoftwareConfig(other.getSoftwareConfig());
}
if (initializationActionsBuilder_ == null) {
if (!other.initializationActions_.isEmpty()) {
if (initializationActions_.isEmpty()) {
initializationActions_ = other.initializationActions_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureInitializationActionsIsMutable();
initializationActions_.addAll(other.initializationActions_);
}
onChanged();
}
} else {
if (!other.initializationActions_.isEmpty()) {
if (initializationActionsBuilder_.isEmpty()) {
initializationActionsBuilder_.dispose();
initializationActionsBuilder_ = null;
initializationActions_ = other.initializationActions_;
bitField0_ = (bitField0_ & ~0x00000080);
initializationActionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getInitializationActionsFieldBuilder()
: null;
} else {
initializationActionsBuilder_.addAllMessages(other.initializationActions_);
}
}
}
if (other.hasEncryptionConfig()) {
mergeEncryptionConfig(other.getEncryptionConfig());
}
if (other.hasAutoscalingConfig()) {
mergeAutoscalingConfig(other.getAutoscalingConfig());
}
if (other.hasSecurityConfig()) {
mergeSecurityConfig(other.getSecurityConfig());
}
if (other.hasLifecycleConfig()) {
mergeLifecycleConfig(other.getLifecycleConfig());
}
if (other.hasEndpointConfig()) {
mergeEndpointConfig(other.getEndpointConfig());
}
if (other.hasMetastoreConfig()) {
mergeMetastoreConfig(other.getMetastoreConfig());
}
if (other.hasDataprocMetricConfig()) {
mergeDataprocMetricConfig(other.getDataprocMetricConfig());
}
if (auxiliaryNodeGroupsBuilder_ == null) {
if (!other.auxiliaryNodeGroups_.isEmpty()) {
if (auxiliaryNodeGroups_.isEmpty()) {
auxiliaryNodeGroups_ = other.auxiliaryNodeGroups_;
bitField0_ = (bitField0_ & ~0x00008000);
} else {
ensureAuxiliaryNodeGroupsIsMutable();
auxiliaryNodeGroups_.addAll(other.auxiliaryNodeGroups_);
}
onChanged();
}
} else {
if (!other.auxiliaryNodeGroups_.isEmpty()) {
if (auxiliaryNodeGroupsBuilder_.isEmpty()) {
auxiliaryNodeGroupsBuilder_.dispose();
auxiliaryNodeGroupsBuilder_ = null;
auxiliaryNodeGroups_ = other.auxiliaryNodeGroups_;
bitField0_ = (bitField0_ & ~0x00008000);
auxiliaryNodeGroupsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getAuxiliaryNodeGroupsFieldBuilder()
: null;
} else {
auxiliaryNodeGroupsBuilder_.addAllMessages(other.auxiliaryNodeGroups_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
configBucket_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
tempBucket_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 66:
{
input.readMessage(
getGceClusterConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 66
case 74:
{
input.readMessage(getMasterConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 74
case 82:
{
input.readMessage(getWorkerConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 82
case 90:
{
com.google.cloud.dataproc.v1.NodeInitializationAction m =
input.readMessage(
com.google.cloud.dataproc.v1.NodeInitializationAction.parser(),
extensionRegistry);
if (initializationActionsBuilder_ == null) {
ensureInitializationActionsIsMutable();
initializationActions_.add(m);
} else {
initializationActionsBuilder_.addMessage(m);
}
break;
} // case 90
case 98:
{
input.readMessage(
getSecondaryWorkerConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 98
case 106:
{
input.readMessage(getSoftwareConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000040;
break;
} // case 106
case 122:
{
input.readMessage(
getEncryptionConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 122
case 130:
{
input.readMessage(getSecurityConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000400;
break;
} // case 130
case 138:
{
input.readMessage(getLifecycleConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000800;
break;
} // case 138
case 146:
{
input.readMessage(
getAutoscalingConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000200;
break;
} // case 146
case 154:
{
input.readMessage(getEndpointConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00001000;
break;
} // case 154
case 162:
{
input.readMessage(getMetastoreConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00002000;
break;
} // case 162
case 186:
{
input.readMessage(
getDataprocMetricConfigFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00004000;
break;
} // case 186
case 202:
{
com.google.cloud.dataproc.v1.AuxiliaryNodeGroup m =
input.readMessage(
com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.parser(),
extensionRegistry);
if (auxiliaryNodeGroupsBuilder_ == null) {
ensureAuxiliaryNodeGroupsIsMutable();
auxiliaryNodeGroups_.add(m);
} else {
auxiliaryNodeGroupsBuilder_.addMessage(m);
}
break;
} // case 202
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object configBucket_ = "";
/**
*
*
*
* Optional. A Cloud Storage bucket used to stage job
* dependencies, config files, and job driver console output.
* If you do not specify a staging bucket, Cloud
* Dataproc will determine a Cloud Storage location (US,
* ASIA, or EU) for your cluster's staging bucket according to the
* Compute Engine zone where your cluster is deployed, and then create
* and manage this project-level, per-location bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string config_bucket = 1 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The configBucket.
*/
public java.lang.String getConfigBucket() {
java.lang.Object ref = configBucket_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
configBucket_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Optional. A Cloud Storage bucket used to stage job
* dependencies, config files, and job driver console output.
* If you do not specify a staging bucket, Cloud
* Dataproc will determine a Cloud Storage location (US,
* ASIA, or EU) for your cluster's staging bucket according to the
* Compute Engine zone where your cluster is deployed, and then create
* and manage this project-level, per-location bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string config_bucket = 1 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for configBucket.
*/
public com.google.protobuf.ByteString getConfigBucketBytes() {
java.lang.Object ref = configBucket_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
configBucket_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Optional. A Cloud Storage bucket used to stage job
* dependencies, config files, and job driver console output.
* If you do not specify a staging bucket, Cloud
* Dataproc will determine a Cloud Storage location (US,
* ASIA, or EU) for your cluster's staging bucket according to the
* Compute Engine zone where your cluster is deployed, and then create
* and manage this project-level, per-location bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string config_bucket = 1 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The configBucket to set.
* @return This builder for chaining.
*/
public Builder setConfigBucket(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
configBucket_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Optional. A Cloud Storage bucket used to stage job
* dependencies, config files, and job driver console output.
* If you do not specify a staging bucket, Cloud
* Dataproc will determine a Cloud Storage location (US,
* ASIA, or EU) for your cluster's staging bucket according to the
* Compute Engine zone where your cluster is deployed, and then create
* and manage this project-level, per-location bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string config_bucket = 1 [(.google.api.field_behavior) = OPTIONAL];
*
* @return This builder for chaining.
*/
public Builder clearConfigBucket() {
configBucket_ = getDefaultInstance().getConfigBucket();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* Optional. A Cloud Storage bucket used to stage job
* dependencies, config files, and job driver console output.
* If you do not specify a staging bucket, Cloud
* Dataproc will determine a Cloud Storage location (US,
* ASIA, or EU) for your cluster's staging bucket according to the
* Compute Engine zone where your cluster is deployed, and then create
* and manage this project-level, per-location bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string config_bucket = 1 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The bytes for configBucket to set.
* @return This builder for chaining.
*/
public Builder setConfigBucketBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
configBucket_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object tempBucket_ = "";
/**
*
*
*
* Optional. A Cloud Storage bucket used to store ephemeral cluster and jobs
* data, such as Spark and MapReduce history files. If you do not specify a
* temp bucket, Dataproc will determine a Cloud Storage location (US, ASIA, or
* EU) for your cluster's temp bucket according to the Compute Engine zone
* where your cluster is deployed, and then create and manage this
* project-level, per-location bucket. The default bucket has a TTL of 90
* days, but you can use any TTL (or none) if you specify a bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string temp_bucket = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The tempBucket.
*/
public java.lang.String getTempBucket() {
java.lang.Object ref = tempBucket_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tempBucket_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Optional. A Cloud Storage bucket used to store ephemeral cluster and jobs
* data, such as Spark and MapReduce history files. If you do not specify a
* temp bucket, Dataproc will determine a Cloud Storage location (US, ASIA, or
* EU) for your cluster's temp bucket according to the Compute Engine zone
* where your cluster is deployed, and then create and manage this
* project-level, per-location bucket. The default bucket has a TTL of 90
* days, but you can use any TTL (or none) if you specify a bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string temp_bucket = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for tempBucket.
*/
public com.google.protobuf.ByteString getTempBucketBytes() {
java.lang.Object ref = tempBucket_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
tempBucket_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Optional. A Cloud Storage bucket used to store ephemeral cluster and jobs
* data, such as Spark and MapReduce history files. If you do not specify a
* temp bucket, Dataproc will determine a Cloud Storage location (US, ASIA, or
* EU) for your cluster's temp bucket according to the Compute Engine zone
* where your cluster is deployed, and then create and manage this
* project-level, per-location bucket. The default bucket has a TTL of 90
* days, but you can use any TTL (or none) if you specify a bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string temp_bucket = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The tempBucket to set.
* @return This builder for chaining.
*/
public Builder setTempBucket(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tempBucket_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Optional. A Cloud Storage bucket used to store ephemeral cluster and jobs
* data, such as Spark and MapReduce history files. If you do not specify a
* temp bucket, Dataproc will determine a Cloud Storage location (US, ASIA, or
* EU) for your cluster's temp bucket according to the Compute Engine zone
* where your cluster is deployed, and then create and manage this
* project-level, per-location bucket. The default bucket has a TTL of 90
* days, but you can use any TTL (or none) if you specify a bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string temp_bucket = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @return This builder for chaining.
*/
public Builder clearTempBucket() {
tempBucket_ = getDefaultInstance().getTempBucket();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* Optional. A Cloud Storage bucket used to store ephemeral cluster and jobs
* data, such as Spark and MapReduce history files. If you do not specify a
* temp bucket, Dataproc will determine a Cloud Storage location (US, ASIA, or
* EU) for your cluster's temp bucket according to the Compute Engine zone
* where your cluster is deployed, and then create and manage this
* project-level, per-location bucket. The default bucket has a TTL of 90
* days, but you can use any TTL (or none) if you specify a bucket (see
* [Dataproc staging and temp
* buckets](https://cloud.google.com/dataproc/docs/concepts/configuring-clusters/staging-bucket)).
* **This field requires a Cloud Storage bucket name, not a `gs://...` URI to
* a Cloud Storage bucket.**
*
*
* string temp_bucket = 2 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The bytes for tempBucket to set.
* @return This builder for chaining.
*/
public Builder setTempBucketBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tempBucket_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.cloud.dataproc.v1.GceClusterConfig gceClusterConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.GceClusterConfig,
com.google.cloud.dataproc.v1.GceClusterConfig.Builder,
com.google.cloud.dataproc.v1.GceClusterConfigOrBuilder>
gceClusterConfigBuilder_;
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the gceClusterConfig field is set.
*/
public boolean hasGceClusterConfig() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The gceClusterConfig.
*/
public com.google.cloud.dataproc.v1.GceClusterConfig getGceClusterConfig() {
if (gceClusterConfigBuilder_ == null) {
return gceClusterConfig_ == null
? com.google.cloud.dataproc.v1.GceClusterConfig.getDefaultInstance()
: gceClusterConfig_;
} else {
return gceClusterConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setGceClusterConfig(com.google.cloud.dataproc.v1.GceClusterConfig value) {
if (gceClusterConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
gceClusterConfig_ = value;
} else {
gceClusterConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setGceClusterConfig(
com.google.cloud.dataproc.v1.GceClusterConfig.Builder builderForValue) {
if (gceClusterConfigBuilder_ == null) {
gceClusterConfig_ = builderForValue.build();
} else {
gceClusterConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeGceClusterConfig(com.google.cloud.dataproc.v1.GceClusterConfig value) {
if (gceClusterConfigBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)
&& gceClusterConfig_ != null
&& gceClusterConfig_
!= com.google.cloud.dataproc.v1.GceClusterConfig.getDefaultInstance()) {
getGceClusterConfigBuilder().mergeFrom(value);
} else {
gceClusterConfig_ = value;
}
} else {
gceClusterConfigBuilder_.mergeFrom(value);
}
if (gceClusterConfig_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearGceClusterConfig() {
bitField0_ = (bitField0_ & ~0x00000004);
gceClusterConfig_ = null;
if (gceClusterConfigBuilder_ != null) {
gceClusterConfigBuilder_.dispose();
gceClusterConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.GceClusterConfig.Builder getGceClusterConfigBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getGceClusterConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.GceClusterConfigOrBuilder getGceClusterConfigOrBuilder() {
if (gceClusterConfigBuilder_ != null) {
return gceClusterConfigBuilder_.getMessageOrBuilder();
} else {
return gceClusterConfig_ == null
? com.google.cloud.dataproc.v1.GceClusterConfig.getDefaultInstance()
: gceClusterConfig_;
}
}
/**
*
*
*
* Optional. The shared Compute Engine config settings for
* all instances in a cluster.
*
*
*
* .google.cloud.dataproc.v1.GceClusterConfig gce_cluster_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.GceClusterConfig,
com.google.cloud.dataproc.v1.GceClusterConfig.Builder,
com.google.cloud.dataproc.v1.GceClusterConfigOrBuilder>
getGceClusterConfigFieldBuilder() {
if (gceClusterConfigBuilder_ == null) {
gceClusterConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.GceClusterConfig,
com.google.cloud.dataproc.v1.GceClusterConfig.Builder,
com.google.cloud.dataproc.v1.GceClusterConfigOrBuilder>(
getGceClusterConfig(), getParentForChildren(), isClean());
gceClusterConfig_ = null;
}
return gceClusterConfigBuilder_;
}
private com.google.cloud.dataproc.v1.InstanceGroupConfig masterConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.InstanceGroupConfig,
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder,
com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder>
masterConfigBuilder_;
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the masterConfig field is set.
*/
public boolean hasMasterConfig() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The masterConfig.
*/
public com.google.cloud.dataproc.v1.InstanceGroupConfig getMasterConfig() {
if (masterConfigBuilder_ == null) {
return masterConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: masterConfig_;
} else {
return masterConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setMasterConfig(com.google.cloud.dataproc.v1.InstanceGroupConfig value) {
if (masterConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
masterConfig_ = value;
} else {
masterConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setMasterConfig(
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder builderForValue) {
if (masterConfigBuilder_ == null) {
masterConfig_ = builderForValue.build();
} else {
masterConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeMasterConfig(com.google.cloud.dataproc.v1.InstanceGroupConfig value) {
if (masterConfigBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& masterConfig_ != null
&& masterConfig_
!= com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()) {
getMasterConfigBuilder().mergeFrom(value);
} else {
masterConfig_ = value;
}
} else {
masterConfigBuilder_.mergeFrom(value);
}
if (masterConfig_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearMasterConfig() {
bitField0_ = (bitField0_ & ~0x00000008);
masterConfig_ = null;
if (masterConfigBuilder_ != null) {
masterConfigBuilder_.dispose();
masterConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder getMasterConfigBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getMasterConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder getMasterConfigOrBuilder() {
if (masterConfigBuilder_ != null) {
return masterConfigBuilder_.getMessageOrBuilder();
} else {
return masterConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: masterConfig_;
}
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's master instance.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig master_config = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.InstanceGroupConfig,
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder,
com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder>
getMasterConfigFieldBuilder() {
if (masterConfigBuilder_ == null) {
masterConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.InstanceGroupConfig,
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder,
com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder>(
getMasterConfig(), getParentForChildren(), isClean());
masterConfig_ = null;
}
return masterConfigBuilder_;
}
private com.google.cloud.dataproc.v1.InstanceGroupConfig workerConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.InstanceGroupConfig,
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder,
com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder>
workerConfigBuilder_;
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the workerConfig field is set.
*/
public boolean hasWorkerConfig() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The workerConfig.
*/
public com.google.cloud.dataproc.v1.InstanceGroupConfig getWorkerConfig() {
if (workerConfigBuilder_ == null) {
return workerConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: workerConfig_;
} else {
return workerConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setWorkerConfig(com.google.cloud.dataproc.v1.InstanceGroupConfig value) {
if (workerConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
workerConfig_ = value;
} else {
workerConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setWorkerConfig(
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder builderForValue) {
if (workerConfigBuilder_ == null) {
workerConfig_ = builderForValue.build();
} else {
workerConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeWorkerConfig(com.google.cloud.dataproc.v1.InstanceGroupConfig value) {
if (workerConfigBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)
&& workerConfig_ != null
&& workerConfig_
!= com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()) {
getWorkerConfigBuilder().mergeFrom(value);
} else {
workerConfig_ = value;
}
} else {
workerConfigBuilder_.mergeFrom(value);
}
if (workerConfig_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearWorkerConfig() {
bitField0_ = (bitField0_ & ~0x00000010);
workerConfig_ = null;
if (workerConfigBuilder_ != null) {
workerConfigBuilder_.dispose();
workerConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder getWorkerConfigBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getWorkerConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder getWorkerConfigOrBuilder() {
if (workerConfigBuilder_ != null) {
return workerConfigBuilder_.getMessageOrBuilder();
} else {
return workerConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: workerConfig_;
}
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* the cluster's worker instances.
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig worker_config = 10 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.InstanceGroupConfig,
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder,
com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder>
getWorkerConfigFieldBuilder() {
if (workerConfigBuilder_ == null) {
workerConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.InstanceGroupConfig,
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder,
com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder>(
getWorkerConfig(), getParentForChildren(), isClean());
workerConfig_ = null;
}
return workerConfigBuilder_;
}
private com.google.cloud.dataproc.v1.InstanceGroupConfig secondaryWorkerConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.InstanceGroupConfig,
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder,
com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder>
secondaryWorkerConfigBuilder_;
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the secondaryWorkerConfig field is set.
*/
public boolean hasSecondaryWorkerConfig() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The secondaryWorkerConfig.
*/
public com.google.cloud.dataproc.v1.InstanceGroupConfig getSecondaryWorkerConfig() {
if (secondaryWorkerConfigBuilder_ == null) {
return secondaryWorkerConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: secondaryWorkerConfig_;
} else {
return secondaryWorkerConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setSecondaryWorkerConfig(
com.google.cloud.dataproc.v1.InstanceGroupConfig value) {
if (secondaryWorkerConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
secondaryWorkerConfig_ = value;
} else {
secondaryWorkerConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setSecondaryWorkerConfig(
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder builderForValue) {
if (secondaryWorkerConfigBuilder_ == null) {
secondaryWorkerConfig_ = builderForValue.build();
} else {
secondaryWorkerConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeSecondaryWorkerConfig(
com.google.cloud.dataproc.v1.InstanceGroupConfig value) {
if (secondaryWorkerConfigBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)
&& secondaryWorkerConfig_ != null
&& secondaryWorkerConfig_
!= com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()) {
getSecondaryWorkerConfigBuilder().mergeFrom(value);
} else {
secondaryWorkerConfig_ = value;
}
} else {
secondaryWorkerConfigBuilder_.mergeFrom(value);
}
if (secondaryWorkerConfig_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearSecondaryWorkerConfig() {
bitField0_ = (bitField0_ & ~0x00000020);
secondaryWorkerConfig_ = null;
if (secondaryWorkerConfigBuilder_ != null) {
secondaryWorkerConfigBuilder_.dispose();
secondaryWorkerConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder
getSecondaryWorkerConfigBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getSecondaryWorkerConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder
getSecondaryWorkerConfigOrBuilder() {
if (secondaryWorkerConfigBuilder_ != null) {
return secondaryWorkerConfigBuilder_.getMessageOrBuilder();
} else {
return secondaryWorkerConfig_ == null
? com.google.cloud.dataproc.v1.InstanceGroupConfig.getDefaultInstance()
: secondaryWorkerConfig_;
}
}
/**
*
*
*
* Optional. The Compute Engine config settings for
* a cluster's secondary worker instances
*
*
*
* .google.cloud.dataproc.v1.InstanceGroupConfig secondary_worker_config = 12 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.InstanceGroupConfig,
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder,
com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder>
getSecondaryWorkerConfigFieldBuilder() {
if (secondaryWorkerConfigBuilder_ == null) {
secondaryWorkerConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.InstanceGroupConfig,
com.google.cloud.dataproc.v1.InstanceGroupConfig.Builder,
com.google.cloud.dataproc.v1.InstanceGroupConfigOrBuilder>(
getSecondaryWorkerConfig(), getParentForChildren(), isClean());
secondaryWorkerConfig_ = null;
}
return secondaryWorkerConfigBuilder_;
}
private com.google.cloud.dataproc.v1.SoftwareConfig softwareConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.SoftwareConfig,
com.google.cloud.dataproc.v1.SoftwareConfig.Builder,
com.google.cloud.dataproc.v1.SoftwareConfigOrBuilder>
softwareConfigBuilder_;
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the softwareConfig field is set.
*/
public boolean hasSoftwareConfig() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The softwareConfig.
*/
public com.google.cloud.dataproc.v1.SoftwareConfig getSoftwareConfig() {
if (softwareConfigBuilder_ == null) {
return softwareConfig_ == null
? com.google.cloud.dataproc.v1.SoftwareConfig.getDefaultInstance()
: softwareConfig_;
} else {
return softwareConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setSoftwareConfig(com.google.cloud.dataproc.v1.SoftwareConfig value) {
if (softwareConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
softwareConfig_ = value;
} else {
softwareConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setSoftwareConfig(
com.google.cloud.dataproc.v1.SoftwareConfig.Builder builderForValue) {
if (softwareConfigBuilder_ == null) {
softwareConfig_ = builderForValue.build();
} else {
softwareConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeSoftwareConfig(com.google.cloud.dataproc.v1.SoftwareConfig value) {
if (softwareConfigBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)
&& softwareConfig_ != null
&& softwareConfig_
!= com.google.cloud.dataproc.v1.SoftwareConfig.getDefaultInstance()) {
getSoftwareConfigBuilder().mergeFrom(value);
} else {
softwareConfig_ = value;
}
} else {
softwareConfigBuilder_.mergeFrom(value);
}
if (softwareConfig_ != null) {
bitField0_ |= 0x00000040;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearSoftwareConfig() {
bitField0_ = (bitField0_ & ~0x00000040);
softwareConfig_ = null;
if (softwareConfigBuilder_ != null) {
softwareConfigBuilder_.dispose();
softwareConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.SoftwareConfig.Builder getSoftwareConfigBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getSoftwareConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.SoftwareConfigOrBuilder getSoftwareConfigOrBuilder() {
if (softwareConfigBuilder_ != null) {
return softwareConfigBuilder_.getMessageOrBuilder();
} else {
return softwareConfig_ == null
? com.google.cloud.dataproc.v1.SoftwareConfig.getDefaultInstance()
: softwareConfig_;
}
}
/**
*
*
*
* Optional. The config settings for cluster software.
*
*
*
* .google.cloud.dataproc.v1.SoftwareConfig software_config = 13 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.SoftwareConfig,
com.google.cloud.dataproc.v1.SoftwareConfig.Builder,
com.google.cloud.dataproc.v1.SoftwareConfigOrBuilder>
getSoftwareConfigFieldBuilder() {
if (softwareConfigBuilder_ == null) {
softwareConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.SoftwareConfig,
com.google.cloud.dataproc.v1.SoftwareConfig.Builder,
com.google.cloud.dataproc.v1.SoftwareConfigOrBuilder>(
getSoftwareConfig(), getParentForChildren(), isClean());
softwareConfig_ = null;
}
return softwareConfigBuilder_;
}
private java.util.List
initializationActions_ = java.util.Collections.emptyList();
private void ensureInitializationActionsIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
initializationActions_ =
new java.util.ArrayList(
initializationActions_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dataproc.v1.NodeInitializationAction,
com.google.cloud.dataproc.v1.NodeInitializationAction.Builder,
com.google.cloud.dataproc.v1.NodeInitializationActionOrBuilder>
initializationActionsBuilder_;
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List
getInitializationActionsList() {
if (initializationActionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(initializationActions_);
} else {
return initializationActionsBuilder_.getMessageList();
}
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public int getInitializationActionsCount() {
if (initializationActionsBuilder_ == null) {
return initializationActions_.size();
} else {
return initializationActionsBuilder_.getCount();
}
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.NodeInitializationAction getInitializationActions(
int index) {
if (initializationActionsBuilder_ == null) {
return initializationActions_.get(index);
} else {
return initializationActionsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setInitializationActions(
int index, com.google.cloud.dataproc.v1.NodeInitializationAction value) {
if (initializationActionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInitializationActionsIsMutable();
initializationActions_.set(index, value);
onChanged();
} else {
initializationActionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setInitializationActions(
int index, com.google.cloud.dataproc.v1.NodeInitializationAction.Builder builderForValue) {
if (initializationActionsBuilder_ == null) {
ensureInitializationActionsIsMutable();
initializationActions_.set(index, builderForValue.build());
onChanged();
} else {
initializationActionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addInitializationActions(
com.google.cloud.dataproc.v1.NodeInitializationAction value) {
if (initializationActionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInitializationActionsIsMutable();
initializationActions_.add(value);
onChanged();
} else {
initializationActionsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addInitializationActions(
int index, com.google.cloud.dataproc.v1.NodeInitializationAction value) {
if (initializationActionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureInitializationActionsIsMutable();
initializationActions_.add(index, value);
onChanged();
} else {
initializationActionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addInitializationActions(
com.google.cloud.dataproc.v1.NodeInitializationAction.Builder builderForValue) {
if (initializationActionsBuilder_ == null) {
ensureInitializationActionsIsMutable();
initializationActions_.add(builderForValue.build());
onChanged();
} else {
initializationActionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addInitializationActions(
int index, com.google.cloud.dataproc.v1.NodeInitializationAction.Builder builderForValue) {
if (initializationActionsBuilder_ == null) {
ensureInitializationActionsIsMutable();
initializationActions_.add(index, builderForValue.build());
onChanged();
} else {
initializationActionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addAllInitializationActions(
java.lang.Iterable extends com.google.cloud.dataproc.v1.NodeInitializationAction>
values) {
if (initializationActionsBuilder_ == null) {
ensureInitializationActionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, initializationActions_);
onChanged();
} else {
initializationActionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearInitializationActions() {
if (initializationActionsBuilder_ == null) {
initializationActions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
initializationActionsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder removeInitializationActions(int index) {
if (initializationActionsBuilder_ == null) {
ensureInitializationActionsIsMutable();
initializationActions_.remove(index);
onChanged();
} else {
initializationActionsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.NodeInitializationAction.Builder
getInitializationActionsBuilder(int index) {
return getInitializationActionsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.NodeInitializationActionOrBuilder
getInitializationActionsOrBuilder(int index) {
if (initializationActionsBuilder_ == null) {
return initializationActions_.get(index);
} else {
return initializationActionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List extends com.google.cloud.dataproc.v1.NodeInitializationActionOrBuilder>
getInitializationActionsOrBuilderList() {
if (initializationActionsBuilder_ != null) {
return initializationActionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(initializationActions_);
}
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.NodeInitializationAction.Builder
addInitializationActionsBuilder() {
return getInitializationActionsFieldBuilder()
.addBuilder(com.google.cloud.dataproc.v1.NodeInitializationAction.getDefaultInstance());
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.NodeInitializationAction.Builder
addInitializationActionsBuilder(int index) {
return getInitializationActionsFieldBuilder()
.addBuilder(
index, com.google.cloud.dataproc.v1.NodeInitializationAction.getDefaultInstance());
}
/**
*
*
*
* Optional. Commands to execute on each node after config is
* completed. By default, executables are run on master and all worker nodes.
* You can test a node's `role` metadata to run an executable on
* a master or worker node, as shown below using `curl` (you can also use
* `wget`):
*
* ROLE=$(curl -H Metadata-Flavor:Google
* http://metadata/computeMetadata/v1/instance/attributes/dataproc-role)
* if [[ "${ROLE}" == 'Master' ]]; then
* ... master specific actions ...
* else
* ... worker specific actions ...
* fi
*
*
*
* repeated .google.cloud.dataproc.v1.NodeInitializationAction initialization_actions = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List
getInitializationActionsBuilderList() {
return getInitializationActionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dataproc.v1.NodeInitializationAction,
com.google.cloud.dataproc.v1.NodeInitializationAction.Builder,
com.google.cloud.dataproc.v1.NodeInitializationActionOrBuilder>
getInitializationActionsFieldBuilder() {
if (initializationActionsBuilder_ == null) {
initializationActionsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dataproc.v1.NodeInitializationAction,
com.google.cloud.dataproc.v1.NodeInitializationAction.Builder,
com.google.cloud.dataproc.v1.NodeInitializationActionOrBuilder>(
initializationActions_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
initializationActions_ = null;
}
return initializationActionsBuilder_;
}
private com.google.cloud.dataproc.v1.EncryptionConfig encryptionConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.EncryptionConfig,
com.google.cloud.dataproc.v1.EncryptionConfig.Builder,
com.google.cloud.dataproc.v1.EncryptionConfigOrBuilder>
encryptionConfigBuilder_;
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the encryptionConfig field is set.
*/
public boolean hasEncryptionConfig() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The encryptionConfig.
*/
public com.google.cloud.dataproc.v1.EncryptionConfig getEncryptionConfig() {
if (encryptionConfigBuilder_ == null) {
return encryptionConfig_ == null
? com.google.cloud.dataproc.v1.EncryptionConfig.getDefaultInstance()
: encryptionConfig_;
} else {
return encryptionConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setEncryptionConfig(com.google.cloud.dataproc.v1.EncryptionConfig value) {
if (encryptionConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
encryptionConfig_ = value;
} else {
encryptionConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setEncryptionConfig(
com.google.cloud.dataproc.v1.EncryptionConfig.Builder builderForValue) {
if (encryptionConfigBuilder_ == null) {
encryptionConfig_ = builderForValue.build();
} else {
encryptionConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeEncryptionConfig(com.google.cloud.dataproc.v1.EncryptionConfig value) {
if (encryptionConfigBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)
&& encryptionConfig_ != null
&& encryptionConfig_
!= com.google.cloud.dataproc.v1.EncryptionConfig.getDefaultInstance()) {
getEncryptionConfigBuilder().mergeFrom(value);
} else {
encryptionConfig_ = value;
}
} else {
encryptionConfigBuilder_.mergeFrom(value);
}
if (encryptionConfig_ != null) {
bitField0_ |= 0x00000100;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearEncryptionConfig() {
bitField0_ = (bitField0_ & ~0x00000100);
encryptionConfig_ = null;
if (encryptionConfigBuilder_ != null) {
encryptionConfigBuilder_.dispose();
encryptionConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.EncryptionConfig.Builder getEncryptionConfigBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getEncryptionConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.EncryptionConfigOrBuilder getEncryptionConfigOrBuilder() {
if (encryptionConfigBuilder_ != null) {
return encryptionConfigBuilder_.getMessageOrBuilder();
} else {
return encryptionConfig_ == null
? com.google.cloud.dataproc.v1.EncryptionConfig.getDefaultInstance()
: encryptionConfig_;
}
}
/**
*
*
*
* Optional. Encryption settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.EncryptionConfig encryption_config = 15 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.EncryptionConfig,
com.google.cloud.dataproc.v1.EncryptionConfig.Builder,
com.google.cloud.dataproc.v1.EncryptionConfigOrBuilder>
getEncryptionConfigFieldBuilder() {
if (encryptionConfigBuilder_ == null) {
encryptionConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.EncryptionConfig,
com.google.cloud.dataproc.v1.EncryptionConfig.Builder,
com.google.cloud.dataproc.v1.EncryptionConfigOrBuilder>(
getEncryptionConfig(), getParentForChildren(), isClean());
encryptionConfig_ = null;
}
return encryptionConfigBuilder_;
}
private com.google.cloud.dataproc.v1.AutoscalingConfig autoscalingConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.AutoscalingConfig,
com.google.cloud.dataproc.v1.AutoscalingConfig.Builder,
com.google.cloud.dataproc.v1.AutoscalingConfigOrBuilder>
autoscalingConfigBuilder_;
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the autoscalingConfig field is set.
*/
public boolean hasAutoscalingConfig() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The autoscalingConfig.
*/
public com.google.cloud.dataproc.v1.AutoscalingConfig getAutoscalingConfig() {
if (autoscalingConfigBuilder_ == null) {
return autoscalingConfig_ == null
? com.google.cloud.dataproc.v1.AutoscalingConfig.getDefaultInstance()
: autoscalingConfig_;
} else {
return autoscalingConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setAutoscalingConfig(com.google.cloud.dataproc.v1.AutoscalingConfig value) {
if (autoscalingConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
autoscalingConfig_ = value;
} else {
autoscalingConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setAutoscalingConfig(
com.google.cloud.dataproc.v1.AutoscalingConfig.Builder builderForValue) {
if (autoscalingConfigBuilder_ == null) {
autoscalingConfig_ = builderForValue.build();
} else {
autoscalingConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeAutoscalingConfig(com.google.cloud.dataproc.v1.AutoscalingConfig value) {
if (autoscalingConfigBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)
&& autoscalingConfig_ != null
&& autoscalingConfig_
!= com.google.cloud.dataproc.v1.AutoscalingConfig.getDefaultInstance()) {
getAutoscalingConfigBuilder().mergeFrom(value);
} else {
autoscalingConfig_ = value;
}
} else {
autoscalingConfigBuilder_.mergeFrom(value);
}
if (autoscalingConfig_ != null) {
bitField0_ |= 0x00000200;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearAutoscalingConfig() {
bitField0_ = (bitField0_ & ~0x00000200);
autoscalingConfig_ = null;
if (autoscalingConfigBuilder_ != null) {
autoscalingConfigBuilder_.dispose();
autoscalingConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.AutoscalingConfig.Builder getAutoscalingConfigBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getAutoscalingConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.AutoscalingConfigOrBuilder getAutoscalingConfigOrBuilder() {
if (autoscalingConfigBuilder_ != null) {
return autoscalingConfigBuilder_.getMessageOrBuilder();
} else {
return autoscalingConfig_ == null
? com.google.cloud.dataproc.v1.AutoscalingConfig.getDefaultInstance()
: autoscalingConfig_;
}
}
/**
*
*
*
* Optional. Autoscaling config for the policy associated with the cluster.
* Cluster does not autoscale if this field is unset.
*
*
*
* .google.cloud.dataproc.v1.AutoscalingConfig autoscaling_config = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.AutoscalingConfig,
com.google.cloud.dataproc.v1.AutoscalingConfig.Builder,
com.google.cloud.dataproc.v1.AutoscalingConfigOrBuilder>
getAutoscalingConfigFieldBuilder() {
if (autoscalingConfigBuilder_ == null) {
autoscalingConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.AutoscalingConfig,
com.google.cloud.dataproc.v1.AutoscalingConfig.Builder,
com.google.cloud.dataproc.v1.AutoscalingConfigOrBuilder>(
getAutoscalingConfig(), getParentForChildren(), isClean());
autoscalingConfig_ = null;
}
return autoscalingConfigBuilder_;
}
private com.google.cloud.dataproc.v1.SecurityConfig securityConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.SecurityConfig,
com.google.cloud.dataproc.v1.SecurityConfig.Builder,
com.google.cloud.dataproc.v1.SecurityConfigOrBuilder>
securityConfigBuilder_;
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the securityConfig field is set.
*/
public boolean hasSecurityConfig() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The securityConfig.
*/
public com.google.cloud.dataproc.v1.SecurityConfig getSecurityConfig() {
if (securityConfigBuilder_ == null) {
return securityConfig_ == null
? com.google.cloud.dataproc.v1.SecurityConfig.getDefaultInstance()
: securityConfig_;
} else {
return securityConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setSecurityConfig(com.google.cloud.dataproc.v1.SecurityConfig value) {
if (securityConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
securityConfig_ = value;
} else {
securityConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setSecurityConfig(
com.google.cloud.dataproc.v1.SecurityConfig.Builder builderForValue) {
if (securityConfigBuilder_ == null) {
securityConfig_ = builderForValue.build();
} else {
securityConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeSecurityConfig(com.google.cloud.dataproc.v1.SecurityConfig value) {
if (securityConfigBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0)
&& securityConfig_ != null
&& securityConfig_
!= com.google.cloud.dataproc.v1.SecurityConfig.getDefaultInstance()) {
getSecurityConfigBuilder().mergeFrom(value);
} else {
securityConfig_ = value;
}
} else {
securityConfigBuilder_.mergeFrom(value);
}
if (securityConfig_ != null) {
bitField0_ |= 0x00000400;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearSecurityConfig() {
bitField0_ = (bitField0_ & ~0x00000400);
securityConfig_ = null;
if (securityConfigBuilder_ != null) {
securityConfigBuilder_.dispose();
securityConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.SecurityConfig.Builder getSecurityConfigBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getSecurityConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.SecurityConfigOrBuilder getSecurityConfigOrBuilder() {
if (securityConfigBuilder_ != null) {
return securityConfigBuilder_.getMessageOrBuilder();
} else {
return securityConfig_ == null
? com.google.cloud.dataproc.v1.SecurityConfig.getDefaultInstance()
: securityConfig_;
}
}
/**
*
*
*
* Optional. Security settings for the cluster.
*
*
*
* .google.cloud.dataproc.v1.SecurityConfig security_config = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.SecurityConfig,
com.google.cloud.dataproc.v1.SecurityConfig.Builder,
com.google.cloud.dataproc.v1.SecurityConfigOrBuilder>
getSecurityConfigFieldBuilder() {
if (securityConfigBuilder_ == null) {
securityConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.SecurityConfig,
com.google.cloud.dataproc.v1.SecurityConfig.Builder,
com.google.cloud.dataproc.v1.SecurityConfigOrBuilder>(
getSecurityConfig(), getParentForChildren(), isClean());
securityConfig_ = null;
}
return securityConfigBuilder_;
}
private com.google.cloud.dataproc.v1.LifecycleConfig lifecycleConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.LifecycleConfig,
com.google.cloud.dataproc.v1.LifecycleConfig.Builder,
com.google.cloud.dataproc.v1.LifecycleConfigOrBuilder>
lifecycleConfigBuilder_;
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the lifecycleConfig field is set.
*/
public boolean hasLifecycleConfig() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The lifecycleConfig.
*/
public com.google.cloud.dataproc.v1.LifecycleConfig getLifecycleConfig() {
if (lifecycleConfigBuilder_ == null) {
return lifecycleConfig_ == null
? com.google.cloud.dataproc.v1.LifecycleConfig.getDefaultInstance()
: lifecycleConfig_;
} else {
return lifecycleConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setLifecycleConfig(com.google.cloud.dataproc.v1.LifecycleConfig value) {
if (lifecycleConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lifecycleConfig_ = value;
} else {
lifecycleConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setLifecycleConfig(
com.google.cloud.dataproc.v1.LifecycleConfig.Builder builderForValue) {
if (lifecycleConfigBuilder_ == null) {
lifecycleConfig_ = builderForValue.build();
} else {
lifecycleConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeLifecycleConfig(com.google.cloud.dataproc.v1.LifecycleConfig value) {
if (lifecycleConfigBuilder_ == null) {
if (((bitField0_ & 0x00000800) != 0)
&& lifecycleConfig_ != null
&& lifecycleConfig_
!= com.google.cloud.dataproc.v1.LifecycleConfig.getDefaultInstance()) {
getLifecycleConfigBuilder().mergeFrom(value);
} else {
lifecycleConfig_ = value;
}
} else {
lifecycleConfigBuilder_.mergeFrom(value);
}
if (lifecycleConfig_ != null) {
bitField0_ |= 0x00000800;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearLifecycleConfig() {
bitField0_ = (bitField0_ & ~0x00000800);
lifecycleConfig_ = null;
if (lifecycleConfigBuilder_ != null) {
lifecycleConfigBuilder_.dispose();
lifecycleConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.LifecycleConfig.Builder getLifecycleConfigBuilder() {
bitField0_ |= 0x00000800;
onChanged();
return getLifecycleConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.LifecycleConfigOrBuilder getLifecycleConfigOrBuilder() {
if (lifecycleConfigBuilder_ != null) {
return lifecycleConfigBuilder_.getMessageOrBuilder();
} else {
return lifecycleConfig_ == null
? com.google.cloud.dataproc.v1.LifecycleConfig.getDefaultInstance()
: lifecycleConfig_;
}
}
/**
*
*
*
* Optional. Lifecycle setting for the cluster.
*
*
*
* .google.cloud.dataproc.v1.LifecycleConfig lifecycle_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.LifecycleConfig,
com.google.cloud.dataproc.v1.LifecycleConfig.Builder,
com.google.cloud.dataproc.v1.LifecycleConfigOrBuilder>
getLifecycleConfigFieldBuilder() {
if (lifecycleConfigBuilder_ == null) {
lifecycleConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.LifecycleConfig,
com.google.cloud.dataproc.v1.LifecycleConfig.Builder,
com.google.cloud.dataproc.v1.LifecycleConfigOrBuilder>(
getLifecycleConfig(), getParentForChildren(), isClean());
lifecycleConfig_ = null;
}
return lifecycleConfigBuilder_;
}
private com.google.cloud.dataproc.v1.EndpointConfig endpointConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.EndpointConfig,
com.google.cloud.dataproc.v1.EndpointConfig.Builder,
com.google.cloud.dataproc.v1.EndpointConfigOrBuilder>
endpointConfigBuilder_;
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the endpointConfig field is set.
*/
public boolean hasEndpointConfig() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The endpointConfig.
*/
public com.google.cloud.dataproc.v1.EndpointConfig getEndpointConfig() {
if (endpointConfigBuilder_ == null) {
return endpointConfig_ == null
? com.google.cloud.dataproc.v1.EndpointConfig.getDefaultInstance()
: endpointConfig_;
} else {
return endpointConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setEndpointConfig(com.google.cloud.dataproc.v1.EndpointConfig value) {
if (endpointConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
endpointConfig_ = value;
} else {
endpointConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setEndpointConfig(
com.google.cloud.dataproc.v1.EndpointConfig.Builder builderForValue) {
if (endpointConfigBuilder_ == null) {
endpointConfig_ = builderForValue.build();
} else {
endpointConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeEndpointConfig(com.google.cloud.dataproc.v1.EndpointConfig value) {
if (endpointConfigBuilder_ == null) {
if (((bitField0_ & 0x00001000) != 0)
&& endpointConfig_ != null
&& endpointConfig_
!= com.google.cloud.dataproc.v1.EndpointConfig.getDefaultInstance()) {
getEndpointConfigBuilder().mergeFrom(value);
} else {
endpointConfig_ = value;
}
} else {
endpointConfigBuilder_.mergeFrom(value);
}
if (endpointConfig_ != null) {
bitField0_ |= 0x00001000;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearEndpointConfig() {
bitField0_ = (bitField0_ & ~0x00001000);
endpointConfig_ = null;
if (endpointConfigBuilder_ != null) {
endpointConfigBuilder_.dispose();
endpointConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.EndpointConfig.Builder getEndpointConfigBuilder() {
bitField0_ |= 0x00001000;
onChanged();
return getEndpointConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.EndpointConfigOrBuilder getEndpointConfigOrBuilder() {
if (endpointConfigBuilder_ != null) {
return endpointConfigBuilder_.getMessageOrBuilder();
} else {
return endpointConfig_ == null
? com.google.cloud.dataproc.v1.EndpointConfig.getDefaultInstance()
: endpointConfig_;
}
}
/**
*
*
*
* Optional. Port/endpoint configuration for this cluster
*
*
*
* .google.cloud.dataproc.v1.EndpointConfig endpoint_config = 19 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.EndpointConfig,
com.google.cloud.dataproc.v1.EndpointConfig.Builder,
com.google.cloud.dataproc.v1.EndpointConfigOrBuilder>
getEndpointConfigFieldBuilder() {
if (endpointConfigBuilder_ == null) {
endpointConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.EndpointConfig,
com.google.cloud.dataproc.v1.EndpointConfig.Builder,
com.google.cloud.dataproc.v1.EndpointConfigOrBuilder>(
getEndpointConfig(), getParentForChildren(), isClean());
endpointConfig_ = null;
}
return endpointConfigBuilder_;
}
private com.google.cloud.dataproc.v1.MetastoreConfig metastoreConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.MetastoreConfig,
com.google.cloud.dataproc.v1.MetastoreConfig.Builder,
com.google.cloud.dataproc.v1.MetastoreConfigOrBuilder>
metastoreConfigBuilder_;
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the metastoreConfig field is set.
*/
public boolean hasMetastoreConfig() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The metastoreConfig.
*/
public com.google.cloud.dataproc.v1.MetastoreConfig getMetastoreConfig() {
if (metastoreConfigBuilder_ == null) {
return metastoreConfig_ == null
? com.google.cloud.dataproc.v1.MetastoreConfig.getDefaultInstance()
: metastoreConfig_;
} else {
return metastoreConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setMetastoreConfig(com.google.cloud.dataproc.v1.MetastoreConfig value) {
if (metastoreConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
metastoreConfig_ = value;
} else {
metastoreConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setMetastoreConfig(
com.google.cloud.dataproc.v1.MetastoreConfig.Builder builderForValue) {
if (metastoreConfigBuilder_ == null) {
metastoreConfig_ = builderForValue.build();
} else {
metastoreConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeMetastoreConfig(com.google.cloud.dataproc.v1.MetastoreConfig value) {
if (metastoreConfigBuilder_ == null) {
if (((bitField0_ & 0x00002000) != 0)
&& metastoreConfig_ != null
&& metastoreConfig_
!= com.google.cloud.dataproc.v1.MetastoreConfig.getDefaultInstance()) {
getMetastoreConfigBuilder().mergeFrom(value);
} else {
metastoreConfig_ = value;
}
} else {
metastoreConfigBuilder_.mergeFrom(value);
}
if (metastoreConfig_ != null) {
bitField0_ |= 0x00002000;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearMetastoreConfig() {
bitField0_ = (bitField0_ & ~0x00002000);
metastoreConfig_ = null;
if (metastoreConfigBuilder_ != null) {
metastoreConfigBuilder_.dispose();
metastoreConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.MetastoreConfig.Builder getMetastoreConfigBuilder() {
bitField0_ |= 0x00002000;
onChanged();
return getMetastoreConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.MetastoreConfigOrBuilder getMetastoreConfigOrBuilder() {
if (metastoreConfigBuilder_ != null) {
return metastoreConfigBuilder_.getMessageOrBuilder();
} else {
return metastoreConfig_ == null
? com.google.cloud.dataproc.v1.MetastoreConfig.getDefaultInstance()
: metastoreConfig_;
}
}
/**
*
*
*
* Optional. Metastore configuration.
*
*
*
* .google.cloud.dataproc.v1.MetastoreConfig metastore_config = 20 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.MetastoreConfig,
com.google.cloud.dataproc.v1.MetastoreConfig.Builder,
com.google.cloud.dataproc.v1.MetastoreConfigOrBuilder>
getMetastoreConfigFieldBuilder() {
if (metastoreConfigBuilder_ == null) {
metastoreConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.MetastoreConfig,
com.google.cloud.dataproc.v1.MetastoreConfig.Builder,
com.google.cloud.dataproc.v1.MetastoreConfigOrBuilder>(
getMetastoreConfig(), getParentForChildren(), isClean());
metastoreConfig_ = null;
}
return metastoreConfigBuilder_;
}
private com.google.cloud.dataproc.v1.DataprocMetricConfig dataprocMetricConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.DataprocMetricConfig,
com.google.cloud.dataproc.v1.DataprocMetricConfig.Builder,
com.google.cloud.dataproc.v1.DataprocMetricConfigOrBuilder>
dataprocMetricConfigBuilder_;
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the dataprocMetricConfig field is set.
*/
public boolean hasDataprocMetricConfig() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The dataprocMetricConfig.
*/
public com.google.cloud.dataproc.v1.DataprocMetricConfig getDataprocMetricConfig() {
if (dataprocMetricConfigBuilder_ == null) {
return dataprocMetricConfig_ == null
? com.google.cloud.dataproc.v1.DataprocMetricConfig.getDefaultInstance()
: dataprocMetricConfig_;
} else {
return dataprocMetricConfigBuilder_.getMessage();
}
}
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setDataprocMetricConfig(
com.google.cloud.dataproc.v1.DataprocMetricConfig value) {
if (dataprocMetricConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dataprocMetricConfig_ = value;
} else {
dataprocMetricConfigBuilder_.setMessage(value);
}
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setDataprocMetricConfig(
com.google.cloud.dataproc.v1.DataprocMetricConfig.Builder builderForValue) {
if (dataprocMetricConfigBuilder_ == null) {
dataprocMetricConfig_ = builderForValue.build();
} else {
dataprocMetricConfigBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeDataprocMetricConfig(
com.google.cloud.dataproc.v1.DataprocMetricConfig value) {
if (dataprocMetricConfigBuilder_ == null) {
if (((bitField0_ & 0x00004000) != 0)
&& dataprocMetricConfig_ != null
&& dataprocMetricConfig_
!= com.google.cloud.dataproc.v1.DataprocMetricConfig.getDefaultInstance()) {
getDataprocMetricConfigBuilder().mergeFrom(value);
} else {
dataprocMetricConfig_ = value;
}
} else {
dataprocMetricConfigBuilder_.mergeFrom(value);
}
if (dataprocMetricConfig_ != null) {
bitField0_ |= 0x00004000;
onChanged();
}
return this;
}
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearDataprocMetricConfig() {
bitField0_ = (bitField0_ & ~0x00004000);
dataprocMetricConfig_ = null;
if (dataprocMetricConfigBuilder_ != null) {
dataprocMetricConfigBuilder_.dispose();
dataprocMetricConfigBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.DataprocMetricConfig.Builder
getDataprocMetricConfigBuilder() {
bitField0_ |= 0x00004000;
onChanged();
return getDataprocMetricConfigFieldBuilder().getBuilder();
}
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.DataprocMetricConfigOrBuilder
getDataprocMetricConfigOrBuilder() {
if (dataprocMetricConfigBuilder_ != null) {
return dataprocMetricConfigBuilder_.getMessageOrBuilder();
} else {
return dataprocMetricConfig_ == null
? com.google.cloud.dataproc.v1.DataprocMetricConfig.getDefaultInstance()
: dataprocMetricConfig_;
}
}
/**
*
*
*
* Optional. The config for Dataproc metrics.
*
*
*
* .google.cloud.dataproc.v1.DataprocMetricConfig dataproc_metric_config = 23 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.DataprocMetricConfig,
com.google.cloud.dataproc.v1.DataprocMetricConfig.Builder,
com.google.cloud.dataproc.v1.DataprocMetricConfigOrBuilder>
getDataprocMetricConfigFieldBuilder() {
if (dataprocMetricConfigBuilder_ == null) {
dataprocMetricConfigBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dataproc.v1.DataprocMetricConfig,
com.google.cloud.dataproc.v1.DataprocMetricConfig.Builder,
com.google.cloud.dataproc.v1.DataprocMetricConfigOrBuilder>(
getDataprocMetricConfig(), getParentForChildren(), isClean());
dataprocMetricConfig_ = null;
}
return dataprocMetricConfigBuilder_;
}
private java.util.List auxiliaryNodeGroups_ =
java.util.Collections.emptyList();
private void ensureAuxiliaryNodeGroupsIsMutable() {
if (!((bitField0_ & 0x00008000) != 0)) {
auxiliaryNodeGroups_ =
new java.util.ArrayList(
auxiliaryNodeGroups_);
bitField0_ |= 0x00008000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dataproc.v1.AuxiliaryNodeGroup,
com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.Builder,
com.google.cloud.dataproc.v1.AuxiliaryNodeGroupOrBuilder>
auxiliaryNodeGroupsBuilder_;
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List
getAuxiliaryNodeGroupsList() {
if (auxiliaryNodeGroupsBuilder_ == null) {
return java.util.Collections.unmodifiableList(auxiliaryNodeGroups_);
} else {
return auxiliaryNodeGroupsBuilder_.getMessageList();
}
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public int getAuxiliaryNodeGroupsCount() {
if (auxiliaryNodeGroupsBuilder_ == null) {
return auxiliaryNodeGroups_.size();
} else {
return auxiliaryNodeGroupsBuilder_.getCount();
}
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.AuxiliaryNodeGroup getAuxiliaryNodeGroups(int index) {
if (auxiliaryNodeGroupsBuilder_ == null) {
return auxiliaryNodeGroups_.get(index);
} else {
return auxiliaryNodeGroupsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setAuxiliaryNodeGroups(
int index, com.google.cloud.dataproc.v1.AuxiliaryNodeGroup value) {
if (auxiliaryNodeGroupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAuxiliaryNodeGroupsIsMutable();
auxiliaryNodeGroups_.set(index, value);
onChanged();
} else {
auxiliaryNodeGroupsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setAuxiliaryNodeGroups(
int index, com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.Builder builderForValue) {
if (auxiliaryNodeGroupsBuilder_ == null) {
ensureAuxiliaryNodeGroupsIsMutable();
auxiliaryNodeGroups_.set(index, builderForValue.build());
onChanged();
} else {
auxiliaryNodeGroupsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addAuxiliaryNodeGroups(com.google.cloud.dataproc.v1.AuxiliaryNodeGroup value) {
if (auxiliaryNodeGroupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAuxiliaryNodeGroupsIsMutable();
auxiliaryNodeGroups_.add(value);
onChanged();
} else {
auxiliaryNodeGroupsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addAuxiliaryNodeGroups(
int index, com.google.cloud.dataproc.v1.AuxiliaryNodeGroup value) {
if (auxiliaryNodeGroupsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAuxiliaryNodeGroupsIsMutable();
auxiliaryNodeGroups_.add(index, value);
onChanged();
} else {
auxiliaryNodeGroupsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addAuxiliaryNodeGroups(
com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.Builder builderForValue) {
if (auxiliaryNodeGroupsBuilder_ == null) {
ensureAuxiliaryNodeGroupsIsMutable();
auxiliaryNodeGroups_.add(builderForValue.build());
onChanged();
} else {
auxiliaryNodeGroupsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addAuxiliaryNodeGroups(
int index, com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.Builder builderForValue) {
if (auxiliaryNodeGroupsBuilder_ == null) {
ensureAuxiliaryNodeGroupsIsMutable();
auxiliaryNodeGroups_.add(index, builderForValue.build());
onChanged();
} else {
auxiliaryNodeGroupsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder addAllAuxiliaryNodeGroups(
java.lang.Iterable extends com.google.cloud.dataproc.v1.AuxiliaryNodeGroup> values) {
if (auxiliaryNodeGroupsBuilder_ == null) {
ensureAuxiliaryNodeGroupsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, auxiliaryNodeGroups_);
onChanged();
} else {
auxiliaryNodeGroupsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearAuxiliaryNodeGroups() {
if (auxiliaryNodeGroupsBuilder_ == null) {
auxiliaryNodeGroups_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00008000);
onChanged();
} else {
auxiliaryNodeGroupsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder removeAuxiliaryNodeGroups(int index) {
if (auxiliaryNodeGroupsBuilder_ == null) {
ensureAuxiliaryNodeGroupsIsMutable();
auxiliaryNodeGroups_.remove(index);
onChanged();
} else {
auxiliaryNodeGroupsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.Builder getAuxiliaryNodeGroupsBuilder(
int index) {
return getAuxiliaryNodeGroupsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.AuxiliaryNodeGroupOrBuilder getAuxiliaryNodeGroupsOrBuilder(
int index) {
if (auxiliaryNodeGroupsBuilder_ == null) {
return auxiliaryNodeGroups_.get(index);
} else {
return auxiliaryNodeGroupsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List extends com.google.cloud.dataproc.v1.AuxiliaryNodeGroupOrBuilder>
getAuxiliaryNodeGroupsOrBuilderList() {
if (auxiliaryNodeGroupsBuilder_ != null) {
return auxiliaryNodeGroupsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(auxiliaryNodeGroups_);
}
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.Builder addAuxiliaryNodeGroupsBuilder() {
return getAuxiliaryNodeGroupsFieldBuilder()
.addBuilder(com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.getDefaultInstance());
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.Builder addAuxiliaryNodeGroupsBuilder(
int index) {
return getAuxiliaryNodeGroupsFieldBuilder()
.addBuilder(index, com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.getDefaultInstance());
}
/**
*
*
*
* Optional. The node group settings.
*
*
*
* repeated .google.cloud.dataproc.v1.AuxiliaryNodeGroup auxiliary_node_groups = 25 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public java.util.List
getAuxiliaryNodeGroupsBuilderList() {
return getAuxiliaryNodeGroupsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dataproc.v1.AuxiliaryNodeGroup,
com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.Builder,
com.google.cloud.dataproc.v1.AuxiliaryNodeGroupOrBuilder>
getAuxiliaryNodeGroupsFieldBuilder() {
if (auxiliaryNodeGroupsBuilder_ == null) {
auxiliaryNodeGroupsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dataproc.v1.AuxiliaryNodeGroup,
com.google.cloud.dataproc.v1.AuxiliaryNodeGroup.Builder,
com.google.cloud.dataproc.v1.AuxiliaryNodeGroupOrBuilder>(
auxiliaryNodeGroups_,
((bitField0_ & 0x00008000) != 0),
getParentForChildren(),
isClean());
auxiliaryNodeGroups_ = null;
}
return auxiliaryNodeGroupsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.dataproc.v1.ClusterConfig)
}
// @@protoc_insertion_point(class_scope:google.cloud.dataproc.v1.ClusterConfig)
private static final com.google.cloud.dataproc.v1.ClusterConfig DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.dataproc.v1.ClusterConfig();
}
public static com.google.cloud.dataproc.v1.ClusterConfig getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ClusterConfig parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.dataproc.v1.ClusterConfig getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy