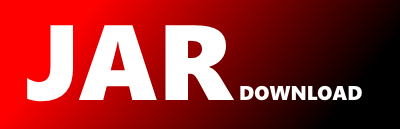
google.cloud.datastream.v1.datastream.proto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-datastream-v1 Show documentation
Show all versions of proto-google-cloud-datastream-v1 Show documentation
Proto library for google-cloud-datastream
// Copyright 2024 Google LLC
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
syntax = "proto3";
package google.cloud.datastream.v1;
import "google/api/annotations.proto";
import "google/api/client.proto";
import "google/api/field_behavior.proto";
import "google/api/resource.proto";
import "google/cloud/datastream/v1/datastream_resources.proto";
import "google/longrunning/operations.proto";
import "google/protobuf/empty.proto";
import "google/protobuf/field_mask.proto";
import "google/protobuf/timestamp.proto";
option csharp_namespace = "Google.Cloud.Datastream.V1";
option go_package = "cloud.google.com/go/datastream/apiv1/datastreampb;datastreampb";
option java_multiple_files = true;
option java_outer_classname = "DatastreamProto";
option java_package = "com.google.cloud.datastream.v1";
option php_namespace = "Google\\Cloud\\Datastream\\V1";
option ruby_package = "Google::Cloud::Datastream::V1";
// Datastream service
service Datastream {
option (google.api.default_host) = "datastream.googleapis.com";
option (google.api.oauth_scopes) =
"https://www.googleapis.com/auth/cloud-platform";
// Use this method to list connection profiles created in a project and
// location.
rpc ListConnectionProfiles(ListConnectionProfilesRequest)
returns (ListConnectionProfilesResponse) {
option (google.api.http) = {
get: "/v1/{parent=projects/*/locations/*}/connectionProfiles"
};
option (google.api.method_signature) = "parent";
}
// Use this method to get details about a connection profile.
rpc GetConnectionProfile(GetConnectionProfileRequest)
returns (ConnectionProfile) {
option (google.api.http) = {
get: "/v1/{name=projects/*/locations/*/connectionProfiles/*}"
};
option (google.api.method_signature) = "name";
}
// Use this method to create a connection profile in a project and location.
rpc CreateConnectionProfile(CreateConnectionProfileRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1/{parent=projects/*/locations/*}/connectionProfiles"
body: "connection_profile"
};
option (google.api.method_signature) =
"parent,connection_profile,connection_profile_id";
option (google.longrunning.operation_info) = {
response_type: "ConnectionProfile"
metadata_type: "OperationMetadata"
};
}
// Use this method to update the parameters of a connection profile.
rpc UpdateConnectionProfile(UpdateConnectionProfileRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
patch: "/v1/{connection_profile.name=projects/*/locations/*/connectionProfiles/*}"
body: "connection_profile"
};
option (google.api.method_signature) = "connection_profile,update_mask";
option (google.longrunning.operation_info) = {
response_type: "ConnectionProfile"
metadata_type: "OperationMetadata"
};
}
// Use this method to delete a connection profile.
rpc DeleteConnectionProfile(DeleteConnectionProfileRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
delete: "/v1/{name=projects/*/locations/*/connectionProfiles/*}"
};
option (google.api.method_signature) = "name";
option (google.longrunning.operation_info) = {
response_type: "google.protobuf.Empty"
metadata_type: "OperationMetadata"
};
}
// Use this method to discover a connection profile.
// The discover API call exposes the data objects and metadata belonging to
// the profile. Typically, a request returns children data objects of a
// parent data object that's optionally supplied in the request.
rpc DiscoverConnectionProfile(DiscoverConnectionProfileRequest)
returns (DiscoverConnectionProfileResponse) {
option (google.api.http) = {
post: "/v1/{parent=projects/*/locations/*}/connectionProfiles:discover"
body: "*"
};
}
// Use this method to list streams in a project and location.
rpc ListStreams(ListStreamsRequest) returns (ListStreamsResponse) {
option (google.api.http) = {
get: "/v1/{parent=projects/*/locations/*}/streams"
};
option (google.api.method_signature) = "parent";
}
// Use this method to get details about a stream.
rpc GetStream(GetStreamRequest) returns (Stream) {
option (google.api.http) = {
get: "/v1/{name=projects/*/locations/*/streams/*}"
};
option (google.api.method_signature) = "name";
}
// Use this method to create a stream.
rpc CreateStream(CreateStreamRequest) returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1/{parent=projects/*/locations/*}/streams"
body: "stream"
};
option (google.api.method_signature) = "parent,stream,stream_id";
option (google.longrunning.operation_info) = {
response_type: "Stream"
metadata_type: "OperationMetadata"
};
}
// Use this method to update the configuration of a stream.
rpc UpdateStream(UpdateStreamRequest) returns (google.longrunning.Operation) {
option (google.api.http) = {
patch: "/v1/{stream.name=projects/*/locations/*/streams/*}"
body: "stream"
};
option (google.api.method_signature) = "stream,update_mask";
option (google.longrunning.operation_info) = {
response_type: "Stream"
metadata_type: "OperationMetadata"
};
}
// Use this method to delete a stream.
rpc DeleteStream(DeleteStreamRequest) returns (google.longrunning.Operation) {
option (google.api.http) = {
delete: "/v1/{name=projects/*/locations/*/streams/*}"
};
option (google.api.method_signature) = "name";
option (google.longrunning.operation_info) = {
response_type: "google.protobuf.Empty"
metadata_type: "OperationMetadata"
};
}
// Use this method to get details about a stream object.
rpc GetStreamObject(GetStreamObjectRequest) returns (StreamObject) {
option (google.api.http) = {
get: "/v1/{name=projects/*/locations/*/streams/*/objects/*}"
};
option (google.api.method_signature) = "name";
}
// Use this method to look up a stream object by its source object identifier.
rpc LookupStreamObject(LookupStreamObjectRequest) returns (StreamObject) {
option (google.api.http) = {
post: "/v1/{parent=projects/*/locations/*/streams/*}/objects:lookup"
body: "*"
};
}
// Use this method to list the objects of a specific stream.
rpc ListStreamObjects(ListStreamObjectsRequest)
returns (ListStreamObjectsResponse) {
option (google.api.http) = {
get: "/v1/{parent=projects/*/locations/*/streams/*}/objects"
};
option (google.api.method_signature) = "parent";
}
// Use this method to start a backfill job for the specified stream object.
rpc StartBackfillJob(StartBackfillJobRequest)
returns (StartBackfillJobResponse) {
option (google.api.http) = {
post: "/v1/{object=projects/*/locations/*/streams/*/objects/*}:startBackfillJob"
body: "*"
};
option (google.api.method_signature) = "object";
}
// Use this method to stop a backfill job for the specified stream object.
rpc StopBackfillJob(StopBackfillJobRequest)
returns (StopBackfillJobResponse) {
option (google.api.http) = {
post: "/v1/{object=projects/*/locations/*/streams/*/objects/*}:stopBackfillJob"
body: "*"
};
option (google.api.method_signature) = "object";
}
// The FetchStaticIps API call exposes the static IP addresses used by
// Datastream.
rpc FetchStaticIps(FetchStaticIpsRequest) returns (FetchStaticIpsResponse) {
option (google.api.http) = {
get: "/v1/{name=projects/*/locations/*}:fetchStaticIps"
};
option (google.api.method_signature) = "name";
}
// Use this method to create a private connectivity configuration.
rpc CreatePrivateConnection(CreatePrivateConnectionRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1/{parent=projects/*/locations/*}/privateConnections"
body: "private_connection"
};
option (google.api.method_signature) =
"parent,private_connection,private_connection_id";
option (google.longrunning.operation_info) = {
response_type: "PrivateConnection"
metadata_type: "OperationMetadata"
};
}
// Use this method to get details about a private connectivity configuration.
rpc GetPrivateConnection(GetPrivateConnectionRequest)
returns (PrivateConnection) {
option (google.api.http) = {
get: "/v1/{name=projects/*/locations/*/privateConnections/*}"
};
option (google.api.method_signature) = "name";
}
// Use this method to list private connectivity configurations in a project
// and location.
rpc ListPrivateConnections(ListPrivateConnectionsRequest)
returns (ListPrivateConnectionsResponse) {
option (google.api.http) = {
get: "/v1/{parent=projects/*/locations/*}/privateConnections"
};
option (google.api.method_signature) = "parent";
}
// Use this method to delete a private connectivity configuration.
rpc DeletePrivateConnection(DeletePrivateConnectionRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
delete: "/v1/{name=projects/*/locations/*/privateConnections/*}"
};
option (google.api.method_signature) = "name";
option (google.longrunning.operation_info) = {
response_type: "google.protobuf.Empty"
metadata_type: "OperationMetadata"
};
}
// Use this method to create a route for a private connectivity configuration
// in a project and location.
rpc CreateRoute(CreateRouteRequest) returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1/{parent=projects/*/locations/*/privateConnections/*}/routes"
body: "route"
};
option (google.api.method_signature) = "parent,route,route_id";
option (google.longrunning.operation_info) = {
response_type: "Route"
metadata_type: "OperationMetadata"
};
}
// Use this method to get details about a route.
rpc GetRoute(GetRouteRequest) returns (Route) {
option (google.api.http) = {
get: "/v1/{name=projects/*/locations/*/privateConnections/*/routes/*}"
};
option (google.api.method_signature) = "name";
}
// Use this method to list routes created for a private connectivity
// configuration in a project and location.
rpc ListRoutes(ListRoutesRequest) returns (ListRoutesResponse) {
option (google.api.http) = {
get: "/v1/{parent=projects/*/locations/*/privateConnections/*}/routes"
};
option (google.api.method_signature) = "parent";
}
// Use this method to delete a route.
rpc DeleteRoute(DeleteRouteRequest) returns (google.longrunning.Operation) {
option (google.api.http) = {
delete: "/v1/{name=projects/*/locations/*/privateConnections/*/routes/*}"
};
option (google.api.method_signature) = "name";
option (google.longrunning.operation_info) = {
response_type: "google.protobuf.Empty"
metadata_type: "OperationMetadata"
};
}
}
// Request message for 'discover' ConnectionProfile request.
message DiscoverConnectionProfileRequest {
// Required. The parent resource of the connection profile type. Must be in
// the format `projects/*/locations/*`.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "datastream.googleapis.com/ConnectionProfile"
}
];
// The connection profile on which to run discover.
oneof target {
// An ad-hoc connection profile configuration.
ConnectionProfile connection_profile = 200;
// A reference to an existing connection profile.
string connection_profile_name = 201;
}
// The depth of the retrieved hierarchy of data objects.
oneof hierarchy {
// Whether to retrieve the full hierarchy of data objects (TRUE) or only the
// current level (FALSE).
bool full_hierarchy = 3;
// The number of hierarchy levels below the current level to be retrieved.
int32 hierarchy_depth = 4;
}
// The data object to populate with child data objects and metadata.
oneof data_object {
// Oracle RDBMS to enrich with child data objects and metadata.
OracleRdbms oracle_rdbms = 100;
// MySQL RDBMS to enrich with child data objects and metadata.
MysqlRdbms mysql_rdbms = 101;
// PostgreSQL RDBMS to enrich with child data objects and metadata.
PostgresqlRdbms postgresql_rdbms = 102;
}
}
// Response from a discover request.
message DiscoverConnectionProfileResponse {
// The data object that has been enriched by the discover API call.
oneof data_object {
// Enriched Oracle RDBMS object.
OracleRdbms oracle_rdbms = 100;
// Enriched MySQL RDBMS object.
MysqlRdbms mysql_rdbms = 101;
// Enriched PostgreSQL RDBMS object.
PostgresqlRdbms postgresql_rdbms = 102;
}
}
// Request message for 'FetchStaticIps' request.
message FetchStaticIpsRequest {
// Required. The resource name for the location for which static IPs should be
// returned. Must be in the format `projects/*/locations/*`.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "locations.googleapis.com/Location"
}
];
// Maximum number of Ips to return, will likely not be specified.
int32 page_size = 2;
// A page token, received from a previous `ListStaticIps` call.
// will likely not be specified.
string page_token = 3;
}
// Response message for a 'FetchStaticIps' response.
message FetchStaticIpsResponse {
// list of static ips by account
repeated string static_ips = 1;
// A token that can be sent as `page_token` to retrieve the next page.
// If this field is omitted, there are no subsequent pages.
string next_page_token = 2;
}
// Request message for listing connection profiles.
message ListConnectionProfilesRequest {
// Required. The parent that owns the collection of connection profiles.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "datastream.googleapis.com/ConnectionProfile"
}
];
// Maximum number of connection profiles to return.
// If unspecified, at most 50 connection profiles will be returned.
// The maximum value is 1000; values above 1000 will be coerced to 1000.
int32 page_size = 2;
// Page token received from a previous `ListConnectionProfiles` call.
// Provide this to retrieve the subsequent page.
//
// When paginating, all other parameters provided to `ListConnectionProfiles`
// must match the call that provided the page token.
string page_token = 3;
// Filter request.
string filter = 4;
// Order by fields for the result.
string order_by = 5;
}
// Response message for listing connection profiles.
message ListConnectionProfilesResponse {
// List of connection profiles.
repeated ConnectionProfile connection_profiles = 1;
// A token, which can be sent as `page_token` to retrieve the next page.
// If this field is omitted, there are no subsequent pages.
string next_page_token = 2;
// Locations that could not be reached.
repeated string unreachable = 3;
}
// Request message for getting a connection profile.
message GetConnectionProfileRequest {
// Required. The name of the connection profile resource to get.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/ConnectionProfile"
}
];
}
// Request message for creating a connection profile.
message CreateConnectionProfileRequest {
// Required. The parent that owns the collection of ConnectionProfiles.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "datastream.googleapis.com/ConnectionProfile"
}
];
// Required. The connection profile identifier.
string connection_profile_id = 2 [(google.api.field_behavior) = REQUIRED];
// Required. The connection profile resource to create.
ConnectionProfile connection_profile = 3
[(google.api.field_behavior) = REQUIRED];
// Optional. A request ID to identify requests. Specify a unique request ID
// so that if you must retry your request, the server will know to ignore
// the request if it has already been completed. The server will guarantee
// that for at least 60 minutes since the first request.
//
// For example, consider a situation where you make an initial request and the
// request times out. If you make the request again with the same request ID,
// the server can check if original operation with the same request ID was
// received, and if so, will ignore the second request. This prevents clients
// from accidentally creating duplicate commitments.
//
// The request ID must be a valid UUID with the exception that zero UUID is
// not supported (00000000-0000-0000-0000-000000000000).
string request_id = 4 [(google.api.field_behavior) = OPTIONAL];
// Optional. Only validate the connection profile, but don't create any
// resources. The default is false.
bool validate_only = 5 [(google.api.field_behavior) = OPTIONAL];
// Optional. Create the connection profile without validating it.
bool force = 6 [(google.api.field_behavior) = OPTIONAL];
}
// Connection profile update message.
message UpdateConnectionProfileRequest {
// Optional. Field mask is used to specify the fields to be overwritten in the
// ConnectionProfile resource by the update.
// The fields specified in the update_mask are relative to the resource, not
// the full request. A field will be overwritten if it is in the mask. If the
// user does not provide a mask then all fields will be overwritten.
google.protobuf.FieldMask update_mask = 1
[(google.api.field_behavior) = OPTIONAL];
// Required. The connection profile to update.
ConnectionProfile connection_profile = 2
[(google.api.field_behavior) = REQUIRED];
// Optional. A request ID to identify requests. Specify a unique request ID
// so that if you must retry your request, the server will know to ignore
// the request if it has already been completed. The server will guarantee
// that for at least 60 minutes since the first request.
//
// For example, consider a situation where you make an initial request and the
// request times out. If you make the request again with the same request ID,
// the server can check if original operation with the same request ID was
// received, and if so, will ignore the second request. This prevents clients
// from accidentally creating duplicate commitments.
//
// The request ID must be a valid UUID with the exception that zero UUID is
// not supported (00000000-0000-0000-0000-000000000000).
string request_id = 3 [(google.api.field_behavior) = OPTIONAL];
// Optional. Only validate the connection profile, but don't update any
// resources. The default is false.
bool validate_only = 4 [(google.api.field_behavior) = OPTIONAL];
// Optional. Update the connection profile without validating it.
bool force = 5 [(google.api.field_behavior) = OPTIONAL];
}
// Request message for deleting a connection profile.
message DeleteConnectionProfileRequest {
// Required. The name of the connection profile resource to delete.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/ConnectionProfile"
}
];
// Optional. A request ID to identify requests. Specify a unique request ID
// so that if you must retry your request, the server will know to ignore
// the request if it has already been completed. The server will guarantee
// that for at least 60 minutes after the first request.
//
// For example, consider a situation where you make an initial request and the
// request times out. If you make the request again with the same request ID,
// the server can check if original operation with the same request ID was
// received, and if so, will ignore the second request. This prevents clients
// from accidentally creating duplicate commitments.
//
// The request ID must be a valid UUID with the exception that zero UUID is
// not supported (00000000-0000-0000-0000-000000000000).
string request_id = 2 [(google.api.field_behavior) = OPTIONAL];
}
// Request message for listing streams.
message ListStreamsRequest {
// Required. The parent that owns the collection of streams.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "datastream.googleapis.com/Stream"
}
];
// Maximum number of streams to return.
// If unspecified, at most 50 streams will be returned. The maximum
// value is 1000; values above 1000 will be coerced to 1000.
int32 page_size = 2;
// Page token received from a previous `ListStreams` call.
// Provide this to retrieve the subsequent page.
//
// When paginating, all other parameters provided to `ListStreams`
// must match the call that provided the page token.
string page_token = 3;
// Filter request.
string filter = 4;
// Order by fields for the result.
string order_by = 5;
}
// Response message for listing streams.
message ListStreamsResponse {
// List of streams
repeated Stream streams = 1;
// A token, which can be sent as `page_token` to retrieve the next page.
// If this field is omitted, there are no subsequent pages.
string next_page_token = 2;
// Locations that could not be reached.
repeated string unreachable = 3;
}
// Request message for getting a stream.
message GetStreamRequest {
// Required. The name of the stream resource to get.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/Stream"
}
];
}
// Request message for creating a stream.
message CreateStreamRequest {
// Required. The parent that owns the collection of streams.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "datastream.googleapis.com/Stream"
}
];
// Required. The stream identifier.
string stream_id = 2 [(google.api.field_behavior) = REQUIRED];
// Required. The stream resource to create.
Stream stream = 3 [(google.api.field_behavior) = REQUIRED];
// Optional. A request ID to identify requests. Specify a unique request ID
// so that if you must retry your request, the server will know to ignore
// the request if it has already been completed. The server will guarantee
// that for at least 60 minutes since the first request.
//
// For example, consider a situation where you make an initial request and the
// request times out. If you make the request again with the same request ID,
// the server can check if original operation with the same request ID was
// received, and if so, will ignore the second request. This prevents clients
// from accidentally creating duplicate commitments.
//
// The request ID must be a valid UUID with the exception that zero UUID is
// not supported (00000000-0000-0000-0000-000000000000).
string request_id = 4 [(google.api.field_behavior) = OPTIONAL];
// Optional. Only validate the stream, but don't create any resources.
// The default is false.
bool validate_only = 5 [(google.api.field_behavior) = OPTIONAL];
// Optional. Create the stream without validating it.
bool force = 6 [(google.api.field_behavior) = OPTIONAL];
}
// Request message for updating a stream.
message UpdateStreamRequest {
// Optional. Field mask is used to specify the fields to be overwritten in the
// stream resource by the update.
// The fields specified in the update_mask are relative to the resource, not
// the full request. A field will be overwritten if it is in the mask. If the
// user does not provide a mask then all fields will be overwritten.
google.protobuf.FieldMask update_mask = 1
[(google.api.field_behavior) = OPTIONAL];
// Required. The stream resource to update.
Stream stream = 2 [(google.api.field_behavior) = REQUIRED];
// Optional. A request ID to identify requests. Specify a unique request ID
// so that if you must retry your request, the server will know to ignore
// the request if it has already been completed. The server will guarantee
// that for at least 60 minutes since the first request.
//
// For example, consider a situation where you make an initial request and the
// request times out. If you make the request again with the same request ID,
// the server can check if original operation with the same request ID was
// received, and if so, will ignore the second request. This prevents clients
// from accidentally creating duplicate commitments.
//
// The request ID must be a valid UUID with the exception that zero UUID is
// not supported (00000000-0000-0000-0000-000000000000).
string request_id = 3 [(google.api.field_behavior) = OPTIONAL];
// Optional. Only validate the stream with the changes, without actually
// updating it. The default is false.
bool validate_only = 4 [(google.api.field_behavior) = OPTIONAL];
// Optional. Update the stream without validating it.
bool force = 5 [(google.api.field_behavior) = OPTIONAL];
}
// Request message for deleting a stream.
message DeleteStreamRequest {
// Required. The name of the stream resource to delete.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/Stream"
}
];
// Optional. A request ID to identify requests. Specify a unique request ID
// so that if you must retry your request, the server will know to ignore
// the request if it has already been completed. The server will guarantee
// that for at least 60 minutes after the first request.
//
// For example, consider a situation where you make an initial request and the
// request times out. If you make the request again with the same request ID,
// the server can check if original operation with the same request ID was
// received, and if so, will ignore the second request. This prevents clients
// from accidentally creating duplicate commitments.
//
// The request ID must be a valid UUID with the exception that zero UUID is
// not supported (00000000-0000-0000-0000-000000000000).
string request_id = 2 [(google.api.field_behavior) = OPTIONAL];
}
// Request for fetching a specific stream object.
message GetStreamObjectRequest {
// Required. The name of the stream object resource to get.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/StreamObject"
}
];
}
// Request for looking up a specific stream object by its source object
// identifier.
message LookupStreamObjectRequest {
// Required. The parent stream that owns the collection of objects.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/Stream"
}
];
// Required. The source object identifier which maps to the stream object.
SourceObjectIdentifier source_object_identifier = 2
[(google.api.field_behavior) = REQUIRED];
}
// Request for manually initiating a backfill job for a specific stream object.
message StartBackfillJobRequest {
// Required. The name of the stream object resource to start a backfill job
// for.
string object = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/StreamObject"
}
];
}
// Response for manually initiating a backfill job for a specific stream object.
message StartBackfillJobResponse {
// The stream object resource a backfill job was started for.
StreamObject object = 1;
}
// Request for manually stopping a running backfill job for a specific stream
// object.
message StopBackfillJobRequest {
// Required. The name of the stream object resource to stop the backfill job
// for.
string object = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/StreamObject"
}
];
}
// Response for manually stop a backfill job for a specific stream object.
message StopBackfillJobResponse {
// The stream object resource the backfill job was stopped for.
StreamObject object = 1;
}
// Request for listing all objects for a specific stream.
message ListStreamObjectsRequest {
// Required. The parent stream that owns the collection of objects.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "datastream.googleapis.com/StreamObject"
}
];
// Maximum number of objects to return. Default is 50.
// The maximum value is 1000; values above 1000 will be coerced to 1000.
int32 page_size = 2;
// Page token received from a previous `ListStreamObjectsRequest` call.
// Provide this to retrieve the subsequent page.
//
// When paginating, all other parameters provided to
// `ListStreamObjectsRequest` must match the call that provided the page
// token.
string page_token = 3;
}
// Response containing the objects for a stream.
message ListStreamObjectsResponse {
// List of stream objects.
repeated StreamObject stream_objects = 1;
// A token, which can be sent as `page_token` to retrieve the next page.
string next_page_token = 2;
}
// Represents the metadata of the long-running operation.
message OperationMetadata {
// Output only. The time the operation was created.
google.protobuf.Timestamp create_time = 1
[(google.api.field_behavior) = OUTPUT_ONLY];
// Output only. The time the operation finished running.
google.protobuf.Timestamp end_time = 2
[(google.api.field_behavior) = OUTPUT_ONLY];
// Output only. Server-defined resource path for the target of the operation.
string target = 3 [(google.api.field_behavior) = OUTPUT_ONLY];
// Output only. Name of the verb executed by the operation.
string verb = 4 [(google.api.field_behavior) = OUTPUT_ONLY];
// Output only. Human-readable status of the operation, if any.
string status_message = 5 [(google.api.field_behavior) = OUTPUT_ONLY];
// Output only. Identifies whether the user has requested cancellation
// of the operation. Operations that have successfully been cancelled
// have [Operation.error][] value with a
// [google.rpc.Status.code][google.rpc.Status.code] of 1, corresponding to
// `Code.CANCELLED`.
bool requested_cancellation = 6 [(google.api.field_behavior) = OUTPUT_ONLY];
// Output only. API version used to start the operation.
string api_version = 7 [(google.api.field_behavior) = OUTPUT_ONLY];
// Output only. Results of executed validations if there are any.
ValidationResult validation_result = 8
[(google.api.field_behavior) = OUTPUT_ONLY];
}
// Request for creating a private connection.
message CreatePrivateConnectionRequest {
// Required. The parent that owns the collection of PrivateConnections.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "datastream.googleapis.com/PrivateConnection"
}
];
// Required. The private connectivity identifier.
string private_connection_id = 2 [(google.api.field_behavior) = REQUIRED];
// Required. The Private Connectivity resource to create.
PrivateConnection private_connection = 3
[(google.api.field_behavior) = REQUIRED];
// Optional. A request ID to identify requests. Specify a unique request ID
// so that if you must retry your request, the server will know to ignore
// the request if it has already been completed. The server will guarantee
// that for at least 60 minutes since the first request.
//
// For example, consider a situation where you make an initial request and the
// request times out. If you make the request again with the same request ID,
// the server can check if original operation with the same request ID was
// received, and if so, will ignore the second request. This prevents clients
// from accidentally creating duplicate commitments.
//
// The request ID must be a valid UUID with the exception that zero UUID is
// not supported (00000000-0000-0000-0000-000000000000).
string request_id = 4 [(google.api.field_behavior) = OPTIONAL];
// Optional. If set to true, will skip validations.
bool force = 6 [(google.api.field_behavior) = OPTIONAL];
}
// Request for listing private connections.
message ListPrivateConnectionsRequest {
// Required. The parent that owns the collection of private connectivity
// configurations.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "datastream.googleapis.com/PrivateConnection"
}
];
// Maximum number of private connectivity configurations to return.
// If unspecified, at most 50 private connectivity configurations that will be
// returned. The maximum value is 1000; values above 1000 will be coerced to
// 1000.
int32 page_size = 2;
// Page token received from a previous `ListPrivateConnections` call.
// Provide this to retrieve the subsequent page.
//
// When paginating, all other parameters provided to
// `ListPrivateConnections` must match the call that provided the page
// token.
string page_token = 3;
// Filter request.
string filter = 4;
// Order by fields for the result.
string order_by = 5;
}
// Response containing a list of private connection configurations.
message ListPrivateConnectionsResponse {
// List of private connectivity configurations.
repeated PrivateConnection private_connections = 1;
// A token, which can be sent as `page_token` to retrieve the next page.
// If this field is omitted, there are no subsequent pages.
string next_page_token = 2;
// Locations that could not be reached.
repeated string unreachable = 3;
}
// Request to delete a private connection.
message DeletePrivateConnectionRequest {
// Required. The name of the private connectivity configuration to delete.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/PrivateConnection"
}
];
// Optional. A request ID to identify requests. Specify a unique request ID
// so that if you must retry your request, the server will know to ignore
// the request if it has already been completed. The server will guarantee
// that for at least 60 minutes after the first request.
//
// For example, consider a situation where you make an initial request and the
// request times out. If you make the request again with the same request ID,
// the server can check if original operation with the same request ID was
// received, and if so, will ignore the second request. This prevents clients
// from accidentally creating duplicate commitments.
//
// The request ID must be a valid UUID with the exception that zero UUID is
// not supported (00000000-0000-0000-0000-000000000000).
string request_id = 2 [(google.api.field_behavior) = OPTIONAL];
// Optional. If set to true, any child routes that belong to this
// PrivateConnection will also be deleted.
bool force = 3 [(google.api.field_behavior) = OPTIONAL];
}
// Request to get a private connection configuration.
message GetPrivateConnectionRequest {
// Required. The name of the private connectivity configuration to get.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/PrivateConnection"
}
];
}
// Route creation request.
message CreateRouteRequest {
// Required. The parent that owns the collection of Routes.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "datastream.googleapis.com/Route"
}
];
// Required. The Route identifier.
string route_id = 2 [(google.api.field_behavior) = REQUIRED];
// Required. The Route resource to create.
Route route = 3 [(google.api.field_behavior) = REQUIRED];
// Optional. A request ID to identify requests. Specify a unique request ID
// so that if you must retry your request, the server will know to ignore
// the request if it has already been completed. The server will guarantee
// that for at least 60 minutes since the first request.
//
// For example, consider a situation where you make an initial request and the
// request times out. If you make the request again with the same request ID,
// the server can check if original operation with the same request ID was
// received, and if so, will ignore the second request. This prevents clients
// from accidentally creating duplicate commitments.
//
// The request ID must be a valid UUID with the exception that zero UUID is
// not supported (00000000-0000-0000-0000-000000000000).
string request_id = 4 [(google.api.field_behavior) = OPTIONAL];
}
// Route list request.
message ListRoutesRequest {
// Required. The parent that owns the collection of Routess.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "datastream.googleapis.com/Route"
}
];
// Maximum number of Routes to return. The service may return
// fewer than this value. If unspecified, at most 50 Routes
// will be returned. The maximum value is 1000; values above 1000 will be
// coerced to 1000.
int32 page_size = 2;
// Page token received from a previous `ListRoutes` call.
// Provide this to retrieve the subsequent page.
//
// When paginating, all other parameters provided to
// `ListRoutes` must match the call that provided the page
// token.
string page_token = 3;
// Filter request.
string filter = 4;
// Order by fields for the result.
string order_by = 5;
}
// Route list response.
message ListRoutesResponse {
// List of Routes.
repeated Route routes = 1;
// A token, which can be sent as `page_token` to retrieve the next page.
// If this field is omitted, there are no subsequent pages.
string next_page_token = 2;
// Locations that could not be reached.
repeated string unreachable = 3;
}
// Route deletion request.
message DeleteRouteRequest {
// Required. The name of the Route resource to delete.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/Route"
}
];
// Optional. A request ID to identify requests. Specify a unique request ID
// so that if you must retry your request, the server will know to ignore
// the request if it has already been completed. The server will guarantee
// that for at least 60 minutes after the first request.
//
// For example, consider a situation where you make an initial request and the
// request times out. If you make the request again with the same request ID,
// the server can check if original operation with the same request ID was
// received, and if so, will ignore the second request. This prevents clients
// from accidentally creating duplicate commitments.
//
// The request ID must be a valid UUID with the exception that zero UUID is
// not supported (00000000-0000-0000-0000-000000000000).
string request_id = 2 [(google.api.field_behavior) = OPTIONAL];
}
// Route get request.
message GetRouteRequest {
// Required. The name of the Route resource to get.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "datastream.googleapis.com/Route"
}
];
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy