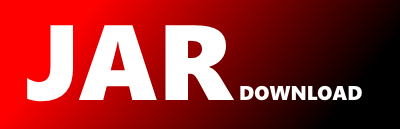
com.google.devtools.clouddebugger.v2.BreakpointOrBuilder Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/devtools/clouddebugger/v2/data.proto
// Protobuf Java Version: 3.25.5
package com.google.devtools.clouddebugger.v2;
public interface BreakpointOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.devtools.clouddebugger.v2.Breakpoint)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Breakpoint identifier, unique in the scope of the debuggee.
*
*
* string id = 1;
*
* @return The id.
*/
java.lang.String getId();
/**
*
*
*
* Breakpoint identifier, unique in the scope of the debuggee.
*
*
* string id = 1;
*
* @return The bytes for id.
*/
com.google.protobuf.ByteString getIdBytes();
/**
*
*
*
* Action that the agent should perform when the code at the
* breakpoint location is hit.
*
*
* .google.devtools.clouddebugger.v2.Breakpoint.Action action = 13;
*
* @return The enum numeric value on the wire for action.
*/
int getActionValue();
/**
*
*
*
* Action that the agent should perform when the code at the
* breakpoint location is hit.
*
*
* .google.devtools.clouddebugger.v2.Breakpoint.Action action = 13;
*
* @return The action.
*/
com.google.devtools.clouddebugger.v2.Breakpoint.Action getAction();
/**
*
*
*
* Breakpoint source location.
*
*
* .google.devtools.clouddebugger.v2.SourceLocation location = 2;
*
* @return Whether the location field is set.
*/
boolean hasLocation();
/**
*
*
*
* Breakpoint source location.
*
*
* .google.devtools.clouddebugger.v2.SourceLocation location = 2;
*
* @return The location.
*/
com.google.devtools.clouddebugger.v2.SourceLocation getLocation();
/**
*
*
*
* Breakpoint source location.
*
*
* .google.devtools.clouddebugger.v2.SourceLocation location = 2;
*/
com.google.devtools.clouddebugger.v2.SourceLocationOrBuilder getLocationOrBuilder();
/**
*
*
*
* Condition that triggers the breakpoint.
* The condition is a compound boolean expression composed using expressions
* in a programming language at the source location.
*
*
* string condition = 3;
*
* @return The condition.
*/
java.lang.String getCondition();
/**
*
*
*
* Condition that triggers the breakpoint.
* The condition is a compound boolean expression composed using expressions
* in a programming language at the source location.
*
*
* string condition = 3;
*
* @return The bytes for condition.
*/
com.google.protobuf.ByteString getConditionBytes();
/**
*
*
*
* List of read-only expressions to evaluate at the breakpoint location.
* The expressions are composed using expressions in the programming language
* at the source location. If the breakpoint action is `LOG`, the evaluated
* expressions are included in log statements.
*
*
* repeated string expressions = 4;
*
* @return A list containing the expressions.
*/
java.util.List getExpressionsList();
/**
*
*
*
* List of read-only expressions to evaluate at the breakpoint location.
* The expressions are composed using expressions in the programming language
* at the source location. If the breakpoint action is `LOG`, the evaluated
* expressions are included in log statements.
*
*
* repeated string expressions = 4;
*
* @return The count of expressions.
*/
int getExpressionsCount();
/**
*
*
*
* List of read-only expressions to evaluate at the breakpoint location.
* The expressions are composed using expressions in the programming language
* at the source location. If the breakpoint action is `LOG`, the evaluated
* expressions are included in log statements.
*
*
* repeated string expressions = 4;
*
* @param index The index of the element to return.
* @return The expressions at the given index.
*/
java.lang.String getExpressions(int index);
/**
*
*
*
* List of read-only expressions to evaluate at the breakpoint location.
* The expressions are composed using expressions in the programming language
* at the source location. If the breakpoint action is `LOG`, the evaluated
* expressions are included in log statements.
*
*
* repeated string expressions = 4;
*
* @param index The index of the value to return.
* @return The bytes of the expressions at the given index.
*/
com.google.protobuf.ByteString getExpressionsBytes(int index);
/**
*
*
*
* Only relevant when action is `LOG`. Defines the message to log when
* the breakpoint hits. The message may include parameter placeholders `$0`,
* `$1`, etc. These placeholders are replaced with the evaluated value
* of the appropriate expression. Expressions not referenced in
* `log_message_format` are not logged.
*
* Example: `Message received, id = $0, count = $1` with
* `expressions` = `[ message.id, message.count ]`.
*
*
* string log_message_format = 14;
*
* @return The logMessageFormat.
*/
java.lang.String getLogMessageFormat();
/**
*
*
*
* Only relevant when action is `LOG`. Defines the message to log when
* the breakpoint hits. The message may include parameter placeholders `$0`,
* `$1`, etc. These placeholders are replaced with the evaluated value
* of the appropriate expression. Expressions not referenced in
* `log_message_format` are not logged.
*
* Example: `Message received, id = $0, count = $1` with
* `expressions` = `[ message.id, message.count ]`.
*
*
* string log_message_format = 14;
*
* @return The bytes for logMessageFormat.
*/
com.google.protobuf.ByteString getLogMessageFormatBytes();
/**
*
*
*
* Indicates the severity of the log. Only relevant when action is `LOG`.
*
*
* .google.devtools.clouddebugger.v2.Breakpoint.LogLevel log_level = 15;
*
* @return The enum numeric value on the wire for logLevel.
*/
int getLogLevelValue();
/**
*
*
*
* Indicates the severity of the log. Only relevant when action is `LOG`.
*
*
* .google.devtools.clouddebugger.v2.Breakpoint.LogLevel log_level = 15;
*
* @return The logLevel.
*/
com.google.devtools.clouddebugger.v2.Breakpoint.LogLevel getLogLevel();
/**
*
*
*
* When true, indicates that this is a final result and the
* breakpoint state will not change from here on.
*
*
* bool is_final_state = 5;
*
* @return The isFinalState.
*/
boolean getIsFinalState();
/**
*
*
*
* Time this breakpoint was created by the server in seconds resolution.
*
*
* .google.protobuf.Timestamp create_time = 11;
*
* @return Whether the createTime field is set.
*/
boolean hasCreateTime();
/**
*
*
*
* Time this breakpoint was created by the server in seconds resolution.
*
*
* .google.protobuf.Timestamp create_time = 11;
*
* @return The createTime.
*/
com.google.protobuf.Timestamp getCreateTime();
/**
*
*
*
* Time this breakpoint was created by the server in seconds resolution.
*
*
* .google.protobuf.Timestamp create_time = 11;
*/
com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder();
/**
*
*
*
* Time this breakpoint was finalized as seen by the server in seconds
* resolution.
*
*
* .google.protobuf.Timestamp final_time = 12;
*
* @return Whether the finalTime field is set.
*/
boolean hasFinalTime();
/**
*
*
*
* Time this breakpoint was finalized as seen by the server in seconds
* resolution.
*
*
* .google.protobuf.Timestamp final_time = 12;
*
* @return The finalTime.
*/
com.google.protobuf.Timestamp getFinalTime();
/**
*
*
*
* Time this breakpoint was finalized as seen by the server in seconds
* resolution.
*
*
* .google.protobuf.Timestamp final_time = 12;
*/
com.google.protobuf.TimestampOrBuilder getFinalTimeOrBuilder();
/**
*
*
*
* E-mail address of the user that created this breakpoint
*
*
* string user_email = 16;
*
* @return The userEmail.
*/
java.lang.String getUserEmail();
/**
*
*
*
* E-mail address of the user that created this breakpoint
*
*
* string user_email = 16;
*
* @return The bytes for userEmail.
*/
com.google.protobuf.ByteString getUserEmailBytes();
/**
*
*
*
* Breakpoint status.
*
* The status includes an error flag and a human readable message.
* This field is usually unset. The message can be either
* informational or an error message. Regardless, clients should always
* display the text message back to the user.
*
* Error status indicates complete failure of the breakpoint.
*
* Example (non-final state): `Still loading symbols...`
*
* Examples (final state):
*
* * `Invalid line number` referring to location
* * `Field f not found in class C` referring to condition
*
*
* .google.devtools.clouddebugger.v2.StatusMessage status = 10;
*
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
*
*
*
* Breakpoint status.
*
* The status includes an error flag and a human readable message.
* This field is usually unset. The message can be either
* informational or an error message. Regardless, clients should always
* display the text message back to the user.
*
* Error status indicates complete failure of the breakpoint.
*
* Example (non-final state): `Still loading symbols...`
*
* Examples (final state):
*
* * `Invalid line number` referring to location
* * `Field f not found in class C` referring to condition
*
*
* .google.devtools.clouddebugger.v2.StatusMessage status = 10;
*
* @return The status.
*/
com.google.devtools.clouddebugger.v2.StatusMessage getStatus();
/**
*
*
*
* Breakpoint status.
*
* The status includes an error flag and a human readable message.
* This field is usually unset. The message can be either
* informational or an error message. Regardless, clients should always
* display the text message back to the user.
*
* Error status indicates complete failure of the breakpoint.
*
* Example (non-final state): `Still loading symbols...`
*
* Examples (final state):
*
* * `Invalid line number` referring to location
* * `Field f not found in class C` referring to condition
*
*
* .google.devtools.clouddebugger.v2.StatusMessage status = 10;
*/
com.google.devtools.clouddebugger.v2.StatusMessageOrBuilder getStatusOrBuilder();
/**
*
*
*
* The stack at breakpoint time, where stack_frames[0] represents the most
* recently entered function.
*
*
* repeated .google.devtools.clouddebugger.v2.StackFrame stack_frames = 7;
*/
java.util.List getStackFramesList();
/**
*
*
*
* The stack at breakpoint time, where stack_frames[0] represents the most
* recently entered function.
*
*
* repeated .google.devtools.clouddebugger.v2.StackFrame stack_frames = 7;
*/
com.google.devtools.clouddebugger.v2.StackFrame getStackFrames(int index);
/**
*
*
*
* The stack at breakpoint time, where stack_frames[0] represents the most
* recently entered function.
*
*
* repeated .google.devtools.clouddebugger.v2.StackFrame stack_frames = 7;
*/
int getStackFramesCount();
/**
*
*
*
* The stack at breakpoint time, where stack_frames[0] represents the most
* recently entered function.
*
*
* repeated .google.devtools.clouddebugger.v2.StackFrame stack_frames = 7;
*/
java.util.List extends com.google.devtools.clouddebugger.v2.StackFrameOrBuilder>
getStackFramesOrBuilderList();
/**
*
*
*
* The stack at breakpoint time, where stack_frames[0] represents the most
* recently entered function.
*
*
* repeated .google.devtools.clouddebugger.v2.StackFrame stack_frames = 7;
*/
com.google.devtools.clouddebugger.v2.StackFrameOrBuilder getStackFramesOrBuilder(int index);
/**
*
*
*
* Values of evaluated expressions at breakpoint time.
* The evaluated expressions appear in exactly the same order they
* are listed in the `expressions` field.
* The `name` field holds the original expression text, the `value` or
* `members` field holds the result of the evaluated expression.
* If the expression cannot be evaluated, the `status` inside the `Variable`
* will indicate an error and contain the error text.
*
*
* repeated .google.devtools.clouddebugger.v2.Variable evaluated_expressions = 8;
*/
java.util.List getEvaluatedExpressionsList();
/**
*
*
*
* Values of evaluated expressions at breakpoint time.
* The evaluated expressions appear in exactly the same order they
* are listed in the `expressions` field.
* The `name` field holds the original expression text, the `value` or
* `members` field holds the result of the evaluated expression.
* If the expression cannot be evaluated, the `status` inside the `Variable`
* will indicate an error and contain the error text.
*
*
* repeated .google.devtools.clouddebugger.v2.Variable evaluated_expressions = 8;
*/
com.google.devtools.clouddebugger.v2.Variable getEvaluatedExpressions(int index);
/**
*
*
*
* Values of evaluated expressions at breakpoint time.
* The evaluated expressions appear in exactly the same order they
* are listed in the `expressions` field.
* The `name` field holds the original expression text, the `value` or
* `members` field holds the result of the evaluated expression.
* If the expression cannot be evaluated, the `status` inside the `Variable`
* will indicate an error and contain the error text.
*
*
* repeated .google.devtools.clouddebugger.v2.Variable evaluated_expressions = 8;
*/
int getEvaluatedExpressionsCount();
/**
*
*
*
* Values of evaluated expressions at breakpoint time.
* The evaluated expressions appear in exactly the same order they
* are listed in the `expressions` field.
* The `name` field holds the original expression text, the `value` or
* `members` field holds the result of the evaluated expression.
* If the expression cannot be evaluated, the `status` inside the `Variable`
* will indicate an error and contain the error text.
*
*
* repeated .google.devtools.clouddebugger.v2.Variable evaluated_expressions = 8;
*/
java.util.List extends com.google.devtools.clouddebugger.v2.VariableOrBuilder>
getEvaluatedExpressionsOrBuilderList();
/**
*
*
*
* Values of evaluated expressions at breakpoint time.
* The evaluated expressions appear in exactly the same order they
* are listed in the `expressions` field.
* The `name` field holds the original expression text, the `value` or
* `members` field holds the result of the evaluated expression.
* If the expression cannot be evaluated, the `status` inside the `Variable`
* will indicate an error and contain the error text.
*
*
* repeated .google.devtools.clouddebugger.v2.Variable evaluated_expressions = 8;
*/
com.google.devtools.clouddebugger.v2.VariableOrBuilder getEvaluatedExpressionsOrBuilder(
int index);
/**
*
*
*
* The `variable_table` exists to aid with computation, memory and network
* traffic optimization. It enables storing a variable once and reference
* it from multiple variables, including variables stored in the
* `variable_table` itself.
* For example, the same `this` object, which may appear at many levels of
* the stack, can have all of its data stored once in this table. The
* stack frame variables then would hold only a reference to it.
*
* The variable `var_table_index` field is an index into this repeated field.
* The stored objects are nameless and get their name from the referencing
* variable. The effective variable is a merge of the referencing variable
* and the referenced variable.
*
*
* repeated .google.devtools.clouddebugger.v2.Variable variable_table = 9;
*/
java.util.List getVariableTableList();
/**
*
*
*
* The `variable_table` exists to aid with computation, memory and network
* traffic optimization. It enables storing a variable once and reference
* it from multiple variables, including variables stored in the
* `variable_table` itself.
* For example, the same `this` object, which may appear at many levels of
* the stack, can have all of its data stored once in this table. The
* stack frame variables then would hold only a reference to it.
*
* The variable `var_table_index` field is an index into this repeated field.
* The stored objects are nameless and get their name from the referencing
* variable. The effective variable is a merge of the referencing variable
* and the referenced variable.
*
*
* repeated .google.devtools.clouddebugger.v2.Variable variable_table = 9;
*/
com.google.devtools.clouddebugger.v2.Variable getVariableTable(int index);
/**
*
*
*
* The `variable_table` exists to aid with computation, memory and network
* traffic optimization. It enables storing a variable once and reference
* it from multiple variables, including variables stored in the
* `variable_table` itself.
* For example, the same `this` object, which may appear at many levels of
* the stack, can have all of its data stored once in this table. The
* stack frame variables then would hold only a reference to it.
*
* The variable `var_table_index` field is an index into this repeated field.
* The stored objects are nameless and get their name from the referencing
* variable. The effective variable is a merge of the referencing variable
* and the referenced variable.
*
*
* repeated .google.devtools.clouddebugger.v2.Variable variable_table = 9;
*/
int getVariableTableCount();
/**
*
*
*
* The `variable_table` exists to aid with computation, memory and network
* traffic optimization. It enables storing a variable once and reference
* it from multiple variables, including variables stored in the
* `variable_table` itself.
* For example, the same `this` object, which may appear at many levels of
* the stack, can have all of its data stored once in this table. The
* stack frame variables then would hold only a reference to it.
*
* The variable `var_table_index` field is an index into this repeated field.
* The stored objects are nameless and get their name from the referencing
* variable. The effective variable is a merge of the referencing variable
* and the referenced variable.
*
*
* repeated .google.devtools.clouddebugger.v2.Variable variable_table = 9;
*/
java.util.List extends com.google.devtools.clouddebugger.v2.VariableOrBuilder>
getVariableTableOrBuilderList();
/**
*
*
*
* The `variable_table` exists to aid with computation, memory and network
* traffic optimization. It enables storing a variable once and reference
* it from multiple variables, including variables stored in the
* `variable_table` itself.
* For example, the same `this` object, which may appear at many levels of
* the stack, can have all of its data stored once in this table. The
* stack frame variables then would hold only a reference to it.
*
* The variable `var_table_index` field is an index into this repeated field.
* The stored objects are nameless and get their name from the referencing
* variable. The effective variable is a merge of the referencing variable
* and the referenced variable.
*
*
* repeated .google.devtools.clouddebugger.v2.Variable variable_table = 9;
*/
com.google.devtools.clouddebugger.v2.VariableOrBuilder getVariableTableOrBuilder(int index);
/**
*
*
*
* A set of custom breakpoint properties, populated by the agent, to be
* displayed to the user.
*
*
* map<string, string> labels = 17;
*/
int getLabelsCount();
/**
*
*
*
* A set of custom breakpoint properties, populated by the agent, to be
* displayed to the user.
*
*
* map<string, string> labels = 17;
*/
boolean containsLabels(java.lang.String key);
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Deprecated
java.util.Map getLabels();
/**
*
*
*
* A set of custom breakpoint properties, populated by the agent, to be
* displayed to the user.
*
*
* map<string, string> labels = 17;
*/
java.util.Map getLabelsMap();
/**
*
*
*
* A set of custom breakpoint properties, populated by the agent, to be
* displayed to the user.
*
*
* map<string, string> labels = 17;
*/
/* nullable */
java.lang.String getLabelsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
*
*
*
* A set of custom breakpoint properties, populated by the agent, to be
* displayed to the user.
*
*
* map<string, string> labels = 17;
*/
java.lang.String getLabelsOrThrow(java.lang.String key);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy