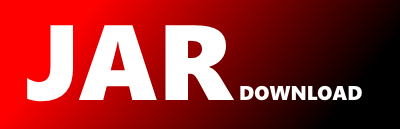
com.google.cloud.dialogflow.v2.DocumentOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-dialogflow-v2 Show documentation
Show all versions of proto-google-cloud-dialogflow-v2 Show documentation
PROTO library for proto-google-cloud-dialogflow-v2
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/dialogflow/v2/document.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.dialogflow.v2;
public interface DocumentOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.dialogflow.v2.Document)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Optional. The document resource name.
* The name must be empty when creating a document.
* Format: `projects/<Project ID>/locations/<Location
* ID>/knowledgeBases/<Knowledge Base ID>/documents/<Document ID>`.
*
*
* string name = 1 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The name.
*/
java.lang.String getName();
/**
*
*
*
* Optional. The document resource name.
* The name must be empty when creating a document.
* Format: `projects/<Project ID>/locations/<Location
* ID>/knowledgeBases/<Knowledge Base ID>/documents/<Document ID>`.
*
*
* string name = 1 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for name.
*/
com.google.protobuf.ByteString getNameBytes();
/**
*
*
*
* Required. The display name of the document. The name must be 1024 bytes or
* less; otherwise, the creation request fails.
*
*
* string display_name = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return The displayName.
*/
java.lang.String getDisplayName();
/**
*
*
*
* Required. The display name of the document. The name must be 1024 bytes or
* less; otherwise, the creation request fails.
*
*
* string display_name = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for displayName.
*/
com.google.protobuf.ByteString getDisplayNameBytes();
/**
*
*
*
* Required. The MIME type of this document.
*
*
* string mime_type = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @return The mimeType.
*/
java.lang.String getMimeType();
/**
*
*
*
* Required. The MIME type of this document.
*
*
* string mime_type = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for mimeType.
*/
com.google.protobuf.ByteString getMimeTypeBytes();
/**
*
*
*
* Required. The knowledge type of document content.
*
*
*
* repeated .google.cloud.dialogflow.v2.Document.KnowledgeType knowledge_types = 4 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return A list containing the knowledgeTypes.
*/
java.util.List getKnowledgeTypesList();
/**
*
*
*
* Required. The knowledge type of document content.
*
*
*
* repeated .google.cloud.dialogflow.v2.Document.KnowledgeType knowledge_types = 4 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The count of knowledgeTypes.
*/
int getKnowledgeTypesCount();
/**
*
*
*
* Required. The knowledge type of document content.
*
*
*
* repeated .google.cloud.dialogflow.v2.Document.KnowledgeType knowledge_types = 4 [(.google.api.field_behavior) = REQUIRED];
*
*
* @param index The index of the element to return.
* @return The knowledgeTypes at the given index.
*/
com.google.cloud.dialogflow.v2.Document.KnowledgeType getKnowledgeTypes(int index);
/**
*
*
*
* Required. The knowledge type of document content.
*
*
*
* repeated .google.cloud.dialogflow.v2.Document.KnowledgeType knowledge_types = 4 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return A list containing the enum numeric values on the wire for knowledgeTypes.
*/
java.util.List getKnowledgeTypesValueList();
/**
*
*
*
* Required. The knowledge type of document content.
*
*
*
* repeated .google.cloud.dialogflow.v2.Document.KnowledgeType knowledge_types = 4 [(.google.api.field_behavior) = REQUIRED];
*
*
* @param index The index of the value to return.
* @return The enum numeric value on the wire of knowledgeTypes at the given index.
*/
int getKnowledgeTypesValue(int index);
/**
*
*
*
* The URI where the file content is located.
*
* For documents stored in Google Cloud Storage, these URIs must have
* the form `gs://<bucket-name>/<object-name>`.
*
* NOTE: External URLs must correspond to public webpages, i.e., they must
* be indexed by Google Search. In particular, URLs for showing documents in
* Google Cloud Storage (i.e. the URL in your browser) are not supported.
* Instead use the `gs://` format URI described above.
*
*
* string content_uri = 5;
*
* @return Whether the contentUri field is set.
*/
boolean hasContentUri();
/**
*
*
*
* The URI where the file content is located.
*
* For documents stored in Google Cloud Storage, these URIs must have
* the form `gs://<bucket-name>/<object-name>`.
*
* NOTE: External URLs must correspond to public webpages, i.e., they must
* be indexed by Google Search. In particular, URLs for showing documents in
* Google Cloud Storage (i.e. the URL in your browser) are not supported.
* Instead use the `gs://` format URI described above.
*
*
* string content_uri = 5;
*
* @return The contentUri.
*/
java.lang.String getContentUri();
/**
*
*
*
* The URI where the file content is located.
*
* For documents stored in Google Cloud Storage, these URIs must have
* the form `gs://<bucket-name>/<object-name>`.
*
* NOTE: External URLs must correspond to public webpages, i.e., they must
* be indexed by Google Search. In particular, URLs for showing documents in
* Google Cloud Storage (i.e. the URL in your browser) are not supported.
* Instead use the `gs://` format URI described above.
*
*
* string content_uri = 5;
*
* @return The bytes for contentUri.
*/
com.google.protobuf.ByteString getContentUriBytes();
/**
*
*
*
* The raw content of the document. This field is only permitted for
* EXTRACTIVE_QA and FAQ knowledge types.
*
*
* bytes raw_content = 9;
*
* @return Whether the rawContent field is set.
*/
boolean hasRawContent();
/**
*
*
*
* The raw content of the document. This field is only permitted for
* EXTRACTIVE_QA and FAQ knowledge types.
*
*
* bytes raw_content = 9;
*
* @return The rawContent.
*/
com.google.protobuf.ByteString getRawContent();
/**
*
*
*
* Optional. If true, we try to automatically reload the document every day
* (at a time picked by the system). If false or unspecified, we don't try
* to automatically reload the document.
*
* Currently you can only enable automatic reload for documents sourced from
* a public url, see `source` field for the source types.
*
* Reload status can be tracked in `latest_reload_status`. If a reload
* fails, we will keep the document unchanged.
*
* If a reload fails with internal errors, the system will try to reload the
* document on the next day.
* If a reload fails with non-retriable errors (e.g. PERMISSION_DENIED), the
* system will not try to reload the document anymore. You need to manually
* reload the document successfully by calling `ReloadDocument` and clear the
* errors.
*
*
* bool enable_auto_reload = 11 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The enableAutoReload.
*/
boolean getEnableAutoReload();
/**
*
*
*
* Output only. The time and status of the latest reload.
* This reload may have been triggered automatically or manually
* and may not have succeeded.
*
*
*
* .google.cloud.dialogflow.v2.Document.ReloadStatus latest_reload_status = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the latestReloadStatus field is set.
*/
boolean hasLatestReloadStatus();
/**
*
*
*
* Output only. The time and status of the latest reload.
* This reload may have been triggered automatically or manually
* and may not have succeeded.
*
*
*
* .google.cloud.dialogflow.v2.Document.ReloadStatus latest_reload_status = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The latestReloadStatus.
*/
com.google.cloud.dialogflow.v2.Document.ReloadStatus getLatestReloadStatus();
/**
*
*
*
* Output only. The time and status of the latest reload.
* This reload may have been triggered automatically or manually
* and may not have succeeded.
*
*
*
* .google.cloud.dialogflow.v2.Document.ReloadStatus latest_reload_status = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.cloud.dialogflow.v2.Document.ReloadStatusOrBuilder getLatestReloadStatusOrBuilder();
/**
*
*
*
* Optional. Metadata for the document. The metadata supports arbitrary
* key-value pairs. Suggested use cases include storing a document's title,
* an external URL distinct from the document's content_uri, etc.
* The max size of a `key` or a `value` of the metadata is 1024 bytes.
*
*
* map<string, string> metadata = 7 [(.google.api.field_behavior) = OPTIONAL];
*/
int getMetadataCount();
/**
*
*
*
* Optional. Metadata for the document. The metadata supports arbitrary
* key-value pairs. Suggested use cases include storing a document's title,
* an external URL distinct from the document's content_uri, etc.
* The max size of a `key` or a `value` of the metadata is 1024 bytes.
*
*
* map<string, string> metadata = 7 [(.google.api.field_behavior) = OPTIONAL];
*/
boolean containsMetadata(java.lang.String key);
/** Use {@link #getMetadataMap()} instead. */
@java.lang.Deprecated
java.util.Map getMetadata();
/**
*
*
*
* Optional. Metadata for the document. The metadata supports arbitrary
* key-value pairs. Suggested use cases include storing a document's title,
* an external URL distinct from the document's content_uri, etc.
* The max size of a `key` or a `value` of the metadata is 1024 bytes.
*
*
* map<string, string> metadata = 7 [(.google.api.field_behavior) = OPTIONAL];
*/
java.util.Map getMetadataMap();
/**
*
*
*
* Optional. Metadata for the document. The metadata supports arbitrary
* key-value pairs. Suggested use cases include storing a document's title,
* an external URL distinct from the document's content_uri, etc.
* The max size of a `key` or a `value` of the metadata is 1024 bytes.
*
*
* map<string, string> metadata = 7 [(.google.api.field_behavior) = OPTIONAL];
*/
/* nullable */
java.lang.String getMetadataOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
*
*
*
* Optional. Metadata for the document. The metadata supports arbitrary
* key-value pairs. Suggested use cases include storing a document's title,
* an external URL distinct from the document's content_uri, etc.
* The max size of a `key` or a `value` of the metadata is 1024 bytes.
*
*
* map<string, string> metadata = 7 [(.google.api.field_behavior) = OPTIONAL];
*/
java.lang.String getMetadataOrThrow(java.lang.String key);
/**
*
*
*
* Output only. The current state of the document.
*
*
*
* .google.cloud.dialogflow.v2.Document.State state = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The enum numeric value on the wire for state.
*/
int getStateValue();
/**
*
*
*
* Output only. The current state of the document.
*
*
*
* .google.cloud.dialogflow.v2.Document.State state = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The state.
*/
com.google.cloud.dialogflow.v2.Document.State getState();
com.google.cloud.dialogflow.v2.Document.SourceCase getSourceCase();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy