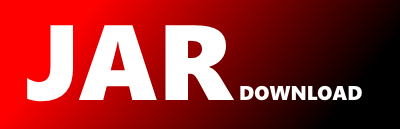
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-dialogflow-v2 Show documentation
Show all versions of proto-google-cloud-dialogflow-v2 Show documentation
PROTO library for proto-google-cloud-dialogflow-v2
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/dialogflow/v2/participant.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.dialogflow.v2;
/**
*
*
*
* The top-level message returned from the `StreamingAnalyzeContent` method.
*
* Multiple response messages can be returned in order:
*
* 1. If the input was set to streaming audio, the first one or more messages
* contain `recognition_result`. Each `recognition_result` represents a more
* complete transcript of what the user said. The last `recognition_result`
* has `is_final` set to `true`.
*
* 2. In virtual agent stage: if `enable_partial_automated_agent_reply` is
* true, the following N (currently 1 <= N <= 4) messages
* contain `automated_agent_reply` and optionally `reply_audio`
* returned by the virtual agent. The first (N-1)
* `automated_agent_reply`s will have `automated_agent_reply_type` set to
* `PARTIAL`. The last `automated_agent_reply` has
* `automated_agent_reply_type` set to `FINAL`.
* If `enable_partial_automated_agent_reply` is not enabled, response stream
* only contains the final reply.
*
* In human assist stage: the following N (N >= 1) messages contain
* `human_agent_suggestion_results`, `end_user_suggestion_results` or
* `message`.
*
*
* Protobuf type {@code google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse}
*/
public final class StreamingAnalyzeContentResponse extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse)
StreamingAnalyzeContentResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use StreamingAnalyzeContentResponse.newBuilder() to construct.
private StreamingAnalyzeContentResponse(
com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StreamingAnalyzeContentResponse() {
replyText_ = "";
humanAgentSuggestionResults_ = java.util.Collections.emptyList();
endUserSuggestionResults_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new StreamingAnalyzeContentResponse();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.dialogflow.v2.ParticipantProto
.internal_static_google_cloud_dialogflow_v2_StreamingAnalyzeContentResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.dialogflow.v2.ParticipantProto
.internal_static_google_cloud_dialogflow_v2_StreamingAnalyzeContentResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.class,
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.Builder.class);
}
private int bitField0_;
public static final int RECOGNITION_RESULT_FIELD_NUMBER = 1;
private com.google.cloud.dialogflow.v2.StreamingRecognitionResult recognitionResult_;
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*
* @return Whether the recognitionResult field is set.
*/
@java.lang.Override
public boolean hasRecognitionResult() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*
* @return The recognitionResult.
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.StreamingRecognitionResult getRecognitionResult() {
return recognitionResult_ == null
? com.google.cloud.dialogflow.v2.StreamingRecognitionResult.getDefaultInstance()
: recognitionResult_;
}
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.StreamingRecognitionResultOrBuilder
getRecognitionResultOrBuilder() {
return recognitionResult_ == null
? com.google.cloud.dialogflow.v2.StreamingRecognitionResult.getDefaultInstance()
: recognitionResult_;
}
public static final int REPLY_TEXT_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object replyText_ = "";
/**
*
*
*
* The output text content.
* This field is set if an automated agent responded with a text for the user.
*
*
* string reply_text = 2;
*
* @return The replyText.
*/
@java.lang.Override
public java.lang.String getReplyText() {
java.lang.Object ref = replyText_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
replyText_ = s;
return s;
}
}
/**
*
*
*
* The output text content.
* This field is set if an automated agent responded with a text for the user.
*
*
* string reply_text = 2;
*
* @return The bytes for replyText.
*/
@java.lang.Override
public com.google.protobuf.ByteString getReplyTextBytes() {
java.lang.Object ref = replyText_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
replyText_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REPLY_AUDIO_FIELD_NUMBER = 3;
private com.google.cloud.dialogflow.v2.OutputAudio replyAudio_;
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*
* @return Whether the replyAudio field is set.
*/
@java.lang.Override
public boolean hasReplyAudio() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*
* @return The replyAudio.
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.OutputAudio getReplyAudio() {
return replyAudio_ == null
? com.google.cloud.dialogflow.v2.OutputAudio.getDefaultInstance()
: replyAudio_;
}
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.OutputAudioOrBuilder getReplyAudioOrBuilder() {
return replyAudio_ == null
? com.google.cloud.dialogflow.v2.OutputAudio.getDefaultInstance()
: replyAudio_;
}
public static final int AUTOMATED_AGENT_REPLY_FIELD_NUMBER = 4;
private com.google.cloud.dialogflow.v2.AutomatedAgentReply automatedAgentReply_;
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*
* @return Whether the automatedAgentReply field is set.
*/
@java.lang.Override
public boolean hasAutomatedAgentReply() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*
* @return The automatedAgentReply.
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.AutomatedAgentReply getAutomatedAgentReply() {
return automatedAgentReply_ == null
? com.google.cloud.dialogflow.v2.AutomatedAgentReply.getDefaultInstance()
: automatedAgentReply_;
}
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.AutomatedAgentReplyOrBuilder
getAutomatedAgentReplyOrBuilder() {
return automatedAgentReply_ == null
? com.google.cloud.dialogflow.v2.AutomatedAgentReply.getDefaultInstance()
: automatedAgentReply_;
}
public static final int MESSAGE_FIELD_NUMBER = 6;
private com.google.cloud.dialogflow.v2.Message message_;
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*
* @return Whether the message field is set.
*/
@java.lang.Override
public boolean hasMessage() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*
* @return The message.
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.Message getMessage() {
return message_ == null
? com.google.cloud.dialogflow.v2.Message.getDefaultInstance()
: message_;
}
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.MessageOrBuilder getMessageOrBuilder() {
return message_ == null
? com.google.cloud.dialogflow.v2.Message.getDefaultInstance()
: message_;
}
public static final int HUMAN_AGENT_SUGGESTION_RESULTS_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private java.util.List
humanAgentSuggestionResults_;
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
@java.lang.Override
public java.util.List
getHumanAgentSuggestionResultsList() {
return humanAgentSuggestionResults_;
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
@java.lang.Override
public java.util.List extends com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder>
getHumanAgentSuggestionResultsOrBuilderList() {
return humanAgentSuggestionResults_;
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
@java.lang.Override
public int getHumanAgentSuggestionResultsCount() {
return humanAgentSuggestionResults_.size();
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.SuggestionResult getHumanAgentSuggestionResults(int index) {
return humanAgentSuggestionResults_.get(index);
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder
getHumanAgentSuggestionResultsOrBuilder(int index) {
return humanAgentSuggestionResults_.get(index);
}
public static final int END_USER_SUGGESTION_RESULTS_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private java.util.List endUserSuggestionResults_;
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
@java.lang.Override
public java.util.List
getEndUserSuggestionResultsList() {
return endUserSuggestionResults_;
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
@java.lang.Override
public java.util.List extends com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder>
getEndUserSuggestionResultsOrBuilderList() {
return endUserSuggestionResults_;
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
@java.lang.Override
public int getEndUserSuggestionResultsCount() {
return endUserSuggestionResults_.size();
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.SuggestionResult getEndUserSuggestionResults(int index) {
return endUserSuggestionResults_.get(index);
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder
getEndUserSuggestionResultsOrBuilder(int index) {
return endUserSuggestionResults_.get(index);
}
public static final int DTMF_PARAMETERS_FIELD_NUMBER = 10;
private com.google.cloud.dialogflow.v2.DtmfParameters dtmfParameters_;
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*
* @return Whether the dtmfParameters field is set.
*/
@java.lang.Override
public boolean hasDtmfParameters() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*
* @return The dtmfParameters.
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.DtmfParameters getDtmfParameters() {
return dtmfParameters_ == null
? com.google.cloud.dialogflow.v2.DtmfParameters.getDefaultInstance()
: dtmfParameters_;
}
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.DtmfParametersOrBuilder getDtmfParametersOrBuilder() {
return dtmfParameters_ == null
? com.google.cloud.dialogflow.v2.DtmfParameters.getDefaultInstance()
: dtmfParameters_;
}
public static final int DEBUGGING_INFO_FIELD_NUMBER = 11;
private com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debuggingInfo_;
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*
* @return Whether the debuggingInfo field is set.
*/
@java.lang.Override
public boolean hasDebuggingInfo() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*
* @return The debuggingInfo.
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo getDebuggingInfo() {
return debuggingInfo_ == null
? com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo.getDefaultInstance()
: debuggingInfo_;
}
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*/
@java.lang.Override
public com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfoOrBuilder
getDebuggingInfoOrBuilder() {
return debuggingInfo_ == null
? com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo.getDefaultInstance()
: debuggingInfo_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getRecognitionResult());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(replyText_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, replyText_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(3, getReplyAudio());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(4, getAutomatedAgentReply());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(6, getMessage());
}
for (int i = 0; i < humanAgentSuggestionResults_.size(); i++) {
output.writeMessage(7, humanAgentSuggestionResults_.get(i));
}
for (int i = 0; i < endUserSuggestionResults_.size(); i++) {
output.writeMessage(8, endUserSuggestionResults_.get(i));
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(10, getDtmfParameters());
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(11, getDebuggingInfo());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(1, getRecognitionResult());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(replyText_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, replyText_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(3, getReplyAudio());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(4, getAutomatedAgentReply());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(6, getMessage());
}
for (int i = 0; i < humanAgentSuggestionResults_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
7, humanAgentSuggestionResults_.get(i));
}
for (int i = 0; i < endUserSuggestionResults_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
8, endUserSuggestionResults_.get(i));
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(10, getDtmfParameters());
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(11, getDebuggingInfo());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse)) {
return super.equals(obj);
}
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse other =
(com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse) obj;
if (hasRecognitionResult() != other.hasRecognitionResult()) return false;
if (hasRecognitionResult()) {
if (!getRecognitionResult().equals(other.getRecognitionResult())) return false;
}
if (!getReplyText().equals(other.getReplyText())) return false;
if (hasReplyAudio() != other.hasReplyAudio()) return false;
if (hasReplyAudio()) {
if (!getReplyAudio().equals(other.getReplyAudio())) return false;
}
if (hasAutomatedAgentReply() != other.hasAutomatedAgentReply()) return false;
if (hasAutomatedAgentReply()) {
if (!getAutomatedAgentReply().equals(other.getAutomatedAgentReply())) return false;
}
if (hasMessage() != other.hasMessage()) return false;
if (hasMessage()) {
if (!getMessage().equals(other.getMessage())) return false;
}
if (!getHumanAgentSuggestionResultsList().equals(other.getHumanAgentSuggestionResultsList()))
return false;
if (!getEndUserSuggestionResultsList().equals(other.getEndUserSuggestionResultsList()))
return false;
if (hasDtmfParameters() != other.hasDtmfParameters()) return false;
if (hasDtmfParameters()) {
if (!getDtmfParameters().equals(other.getDtmfParameters())) return false;
}
if (hasDebuggingInfo() != other.hasDebuggingInfo()) return false;
if (hasDebuggingInfo()) {
if (!getDebuggingInfo().equals(other.getDebuggingInfo())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRecognitionResult()) {
hash = (37 * hash) + RECOGNITION_RESULT_FIELD_NUMBER;
hash = (53 * hash) + getRecognitionResult().hashCode();
}
hash = (37 * hash) + REPLY_TEXT_FIELD_NUMBER;
hash = (53 * hash) + getReplyText().hashCode();
if (hasReplyAudio()) {
hash = (37 * hash) + REPLY_AUDIO_FIELD_NUMBER;
hash = (53 * hash) + getReplyAudio().hashCode();
}
if (hasAutomatedAgentReply()) {
hash = (37 * hash) + AUTOMATED_AGENT_REPLY_FIELD_NUMBER;
hash = (53 * hash) + getAutomatedAgentReply().hashCode();
}
if (hasMessage()) {
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
}
if (getHumanAgentSuggestionResultsCount() > 0) {
hash = (37 * hash) + HUMAN_AGENT_SUGGESTION_RESULTS_FIELD_NUMBER;
hash = (53 * hash) + getHumanAgentSuggestionResultsList().hashCode();
}
if (getEndUserSuggestionResultsCount() > 0) {
hash = (37 * hash) + END_USER_SUGGESTION_RESULTS_FIELD_NUMBER;
hash = (53 * hash) + getEndUserSuggestionResultsList().hashCode();
}
if (hasDtmfParameters()) {
hash = (37 * hash) + DTMF_PARAMETERS_FIELD_NUMBER;
hash = (53 * hash) + getDtmfParameters().hashCode();
}
if (hasDebuggingInfo()) {
hash = (37 * hash) + DEBUGGING_INFO_FIELD_NUMBER;
hash = (53 * hash) + getDebuggingInfo().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseFrom(
byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* The top-level message returned from the `StreamingAnalyzeContent` method.
*
* Multiple response messages can be returned in order:
*
* 1. If the input was set to streaming audio, the first one or more messages
* contain `recognition_result`. Each `recognition_result` represents a more
* complete transcript of what the user said. The last `recognition_result`
* has `is_final` set to `true`.
*
* 2. In virtual agent stage: if `enable_partial_automated_agent_reply` is
* true, the following N (currently 1 <= N <= 4) messages
* contain `automated_agent_reply` and optionally `reply_audio`
* returned by the virtual agent. The first (N-1)
* `automated_agent_reply`s will have `automated_agent_reply_type` set to
* `PARTIAL`. The last `automated_agent_reply` has
* `automated_agent_reply_type` set to `FINAL`.
* If `enable_partial_automated_agent_reply` is not enabled, response stream
* only contains the final reply.
*
* In human assist stage: the following N (N >= 1) messages contain
* `human_agent_suggestion_results`, `end_user_suggestion_results` or
* `message`.
*
*
* Protobuf type {@code google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse)
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.dialogflow.v2.ParticipantProto
.internal_static_google_cloud_dialogflow_v2_StreamingAnalyzeContentResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.dialogflow.v2.ParticipantProto
.internal_static_google_cloud_dialogflow_v2_StreamingAnalyzeContentResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.class,
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.Builder.class);
}
// Construct using com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getRecognitionResultFieldBuilder();
getReplyAudioFieldBuilder();
getAutomatedAgentReplyFieldBuilder();
getMessageFieldBuilder();
getHumanAgentSuggestionResultsFieldBuilder();
getEndUserSuggestionResultsFieldBuilder();
getDtmfParametersFieldBuilder();
getDebuggingInfoFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
recognitionResult_ = null;
if (recognitionResultBuilder_ != null) {
recognitionResultBuilder_.dispose();
recognitionResultBuilder_ = null;
}
replyText_ = "";
replyAudio_ = null;
if (replyAudioBuilder_ != null) {
replyAudioBuilder_.dispose();
replyAudioBuilder_ = null;
}
automatedAgentReply_ = null;
if (automatedAgentReplyBuilder_ != null) {
automatedAgentReplyBuilder_.dispose();
automatedAgentReplyBuilder_ = null;
}
message_ = null;
if (messageBuilder_ != null) {
messageBuilder_.dispose();
messageBuilder_ = null;
}
if (humanAgentSuggestionResultsBuilder_ == null) {
humanAgentSuggestionResults_ = java.util.Collections.emptyList();
} else {
humanAgentSuggestionResults_ = null;
humanAgentSuggestionResultsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
if (endUserSuggestionResultsBuilder_ == null) {
endUserSuggestionResults_ = java.util.Collections.emptyList();
} else {
endUserSuggestionResults_ = null;
endUserSuggestionResultsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
dtmfParameters_ = null;
if (dtmfParametersBuilder_ != null) {
dtmfParametersBuilder_.dispose();
dtmfParametersBuilder_ = null;
}
debuggingInfo_ = null;
if (debuggingInfoBuilder_ != null) {
debuggingInfoBuilder_.dispose();
debuggingInfoBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.dialogflow.v2.ParticipantProto
.internal_static_google_cloud_dialogflow_v2_StreamingAnalyzeContentResponse_descriptor;
}
@java.lang.Override
public com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse
getDefaultInstanceForType() {
return com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse build() {
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse buildPartial() {
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse result =
new com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse result) {
if (humanAgentSuggestionResultsBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)) {
humanAgentSuggestionResults_ =
java.util.Collections.unmodifiableList(humanAgentSuggestionResults_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.humanAgentSuggestionResults_ = humanAgentSuggestionResults_;
} else {
result.humanAgentSuggestionResults_ = humanAgentSuggestionResultsBuilder_.build();
}
if (endUserSuggestionResultsBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
endUserSuggestionResults_ =
java.util.Collections.unmodifiableList(endUserSuggestionResults_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.endUserSuggestionResults_ = endUserSuggestionResults_;
} else {
result.endUserSuggestionResults_ = endUserSuggestionResultsBuilder_.build();
}
}
private void buildPartial0(
com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.recognitionResult_ =
recognitionResultBuilder_ == null
? recognitionResult_
: recognitionResultBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.replyText_ = replyText_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.replyAudio_ = replyAudioBuilder_ == null ? replyAudio_ : replyAudioBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.automatedAgentReply_ =
automatedAgentReplyBuilder_ == null
? automatedAgentReply_
: automatedAgentReplyBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.message_ = messageBuilder_ == null ? message_ : messageBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.dtmfParameters_ =
dtmfParametersBuilder_ == null ? dtmfParameters_ : dtmfParametersBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.debuggingInfo_ =
debuggingInfoBuilder_ == null ? debuggingInfo_ : debuggingInfoBuilder_.build();
to_bitField0_ |= 0x00000020;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse) {
return mergeFrom((com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse other) {
if (other
== com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.getDefaultInstance())
return this;
if (other.hasRecognitionResult()) {
mergeRecognitionResult(other.getRecognitionResult());
}
if (!other.getReplyText().isEmpty()) {
replyText_ = other.replyText_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasReplyAudio()) {
mergeReplyAudio(other.getReplyAudio());
}
if (other.hasAutomatedAgentReply()) {
mergeAutomatedAgentReply(other.getAutomatedAgentReply());
}
if (other.hasMessage()) {
mergeMessage(other.getMessage());
}
if (humanAgentSuggestionResultsBuilder_ == null) {
if (!other.humanAgentSuggestionResults_.isEmpty()) {
if (humanAgentSuggestionResults_.isEmpty()) {
humanAgentSuggestionResults_ = other.humanAgentSuggestionResults_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureHumanAgentSuggestionResultsIsMutable();
humanAgentSuggestionResults_.addAll(other.humanAgentSuggestionResults_);
}
onChanged();
}
} else {
if (!other.humanAgentSuggestionResults_.isEmpty()) {
if (humanAgentSuggestionResultsBuilder_.isEmpty()) {
humanAgentSuggestionResultsBuilder_.dispose();
humanAgentSuggestionResultsBuilder_ = null;
humanAgentSuggestionResults_ = other.humanAgentSuggestionResults_;
bitField0_ = (bitField0_ & ~0x00000020);
humanAgentSuggestionResultsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getHumanAgentSuggestionResultsFieldBuilder()
: null;
} else {
humanAgentSuggestionResultsBuilder_.addAllMessages(other.humanAgentSuggestionResults_);
}
}
}
if (endUserSuggestionResultsBuilder_ == null) {
if (!other.endUserSuggestionResults_.isEmpty()) {
if (endUserSuggestionResults_.isEmpty()) {
endUserSuggestionResults_ = other.endUserSuggestionResults_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureEndUserSuggestionResultsIsMutable();
endUserSuggestionResults_.addAll(other.endUserSuggestionResults_);
}
onChanged();
}
} else {
if (!other.endUserSuggestionResults_.isEmpty()) {
if (endUserSuggestionResultsBuilder_.isEmpty()) {
endUserSuggestionResultsBuilder_.dispose();
endUserSuggestionResultsBuilder_ = null;
endUserSuggestionResults_ = other.endUserSuggestionResults_;
bitField0_ = (bitField0_ & ~0x00000040);
endUserSuggestionResultsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getEndUserSuggestionResultsFieldBuilder()
: null;
} else {
endUserSuggestionResultsBuilder_.addAllMessages(other.endUserSuggestionResults_);
}
}
}
if (other.hasDtmfParameters()) {
mergeDtmfParameters(other.getDtmfParameters());
}
if (other.hasDebuggingInfo()) {
mergeDebuggingInfo(other.getDebuggingInfo());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
input.readMessage(
getRecognitionResultFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
replyText_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26:
{
input.readMessage(getReplyAudioFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 34:
{
input.readMessage(
getAutomatedAgentReplyFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 50:
{
input.readMessage(getMessageFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 50
case 58:
{
com.google.cloud.dialogflow.v2.SuggestionResult m =
input.readMessage(
com.google.cloud.dialogflow.v2.SuggestionResult.parser(),
extensionRegistry);
if (humanAgentSuggestionResultsBuilder_ == null) {
ensureHumanAgentSuggestionResultsIsMutable();
humanAgentSuggestionResults_.add(m);
} else {
humanAgentSuggestionResultsBuilder_.addMessage(m);
}
break;
} // case 58
case 66:
{
com.google.cloud.dialogflow.v2.SuggestionResult m =
input.readMessage(
com.google.cloud.dialogflow.v2.SuggestionResult.parser(),
extensionRegistry);
if (endUserSuggestionResultsBuilder_ == null) {
ensureEndUserSuggestionResultsIsMutable();
endUserSuggestionResults_.add(m);
} else {
endUserSuggestionResultsBuilder_.addMessage(m);
}
break;
} // case 66
case 82:
{
input.readMessage(getDtmfParametersFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 82
case 90:
{
input.readMessage(getDebuggingInfoFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 90
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.cloud.dialogflow.v2.StreamingRecognitionResult recognitionResult_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.StreamingRecognitionResult,
com.google.cloud.dialogflow.v2.StreamingRecognitionResult.Builder,
com.google.cloud.dialogflow.v2.StreamingRecognitionResultOrBuilder>
recognitionResultBuilder_;
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*
* @return Whether the recognitionResult field is set.
*/
public boolean hasRecognitionResult() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*
* @return The recognitionResult.
*/
public com.google.cloud.dialogflow.v2.StreamingRecognitionResult getRecognitionResult() {
if (recognitionResultBuilder_ == null) {
return recognitionResult_ == null
? com.google.cloud.dialogflow.v2.StreamingRecognitionResult.getDefaultInstance()
: recognitionResult_;
} else {
return recognitionResultBuilder_.getMessage();
}
}
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*/
public Builder setRecognitionResult(
com.google.cloud.dialogflow.v2.StreamingRecognitionResult value) {
if (recognitionResultBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
recognitionResult_ = value;
} else {
recognitionResultBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*/
public Builder setRecognitionResult(
com.google.cloud.dialogflow.v2.StreamingRecognitionResult.Builder builderForValue) {
if (recognitionResultBuilder_ == null) {
recognitionResult_ = builderForValue.build();
} else {
recognitionResultBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*/
public Builder mergeRecognitionResult(
com.google.cloud.dialogflow.v2.StreamingRecognitionResult value) {
if (recognitionResultBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)
&& recognitionResult_ != null
&& recognitionResult_
!= com.google.cloud.dialogflow.v2.StreamingRecognitionResult.getDefaultInstance()) {
getRecognitionResultBuilder().mergeFrom(value);
} else {
recognitionResult_ = value;
}
} else {
recognitionResultBuilder_.mergeFrom(value);
}
if (recognitionResult_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*/
public Builder clearRecognitionResult() {
bitField0_ = (bitField0_ & ~0x00000001);
recognitionResult_ = null;
if (recognitionResultBuilder_ != null) {
recognitionResultBuilder_.dispose();
recognitionResultBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*/
public com.google.cloud.dialogflow.v2.StreamingRecognitionResult.Builder
getRecognitionResultBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getRecognitionResultFieldBuilder().getBuilder();
}
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*/
public com.google.cloud.dialogflow.v2.StreamingRecognitionResultOrBuilder
getRecognitionResultOrBuilder() {
if (recognitionResultBuilder_ != null) {
return recognitionResultBuilder_.getMessageOrBuilder();
} else {
return recognitionResult_ == null
? com.google.cloud.dialogflow.v2.StreamingRecognitionResult.getDefaultInstance()
: recognitionResult_;
}
}
/**
*
*
*
* The result of speech recognition.
*
*
* .google.cloud.dialogflow.v2.StreamingRecognitionResult recognition_result = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.StreamingRecognitionResult,
com.google.cloud.dialogflow.v2.StreamingRecognitionResult.Builder,
com.google.cloud.dialogflow.v2.StreamingRecognitionResultOrBuilder>
getRecognitionResultFieldBuilder() {
if (recognitionResultBuilder_ == null) {
recognitionResultBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.StreamingRecognitionResult,
com.google.cloud.dialogflow.v2.StreamingRecognitionResult.Builder,
com.google.cloud.dialogflow.v2.StreamingRecognitionResultOrBuilder>(
getRecognitionResult(), getParentForChildren(), isClean());
recognitionResult_ = null;
}
return recognitionResultBuilder_;
}
private java.lang.Object replyText_ = "";
/**
*
*
*
* The output text content.
* This field is set if an automated agent responded with a text for the user.
*
*
* string reply_text = 2;
*
* @return The replyText.
*/
public java.lang.String getReplyText() {
java.lang.Object ref = replyText_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
replyText_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The output text content.
* This field is set if an automated agent responded with a text for the user.
*
*
* string reply_text = 2;
*
* @return The bytes for replyText.
*/
public com.google.protobuf.ByteString getReplyTextBytes() {
java.lang.Object ref = replyText_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
replyText_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The output text content.
* This field is set if an automated agent responded with a text for the user.
*
*
* string reply_text = 2;
*
* @param value The replyText to set.
* @return This builder for chaining.
*/
public Builder setReplyText(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
replyText_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* The output text content.
* This field is set if an automated agent responded with a text for the user.
*
*
* string reply_text = 2;
*
* @return This builder for chaining.
*/
public Builder clearReplyText() {
replyText_ = getDefaultInstance().getReplyText();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* The output text content.
* This field is set if an automated agent responded with a text for the user.
*
*
* string reply_text = 2;
*
* @param value The bytes for replyText to set.
* @return This builder for chaining.
*/
public Builder setReplyTextBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
replyText_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.cloud.dialogflow.v2.OutputAudio replyAudio_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.OutputAudio,
com.google.cloud.dialogflow.v2.OutputAudio.Builder,
com.google.cloud.dialogflow.v2.OutputAudioOrBuilder>
replyAudioBuilder_;
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*
* @return Whether the replyAudio field is set.
*/
public boolean hasReplyAudio() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*
* @return The replyAudio.
*/
public com.google.cloud.dialogflow.v2.OutputAudio getReplyAudio() {
if (replyAudioBuilder_ == null) {
return replyAudio_ == null
? com.google.cloud.dialogflow.v2.OutputAudio.getDefaultInstance()
: replyAudio_;
} else {
return replyAudioBuilder_.getMessage();
}
}
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*/
public Builder setReplyAudio(com.google.cloud.dialogflow.v2.OutputAudio value) {
if (replyAudioBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
replyAudio_ = value;
} else {
replyAudioBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*/
public Builder setReplyAudio(
com.google.cloud.dialogflow.v2.OutputAudio.Builder builderForValue) {
if (replyAudioBuilder_ == null) {
replyAudio_ = builderForValue.build();
} else {
replyAudioBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*/
public Builder mergeReplyAudio(com.google.cloud.dialogflow.v2.OutputAudio value) {
if (replyAudioBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)
&& replyAudio_ != null
&& replyAudio_ != com.google.cloud.dialogflow.v2.OutputAudio.getDefaultInstance()) {
getReplyAudioBuilder().mergeFrom(value);
} else {
replyAudio_ = value;
}
} else {
replyAudioBuilder_.mergeFrom(value);
}
if (replyAudio_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*/
public Builder clearReplyAudio() {
bitField0_ = (bitField0_ & ~0x00000004);
replyAudio_ = null;
if (replyAudioBuilder_ != null) {
replyAudioBuilder_.dispose();
replyAudioBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*/
public com.google.cloud.dialogflow.v2.OutputAudio.Builder getReplyAudioBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getReplyAudioFieldBuilder().getBuilder();
}
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*/
public com.google.cloud.dialogflow.v2.OutputAudioOrBuilder getReplyAudioOrBuilder() {
if (replyAudioBuilder_ != null) {
return replyAudioBuilder_.getMessageOrBuilder();
} else {
return replyAudio_ == null
? com.google.cloud.dialogflow.v2.OutputAudio.getDefaultInstance()
: replyAudio_;
}
}
/**
*
*
*
* The audio data bytes encoded as specified in the request.
* This field is set if:
*
* - The `reply_audio_config` field is specified in the request.
* - The automated agent, which this output comes from, responded with audio.
* In such case, the `reply_audio.config` field contains settings used to
* synthesize the speech.
*
* In some scenarios, multiple output audio fields may be present in the
* response structure. In these cases, only the top-most-level audio output
* has content.
*
*
* .google.cloud.dialogflow.v2.OutputAudio reply_audio = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.OutputAudio,
com.google.cloud.dialogflow.v2.OutputAudio.Builder,
com.google.cloud.dialogflow.v2.OutputAudioOrBuilder>
getReplyAudioFieldBuilder() {
if (replyAudioBuilder_ == null) {
replyAudioBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.OutputAudio,
com.google.cloud.dialogflow.v2.OutputAudio.Builder,
com.google.cloud.dialogflow.v2.OutputAudioOrBuilder>(
getReplyAudio(), getParentForChildren(), isClean());
replyAudio_ = null;
}
return replyAudioBuilder_;
}
private com.google.cloud.dialogflow.v2.AutomatedAgentReply automatedAgentReply_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.AutomatedAgentReply,
com.google.cloud.dialogflow.v2.AutomatedAgentReply.Builder,
com.google.cloud.dialogflow.v2.AutomatedAgentReplyOrBuilder>
automatedAgentReplyBuilder_;
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*
* @return Whether the automatedAgentReply field is set.
*/
public boolean hasAutomatedAgentReply() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*
* @return The automatedAgentReply.
*/
public com.google.cloud.dialogflow.v2.AutomatedAgentReply getAutomatedAgentReply() {
if (automatedAgentReplyBuilder_ == null) {
return automatedAgentReply_ == null
? com.google.cloud.dialogflow.v2.AutomatedAgentReply.getDefaultInstance()
: automatedAgentReply_;
} else {
return automatedAgentReplyBuilder_.getMessage();
}
}
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*/
public Builder setAutomatedAgentReply(
com.google.cloud.dialogflow.v2.AutomatedAgentReply value) {
if (automatedAgentReplyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
automatedAgentReply_ = value;
} else {
automatedAgentReplyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*/
public Builder setAutomatedAgentReply(
com.google.cloud.dialogflow.v2.AutomatedAgentReply.Builder builderForValue) {
if (automatedAgentReplyBuilder_ == null) {
automatedAgentReply_ = builderForValue.build();
} else {
automatedAgentReplyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*/
public Builder mergeAutomatedAgentReply(
com.google.cloud.dialogflow.v2.AutomatedAgentReply value) {
if (automatedAgentReplyBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& automatedAgentReply_ != null
&& automatedAgentReply_
!= com.google.cloud.dialogflow.v2.AutomatedAgentReply.getDefaultInstance()) {
getAutomatedAgentReplyBuilder().mergeFrom(value);
} else {
automatedAgentReply_ = value;
}
} else {
automatedAgentReplyBuilder_.mergeFrom(value);
}
if (automatedAgentReply_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*/
public Builder clearAutomatedAgentReply() {
bitField0_ = (bitField0_ & ~0x00000008);
automatedAgentReply_ = null;
if (automatedAgentReplyBuilder_ != null) {
automatedAgentReplyBuilder_.dispose();
automatedAgentReplyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*/
public com.google.cloud.dialogflow.v2.AutomatedAgentReply.Builder
getAutomatedAgentReplyBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getAutomatedAgentReplyFieldBuilder().getBuilder();
}
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*/
public com.google.cloud.dialogflow.v2.AutomatedAgentReplyOrBuilder
getAutomatedAgentReplyOrBuilder() {
if (automatedAgentReplyBuilder_ != null) {
return automatedAgentReplyBuilder_.getMessageOrBuilder();
} else {
return automatedAgentReply_ == null
? com.google.cloud.dialogflow.v2.AutomatedAgentReply.getDefaultInstance()
: automatedAgentReply_;
}
}
/**
*
*
*
* Only set if a Dialogflow automated agent has responded.
* Note that: [AutomatedAgentReply.detect_intent_response.output_audio][]
* and [AutomatedAgentReply.detect_intent_response.output_audio_config][]
* are always empty, use
* [reply_audio][google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse.reply_audio]
* instead.
*
*
* .google.cloud.dialogflow.v2.AutomatedAgentReply automated_agent_reply = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.AutomatedAgentReply,
com.google.cloud.dialogflow.v2.AutomatedAgentReply.Builder,
com.google.cloud.dialogflow.v2.AutomatedAgentReplyOrBuilder>
getAutomatedAgentReplyFieldBuilder() {
if (automatedAgentReplyBuilder_ == null) {
automatedAgentReplyBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.AutomatedAgentReply,
com.google.cloud.dialogflow.v2.AutomatedAgentReply.Builder,
com.google.cloud.dialogflow.v2.AutomatedAgentReplyOrBuilder>(
getAutomatedAgentReply(), getParentForChildren(), isClean());
automatedAgentReply_ = null;
}
return automatedAgentReplyBuilder_;
}
private com.google.cloud.dialogflow.v2.Message message_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.Message,
com.google.cloud.dialogflow.v2.Message.Builder,
com.google.cloud.dialogflow.v2.MessageOrBuilder>
messageBuilder_;
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*
* @return Whether the message field is set.
*/
public boolean hasMessage() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*
* @return The message.
*/
public com.google.cloud.dialogflow.v2.Message getMessage() {
if (messageBuilder_ == null) {
return message_ == null
? com.google.cloud.dialogflow.v2.Message.getDefaultInstance()
: message_;
} else {
return messageBuilder_.getMessage();
}
}
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*/
public Builder setMessage(com.google.cloud.dialogflow.v2.Message value) {
if (messageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
} else {
messageBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*/
public Builder setMessage(com.google.cloud.dialogflow.v2.Message.Builder builderForValue) {
if (messageBuilder_ == null) {
message_ = builderForValue.build();
} else {
messageBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*/
public Builder mergeMessage(com.google.cloud.dialogflow.v2.Message value) {
if (messageBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)
&& message_ != null
&& message_ != com.google.cloud.dialogflow.v2.Message.getDefaultInstance()) {
getMessageBuilder().mergeFrom(value);
} else {
message_ = value;
}
} else {
messageBuilder_.mergeFrom(value);
}
if (message_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*/
public Builder clearMessage() {
bitField0_ = (bitField0_ & ~0x00000010);
message_ = null;
if (messageBuilder_ != null) {
messageBuilder_.dispose();
messageBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*/
public com.google.cloud.dialogflow.v2.Message.Builder getMessageBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getMessageFieldBuilder().getBuilder();
}
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*/
public com.google.cloud.dialogflow.v2.MessageOrBuilder getMessageOrBuilder() {
if (messageBuilder_ != null) {
return messageBuilder_.getMessageOrBuilder();
} else {
return message_ == null
? com.google.cloud.dialogflow.v2.Message.getDefaultInstance()
: message_;
}
}
/**
*
*
*
* Message analyzed by CCAI.
*
*
* .google.cloud.dialogflow.v2.Message message = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.Message,
com.google.cloud.dialogflow.v2.Message.Builder,
com.google.cloud.dialogflow.v2.MessageOrBuilder>
getMessageFieldBuilder() {
if (messageBuilder_ == null) {
messageBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.Message,
com.google.cloud.dialogflow.v2.Message.Builder,
com.google.cloud.dialogflow.v2.MessageOrBuilder>(
getMessage(), getParentForChildren(), isClean());
message_ = null;
}
return messageBuilder_;
}
private java.util.List
humanAgentSuggestionResults_ = java.util.Collections.emptyList();
private void ensureHumanAgentSuggestionResultsIsMutable() {
if (!((bitField0_ & 0x00000020) != 0)) {
humanAgentSuggestionResults_ =
new java.util.ArrayList(
humanAgentSuggestionResults_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dialogflow.v2.SuggestionResult,
com.google.cloud.dialogflow.v2.SuggestionResult.Builder,
com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder>
humanAgentSuggestionResultsBuilder_;
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public java.util.List
getHumanAgentSuggestionResultsList() {
if (humanAgentSuggestionResultsBuilder_ == null) {
return java.util.Collections.unmodifiableList(humanAgentSuggestionResults_);
} else {
return humanAgentSuggestionResultsBuilder_.getMessageList();
}
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public int getHumanAgentSuggestionResultsCount() {
if (humanAgentSuggestionResultsBuilder_ == null) {
return humanAgentSuggestionResults_.size();
} else {
return humanAgentSuggestionResultsBuilder_.getCount();
}
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public com.google.cloud.dialogflow.v2.SuggestionResult getHumanAgentSuggestionResults(
int index) {
if (humanAgentSuggestionResultsBuilder_ == null) {
return humanAgentSuggestionResults_.get(index);
} else {
return humanAgentSuggestionResultsBuilder_.getMessage(index);
}
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public Builder setHumanAgentSuggestionResults(
int index, com.google.cloud.dialogflow.v2.SuggestionResult value) {
if (humanAgentSuggestionResultsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHumanAgentSuggestionResultsIsMutable();
humanAgentSuggestionResults_.set(index, value);
onChanged();
} else {
humanAgentSuggestionResultsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public Builder setHumanAgentSuggestionResults(
int index, com.google.cloud.dialogflow.v2.SuggestionResult.Builder builderForValue) {
if (humanAgentSuggestionResultsBuilder_ == null) {
ensureHumanAgentSuggestionResultsIsMutable();
humanAgentSuggestionResults_.set(index, builderForValue.build());
onChanged();
} else {
humanAgentSuggestionResultsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public Builder addHumanAgentSuggestionResults(
com.google.cloud.dialogflow.v2.SuggestionResult value) {
if (humanAgentSuggestionResultsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHumanAgentSuggestionResultsIsMutable();
humanAgentSuggestionResults_.add(value);
onChanged();
} else {
humanAgentSuggestionResultsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public Builder addHumanAgentSuggestionResults(
int index, com.google.cloud.dialogflow.v2.SuggestionResult value) {
if (humanAgentSuggestionResultsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureHumanAgentSuggestionResultsIsMutable();
humanAgentSuggestionResults_.add(index, value);
onChanged();
} else {
humanAgentSuggestionResultsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public Builder addHumanAgentSuggestionResults(
com.google.cloud.dialogflow.v2.SuggestionResult.Builder builderForValue) {
if (humanAgentSuggestionResultsBuilder_ == null) {
ensureHumanAgentSuggestionResultsIsMutable();
humanAgentSuggestionResults_.add(builderForValue.build());
onChanged();
} else {
humanAgentSuggestionResultsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public Builder addHumanAgentSuggestionResults(
int index, com.google.cloud.dialogflow.v2.SuggestionResult.Builder builderForValue) {
if (humanAgentSuggestionResultsBuilder_ == null) {
ensureHumanAgentSuggestionResultsIsMutable();
humanAgentSuggestionResults_.add(index, builderForValue.build());
onChanged();
} else {
humanAgentSuggestionResultsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public Builder addAllHumanAgentSuggestionResults(
java.lang.Iterable extends com.google.cloud.dialogflow.v2.SuggestionResult> values) {
if (humanAgentSuggestionResultsBuilder_ == null) {
ensureHumanAgentSuggestionResultsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, humanAgentSuggestionResults_);
onChanged();
} else {
humanAgentSuggestionResultsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public Builder clearHumanAgentSuggestionResults() {
if (humanAgentSuggestionResultsBuilder_ == null) {
humanAgentSuggestionResults_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
humanAgentSuggestionResultsBuilder_.clear();
}
return this;
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public Builder removeHumanAgentSuggestionResults(int index) {
if (humanAgentSuggestionResultsBuilder_ == null) {
ensureHumanAgentSuggestionResultsIsMutable();
humanAgentSuggestionResults_.remove(index);
onChanged();
} else {
humanAgentSuggestionResultsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public com.google.cloud.dialogflow.v2.SuggestionResult.Builder
getHumanAgentSuggestionResultsBuilder(int index) {
return getHumanAgentSuggestionResultsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder
getHumanAgentSuggestionResultsOrBuilder(int index) {
if (humanAgentSuggestionResultsBuilder_ == null) {
return humanAgentSuggestionResults_.get(index);
} else {
return humanAgentSuggestionResultsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public java.util.List extends com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder>
getHumanAgentSuggestionResultsOrBuilderList() {
if (humanAgentSuggestionResultsBuilder_ != null) {
return humanAgentSuggestionResultsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(humanAgentSuggestionResults_);
}
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public com.google.cloud.dialogflow.v2.SuggestionResult.Builder
addHumanAgentSuggestionResultsBuilder() {
return getHumanAgentSuggestionResultsFieldBuilder()
.addBuilder(com.google.cloud.dialogflow.v2.SuggestionResult.getDefaultInstance());
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public com.google.cloud.dialogflow.v2.SuggestionResult.Builder
addHumanAgentSuggestionResultsBuilder(int index) {
return getHumanAgentSuggestionResultsFieldBuilder()
.addBuilder(index, com.google.cloud.dialogflow.v2.SuggestionResult.getDefaultInstance());
}
/**
*
*
*
* The suggestions for most recent human agent. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.human_agent_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.human_agent_suggestion_config].
*
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult human_agent_suggestion_results = 7;
*
*/
public java.util.List
getHumanAgentSuggestionResultsBuilderList() {
return getHumanAgentSuggestionResultsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dialogflow.v2.SuggestionResult,
com.google.cloud.dialogflow.v2.SuggestionResult.Builder,
com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder>
getHumanAgentSuggestionResultsFieldBuilder() {
if (humanAgentSuggestionResultsBuilder_ == null) {
humanAgentSuggestionResultsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dialogflow.v2.SuggestionResult,
com.google.cloud.dialogflow.v2.SuggestionResult.Builder,
com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder>(
humanAgentSuggestionResults_,
((bitField0_ & 0x00000020) != 0),
getParentForChildren(),
isClean());
humanAgentSuggestionResults_ = null;
}
return humanAgentSuggestionResultsBuilder_;
}
private java.util.List
endUserSuggestionResults_ = java.util.Collections.emptyList();
private void ensureEndUserSuggestionResultsIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
endUserSuggestionResults_ =
new java.util.ArrayList(
endUserSuggestionResults_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dialogflow.v2.SuggestionResult,
com.google.cloud.dialogflow.v2.SuggestionResult.Builder,
com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder>
endUserSuggestionResultsBuilder_;
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public java.util.List
getEndUserSuggestionResultsList() {
if (endUserSuggestionResultsBuilder_ == null) {
return java.util.Collections.unmodifiableList(endUserSuggestionResults_);
} else {
return endUserSuggestionResultsBuilder_.getMessageList();
}
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public int getEndUserSuggestionResultsCount() {
if (endUserSuggestionResultsBuilder_ == null) {
return endUserSuggestionResults_.size();
} else {
return endUserSuggestionResultsBuilder_.getCount();
}
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public com.google.cloud.dialogflow.v2.SuggestionResult getEndUserSuggestionResults(int index) {
if (endUserSuggestionResultsBuilder_ == null) {
return endUserSuggestionResults_.get(index);
} else {
return endUserSuggestionResultsBuilder_.getMessage(index);
}
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public Builder setEndUserSuggestionResults(
int index, com.google.cloud.dialogflow.v2.SuggestionResult value) {
if (endUserSuggestionResultsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEndUserSuggestionResultsIsMutable();
endUserSuggestionResults_.set(index, value);
onChanged();
} else {
endUserSuggestionResultsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public Builder setEndUserSuggestionResults(
int index, com.google.cloud.dialogflow.v2.SuggestionResult.Builder builderForValue) {
if (endUserSuggestionResultsBuilder_ == null) {
ensureEndUserSuggestionResultsIsMutable();
endUserSuggestionResults_.set(index, builderForValue.build());
onChanged();
} else {
endUserSuggestionResultsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public Builder addEndUserSuggestionResults(
com.google.cloud.dialogflow.v2.SuggestionResult value) {
if (endUserSuggestionResultsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEndUserSuggestionResultsIsMutable();
endUserSuggestionResults_.add(value);
onChanged();
} else {
endUserSuggestionResultsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public Builder addEndUserSuggestionResults(
int index, com.google.cloud.dialogflow.v2.SuggestionResult value) {
if (endUserSuggestionResultsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEndUserSuggestionResultsIsMutable();
endUserSuggestionResults_.add(index, value);
onChanged();
} else {
endUserSuggestionResultsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public Builder addEndUserSuggestionResults(
com.google.cloud.dialogflow.v2.SuggestionResult.Builder builderForValue) {
if (endUserSuggestionResultsBuilder_ == null) {
ensureEndUserSuggestionResultsIsMutable();
endUserSuggestionResults_.add(builderForValue.build());
onChanged();
} else {
endUserSuggestionResultsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public Builder addEndUserSuggestionResults(
int index, com.google.cloud.dialogflow.v2.SuggestionResult.Builder builderForValue) {
if (endUserSuggestionResultsBuilder_ == null) {
ensureEndUserSuggestionResultsIsMutable();
endUserSuggestionResults_.add(index, builderForValue.build());
onChanged();
} else {
endUserSuggestionResultsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public Builder addAllEndUserSuggestionResults(
java.lang.Iterable extends com.google.cloud.dialogflow.v2.SuggestionResult> values) {
if (endUserSuggestionResultsBuilder_ == null) {
ensureEndUserSuggestionResultsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, endUserSuggestionResults_);
onChanged();
} else {
endUserSuggestionResultsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public Builder clearEndUserSuggestionResults() {
if (endUserSuggestionResultsBuilder_ == null) {
endUserSuggestionResults_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
endUserSuggestionResultsBuilder_.clear();
}
return this;
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public Builder removeEndUserSuggestionResults(int index) {
if (endUserSuggestionResultsBuilder_ == null) {
ensureEndUserSuggestionResultsIsMutable();
endUserSuggestionResults_.remove(index);
onChanged();
} else {
endUserSuggestionResultsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public com.google.cloud.dialogflow.v2.SuggestionResult.Builder
getEndUserSuggestionResultsBuilder(int index) {
return getEndUserSuggestionResultsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder
getEndUserSuggestionResultsOrBuilder(int index) {
if (endUserSuggestionResultsBuilder_ == null) {
return endUserSuggestionResults_.get(index);
} else {
return endUserSuggestionResultsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public java.util.List extends com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder>
getEndUserSuggestionResultsOrBuilderList() {
if (endUserSuggestionResultsBuilder_ != null) {
return endUserSuggestionResultsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(endUserSuggestionResults_);
}
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public com.google.cloud.dialogflow.v2.SuggestionResult.Builder
addEndUserSuggestionResultsBuilder() {
return getEndUserSuggestionResultsFieldBuilder()
.addBuilder(com.google.cloud.dialogflow.v2.SuggestionResult.getDefaultInstance());
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public com.google.cloud.dialogflow.v2.SuggestionResult.Builder
addEndUserSuggestionResultsBuilder(int index) {
return getEndUserSuggestionResultsFieldBuilder()
.addBuilder(index, com.google.cloud.dialogflow.v2.SuggestionResult.getDefaultInstance());
}
/**
*
*
*
* The suggestions for end user. The order is the same as
* [HumanAgentAssistantConfig.SuggestionConfig.feature_configs][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.SuggestionConfig.feature_configs]
* of
* [HumanAgentAssistantConfig.end_user_suggestion_config][google.cloud.dialogflow.v2.HumanAgentAssistantConfig.end_user_suggestion_config].
*
*
* repeated .google.cloud.dialogflow.v2.SuggestionResult end_user_suggestion_results = 8;
*
*/
public java.util.List
getEndUserSuggestionResultsBuilderList() {
return getEndUserSuggestionResultsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dialogflow.v2.SuggestionResult,
com.google.cloud.dialogflow.v2.SuggestionResult.Builder,
com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder>
getEndUserSuggestionResultsFieldBuilder() {
if (endUserSuggestionResultsBuilder_ == null) {
endUserSuggestionResultsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.dialogflow.v2.SuggestionResult,
com.google.cloud.dialogflow.v2.SuggestionResult.Builder,
com.google.cloud.dialogflow.v2.SuggestionResultOrBuilder>(
endUserSuggestionResults_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
endUserSuggestionResults_ = null;
}
return endUserSuggestionResultsBuilder_;
}
private com.google.cloud.dialogflow.v2.DtmfParameters dtmfParameters_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.DtmfParameters,
com.google.cloud.dialogflow.v2.DtmfParameters.Builder,
com.google.cloud.dialogflow.v2.DtmfParametersOrBuilder>
dtmfParametersBuilder_;
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*
* @return Whether the dtmfParameters field is set.
*/
public boolean hasDtmfParameters() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*
* @return The dtmfParameters.
*/
public com.google.cloud.dialogflow.v2.DtmfParameters getDtmfParameters() {
if (dtmfParametersBuilder_ == null) {
return dtmfParameters_ == null
? com.google.cloud.dialogflow.v2.DtmfParameters.getDefaultInstance()
: dtmfParameters_;
} else {
return dtmfParametersBuilder_.getMessage();
}
}
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*/
public Builder setDtmfParameters(com.google.cloud.dialogflow.v2.DtmfParameters value) {
if (dtmfParametersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dtmfParameters_ = value;
} else {
dtmfParametersBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*/
public Builder setDtmfParameters(
com.google.cloud.dialogflow.v2.DtmfParameters.Builder builderForValue) {
if (dtmfParametersBuilder_ == null) {
dtmfParameters_ = builderForValue.build();
} else {
dtmfParametersBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*/
public Builder mergeDtmfParameters(com.google.cloud.dialogflow.v2.DtmfParameters value) {
if (dtmfParametersBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)
&& dtmfParameters_ != null
&& dtmfParameters_
!= com.google.cloud.dialogflow.v2.DtmfParameters.getDefaultInstance()) {
getDtmfParametersBuilder().mergeFrom(value);
} else {
dtmfParameters_ = value;
}
} else {
dtmfParametersBuilder_.mergeFrom(value);
}
if (dtmfParameters_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*/
public Builder clearDtmfParameters() {
bitField0_ = (bitField0_ & ~0x00000080);
dtmfParameters_ = null;
if (dtmfParametersBuilder_ != null) {
dtmfParametersBuilder_.dispose();
dtmfParametersBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*/
public com.google.cloud.dialogflow.v2.DtmfParameters.Builder getDtmfParametersBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getDtmfParametersFieldBuilder().getBuilder();
}
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*/
public com.google.cloud.dialogflow.v2.DtmfParametersOrBuilder getDtmfParametersOrBuilder() {
if (dtmfParametersBuilder_ != null) {
return dtmfParametersBuilder_.getMessageOrBuilder();
} else {
return dtmfParameters_ == null
? com.google.cloud.dialogflow.v2.DtmfParameters.getDefaultInstance()
: dtmfParameters_;
}
}
/**
*
*
*
* Indicates the parameters of DTMF.
*
*
* .google.cloud.dialogflow.v2.DtmfParameters dtmf_parameters = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.DtmfParameters,
com.google.cloud.dialogflow.v2.DtmfParameters.Builder,
com.google.cloud.dialogflow.v2.DtmfParametersOrBuilder>
getDtmfParametersFieldBuilder() {
if (dtmfParametersBuilder_ == null) {
dtmfParametersBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.DtmfParameters,
com.google.cloud.dialogflow.v2.DtmfParameters.Builder,
com.google.cloud.dialogflow.v2.DtmfParametersOrBuilder>(
getDtmfParameters(), getParentForChildren(), isClean());
dtmfParameters_ = null;
}
return dtmfParametersBuilder_;
}
private com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debuggingInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo,
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo.Builder,
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfoOrBuilder>
debuggingInfoBuilder_;
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*
* @return Whether the debuggingInfo field is set.
*/
public boolean hasDebuggingInfo() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*
* @return The debuggingInfo.
*/
public com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo getDebuggingInfo() {
if (debuggingInfoBuilder_ == null) {
return debuggingInfo_ == null
? com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo.getDefaultInstance()
: debuggingInfo_;
} else {
return debuggingInfoBuilder_.getMessage();
}
}
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*/
public Builder setDebuggingInfo(
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo value) {
if (debuggingInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
debuggingInfo_ = value;
} else {
debuggingInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*/
public Builder setDebuggingInfo(
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo.Builder builderForValue) {
if (debuggingInfoBuilder_ == null) {
debuggingInfo_ = builderForValue.build();
} else {
debuggingInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*/
public Builder mergeDebuggingInfo(
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo value) {
if (debuggingInfoBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)
&& debuggingInfo_ != null
&& debuggingInfo_
!= com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo
.getDefaultInstance()) {
getDebuggingInfoBuilder().mergeFrom(value);
} else {
debuggingInfo_ = value;
}
} else {
debuggingInfoBuilder_.mergeFrom(value);
}
if (debuggingInfo_ != null) {
bitField0_ |= 0x00000100;
onChanged();
}
return this;
}
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*/
public Builder clearDebuggingInfo() {
bitField0_ = (bitField0_ & ~0x00000100);
debuggingInfo_ = null;
if (debuggingInfoBuilder_ != null) {
debuggingInfoBuilder_.dispose();
debuggingInfoBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*/
public com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo.Builder
getDebuggingInfoBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getDebuggingInfoFieldBuilder().getBuilder();
}
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*/
public com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfoOrBuilder
getDebuggingInfoOrBuilder() {
if (debuggingInfoBuilder_ != null) {
return debuggingInfoBuilder_.getMessageOrBuilder();
} else {
return debuggingInfo_ == null
? com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo.getDefaultInstance()
: debuggingInfo_;
}
}
/**
*
*
*
* Debugging info that would get populated when
* `StreamingAnalyzeContentRequest.enable_debugging_info` is set to true.
*
*
* .google.cloud.dialogflow.v2.CloudConversationDebuggingInfo debugging_info = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo,
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo.Builder,
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfoOrBuilder>
getDebuggingInfoFieldBuilder() {
if (debuggingInfoBuilder_ == null) {
debuggingInfoBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo,
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfo.Builder,
com.google.cloud.dialogflow.v2.CloudConversationDebuggingInfoOrBuilder>(
getDebuggingInfo(), getParentForChildren(), isClean());
debuggingInfo_ = null;
}
return debuggingInfoBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse)
}
// @@protoc_insertion_point(class_scope:google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse)
private static final com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse();
}
public static com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StreamingAnalyzeContentResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.dialogflow.v2.StreamingAnalyzeContentResponse
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy