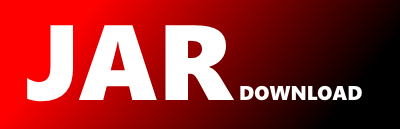
google.cloud.documentai.v1beta3.document_processor_service.proto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-document-ai-v1beta3 Show documentation
Show all versions of proto-google-cloud-document-ai-v1beta3 Show documentation
PROTO library for proto-google-cloud-document-ai-v1beta3
// Copyright 2023 Google LLC
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
syntax = "proto3";
package google.cloud.documentai.v1beta3;
import "google/api/annotations.proto";
import "google/api/client.proto";
import "google/api/field_behavior.proto";
import "google/api/resource.proto";
import "google/cloud/documentai/v1beta3/document.proto";
import "google/cloud/documentai/v1beta3/document_io.proto";
import "google/cloud/documentai/v1beta3/document_schema.proto";
import "google/cloud/documentai/v1beta3/evaluation.proto";
import "google/cloud/documentai/v1beta3/operation_metadata.proto";
import "google/cloud/documentai/v1beta3/processor.proto";
import "google/cloud/documentai/v1beta3/processor_type.proto";
import "google/longrunning/operations.proto";
import "google/protobuf/empty.proto";
import "google/protobuf/field_mask.proto";
import "google/protobuf/timestamp.proto";
import "google/rpc/status.proto";
option csharp_namespace = "Google.Cloud.DocumentAI.V1Beta3";
option go_package = "cloud.google.com/go/documentai/apiv1beta3/documentaipb;documentaipb";
option java_multiple_files = true;
option java_outer_classname = "DocumentAiProcessorService";
option java_package = "com.google.cloud.documentai.v1beta3";
option php_namespace = "Google\\Cloud\\DocumentAI\\V1beta3";
option ruby_package = "Google::Cloud::DocumentAI::V1beta3";
option (google.api.resource_definition) = {
type: "documentai.googleapis.com/HumanReviewConfig"
pattern: "projects/{project}/locations/{location}/processors/{processor}/humanReviewConfig"
};
option (google.api.resource_definition) = {
type: "documentai.googleapis.com/Location"
pattern: "projects/{project}/locations/{location}"
};
// Service to call Document AI to process documents according to the
// processor's definition. Processors are built using state-of-the-art Google
// AI such as natural language, computer vision, and translation to extract
// structured information from unstructured or semi-structured documents.
service DocumentProcessorService {
option (google.api.default_host) = "documentai.googleapis.com";
option (google.api.oauth_scopes) =
"https://www.googleapis.com/auth/cloud-platform";
// Processes a single document.
rpc ProcessDocument(ProcessRequest) returns (ProcessResponse) {
option (google.api.http) = {
post: "/v1beta3/{name=projects/*/locations/*/processors/*}:process"
body: "*"
additional_bindings {
post: "/v1beta3/{name=projects/*/locations/*/processors/*/processorVersions/*}:process"
body: "*"
}
};
option (google.api.method_signature) = "name";
}
// LRO endpoint to batch process many documents. The output is written
// to Cloud Storage as JSON in the [Document] format.
rpc BatchProcessDocuments(BatchProcessRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1beta3/{name=projects/*/locations/*/processors/*}:batchProcess"
body: "*"
additional_bindings {
post: "/v1beta3/{name=projects/*/locations/*/processors/*/processorVersions/*}:batchProcess"
body: "*"
}
};
option (google.api.method_signature) = "name";
option (google.longrunning.operation_info) = {
response_type: "BatchProcessResponse"
metadata_type: "BatchProcessMetadata"
};
}
// Fetches processor types. Note that we don't use
// [ListProcessorTypes][google.cloud.documentai.v1beta3.DocumentProcessorService.ListProcessorTypes]
// here, because it isn't paginated.
rpc FetchProcessorTypes(FetchProcessorTypesRequest)
returns (FetchProcessorTypesResponse) {
option (google.api.http) = {
get: "/v1beta3/{parent=projects/*/locations/*}:fetchProcessorTypes"
};
option (google.api.method_signature) = "parent";
}
// Lists the processor types that exist.
rpc ListProcessorTypes(ListProcessorTypesRequest)
returns (ListProcessorTypesResponse) {
option (google.api.http) = {
get: "/v1beta3/{parent=projects/*/locations/*}/processorTypes"
};
option (google.api.method_signature) = "parent";
}
// Gets a processor type detail.
rpc GetProcessorType(GetProcessorTypeRequest) returns (ProcessorType) {
option (google.api.http) = {
get: "/v1beta3/{name=projects/*/locations/*/processorTypes/*}"
};
option (google.api.method_signature) = "name";
}
// Lists all processors which belong to this project.
rpc ListProcessors(ListProcessorsRequest) returns (ListProcessorsResponse) {
option (google.api.http) = {
get: "/v1beta3/{parent=projects/*/locations/*}/processors"
};
option (google.api.method_signature) = "parent";
}
// Gets a processor detail.
rpc GetProcessor(GetProcessorRequest) returns (Processor) {
option (google.api.http) = {
get: "/v1beta3/{name=projects/*/locations/*/processors/*}"
};
option (google.api.method_signature) = "name";
}
// Trains a new processor version.
// Operation metadata is returned as
// [TrainProcessorVersionMetadata][google.cloud.documentai.v1beta3.TrainProcessorVersionMetadata].
rpc TrainProcessorVersion(TrainProcessorVersionRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1beta3/{parent=projects/*/locations/*/processors/*}/processorVersions:train"
body: "*"
};
option (google.api.method_signature) = "parent,processor_version";
option (google.longrunning.operation_info) = {
response_type: "TrainProcessorVersionResponse"
metadata_type: "TrainProcessorVersionMetadata"
};
}
// Gets a processor version detail.
rpc GetProcessorVersion(GetProcessorVersionRequest)
returns (ProcessorVersion) {
option (google.api.http) = {
get: "/v1beta3/{name=projects/*/locations/*/processors/*/processorVersions/*}"
};
option (google.api.method_signature) = "name";
}
// Lists all versions of a processor.
rpc ListProcessorVersions(ListProcessorVersionsRequest)
returns (ListProcessorVersionsResponse) {
option (google.api.http) = {
get: "/v1beta3/{parent=projects/*/locations/*/processors/*}/processorVersions"
};
option (google.api.method_signature) = "parent";
}
// Deletes the processor version, all artifacts under the processor version
// will be deleted.
rpc DeleteProcessorVersion(DeleteProcessorVersionRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
delete: "/v1beta3/{name=projects/*/locations/*/processors/*/processorVersions/*}"
};
option (google.api.method_signature) = "name";
option (google.longrunning.operation_info) = {
response_type: "google.protobuf.Empty"
metadata_type: "DeleteProcessorVersionMetadata"
};
}
// Deploys the processor version.
rpc DeployProcessorVersion(DeployProcessorVersionRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1beta3/{name=projects/*/locations/*/processors/*/processorVersions/*}:deploy"
body: "*"
};
option (google.api.method_signature) = "name";
option (google.longrunning.operation_info) = {
response_type: "DeployProcessorVersionResponse"
metadata_type: "DeployProcessorVersionMetadata"
};
}
// Undeploys the processor version.
rpc UndeployProcessorVersion(UndeployProcessorVersionRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1beta3/{name=projects/*/locations/*/processors/*/processorVersions/*}:undeploy"
body: "*"
};
option (google.api.method_signature) = "name";
option (google.longrunning.operation_info) = {
response_type: "UndeployProcessorVersionResponse"
metadata_type: "UndeployProcessorVersionMetadata"
};
}
// Creates a processor from the
// [ProcessorType][google.cloud.documentai.v1beta3.ProcessorType] provided.
// The processor will be at `ENABLED` state by default after its creation.
rpc CreateProcessor(CreateProcessorRequest) returns (Processor) {
option (google.api.http) = {
post: "/v1beta3/{parent=projects/*/locations/*}/processors"
body: "processor"
};
option (google.api.method_signature) = "parent,processor";
}
// Deletes the processor, unloads all deployed model artifacts if it was
// enabled and then deletes all artifacts associated with this processor.
rpc DeleteProcessor(DeleteProcessorRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
delete: "/v1beta3/{name=projects/*/locations/*/processors/*}"
};
option (google.api.method_signature) = "name";
option (google.longrunning.operation_info) = {
response_type: "google.protobuf.Empty"
metadata_type: "DeleteProcessorMetadata"
};
}
// Enables a processor
rpc EnableProcessor(EnableProcessorRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1beta3/{name=projects/*/locations/*/processors/*}:enable"
body: "*"
};
option (google.longrunning.operation_info) = {
response_type: "EnableProcessorResponse"
metadata_type: "EnableProcessorMetadata"
};
}
// Disables a processor
rpc DisableProcessor(DisableProcessorRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1beta3/{name=projects/*/locations/*/processors/*}:disable"
body: "*"
};
option (google.longrunning.operation_info) = {
response_type: "DisableProcessorResponse"
metadata_type: "DisableProcessorMetadata"
};
}
// Set the default (active) version of a
// [Processor][google.cloud.documentai.v1beta3.Processor] that will be used in
// [ProcessDocument][google.cloud.documentai.v1beta3.DocumentProcessorService.ProcessDocument]
// and
// [BatchProcessDocuments][google.cloud.documentai.v1beta3.DocumentProcessorService.BatchProcessDocuments].
rpc SetDefaultProcessorVersion(SetDefaultProcessorVersionRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1beta3/{processor=projects/*/locations/*/processors/*}:setDefaultProcessorVersion"
body: "*"
};
option (google.longrunning.operation_info) = {
response_type: "SetDefaultProcessorVersionResponse"
metadata_type: "SetDefaultProcessorVersionMetadata"
};
}
// Send a document for Human Review. The input document should be processed by
// the specified processor.
rpc ReviewDocument(ReviewDocumentRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1beta3/{human_review_config=projects/*/locations/*/processors/*/humanReviewConfig}:reviewDocument"
body: "*"
};
option (google.api.method_signature) = "human_review_config";
option (google.longrunning.operation_info) = {
response_type: "ReviewDocumentResponse"
metadata_type: "ReviewDocumentOperationMetadata"
};
}
// Evaluates a ProcessorVersion against annotated documents, producing an
// Evaluation.
rpc EvaluateProcessorVersion(EvaluateProcessorVersionRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1beta3/{processor_version=projects/*/locations/*/processors/*/processorVersions/*}:evaluateProcessorVersion"
body: "*"
};
option (google.api.method_signature) = "processor_version";
option (google.longrunning.operation_info) = {
response_type: "EvaluateProcessorVersionResponse"
metadata_type: "EvaluateProcessorVersionMetadata"
};
}
// Retrieves a specific evaluation.
rpc GetEvaluation(GetEvaluationRequest) returns (Evaluation) {
option (google.api.http) = {
get: "/v1beta3/{name=projects/*/locations/*/processors/*/processorVersions/*/evaluations/*}"
};
option (google.api.method_signature) = "name";
}
// Retrieves a set of evaluations for a given processor version.
rpc ListEvaluations(ListEvaluationsRequest)
returns (ListEvaluationsResponse) {
option (google.api.http) = {
get: "/v1beta3/{parent=projects/*/locations/*/processors/*/processorVersions/*}/evaluations"
};
option (google.api.method_signature) = "parent";
}
// Imports a processor version from source processor version.
rpc ImportProcessorVersion(ImportProcessorVersionRequest)
returns (google.longrunning.Operation) {
option (google.api.http) = {
post: "/v1beta3/{parent=projects/*/locations/*/processors/*}/processorVersions:importProcessorVersion"
body: "*"
};
option (google.api.method_signature) = "parent";
option (google.longrunning.operation_info) = {
response_type: "ImportProcessorVersionResponse"
metadata_type: "ImportProcessorVersionMetadata"
};
}
}
// Options for Process API
message ProcessOptions {
// A list of individual page numbers.
message IndividualPageSelector {
// Optional. Indices of the pages (starting from 1).
repeated int32 pages = 1 [(google.api.field_behavior) = OPTIONAL];
}
// A subset of pages to process. If not specified, all pages are processed.
// If a page range is set, only the given pages are extracted and processed
// from the document. In the output document,
// [Document.Page.page_number][google.cloud.documentai.v1beta3.Document.Page.page_number]
// refers to the page number in the original document. This configuration
// only applies to sync requests.
oneof page_range {
// Which pages to process (1-indexed).
IndividualPageSelector individual_page_selector = 5;
// Only process certain pages from the start. Process all if the document
// has fewer pages.
int32 from_start = 6;
// Only process certain pages from the end, same as above.
int32 from_end = 7;
}
// Only applicable to `OCR_PROCESSOR` and `FORM_PARSER_PROCESSOR`.
// Returns error if set on other processor types.
OcrConfig ocr_config = 1;
// Optional. Override the schema of the
// [ProcessorVersion][google.cloud.documentai.v1beta3.ProcessorVersion]. Will
// return an Invalid Argument error if this field is set when the underlying
// [ProcessorVersion][google.cloud.documentai.v1beta3.ProcessorVersion]
// doesn't support schema override.
DocumentSchema schema_override = 8 [(google.api.field_behavior) = OPTIONAL];
}
// Request message for the
// [ProcessDocument][google.cloud.documentai.v1beta3.DocumentProcessorService.ProcessDocument]
// method.
message ProcessRequest {
// The document payload.
oneof source {
// An inline document proto.
Document inline_document = 4;
// A raw document content (bytes).
RawDocument raw_document = 5;
// A raw document on Google Cloud Storage.
GcsDocument gcs_document = 8;
}
// Required. The resource name of the
// [Processor][google.cloud.documentai.v1beta3.Processor] or
// [ProcessorVersion][google.cloud.documentai.v1beta3.ProcessorVersion]
// to use for processing. If a
// [Processor][google.cloud.documentai.v1beta3.Processor] is specified, the
// server will use its [default
// version][google.cloud.documentai.v1beta3.Processor.default_processor_version].
// Format: `projects/{project}/locations/{location}/processors/{processor}`,
// or
// `projects/{project}/locations/{location}/processors/{processor}/processorVersions/{processorVersion}`
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = { type: "*" }
];
// The document payload, the
// [content][google.cloud.documentai.v1beta3.Document.content] and
// [mime_type][google.cloud.documentai.v1beta3.Document.mime_type] fields must
// be set.
Document document = 2 [deprecated = true];
// Whether human review should be skipped for this request. Default to
// `false`.
bool skip_human_review = 3;
// Specifies which fields to include in the
// [ProcessResponse.document][google.cloud.documentai.v1beta3.ProcessResponse.document]
// output. Only supports top-level document and pages field, so it must be in
// the form of `{document_field_name}` or `pages.{page_field_name}`.
google.protobuf.FieldMask field_mask = 6;
// Inference-time options for the process API
ProcessOptions process_options = 7;
// Optional. The labels with user-defined metadata for the request.
//
// Label keys and values can be no longer than 63 characters
// (Unicode codepoints) and can only contain lowercase letters, numeric
// characters, underscores, and dashes. International characters are allowed.
// Label values are optional. Label keys must start with a letter.
map labels = 10 [(google.api.field_behavior) = OPTIONAL];
}
// The status of human review on a processed document.
message HumanReviewStatus {
// The final state of human review on a processed document.
enum State {
// Human review state is unspecified. Most likely due to an internal error.
STATE_UNSPECIFIED = 0;
// Human review is skipped for the document. This can happen because human
// review isn't enabled on the processor or the processing request has
// been set to skip this document.
SKIPPED = 1;
// Human review validation is triggered and passed, so no review is needed.
VALIDATION_PASSED = 2;
// Human review validation is triggered and the document is under review.
IN_PROGRESS = 3;
// Some error happened during triggering human review, see the
// [state_message][google.cloud.documentai.v1beta3.HumanReviewStatus.state_message]
// for details.
ERROR = 4;
}
// The state of human review on the processing request.
State state = 1;
// A message providing more details about the human review state.
string state_message = 2;
// The name of the operation triggered by the processed document. This field
// is populated only when the
// [state][google.cloud.documentai.v1beta3.HumanReviewStatus.state] is
// `HUMAN_REVIEW_IN_PROGRESS`. It has the same response type and metadata as
// the long-running operation returned by
// [ReviewDocument][google.cloud.documentai.v1beta3.DocumentProcessorService.ReviewDocument].
string human_review_operation = 3;
}
// Response message for the
// [ProcessDocument][google.cloud.documentai.v1beta3.DocumentProcessorService.ProcessDocument]
// method.
message ProcessResponse {
// The document payload, will populate fields based on the processor's
// behavior.
Document document = 1;
// The name of the operation triggered by the processed document. If the human
// review process isn't triggered, this field is empty. It has the same
// response type and metadata as the long-running operation returned by
// [ReviewDocument][google.cloud.documentai.v1beta3.DocumentProcessorService.ReviewDocument].
string human_review_operation = 2 [deprecated = true];
// The status of human review on the processed document.
HumanReviewStatus human_review_status = 3;
}
// Request message for
// [BatchProcessDocuments][google.cloud.documentai.v1beta3.DocumentProcessorService.BatchProcessDocuments].
message BatchProcessRequest {
// The message for input config in batch process.
message BatchInputConfig {
option deprecated = true;
// The Cloud Storage location as the source of the document.
string gcs_source = 1;
// An IANA published [media type (MIME
// type)](https://www.iana.org/assignments/media-types/media-types.xhtml) of
// the input. If the input is a raw document, refer to [supported file
// types](https://cloud.google.com/document-ai/docs/file-types) for the list
// of media types. If the input is a
// [Document][google.cloud.documentai.v1beta3.Document], the type should be
// `application/json`.
string mime_type = 2;
}
// The output configuration in the
// [BatchProcessDocuments][google.cloud.documentai.v1beta3.DocumentProcessorService.BatchProcessDocuments]
// method.
message BatchOutputConfig {
option deprecated = true;
// The output Cloud Storage directory to put the processed documents.
string gcs_destination = 1;
}
// Required. The resource name of
// [Processor][google.cloud.documentai.v1beta3.Processor] or
// [ProcessorVersion][google.cloud.documentai.v1beta3.ProcessorVersion].
// Format: `projects/{project}/locations/{location}/processors/{processor}`,
// or
// `projects/{project}/locations/{location}/processors/{processor}/processorVersions/{processorVersion}`
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = { type: "*" }
];
// The input config for each single document in the batch process.
repeated BatchInputConfig input_configs = 2 [deprecated = true];
// The overall output config for batch process.
BatchOutputConfig output_config = 3 [deprecated = true];
// The input documents for the
// [BatchProcessDocuments][google.cloud.documentai.v1beta3.DocumentProcessorService.BatchProcessDocuments]
// method.
BatchDocumentsInputConfig input_documents = 5;
// The output configuration for the
// [BatchProcessDocuments][google.cloud.documentai.v1beta3.DocumentProcessorService.BatchProcessDocuments]
// method.
DocumentOutputConfig document_output_config = 6;
// Whether human review should be skipped for this request. Default to
// `false`.
bool skip_human_review = 4;
// Inference-time options for the process API
ProcessOptions process_options = 7;
// Optional. The labels with user-defined metadata for the request.
//
// Label keys and values can be no longer than 63 characters
// (Unicode codepoints) and can only contain lowercase letters, numeric
// characters, underscores, and dashes. International characters are allowed.
// Label values are optional. Label keys must start with a letter.
map labels = 9 [(google.api.field_behavior) = OPTIONAL];
}
// Response message for
// [BatchProcessDocuments][google.cloud.documentai.v1beta3.DocumentProcessorService.BatchProcessDocuments].
message BatchProcessResponse {}
// The long-running operation metadata for
// [BatchProcessDocuments][google.cloud.documentai.v1beta3.DocumentProcessorService.BatchProcessDocuments].
message BatchProcessMetadata {
// The status of a each individual document in the batch process.
message IndividualProcessStatus {
// The source of the document, same as the
// [input_gcs_source][google.cloud.documentai.v1beta3.BatchProcessMetadata.IndividualProcessStatus.input_gcs_source]
// field in the request when the batch process started.
string input_gcs_source = 1;
// The status processing the document.
google.rpc.Status status = 2;
// The Cloud Storage output destination (in the request as
// [DocumentOutputConfig.GcsOutputConfig.gcs_uri][google.cloud.documentai.v1beta3.DocumentOutputConfig.GcsOutputConfig.gcs_uri])
// of the processed document if it was successful, otherwise empty.
string output_gcs_destination = 3;
// The name of the operation triggered by the processed document. If the
// human review process isn't triggered, this field will be empty. It has
// the same response type and metadata as the long-running operation
// returned by the
// [ReviewDocument][google.cloud.documentai.v1beta3.DocumentProcessorService.ReviewDocument]
// method.
string human_review_operation = 4 [deprecated = true];
// The status of human review on the processed document.
HumanReviewStatus human_review_status = 5;
}
// Possible states of the batch processing operation.
enum State {
// The default value. This value is used if the state is omitted.
STATE_UNSPECIFIED = 0;
// Request operation is waiting for scheduling.
WAITING = 1;
// Request is being processed.
RUNNING = 2;
// The batch processing completed successfully.
SUCCEEDED = 3;
// The batch processing was being cancelled.
CANCELLING = 4;
// The batch processing was cancelled.
CANCELLED = 5;
// The batch processing has failed.
FAILED = 6;
}
// The state of the current batch processing.
State state = 1;
// A message providing more details about the current state of processing.
// For example, the error message if the operation is failed.
string state_message = 2;
// The creation time of the operation.
google.protobuf.Timestamp create_time = 3;
// The last update time of the operation.
google.protobuf.Timestamp update_time = 4;
// The list of response details of each document.
repeated IndividualProcessStatus individual_process_statuses = 5;
}
// Request message for the
// [FetchProcessorTypes][google.cloud.documentai.v1beta3.DocumentProcessorService.FetchProcessorTypes]
// method. Some processor types may require the project be added to an
// allowlist.
message FetchProcessorTypesRequest {
// Required. The location of processor types to list.
// Format: `projects/{project}/locations/{location}`.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "documentai.googleapis.com/ProcessorType"
}
];
}
// Response message for the
// [FetchProcessorTypes][google.cloud.documentai.v1beta3.DocumentProcessorService.FetchProcessorTypes]
// method.
message FetchProcessorTypesResponse {
// The list of processor types.
repeated ProcessorType processor_types = 1;
}
// Request message for the
// [ListProcessorTypes][google.cloud.documentai.v1beta3.DocumentProcessorService.ListProcessorTypes]
// method. Some processor types may require the project be added to an
// allowlist.
message ListProcessorTypesRequest {
// Required. The location of processor types to list.
// Format: `projects/{project}/locations/{location}`.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "documentai.googleapis.com/ProcessorType"
}
];
// The maximum number of processor types to return.
// If unspecified, at most `100` processor types will be returned.
// The maximum value is `500`. Values above `500` will be coerced to `500`.
int32 page_size = 2;
// Used to retrieve the next page of results, empty if at the end of the list.
string page_token = 3;
}
// Response message for the
// [ListProcessorTypes][google.cloud.documentai.v1beta3.DocumentProcessorService.ListProcessorTypes]
// method.
message ListProcessorTypesResponse {
// The processor types.
repeated ProcessorType processor_types = 1;
// Points to the next page, otherwise empty.
string next_page_token = 2;
}
// Request message for list all processors belongs to a project.
message ListProcessorsRequest {
// Required. The parent (project and location) which owns this collection of
// Processors. Format: `projects/{project}/locations/{location}`
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "documentai.googleapis.com/Processor"
}
];
// The maximum number of processors to return.
// If unspecified, at most `50` processors will be returned.
// The maximum value is `100`. Values above `100` will be coerced to `100`.
int32 page_size = 2;
// We will return the processors sorted by creation time. The page token
// will point to the next processor.
string page_token = 3;
}
// Response message for the
// [ListProcessors][google.cloud.documentai.v1beta3.DocumentProcessorService.ListProcessors]
// method.
message ListProcessorsResponse {
// The list of processors.
repeated Processor processors = 1;
// Points to the next processor, otherwise empty.
string next_page_token = 2;
}
// Request message for the
// [GetProcessorType][google.cloud.documentai.v1beta3.DocumentProcessorService.GetProcessorType]
// method.
message GetProcessorTypeRequest {
// Required. The processor type resource name.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/ProcessorType"
}
];
}
// Request message for the
// [GetProcessor][google.cloud.documentai.v1beta3.DocumentProcessorService.GetProcessor]
// method.
message GetProcessorRequest {
// Required. The processor resource name.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/Processor"
}
];
}
// Request message for the
// [GetProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.GetProcessorVersion]
// method.
message GetProcessorVersionRequest {
// Required. The processor resource name.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/ProcessorVersion"
}
];
}
// Request message for list all processor versions belongs to a processor.
message ListProcessorVersionsRequest {
// Required. The parent (project, location and processor) to list all
// versions. Format:
// `projects/{project}/locations/{location}/processors/{processor}`
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "documentai.googleapis.com/ProcessorVersion"
}
];
// The maximum number of processor versions to return.
// If unspecified, at most `10` processor versions will be returned.
// The maximum value is `20`. Values above `20` will be coerced to `20`.
int32 page_size = 2;
// We will return the processor versions sorted by creation time. The page
// token will point to the next processor version.
string page_token = 3;
}
// Response message for the
// [ListProcessorVersions][google.cloud.documentai.v1beta3.DocumentProcessorService.ListProcessorVersions]
// method.
message ListProcessorVersionsResponse {
// The list of processors.
repeated ProcessorVersion processor_versions = 1;
// Points to the next processor, otherwise empty.
string next_page_token = 2;
}
// Request message for the
// [DeleteProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.DeleteProcessorVersion]
// method.
message DeleteProcessorVersionRequest {
// Required. The processor version resource name to be deleted.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/ProcessorVersion"
}
];
}
// The long-running operation metadata for the
// [DeleteProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.DeleteProcessorVersion]
// method.
message DeleteProcessorVersionMetadata {
// The basic metadata of the long-running operation.
CommonOperationMetadata common_metadata = 1;
}
// Request message for the
// [DeployProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.DeployProcessorVersion]
// method.
message DeployProcessorVersionRequest {
// Required. The processor version resource name to be deployed.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/ProcessorVersion"
}
];
}
// Response message for the
// [DeployProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.DeployProcessorVersion]
// method.
message DeployProcessorVersionResponse {}
// The long-running operation metadata for the
// [DeployProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.DeployProcessorVersion]
// method.
message DeployProcessorVersionMetadata {
// The basic metadata of the long-running operation.
CommonOperationMetadata common_metadata = 1;
}
// Request message for the
// [UndeployProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.UndeployProcessorVersion]
// method.
message UndeployProcessorVersionRequest {
// Required. The processor version resource name to be undeployed.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/ProcessorVersion"
}
];
}
// Response message for the
// [UndeployProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.UndeployProcessorVersion]
// method.
message UndeployProcessorVersionResponse {}
// The long-running operation metadata for the
// [UndeployProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.UndeployProcessorVersion]
// method.
message UndeployProcessorVersionMetadata {
// The basic metadata of the long-running operation.
CommonOperationMetadata common_metadata = 1;
}
// Request message for the
// [CreateProcessor][google.cloud.documentai.v1beta3.DocumentProcessorService.CreateProcessor]
// method. Notice this request is sent to a regionalized backend service. If the
// [ProcessorType][google.cloud.documentai.v1beta3.ProcessorType] isn't
// available in that region, the creation fails.
message CreateProcessorRequest {
// Required. The parent (project and location) under which to create the
// processor. Format: `projects/{project}/locations/{location}`
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "documentai.googleapis.com/Processor"
}
];
// Required. The processor to be created, requires
// [Processor.type][google.cloud.documentai.v1beta3.Processor.type] and
// [Processor.display_name][google.cloud.documentai.v1beta3.Processor.display_name]
// to be set. Also, the
// [Processor.kms_key_name][google.cloud.documentai.v1beta3.Processor.kms_key_name]
// field must be set if the processor is under CMEK.
Processor processor = 2 [(google.api.field_behavior) = REQUIRED];
}
// Request message for the
// [DeleteProcessor][google.cloud.documentai.v1beta3.DocumentProcessorService.DeleteProcessor]
// method.
message DeleteProcessorRequest {
// Required. The processor resource name to be deleted.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/Processor"
}
];
}
// The long-running operation metadata for the
// [DeleteProcessor][google.cloud.documentai.v1beta3.DocumentProcessorService.DeleteProcessor]
// method.
message DeleteProcessorMetadata {
// The basic metadata of the long-running operation.
CommonOperationMetadata common_metadata = 5;
}
// Request message for the
// [EnableProcessor][google.cloud.documentai.v1beta3.DocumentProcessorService.EnableProcessor]
// method.
message EnableProcessorRequest {
// Required. The processor resource name to be enabled.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/Processor"
}
];
}
// Response message for the
// [EnableProcessor][google.cloud.documentai.v1beta3.DocumentProcessorService.EnableProcessor]
// method. Intentionally empty proto for adding fields in future.
message EnableProcessorResponse {}
// The long-running operation metadata for the
// [EnableProcessor][google.cloud.documentai.v1beta3.DocumentProcessorService.EnableProcessor]
// method.
message EnableProcessorMetadata {
// The basic metadata of the long-running operation.
CommonOperationMetadata common_metadata = 5;
}
// Request message for the
// [DisableProcessor][google.cloud.documentai.v1beta3.DocumentProcessorService.DisableProcessor]
// method.
message DisableProcessorRequest {
// Required. The processor resource name to be disabled.
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/Processor"
}
];
}
// Response message for the
// [DisableProcessor][google.cloud.documentai.v1beta3.DocumentProcessorService.DisableProcessor]
// method. Intentionally empty proto for adding fields in future.
message DisableProcessorResponse {}
// The long-running operation metadata for the
// [DisableProcessor][google.cloud.documentai.v1beta3.DocumentProcessorService.DisableProcessor]
// method.
message DisableProcessorMetadata {
// The basic metadata of the long-running operation.
CommonOperationMetadata common_metadata = 5;
}
// Request message for the
// [SetDefaultProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.SetDefaultProcessorVersion]
// method.
message SetDefaultProcessorVersionRequest {
// Required. The resource name of the
// [Processor][google.cloud.documentai.v1beta3.Processor] to change default
// version.
string processor = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/Processor"
}
];
// Required. The resource name of child
// [ProcessorVersion][google.cloud.documentai.v1beta3.ProcessorVersion] to use
// as default. Format:
// `projects/{project}/locations/{location}/processors/{processor}/processorVersions/{version}`
string default_processor_version = 2 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/ProcessorVersion"
}
];
}
// Response message for the
// [SetDefaultProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.SetDefaultProcessorVersion]
// method.
message SetDefaultProcessorVersionResponse {}
// The long-running operation metadata for the
// [SetDefaultProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.SetDefaultProcessorVersion]
// method.
message SetDefaultProcessorVersionMetadata {
// The basic metadata of the long-running operation.
CommonOperationMetadata common_metadata = 1;
}
// Request message for the
// [TrainProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.TrainProcessorVersion]
// method.
message TrainProcessorVersionRequest {
// The input data used to train a new
// [ProcessorVersion][google.cloud.documentai.v1beta3.ProcessorVersion].
message InputData {
// The documents used for training the new version.
BatchDocumentsInputConfig training_documents = 3;
// The documents used for testing the trained version.
BatchDocumentsInputConfig test_documents = 4;
}
// Options to control the training of the Custom Document Extraction (CDE)
// Processor.
message CustomDocumentExtractionOptions {
// Training Method for CDE. `TRAINING_METHOD_UNSPECIFIED` will fall back to
// `MODEL_BASED`.
enum TrainingMethod {
TRAINING_METHOD_UNSPECIFIED = 0;
MODEL_BASED = 1;
TEMPLATE_BASED = 2;
}
// Training method to use for CDE training.
TrainingMethod training_method = 3;
}
// Options to control foundation model tuning of the processor.
message FoundationModelTuningOptions {
// Optional. The number of steps to run for model tuning. Valid values are
// between 1 and 400. If not provided, recommended steps will be used.
int32 train_steps = 2 [(google.api.field_behavior) = OPTIONAL];
// Optional. The multiplier to apply to the recommended learning rate. Valid
// values are between 0.1 and 10. If not provided, recommended learning rate
// will be used.
float learning_rate_multiplier = 3 [(google.api.field_behavior) = OPTIONAL];
}
oneof processor_flags {
// Options to control Custom Document Extraction (CDE) Processor.
CustomDocumentExtractionOptions custom_document_extraction_options = 5;
// Options to control foundation model tuning of a processor.
FoundationModelTuningOptions foundation_model_tuning_options = 12;
}
// Required. The parent (project, location and processor) to create the new
// version for. Format:
// `projects/{project}/locations/{location}/processors/{processor}`.
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/Processor"
}
];
// Required. The processor version to be created.
ProcessorVersion processor_version = 2
[(google.api.field_behavior) = REQUIRED];
// Optional. The schema the processor version will be trained with.
DocumentSchema document_schema = 10 [(google.api.field_behavior) = OPTIONAL];
// Optional. The input data used to train the
// [ProcessorVersion][google.cloud.documentai.v1beta3.ProcessorVersion].
InputData input_data = 4 [(google.api.field_behavior) = OPTIONAL];
// Optional. The processor version to use as a base for training. This
// processor version must be a child of `parent`. Format:
// `projects/{project}/locations/{location}/processors/{processor}/processorVersions/{processorVersion}`.
string base_processor_version = 8 [(google.api.field_behavior) = OPTIONAL];
}
// The response for
// [TrainProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.TrainProcessorVersion].
message TrainProcessorVersionResponse {
// The resource name of the processor version produced by training.
string processor_version = 1;
}
// The metadata that represents a processor version being created.
message TrainProcessorVersionMetadata {
// The dataset validation information.
// This includes any and all errors with documents and the dataset.
message DatasetValidation {
// The total number of document errors.
int32 document_error_count = 3;
// The total number of dataset errors.
int32 dataset_error_count = 4;
// Error information pertaining to specific documents. A maximum of 10
// document errors will be returned.
// Any document with errors will not be used throughout training.
repeated google.rpc.Status document_errors = 1;
// Error information for the dataset as a whole. A maximum of 10 dataset
// errors will be returned.
// A single dataset error is terminal for training.
repeated google.rpc.Status dataset_errors = 2;
}
// The basic metadata of the long-running operation.
CommonOperationMetadata common_metadata = 1;
// The training dataset validation information.
DatasetValidation training_dataset_validation = 2;
// The test dataset validation information.
DatasetValidation test_dataset_validation = 3;
}
// Request message for the
// [ReviewDocument][google.cloud.documentai.v1beta3.DocumentProcessorService.ReviewDocument]
// method.
message ReviewDocumentRequest {
// The priority level of the human review task.
enum Priority {
// The default priority level.
DEFAULT = 0;
// The urgent priority level. The labeling manager should allocate labeler
// resource to the urgent task queue to respect this priority level.
URGENT = 1;
}
// The document payload.
oneof source {
// An inline document proto.
Document inline_document = 4;
}
// Required. The resource name of the
// [HumanReviewConfig][google.cloud.documentai.v1beta3.HumanReviewConfig] that
// the document will be reviewed with.
string human_review_config = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/HumanReviewConfig"
}
];
// The document that needs human review.
Document document = 2 [deprecated = true];
// Whether the validation should be performed on the ad-hoc review request.
bool enable_schema_validation = 3;
// The priority of the human review task.
Priority priority = 5;
// The document schema of the human review task.
DocumentSchema document_schema = 6;
}
// Response message for the
// [ReviewDocument][google.cloud.documentai.v1beta3.DocumentProcessorService.ReviewDocument]
// method.
message ReviewDocumentResponse {
// Possible states of the review operation.
enum State {
// The default value. This value is used if the state is omitted.
STATE_UNSPECIFIED = 0;
// The review operation is rejected by the reviewer.
REJECTED = 1;
// The review operation is succeeded.
SUCCEEDED = 2;
}
// The Cloud Storage uri for the human reviewed document if the review is
// succeeded.
string gcs_destination = 1;
// The state of the review operation.
State state = 2;
// The reason why the review is rejected by reviewer.
string rejection_reason = 3;
}
// The long-running operation metadata for the
// [ReviewDocument][google.cloud.documentai.v1beta3.DocumentProcessorService.ReviewDocument]
// method.
message ReviewDocumentOperationMetadata {
// State of the long-running operation.
enum State {
// Unspecified state.
STATE_UNSPECIFIED = 0;
// Operation is still running.
RUNNING = 1;
// Operation is being cancelled.
CANCELLING = 2;
// Operation succeeded.
SUCCEEDED = 3;
// Operation failed.
FAILED = 4;
// Operation is cancelled.
CANCELLED = 5;
}
// Used only when Operation.done is false.
State state = 1;
// A message providing more details about the current state of processing.
// For example, the error message if the operation is failed.
string state_message = 2;
// The creation time of the operation.
google.protobuf.Timestamp create_time = 3;
// The last update time of the operation.
google.protobuf.Timestamp update_time = 4;
// The basic metadata of the long-running operation.
CommonOperationMetadata common_metadata = 5;
// The Crowd Compute question ID.
string question_id = 6;
}
// Evaluates the given
// [ProcessorVersion][google.cloud.documentai.v1beta3.ProcessorVersion] against
// the supplied documents.
message EvaluateProcessorVersionRequest {
// Required. The resource name of the
// [ProcessorVersion][google.cloud.documentai.v1beta3.ProcessorVersion] to
// evaluate.
// `projects/{project}/locations/{location}/processors/{processor}/processorVersions/{processorVersion}`
string processor_version = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/ProcessorVersion"
}
];
// Optional. The documents used in the evaluation. If unspecified, use the
// processor's dataset as evaluation input.
BatchDocumentsInputConfig evaluation_documents = 3
[(google.api.field_behavior) = OPTIONAL];
}
// Metadata of the
// [EvaluateProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.EvaluateProcessorVersion]
// method.
message EvaluateProcessorVersionMetadata {
// The basic metadata of the long-running operation.
CommonOperationMetadata common_metadata = 1;
}
// Response of the
// [EvaluateProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.EvaluateProcessorVersion]
// method.
message EvaluateProcessorVersionResponse {
// The resource name of the created evaluation.
string evaluation = 2;
}
// Retrieves a specific Evaluation.
message GetEvaluationRequest {
// Required. The resource name of the
// [Evaluation][google.cloud.documentai.v1beta3.Evaluation] to get.
// `projects/{project}/locations/{location}/processors/{processor}/processorVersions/{processorVersion}/evaluations/{evaluation}`
string name = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/Evaluation"
}
];
}
// Retrieves a list of evaluations for a given
// [ProcessorVersion][google.cloud.documentai.v1beta3.ProcessorVersion].
message ListEvaluationsRequest {
// Required. The resource name of the
// [ProcessorVersion][google.cloud.documentai.v1beta3.ProcessorVersion] to
// list evaluations for.
// `projects/{project}/locations/{location}/processors/{processor}/processorVersions/{processorVersion}`
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
type: "documentai.googleapis.com/ProcessorVersion"
}
];
// The standard list page size.
// If unspecified, at most `5` evaluations are returned.
// The maximum value is `100`. Values above `100` are coerced to `100`.
int32 page_size = 2;
// A page token, received from a previous `ListEvaluations` call.
// Provide this to retrieve the subsequent page.
string page_token = 3;
}
// The response from `ListEvaluations`.
message ListEvaluationsResponse {
// The evaluations requested.
repeated Evaluation evaluations = 1;
// A token, which can be sent as `page_token` to retrieve the next page.
// If this field is omitted, there are no subsequent pages.
string next_page_token = 2;
}
// The request message for the
// [ImportProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.ImportProcessorVersion]
// method.
//
// The Document AI [Service
// Agent](https://cloud.google.com/iam/docs/service-agents) of the destination
// project must have [Document AI Editor
// role](https://cloud.google.com/document-ai/docs/access-control/iam-roles) on
// the source project.
//
// The destination project is specified as part of the
// [parent][google.cloud.documentai.v1beta3.ImportProcessorVersionRequest.parent]
// field. The source project is specified as part of the
// [source][google.cloud.documentai.v1beta3.ImportProcessorVersionRequest.processor_version_source]
// or
// [external_processor_version_source][google.cloud.documentai.v1beta3.ImportProcessorVersionRequest.external_processor_version_source]
// field.
message ImportProcessorVersionRequest {
// The external source processor version.
message ExternalProcessorVersionSource {
// Required. The processor version name. Format:
// `projects/{project}/locations/{location}/processors/{processor}/processorVersions/{processorVersion}`
string processor_version = 1 [(google.api.field_behavior) = REQUIRED];
// Optional. The Document AI service endpoint. For example,
// 'https://us-documentai.googleapis.com'
string service_endpoint = 2 [(google.api.field_behavior) = OPTIONAL];
}
oneof source {
// The source processor version to import from. The source processor version
// and destination processor need to be in the same environment and region.
// Note that ProcessorVersions with `model_type` `MODEL_TYPE_LLM` are not
// supported.
string processor_version_source = 2 [(google.api.resource_reference) = {
type: "documentai.googleapis.com/ProcessorVersion"
}];
// The source processor version to import from. It can be from a different
// environment and region than the destination processor.
ExternalProcessorVersionSource external_processor_version_source = 3;
}
// Required. The destination processor name to create the processor version
// in. Format:
// `projects/{project}/locations/{location}/processors/{processor}`
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = {
child_type: "documentai.googleapis.com/ProcessorVersion"
}
];
}
// The response message for the
// [ImportProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.ImportProcessorVersion]
// method.
message ImportProcessorVersionResponse {
// The destination processor version name.
string processor_version = 1 [(google.api.resource_reference) = {
type: "documentai.googleapis.com/ProcessorVersion"
}];
}
// The long-running operation metadata for the
// [ImportProcessorVersion][google.cloud.documentai.v1beta3.DocumentProcessorService.ImportProcessorVersion]
// method.
message ImportProcessorVersionMetadata {
// The basic metadata for the long-running operation.
CommonOperationMetadata common_metadata = 1;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy