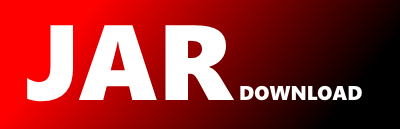
com.google.firestore.v1.StructuredQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-firestore-v1 Show documentation
Show all versions of proto-google-cloud-firestore-v1 Show documentation
PROTO library for proto-google-cloud-firestore-v1
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/firestore/v1/query.proto
// Protobuf Java Version: 3.25.4
package com.google.firestore.v1;
/**
*
*
* * A Firestore query.
*
* The query stages are executed in the following order:
* 1. from
* 2. where
* 3. select
* 4. order_by + start_at + end_at
* 5. offset
* 6. limit
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery}
*/
public final class StructuredQuery extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.firestore.v1.StructuredQuery)
StructuredQueryOrBuilder {
private static final long serialVersionUID = 0L;
// Use StructuredQuery.newBuilder() to construct.
private StructuredQuery(com.google.protobuf.GeneratedMessageV3.Builder builder) {
super(builder);
}
private StructuredQuery() {
from_ = java.util.Collections.emptyList();
orderBy_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new StructuredQuery();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.class,
com.google.firestore.v1.StructuredQuery.Builder.class);
}
/**
*
*
* * A sort direction.
*
*
* Protobuf enum {@code google.firestore.v1.StructuredQuery.Direction}
*/
public enum Direction implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
* * Unspecified.
*
*
* DIRECTION_UNSPECIFIED = 0;
*/
DIRECTION_UNSPECIFIED(0),
/**
*
*
* * Ascending.
*
*
* ASCENDING = 1;
*/
ASCENDING(1),
/**
*
*
* * Descending.
*
*
* DESCENDING = 2;
*/
DESCENDING(2),
UNRECOGNIZED(-1),
;
/**
*
*
* * Unspecified.
*
*
* DIRECTION_UNSPECIFIED = 0;
*/
public static final int DIRECTION_UNSPECIFIED_VALUE = 0;
/**
*
*
* * Ascending.
*
*
* ASCENDING = 1;
*/
public static final int ASCENDING_VALUE = 1;
/**
*
*
* * Descending.
*
*
* DESCENDING = 2;
*/
public static final int DESCENDING_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Direction valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Direction forNumber(int value) {
switch (value) {
case 0:
return DIRECTION_UNSPECIFIED;
case 1:
return ASCENDING;
case 2:
return DESCENDING;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Direction findValueByNumber(int number) {
return Direction.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.firestore.v1.StructuredQuery.getDescriptor().getEnumTypes().get(0);
}
private static final Direction[] VALUES = values();
public static Direction valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Direction(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.firestore.v1.StructuredQuery.Direction)
}
public interface CollectionSelectorOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.firestore.v1.StructuredQuery.CollectionSelector)
com.google.protobuf.MessageOrBuilder {
/**
*
*
* * The collection ID.
* When set, selects only collections with this ID.
*
*
* string collection_id = 2;
*
* @return The collectionId.
*/
java.lang.String getCollectionId();
/**
*
*
* * The collection ID.
* When set, selects only collections with this ID.
*
*
* string collection_id = 2;
*
* @return The bytes for collectionId.
*/
com.google.protobuf.ByteString getCollectionIdBytes();
/**
*
*
* * When false, selects only collections that are immediate children of
* the `parent` specified in the containing `RunQueryRequest`.
* When true, selects all descendant collections.
*
*
* bool all_descendants = 3;
*
* @return The allDescendants.
*/
boolean getAllDescendants();
}
/**
*
*
* * A selection of a collection, such as `messages as m1`.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.CollectionSelector}
*/
public static final class CollectionSelector extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.firestore.v1.StructuredQuery.CollectionSelector)
CollectionSelectorOrBuilder {
private static final long serialVersionUID = 0L;
// Use CollectionSelector.newBuilder() to construct.
private CollectionSelector(com.google.protobuf.GeneratedMessageV3.Builder builder) {
super(builder);
}
private CollectionSelector() {
collectionId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new CollectionSelector();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_CollectionSelector_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_CollectionSelector_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.CollectionSelector.class,
com.google.firestore.v1.StructuredQuery.CollectionSelector.Builder.class);
}
public static final int COLLECTION_ID_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object collectionId_ = "";
/**
*
*
* * The collection ID.
* When set, selects only collections with this ID.
*
*
* string collection_id = 2;
*
* @return The collectionId.
*/
@java.lang.Override
public java.lang.String getCollectionId() {
java.lang.Object ref = collectionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
collectionId_ = s;
return s;
}
}
/**
*
*
* * The collection ID.
* When set, selects only collections with this ID.
*
*
* string collection_id = 2;
*
* @return The bytes for collectionId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCollectionIdBytes() {
java.lang.Object ref = collectionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
collectionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ALL_DESCENDANTS_FIELD_NUMBER = 3;
private boolean allDescendants_ = false;
/**
*
*
* * When false, selects only collections that are immediate children of
* the `parent` specified in the containing `RunQueryRequest`.
* When true, selects all descendant collections.
*
*
* bool all_descendants = 3;
*
* @return The allDescendants.
*/
@java.lang.Override
public boolean getAllDescendants() {
return allDescendants_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(collectionId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, collectionId_);
}
if (allDescendants_ != false) {
output.writeBool(3, allDescendants_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(collectionId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, collectionId_);
}
if (allDescendants_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(3, allDescendants_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.firestore.v1.StructuredQuery.CollectionSelector)) {
return super.equals(obj);
}
com.google.firestore.v1.StructuredQuery.CollectionSelector other =
(com.google.firestore.v1.StructuredQuery.CollectionSelector) obj;
if (!getCollectionId().equals(other.getCollectionId())) return false;
if (getAllDescendants() != other.getAllDescendants()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COLLECTION_ID_FIELD_NUMBER;
hash = (53 * hash) + getCollectionId().hashCode();
hash = (37 * hash) + ALL_DESCENDANTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getAllDescendants());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.firestore.v1.StructuredQuery.CollectionSelector prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
* * A selection of a collection, such as `messages as m1`.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.CollectionSelector}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.firestore.v1.StructuredQuery.CollectionSelector)
com.google.firestore.v1.StructuredQuery.CollectionSelectorOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_CollectionSelector_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_CollectionSelector_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.CollectionSelector.class,
com.google.firestore.v1.StructuredQuery.CollectionSelector.Builder.class);
}
// Construct using com.google.firestore.v1.StructuredQuery.CollectionSelector.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
collectionId_ = "";
allDescendants_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_CollectionSelector_descriptor;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CollectionSelector
getDefaultInstanceForType() {
return com.google.firestore.v1.StructuredQuery.CollectionSelector.getDefaultInstance();
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CollectionSelector build() {
com.google.firestore.v1.StructuredQuery.CollectionSelector result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CollectionSelector buildPartial() {
com.google.firestore.v1.StructuredQuery.CollectionSelector result =
new com.google.firestore.v1.StructuredQuery.CollectionSelector(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.firestore.v1.StructuredQuery.CollectionSelector result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.collectionId_ = collectionId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.allDescendants_ = allDescendants_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.firestore.v1.StructuredQuery.CollectionSelector) {
return mergeFrom((com.google.firestore.v1.StructuredQuery.CollectionSelector) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.firestore.v1.StructuredQuery.CollectionSelector other) {
if (other
== com.google.firestore.v1.StructuredQuery.CollectionSelector.getDefaultInstance())
return this;
if (!other.getCollectionId().isEmpty()) {
collectionId_ = other.collectionId_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.getAllDescendants() != false) {
setAllDescendants(other.getAllDescendants());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18:
{
collectionId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 18
case 24:
{
allDescendants_ = input.readBool();
bitField0_ |= 0x00000002;
break;
} // case 24
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object collectionId_ = "";
/**
*
*
* * The collection ID.
* When set, selects only collections with this ID.
*
*
* string collection_id = 2;
*
* @return The collectionId.
*/
public java.lang.String getCollectionId() {
java.lang.Object ref = collectionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
collectionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
* * The collection ID.
* When set, selects only collections with this ID.
*
*
* string collection_id = 2;
*
* @return The bytes for collectionId.
*/
public com.google.protobuf.ByteString getCollectionIdBytes() {
java.lang.Object ref = collectionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
collectionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
* * The collection ID.
* When set, selects only collections with this ID.
*
*
* string collection_id = 2;
*
* @param value The collectionId to set.
* @return This builder for chaining.
*/
public Builder setCollectionId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
collectionId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * The collection ID.
* When set, selects only collections with this ID.
*
*
* string collection_id = 2;
*
* @return This builder for chaining.
*/
public Builder clearCollectionId() {
collectionId_ = getDefaultInstance().getCollectionId();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
* * The collection ID.
* When set, selects only collections with this ID.
*
*
* string collection_id = 2;
*
* @param value The bytes for collectionId to set.
* @return This builder for chaining.
*/
public Builder setCollectionIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
collectionId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private boolean allDescendants_;
/**
*
*
* * When false, selects only collections that are immediate children of
* the `parent` specified in the containing `RunQueryRequest`.
* When true, selects all descendant collections.
*
*
* bool all_descendants = 3;
*
* @return The allDescendants.
*/
@java.lang.Override
public boolean getAllDescendants() {
return allDescendants_;
}
/**
*
*
* * When false, selects only collections that are immediate children of
* the `parent` specified in the containing `RunQueryRequest`.
* When true, selects all descendant collections.
*
*
* bool all_descendants = 3;
*
* @param value The allDescendants to set.
* @return This builder for chaining.
*/
public Builder setAllDescendants(boolean value) {
allDescendants_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
* * When false, selects only collections that are immediate children of
* the `parent` specified in the containing `RunQueryRequest`.
* When true, selects all descendant collections.
*
*
* bool all_descendants = 3;
*
* @return This builder for chaining.
*/
public Builder clearAllDescendants() {
bitField0_ = (bitField0_ & ~0x00000002);
allDescendants_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.firestore.v1.StructuredQuery.CollectionSelector)
}
// @@protoc_insertion_point(class_scope:google.firestore.v1.StructuredQuery.CollectionSelector)
private static final com.google.firestore.v1.StructuredQuery.CollectionSelector
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.firestore.v1.StructuredQuery.CollectionSelector();
}
public static com.google.firestore.v1.StructuredQuery.CollectionSelector getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CollectionSelector parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CollectionSelector getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FilterOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.firestore.v1.StructuredQuery.Filter)
com.google.protobuf.MessageOrBuilder {
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*
* @return Whether the compositeFilter field is set.
*/
boolean hasCompositeFilter();
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*
* @return The compositeFilter.
*/
com.google.firestore.v1.StructuredQuery.CompositeFilter getCompositeFilter();
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*/
com.google.firestore.v1.StructuredQuery.CompositeFilterOrBuilder getCompositeFilterOrBuilder();
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*
* @return Whether the fieldFilter field is set.
*/
boolean hasFieldFilter();
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*
* @return The fieldFilter.
*/
com.google.firestore.v1.StructuredQuery.FieldFilter getFieldFilter();
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*/
com.google.firestore.v1.StructuredQuery.FieldFilterOrBuilder getFieldFilterOrBuilder();
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*
* @return Whether the unaryFilter field is set.
*/
boolean hasUnaryFilter();
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*
* @return The unaryFilter.
*/
com.google.firestore.v1.StructuredQuery.UnaryFilter getUnaryFilter();
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*/
com.google.firestore.v1.StructuredQuery.UnaryFilterOrBuilder getUnaryFilterOrBuilder();
com.google.firestore.v1.StructuredQuery.Filter.FilterTypeCase getFilterTypeCase();
}
/**
*
*
* * A filter.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.Filter}
*/
public static final class Filter extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.firestore.v1.StructuredQuery.Filter)
FilterOrBuilder {
private static final long serialVersionUID = 0L;
// Use Filter.newBuilder() to construct.
private Filter(com.google.protobuf.GeneratedMessageV3.Builder builder) {
super(builder);
}
private Filter() {}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Filter();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Filter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Filter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.Filter.class,
com.google.firestore.v1.StructuredQuery.Filter.Builder.class);
}
private int filterTypeCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object filterType_;
public enum FilterTypeCase
implements
com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
COMPOSITE_FILTER(1),
FIELD_FILTER(2),
UNARY_FILTER(3),
FILTERTYPE_NOT_SET(0);
private final int value;
private FilterTypeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static FilterTypeCase valueOf(int value) {
return forNumber(value);
}
public static FilterTypeCase forNumber(int value) {
switch (value) {
case 1:
return COMPOSITE_FILTER;
case 2:
return FIELD_FILTER;
case 3:
return UNARY_FILTER;
case 0:
return FILTERTYPE_NOT_SET;
default:
return null;
}
}
public int getNumber() {
return this.value;
}
};
public FilterTypeCase getFilterTypeCase() {
return FilterTypeCase.forNumber(filterTypeCase_);
}
public static final int COMPOSITE_FILTER_FIELD_NUMBER = 1;
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*
* @return Whether the compositeFilter field is set.
*/
@java.lang.Override
public boolean hasCompositeFilter() {
return filterTypeCase_ == 1;
}
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*
* @return The compositeFilter.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CompositeFilter getCompositeFilter() {
if (filterTypeCase_ == 1) {
return (com.google.firestore.v1.StructuredQuery.CompositeFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.CompositeFilter.getDefaultInstance();
}
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CompositeFilterOrBuilder
getCompositeFilterOrBuilder() {
if (filterTypeCase_ == 1) {
return (com.google.firestore.v1.StructuredQuery.CompositeFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.CompositeFilter.getDefaultInstance();
}
public static final int FIELD_FILTER_FIELD_NUMBER = 2;
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*
* @return Whether the fieldFilter field is set.
*/
@java.lang.Override
public boolean hasFieldFilter() {
return filterTypeCase_ == 2;
}
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*
* @return The fieldFilter.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldFilter getFieldFilter() {
if (filterTypeCase_ == 2) {
return (com.google.firestore.v1.StructuredQuery.FieldFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.FieldFilter.getDefaultInstance();
}
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldFilterOrBuilder getFieldFilterOrBuilder() {
if (filterTypeCase_ == 2) {
return (com.google.firestore.v1.StructuredQuery.FieldFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.FieldFilter.getDefaultInstance();
}
public static final int UNARY_FILTER_FIELD_NUMBER = 3;
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*
* @return Whether the unaryFilter field is set.
*/
@java.lang.Override
public boolean hasUnaryFilter() {
return filterTypeCase_ == 3;
}
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*
* @return The unaryFilter.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.UnaryFilter getUnaryFilter() {
if (filterTypeCase_ == 3) {
return (com.google.firestore.v1.StructuredQuery.UnaryFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.UnaryFilter.getDefaultInstance();
}
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.UnaryFilterOrBuilder getUnaryFilterOrBuilder() {
if (filterTypeCase_ == 3) {
return (com.google.firestore.v1.StructuredQuery.UnaryFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.UnaryFilter.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (filterTypeCase_ == 1) {
output.writeMessage(
1, (com.google.firestore.v1.StructuredQuery.CompositeFilter) filterType_);
}
if (filterTypeCase_ == 2) {
output.writeMessage(2, (com.google.firestore.v1.StructuredQuery.FieldFilter) filterType_);
}
if (filterTypeCase_ == 3) {
output.writeMessage(3, (com.google.firestore.v1.StructuredQuery.UnaryFilter) filterType_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (filterTypeCase_ == 1) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
1, (com.google.firestore.v1.StructuredQuery.CompositeFilter) filterType_);
}
if (filterTypeCase_ == 2) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
2, (com.google.firestore.v1.StructuredQuery.FieldFilter) filterType_);
}
if (filterTypeCase_ == 3) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
3, (com.google.firestore.v1.StructuredQuery.UnaryFilter) filterType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.firestore.v1.StructuredQuery.Filter)) {
return super.equals(obj);
}
com.google.firestore.v1.StructuredQuery.Filter other =
(com.google.firestore.v1.StructuredQuery.Filter) obj;
if (!getFilterTypeCase().equals(other.getFilterTypeCase())) return false;
switch (filterTypeCase_) {
case 1:
if (!getCompositeFilter().equals(other.getCompositeFilter())) return false;
break;
case 2:
if (!getFieldFilter().equals(other.getFieldFilter())) return false;
break;
case 3:
if (!getUnaryFilter().equals(other.getUnaryFilter())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (filterTypeCase_) {
case 1:
hash = (37 * hash) + COMPOSITE_FILTER_FIELD_NUMBER;
hash = (53 * hash) + getCompositeFilter().hashCode();
break;
case 2:
hash = (37 * hash) + FIELD_FILTER_FIELD_NUMBER;
hash = (53 * hash) + getFieldFilter().hashCode();
break;
case 3:
hash = (37 * hash) + UNARY_FILTER_FIELD_NUMBER;
hash = (53 * hash) + getUnaryFilter().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.firestore.v1.StructuredQuery.Filter parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.Filter parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Filter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.Filter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Filter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.Filter parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Filter parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.Filter parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Filter parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.Filter parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Filter parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.Filter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.firestore.v1.StructuredQuery.Filter prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
* * A filter.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.Filter}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.firestore.v1.StructuredQuery.Filter)
com.google.firestore.v1.StructuredQuery.FilterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Filter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Filter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.Filter.class,
com.google.firestore.v1.StructuredQuery.Filter.Builder.class);
}
// Construct using com.google.firestore.v1.StructuredQuery.Filter.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (compositeFilterBuilder_ != null) {
compositeFilterBuilder_.clear();
}
if (fieldFilterBuilder_ != null) {
fieldFilterBuilder_.clear();
}
if (unaryFilterBuilder_ != null) {
unaryFilterBuilder_.clear();
}
filterTypeCase_ = 0;
filterType_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Filter_descriptor;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Filter getDefaultInstanceForType() {
return com.google.firestore.v1.StructuredQuery.Filter.getDefaultInstance();
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Filter build() {
com.google.firestore.v1.StructuredQuery.Filter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Filter buildPartial() {
com.google.firestore.v1.StructuredQuery.Filter result =
new com.google.firestore.v1.StructuredQuery.Filter(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.google.firestore.v1.StructuredQuery.Filter result) {
int from_bitField0_ = bitField0_;
}
private void buildPartialOneofs(com.google.firestore.v1.StructuredQuery.Filter result) {
result.filterTypeCase_ = filterTypeCase_;
result.filterType_ = this.filterType_;
if (filterTypeCase_ == 1 && compositeFilterBuilder_ != null) {
result.filterType_ = compositeFilterBuilder_.build();
}
if (filterTypeCase_ == 2 && fieldFilterBuilder_ != null) {
result.filterType_ = fieldFilterBuilder_.build();
}
if (filterTypeCase_ == 3 && unaryFilterBuilder_ != null) {
result.filterType_ = unaryFilterBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.firestore.v1.StructuredQuery.Filter) {
return mergeFrom((com.google.firestore.v1.StructuredQuery.Filter) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.firestore.v1.StructuredQuery.Filter other) {
if (other == com.google.firestore.v1.StructuredQuery.Filter.getDefaultInstance())
return this;
switch (other.getFilterTypeCase()) {
case COMPOSITE_FILTER:
{
mergeCompositeFilter(other.getCompositeFilter());
break;
}
case FIELD_FILTER:
{
mergeFieldFilter(other.getFieldFilter());
break;
}
case UNARY_FILTER:
{
mergeUnaryFilter(other.getUnaryFilter());
break;
}
case FILTERTYPE_NOT_SET:
{
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
input.readMessage(
getCompositeFilterFieldBuilder().getBuilder(), extensionRegistry);
filterTypeCase_ = 1;
break;
} // case 10
case 18:
{
input.readMessage(getFieldFilterFieldBuilder().getBuilder(), extensionRegistry);
filterTypeCase_ = 2;
break;
} // case 18
case 26:
{
input.readMessage(getUnaryFilterFieldBuilder().getBuilder(), extensionRegistry);
filterTypeCase_ = 3;
break;
} // case 26
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int filterTypeCase_ = 0;
private java.lang.Object filterType_;
public FilterTypeCase getFilterTypeCase() {
return FilterTypeCase.forNumber(filterTypeCase_);
}
public Builder clearFilterType() {
filterTypeCase_ = 0;
filterType_ = null;
onChanged();
return this;
}
private int bitField0_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.CompositeFilter,
com.google.firestore.v1.StructuredQuery.CompositeFilter.Builder,
com.google.firestore.v1.StructuredQuery.CompositeFilterOrBuilder>
compositeFilterBuilder_;
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*
* @return Whether the compositeFilter field is set.
*/
@java.lang.Override
public boolean hasCompositeFilter() {
return filterTypeCase_ == 1;
}
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*
* @return The compositeFilter.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CompositeFilter getCompositeFilter() {
if (compositeFilterBuilder_ == null) {
if (filterTypeCase_ == 1) {
return (com.google.firestore.v1.StructuredQuery.CompositeFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.CompositeFilter.getDefaultInstance();
} else {
if (filterTypeCase_ == 1) {
return compositeFilterBuilder_.getMessage();
}
return com.google.firestore.v1.StructuredQuery.CompositeFilter.getDefaultInstance();
}
}
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*/
public Builder setCompositeFilter(
com.google.firestore.v1.StructuredQuery.CompositeFilter value) {
if (compositeFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
filterType_ = value;
onChanged();
} else {
compositeFilterBuilder_.setMessage(value);
}
filterTypeCase_ = 1;
return this;
}
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*/
public Builder setCompositeFilter(
com.google.firestore.v1.StructuredQuery.CompositeFilter.Builder builderForValue) {
if (compositeFilterBuilder_ == null) {
filterType_ = builderForValue.build();
onChanged();
} else {
compositeFilterBuilder_.setMessage(builderForValue.build());
}
filterTypeCase_ = 1;
return this;
}
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*/
public Builder mergeCompositeFilter(
com.google.firestore.v1.StructuredQuery.CompositeFilter value) {
if (compositeFilterBuilder_ == null) {
if (filterTypeCase_ == 1
&& filterType_
!= com.google.firestore.v1.StructuredQuery.CompositeFilter.getDefaultInstance()) {
filterType_ =
com.google.firestore.v1.StructuredQuery.CompositeFilter.newBuilder(
(com.google.firestore.v1.StructuredQuery.CompositeFilter) filterType_)
.mergeFrom(value)
.buildPartial();
} else {
filterType_ = value;
}
onChanged();
} else {
if (filterTypeCase_ == 1) {
compositeFilterBuilder_.mergeFrom(value);
} else {
compositeFilterBuilder_.setMessage(value);
}
}
filterTypeCase_ = 1;
return this;
}
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*/
public Builder clearCompositeFilter() {
if (compositeFilterBuilder_ == null) {
if (filterTypeCase_ == 1) {
filterTypeCase_ = 0;
filterType_ = null;
onChanged();
}
} else {
if (filterTypeCase_ == 1) {
filterTypeCase_ = 0;
filterType_ = null;
}
compositeFilterBuilder_.clear();
}
return this;
}
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*/
public com.google.firestore.v1.StructuredQuery.CompositeFilter.Builder
getCompositeFilterBuilder() {
return getCompositeFilterFieldBuilder().getBuilder();
}
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CompositeFilterOrBuilder
getCompositeFilterOrBuilder() {
if ((filterTypeCase_ == 1) && (compositeFilterBuilder_ != null)) {
return compositeFilterBuilder_.getMessageOrBuilder();
} else {
if (filterTypeCase_ == 1) {
return (com.google.firestore.v1.StructuredQuery.CompositeFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.CompositeFilter.getDefaultInstance();
}
}
/**
*
*
* * A composite filter.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter composite_filter = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.CompositeFilter,
com.google.firestore.v1.StructuredQuery.CompositeFilter.Builder,
com.google.firestore.v1.StructuredQuery.CompositeFilterOrBuilder>
getCompositeFilterFieldBuilder() {
if (compositeFilterBuilder_ == null) {
if (!(filterTypeCase_ == 1)) {
filterType_ =
com.google.firestore.v1.StructuredQuery.CompositeFilter.getDefaultInstance();
}
compositeFilterBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.CompositeFilter,
com.google.firestore.v1.StructuredQuery.CompositeFilter.Builder,
com.google.firestore.v1.StructuredQuery.CompositeFilterOrBuilder>(
(com.google.firestore.v1.StructuredQuery.CompositeFilter) filterType_,
getParentForChildren(),
isClean());
filterType_ = null;
}
filterTypeCase_ = 1;
onChanged();
return compositeFilterBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldFilter,
com.google.firestore.v1.StructuredQuery.FieldFilter.Builder,
com.google.firestore.v1.StructuredQuery.FieldFilterOrBuilder>
fieldFilterBuilder_;
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*
* @return Whether the fieldFilter field is set.
*/
@java.lang.Override
public boolean hasFieldFilter() {
return filterTypeCase_ == 2;
}
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*
* @return The fieldFilter.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldFilter getFieldFilter() {
if (fieldFilterBuilder_ == null) {
if (filterTypeCase_ == 2) {
return (com.google.firestore.v1.StructuredQuery.FieldFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.FieldFilter.getDefaultInstance();
} else {
if (filterTypeCase_ == 2) {
return fieldFilterBuilder_.getMessage();
}
return com.google.firestore.v1.StructuredQuery.FieldFilter.getDefaultInstance();
}
}
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*/
public Builder setFieldFilter(com.google.firestore.v1.StructuredQuery.FieldFilter value) {
if (fieldFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
filterType_ = value;
onChanged();
} else {
fieldFilterBuilder_.setMessage(value);
}
filterTypeCase_ = 2;
return this;
}
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*/
public Builder setFieldFilter(
com.google.firestore.v1.StructuredQuery.FieldFilter.Builder builderForValue) {
if (fieldFilterBuilder_ == null) {
filterType_ = builderForValue.build();
onChanged();
} else {
fieldFilterBuilder_.setMessage(builderForValue.build());
}
filterTypeCase_ = 2;
return this;
}
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*/
public Builder mergeFieldFilter(com.google.firestore.v1.StructuredQuery.FieldFilter value) {
if (fieldFilterBuilder_ == null) {
if (filterTypeCase_ == 2
&& filterType_
!= com.google.firestore.v1.StructuredQuery.FieldFilter.getDefaultInstance()) {
filterType_ =
com.google.firestore.v1.StructuredQuery.FieldFilter.newBuilder(
(com.google.firestore.v1.StructuredQuery.FieldFilter) filterType_)
.mergeFrom(value)
.buildPartial();
} else {
filterType_ = value;
}
onChanged();
} else {
if (filterTypeCase_ == 2) {
fieldFilterBuilder_.mergeFrom(value);
} else {
fieldFilterBuilder_.setMessage(value);
}
}
filterTypeCase_ = 2;
return this;
}
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*/
public Builder clearFieldFilter() {
if (fieldFilterBuilder_ == null) {
if (filterTypeCase_ == 2) {
filterTypeCase_ = 0;
filterType_ = null;
onChanged();
}
} else {
if (filterTypeCase_ == 2) {
filterTypeCase_ = 0;
filterType_ = null;
}
fieldFilterBuilder_.clear();
}
return this;
}
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*/
public com.google.firestore.v1.StructuredQuery.FieldFilter.Builder getFieldFilterBuilder() {
return getFieldFilterFieldBuilder().getBuilder();
}
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldFilterOrBuilder
getFieldFilterOrBuilder() {
if ((filterTypeCase_ == 2) && (fieldFilterBuilder_ != null)) {
return fieldFilterBuilder_.getMessageOrBuilder();
} else {
if (filterTypeCase_ == 2) {
return (com.google.firestore.v1.StructuredQuery.FieldFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.FieldFilter.getDefaultInstance();
}
}
/**
*
*
* * A filter on a document field.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter field_filter = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldFilter,
com.google.firestore.v1.StructuredQuery.FieldFilter.Builder,
com.google.firestore.v1.StructuredQuery.FieldFilterOrBuilder>
getFieldFilterFieldBuilder() {
if (fieldFilterBuilder_ == null) {
if (!(filterTypeCase_ == 2)) {
filterType_ = com.google.firestore.v1.StructuredQuery.FieldFilter.getDefaultInstance();
}
fieldFilterBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldFilter,
com.google.firestore.v1.StructuredQuery.FieldFilter.Builder,
com.google.firestore.v1.StructuredQuery.FieldFilterOrBuilder>(
(com.google.firestore.v1.StructuredQuery.FieldFilter) filterType_,
getParentForChildren(),
isClean());
filterType_ = null;
}
filterTypeCase_ = 2;
onChanged();
return fieldFilterBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.UnaryFilter,
com.google.firestore.v1.StructuredQuery.UnaryFilter.Builder,
com.google.firestore.v1.StructuredQuery.UnaryFilterOrBuilder>
unaryFilterBuilder_;
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*
* @return Whether the unaryFilter field is set.
*/
@java.lang.Override
public boolean hasUnaryFilter() {
return filterTypeCase_ == 3;
}
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*
* @return The unaryFilter.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.UnaryFilter getUnaryFilter() {
if (unaryFilterBuilder_ == null) {
if (filterTypeCase_ == 3) {
return (com.google.firestore.v1.StructuredQuery.UnaryFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.UnaryFilter.getDefaultInstance();
} else {
if (filterTypeCase_ == 3) {
return unaryFilterBuilder_.getMessage();
}
return com.google.firestore.v1.StructuredQuery.UnaryFilter.getDefaultInstance();
}
}
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*/
public Builder setUnaryFilter(com.google.firestore.v1.StructuredQuery.UnaryFilter value) {
if (unaryFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
filterType_ = value;
onChanged();
} else {
unaryFilterBuilder_.setMessage(value);
}
filterTypeCase_ = 3;
return this;
}
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*/
public Builder setUnaryFilter(
com.google.firestore.v1.StructuredQuery.UnaryFilter.Builder builderForValue) {
if (unaryFilterBuilder_ == null) {
filterType_ = builderForValue.build();
onChanged();
} else {
unaryFilterBuilder_.setMessage(builderForValue.build());
}
filterTypeCase_ = 3;
return this;
}
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*/
public Builder mergeUnaryFilter(com.google.firestore.v1.StructuredQuery.UnaryFilter value) {
if (unaryFilterBuilder_ == null) {
if (filterTypeCase_ == 3
&& filterType_
!= com.google.firestore.v1.StructuredQuery.UnaryFilter.getDefaultInstance()) {
filterType_ =
com.google.firestore.v1.StructuredQuery.UnaryFilter.newBuilder(
(com.google.firestore.v1.StructuredQuery.UnaryFilter) filterType_)
.mergeFrom(value)
.buildPartial();
} else {
filterType_ = value;
}
onChanged();
} else {
if (filterTypeCase_ == 3) {
unaryFilterBuilder_.mergeFrom(value);
} else {
unaryFilterBuilder_.setMessage(value);
}
}
filterTypeCase_ = 3;
return this;
}
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*/
public Builder clearUnaryFilter() {
if (unaryFilterBuilder_ == null) {
if (filterTypeCase_ == 3) {
filterTypeCase_ = 0;
filterType_ = null;
onChanged();
}
} else {
if (filterTypeCase_ == 3) {
filterTypeCase_ = 0;
filterType_ = null;
}
unaryFilterBuilder_.clear();
}
return this;
}
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*/
public com.google.firestore.v1.StructuredQuery.UnaryFilter.Builder getUnaryFilterBuilder() {
return getUnaryFilterFieldBuilder().getBuilder();
}
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.UnaryFilterOrBuilder
getUnaryFilterOrBuilder() {
if ((filterTypeCase_ == 3) && (unaryFilterBuilder_ != null)) {
return unaryFilterBuilder_.getMessageOrBuilder();
} else {
if (filterTypeCase_ == 3) {
return (com.google.firestore.v1.StructuredQuery.UnaryFilter) filterType_;
}
return com.google.firestore.v1.StructuredQuery.UnaryFilter.getDefaultInstance();
}
}
/**
*
*
* * A filter that takes exactly one argument.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter unary_filter = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.UnaryFilter,
com.google.firestore.v1.StructuredQuery.UnaryFilter.Builder,
com.google.firestore.v1.StructuredQuery.UnaryFilterOrBuilder>
getUnaryFilterFieldBuilder() {
if (unaryFilterBuilder_ == null) {
if (!(filterTypeCase_ == 3)) {
filterType_ = com.google.firestore.v1.StructuredQuery.UnaryFilter.getDefaultInstance();
}
unaryFilterBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.UnaryFilter,
com.google.firestore.v1.StructuredQuery.UnaryFilter.Builder,
com.google.firestore.v1.StructuredQuery.UnaryFilterOrBuilder>(
(com.google.firestore.v1.StructuredQuery.UnaryFilter) filterType_,
getParentForChildren(),
isClean());
filterType_ = null;
}
filterTypeCase_ = 3;
onChanged();
return unaryFilterBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.firestore.v1.StructuredQuery.Filter)
}
// @@protoc_insertion_point(class_scope:google.firestore.v1.StructuredQuery.Filter)
private static final com.google.firestore.v1.StructuredQuery.Filter DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.firestore.v1.StructuredQuery.Filter();
}
public static com.google.firestore.v1.StructuredQuery.Filter getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Filter parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Filter getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CompositeFilterOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.firestore.v1.StructuredQuery.CompositeFilter)
com.google.protobuf.MessageOrBuilder {
/**
*
*
* * The operator for combining multiple filters.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter.Operator op = 1;
*
* @return The enum numeric value on the wire for op.
*/
int getOpValue();
/**
*
*
* * The operator for combining multiple filters.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter.Operator op = 1;
*
* @return The op.
*/
com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator getOp();
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
java.util.List getFiltersList();
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
com.google.firestore.v1.StructuredQuery.Filter getFilters(int index);
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
int getFiltersCount();
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
java.util.List
getFiltersOrBuilderList();
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
com.google.firestore.v1.StructuredQuery.FilterOrBuilder getFiltersOrBuilder(int index);
}
/**
*
*
* * A filter that merges multiple other filters using the given operator.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.CompositeFilter}
*/
public static final class CompositeFilter extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.firestore.v1.StructuredQuery.CompositeFilter)
CompositeFilterOrBuilder {
private static final long serialVersionUID = 0L;
// Use CompositeFilter.newBuilder() to construct.
private CompositeFilter(com.google.protobuf.GeneratedMessageV3.Builder builder) {
super(builder);
}
private CompositeFilter() {
op_ = 0;
filters_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new CompositeFilter();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_CompositeFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_CompositeFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.CompositeFilter.class,
com.google.firestore.v1.StructuredQuery.CompositeFilter.Builder.class);
}
/**
*
*
* * A composite filter operator.
*
*
* Protobuf enum {@code google.firestore.v1.StructuredQuery.CompositeFilter.Operator}
*/
public enum Operator implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
* * Unspecified. This value must not be used.
*
*
* OPERATOR_UNSPECIFIED = 0;
*/
OPERATOR_UNSPECIFIED(0),
/**
*
*
* * Documents are required to satisfy all of the combined filters.
*
*
* AND = 1;
*/
AND(1),
/**
*
*
* * Documents are required to satisfy at least one of the combined filters.
*
*
* OR = 2;
*/
OR(2),
UNRECOGNIZED(-1),
;
/**
*
*
* * Unspecified. This value must not be used.
*
*
* OPERATOR_UNSPECIFIED = 0;
*/
public static final int OPERATOR_UNSPECIFIED_VALUE = 0;
/**
*
*
* * Documents are required to satisfy all of the combined filters.
*
*
* AND = 1;
*/
public static final int AND_VALUE = 1;
/**
*
*
* * Documents are required to satisfy at least one of the combined filters.
*
*
* OR = 2;
*/
public static final int OR_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Operator valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Operator forNumber(int value) {
switch (value) {
case 0:
return OPERATOR_UNSPECIFIED;
case 1:
return AND;
case 2:
return OR;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Operator findValueByNumber(int number) {
return Operator.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.firestore.v1.StructuredQuery.CompositeFilter.getDescriptor()
.getEnumTypes()
.get(0);
}
private static final Operator[] VALUES = values();
public static Operator valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Operator(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.firestore.v1.StructuredQuery.CompositeFilter.Operator)
}
public static final int OP_FIELD_NUMBER = 1;
private int op_ = 0;
/**
*
*
* * The operator for combining multiple filters.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter.Operator op = 1;
*
* @return The enum numeric value on the wire for op.
*/
@java.lang.Override
public int getOpValue() {
return op_;
}
/**
*
*
* * The operator for combining multiple filters.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter.Operator op = 1;
*
* @return The op.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator getOp() {
com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator result =
com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator.forNumber(op_);
return result == null
? com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator.UNRECOGNIZED
: result;
}
public static final int FILTERS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List filters_;
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
@java.lang.Override
public java.util.List getFiltersList() {
return filters_;
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
@java.lang.Override
public java.util.List
getFiltersOrBuilderList() {
return filters_;
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
@java.lang.Override
public int getFiltersCount() {
return filters_.size();
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Filter getFilters(int index) {
return filters_.get(index);
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FilterOrBuilder getFiltersOrBuilder(int index) {
return filters_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (op_
!= com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator.OPERATOR_UNSPECIFIED
.getNumber()) {
output.writeEnum(1, op_);
}
for (int i = 0; i < filters_.size(); i++) {
output.writeMessage(2, filters_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (op_
!= com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator.OPERATOR_UNSPECIFIED
.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(1, op_);
}
for (int i = 0; i < filters_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(2, filters_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.firestore.v1.StructuredQuery.CompositeFilter)) {
return super.equals(obj);
}
com.google.firestore.v1.StructuredQuery.CompositeFilter other =
(com.google.firestore.v1.StructuredQuery.CompositeFilter) obj;
if (op_ != other.op_) return false;
if (!getFiltersList().equals(other.getFiltersList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OP_FIELD_NUMBER;
hash = (53 * hash) + op_;
if (getFiltersCount() > 0) {
hash = (37 * hash) + FILTERS_FIELD_NUMBER;
hash = (53 * hash) + getFiltersList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.firestore.v1.StructuredQuery.CompositeFilter prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
* * A filter that merges multiple other filters using the given operator.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.CompositeFilter}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.firestore.v1.StructuredQuery.CompositeFilter)
com.google.firestore.v1.StructuredQuery.CompositeFilterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_CompositeFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_CompositeFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.CompositeFilter.class,
com.google.firestore.v1.StructuredQuery.CompositeFilter.Builder.class);
}
// Construct using com.google.firestore.v1.StructuredQuery.CompositeFilter.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
op_ = 0;
if (filtersBuilder_ == null) {
filters_ = java.util.Collections.emptyList();
} else {
filters_ = null;
filtersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_CompositeFilter_descriptor;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CompositeFilter getDefaultInstanceForType() {
return com.google.firestore.v1.StructuredQuery.CompositeFilter.getDefaultInstance();
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CompositeFilter build() {
com.google.firestore.v1.StructuredQuery.CompositeFilter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CompositeFilter buildPartial() {
com.google.firestore.v1.StructuredQuery.CompositeFilter result =
new com.google.firestore.v1.StructuredQuery.CompositeFilter(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(
com.google.firestore.v1.StructuredQuery.CompositeFilter result) {
if (filtersBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
filters_ = java.util.Collections.unmodifiableList(filters_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.filters_ = filters_;
} else {
result.filters_ = filtersBuilder_.build();
}
}
private void buildPartial0(com.google.firestore.v1.StructuredQuery.CompositeFilter result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.op_ = op_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.firestore.v1.StructuredQuery.CompositeFilter) {
return mergeFrom((com.google.firestore.v1.StructuredQuery.CompositeFilter) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.firestore.v1.StructuredQuery.CompositeFilter other) {
if (other == com.google.firestore.v1.StructuredQuery.CompositeFilter.getDefaultInstance())
return this;
if (other.op_ != 0) {
setOpValue(other.getOpValue());
}
if (filtersBuilder_ == null) {
if (!other.filters_.isEmpty()) {
if (filters_.isEmpty()) {
filters_ = other.filters_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureFiltersIsMutable();
filters_.addAll(other.filters_);
}
onChanged();
}
} else {
if (!other.filters_.isEmpty()) {
if (filtersBuilder_.isEmpty()) {
filtersBuilder_.dispose();
filtersBuilder_ = null;
filters_ = other.filters_;
bitField0_ = (bitField0_ & ~0x00000002);
filtersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getFiltersFieldBuilder()
: null;
} else {
filtersBuilder_.addAllMessages(other.filters_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
op_ = input.readEnum();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18:
{
com.google.firestore.v1.StructuredQuery.Filter m =
input.readMessage(
com.google.firestore.v1.StructuredQuery.Filter.parser(),
extensionRegistry);
if (filtersBuilder_ == null) {
ensureFiltersIsMutable();
filters_.add(m);
} else {
filtersBuilder_.addMessage(m);
}
break;
} // case 18
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int op_ = 0;
/**
*
*
* * The operator for combining multiple filters.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter.Operator op = 1;
*
* @return The enum numeric value on the wire for op.
*/
@java.lang.Override
public int getOpValue() {
return op_;
}
/**
*
*
* * The operator for combining multiple filters.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter.Operator op = 1;
*
* @param value The enum numeric value on the wire for op to set.
* @return This builder for chaining.
*/
public Builder setOpValue(int value) {
op_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * The operator for combining multiple filters.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter.Operator op = 1;
*
* @return The op.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator getOp() {
com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator result =
com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator.forNumber(op_);
return result == null
? com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator.UNRECOGNIZED
: result;
}
/**
*
*
* * The operator for combining multiple filters.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter.Operator op = 1;
*
* @param value The op to set.
* @return This builder for chaining.
*/
public Builder setOp(com.google.firestore.v1.StructuredQuery.CompositeFilter.Operator value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
op_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
* * The operator for combining multiple filters.
*
*
* .google.firestore.v1.StructuredQuery.CompositeFilter.Operator op = 1;
*
* @return This builder for chaining.
*/
public Builder clearOp() {
bitField0_ = (bitField0_ & ~0x00000001);
op_ = 0;
onChanged();
return this;
}
private java.util.List filters_ =
java.util.Collections.emptyList();
private void ensureFiltersIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
filters_ =
new java.util.ArrayList(filters_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Filter,
com.google.firestore.v1.StructuredQuery.Filter.Builder,
com.google.firestore.v1.StructuredQuery.FilterOrBuilder>
filtersBuilder_;
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public java.util.List getFiltersList() {
if (filtersBuilder_ == null) {
return java.util.Collections.unmodifiableList(filters_);
} else {
return filtersBuilder_.getMessageList();
}
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public int getFiltersCount() {
if (filtersBuilder_ == null) {
return filters_.size();
} else {
return filtersBuilder_.getCount();
}
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public com.google.firestore.v1.StructuredQuery.Filter getFilters(int index) {
if (filtersBuilder_ == null) {
return filters_.get(index);
} else {
return filtersBuilder_.getMessage(index);
}
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public Builder setFilters(int index, com.google.firestore.v1.StructuredQuery.Filter value) {
if (filtersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFiltersIsMutable();
filters_.set(index, value);
onChanged();
} else {
filtersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public Builder setFilters(
int index, com.google.firestore.v1.StructuredQuery.Filter.Builder builderForValue) {
if (filtersBuilder_ == null) {
ensureFiltersIsMutable();
filters_.set(index, builderForValue.build());
onChanged();
} else {
filtersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public Builder addFilters(com.google.firestore.v1.StructuredQuery.Filter value) {
if (filtersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFiltersIsMutable();
filters_.add(value);
onChanged();
} else {
filtersBuilder_.addMessage(value);
}
return this;
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public Builder addFilters(int index, com.google.firestore.v1.StructuredQuery.Filter value) {
if (filtersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFiltersIsMutable();
filters_.add(index, value);
onChanged();
} else {
filtersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public Builder addFilters(
com.google.firestore.v1.StructuredQuery.Filter.Builder builderForValue) {
if (filtersBuilder_ == null) {
ensureFiltersIsMutable();
filters_.add(builderForValue.build());
onChanged();
} else {
filtersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public Builder addFilters(
int index, com.google.firestore.v1.StructuredQuery.Filter.Builder builderForValue) {
if (filtersBuilder_ == null) {
ensureFiltersIsMutable();
filters_.add(index, builderForValue.build());
onChanged();
} else {
filtersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public Builder addAllFilters(
java.lang.Iterable values) {
if (filtersBuilder_ == null) {
ensureFiltersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, filters_);
onChanged();
} else {
filtersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public Builder clearFilters() {
if (filtersBuilder_ == null) {
filters_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
filtersBuilder_.clear();
}
return this;
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public Builder removeFilters(int index) {
if (filtersBuilder_ == null) {
ensureFiltersIsMutable();
filters_.remove(index);
onChanged();
} else {
filtersBuilder_.remove(index);
}
return this;
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public com.google.firestore.v1.StructuredQuery.Filter.Builder getFiltersBuilder(int index) {
return getFiltersFieldBuilder().getBuilder(index);
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public com.google.firestore.v1.StructuredQuery.FilterOrBuilder getFiltersOrBuilder(
int index) {
if (filtersBuilder_ == null) {
return filters_.get(index);
} else {
return filtersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public java.util.List
getFiltersOrBuilderList() {
if (filtersBuilder_ != null) {
return filtersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(filters_);
}
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public com.google.firestore.v1.StructuredQuery.Filter.Builder addFiltersBuilder() {
return getFiltersFieldBuilder()
.addBuilder(com.google.firestore.v1.StructuredQuery.Filter.getDefaultInstance());
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public com.google.firestore.v1.StructuredQuery.Filter.Builder addFiltersBuilder(int index) {
return getFiltersFieldBuilder()
.addBuilder(index, com.google.firestore.v1.StructuredQuery.Filter.getDefaultInstance());
}
/**
*
*
* * The list of filters to combine.
*
* Requires:
*
* * At least one filter is present.
*
*
* repeated .google.firestore.v1.StructuredQuery.Filter filters = 2;
*/
public java.util.List
getFiltersBuilderList() {
return getFiltersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Filter,
com.google.firestore.v1.StructuredQuery.Filter.Builder,
com.google.firestore.v1.StructuredQuery.FilterOrBuilder>
getFiltersFieldBuilder() {
if (filtersBuilder_ == null) {
filtersBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Filter,
com.google.firestore.v1.StructuredQuery.Filter.Builder,
com.google.firestore.v1.StructuredQuery.FilterOrBuilder>(
filters_, ((bitField0_ & 0x00000002) != 0), getParentForChildren(), isClean());
filters_ = null;
}
return filtersBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.firestore.v1.StructuredQuery.CompositeFilter)
}
// @@protoc_insertion_point(class_scope:google.firestore.v1.StructuredQuery.CompositeFilter)
private static final com.google.firestore.v1.StructuredQuery.CompositeFilter DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.firestore.v1.StructuredQuery.CompositeFilter();
}
public static com.google.firestore.v1.StructuredQuery.CompositeFilter getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CompositeFilter parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CompositeFilter getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FieldFilterOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.firestore.v1.StructuredQuery.FieldFilter)
com.google.protobuf.MessageOrBuilder {
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return Whether the field field is set.
*/
boolean hasField();
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return The field.
*/
com.google.firestore.v1.StructuredQuery.FieldReference getField();
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldOrBuilder();
/**
*
*
* * The operator to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter.Operator op = 2;
*
* @return The enum numeric value on the wire for op.
*/
int getOpValue();
/**
*
*
* * The operator to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter.Operator op = 2;
*
* @return The op.
*/
com.google.firestore.v1.StructuredQuery.FieldFilter.Operator getOp();
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*
* @return Whether the value field is set.
*/
boolean hasValue();
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*
* @return The value.
*/
com.google.firestore.v1.Value getValue();
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*/
com.google.firestore.v1.ValueOrBuilder getValueOrBuilder();
}
/**
*
*
* * A filter on a specific field.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.FieldFilter}
*/
public static final class FieldFilter extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.firestore.v1.StructuredQuery.FieldFilter)
FieldFilterOrBuilder {
private static final long serialVersionUID = 0L;
// Use FieldFilter.newBuilder() to construct.
private FieldFilter(com.google.protobuf.GeneratedMessageV3.Builder builder) {
super(builder);
}
private FieldFilter() {
op_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new FieldFilter();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FieldFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FieldFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.FieldFilter.class,
com.google.firestore.v1.StructuredQuery.FieldFilter.Builder.class);
}
/**
*
*
* * A field filter operator.
*
*
* Protobuf enum {@code google.firestore.v1.StructuredQuery.FieldFilter.Operator}
*/
public enum Operator implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
* * Unspecified. This value must not be used.
*
*
* OPERATOR_UNSPECIFIED = 0;
*/
OPERATOR_UNSPECIFIED(0),
/**
*
*
* * The given `field` is less than the given `value`.
*
* Requires:
*
* * That `field` come first in `order_by`.
*
*
* LESS_THAN = 1;
*/
LESS_THAN(1),
/**
*
*
* * The given `field` is less than or equal to the given `value`.
*
* Requires:
*
* * That `field` come first in `order_by`.
*
*
* LESS_THAN_OR_EQUAL = 2;
*/
LESS_THAN_OR_EQUAL(2),
/**
*
*
* * The given `field` is greater than the given `value`.
*
* Requires:
*
* * That `field` come first in `order_by`.
*
*
* GREATER_THAN = 3;
*/
GREATER_THAN(3),
/**
*
*
* * The given `field` is greater than or equal to the given `value`.
*
* Requires:
*
* * That `field` come first in `order_by`.
*
*
* GREATER_THAN_OR_EQUAL = 4;
*/
GREATER_THAN_OR_EQUAL(4),
/**
*
*
* * The given `field` is equal to the given `value`.
*
*
* EQUAL = 5;
*/
EQUAL(5),
/**
*
*
* * The given `field` is not equal to the given `value`.
*
* Requires:
*
* * No other `NOT_EQUAL`, `NOT_IN`, `IS_NOT_NULL`, or `IS_NOT_NAN`.
* * That `field` comes first in the `order_by`.
*
*
* NOT_EQUAL = 6;
*/
NOT_EQUAL(6),
/**
*
*
* * The given `field` is an array that contains the given `value`.
*
*
* ARRAY_CONTAINS = 7;
*/
ARRAY_CONTAINS(7),
/**
*
*
* * The given `field` is equal to at least one value in the given array.
*
* Requires:
*
* * That `value` is a non-empty `ArrayValue`, subject to disjunction
* limits.
* * No `NOT_IN` filters in the same query.
*
*
* IN = 8;
*/
IN(8),
/**
*
*
* * The given `field` is an array that contains any of the values in the
* given array.
*
* Requires:
*
* * That `value` is a non-empty `ArrayValue`, subject to disjunction
* limits.
* * No other `ARRAY_CONTAINS_ANY` filters within the same disjunction.
* * No `NOT_IN` filters in the same query.
*
*
* ARRAY_CONTAINS_ANY = 9;
*/
ARRAY_CONTAINS_ANY(9),
/**
*
*
* * The value of the `field` is not in the given array.
*
* Requires:
*
* * That `value` is a non-empty `ArrayValue` with at most 10 values.
* * No other `OR`, `IN`, `ARRAY_CONTAINS_ANY`, `NOT_IN`, `NOT_EQUAL`,
* `IS_NOT_NULL`, or `IS_NOT_NAN`.
* * That `field` comes first in the `order_by`.
*
*
* NOT_IN = 10;
*/
NOT_IN(10),
UNRECOGNIZED(-1),
;
/**
*
*
* * Unspecified. This value must not be used.
*
*
* OPERATOR_UNSPECIFIED = 0;
*/
public static final int OPERATOR_UNSPECIFIED_VALUE = 0;
/**
*
*
* * The given `field` is less than the given `value`.
*
* Requires:
*
* * That `field` come first in `order_by`.
*
*
* LESS_THAN = 1;
*/
public static final int LESS_THAN_VALUE = 1;
/**
*
*
* * The given `field` is less than or equal to the given `value`.
*
* Requires:
*
* * That `field` come first in `order_by`.
*
*
* LESS_THAN_OR_EQUAL = 2;
*/
public static final int LESS_THAN_OR_EQUAL_VALUE = 2;
/**
*
*
* * The given `field` is greater than the given `value`.
*
* Requires:
*
* * That `field` come first in `order_by`.
*
*
* GREATER_THAN = 3;
*/
public static final int GREATER_THAN_VALUE = 3;
/**
*
*
* * The given `field` is greater than or equal to the given `value`.
*
* Requires:
*
* * That `field` come first in `order_by`.
*
*
* GREATER_THAN_OR_EQUAL = 4;
*/
public static final int GREATER_THAN_OR_EQUAL_VALUE = 4;
/**
*
*
* * The given `field` is equal to the given `value`.
*
*
* EQUAL = 5;
*/
public static final int EQUAL_VALUE = 5;
/**
*
*
* * The given `field` is not equal to the given `value`.
*
* Requires:
*
* * No other `NOT_EQUAL`, `NOT_IN`, `IS_NOT_NULL`, or `IS_NOT_NAN`.
* * That `field` comes first in the `order_by`.
*
*
* NOT_EQUAL = 6;
*/
public static final int NOT_EQUAL_VALUE = 6;
/**
*
*
* * The given `field` is an array that contains the given `value`.
*
*
* ARRAY_CONTAINS = 7;
*/
public static final int ARRAY_CONTAINS_VALUE = 7;
/**
*
*
* * The given `field` is equal to at least one value in the given array.
*
* Requires:
*
* * That `value` is a non-empty `ArrayValue`, subject to disjunction
* limits.
* * No `NOT_IN` filters in the same query.
*
*
* IN = 8;
*/
public static final int IN_VALUE = 8;
/**
*
*
* * The given `field` is an array that contains any of the values in the
* given array.
*
* Requires:
*
* * That `value` is a non-empty `ArrayValue`, subject to disjunction
* limits.
* * No other `ARRAY_CONTAINS_ANY` filters within the same disjunction.
* * No `NOT_IN` filters in the same query.
*
*
* ARRAY_CONTAINS_ANY = 9;
*/
public static final int ARRAY_CONTAINS_ANY_VALUE = 9;
/**
*
*
* * The value of the `field` is not in the given array.
*
* Requires:
*
* * That `value` is a non-empty `ArrayValue` with at most 10 values.
* * No other `OR`, `IN`, `ARRAY_CONTAINS_ANY`, `NOT_IN`, `NOT_EQUAL`,
* `IS_NOT_NULL`, or `IS_NOT_NAN`.
* * That `field` comes first in the `order_by`.
*
*
* NOT_IN = 10;
*/
public static final int NOT_IN_VALUE = 10;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Operator valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Operator forNumber(int value) {
switch (value) {
case 0:
return OPERATOR_UNSPECIFIED;
case 1:
return LESS_THAN;
case 2:
return LESS_THAN_OR_EQUAL;
case 3:
return GREATER_THAN;
case 4:
return GREATER_THAN_OR_EQUAL;
case 5:
return EQUAL;
case 6:
return NOT_EQUAL;
case 7:
return ARRAY_CONTAINS;
case 8:
return IN;
case 9:
return ARRAY_CONTAINS_ANY;
case 10:
return NOT_IN;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Operator findValueByNumber(int number) {
return Operator.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.firestore.v1.StructuredQuery.FieldFilter.getDescriptor()
.getEnumTypes()
.get(0);
}
private static final Operator[] VALUES = values();
public static Operator valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Operator(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.firestore.v1.StructuredQuery.FieldFilter.Operator)
}
private int bitField0_;
public static final int FIELD_FIELD_NUMBER = 1;
private com.google.firestore.v1.StructuredQuery.FieldReference field_;
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return Whether the field field is set.
*/
@java.lang.Override
public boolean hasField() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return The field.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReference getField() {
return field_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: field_;
}
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldOrBuilder() {
return field_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: field_;
}
public static final int OP_FIELD_NUMBER = 2;
private int op_ = 0;
/**
*
*
* * The operator to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter.Operator op = 2;
*
* @return The enum numeric value on the wire for op.
*/
@java.lang.Override
public int getOpValue() {
return op_;
}
/**
*
*
* * The operator to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter.Operator op = 2;
*
* @return The op.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldFilter.Operator getOp() {
com.google.firestore.v1.StructuredQuery.FieldFilter.Operator result =
com.google.firestore.v1.StructuredQuery.FieldFilter.Operator.forNumber(op_);
return result == null
? com.google.firestore.v1.StructuredQuery.FieldFilter.Operator.UNRECOGNIZED
: result;
}
public static final int VALUE_FIELD_NUMBER = 3;
private com.google.firestore.v1.Value value_;
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*
* @return Whether the value field is set.
*/
@java.lang.Override
public boolean hasValue() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*
* @return The value.
*/
@java.lang.Override
public com.google.firestore.v1.Value getValue() {
return value_ == null ? com.google.firestore.v1.Value.getDefaultInstance() : value_;
}
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*/
@java.lang.Override
public com.google.firestore.v1.ValueOrBuilder getValueOrBuilder() {
return value_ == null ? com.google.firestore.v1.Value.getDefaultInstance() : value_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getField());
}
if (op_
!= com.google.firestore.v1.StructuredQuery.FieldFilter.Operator.OPERATOR_UNSPECIFIED
.getNumber()) {
output.writeEnum(2, op_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(3, getValue());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(1, getField());
}
if (op_
!= com.google.firestore.v1.StructuredQuery.FieldFilter.Operator.OPERATOR_UNSPECIFIED
.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(2, op_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(3, getValue());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.firestore.v1.StructuredQuery.FieldFilter)) {
return super.equals(obj);
}
com.google.firestore.v1.StructuredQuery.FieldFilter other =
(com.google.firestore.v1.StructuredQuery.FieldFilter) obj;
if (hasField() != other.hasField()) return false;
if (hasField()) {
if (!getField().equals(other.getField())) return false;
}
if (op_ != other.op_) return false;
if (hasValue() != other.hasValue()) return false;
if (hasValue()) {
if (!getValue().equals(other.getValue())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasField()) {
hash = (37 * hash) + FIELD_FIELD_NUMBER;
hash = (53 * hash) + getField().hashCode();
}
hash = (37 * hash) + OP_FIELD_NUMBER;
hash = (53 * hash) + op_;
if (hasValue()) {
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.firestore.v1.StructuredQuery.FieldFilter prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
* * A filter on a specific field.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.FieldFilter}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.firestore.v1.StructuredQuery.FieldFilter)
com.google.firestore.v1.StructuredQuery.FieldFilterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FieldFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FieldFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.FieldFilter.class,
com.google.firestore.v1.StructuredQuery.FieldFilter.Builder.class);
}
// Construct using com.google.firestore.v1.StructuredQuery.FieldFilter.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getFieldFieldBuilder();
getValueFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
field_ = null;
if (fieldBuilder_ != null) {
fieldBuilder_.dispose();
fieldBuilder_ = null;
}
op_ = 0;
value_ = null;
if (valueBuilder_ != null) {
valueBuilder_.dispose();
valueBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FieldFilter_descriptor;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldFilter getDefaultInstanceForType() {
return com.google.firestore.v1.StructuredQuery.FieldFilter.getDefaultInstance();
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldFilter build() {
com.google.firestore.v1.StructuredQuery.FieldFilter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldFilter buildPartial() {
com.google.firestore.v1.StructuredQuery.FieldFilter result =
new com.google.firestore.v1.StructuredQuery.FieldFilter(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.firestore.v1.StructuredQuery.FieldFilter result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.field_ = fieldBuilder_ == null ? field_ : fieldBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.op_ = op_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.value_ = valueBuilder_ == null ? value_ : valueBuilder_.build();
to_bitField0_ |= 0x00000002;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.firestore.v1.StructuredQuery.FieldFilter) {
return mergeFrom((com.google.firestore.v1.StructuredQuery.FieldFilter) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.firestore.v1.StructuredQuery.FieldFilter other) {
if (other == com.google.firestore.v1.StructuredQuery.FieldFilter.getDefaultInstance())
return this;
if (other.hasField()) {
mergeField(other.getField());
}
if (other.op_ != 0) {
setOpValue(other.getOpValue());
}
if (other.hasValue()) {
mergeValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
input.readMessage(getFieldFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 16:
{
op_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26:
{
input.readMessage(getValueFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.firestore.v1.StructuredQuery.FieldReference field_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>
fieldBuilder_;
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return Whether the field field is set.
*/
public boolean hasField() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return The field.
*/
public com.google.firestore.v1.StructuredQuery.FieldReference getField() {
if (fieldBuilder_ == null) {
return field_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: field_;
} else {
return fieldBuilder_.getMessage();
}
}
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public Builder setField(com.google.firestore.v1.StructuredQuery.FieldReference value) {
if (fieldBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
field_ = value;
} else {
fieldBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public Builder setField(
com.google.firestore.v1.StructuredQuery.FieldReference.Builder builderForValue) {
if (fieldBuilder_ == null) {
field_ = builderForValue.build();
} else {
fieldBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public Builder mergeField(com.google.firestore.v1.StructuredQuery.FieldReference value) {
if (fieldBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)
&& field_ != null
&& field_
!= com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()) {
getFieldBuilder().mergeFrom(value);
} else {
field_ = value;
}
} else {
fieldBuilder_.mergeFrom(value);
}
if (field_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public Builder clearField() {
bitField0_ = (bitField0_ & ~0x00000001);
field_ = null;
if (fieldBuilder_ != null) {
fieldBuilder_.dispose();
fieldBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public com.google.firestore.v1.StructuredQuery.FieldReference.Builder getFieldBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getFieldFieldBuilder().getBuilder();
}
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldOrBuilder() {
if (fieldBuilder_ != null) {
return fieldBuilder_.getMessageOrBuilder();
} else {
return field_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: field_;
}
}
/**
*
*
* * The field to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>
getFieldFieldBuilder() {
if (fieldBuilder_ == null) {
fieldBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>(
getField(), getParentForChildren(), isClean());
field_ = null;
}
return fieldBuilder_;
}
private int op_ = 0;
/**
*
*
* * The operator to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter.Operator op = 2;
*
* @return The enum numeric value on the wire for op.
*/
@java.lang.Override
public int getOpValue() {
return op_;
}
/**
*
*
* * The operator to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter.Operator op = 2;
*
* @param value The enum numeric value on the wire for op to set.
* @return This builder for chaining.
*/
public Builder setOpValue(int value) {
op_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
* * The operator to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter.Operator op = 2;
*
* @return The op.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldFilter.Operator getOp() {
com.google.firestore.v1.StructuredQuery.FieldFilter.Operator result =
com.google.firestore.v1.StructuredQuery.FieldFilter.Operator.forNumber(op_);
return result == null
? com.google.firestore.v1.StructuredQuery.FieldFilter.Operator.UNRECOGNIZED
: result;
}
/**
*
*
* * The operator to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter.Operator op = 2;
*
* @param value The op to set.
* @return This builder for chaining.
*/
public Builder setOp(com.google.firestore.v1.StructuredQuery.FieldFilter.Operator value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
op_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
* * The operator to filter by.
*
*
* .google.firestore.v1.StructuredQuery.FieldFilter.Operator op = 2;
*
* @return This builder for chaining.
*/
public Builder clearOp() {
bitField0_ = (bitField0_ & ~0x00000002);
op_ = 0;
onChanged();
return this;
}
private com.google.firestore.v1.Value value_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Value,
com.google.firestore.v1.Value.Builder,
com.google.firestore.v1.ValueOrBuilder>
valueBuilder_;
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*
* @return Whether the value field is set.
*/
public boolean hasValue() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*
* @return The value.
*/
public com.google.firestore.v1.Value getValue() {
if (valueBuilder_ == null) {
return value_ == null ? com.google.firestore.v1.Value.getDefaultInstance() : value_;
} else {
return valueBuilder_.getMessage();
}
}
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*/
public Builder setValue(com.google.firestore.v1.Value value) {
if (valueBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
} else {
valueBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*/
public Builder setValue(com.google.firestore.v1.Value.Builder builderForValue) {
if (valueBuilder_ == null) {
value_ = builderForValue.build();
} else {
valueBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*/
public Builder mergeValue(com.google.firestore.v1.Value value) {
if (valueBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)
&& value_ != null
&& value_ != com.google.firestore.v1.Value.getDefaultInstance()) {
getValueBuilder().mergeFrom(value);
} else {
value_ = value;
}
} else {
valueBuilder_.mergeFrom(value);
}
if (value_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*/
public Builder clearValue() {
bitField0_ = (bitField0_ & ~0x00000004);
value_ = null;
if (valueBuilder_ != null) {
valueBuilder_.dispose();
valueBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*/
public com.google.firestore.v1.Value.Builder getValueBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getValueFieldBuilder().getBuilder();
}
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*/
public com.google.firestore.v1.ValueOrBuilder getValueOrBuilder() {
if (valueBuilder_ != null) {
return valueBuilder_.getMessageOrBuilder();
} else {
return value_ == null ? com.google.firestore.v1.Value.getDefaultInstance() : value_;
}
}
/**
*
*
* * The value to compare to.
*
*
* .google.firestore.v1.Value value = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Value,
com.google.firestore.v1.Value.Builder,
com.google.firestore.v1.ValueOrBuilder>
getValueFieldBuilder() {
if (valueBuilder_ == null) {
valueBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Value,
com.google.firestore.v1.Value.Builder,
com.google.firestore.v1.ValueOrBuilder>(
getValue(), getParentForChildren(), isClean());
value_ = null;
}
return valueBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.firestore.v1.StructuredQuery.FieldFilter)
}
// @@protoc_insertion_point(class_scope:google.firestore.v1.StructuredQuery.FieldFilter)
private static final com.google.firestore.v1.StructuredQuery.FieldFilter DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.firestore.v1.StructuredQuery.FieldFilter();
}
public static com.google.firestore.v1.StructuredQuery.FieldFilter getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FieldFilter parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldFilter getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface UnaryFilterOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.firestore.v1.StructuredQuery.UnaryFilter)
com.google.protobuf.MessageOrBuilder {
/**
*
*
* * The unary operator to apply.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter.Operator op = 1;
*
* @return The enum numeric value on the wire for op.
*/
int getOpValue();
/**
*
*
* * The unary operator to apply.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter.Operator op = 1;
*
* @return The op.
*/
com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator getOp();
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*
* @return Whether the field field is set.
*/
boolean hasField();
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*
* @return The field.
*/
com.google.firestore.v1.StructuredQuery.FieldReference getField();
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*/
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldOrBuilder();
com.google.firestore.v1.StructuredQuery.UnaryFilter.OperandTypeCase getOperandTypeCase();
}
/**
*
*
* * A filter with a single operand.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.UnaryFilter}
*/
public static final class UnaryFilter extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.firestore.v1.StructuredQuery.UnaryFilter)
UnaryFilterOrBuilder {
private static final long serialVersionUID = 0L;
// Use UnaryFilter.newBuilder() to construct.
private UnaryFilter(com.google.protobuf.GeneratedMessageV3.Builder builder) {
super(builder);
}
private UnaryFilter() {
op_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new UnaryFilter();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_UnaryFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_UnaryFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.UnaryFilter.class,
com.google.firestore.v1.StructuredQuery.UnaryFilter.Builder.class);
}
/**
*
*
* * A unary operator.
*
*
* Protobuf enum {@code google.firestore.v1.StructuredQuery.UnaryFilter.Operator}
*/
public enum Operator implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
* * Unspecified. This value must not be used.
*
*
* OPERATOR_UNSPECIFIED = 0;
*/
OPERATOR_UNSPECIFIED(0),
/**
*
*
* * The given `field` is equal to `NaN`.
*
*
* IS_NAN = 2;
*/
IS_NAN(2),
/**
*
*
* * The given `field` is equal to `NULL`.
*
*
* IS_NULL = 3;
*/
IS_NULL(3),
/**
*
*
* * The given `field` is not equal to `NaN`.
*
* Requires:
*
* * No other `NOT_EQUAL`, `NOT_IN`, `IS_NOT_NULL`, or `IS_NOT_NAN`.
* * That `field` comes first in the `order_by`.
*
*
* IS_NOT_NAN = 4;
*/
IS_NOT_NAN(4),
/**
*
*
* * The given `field` is not equal to `NULL`.
*
* Requires:
*
* * A single `NOT_EQUAL`, `NOT_IN`, `IS_NOT_NULL`, or `IS_NOT_NAN`.
* * That `field` comes first in the `order_by`.
*
*
* IS_NOT_NULL = 5;
*/
IS_NOT_NULL(5),
UNRECOGNIZED(-1),
;
/**
*
*
* * Unspecified. This value must not be used.
*
*
* OPERATOR_UNSPECIFIED = 0;
*/
public static final int OPERATOR_UNSPECIFIED_VALUE = 0;
/**
*
*
* * The given `field` is equal to `NaN`.
*
*
* IS_NAN = 2;
*/
public static final int IS_NAN_VALUE = 2;
/**
*
*
* * The given `field` is equal to `NULL`.
*
*
* IS_NULL = 3;
*/
public static final int IS_NULL_VALUE = 3;
/**
*
*
* * The given `field` is not equal to `NaN`.
*
* Requires:
*
* * No other `NOT_EQUAL`, `NOT_IN`, `IS_NOT_NULL`, or `IS_NOT_NAN`.
* * That `field` comes first in the `order_by`.
*
*
* IS_NOT_NAN = 4;
*/
public static final int IS_NOT_NAN_VALUE = 4;
/**
*
*
* * The given `field` is not equal to `NULL`.
*
* Requires:
*
* * A single `NOT_EQUAL`, `NOT_IN`, `IS_NOT_NULL`, or `IS_NOT_NAN`.
* * That `field` comes first in the `order_by`.
*
*
* IS_NOT_NULL = 5;
*/
public static final int IS_NOT_NULL_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Operator valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Operator forNumber(int value) {
switch (value) {
case 0:
return OPERATOR_UNSPECIFIED;
case 2:
return IS_NAN;
case 3:
return IS_NULL;
case 4:
return IS_NOT_NAN;
case 5:
return IS_NOT_NULL;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Operator findValueByNumber(int number) {
return Operator.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.firestore.v1.StructuredQuery.UnaryFilter.getDescriptor()
.getEnumTypes()
.get(0);
}
private static final Operator[] VALUES = values();
public static Operator valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Operator(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.firestore.v1.StructuredQuery.UnaryFilter.Operator)
}
private int operandTypeCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object operandType_;
public enum OperandTypeCase
implements
com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
FIELD(2),
OPERANDTYPE_NOT_SET(0);
private final int value;
private OperandTypeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OperandTypeCase valueOf(int value) {
return forNumber(value);
}
public static OperandTypeCase forNumber(int value) {
switch (value) {
case 2:
return FIELD;
case 0:
return OPERANDTYPE_NOT_SET;
default:
return null;
}
}
public int getNumber() {
return this.value;
}
};
public OperandTypeCase getOperandTypeCase() {
return OperandTypeCase.forNumber(operandTypeCase_);
}
public static final int OP_FIELD_NUMBER = 1;
private int op_ = 0;
/**
*
*
* * The unary operator to apply.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter.Operator op = 1;
*
* @return The enum numeric value on the wire for op.
*/
@java.lang.Override
public int getOpValue() {
return op_;
}
/**
*
*
* * The unary operator to apply.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter.Operator op = 1;
*
* @return The op.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator getOp() {
com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator result =
com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator.forNumber(op_);
return result == null
? com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator.UNRECOGNIZED
: result;
}
public static final int FIELD_FIELD_NUMBER = 2;
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*
* @return Whether the field field is set.
*/
@java.lang.Override
public boolean hasField() {
return operandTypeCase_ == 2;
}
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*
* @return The field.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReference getField() {
if (operandTypeCase_ == 2) {
return (com.google.firestore.v1.StructuredQuery.FieldReference) operandType_;
}
return com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance();
}
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldOrBuilder() {
if (operandTypeCase_ == 2) {
return (com.google.firestore.v1.StructuredQuery.FieldReference) operandType_;
}
return com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (op_
!= com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator.OPERATOR_UNSPECIFIED
.getNumber()) {
output.writeEnum(1, op_);
}
if (operandTypeCase_ == 2) {
output.writeMessage(
2, (com.google.firestore.v1.StructuredQuery.FieldReference) operandType_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (op_
!= com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator.OPERATOR_UNSPECIFIED
.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(1, op_);
}
if (operandTypeCase_ == 2) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
2, (com.google.firestore.v1.StructuredQuery.FieldReference) operandType_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.firestore.v1.StructuredQuery.UnaryFilter)) {
return super.equals(obj);
}
com.google.firestore.v1.StructuredQuery.UnaryFilter other =
(com.google.firestore.v1.StructuredQuery.UnaryFilter) obj;
if (op_ != other.op_) return false;
if (!getOperandTypeCase().equals(other.getOperandTypeCase())) return false;
switch (operandTypeCase_) {
case 2:
if (!getField().equals(other.getField())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OP_FIELD_NUMBER;
hash = (53 * hash) + op_;
switch (operandTypeCase_) {
case 2:
hash = (37 * hash) + FIELD_FIELD_NUMBER;
hash = (53 * hash) + getField().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.firestore.v1.StructuredQuery.UnaryFilter prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
* * A filter with a single operand.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.UnaryFilter}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.firestore.v1.StructuredQuery.UnaryFilter)
com.google.firestore.v1.StructuredQuery.UnaryFilterOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_UnaryFilter_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_UnaryFilter_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.UnaryFilter.class,
com.google.firestore.v1.StructuredQuery.UnaryFilter.Builder.class);
}
// Construct using com.google.firestore.v1.StructuredQuery.UnaryFilter.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
op_ = 0;
if (fieldBuilder_ != null) {
fieldBuilder_.clear();
}
operandTypeCase_ = 0;
operandType_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_UnaryFilter_descriptor;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.UnaryFilter getDefaultInstanceForType() {
return com.google.firestore.v1.StructuredQuery.UnaryFilter.getDefaultInstance();
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.UnaryFilter build() {
com.google.firestore.v1.StructuredQuery.UnaryFilter result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.UnaryFilter buildPartial() {
com.google.firestore.v1.StructuredQuery.UnaryFilter result =
new com.google.firestore.v1.StructuredQuery.UnaryFilter(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.google.firestore.v1.StructuredQuery.UnaryFilter result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.op_ = op_;
}
}
private void buildPartialOneofs(com.google.firestore.v1.StructuredQuery.UnaryFilter result) {
result.operandTypeCase_ = operandTypeCase_;
result.operandType_ = this.operandType_;
if (operandTypeCase_ == 2 && fieldBuilder_ != null) {
result.operandType_ = fieldBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.firestore.v1.StructuredQuery.UnaryFilter) {
return mergeFrom((com.google.firestore.v1.StructuredQuery.UnaryFilter) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.firestore.v1.StructuredQuery.UnaryFilter other) {
if (other == com.google.firestore.v1.StructuredQuery.UnaryFilter.getDefaultInstance())
return this;
if (other.op_ != 0) {
setOpValue(other.getOpValue());
}
switch (other.getOperandTypeCase()) {
case FIELD:
{
mergeField(other.getField());
break;
}
case OPERANDTYPE_NOT_SET:
{
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
op_ = input.readEnum();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18:
{
input.readMessage(getFieldFieldBuilder().getBuilder(), extensionRegistry);
operandTypeCase_ = 2;
break;
} // case 18
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int operandTypeCase_ = 0;
private java.lang.Object operandType_;
public OperandTypeCase getOperandTypeCase() {
return OperandTypeCase.forNumber(operandTypeCase_);
}
public Builder clearOperandType() {
operandTypeCase_ = 0;
operandType_ = null;
onChanged();
return this;
}
private int bitField0_;
private int op_ = 0;
/**
*
*
* * The unary operator to apply.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter.Operator op = 1;
*
* @return The enum numeric value on the wire for op.
*/
@java.lang.Override
public int getOpValue() {
return op_;
}
/**
*
*
* * The unary operator to apply.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter.Operator op = 1;
*
* @param value The enum numeric value on the wire for op to set.
* @return This builder for chaining.
*/
public Builder setOpValue(int value) {
op_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * The unary operator to apply.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter.Operator op = 1;
*
* @return The op.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator getOp() {
com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator result =
com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator.forNumber(op_);
return result == null
? com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator.UNRECOGNIZED
: result;
}
/**
*
*
* * The unary operator to apply.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter.Operator op = 1;
*
* @param value The op to set.
* @return This builder for chaining.
*/
public Builder setOp(com.google.firestore.v1.StructuredQuery.UnaryFilter.Operator value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
op_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
* * The unary operator to apply.
*
*
* .google.firestore.v1.StructuredQuery.UnaryFilter.Operator op = 1;
*
* @return This builder for chaining.
*/
public Builder clearOp() {
bitField0_ = (bitField0_ & ~0x00000001);
op_ = 0;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>
fieldBuilder_;
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*
* @return Whether the field field is set.
*/
@java.lang.Override
public boolean hasField() {
return operandTypeCase_ == 2;
}
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*
* @return The field.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReference getField() {
if (fieldBuilder_ == null) {
if (operandTypeCase_ == 2) {
return (com.google.firestore.v1.StructuredQuery.FieldReference) operandType_;
}
return com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance();
} else {
if (operandTypeCase_ == 2) {
return fieldBuilder_.getMessage();
}
return com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance();
}
}
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*/
public Builder setField(com.google.firestore.v1.StructuredQuery.FieldReference value) {
if (fieldBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
operandType_ = value;
onChanged();
} else {
fieldBuilder_.setMessage(value);
}
operandTypeCase_ = 2;
return this;
}
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*/
public Builder setField(
com.google.firestore.v1.StructuredQuery.FieldReference.Builder builderForValue) {
if (fieldBuilder_ == null) {
operandType_ = builderForValue.build();
onChanged();
} else {
fieldBuilder_.setMessage(builderForValue.build());
}
operandTypeCase_ = 2;
return this;
}
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*/
public Builder mergeField(com.google.firestore.v1.StructuredQuery.FieldReference value) {
if (fieldBuilder_ == null) {
if (operandTypeCase_ == 2
&& operandType_
!= com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()) {
operandType_ =
com.google.firestore.v1.StructuredQuery.FieldReference.newBuilder(
(com.google.firestore.v1.StructuredQuery.FieldReference) operandType_)
.mergeFrom(value)
.buildPartial();
} else {
operandType_ = value;
}
onChanged();
} else {
if (operandTypeCase_ == 2) {
fieldBuilder_.mergeFrom(value);
} else {
fieldBuilder_.setMessage(value);
}
}
operandTypeCase_ = 2;
return this;
}
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*/
public Builder clearField() {
if (fieldBuilder_ == null) {
if (operandTypeCase_ == 2) {
operandTypeCase_ = 0;
operandType_ = null;
onChanged();
}
} else {
if (operandTypeCase_ == 2) {
operandTypeCase_ = 0;
operandType_ = null;
}
fieldBuilder_.clear();
}
return this;
}
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*/
public com.google.firestore.v1.StructuredQuery.FieldReference.Builder getFieldBuilder() {
return getFieldFieldBuilder().getBuilder();
}
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldOrBuilder() {
if ((operandTypeCase_ == 2) && (fieldBuilder_ != null)) {
return fieldBuilder_.getMessageOrBuilder();
} else {
if (operandTypeCase_ == 2) {
return (com.google.firestore.v1.StructuredQuery.FieldReference) operandType_;
}
return com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance();
}
}
/**
*
*
* * The field to which to apply the operator.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>
getFieldFieldBuilder() {
if (fieldBuilder_ == null) {
if (!(operandTypeCase_ == 2)) {
operandType_ =
com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance();
}
fieldBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>(
(com.google.firestore.v1.StructuredQuery.FieldReference) operandType_,
getParentForChildren(),
isClean());
operandType_ = null;
}
operandTypeCase_ = 2;
onChanged();
return fieldBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.firestore.v1.StructuredQuery.UnaryFilter)
}
// @@protoc_insertion_point(class_scope:google.firestore.v1.StructuredQuery.UnaryFilter)
private static final com.google.firestore.v1.StructuredQuery.UnaryFilter DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.firestore.v1.StructuredQuery.UnaryFilter();
}
public static com.google.firestore.v1.StructuredQuery.UnaryFilter getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UnaryFilter parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.UnaryFilter getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OrderOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.firestore.v1.StructuredQuery.Order)
com.google.protobuf.MessageOrBuilder {
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return Whether the field field is set.
*/
boolean hasField();
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return The field.
*/
com.google.firestore.v1.StructuredQuery.FieldReference getField();
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldOrBuilder();
/**
*
*
* * The direction to order by. Defaults to `ASCENDING`.
*
*
* .google.firestore.v1.StructuredQuery.Direction direction = 2;
*
* @return The enum numeric value on the wire for direction.
*/
int getDirectionValue();
/**
*
*
* * The direction to order by. Defaults to `ASCENDING`.
*
*
* .google.firestore.v1.StructuredQuery.Direction direction = 2;
*
* @return The direction.
*/
com.google.firestore.v1.StructuredQuery.Direction getDirection();
}
/**
*
*
* * An order on a field.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.Order}
*/
public static final class Order extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.firestore.v1.StructuredQuery.Order)
OrderOrBuilder {
private static final long serialVersionUID = 0L;
// Use Order.newBuilder() to construct.
private Order(com.google.protobuf.GeneratedMessageV3.Builder builder) {
super(builder);
}
private Order() {
direction_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Order();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Order_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Order_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.Order.class,
com.google.firestore.v1.StructuredQuery.Order.Builder.class);
}
private int bitField0_;
public static final int FIELD_FIELD_NUMBER = 1;
private com.google.firestore.v1.StructuredQuery.FieldReference field_;
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return Whether the field field is set.
*/
@java.lang.Override
public boolean hasField() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return The field.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReference getField() {
return field_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: field_;
}
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldOrBuilder() {
return field_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: field_;
}
public static final int DIRECTION_FIELD_NUMBER = 2;
private int direction_ = 0;
/**
*
*
* * The direction to order by. Defaults to `ASCENDING`.
*
*
* .google.firestore.v1.StructuredQuery.Direction direction = 2;
*
* @return The enum numeric value on the wire for direction.
*/
@java.lang.Override
public int getDirectionValue() {
return direction_;
}
/**
*
*
* * The direction to order by. Defaults to `ASCENDING`.
*
*
* .google.firestore.v1.StructuredQuery.Direction direction = 2;
*
* @return The direction.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Direction getDirection() {
com.google.firestore.v1.StructuredQuery.Direction result =
com.google.firestore.v1.StructuredQuery.Direction.forNumber(direction_);
return result == null
? com.google.firestore.v1.StructuredQuery.Direction.UNRECOGNIZED
: result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getField());
}
if (direction_
!= com.google.firestore.v1.StructuredQuery.Direction.DIRECTION_UNSPECIFIED.getNumber()) {
output.writeEnum(2, direction_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(1, getField());
}
if (direction_
!= com.google.firestore.v1.StructuredQuery.Direction.DIRECTION_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(2, direction_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.firestore.v1.StructuredQuery.Order)) {
return super.equals(obj);
}
com.google.firestore.v1.StructuredQuery.Order other =
(com.google.firestore.v1.StructuredQuery.Order) obj;
if (hasField() != other.hasField()) return false;
if (hasField()) {
if (!getField().equals(other.getField())) return false;
}
if (direction_ != other.direction_) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasField()) {
hash = (37 * hash) + FIELD_FIELD_NUMBER;
hash = (53 * hash) + getField().hashCode();
}
hash = (37 * hash) + DIRECTION_FIELD_NUMBER;
hash = (53 * hash) + direction_;
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.firestore.v1.StructuredQuery.Order parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.Order parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Order parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.Order parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Order parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.Order parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Order parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.Order parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Order parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.Order parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Order parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.Order parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.firestore.v1.StructuredQuery.Order prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
* * An order on a field.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.Order}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.firestore.v1.StructuredQuery.Order)
com.google.firestore.v1.StructuredQuery.OrderOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Order_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Order_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.Order.class,
com.google.firestore.v1.StructuredQuery.Order.Builder.class);
}
// Construct using com.google.firestore.v1.StructuredQuery.Order.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getFieldFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
field_ = null;
if (fieldBuilder_ != null) {
fieldBuilder_.dispose();
fieldBuilder_ = null;
}
direction_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Order_descriptor;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Order getDefaultInstanceForType() {
return com.google.firestore.v1.StructuredQuery.Order.getDefaultInstance();
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Order build() {
com.google.firestore.v1.StructuredQuery.Order result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Order buildPartial() {
com.google.firestore.v1.StructuredQuery.Order result =
new com.google.firestore.v1.StructuredQuery.Order(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.firestore.v1.StructuredQuery.Order result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.field_ = fieldBuilder_ == null ? field_ : fieldBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.direction_ = direction_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.firestore.v1.StructuredQuery.Order) {
return mergeFrom((com.google.firestore.v1.StructuredQuery.Order) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.firestore.v1.StructuredQuery.Order other) {
if (other == com.google.firestore.v1.StructuredQuery.Order.getDefaultInstance())
return this;
if (other.hasField()) {
mergeField(other.getField());
}
if (other.direction_ != 0) {
setDirectionValue(other.getDirectionValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
input.readMessage(getFieldFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 16:
{
direction_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.firestore.v1.StructuredQuery.FieldReference field_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>
fieldBuilder_;
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return Whether the field field is set.
*/
public boolean hasField() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*
* @return The field.
*/
public com.google.firestore.v1.StructuredQuery.FieldReference getField() {
if (fieldBuilder_ == null) {
return field_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: field_;
} else {
return fieldBuilder_.getMessage();
}
}
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public Builder setField(com.google.firestore.v1.StructuredQuery.FieldReference value) {
if (fieldBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
field_ = value;
} else {
fieldBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public Builder setField(
com.google.firestore.v1.StructuredQuery.FieldReference.Builder builderForValue) {
if (fieldBuilder_ == null) {
field_ = builderForValue.build();
} else {
fieldBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public Builder mergeField(com.google.firestore.v1.StructuredQuery.FieldReference value) {
if (fieldBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)
&& field_ != null
&& field_
!= com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()) {
getFieldBuilder().mergeFrom(value);
} else {
field_ = value;
}
} else {
fieldBuilder_.mergeFrom(value);
}
if (field_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public Builder clearField() {
bitField0_ = (bitField0_ & ~0x00000001);
field_ = null;
if (fieldBuilder_ != null) {
fieldBuilder_.dispose();
fieldBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public com.google.firestore.v1.StructuredQuery.FieldReference.Builder getFieldBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getFieldFieldBuilder().getBuilder();
}
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
public com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldOrBuilder() {
if (fieldBuilder_ != null) {
return fieldBuilder_.getMessageOrBuilder();
} else {
return field_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: field_;
}
}
/**
*
*
* * The field to order by.
*
*
* .google.firestore.v1.StructuredQuery.FieldReference field = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>
getFieldFieldBuilder() {
if (fieldBuilder_ == null) {
fieldBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>(
getField(), getParentForChildren(), isClean());
field_ = null;
}
return fieldBuilder_;
}
private int direction_ = 0;
/**
*
*
* * The direction to order by. Defaults to `ASCENDING`.
*
*
* .google.firestore.v1.StructuredQuery.Direction direction = 2;
*
* @return The enum numeric value on the wire for direction.
*/
@java.lang.Override
public int getDirectionValue() {
return direction_;
}
/**
*
*
* * The direction to order by. Defaults to `ASCENDING`.
*
*
* .google.firestore.v1.StructuredQuery.Direction direction = 2;
*
* @param value The enum numeric value on the wire for direction to set.
* @return This builder for chaining.
*/
public Builder setDirectionValue(int value) {
direction_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
* * The direction to order by. Defaults to `ASCENDING`.
*
*
* .google.firestore.v1.StructuredQuery.Direction direction = 2;
*
* @return The direction.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Direction getDirection() {
com.google.firestore.v1.StructuredQuery.Direction result =
com.google.firestore.v1.StructuredQuery.Direction.forNumber(direction_);
return result == null
? com.google.firestore.v1.StructuredQuery.Direction.UNRECOGNIZED
: result;
}
/**
*
*
* * The direction to order by. Defaults to `ASCENDING`.
*
*
* .google.firestore.v1.StructuredQuery.Direction direction = 2;
*
* @param value The direction to set.
* @return This builder for chaining.
*/
public Builder setDirection(com.google.firestore.v1.StructuredQuery.Direction value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
direction_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
* * The direction to order by. Defaults to `ASCENDING`.
*
*
* .google.firestore.v1.StructuredQuery.Direction direction = 2;
*
* @return This builder for chaining.
*/
public Builder clearDirection() {
bitField0_ = (bitField0_ & ~0x00000002);
direction_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.firestore.v1.StructuredQuery.Order)
}
// @@protoc_insertion_point(class_scope:google.firestore.v1.StructuredQuery.Order)
private static final com.google.firestore.v1.StructuredQuery.Order DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.firestore.v1.StructuredQuery.Order();
}
public static com.google.firestore.v1.StructuredQuery.Order getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Order parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Order getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FieldReferenceOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.firestore.v1.StructuredQuery.FieldReference)
com.google.protobuf.MessageOrBuilder {
/**
*
*
* * A reference to a field in a document.
*
* Requires:
*
* * MUST be a dot-delimited (`.`) string of segments, where each segment
* conforms to [document field name][google.firestore.v1.Document.fields]
* limitations.
*
*
* string field_path = 2;
*
* @return The fieldPath.
*/
java.lang.String getFieldPath();
/**
*
*
* * A reference to a field in a document.
*
* Requires:
*
* * MUST be a dot-delimited (`.`) string of segments, where each segment
* conforms to [document field name][google.firestore.v1.Document.fields]
* limitations.
*
*
* string field_path = 2;
*
* @return The bytes for fieldPath.
*/
com.google.protobuf.ByteString getFieldPathBytes();
}
/**
*
*
* * A reference to a field in a document, ex: `stats.operations`.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.FieldReference}
*/
public static final class FieldReference extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.firestore.v1.StructuredQuery.FieldReference)
FieldReferenceOrBuilder {
private static final long serialVersionUID = 0L;
// Use FieldReference.newBuilder() to construct.
private FieldReference(com.google.protobuf.GeneratedMessageV3.Builder builder) {
super(builder);
}
private FieldReference() {
fieldPath_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new FieldReference();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FieldReference_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FieldReference_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.FieldReference.class,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder.class);
}
public static final int FIELD_PATH_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object fieldPath_ = "";
/**
*
*
* * A reference to a field in a document.
*
* Requires:
*
* * MUST be a dot-delimited (`.`) string of segments, where each segment
* conforms to [document field name][google.firestore.v1.Document.fields]
* limitations.
*
*
* string field_path = 2;
*
* @return The fieldPath.
*/
@java.lang.Override
public java.lang.String getFieldPath() {
java.lang.Object ref = fieldPath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fieldPath_ = s;
return s;
}
}
/**
*
*
* * A reference to a field in a document.
*
* Requires:
*
* * MUST be a dot-delimited (`.`) string of segments, where each segment
* conforms to [document field name][google.firestore.v1.Document.fields]
* limitations.
*
*
* string field_path = 2;
*
* @return The bytes for fieldPath.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFieldPathBytes() {
java.lang.Object ref = fieldPath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
fieldPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(fieldPath_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, fieldPath_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(fieldPath_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, fieldPath_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.firestore.v1.StructuredQuery.FieldReference)) {
return super.equals(obj);
}
com.google.firestore.v1.StructuredQuery.FieldReference other =
(com.google.firestore.v1.StructuredQuery.FieldReference) obj;
if (!getFieldPath().equals(other.getFieldPath())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FIELD_PATH_FIELD_NUMBER;
hash = (53 * hash) + getFieldPath().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.FieldReference parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.firestore.v1.StructuredQuery.FieldReference prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
* * A reference to a field in a document, ex: `stats.operations`.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.FieldReference}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.firestore.v1.StructuredQuery.FieldReference)
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FieldReference_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FieldReference_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.FieldReference.class,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder.class);
}
// Construct using com.google.firestore.v1.StructuredQuery.FieldReference.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
fieldPath_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FieldReference_descriptor;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReference getDefaultInstanceForType() {
return com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance();
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReference build() {
com.google.firestore.v1.StructuredQuery.FieldReference result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReference buildPartial() {
com.google.firestore.v1.StructuredQuery.FieldReference result =
new com.google.firestore.v1.StructuredQuery.FieldReference(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.firestore.v1.StructuredQuery.FieldReference result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.fieldPath_ = fieldPath_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.firestore.v1.StructuredQuery.FieldReference) {
return mergeFrom((com.google.firestore.v1.StructuredQuery.FieldReference) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.firestore.v1.StructuredQuery.FieldReference other) {
if (other == com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance())
return this;
if (!other.getFieldPath().isEmpty()) {
fieldPath_ = other.fieldPath_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18:
{
fieldPath_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 18
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object fieldPath_ = "";
/**
*
*
* * A reference to a field in a document.
*
* Requires:
*
* * MUST be a dot-delimited (`.`) string of segments, where each segment
* conforms to [document field name][google.firestore.v1.Document.fields]
* limitations.
*
*
* string field_path = 2;
*
* @return The fieldPath.
*/
public java.lang.String getFieldPath() {
java.lang.Object ref = fieldPath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
fieldPath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
* * A reference to a field in a document.
*
* Requires:
*
* * MUST be a dot-delimited (`.`) string of segments, where each segment
* conforms to [document field name][google.firestore.v1.Document.fields]
* limitations.
*
*
* string field_path = 2;
*
* @return The bytes for fieldPath.
*/
public com.google.protobuf.ByteString getFieldPathBytes() {
java.lang.Object ref = fieldPath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
fieldPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
* * A reference to a field in a document.
*
* Requires:
*
* * MUST be a dot-delimited (`.`) string of segments, where each segment
* conforms to [document field name][google.firestore.v1.Document.fields]
* limitations.
*
*
* string field_path = 2;
*
* @param value The fieldPath to set.
* @return This builder for chaining.
*/
public Builder setFieldPath(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
fieldPath_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * A reference to a field in a document.
*
* Requires:
*
* * MUST be a dot-delimited (`.`) string of segments, where each segment
* conforms to [document field name][google.firestore.v1.Document.fields]
* limitations.
*
*
* string field_path = 2;
*
* @return This builder for chaining.
*/
public Builder clearFieldPath() {
fieldPath_ = getDefaultInstance().getFieldPath();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
* * A reference to a field in a document.
*
* Requires:
*
* * MUST be a dot-delimited (`.`) string of segments, where each segment
* conforms to [document field name][google.firestore.v1.Document.fields]
* limitations.
*
*
* string field_path = 2;
*
* @param value The bytes for fieldPath to set.
* @return This builder for chaining.
*/
public Builder setFieldPathBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
fieldPath_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.firestore.v1.StructuredQuery.FieldReference)
}
// @@protoc_insertion_point(class_scope:google.firestore.v1.StructuredQuery.FieldReference)
private static final com.google.firestore.v1.StructuredQuery.FieldReference DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.firestore.v1.StructuredQuery.FieldReference();
}
public static com.google.firestore.v1.StructuredQuery.FieldReference getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FieldReference parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReference getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProjectionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.firestore.v1.StructuredQuery.Projection)
com.google.protobuf.MessageOrBuilder {
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
java.util.List getFieldsList();
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
com.google.firestore.v1.StructuredQuery.FieldReference getFields(int index);
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
int getFieldsCount();
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
java.util.List
getFieldsOrBuilderList();
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldsOrBuilder(int index);
}
/**
*
*
* * The projection of document's fields to return.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.Projection}
*/
public static final class Projection extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.firestore.v1.StructuredQuery.Projection)
ProjectionOrBuilder {
private static final long serialVersionUID = 0L;
// Use Projection.newBuilder() to construct.
private Projection(com.google.protobuf.GeneratedMessageV3.Builder builder) {
super(builder);
}
private Projection() {
fields_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Projection();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Projection_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Projection_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.Projection.class,
com.google.firestore.v1.StructuredQuery.Projection.Builder.class);
}
public static final int FIELDS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List fields_;
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
@java.lang.Override
public java.util.List getFieldsList() {
return fields_;
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
@java.lang.Override
public java.util.List
getFieldsOrBuilderList() {
return fields_;
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
@java.lang.Override
public int getFieldsCount() {
return fields_.size();
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReference getFields(int index) {
return fields_.get(index);
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldsOrBuilder(
int index) {
return fields_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
for (int i = 0; i < fields_.size(); i++) {
output.writeMessage(2, fields_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < fields_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(2, fields_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.firestore.v1.StructuredQuery.Projection)) {
return super.equals(obj);
}
com.google.firestore.v1.StructuredQuery.Projection other =
(com.google.firestore.v1.StructuredQuery.Projection) obj;
if (!getFieldsList().equals(other.getFieldsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getFieldsCount() > 0) {
hash = (37 * hash) + FIELDS_FIELD_NUMBER;
hash = (53 * hash) + getFieldsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.firestore.v1.StructuredQuery.Projection parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.Projection parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Projection parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.Projection parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Projection parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.Projection parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Projection parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.Projection parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Projection parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.Projection parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.Projection parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.Projection parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.firestore.v1.StructuredQuery.Projection prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
* * The projection of document's fields to return.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.Projection}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.firestore.v1.StructuredQuery.Projection)
com.google.firestore.v1.StructuredQuery.ProjectionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Projection_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Projection_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.Projection.class,
com.google.firestore.v1.StructuredQuery.Projection.Builder.class);
}
// Construct using com.google.firestore.v1.StructuredQuery.Projection.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
} else {
fields_ = null;
fieldsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_Projection_descriptor;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Projection getDefaultInstanceForType() {
return com.google.firestore.v1.StructuredQuery.Projection.getDefaultInstance();
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Projection build() {
com.google.firestore.v1.StructuredQuery.Projection result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Projection buildPartial() {
com.google.firestore.v1.StructuredQuery.Projection result =
new com.google.firestore.v1.StructuredQuery.Projection(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(
com.google.firestore.v1.StructuredQuery.Projection result) {
if (fieldsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
fields_ = java.util.Collections.unmodifiableList(fields_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.fields_ = fields_;
} else {
result.fields_ = fieldsBuilder_.build();
}
}
private void buildPartial0(com.google.firestore.v1.StructuredQuery.Projection result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.firestore.v1.StructuredQuery.Projection) {
return mergeFrom((com.google.firestore.v1.StructuredQuery.Projection) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.firestore.v1.StructuredQuery.Projection other) {
if (other == com.google.firestore.v1.StructuredQuery.Projection.getDefaultInstance())
return this;
if (fieldsBuilder_ == null) {
if (!other.fields_.isEmpty()) {
if (fields_.isEmpty()) {
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFieldsIsMutable();
fields_.addAll(other.fields_);
}
onChanged();
}
} else {
if (!other.fields_.isEmpty()) {
if (fieldsBuilder_.isEmpty()) {
fieldsBuilder_.dispose();
fieldsBuilder_ = null;
fields_ = other.fields_;
bitField0_ = (bitField0_ & ~0x00000001);
fieldsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getFieldsFieldBuilder()
: null;
} else {
fieldsBuilder_.addAllMessages(other.fields_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18:
{
com.google.firestore.v1.StructuredQuery.FieldReference m =
input.readMessage(
com.google.firestore.v1.StructuredQuery.FieldReference.parser(),
extensionRegistry);
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(m);
} else {
fieldsBuilder_.addMessage(m);
}
break;
} // case 18
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List fields_ =
java.util.Collections.emptyList();
private void ensureFieldsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
fields_ =
new java.util.ArrayList(
fields_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>
fieldsBuilder_;
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public java.util.List
getFieldsList() {
if (fieldsBuilder_ == null) {
return java.util.Collections.unmodifiableList(fields_);
} else {
return fieldsBuilder_.getMessageList();
}
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public int getFieldsCount() {
if (fieldsBuilder_ == null) {
return fields_.size();
} else {
return fieldsBuilder_.getCount();
}
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public com.google.firestore.v1.StructuredQuery.FieldReference getFields(int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index);
} else {
return fieldsBuilder_.getMessage(index);
}
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public Builder setFields(
int index, com.google.firestore.v1.StructuredQuery.FieldReference value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.set(index, value);
onChanged();
} else {
fieldsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public Builder setFields(
int index,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.set(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public Builder addFields(com.google.firestore.v1.StructuredQuery.FieldReference value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(value);
onChanged();
} else {
fieldsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public Builder addFields(
int index, com.google.firestore.v1.StructuredQuery.FieldReference value) {
if (fieldsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFieldsIsMutable();
fields_.add(index, value);
onChanged();
} else {
fieldsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public Builder addFields(
com.google.firestore.v1.StructuredQuery.FieldReference.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public Builder addFields(
int index,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder builderForValue) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.add(index, builderForValue.build());
onChanged();
} else {
fieldsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public Builder addAllFields(
java.lang.Iterable
values) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, fields_);
onChanged();
} else {
fieldsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public Builder clearFields() {
if (fieldsBuilder_ == null) {
fields_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
fieldsBuilder_.clear();
}
return this;
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public Builder removeFields(int index) {
if (fieldsBuilder_ == null) {
ensureFieldsIsMutable();
fields_.remove(index);
onChanged();
} else {
fieldsBuilder_.remove(index);
}
return this;
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public com.google.firestore.v1.StructuredQuery.FieldReference.Builder getFieldsBuilder(
int index) {
return getFieldsFieldBuilder().getBuilder(index);
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getFieldsOrBuilder(
int index) {
if (fieldsBuilder_ == null) {
return fields_.get(index);
} else {
return fieldsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public java.util.List<
? extends com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>
getFieldsOrBuilderList() {
if (fieldsBuilder_ != null) {
return fieldsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(fields_);
}
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public com.google.firestore.v1.StructuredQuery.FieldReference.Builder addFieldsBuilder() {
return getFieldsFieldBuilder()
.addBuilder(
com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance());
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public com.google.firestore.v1.StructuredQuery.FieldReference.Builder addFieldsBuilder(
int index) {
return getFieldsFieldBuilder()
.addBuilder(
index, com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance());
}
/**
*
*
* * The fields to return.
*
* If empty, all fields are returned. To only return the name
* of the document, use `['__name__']`.
*
*
* repeated .google.firestore.v1.StructuredQuery.FieldReference fields = 2;
*/
public java.util.List
getFieldsBuilderList() {
return getFieldsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>
getFieldsFieldBuilder() {
if (fieldsBuilder_ == null) {
fieldsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>(
fields_, ((bitField0_ & 0x00000001) != 0), getParentForChildren(), isClean());
fields_ = null;
}
return fieldsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.firestore.v1.StructuredQuery.Projection)
}
// @@protoc_insertion_point(class_scope:google.firestore.v1.StructuredQuery.Projection)
private static final com.google.firestore.v1.StructuredQuery.Projection DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.firestore.v1.StructuredQuery.Projection();
}
public static com.google.firestore.v1.StructuredQuery.Projection getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Projection parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Projection getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FindNearestOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.firestore.v1.StructuredQuery.FindNearest)
com.google.protobuf.MessageOrBuilder {
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the vectorField field is set.
*/
boolean hasVectorField();
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The vectorField.
*/
com.google.firestore.v1.StructuredQuery.FieldReference getVectorField();
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder getVectorFieldOrBuilder();
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the queryVector field is set.
*/
boolean hasQueryVector();
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The queryVector.
*/
com.google.firestore.v1.Value getQueryVector();
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*/
com.google.firestore.v1.ValueOrBuilder getQueryVectorOrBuilder();
/**
*
*
* * Required. The distance measure to use, required.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure distance_measure = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The enum numeric value on the wire for distanceMeasure.
*/
int getDistanceMeasureValue();
/**
*
*
* * Required. The distance measure to use, required.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure distance_measure = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The distanceMeasure.
*/
com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure getDistanceMeasure();
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
* @return Whether the limit field is set.
*/
boolean hasLimit();
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
* @return The limit.
*/
com.google.protobuf.Int32Value getLimit();
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*/
com.google.protobuf.Int32ValueOrBuilder getLimitOrBuilder();
/**
*
*
* * Optional. Optional name of the field to output the result of the vector
* distance calculation. Must conform to [document field
* name][google.firestore.v1.Document.fields] limitations.
*
*
* string distance_result_field = 5 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The distanceResultField.
*/
java.lang.String getDistanceResultField();
/**
*
*
* * Optional. Optional name of the field to output the result of the vector
* distance calculation. Must conform to [document field
* name][google.firestore.v1.Document.fields] limitations.
*
*
* string distance_result_field = 5 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for distanceResultField.
*/
com.google.protobuf.ByteString getDistanceResultFieldBytes();
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the distanceThreshold field is set.
*/
boolean hasDistanceThreshold();
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The distanceThreshold.
*/
com.google.protobuf.DoubleValue getDistanceThreshold();
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.protobuf.DoubleValueOrBuilder getDistanceThresholdOrBuilder();
}
/**
*
*
* * Nearest Neighbors search config. The ordering provided by FindNearest
* supersedes the order_by stage. If multiple documents have the same vector
* distance, the returned document order is not guaranteed to be stable
* between queries.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.FindNearest}
*/
public static final class FindNearest extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.firestore.v1.StructuredQuery.FindNearest)
FindNearestOrBuilder {
private static final long serialVersionUID = 0L;
// Use FindNearest.newBuilder() to construct.
private FindNearest(com.google.protobuf.GeneratedMessageV3.Builder builder) {
super(builder);
}
private FindNearest() {
distanceMeasure_ = 0;
distanceResultField_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new FindNearest();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FindNearest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FindNearest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.FindNearest.class,
com.google.firestore.v1.StructuredQuery.FindNearest.Builder.class);
}
/**
*
*
* * The distance measure to use when comparing vectors.
*
*
* Protobuf enum {@code google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure}
*/
public enum DistanceMeasure implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
* * Should not be set.
*
*
* DISTANCE_MEASURE_UNSPECIFIED = 0;
*/
DISTANCE_MEASURE_UNSPECIFIED(0),
/**
*
*
* * Measures the EUCLIDEAN distance between the vectors. See
* [Euclidean](https://en.wikipedia.org/wiki/Euclidean_distance) to learn
* more. The resulting distance decreases the more similar two vectors
* are.
*
*
* EUCLIDEAN = 1;
*/
EUCLIDEAN(1),
/**
*
*
* * COSINE distance compares vectors based on the angle between them, which
* allows you to measure similarity that isn't based on the vectors
* magnitude. We recommend using DOT_PRODUCT with unit normalized vectors
* instead of COSINE distance, which is mathematically equivalent with
* better performance. See [Cosine
* Similarity](https://en.wikipedia.org/wiki/Cosine_similarity) to learn
* more about COSINE similarity and COSINE distance. The resulting
* COSINE distance decreases the more similar two vectors are.
*
*
* COSINE = 2;
*/
COSINE(2),
/**
*
*
* * Similar to cosine but is affected by the magnitude of the vectors. See
* [Dot Product](https://en.wikipedia.org/wiki/Dot_product) to learn more.
* The resulting distance increases the more similar two vectors are.
*
*
* DOT_PRODUCT = 3;
*/
DOT_PRODUCT(3),
UNRECOGNIZED(-1),
;
/**
*
*
* * Should not be set.
*
*
* DISTANCE_MEASURE_UNSPECIFIED = 0;
*/
public static final int DISTANCE_MEASURE_UNSPECIFIED_VALUE = 0;
/**
*
*
* * Measures the EUCLIDEAN distance between the vectors. See
* [Euclidean](https://en.wikipedia.org/wiki/Euclidean_distance) to learn
* more. The resulting distance decreases the more similar two vectors
* are.
*
*
* EUCLIDEAN = 1;
*/
public static final int EUCLIDEAN_VALUE = 1;
/**
*
*
* * COSINE distance compares vectors based on the angle between them, which
* allows you to measure similarity that isn't based on the vectors
* magnitude. We recommend using DOT_PRODUCT with unit normalized vectors
* instead of COSINE distance, which is mathematically equivalent with
* better performance. See [Cosine
* Similarity](https://en.wikipedia.org/wiki/Cosine_similarity) to learn
* more about COSINE similarity and COSINE distance. The resulting
* COSINE distance decreases the more similar two vectors are.
*
*
* COSINE = 2;
*/
public static final int COSINE_VALUE = 2;
/**
*
*
* * Similar to cosine but is affected by the magnitude of the vectors. See
* [Dot Product](https://en.wikipedia.org/wiki/Dot_product) to learn more.
* The resulting distance increases the more similar two vectors are.
*
*
* DOT_PRODUCT = 3;
*/
public static final int DOT_PRODUCT_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DistanceMeasure valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static DistanceMeasure forNumber(int value) {
switch (value) {
case 0:
return DISTANCE_MEASURE_UNSPECIFIED;
case 1:
return EUCLIDEAN;
case 2:
return COSINE;
case 3:
return DOT_PRODUCT;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public DistanceMeasure findValueByNumber(int number) {
return DistanceMeasure.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.firestore.v1.StructuredQuery.FindNearest.getDescriptor()
.getEnumTypes()
.get(0);
}
private static final DistanceMeasure[] VALUES = values();
public static DistanceMeasure valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private DistanceMeasure(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure)
}
private int bitField0_;
public static final int VECTOR_FIELD_FIELD_NUMBER = 1;
private com.google.firestore.v1.StructuredQuery.FieldReference vectorField_;
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the vectorField field is set.
*/
@java.lang.Override
public boolean hasVectorField() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The vectorField.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReference getVectorField() {
return vectorField_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: vectorField_;
}
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder
getVectorFieldOrBuilder() {
return vectorField_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: vectorField_;
}
public static final int QUERY_VECTOR_FIELD_NUMBER = 2;
private com.google.firestore.v1.Value queryVector_;
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the queryVector field is set.
*/
@java.lang.Override
public boolean hasQueryVector() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The queryVector.
*/
@java.lang.Override
public com.google.firestore.v1.Value getQueryVector() {
return queryVector_ == null
? com.google.firestore.v1.Value.getDefaultInstance()
: queryVector_;
}
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*/
@java.lang.Override
public com.google.firestore.v1.ValueOrBuilder getQueryVectorOrBuilder() {
return queryVector_ == null
? com.google.firestore.v1.Value.getDefaultInstance()
: queryVector_;
}
public static final int DISTANCE_MEASURE_FIELD_NUMBER = 3;
private int distanceMeasure_ = 0;
/**
*
*
* * Required. The distance measure to use, required.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure distance_measure = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The enum numeric value on the wire for distanceMeasure.
*/
@java.lang.Override
public int getDistanceMeasureValue() {
return distanceMeasure_;
}
/**
*
*
* * Required. The distance measure to use, required.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure distance_measure = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The distanceMeasure.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure
getDistanceMeasure() {
com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure result =
com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure.forNumber(
distanceMeasure_);
return result == null
? com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure.UNRECOGNIZED
: result;
}
public static final int LIMIT_FIELD_NUMBER = 4;
private com.google.protobuf.Int32Value limit_;
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
* @return Whether the limit field is set.
*/
@java.lang.Override
public boolean hasLimit() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
* @return The limit.
*/
@java.lang.Override
public com.google.protobuf.Int32Value getLimit() {
return limit_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : limit_;
}
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*/
@java.lang.Override
public com.google.protobuf.Int32ValueOrBuilder getLimitOrBuilder() {
return limit_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : limit_;
}
public static final int DISTANCE_RESULT_FIELD_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object distanceResultField_ = "";
/**
*
*
* * Optional. Optional name of the field to output the result of the vector
* distance calculation. Must conform to [document field
* name][google.firestore.v1.Document.fields] limitations.
*
*
* string distance_result_field = 5 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The distanceResultField.
*/
@java.lang.Override
public java.lang.String getDistanceResultField() {
java.lang.Object ref = distanceResultField_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
distanceResultField_ = s;
return s;
}
}
/**
*
*
* * Optional. Optional name of the field to output the result of the vector
* distance calculation. Must conform to [document field
* name][google.firestore.v1.Document.fields] limitations.
*
*
* string distance_result_field = 5 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for distanceResultField.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDistanceResultFieldBytes() {
java.lang.Object ref = distanceResultField_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
distanceResultField_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DISTANCE_THRESHOLD_FIELD_NUMBER = 6;
private com.google.protobuf.DoubleValue distanceThreshold_;
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the distanceThreshold field is set.
*/
@java.lang.Override
public boolean hasDistanceThreshold() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The distanceThreshold.
*/
@java.lang.Override
public com.google.protobuf.DoubleValue getDistanceThreshold() {
return distanceThreshold_ == null
? com.google.protobuf.DoubleValue.getDefaultInstance()
: distanceThreshold_;
}
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.protobuf.DoubleValueOrBuilder getDistanceThresholdOrBuilder() {
return distanceThreshold_ == null
? com.google.protobuf.DoubleValue.getDefaultInstance()
: distanceThreshold_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getVectorField());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getQueryVector());
}
if (distanceMeasure_
!= com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure
.DISTANCE_MEASURE_UNSPECIFIED
.getNumber()) {
output.writeEnum(3, distanceMeasure_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(4, getLimit());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(distanceResultField_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, distanceResultField_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(6, getDistanceThreshold());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(1, getVectorField());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(2, getQueryVector());
}
if (distanceMeasure_
!= com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure
.DISTANCE_MEASURE_UNSPECIFIED
.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(3, distanceMeasure_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(4, getLimit());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(distanceResultField_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, distanceResultField_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(6, getDistanceThreshold());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.firestore.v1.StructuredQuery.FindNearest)) {
return super.equals(obj);
}
com.google.firestore.v1.StructuredQuery.FindNearest other =
(com.google.firestore.v1.StructuredQuery.FindNearest) obj;
if (hasVectorField() != other.hasVectorField()) return false;
if (hasVectorField()) {
if (!getVectorField().equals(other.getVectorField())) return false;
}
if (hasQueryVector() != other.hasQueryVector()) return false;
if (hasQueryVector()) {
if (!getQueryVector().equals(other.getQueryVector())) return false;
}
if (distanceMeasure_ != other.distanceMeasure_) return false;
if (hasLimit() != other.hasLimit()) return false;
if (hasLimit()) {
if (!getLimit().equals(other.getLimit())) return false;
}
if (!getDistanceResultField().equals(other.getDistanceResultField())) return false;
if (hasDistanceThreshold() != other.hasDistanceThreshold()) return false;
if (hasDistanceThreshold()) {
if (!getDistanceThreshold().equals(other.getDistanceThreshold())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasVectorField()) {
hash = (37 * hash) + VECTOR_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getVectorField().hashCode();
}
if (hasQueryVector()) {
hash = (37 * hash) + QUERY_VECTOR_FIELD_NUMBER;
hash = (53 * hash) + getQueryVector().hashCode();
}
hash = (37 * hash) + DISTANCE_MEASURE_FIELD_NUMBER;
hash = (53 * hash) + distanceMeasure_;
if (hasLimit()) {
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getLimit().hashCode();
}
hash = (37 * hash) + DISTANCE_RESULT_FIELD_FIELD_NUMBER;
hash = (53 * hash) + getDistanceResultField().hashCode();
if (hasDistanceThreshold()) {
hash = (37 * hash) + DISTANCE_THRESHOLD_FIELD_NUMBER;
hash = (53 * hash) + getDistanceThreshold().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery.FindNearest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.firestore.v1.StructuredQuery.FindNearest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
* * Nearest Neighbors search config. The ordering provided by FindNearest
* supersedes the order_by stage. If multiple documents have the same vector
* distance, the returned document order is not guaranteed to be stable
* between queries.
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery.FindNearest}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.firestore.v1.StructuredQuery.FindNearest)
com.google.firestore.v1.StructuredQuery.FindNearestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FindNearest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FindNearest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.FindNearest.class,
com.google.firestore.v1.StructuredQuery.FindNearest.Builder.class);
}
// Construct using com.google.firestore.v1.StructuredQuery.FindNearest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getVectorFieldFieldBuilder();
getQueryVectorFieldBuilder();
getLimitFieldBuilder();
getDistanceThresholdFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
vectorField_ = null;
if (vectorFieldBuilder_ != null) {
vectorFieldBuilder_.dispose();
vectorFieldBuilder_ = null;
}
queryVector_ = null;
if (queryVectorBuilder_ != null) {
queryVectorBuilder_.dispose();
queryVectorBuilder_ = null;
}
distanceMeasure_ = 0;
limit_ = null;
if (limitBuilder_ != null) {
limitBuilder_.dispose();
limitBuilder_ = null;
}
distanceResultField_ = "";
distanceThreshold_ = null;
if (distanceThresholdBuilder_ != null) {
distanceThresholdBuilder_.dispose();
distanceThresholdBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_FindNearest_descriptor;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FindNearest getDefaultInstanceForType() {
return com.google.firestore.v1.StructuredQuery.FindNearest.getDefaultInstance();
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FindNearest build() {
com.google.firestore.v1.StructuredQuery.FindNearest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FindNearest buildPartial() {
com.google.firestore.v1.StructuredQuery.FindNearest result =
new com.google.firestore.v1.StructuredQuery.FindNearest(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.firestore.v1.StructuredQuery.FindNearest result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.vectorField_ =
vectorFieldBuilder_ == null ? vectorField_ : vectorFieldBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.queryVector_ =
queryVectorBuilder_ == null ? queryVector_ : queryVectorBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.distanceMeasure_ = distanceMeasure_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.limit_ = limitBuilder_ == null ? limit_ : limitBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.distanceResultField_ = distanceResultField_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.distanceThreshold_ =
distanceThresholdBuilder_ == null
? distanceThreshold_
: distanceThresholdBuilder_.build();
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.firestore.v1.StructuredQuery.FindNearest) {
return mergeFrom((com.google.firestore.v1.StructuredQuery.FindNearest) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.firestore.v1.StructuredQuery.FindNearest other) {
if (other == com.google.firestore.v1.StructuredQuery.FindNearest.getDefaultInstance())
return this;
if (other.hasVectorField()) {
mergeVectorField(other.getVectorField());
}
if (other.hasQueryVector()) {
mergeQueryVector(other.getQueryVector());
}
if (other.distanceMeasure_ != 0) {
setDistanceMeasureValue(other.getDistanceMeasureValue());
}
if (other.hasLimit()) {
mergeLimit(other.getLimit());
}
if (!other.getDistanceResultField().isEmpty()) {
distanceResultField_ = other.distanceResultField_;
bitField0_ |= 0x00000010;
onChanged();
}
if (other.hasDistanceThreshold()) {
mergeDistanceThreshold(other.getDistanceThreshold());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
input.readMessage(getVectorFieldFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
input.readMessage(getQueryVectorFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 24:
{
distanceMeasure_ = input.readEnum();
bitField0_ |= 0x00000004;
break;
} // case 24
case 34:
{
input.readMessage(getLimitFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 42:
{
distanceResultField_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 42
case 50:
{
input.readMessage(
getDistanceThresholdFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 50
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.firestore.v1.StructuredQuery.FieldReference vectorField_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>
vectorFieldBuilder_;
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the vectorField field is set.
*/
public boolean hasVectorField() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The vectorField.
*/
public com.google.firestore.v1.StructuredQuery.FieldReference getVectorField() {
if (vectorFieldBuilder_ == null) {
return vectorField_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: vectorField_;
} else {
return vectorFieldBuilder_.getMessage();
}
}
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setVectorField(com.google.firestore.v1.StructuredQuery.FieldReference value) {
if (vectorFieldBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
vectorField_ = value;
} else {
vectorFieldBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setVectorField(
com.google.firestore.v1.StructuredQuery.FieldReference.Builder builderForValue) {
if (vectorFieldBuilder_ == null) {
vectorField_ = builderForValue.build();
} else {
vectorFieldBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder mergeVectorField(
com.google.firestore.v1.StructuredQuery.FieldReference value) {
if (vectorFieldBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)
&& vectorField_ != null
&& vectorField_
!= com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()) {
getVectorFieldBuilder().mergeFrom(value);
} else {
vectorField_ = value;
}
} else {
vectorFieldBuilder_.mergeFrom(value);
}
if (vectorField_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder clearVectorField() {
bitField0_ = (bitField0_ & ~0x00000001);
vectorField_ = null;
if (vectorFieldBuilder_ != null) {
vectorFieldBuilder_.dispose();
vectorFieldBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.firestore.v1.StructuredQuery.FieldReference.Builder
getVectorFieldBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getVectorFieldFieldBuilder().getBuilder();
}
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder
getVectorFieldOrBuilder() {
if (vectorFieldBuilder_ != null) {
return vectorFieldBuilder_.getMessageOrBuilder();
} else {
return vectorField_ == null
? com.google.firestore.v1.StructuredQuery.FieldReference.getDefaultInstance()
: vectorField_;
}
}
/**
*
*
* * Required. An indexed vector field to search upon. Only documents which
* contain vectors whose dimensionality match the query_vector can be
* returned.
*
*
*
* .google.firestore.v1.StructuredQuery.FieldReference vector_field = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>
getVectorFieldFieldBuilder() {
if (vectorFieldBuilder_ == null) {
vectorFieldBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FieldReference,
com.google.firestore.v1.StructuredQuery.FieldReference.Builder,
com.google.firestore.v1.StructuredQuery.FieldReferenceOrBuilder>(
getVectorField(), getParentForChildren(), isClean());
vectorField_ = null;
}
return vectorFieldBuilder_;
}
private com.google.firestore.v1.Value queryVector_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Value,
com.google.firestore.v1.Value.Builder,
com.google.firestore.v1.ValueOrBuilder>
queryVectorBuilder_;
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the queryVector field is set.
*/
public boolean hasQueryVector() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The queryVector.
*/
public com.google.firestore.v1.Value getQueryVector() {
if (queryVectorBuilder_ == null) {
return queryVector_ == null
? com.google.firestore.v1.Value.getDefaultInstance()
: queryVector_;
} else {
return queryVectorBuilder_.getMessage();
}
}
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setQueryVector(com.google.firestore.v1.Value value) {
if (queryVectorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
queryVector_ = value;
} else {
queryVectorBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setQueryVector(com.google.firestore.v1.Value.Builder builderForValue) {
if (queryVectorBuilder_ == null) {
queryVector_ = builderForValue.build();
} else {
queryVectorBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder mergeQueryVector(com.google.firestore.v1.Value value) {
if (queryVectorBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)
&& queryVector_ != null
&& queryVector_ != com.google.firestore.v1.Value.getDefaultInstance()) {
getQueryVectorBuilder().mergeFrom(value);
} else {
queryVector_ = value;
}
} else {
queryVectorBuilder_.mergeFrom(value);
}
if (queryVector_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder clearQueryVector() {
bitField0_ = (bitField0_ & ~0x00000002);
queryVector_ = null;
if (queryVectorBuilder_ != null) {
queryVectorBuilder_.dispose();
queryVectorBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.firestore.v1.Value.Builder getQueryVectorBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getQueryVectorFieldBuilder().getBuilder();
}
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.firestore.v1.ValueOrBuilder getQueryVectorOrBuilder() {
if (queryVectorBuilder_ != null) {
return queryVectorBuilder_.getMessageOrBuilder();
} else {
return queryVector_ == null
? com.google.firestore.v1.Value.getDefaultInstance()
: queryVector_;
}
}
/**
*
*
* * Required. The query vector that we are searching on. Must be a vector of
* no more than 2048 dimensions.
*
*
*
* .google.firestore.v1.Value query_vector = 2 [(.google.api.field_behavior) = REQUIRED];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Value,
com.google.firestore.v1.Value.Builder,
com.google.firestore.v1.ValueOrBuilder>
getQueryVectorFieldBuilder() {
if (queryVectorBuilder_ == null) {
queryVectorBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Value,
com.google.firestore.v1.Value.Builder,
com.google.firestore.v1.ValueOrBuilder>(
getQueryVector(), getParentForChildren(), isClean());
queryVector_ = null;
}
return queryVectorBuilder_;
}
private int distanceMeasure_ = 0;
/**
*
*
* * Required. The distance measure to use, required.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure distance_measure = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The enum numeric value on the wire for distanceMeasure.
*/
@java.lang.Override
public int getDistanceMeasureValue() {
return distanceMeasure_;
}
/**
*
*
* * Required. The distance measure to use, required.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure distance_measure = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @param value The enum numeric value on the wire for distanceMeasure to set.
* @return This builder for chaining.
*/
public Builder setDistanceMeasureValue(int value) {
distanceMeasure_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
* * Required. The distance measure to use, required.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure distance_measure = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The distanceMeasure.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure
getDistanceMeasure() {
com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure result =
com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure.forNumber(
distanceMeasure_);
return result == null
? com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure.UNRECOGNIZED
: result;
}
/**
*
*
* * Required. The distance measure to use, required.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure distance_measure = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @param value The distanceMeasure to set.
* @return This builder for chaining.
*/
public Builder setDistanceMeasure(
com.google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
distanceMeasure_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
* * Required. The distance measure to use, required.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest.DistanceMeasure distance_measure = 3 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return This builder for chaining.
*/
public Builder clearDistanceMeasure() {
bitField0_ = (bitField0_ & ~0x00000004);
distanceMeasure_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Int32Value limit_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value,
com.google.protobuf.Int32Value.Builder,
com.google.protobuf.Int32ValueOrBuilder>
limitBuilder_;
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the limit field is set.
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The limit.
*/
public com.google.protobuf.Int32Value getLimit() {
if (limitBuilder_ == null) {
return limit_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : limit_;
} else {
return limitBuilder_.getMessage();
}
}
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setLimit(com.google.protobuf.Int32Value value) {
if (limitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
limit_ = value;
} else {
limitBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setLimit(com.google.protobuf.Int32Value.Builder builderForValue) {
if (limitBuilder_ == null) {
limit_ = builderForValue.build();
} else {
limitBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder mergeLimit(com.google.protobuf.Int32Value value) {
if (limitBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& limit_ != null
&& limit_ != com.google.protobuf.Int32Value.getDefaultInstance()) {
getLimitBuilder().mergeFrom(value);
} else {
limit_ = value;
}
} else {
limitBuilder_.mergeFrom(value);
}
if (limit_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder clearLimit() {
bitField0_ = (bitField0_ & ~0x00000008);
limit_ = null;
if (limitBuilder_ != null) {
limitBuilder_.dispose();
limitBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.protobuf.Int32Value.Builder getLimitBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getLimitFieldBuilder().getBuilder();
}
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.protobuf.Int32ValueOrBuilder getLimitOrBuilder() {
if (limitBuilder_ != null) {
return limitBuilder_.getMessageOrBuilder();
} else {
return limit_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : limit_;
}
}
/**
*
*
* * Required. The number of nearest neighbors to return. Must be a positive
* integer of no more than 1000.
*
*
* .google.protobuf.Int32Value limit = 4 [(.google.api.field_behavior) = REQUIRED];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value,
com.google.protobuf.Int32Value.Builder,
com.google.protobuf.Int32ValueOrBuilder>
getLimitFieldBuilder() {
if (limitBuilder_ == null) {
limitBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value,
com.google.protobuf.Int32Value.Builder,
com.google.protobuf.Int32ValueOrBuilder>(
getLimit(), getParentForChildren(), isClean());
limit_ = null;
}
return limitBuilder_;
}
private java.lang.Object distanceResultField_ = "";
/**
*
*
* * Optional. Optional name of the field to output the result of the vector
* distance calculation. Must conform to [document field
* name][google.firestore.v1.Document.fields] limitations.
*
*
* string distance_result_field = 5 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The distanceResultField.
*/
public java.lang.String getDistanceResultField() {
java.lang.Object ref = distanceResultField_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
distanceResultField_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
* * Optional. Optional name of the field to output the result of the vector
* distance calculation. Must conform to [document field
* name][google.firestore.v1.Document.fields] limitations.
*
*
* string distance_result_field = 5 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for distanceResultField.
*/
public com.google.protobuf.ByteString getDistanceResultFieldBytes() {
java.lang.Object ref = distanceResultField_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
distanceResultField_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
* * Optional. Optional name of the field to output the result of the vector
* distance calculation. Must conform to [document field
* name][google.firestore.v1.Document.fields] limitations.
*
*
* string distance_result_field = 5 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The distanceResultField to set.
* @return This builder for chaining.
*/
public Builder setDistanceResultField(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
distanceResultField_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
* * Optional. Optional name of the field to output the result of the vector
* distance calculation. Must conform to [document field
* name][google.firestore.v1.Document.fields] limitations.
*
*
* string distance_result_field = 5 [(.google.api.field_behavior) = OPTIONAL];
*
* @return This builder for chaining.
*/
public Builder clearDistanceResultField() {
distanceResultField_ = getDefaultInstance().getDistanceResultField();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
*
* * Optional. Optional name of the field to output the result of the vector
* distance calculation. Must conform to [document field
* name][google.firestore.v1.Document.fields] limitations.
*
*
* string distance_result_field = 5 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The bytes for distanceResultField to set.
* @return This builder for chaining.
*/
public Builder setDistanceResultFieldBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
distanceResultField_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private com.google.protobuf.DoubleValue distanceThreshold_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.DoubleValue,
com.google.protobuf.DoubleValue.Builder,
com.google.protobuf.DoubleValueOrBuilder>
distanceThresholdBuilder_;
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the distanceThreshold field is set.
*/
public boolean hasDistanceThreshold() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The distanceThreshold.
*/
public com.google.protobuf.DoubleValue getDistanceThreshold() {
if (distanceThresholdBuilder_ == null) {
return distanceThreshold_ == null
? com.google.protobuf.DoubleValue.getDefaultInstance()
: distanceThreshold_;
} else {
return distanceThresholdBuilder_.getMessage();
}
}
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setDistanceThreshold(com.google.protobuf.DoubleValue value) {
if (distanceThresholdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
distanceThreshold_ = value;
} else {
distanceThresholdBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setDistanceThreshold(com.google.protobuf.DoubleValue.Builder builderForValue) {
if (distanceThresholdBuilder_ == null) {
distanceThreshold_ = builderForValue.build();
} else {
distanceThresholdBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeDistanceThreshold(com.google.protobuf.DoubleValue value) {
if (distanceThresholdBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)
&& distanceThreshold_ != null
&& distanceThreshold_ != com.google.protobuf.DoubleValue.getDefaultInstance()) {
getDistanceThresholdBuilder().mergeFrom(value);
} else {
distanceThreshold_ = value;
}
} else {
distanceThresholdBuilder_.mergeFrom(value);
}
if (distanceThreshold_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearDistanceThreshold() {
bitField0_ = (bitField0_ & ~0x00000020);
distanceThreshold_ = null;
if (distanceThresholdBuilder_ != null) {
distanceThresholdBuilder_.dispose();
distanceThresholdBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.protobuf.DoubleValue.Builder getDistanceThresholdBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getDistanceThresholdFieldBuilder().getBuilder();
}
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.protobuf.DoubleValueOrBuilder getDistanceThresholdOrBuilder() {
if (distanceThresholdBuilder_ != null) {
return distanceThresholdBuilder_.getMessageOrBuilder();
} else {
return distanceThreshold_ == null
? com.google.protobuf.DoubleValue.getDefaultInstance()
: distanceThreshold_;
}
}
/**
*
*
* * Optional. Option to specify a threshold for which no less similar
* documents will be returned. The behavior of the specified
* `distance_measure` will affect the meaning of the distance threshold.
* Since DOT_PRODUCT distances increase when the vectors are more similar,
* the comparison is inverted.
*
* For EUCLIDEAN, COSINE: WHERE distance <= distance_threshold
* For DOT_PRODUCT: WHERE distance >= distance_threshold
*
*
*
* .google.protobuf.DoubleValue distance_threshold = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.DoubleValue,
com.google.protobuf.DoubleValue.Builder,
com.google.protobuf.DoubleValueOrBuilder>
getDistanceThresholdFieldBuilder() {
if (distanceThresholdBuilder_ == null) {
distanceThresholdBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.DoubleValue,
com.google.protobuf.DoubleValue.Builder,
com.google.protobuf.DoubleValueOrBuilder>(
getDistanceThreshold(), getParentForChildren(), isClean());
distanceThreshold_ = null;
}
return distanceThresholdBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.firestore.v1.StructuredQuery.FindNearest)
}
// @@protoc_insertion_point(class_scope:google.firestore.v1.StructuredQuery.FindNearest)
private static final com.google.firestore.v1.StructuredQuery.FindNearest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.firestore.v1.StructuredQuery.FindNearest();
}
public static com.google.firestore.v1.StructuredQuery.FindNearest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FindNearest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FindNearest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int SELECT_FIELD_NUMBER = 1;
private com.google.firestore.v1.StructuredQuery.Projection select_;
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*
* @return Whether the select field is set.
*/
@java.lang.Override
public boolean hasSelect() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*
* @return The select.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Projection getSelect() {
return select_ == null
? com.google.firestore.v1.StructuredQuery.Projection.getDefaultInstance()
: select_;
}
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.ProjectionOrBuilder getSelectOrBuilder() {
return select_ == null
? com.google.firestore.v1.StructuredQuery.Projection.getDefaultInstance()
: select_;
}
public static final int FROM_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private java.util.List from_;
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
@java.lang.Override
public java.util.List getFromList() {
return from_;
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
@java.lang.Override
public java.util.List<
? extends com.google.firestore.v1.StructuredQuery.CollectionSelectorOrBuilder>
getFromOrBuilderList() {
return from_;
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
@java.lang.Override
public int getFromCount() {
return from_.size();
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CollectionSelector getFrom(int index) {
return from_.get(index);
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.CollectionSelectorOrBuilder getFromOrBuilder(
int index) {
return from_.get(index);
}
public static final int WHERE_FIELD_NUMBER = 3;
private com.google.firestore.v1.StructuredQuery.Filter where_;
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*
* @return Whether the where field is set.
*/
@java.lang.Override
public boolean hasWhere() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*
* @return The where.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Filter getWhere() {
return where_ == null
? com.google.firestore.v1.StructuredQuery.Filter.getDefaultInstance()
: where_;
}
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FilterOrBuilder getWhereOrBuilder() {
return where_ == null
? com.google.firestore.v1.StructuredQuery.Filter.getDefaultInstance()
: where_;
}
public static final int ORDER_BY_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private java.util.List orderBy_;
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
@java.lang.Override
public java.util.List getOrderByList() {
return orderBy_;
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
@java.lang.Override
public java.util.List
getOrderByOrBuilderList() {
return orderBy_;
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
@java.lang.Override
public int getOrderByCount() {
return orderBy_.size();
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.Order getOrderBy(int index) {
return orderBy_.get(index);
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.OrderOrBuilder getOrderByOrBuilder(int index) {
return orderBy_.get(index);
}
public static final int START_AT_FIELD_NUMBER = 7;
private com.google.firestore.v1.Cursor startAt_;
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*
* @return Whether the startAt field is set.
*/
@java.lang.Override
public boolean hasStartAt() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*
* @return The startAt.
*/
@java.lang.Override
public com.google.firestore.v1.Cursor getStartAt() {
return startAt_ == null ? com.google.firestore.v1.Cursor.getDefaultInstance() : startAt_;
}
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*/
@java.lang.Override
public com.google.firestore.v1.CursorOrBuilder getStartAtOrBuilder() {
return startAt_ == null ? com.google.firestore.v1.Cursor.getDefaultInstance() : startAt_;
}
public static final int END_AT_FIELD_NUMBER = 8;
private com.google.firestore.v1.Cursor endAt_;
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*
* @return Whether the endAt field is set.
*/
@java.lang.Override
public boolean hasEndAt() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*
* @return The endAt.
*/
@java.lang.Override
public com.google.firestore.v1.Cursor getEndAt() {
return endAt_ == null ? com.google.firestore.v1.Cursor.getDefaultInstance() : endAt_;
}
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*/
@java.lang.Override
public com.google.firestore.v1.CursorOrBuilder getEndAtOrBuilder() {
return endAt_ == null ? com.google.firestore.v1.Cursor.getDefaultInstance() : endAt_;
}
public static final int OFFSET_FIELD_NUMBER = 6;
private int offset_ = 0;
/**
*
*
* * The number of documents to skip before returning the first result.
*
* This applies after the constraints specified by the `WHERE`, `START AT`, &
* `END AT` but before the `LIMIT` clause.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* int32 offset = 6;
*
* @return The offset.
*/
@java.lang.Override
public int getOffset() {
return offset_;
}
public static final int LIMIT_FIELD_NUMBER = 5;
private com.google.protobuf.Int32Value limit_;
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*
* @return Whether the limit field is set.
*/
@java.lang.Override
public boolean hasLimit() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*
* @return The limit.
*/
@java.lang.Override
public com.google.protobuf.Int32Value getLimit() {
return limit_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : limit_;
}
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*/
@java.lang.Override
public com.google.protobuf.Int32ValueOrBuilder getLimitOrBuilder() {
return limit_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : limit_;
}
public static final int FIND_NEAREST_FIELD_NUMBER = 9;
private com.google.firestore.v1.StructuredQuery.FindNearest findNearest_;
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the findNearest field is set.
*/
@java.lang.Override
public boolean hasFindNearest() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The findNearest.
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FindNearest getFindNearest() {
return findNearest_ == null
? com.google.firestore.v1.StructuredQuery.FindNearest.getDefaultInstance()
: findNearest_;
}
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
@java.lang.Override
public com.google.firestore.v1.StructuredQuery.FindNearestOrBuilder getFindNearestOrBuilder() {
return findNearest_ == null
? com.google.firestore.v1.StructuredQuery.FindNearest.getDefaultInstance()
: findNearest_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getSelect());
}
for (int i = 0; i < from_.size(); i++) {
output.writeMessage(2, from_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(3, getWhere());
}
for (int i = 0; i < orderBy_.size(); i++) {
output.writeMessage(4, orderBy_.get(i));
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(5, getLimit());
}
if (offset_ != 0) {
output.writeInt32(6, offset_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(7, getStartAt());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(8, getEndAt());
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(9, getFindNearest());
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(1, getSelect());
}
for (int i = 0; i < from_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(2, from_.get(i));
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(3, getWhere());
}
for (int i = 0; i < orderBy_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(4, orderBy_.get(i));
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(5, getLimit());
}
if (offset_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(6, offset_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(7, getStartAt());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(8, getEndAt());
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(9, getFindNearest());
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.firestore.v1.StructuredQuery)) {
return super.equals(obj);
}
com.google.firestore.v1.StructuredQuery other = (com.google.firestore.v1.StructuredQuery) obj;
if (hasSelect() != other.hasSelect()) return false;
if (hasSelect()) {
if (!getSelect().equals(other.getSelect())) return false;
}
if (!getFromList().equals(other.getFromList())) return false;
if (hasWhere() != other.hasWhere()) return false;
if (hasWhere()) {
if (!getWhere().equals(other.getWhere())) return false;
}
if (!getOrderByList().equals(other.getOrderByList())) return false;
if (hasStartAt() != other.hasStartAt()) return false;
if (hasStartAt()) {
if (!getStartAt().equals(other.getStartAt())) return false;
}
if (hasEndAt() != other.hasEndAt()) return false;
if (hasEndAt()) {
if (!getEndAt().equals(other.getEndAt())) return false;
}
if (getOffset() != other.getOffset()) return false;
if (hasLimit() != other.hasLimit()) return false;
if (hasLimit()) {
if (!getLimit().equals(other.getLimit())) return false;
}
if (hasFindNearest() != other.hasFindNearest()) return false;
if (hasFindNearest()) {
if (!getFindNearest().equals(other.getFindNearest())) return false;
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasSelect()) {
hash = (37 * hash) + SELECT_FIELD_NUMBER;
hash = (53 * hash) + getSelect().hashCode();
}
if (getFromCount() > 0) {
hash = (37 * hash) + FROM_FIELD_NUMBER;
hash = (53 * hash) + getFromList().hashCode();
}
if (hasWhere()) {
hash = (37 * hash) + WHERE_FIELD_NUMBER;
hash = (53 * hash) + getWhere().hashCode();
}
if (getOrderByCount() > 0) {
hash = (37 * hash) + ORDER_BY_FIELD_NUMBER;
hash = (53 * hash) + getOrderByList().hashCode();
}
if (hasStartAt()) {
hash = (37 * hash) + START_AT_FIELD_NUMBER;
hash = (53 * hash) + getStartAt().hashCode();
}
if (hasEndAt()) {
hash = (37 * hash) + END_AT_FIELD_NUMBER;
hash = (53 * hash) + getEndAt().hashCode();
}
hash = (37 * hash) + OFFSET_FIELD_NUMBER;
hash = (53 * hash) + getOffset();
if (hasLimit()) {
hash = (37 * hash) + LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getLimit().hashCode();
}
if (hasFindNearest()) {
hash = (37 * hash) + FIND_NEAREST_FIELD_NUMBER;
hash = (53 * hash) + getFindNearest().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.firestore.v1.StructuredQuery parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.firestore.v1.StructuredQuery parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.firestore.v1.StructuredQuery parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.firestore.v1.StructuredQuery parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.firestore.v1.StructuredQuery prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
* * A Firestore query.
*
* The query stages are executed in the following order:
* 1. from
* 2. where
* 3. select
* 4. order_by + start_at + end_at
* 5. offset
* 6. limit
*
*
* Protobuf type {@code google.firestore.v1.StructuredQuery}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.firestore.v1.StructuredQuery)
com.google.firestore.v1.StructuredQueryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.firestore.v1.StructuredQuery.class,
com.google.firestore.v1.StructuredQuery.Builder.class);
}
// Construct using com.google.firestore.v1.StructuredQuery.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getSelectFieldBuilder();
getFromFieldBuilder();
getWhereFieldBuilder();
getOrderByFieldBuilder();
getStartAtFieldBuilder();
getEndAtFieldBuilder();
getLimitFieldBuilder();
getFindNearestFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
select_ = null;
if (selectBuilder_ != null) {
selectBuilder_.dispose();
selectBuilder_ = null;
}
if (fromBuilder_ == null) {
from_ = java.util.Collections.emptyList();
} else {
from_ = null;
fromBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
where_ = null;
if (whereBuilder_ != null) {
whereBuilder_.dispose();
whereBuilder_ = null;
}
if (orderByBuilder_ == null) {
orderBy_ = java.util.Collections.emptyList();
} else {
orderBy_ = null;
orderByBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000008);
startAt_ = null;
if (startAtBuilder_ != null) {
startAtBuilder_.dispose();
startAtBuilder_ = null;
}
endAt_ = null;
if (endAtBuilder_ != null) {
endAtBuilder_.dispose();
endAtBuilder_ = null;
}
offset_ = 0;
limit_ = null;
if (limitBuilder_ != null) {
limitBuilder_.dispose();
limitBuilder_ = null;
}
findNearest_ = null;
if (findNearestBuilder_ != null) {
findNearestBuilder_.dispose();
findNearestBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.firestore.v1.QueryProto
.internal_static_google_firestore_v1_StructuredQuery_descriptor;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery getDefaultInstanceForType() {
return com.google.firestore.v1.StructuredQuery.getDefaultInstance();
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery build() {
com.google.firestore.v1.StructuredQuery result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery buildPartial() {
com.google.firestore.v1.StructuredQuery result =
new com.google.firestore.v1.StructuredQuery(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.firestore.v1.StructuredQuery result) {
if (fromBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
from_ = java.util.Collections.unmodifiableList(from_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.from_ = from_;
} else {
result.from_ = fromBuilder_.build();
}
if (orderByBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
orderBy_ = java.util.Collections.unmodifiableList(orderBy_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.orderBy_ = orderBy_;
} else {
result.orderBy_ = orderByBuilder_.build();
}
}
private void buildPartial0(com.google.firestore.v1.StructuredQuery result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.select_ = selectBuilder_ == null ? select_ : selectBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.where_ = whereBuilder_ == null ? where_ : whereBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.startAt_ = startAtBuilder_ == null ? startAt_ : startAtBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.endAt_ = endAtBuilder_ == null ? endAt_ : endAtBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.offset_ = offset_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.limit_ = limitBuilder_ == null ? limit_ : limitBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.findNearest_ =
findNearestBuilder_ == null ? findNearest_ : findNearestBuilder_.build();
to_bitField0_ |= 0x00000020;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.firestore.v1.StructuredQuery) {
return mergeFrom((com.google.firestore.v1.StructuredQuery) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.firestore.v1.StructuredQuery other) {
if (other == com.google.firestore.v1.StructuredQuery.getDefaultInstance()) return this;
if (other.hasSelect()) {
mergeSelect(other.getSelect());
}
if (fromBuilder_ == null) {
if (!other.from_.isEmpty()) {
if (from_.isEmpty()) {
from_ = other.from_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureFromIsMutable();
from_.addAll(other.from_);
}
onChanged();
}
} else {
if (!other.from_.isEmpty()) {
if (fromBuilder_.isEmpty()) {
fromBuilder_.dispose();
fromBuilder_ = null;
from_ = other.from_;
bitField0_ = (bitField0_ & ~0x00000002);
fromBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getFromFieldBuilder()
: null;
} else {
fromBuilder_.addAllMessages(other.from_);
}
}
}
if (other.hasWhere()) {
mergeWhere(other.getWhere());
}
if (orderByBuilder_ == null) {
if (!other.orderBy_.isEmpty()) {
if (orderBy_.isEmpty()) {
orderBy_ = other.orderBy_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureOrderByIsMutable();
orderBy_.addAll(other.orderBy_);
}
onChanged();
}
} else {
if (!other.orderBy_.isEmpty()) {
if (orderByBuilder_.isEmpty()) {
orderByBuilder_.dispose();
orderByBuilder_ = null;
orderBy_ = other.orderBy_;
bitField0_ = (bitField0_ & ~0x00000008);
orderByBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getOrderByFieldBuilder()
: null;
} else {
orderByBuilder_.addAllMessages(other.orderBy_);
}
}
}
if (other.hasStartAt()) {
mergeStartAt(other.getStartAt());
}
if (other.hasEndAt()) {
mergeEndAt(other.getEndAt());
}
if (other.getOffset() != 0) {
setOffset(other.getOffset());
}
if (other.hasLimit()) {
mergeLimit(other.getLimit());
}
if (other.hasFindNearest()) {
mergeFindNearest(other.getFindNearest());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
input.readMessage(getSelectFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
com.google.firestore.v1.StructuredQuery.CollectionSelector m =
input.readMessage(
com.google.firestore.v1.StructuredQuery.CollectionSelector.parser(),
extensionRegistry);
if (fromBuilder_ == null) {
ensureFromIsMutable();
from_.add(m);
} else {
fromBuilder_.addMessage(m);
}
break;
} // case 18
case 26:
{
input.readMessage(getWhereFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 34:
{
com.google.firestore.v1.StructuredQuery.Order m =
input.readMessage(
com.google.firestore.v1.StructuredQuery.Order.parser(), extensionRegistry);
if (orderByBuilder_ == null) {
ensureOrderByIsMutable();
orderBy_.add(m);
} else {
orderByBuilder_.addMessage(m);
}
break;
} // case 34
case 42:
{
input.readMessage(getLimitFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 42
case 48:
{
offset_ = input.readInt32();
bitField0_ |= 0x00000040;
break;
} // case 48
case 58:
{
input.readMessage(getStartAtFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 58
case 66:
{
input.readMessage(getEndAtFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000020;
break;
} // case 66
case 74:
{
input.readMessage(getFindNearestFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 74
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.firestore.v1.StructuredQuery.Projection select_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Projection,
com.google.firestore.v1.StructuredQuery.Projection.Builder,
com.google.firestore.v1.StructuredQuery.ProjectionOrBuilder>
selectBuilder_;
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*
* @return Whether the select field is set.
*/
public boolean hasSelect() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*
* @return The select.
*/
public com.google.firestore.v1.StructuredQuery.Projection getSelect() {
if (selectBuilder_ == null) {
return select_ == null
? com.google.firestore.v1.StructuredQuery.Projection.getDefaultInstance()
: select_;
} else {
return selectBuilder_.getMessage();
}
}
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*/
public Builder setSelect(com.google.firestore.v1.StructuredQuery.Projection value) {
if (selectBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
select_ = value;
} else {
selectBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*/
public Builder setSelect(
com.google.firestore.v1.StructuredQuery.Projection.Builder builderForValue) {
if (selectBuilder_ == null) {
select_ = builderForValue.build();
} else {
selectBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*/
public Builder mergeSelect(com.google.firestore.v1.StructuredQuery.Projection value) {
if (selectBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)
&& select_ != null
&& select_ != com.google.firestore.v1.StructuredQuery.Projection.getDefaultInstance()) {
getSelectBuilder().mergeFrom(value);
} else {
select_ = value;
}
} else {
selectBuilder_.mergeFrom(value);
}
if (select_ != null) {
bitField0_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*/
public Builder clearSelect() {
bitField0_ = (bitField0_ & ~0x00000001);
select_ = null;
if (selectBuilder_ != null) {
selectBuilder_.dispose();
selectBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*/
public com.google.firestore.v1.StructuredQuery.Projection.Builder getSelectBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getSelectFieldBuilder().getBuilder();
}
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*/
public com.google.firestore.v1.StructuredQuery.ProjectionOrBuilder getSelectOrBuilder() {
if (selectBuilder_ != null) {
return selectBuilder_.getMessageOrBuilder();
} else {
return select_ == null
? com.google.firestore.v1.StructuredQuery.Projection.getDefaultInstance()
: select_;
}
}
/**
*
*
* * Optional sub-set of the fields to return.
*
* This acts as a [DocumentMask][google.firestore.v1.DocumentMask] over the
* documents returned from a query. When not set, assumes that the caller
* wants all fields returned.
*
*
* .google.firestore.v1.StructuredQuery.Projection select = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Projection,
com.google.firestore.v1.StructuredQuery.Projection.Builder,
com.google.firestore.v1.StructuredQuery.ProjectionOrBuilder>
getSelectFieldBuilder() {
if (selectBuilder_ == null) {
selectBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Projection,
com.google.firestore.v1.StructuredQuery.Projection.Builder,
com.google.firestore.v1.StructuredQuery.ProjectionOrBuilder>(
getSelect(), getParentForChildren(), isClean());
select_ = null;
}
return selectBuilder_;
}
private java.util.List from_ =
java.util.Collections.emptyList();
private void ensureFromIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
from_ =
new java.util.ArrayList(
from_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.CollectionSelector,
com.google.firestore.v1.StructuredQuery.CollectionSelector.Builder,
com.google.firestore.v1.StructuredQuery.CollectionSelectorOrBuilder>
fromBuilder_;
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public java.util.List
getFromList() {
if (fromBuilder_ == null) {
return java.util.Collections.unmodifiableList(from_);
} else {
return fromBuilder_.getMessageList();
}
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public int getFromCount() {
if (fromBuilder_ == null) {
return from_.size();
} else {
return fromBuilder_.getCount();
}
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public com.google.firestore.v1.StructuredQuery.CollectionSelector getFrom(int index) {
if (fromBuilder_ == null) {
return from_.get(index);
} else {
return fromBuilder_.getMessage(index);
}
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public Builder setFrom(
int index, com.google.firestore.v1.StructuredQuery.CollectionSelector value) {
if (fromBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFromIsMutable();
from_.set(index, value);
onChanged();
} else {
fromBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public Builder setFrom(
int index,
com.google.firestore.v1.StructuredQuery.CollectionSelector.Builder builderForValue) {
if (fromBuilder_ == null) {
ensureFromIsMutable();
from_.set(index, builderForValue.build());
onChanged();
} else {
fromBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public Builder addFrom(com.google.firestore.v1.StructuredQuery.CollectionSelector value) {
if (fromBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFromIsMutable();
from_.add(value);
onChanged();
} else {
fromBuilder_.addMessage(value);
}
return this;
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public Builder addFrom(
int index, com.google.firestore.v1.StructuredQuery.CollectionSelector value) {
if (fromBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFromIsMutable();
from_.add(index, value);
onChanged();
} else {
fromBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public Builder addFrom(
com.google.firestore.v1.StructuredQuery.CollectionSelector.Builder builderForValue) {
if (fromBuilder_ == null) {
ensureFromIsMutable();
from_.add(builderForValue.build());
onChanged();
} else {
fromBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public Builder addFrom(
int index,
com.google.firestore.v1.StructuredQuery.CollectionSelector.Builder builderForValue) {
if (fromBuilder_ == null) {
ensureFromIsMutable();
from_.add(index, builderForValue.build());
onChanged();
} else {
fromBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public Builder addAllFrom(
java.lang.Iterable
values) {
if (fromBuilder_ == null) {
ensureFromIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, from_);
onChanged();
} else {
fromBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public Builder clearFrom() {
if (fromBuilder_ == null) {
from_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
fromBuilder_.clear();
}
return this;
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public Builder removeFrom(int index) {
if (fromBuilder_ == null) {
ensureFromIsMutable();
from_.remove(index);
onChanged();
} else {
fromBuilder_.remove(index);
}
return this;
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public com.google.firestore.v1.StructuredQuery.CollectionSelector.Builder getFromBuilder(
int index) {
return getFromFieldBuilder().getBuilder(index);
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public com.google.firestore.v1.StructuredQuery.CollectionSelectorOrBuilder getFromOrBuilder(
int index) {
if (fromBuilder_ == null) {
return from_.get(index);
} else {
return fromBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public java.util.List<
? extends com.google.firestore.v1.StructuredQuery.CollectionSelectorOrBuilder>
getFromOrBuilderList() {
if (fromBuilder_ != null) {
return fromBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(from_);
}
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public com.google.firestore.v1.StructuredQuery.CollectionSelector.Builder addFromBuilder() {
return getFromFieldBuilder()
.addBuilder(
com.google.firestore.v1.StructuredQuery.CollectionSelector.getDefaultInstance());
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public com.google.firestore.v1.StructuredQuery.CollectionSelector.Builder addFromBuilder(
int index) {
return getFromFieldBuilder()
.addBuilder(
index,
com.google.firestore.v1.StructuredQuery.CollectionSelector.getDefaultInstance());
}
/**
*
*
* * The collections to query.
*
*
* repeated .google.firestore.v1.StructuredQuery.CollectionSelector from = 2;
*/
public java.util.List
getFromBuilderList() {
return getFromFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.CollectionSelector,
com.google.firestore.v1.StructuredQuery.CollectionSelector.Builder,
com.google.firestore.v1.StructuredQuery.CollectionSelectorOrBuilder>
getFromFieldBuilder() {
if (fromBuilder_ == null) {
fromBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.CollectionSelector,
com.google.firestore.v1.StructuredQuery.CollectionSelector.Builder,
com.google.firestore.v1.StructuredQuery.CollectionSelectorOrBuilder>(
from_, ((bitField0_ & 0x00000002) != 0), getParentForChildren(), isClean());
from_ = null;
}
return fromBuilder_;
}
private com.google.firestore.v1.StructuredQuery.Filter where_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Filter,
com.google.firestore.v1.StructuredQuery.Filter.Builder,
com.google.firestore.v1.StructuredQuery.FilterOrBuilder>
whereBuilder_;
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*
* @return Whether the where field is set.
*/
public boolean hasWhere() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*
* @return The where.
*/
public com.google.firestore.v1.StructuredQuery.Filter getWhere() {
if (whereBuilder_ == null) {
return where_ == null
? com.google.firestore.v1.StructuredQuery.Filter.getDefaultInstance()
: where_;
} else {
return whereBuilder_.getMessage();
}
}
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*/
public Builder setWhere(com.google.firestore.v1.StructuredQuery.Filter value) {
if (whereBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
where_ = value;
} else {
whereBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*/
public Builder setWhere(
com.google.firestore.v1.StructuredQuery.Filter.Builder builderForValue) {
if (whereBuilder_ == null) {
where_ = builderForValue.build();
} else {
whereBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*/
public Builder mergeWhere(com.google.firestore.v1.StructuredQuery.Filter value) {
if (whereBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)
&& where_ != null
&& where_ != com.google.firestore.v1.StructuredQuery.Filter.getDefaultInstance()) {
getWhereBuilder().mergeFrom(value);
} else {
where_ = value;
}
} else {
whereBuilder_.mergeFrom(value);
}
if (where_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*/
public Builder clearWhere() {
bitField0_ = (bitField0_ & ~0x00000004);
where_ = null;
if (whereBuilder_ != null) {
whereBuilder_.dispose();
whereBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*/
public com.google.firestore.v1.StructuredQuery.Filter.Builder getWhereBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getWhereFieldBuilder().getBuilder();
}
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*/
public com.google.firestore.v1.StructuredQuery.FilterOrBuilder getWhereOrBuilder() {
if (whereBuilder_ != null) {
return whereBuilder_.getMessageOrBuilder();
} else {
return where_ == null
? com.google.firestore.v1.StructuredQuery.Filter.getDefaultInstance()
: where_;
}
}
/**
*
*
* * The filter to apply.
*
*
* .google.firestore.v1.StructuredQuery.Filter where = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Filter,
com.google.firestore.v1.StructuredQuery.Filter.Builder,
com.google.firestore.v1.StructuredQuery.FilterOrBuilder>
getWhereFieldBuilder() {
if (whereBuilder_ == null) {
whereBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Filter,
com.google.firestore.v1.StructuredQuery.Filter.Builder,
com.google.firestore.v1.StructuredQuery.FilterOrBuilder>(
getWhere(), getParentForChildren(), isClean());
where_ = null;
}
return whereBuilder_;
}
private java.util.List orderBy_ =
java.util.Collections.emptyList();
private void ensureOrderByIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
orderBy_ = new java.util.ArrayList(orderBy_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Order,
com.google.firestore.v1.StructuredQuery.Order.Builder,
com.google.firestore.v1.StructuredQuery.OrderOrBuilder>
orderByBuilder_;
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public java.util.List getOrderByList() {
if (orderByBuilder_ == null) {
return java.util.Collections.unmodifiableList(orderBy_);
} else {
return orderByBuilder_.getMessageList();
}
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public int getOrderByCount() {
if (orderByBuilder_ == null) {
return orderBy_.size();
} else {
return orderByBuilder_.getCount();
}
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public com.google.firestore.v1.StructuredQuery.Order getOrderBy(int index) {
if (orderByBuilder_ == null) {
return orderBy_.get(index);
} else {
return orderByBuilder_.getMessage(index);
}
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public Builder setOrderBy(int index, com.google.firestore.v1.StructuredQuery.Order value) {
if (orderByBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrderByIsMutable();
orderBy_.set(index, value);
onChanged();
} else {
orderByBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public Builder setOrderBy(
int index, com.google.firestore.v1.StructuredQuery.Order.Builder builderForValue) {
if (orderByBuilder_ == null) {
ensureOrderByIsMutable();
orderBy_.set(index, builderForValue.build());
onChanged();
} else {
orderByBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public Builder addOrderBy(com.google.firestore.v1.StructuredQuery.Order value) {
if (orderByBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrderByIsMutable();
orderBy_.add(value);
onChanged();
} else {
orderByBuilder_.addMessage(value);
}
return this;
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public Builder addOrderBy(int index, com.google.firestore.v1.StructuredQuery.Order value) {
if (orderByBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrderByIsMutable();
orderBy_.add(index, value);
onChanged();
} else {
orderByBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public Builder addOrderBy(
com.google.firestore.v1.StructuredQuery.Order.Builder builderForValue) {
if (orderByBuilder_ == null) {
ensureOrderByIsMutable();
orderBy_.add(builderForValue.build());
onChanged();
} else {
orderByBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public Builder addOrderBy(
int index, com.google.firestore.v1.StructuredQuery.Order.Builder builderForValue) {
if (orderByBuilder_ == null) {
ensureOrderByIsMutable();
orderBy_.add(index, builderForValue.build());
onChanged();
} else {
orderByBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public Builder addAllOrderBy(
java.lang.Iterable values) {
if (orderByBuilder_ == null) {
ensureOrderByIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, orderBy_);
onChanged();
} else {
orderByBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public Builder clearOrderBy() {
if (orderByBuilder_ == null) {
orderBy_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
orderByBuilder_.clear();
}
return this;
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public Builder removeOrderBy(int index) {
if (orderByBuilder_ == null) {
ensureOrderByIsMutable();
orderBy_.remove(index);
onChanged();
} else {
orderByBuilder_.remove(index);
}
return this;
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public com.google.firestore.v1.StructuredQuery.Order.Builder getOrderByBuilder(int index) {
return getOrderByFieldBuilder().getBuilder(index);
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public com.google.firestore.v1.StructuredQuery.OrderOrBuilder getOrderByOrBuilder(int index) {
if (orderByBuilder_ == null) {
return orderBy_.get(index);
} else {
return orderByBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public java.util.List
getOrderByOrBuilderList() {
if (orderByBuilder_ != null) {
return orderByBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(orderBy_);
}
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public com.google.firestore.v1.StructuredQuery.Order.Builder addOrderByBuilder() {
return getOrderByFieldBuilder()
.addBuilder(com.google.firestore.v1.StructuredQuery.Order.getDefaultInstance());
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public com.google.firestore.v1.StructuredQuery.Order.Builder addOrderByBuilder(int index) {
return getOrderByFieldBuilder()
.addBuilder(index, com.google.firestore.v1.StructuredQuery.Order.getDefaultInstance());
}
/**
*
*
* * The order to apply to the query results.
*
* Firestore allows callers to provide a full ordering, a partial ordering, or
* no ordering at all. In all cases, Firestore guarantees a stable ordering
* through the following rules:
*
* * The `order_by` is required to reference all fields used with an
* inequality filter.
* * All fields that are required to be in the `order_by` but are not already
* present are appended in lexicographical ordering of the field name.
* * If an order on `__name__` is not specified, it is appended by default.
*
* Fields are appended with the same sort direction as the last order
* specified, or 'ASCENDING' if no order was specified. For example:
*
* * `ORDER BY a` becomes `ORDER BY a ASC, __name__ ASC`
* * `ORDER BY a DESC` becomes `ORDER BY a DESC, __name__ DESC`
* * `WHERE a > 1` becomes `WHERE a > 1 ORDER BY a ASC, __name__ ASC`
* * `WHERE __name__ > ... AND a > 1` becomes
* `WHERE __name__ > ... AND a > 1 ORDER BY a ASC, __name__ ASC`
*
*
* repeated .google.firestore.v1.StructuredQuery.Order order_by = 4;
*/
public java.util.List
getOrderByBuilderList() {
return getOrderByFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Order,
com.google.firestore.v1.StructuredQuery.Order.Builder,
com.google.firestore.v1.StructuredQuery.OrderOrBuilder>
getOrderByFieldBuilder() {
if (orderByBuilder_ == null) {
orderByBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.Order,
com.google.firestore.v1.StructuredQuery.Order.Builder,
com.google.firestore.v1.StructuredQuery.OrderOrBuilder>(
orderBy_, ((bitField0_ & 0x00000008) != 0), getParentForChildren(), isClean());
orderBy_ = null;
}
return orderByBuilder_;
}
private com.google.firestore.v1.Cursor startAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Cursor,
com.google.firestore.v1.Cursor.Builder,
com.google.firestore.v1.CursorOrBuilder>
startAtBuilder_;
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*
* @return Whether the startAt field is set.
*/
public boolean hasStartAt() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*
* @return The startAt.
*/
public com.google.firestore.v1.Cursor getStartAt() {
if (startAtBuilder_ == null) {
return startAt_ == null ? com.google.firestore.v1.Cursor.getDefaultInstance() : startAt_;
} else {
return startAtBuilder_.getMessage();
}
}
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*/
public Builder setStartAt(com.google.firestore.v1.Cursor value) {
if (startAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
startAt_ = value;
} else {
startAtBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*/
public Builder setStartAt(com.google.firestore.v1.Cursor.Builder builderForValue) {
if (startAtBuilder_ == null) {
startAt_ = builderForValue.build();
} else {
startAtBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*/
public Builder mergeStartAt(com.google.firestore.v1.Cursor value) {
if (startAtBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)
&& startAt_ != null
&& startAt_ != com.google.firestore.v1.Cursor.getDefaultInstance()) {
getStartAtBuilder().mergeFrom(value);
} else {
startAt_ = value;
}
} else {
startAtBuilder_.mergeFrom(value);
}
if (startAt_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*/
public Builder clearStartAt() {
bitField0_ = (bitField0_ & ~0x00000010);
startAt_ = null;
if (startAtBuilder_ != null) {
startAtBuilder_.dispose();
startAtBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*/
public com.google.firestore.v1.Cursor.Builder getStartAtBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getStartAtFieldBuilder().getBuilder();
}
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*/
public com.google.firestore.v1.CursorOrBuilder getStartAtOrBuilder() {
if (startAtBuilder_ != null) {
return startAtBuilder_.getMessageOrBuilder();
} else {
return startAt_ == null ? com.google.firestore.v1.Cursor.getDefaultInstance() : startAt_;
}
}
/**
*
*
* * A potential prefix of a position in the result set to start the query at.
*
* The ordering of the result set is based on the `ORDER BY` clause of the
* original query.
*
* ```
* SELECT * FROM k WHERE a = 1 AND b > 2 ORDER BY b ASC, __name__ ASC;
* ```
*
* This query's results are ordered by `(b ASC, __name__ ASC)`.
*
* Cursors can reference either the full ordering or a prefix of the location,
* though it cannot reference more fields than what are in the provided
* `ORDER BY`.
*
* Continuing off the example above, attaching the following start cursors
* will have varying impact:
*
* - `START BEFORE (2, /k/123)`: start the query right before `a = 1 AND
* b > 2 AND __name__ > /k/123`.
* - `START AFTER (10)`: start the query right after `a = 1 AND b > 10`.
*
* Unlike `OFFSET` which requires scanning over the first N results to skip,
* a start cursor allows the query to begin at a logical position. This
* position is not required to match an actual result, it will scan forward
* from this position to find the next document.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor start_at = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Cursor,
com.google.firestore.v1.Cursor.Builder,
com.google.firestore.v1.CursorOrBuilder>
getStartAtFieldBuilder() {
if (startAtBuilder_ == null) {
startAtBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Cursor,
com.google.firestore.v1.Cursor.Builder,
com.google.firestore.v1.CursorOrBuilder>(
getStartAt(), getParentForChildren(), isClean());
startAt_ = null;
}
return startAtBuilder_;
}
private com.google.firestore.v1.Cursor endAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Cursor,
com.google.firestore.v1.Cursor.Builder,
com.google.firestore.v1.CursorOrBuilder>
endAtBuilder_;
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*
* @return Whether the endAt field is set.
*/
public boolean hasEndAt() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*
* @return The endAt.
*/
public com.google.firestore.v1.Cursor getEndAt() {
if (endAtBuilder_ == null) {
return endAt_ == null ? com.google.firestore.v1.Cursor.getDefaultInstance() : endAt_;
} else {
return endAtBuilder_.getMessage();
}
}
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*/
public Builder setEndAt(com.google.firestore.v1.Cursor value) {
if (endAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
endAt_ = value;
} else {
endAtBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*/
public Builder setEndAt(com.google.firestore.v1.Cursor.Builder builderForValue) {
if (endAtBuilder_ == null) {
endAt_ = builderForValue.build();
} else {
endAtBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*/
public Builder mergeEndAt(com.google.firestore.v1.Cursor value) {
if (endAtBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0)
&& endAt_ != null
&& endAt_ != com.google.firestore.v1.Cursor.getDefaultInstance()) {
getEndAtBuilder().mergeFrom(value);
} else {
endAt_ = value;
}
} else {
endAtBuilder_.mergeFrom(value);
}
if (endAt_ != null) {
bitField0_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*/
public Builder clearEndAt() {
bitField0_ = (bitField0_ & ~0x00000020);
endAt_ = null;
if (endAtBuilder_ != null) {
endAtBuilder_.dispose();
endAtBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*/
public com.google.firestore.v1.Cursor.Builder getEndAtBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getEndAtFieldBuilder().getBuilder();
}
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*/
public com.google.firestore.v1.CursorOrBuilder getEndAtOrBuilder() {
if (endAtBuilder_ != null) {
return endAtBuilder_.getMessageOrBuilder();
} else {
return endAt_ == null ? com.google.firestore.v1.Cursor.getDefaultInstance() : endAt_;
}
}
/**
*
*
* * A potential prefix of a position in the result set to end the query at.
*
* This is similar to `START_AT` but with it controlling the end position
* rather than the start position.
*
* Requires:
*
* * The number of values cannot be greater than the number of fields
* specified in the `ORDER BY` clause.
*
*
* .google.firestore.v1.Cursor end_at = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Cursor,
com.google.firestore.v1.Cursor.Builder,
com.google.firestore.v1.CursorOrBuilder>
getEndAtFieldBuilder() {
if (endAtBuilder_ == null) {
endAtBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.Cursor,
com.google.firestore.v1.Cursor.Builder,
com.google.firestore.v1.CursorOrBuilder>(
getEndAt(), getParentForChildren(), isClean());
endAt_ = null;
}
return endAtBuilder_;
}
private int offset_;
/**
*
*
* * The number of documents to skip before returning the first result.
*
* This applies after the constraints specified by the `WHERE`, `START AT`, &
* `END AT` but before the `LIMIT` clause.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* int32 offset = 6;
*
* @return The offset.
*/
@java.lang.Override
public int getOffset() {
return offset_;
}
/**
*
*
* * The number of documents to skip before returning the first result.
*
* This applies after the constraints specified by the `WHERE`, `START AT`, &
* `END AT` but before the `LIMIT` clause.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* int32 offset = 6;
*
* @param value The offset to set.
* @return This builder for chaining.
*/
public Builder setOffset(int value) {
offset_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
* * The number of documents to skip before returning the first result.
*
* This applies after the constraints specified by the `WHERE`, `START AT`, &
* `END AT` but before the `LIMIT` clause.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* int32 offset = 6;
*
* @return This builder for chaining.
*/
public Builder clearOffset() {
bitField0_ = (bitField0_ & ~0x00000040);
offset_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Int32Value limit_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value,
com.google.protobuf.Int32Value.Builder,
com.google.protobuf.Int32ValueOrBuilder>
limitBuilder_;
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*
* @return Whether the limit field is set.
*/
public boolean hasLimit() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*
* @return The limit.
*/
public com.google.protobuf.Int32Value getLimit() {
if (limitBuilder_ == null) {
return limit_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : limit_;
} else {
return limitBuilder_.getMessage();
}
}
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*/
public Builder setLimit(com.google.protobuf.Int32Value value) {
if (limitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
limit_ = value;
} else {
limitBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*/
public Builder setLimit(com.google.protobuf.Int32Value.Builder builderForValue) {
if (limitBuilder_ == null) {
limit_ = builderForValue.build();
} else {
limitBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*/
public Builder mergeLimit(com.google.protobuf.Int32Value value) {
if (limitBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)
&& limit_ != null
&& limit_ != com.google.protobuf.Int32Value.getDefaultInstance()) {
getLimitBuilder().mergeFrom(value);
} else {
limit_ = value;
}
} else {
limitBuilder_.mergeFrom(value);
}
if (limit_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*/
public Builder clearLimit() {
bitField0_ = (bitField0_ & ~0x00000080);
limit_ = null;
if (limitBuilder_ != null) {
limitBuilder_.dispose();
limitBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*/
public com.google.protobuf.Int32Value.Builder getLimitBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getLimitFieldBuilder().getBuilder();
}
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*/
public com.google.protobuf.Int32ValueOrBuilder getLimitOrBuilder() {
if (limitBuilder_ != null) {
return limitBuilder_.getMessageOrBuilder();
} else {
return limit_ == null ? com.google.protobuf.Int32Value.getDefaultInstance() : limit_;
}
}
/**
*
*
* * The maximum number of results to return.
*
* Applies after all other constraints.
*
* Requires:
*
* * The value must be greater than or equal to zero if specified.
*
*
* .google.protobuf.Int32Value limit = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value,
com.google.protobuf.Int32Value.Builder,
com.google.protobuf.Int32ValueOrBuilder>
getLimitFieldBuilder() {
if (limitBuilder_ == null) {
limitBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Int32Value,
com.google.protobuf.Int32Value.Builder,
com.google.protobuf.Int32ValueOrBuilder>(
getLimit(), getParentForChildren(), isClean());
limit_ = null;
}
return limitBuilder_;
}
private com.google.firestore.v1.StructuredQuery.FindNearest findNearest_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FindNearest,
com.google.firestore.v1.StructuredQuery.FindNearest.Builder,
com.google.firestore.v1.StructuredQuery.FindNearestOrBuilder>
findNearestBuilder_;
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the findNearest field is set.
*/
public boolean hasFindNearest() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The findNearest.
*/
public com.google.firestore.v1.StructuredQuery.FindNearest getFindNearest() {
if (findNearestBuilder_ == null) {
return findNearest_ == null
? com.google.firestore.v1.StructuredQuery.FindNearest.getDefaultInstance()
: findNearest_;
} else {
return findNearestBuilder_.getMessage();
}
}
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setFindNearest(com.google.firestore.v1.StructuredQuery.FindNearest value) {
if (findNearestBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
findNearest_ = value;
} else {
findNearestBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder setFindNearest(
com.google.firestore.v1.StructuredQuery.FindNearest.Builder builderForValue) {
if (findNearestBuilder_ == null) {
findNearest_ = builderForValue.build();
} else {
findNearestBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder mergeFindNearest(com.google.firestore.v1.StructuredQuery.FindNearest value) {
if (findNearestBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)
&& findNearest_ != null
&& findNearest_
!= com.google.firestore.v1.StructuredQuery.FindNearest.getDefaultInstance()) {
getFindNearestBuilder().mergeFrom(value);
} else {
findNearest_ = value;
}
} else {
findNearestBuilder_.mergeFrom(value);
}
if (findNearest_ != null) {
bitField0_ |= 0x00000100;
onChanged();
}
return this;
}
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public Builder clearFindNearest() {
bitField0_ = (bitField0_ & ~0x00000100);
findNearest_ = null;
if (findNearestBuilder_ != null) {
findNearestBuilder_.dispose();
findNearestBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.firestore.v1.StructuredQuery.FindNearest.Builder getFindNearestBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getFindNearestFieldBuilder().getBuilder();
}
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
public com.google.firestore.v1.StructuredQuery.FindNearestOrBuilder getFindNearestOrBuilder() {
if (findNearestBuilder_ != null) {
return findNearestBuilder_.getMessageOrBuilder();
} else {
return findNearest_ == null
? com.google.firestore.v1.StructuredQuery.FindNearest.getDefaultInstance()
: findNearest_;
}
}
/**
*
*
* * Optional. A potential nearest neighbors search.
*
* Applies after all other filters and ordering.
*
* Finds the closest vector embeddings to the given query vector.
*
*
*
* .google.firestore.v1.StructuredQuery.FindNearest find_nearest = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FindNearest,
com.google.firestore.v1.StructuredQuery.FindNearest.Builder,
com.google.firestore.v1.StructuredQuery.FindNearestOrBuilder>
getFindNearestFieldBuilder() {
if (findNearestBuilder_ == null) {
findNearestBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.firestore.v1.StructuredQuery.FindNearest,
com.google.firestore.v1.StructuredQuery.FindNearest.Builder,
com.google.firestore.v1.StructuredQuery.FindNearestOrBuilder>(
getFindNearest(), getParentForChildren(), isClean());
findNearest_ = null;
}
return findNearestBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.firestore.v1.StructuredQuery)
}
// @@protoc_insertion_point(class_scope:google.firestore.v1.StructuredQuery)
private static final com.google.firestore.v1.StructuredQuery DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.firestore.v1.StructuredQuery();
}
public static com.google.firestore.v1.StructuredQuery getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public StructuredQuery parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.firestore.v1.StructuredQuery getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}