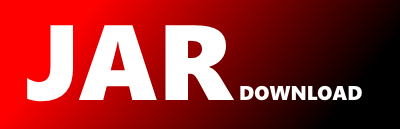
com.google.cloud.gkebackup.v1.Backup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-gke-backup-v1 Show documentation
Show all versions of proto-google-cloud-gke-backup-v1 Show documentation
Proto library for google-cloud-gke-backup
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/gkebackup/v1/backup.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.gkebackup.v1;
/**
*
*
*
* Represents a request to perform a single point-in-time capture of
* some portion of the state of a GKE cluster, the record of the backup
* operation itself, and an anchor for the underlying artifacts that
* comprise the Backup (the config backup and VolumeBackups).
*
*
* Protobuf type {@code google.cloud.gkebackup.v1.Backup}
*/
public final class Backup extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.gkebackup.v1.Backup)
BackupOrBuilder {
private static final long serialVersionUID = 0L;
// Use Backup.newBuilder() to construct.
private Backup(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Backup() {
name_ = "";
uid_ = "";
state_ = 0;
stateReason_ = "";
etag_ = "";
description_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Backup();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 6:
return internalGetLabels();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.gkebackup.v1.Backup.class,
com.google.cloud.gkebackup.v1.Backup.Builder.class);
}
/**
*
*
*
* State
*
*
* Protobuf enum {@code google.cloud.gkebackup.v1.Backup.State}
*/
public enum State implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* The Backup resource is in the process of being created.
*
*
* STATE_UNSPECIFIED = 0;
*/
STATE_UNSPECIFIED(0),
/**
*
*
*
* The Backup resource has been created and the associated BackupJob
* Kubernetes resource has been injected into the source cluster.
*
*
* CREATING = 1;
*/
CREATING(1),
/**
*
*
*
* The gkebackup agent in the cluster has begun executing the backup
* operation.
*
*
* IN_PROGRESS = 2;
*/
IN_PROGRESS(2),
/**
*
*
*
* The backup operation has completed successfully.
*
*
* SUCCEEDED = 3;
*/
SUCCEEDED(3),
/**
*
*
*
* The backup operation has failed.
*
*
* FAILED = 4;
*/
FAILED(4),
/**
*
*
*
* This Backup resource (and its associated artifacts) is in the process
* of being deleted.
*
*
* DELETING = 5;
*/
DELETING(5),
UNRECOGNIZED(-1),
;
/**
*
*
*
* The Backup resource is in the process of being created.
*
*
* STATE_UNSPECIFIED = 0;
*/
public static final int STATE_UNSPECIFIED_VALUE = 0;
/**
*
*
*
* The Backup resource has been created and the associated BackupJob
* Kubernetes resource has been injected into the source cluster.
*
*
* CREATING = 1;
*/
public static final int CREATING_VALUE = 1;
/**
*
*
*
* The gkebackup agent in the cluster has begun executing the backup
* operation.
*
*
* IN_PROGRESS = 2;
*/
public static final int IN_PROGRESS_VALUE = 2;
/**
*
*
*
* The backup operation has completed successfully.
*
*
* SUCCEEDED = 3;
*/
public static final int SUCCEEDED_VALUE = 3;
/**
*
*
*
* The backup operation has failed.
*
*
* FAILED = 4;
*/
public static final int FAILED_VALUE = 4;
/**
*
*
*
* This Backup resource (and its associated artifacts) is in the process
* of being deleted.
*
*
* DELETING = 5;
*/
public static final int DELETING_VALUE = 5;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static State valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static State forNumber(int value) {
switch (value) {
case 0:
return STATE_UNSPECIFIED;
case 1:
return CREATING;
case 2:
return IN_PROGRESS;
case 3:
return SUCCEEDED;
case 4:
return FAILED;
case 5:
return DELETING;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public State findValueByNumber(int number) {
return State.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.gkebackup.v1.Backup.getDescriptor().getEnumTypes().get(0);
}
private static final State[] VALUES = values();
public static State valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private State(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.gkebackup.v1.Backup.State)
}
public interface ClusterMetadataOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.gkebackup.v1.Backup.ClusterMetadata)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Output only. The source cluster from which this Backup was created.
* Valid formats:
*
* - `projects/*/locations/*/clusters/*`
* - `projects/*/zones/*/clusters/*`
*
* This is inherited from the parent BackupPlan's
* [cluster][google.cloud.gkebackup.v1.BackupPlan.cluster] field.
*
*
* string cluster = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The cluster.
*/
java.lang.String getCluster();
/**
*
*
*
* Output only. The source cluster from which this Backup was created.
* Valid formats:
*
* - `projects/*/locations/*/clusters/*`
* - `projects/*/zones/*/clusters/*`
*
* This is inherited from the parent BackupPlan's
* [cluster][google.cloud.gkebackup.v1.BackupPlan.cluster] field.
*
*
* string cluster = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for cluster.
*/
com.google.protobuf.ByteString getClusterBytes();
/**
*
*
*
* Output only. The Kubernetes server version of the source cluster.
*
*
* string k8s_version = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The k8sVersion.
*/
java.lang.String getK8SVersion();
/**
*
*
*
* Output only. The Kubernetes server version of the source cluster.
*
*
* string k8s_version = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for k8sVersion.
*/
com.google.protobuf.ByteString getK8SVersionBytes();
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
int getBackupCrdVersionsCount();
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
boolean containsBackupCrdVersions(java.lang.String key);
/** Use {@link #getBackupCrdVersionsMap()} instead. */
@java.lang.Deprecated
java.util.Map getBackupCrdVersions();
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
java.util.Map getBackupCrdVersionsMap();
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
/* nullable */
java.lang.String getBackupCrdVersionsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
java.lang.String getBackupCrdVersionsOrThrow(java.lang.String key);
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return Whether the gkeVersion field is set.
*/
boolean hasGkeVersion();
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The gkeVersion.
*/
java.lang.String getGkeVersion();
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for gkeVersion.
*/
com.google.protobuf.ByteString getGkeVersionBytes();
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return Whether the anthosVersion field is set.
*/
boolean hasAnthosVersion();
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The anthosVersion.
*/
java.lang.String getAnthosVersion();
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for anthosVersion.
*/
com.google.protobuf.ByteString getAnthosVersionBytes();
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.PlatformVersionCase
getPlatformVersionCase();
}
/**
*
*
*
* Information about the GKE cluster from which this Backup was created.
*
*
* Protobuf type {@code google.cloud.gkebackup.v1.Backup.ClusterMetadata}
*/
public static final class ClusterMetadata extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.gkebackup.v1.Backup.ClusterMetadata)
ClusterMetadataOrBuilder {
private static final long serialVersionUID = 0L;
// Use ClusterMetadata.newBuilder() to construct.
private ClusterMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ClusterMetadata() {
cluster_ = "";
k8SVersion_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new ClusterMetadata();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_ClusterMetadata_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 3:
return internalGetBackupCrdVersions();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_ClusterMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.class,
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.Builder.class);
}
private int platformVersionCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object platformVersion_;
public enum PlatformVersionCase
implements
com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
GKE_VERSION(4),
ANTHOS_VERSION(5),
PLATFORMVERSION_NOT_SET(0);
private final int value;
private PlatformVersionCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PlatformVersionCase valueOf(int value) {
return forNumber(value);
}
public static PlatformVersionCase forNumber(int value) {
switch (value) {
case 4:
return GKE_VERSION;
case 5:
return ANTHOS_VERSION;
case 0:
return PLATFORMVERSION_NOT_SET;
default:
return null;
}
}
public int getNumber() {
return this.value;
}
};
public PlatformVersionCase getPlatformVersionCase() {
return PlatformVersionCase.forNumber(platformVersionCase_);
}
public static final int CLUSTER_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object cluster_ = "";
/**
*
*
*
* Output only. The source cluster from which this Backup was created.
* Valid formats:
*
* - `projects/*/locations/*/clusters/*`
* - `projects/*/zones/*/clusters/*`
*
* This is inherited from the parent BackupPlan's
* [cluster][google.cloud.gkebackup.v1.BackupPlan.cluster] field.
*
*
* string cluster = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The cluster.
*/
@java.lang.Override
public java.lang.String getCluster() {
java.lang.Object ref = cluster_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cluster_ = s;
return s;
}
}
/**
*
*
*
* Output only. The source cluster from which this Backup was created.
* Valid formats:
*
* - `projects/*/locations/*/clusters/*`
* - `projects/*/zones/*/clusters/*`
*
* This is inherited from the parent BackupPlan's
* [cluster][google.cloud.gkebackup.v1.BackupPlan.cluster] field.
*
*
* string cluster = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for cluster.
*/
@java.lang.Override
public com.google.protobuf.ByteString getClusterBytes() {
java.lang.Object ref = cluster_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
cluster_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int K8S_VERSION_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object k8SVersion_ = "";
/**
*
*
*
* Output only. The Kubernetes server version of the source cluster.
*
*
* string k8s_version = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The k8sVersion.
*/
@java.lang.Override
public java.lang.String getK8SVersion() {
java.lang.Object ref = k8SVersion_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
k8SVersion_ = s;
return s;
}
}
/**
*
*
*
* Output only. The Kubernetes server version of the source cluster.
*
*
* string k8s_version = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for k8sVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString getK8SVersionBytes() {
java.lang.Object ref = k8SVersion_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
k8SVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BACKUP_CRD_VERSIONS_FIELD_NUMBER = 3;
private static final class BackupCrdVersionsDefaultEntryHolder {
static final com.google.protobuf.MapEntry defaultEntry =
com.google.protobuf.MapEntry.newDefaultInstance(
com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_ClusterMetadata_BackupCrdVersionsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField backupCrdVersions_;
private com.google.protobuf.MapField
internalGetBackupCrdVersions() {
if (backupCrdVersions_ == null) {
return com.google.protobuf.MapField.emptyMapField(
BackupCrdVersionsDefaultEntryHolder.defaultEntry);
}
return backupCrdVersions_;
}
public int getBackupCrdVersionsCount() {
return internalGetBackupCrdVersions().getMap().size();
}
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public boolean containsBackupCrdVersions(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetBackupCrdVersions().getMap().containsKey(key);
}
/** Use {@link #getBackupCrdVersionsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getBackupCrdVersions() {
return getBackupCrdVersionsMap();
}
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public java.util.Map getBackupCrdVersionsMap() {
return internalGetBackupCrdVersions().getMap();
}
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public /* nullable */ java.lang.String getBackupCrdVersionsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetBackupCrdVersions().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public java.lang.String getBackupCrdVersionsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetBackupCrdVersions().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int GKE_VERSION_FIELD_NUMBER = 4;
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return Whether the gkeVersion field is set.
*/
public boolean hasGkeVersion() {
return platformVersionCase_ == 4;
}
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The gkeVersion.
*/
public java.lang.String getGkeVersion() {
java.lang.Object ref = "";
if (platformVersionCase_ == 4) {
ref = platformVersion_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (platformVersionCase_ == 4) {
platformVersion_ = s;
}
return s;
}
}
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for gkeVersion.
*/
public com.google.protobuf.ByteString getGkeVersionBytes() {
java.lang.Object ref = "";
if (platformVersionCase_ == 4) {
ref = platformVersion_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
if (platformVersionCase_ == 4) {
platformVersion_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ANTHOS_VERSION_FIELD_NUMBER = 5;
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return Whether the anthosVersion field is set.
*/
public boolean hasAnthosVersion() {
return platformVersionCase_ == 5;
}
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The anthosVersion.
*/
public java.lang.String getAnthosVersion() {
java.lang.Object ref = "";
if (platformVersionCase_ == 5) {
ref = platformVersion_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (platformVersionCase_ == 5) {
platformVersion_ = s;
}
return s;
}
}
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for anthosVersion.
*/
public com.google.protobuf.ByteString getAnthosVersionBytes() {
java.lang.Object ref = "";
if (platformVersionCase_ == 5) {
ref = platformVersion_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
if (platformVersionCase_ == 5) {
platformVersion_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(cluster_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, cluster_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(k8SVersion_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, k8SVersion_);
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output,
internalGetBackupCrdVersions(),
BackupCrdVersionsDefaultEntryHolder.defaultEntry,
3);
if (platformVersionCase_ == 4) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, platformVersion_);
}
if (platformVersionCase_ == 5) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, platformVersion_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(cluster_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, cluster_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(k8SVersion_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, k8SVersion_);
}
for (java.util.Map.Entry entry :
internalGetBackupCrdVersions().getMap().entrySet()) {
com.google.protobuf.MapEntry backupCrdVersions__ =
BackupCrdVersionsDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(3, backupCrdVersions__);
}
if (platformVersionCase_ == 4) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, platformVersion_);
}
if (platformVersionCase_ == 5) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, platformVersion_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.gkebackup.v1.Backup.ClusterMetadata)) {
return super.equals(obj);
}
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata other =
(com.google.cloud.gkebackup.v1.Backup.ClusterMetadata) obj;
if (!getCluster().equals(other.getCluster())) return false;
if (!getK8SVersion().equals(other.getK8SVersion())) return false;
if (!internalGetBackupCrdVersions().equals(other.internalGetBackupCrdVersions()))
return false;
if (!getPlatformVersionCase().equals(other.getPlatformVersionCase())) return false;
switch (platformVersionCase_) {
case 4:
if (!getGkeVersion().equals(other.getGkeVersion())) return false;
break;
case 5:
if (!getAnthosVersion().equals(other.getAnthosVersion())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CLUSTER_FIELD_NUMBER;
hash = (53 * hash) + getCluster().hashCode();
hash = (37 * hash) + K8S_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getK8SVersion().hashCode();
if (!internalGetBackupCrdVersions().getMap().isEmpty()) {
hash = (37 * hash) + BACKUP_CRD_VERSIONS_FIELD_NUMBER;
hash = (53 * hash) + internalGetBackupCrdVersions().hashCode();
}
switch (platformVersionCase_) {
case 4:
hash = (37 * hash) + GKE_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getGkeVersion().hashCode();
break;
case 5:
hash = (37 * hash) + ANTHOS_VERSION_FIELD_NUMBER;
hash = (53 * hash) + getAnthosVersion().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Information about the GKE cluster from which this Backup was created.
*
*
* Protobuf type {@code google.cloud.gkebackup.v1.Backup.ClusterMetadata}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.gkebackup.v1.Backup.ClusterMetadata)
com.google.cloud.gkebackup.v1.Backup.ClusterMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_ClusterMetadata_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 3:
return internalGetBackupCrdVersions();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 3:
return internalGetMutableBackupCrdVersions();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_ClusterMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.class,
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.Builder.class);
}
// Construct using com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
cluster_ = "";
k8SVersion_ = "";
internalGetMutableBackupCrdVersions().clear();
platformVersionCase_ = 0;
platformVersion_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_ClusterMetadata_descriptor;
}
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup.ClusterMetadata getDefaultInstanceForType() {
return com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup.ClusterMetadata build() {
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup.ClusterMetadata buildPartial() {
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata result =
new com.google.cloud.gkebackup.v1.Backup.ClusterMetadata(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.gkebackup.v1.Backup.ClusterMetadata result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.cluster_ = cluster_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.k8SVersion_ = k8SVersion_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.backupCrdVersions_ = internalGetBackupCrdVersions();
result.backupCrdVersions_.makeImmutable();
}
}
private void buildPartialOneofs(com.google.cloud.gkebackup.v1.Backup.ClusterMetadata result) {
result.platformVersionCase_ = platformVersionCase_;
result.platformVersion_ = this.platformVersion_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.gkebackup.v1.Backup.ClusterMetadata) {
return mergeFrom((com.google.cloud.gkebackup.v1.Backup.ClusterMetadata) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.gkebackup.v1.Backup.ClusterMetadata other) {
if (other == com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.getDefaultInstance())
return this;
if (!other.getCluster().isEmpty()) {
cluster_ = other.cluster_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getK8SVersion().isEmpty()) {
k8SVersion_ = other.k8SVersion_;
bitField0_ |= 0x00000002;
onChanged();
}
internalGetMutableBackupCrdVersions().mergeFrom(other.internalGetBackupCrdVersions());
bitField0_ |= 0x00000004;
switch (other.getPlatformVersionCase()) {
case GKE_VERSION:
{
platformVersionCase_ = 4;
platformVersion_ = other.platformVersion_;
onChanged();
break;
}
case ANTHOS_VERSION:
{
platformVersionCase_ = 5;
platformVersion_ = other.platformVersion_;
onChanged();
break;
}
case PLATFORMVERSION_NOT_SET:
{
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
cluster_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
k8SVersion_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26:
{
com.google.protobuf.MapEntry
backupCrdVersions__ =
input.readMessage(
BackupCrdVersionsDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableBackupCrdVersions()
.getMutableMap()
.put(backupCrdVersions__.getKey(), backupCrdVersions__.getValue());
bitField0_ |= 0x00000004;
break;
} // case 26
case 34:
{
java.lang.String s = input.readStringRequireUtf8();
platformVersionCase_ = 4;
platformVersion_ = s;
break;
} // case 34
case 42:
{
java.lang.String s = input.readStringRequireUtf8();
platformVersionCase_ = 5;
platformVersion_ = s;
break;
} // case 42
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int platformVersionCase_ = 0;
private java.lang.Object platformVersion_;
public PlatformVersionCase getPlatformVersionCase() {
return PlatformVersionCase.forNumber(platformVersionCase_);
}
public Builder clearPlatformVersion() {
platformVersionCase_ = 0;
platformVersion_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object cluster_ = "";
/**
*
*
*
* Output only. The source cluster from which this Backup was created.
* Valid formats:
*
* - `projects/*/locations/*/clusters/*`
* - `projects/*/zones/*/clusters/*`
*
* This is inherited from the parent BackupPlan's
* [cluster][google.cloud.gkebackup.v1.BackupPlan.cluster] field.
*
*
* string cluster = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The cluster.
*/
public java.lang.String getCluster() {
java.lang.Object ref = cluster_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cluster_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. The source cluster from which this Backup was created.
* Valid formats:
*
* - `projects/*/locations/*/clusters/*`
* - `projects/*/zones/*/clusters/*`
*
* This is inherited from the parent BackupPlan's
* [cluster][google.cloud.gkebackup.v1.BackupPlan.cluster] field.
*
*
* string cluster = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for cluster.
*/
public com.google.protobuf.ByteString getClusterBytes() {
java.lang.Object ref = cluster_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
cluster_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. The source cluster from which this Backup was created.
* Valid formats:
*
* - `projects/*/locations/*/clusters/*`
* - `projects/*/zones/*/clusters/*`
*
* This is inherited from the parent BackupPlan's
* [cluster][google.cloud.gkebackup.v1.BackupPlan.cluster] field.
*
*
* string cluster = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The cluster to set.
* @return This builder for chaining.
*/
public Builder setCluster(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
cluster_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Output only. The source cluster from which this Backup was created.
* Valid formats:
*
* - `projects/*/locations/*/clusters/*`
* - `projects/*/zones/*/clusters/*`
*
* This is inherited from the parent BackupPlan's
* [cluster][google.cloud.gkebackup.v1.BackupPlan.cluster] field.
*
*
* string cluster = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearCluster() {
cluster_ = getDefaultInstance().getCluster();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* Output only. The source cluster from which this Backup was created.
* Valid formats:
*
* - `projects/*/locations/*/clusters/*`
* - `projects/*/zones/*/clusters/*`
*
* This is inherited from the parent BackupPlan's
* [cluster][google.cloud.gkebackup.v1.BackupPlan.cluster] field.
*
*
* string cluster = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The bytes for cluster to set.
* @return This builder for chaining.
*/
public Builder setClusterBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
cluster_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object k8SVersion_ = "";
/**
*
*
*
* Output only. The Kubernetes server version of the source cluster.
*
*
* string k8s_version = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The k8sVersion.
*/
public java.lang.String getK8SVersion() {
java.lang.Object ref = k8SVersion_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
k8SVersion_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. The Kubernetes server version of the source cluster.
*
*
* string k8s_version = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for k8sVersion.
*/
public com.google.protobuf.ByteString getK8SVersionBytes() {
java.lang.Object ref = k8SVersion_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
k8SVersion_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. The Kubernetes server version of the source cluster.
*
*
* string k8s_version = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The k8sVersion to set.
* @return This builder for chaining.
*/
public Builder setK8SVersion(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
k8SVersion_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Output only. The Kubernetes server version of the source cluster.
*
*
* string k8s_version = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearK8SVersion() {
k8SVersion_ = getDefaultInstance().getK8SVersion();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* Output only. The Kubernetes server version of the source cluster.
*
*
* string k8s_version = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The bytes for k8sVersion to set.
* @return This builder for chaining.
*/
public Builder setK8SVersionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
k8SVersion_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.MapField backupCrdVersions_;
private com.google.protobuf.MapField
internalGetBackupCrdVersions() {
if (backupCrdVersions_ == null) {
return com.google.protobuf.MapField.emptyMapField(
BackupCrdVersionsDefaultEntryHolder.defaultEntry);
}
return backupCrdVersions_;
}
private com.google.protobuf.MapField
internalGetMutableBackupCrdVersions() {
if (backupCrdVersions_ == null) {
backupCrdVersions_ =
com.google.protobuf.MapField.newMapField(
BackupCrdVersionsDefaultEntryHolder.defaultEntry);
}
if (!backupCrdVersions_.isMutable()) {
backupCrdVersions_ = backupCrdVersions_.copy();
}
bitField0_ |= 0x00000004;
onChanged();
return backupCrdVersions_;
}
public int getBackupCrdVersionsCount() {
return internalGetBackupCrdVersions().getMap().size();
}
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public boolean containsBackupCrdVersions(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetBackupCrdVersions().getMap().containsKey(key);
}
/** Use {@link #getBackupCrdVersionsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getBackupCrdVersions() {
return getBackupCrdVersionsMap();
}
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public java.util.Map getBackupCrdVersionsMap() {
return internalGetBackupCrdVersions().getMap();
}
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public /* nullable */ java.lang.String getBackupCrdVersionsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetBackupCrdVersions().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public java.lang.String getBackupCrdVersionsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetBackupCrdVersions().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearBackupCrdVersions() {
bitField0_ = (bitField0_ & ~0x00000004);
internalGetMutableBackupCrdVersions().getMutableMap().clear();
return this;
}
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder removeBackupCrdVersions(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableBackupCrdVersions().getMutableMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map getMutableBackupCrdVersions() {
bitField0_ |= 0x00000004;
return internalGetMutableBackupCrdVersions().getMutableMap();
}
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder putBackupCrdVersions(java.lang.String key, java.lang.String value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableBackupCrdVersions().getMutableMap().put(key, value);
bitField0_ |= 0x00000004;
return this;
}
/**
*
*
*
* Output only. A list of the Backup for GKE CRD versions found in the
* cluster.
*
*
*
* map<string, string> backup_crd_versions = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder putAllBackupCrdVersions(
java.util.Map values) {
internalGetMutableBackupCrdVersions().getMutableMap().putAll(values);
bitField0_ |= 0x00000004;
return this;
}
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return Whether the gkeVersion field is set.
*/
@java.lang.Override
public boolean hasGkeVersion() {
return platformVersionCase_ == 4;
}
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The gkeVersion.
*/
@java.lang.Override
public java.lang.String getGkeVersion() {
java.lang.Object ref = "";
if (platformVersionCase_ == 4) {
ref = platformVersion_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (platformVersionCase_ == 4) {
platformVersion_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for gkeVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString getGkeVersionBytes() {
java.lang.Object ref = "";
if (platformVersionCase_ == 4) {
ref = platformVersion_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
if (platformVersionCase_ == 4) {
platformVersion_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The gkeVersion to set.
* @return This builder for chaining.
*/
public Builder setGkeVersion(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
platformVersionCase_ = 4;
platformVersion_ = value;
onChanged();
return this;
}
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearGkeVersion() {
if (platformVersionCase_ == 4) {
platformVersionCase_ = 0;
platformVersion_ = null;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. GKE version
*
*
* string gke_version = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The bytes for gkeVersion to set.
* @return This builder for chaining.
*/
public Builder setGkeVersionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
platformVersionCase_ = 4;
platformVersion_ = value;
onChanged();
return this;
}
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return Whether the anthosVersion field is set.
*/
@java.lang.Override
public boolean hasAnthosVersion() {
return platformVersionCase_ == 5;
}
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The anthosVersion.
*/
@java.lang.Override
public java.lang.String getAnthosVersion() {
java.lang.Object ref = "";
if (platformVersionCase_ == 5) {
ref = platformVersion_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (platformVersionCase_ == 5) {
platformVersion_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for anthosVersion.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAnthosVersionBytes() {
java.lang.Object ref = "";
if (platformVersionCase_ == 5) {
ref = platformVersion_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
if (platformVersionCase_ == 5) {
platformVersion_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The anthosVersion to set.
* @return This builder for chaining.
*/
public Builder setAnthosVersion(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
platformVersionCase_ = 5;
platformVersion_ = value;
onChanged();
return this;
}
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearAnthosVersion() {
if (platformVersionCase_ == 5) {
platformVersionCase_ = 0;
platformVersion_ = null;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. Anthos version
*
*
* string anthos_version = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The bytes for anthosVersion to set.
* @return This builder for chaining.
*/
public Builder setAnthosVersionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
platformVersionCase_ = 5;
platformVersion_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.gkebackup.v1.Backup.ClusterMetadata)
}
// @@protoc_insertion_point(class_scope:google.cloud.gkebackup.v1.Backup.ClusterMetadata)
private static final com.google.cloud.gkebackup.v1.Backup.ClusterMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.gkebackup.v1.Backup.ClusterMetadata();
}
public static com.google.cloud.gkebackup.v1.Backup.ClusterMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ClusterMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup.ClusterMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
private int backupScopeCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object backupScope_;
public enum BackupScopeCase
implements
com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
ALL_NAMESPACES(12),
SELECTED_NAMESPACES(13),
SELECTED_APPLICATIONS(14),
BACKUPSCOPE_NOT_SET(0);
private final int value;
private BackupScopeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static BackupScopeCase valueOf(int value) {
return forNumber(value);
}
public static BackupScopeCase forNumber(int value) {
switch (value) {
case 12:
return ALL_NAMESPACES;
case 13:
return SELECTED_NAMESPACES;
case 14:
return SELECTED_APPLICATIONS;
case 0:
return BACKUPSCOPE_NOT_SET;
default:
return null;
}
}
public int getNumber() {
return this.value;
}
};
public BackupScopeCase getBackupScopeCase() {
return BackupScopeCase.forNumber(backupScopeCase_);
}
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* Output only. The fully qualified name of the Backup.
* `projects/*/locations/*/backupPlans/*/backups/*`
*
*
* string name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* Output only. The fully qualified name of the Backup.
* `projects/*/locations/*/backupPlans/*/backups/*`
*
*
* string name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int UID_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object uid_ = "";
/**
*
*
*
* Output only. Server generated global unique identifier of
* [UUID4](https://en.wikipedia.org/wiki/Universally_unique_identifier)
*
*
* string uid = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The uid.
*/
@java.lang.Override
public java.lang.String getUid() {
java.lang.Object ref = uid_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uid_ = s;
return s;
}
}
/**
*
*
*
* Output only. Server generated global unique identifier of
* [UUID4](https://en.wikipedia.org/wiki/Universally_unique_identifier)
*
*
* string uid = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for uid.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUidBytes() {
java.lang.Object ref = uid_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
uid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CREATE_TIME_FIELD_NUMBER = 3;
private com.google.protobuf.Timestamp createTime_;
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the createTime field is set.
*/
@java.lang.Override
public boolean hasCreateTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The createTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getCreateTime() {
return createTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : createTime_;
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder() {
return createTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : createTime_;
}
public static final int UPDATE_TIME_FIELD_NUMBER = 4;
private com.google.protobuf.Timestamp updateTime_;
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the updateTime field is set.
*/
@java.lang.Override
public boolean hasUpdateTime() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The updateTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getUpdateTime() {
return updateTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : updateTime_;
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getUpdateTimeOrBuilder() {
return updateTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : updateTime_;
}
public static final int MANUAL_FIELD_NUMBER = 5;
private boolean manual_ = false;
/**
*
*
*
* Output only. This flag indicates whether this Backup resource was created
* manually by a user or via a schedule in the BackupPlan. A value of True
* means that the Backup was created manually.
*
*
* bool manual = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The manual.
*/
@java.lang.Override
public boolean getManual() {
return manual_;
}
public static final int LABELS_FIELD_NUMBER = 6;
private static final class LabelsDefaultEntryHolder {
static final com.google.protobuf.MapEntry defaultEntry =
com.google.protobuf.MapEntry.newDefaultInstance(
com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_LabelsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField labels_;
private com.google.protobuf.MapField internalGetLabels() {
if (labels_ == null) {
return com.google.protobuf.MapField.emptyMapField(LabelsDefaultEntryHolder.defaultEntry);
}
return labels_;
}
public int getLabelsCount() {
return internalGetLabels().getMap().size();
}
/**
*
*
*
* Optional. A set of custom labels supplied by user.
*
*
* map<string, string> labels = 6 [(.google.api.field_behavior) = OPTIONAL];
*/
@java.lang.Override
public boolean containsLabels(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetLabels().getMap().containsKey(key);
}
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getLabels() {
return getLabelsMap();
}
/**
*
*
*
* Optional. A set of custom labels supplied by user.
*
*
* map<string, string> labels = 6 [(.google.api.field_behavior) = OPTIONAL];
*/
@java.lang.Override
public java.util.Map getLabelsMap() {
return internalGetLabels().getMap();
}
/**
*
*
*
* Optional. A set of custom labels supplied by user.
*
*
* map<string, string> labels = 6 [(.google.api.field_behavior) = OPTIONAL];
*/
@java.lang.Override
public /* nullable */ java.lang.String getLabelsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Optional. A set of custom labels supplied by user.
*
*
* map<string, string> labels = 6 [(.google.api.field_behavior) = OPTIONAL];
*/
@java.lang.Override
public java.lang.String getLabelsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int DELETE_LOCK_DAYS_FIELD_NUMBER = 7;
private int deleteLockDays_ = 0;
/**
*
*
*
* Optional. Minimum age for this Backup (in days). If this field is set to a
* non-zero value, the Backup will be "locked" against deletion (either manual
* or automatic deletion) for the number of days provided (measured from the
* creation time of the Backup). MUST be an integer value between 0-90
* (inclusive).
*
* Defaults to parent BackupPlan's
* [backup_delete_lock_days][google.cloud.gkebackup.v1.BackupPlan.RetentionPolicy.backup_delete_lock_days]
* setting and may only be increased
* (either at creation time or in a subsequent update).
*
*
* int32 delete_lock_days = 7 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The deleteLockDays.
*/
@java.lang.Override
public int getDeleteLockDays() {
return deleteLockDays_;
}
public static final int DELETE_LOCK_EXPIRE_TIME_FIELD_NUMBER = 8;
private com.google.protobuf.Timestamp deleteLockExpireTime_;
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the deleteLockExpireTime field is set.
*/
@java.lang.Override
public boolean hasDeleteLockExpireTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The deleteLockExpireTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getDeleteLockExpireTime() {
return deleteLockExpireTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: deleteLockExpireTime_;
}
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getDeleteLockExpireTimeOrBuilder() {
return deleteLockExpireTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: deleteLockExpireTime_;
}
public static final int RETAIN_DAYS_FIELD_NUMBER = 9;
private int retainDays_ = 0;
/**
*
*
*
* Optional. The age (in days) after which this Backup will be automatically
* deleted. Must be an integer value >= 0:
*
* - If 0, no automatic deletion will occur for this Backup.
* - If not 0, this must be >=
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days] and
* <= 365.
*
* Once a Backup is created, this value may only be increased.
*
* Defaults to the parent BackupPlan's
* [backup_retain_days][google.cloud.gkebackup.v1.BackupPlan.RetentionPolicy.backup_retain_days]
* value.
*
*
* int32 retain_days = 9 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The retainDays.
*/
@java.lang.Override
public int getRetainDays() {
return retainDays_;
}
public static final int RETAIN_EXPIRE_TIME_FIELD_NUMBER = 10;
private com.google.protobuf.Timestamp retainExpireTime_;
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the retainExpireTime field is set.
*/
@java.lang.Override
public boolean hasRetainExpireTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The retainExpireTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getRetainExpireTime() {
return retainExpireTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: retainExpireTime_;
}
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getRetainExpireTimeOrBuilder() {
return retainExpireTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: retainExpireTime_;
}
public static final int ENCRYPTION_KEY_FIELD_NUMBER = 11;
private com.google.cloud.gkebackup.v1.EncryptionKey encryptionKey_;
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the encryptionKey field is set.
*/
@java.lang.Override
public boolean hasEncryptionKey() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The encryptionKey.
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.EncryptionKey getEncryptionKey() {
return encryptionKey_ == null
? com.google.cloud.gkebackup.v1.EncryptionKey.getDefaultInstance()
: encryptionKey_;
}
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.EncryptionKeyOrBuilder getEncryptionKeyOrBuilder() {
return encryptionKey_ == null
? com.google.cloud.gkebackup.v1.EncryptionKey.getDefaultInstance()
: encryptionKey_;
}
public static final int ALL_NAMESPACES_FIELD_NUMBER = 12;
/**
*
*
*
* Output only. If True, all namespaces were included in the Backup.
*
*
* bool all_namespaces = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return Whether the allNamespaces field is set.
*/
@java.lang.Override
public boolean hasAllNamespaces() {
return backupScopeCase_ == 12;
}
/**
*
*
*
* Output only. If True, all namespaces were included in the Backup.
*
*
* bool all_namespaces = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The allNamespaces.
*/
@java.lang.Override
public boolean getAllNamespaces() {
if (backupScopeCase_ == 12) {
return (java.lang.Boolean) backupScope_;
}
return false;
}
public static final int SELECTED_NAMESPACES_FIELD_NUMBER = 13;
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the selectedNamespaces field is set.
*/
@java.lang.Override
public boolean hasSelectedNamespaces() {
return backupScopeCase_ == 13;
}
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The selectedNamespaces.
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.Namespaces getSelectedNamespaces() {
if (backupScopeCase_ == 13) {
return (com.google.cloud.gkebackup.v1.Namespaces) backupScope_;
}
return com.google.cloud.gkebackup.v1.Namespaces.getDefaultInstance();
}
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.NamespacesOrBuilder getSelectedNamespacesOrBuilder() {
if (backupScopeCase_ == 13) {
return (com.google.cloud.gkebackup.v1.Namespaces) backupScope_;
}
return com.google.cloud.gkebackup.v1.Namespaces.getDefaultInstance();
}
public static final int SELECTED_APPLICATIONS_FIELD_NUMBER = 14;
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the selectedApplications field is set.
*/
@java.lang.Override
public boolean hasSelectedApplications() {
return backupScopeCase_ == 14;
}
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The selectedApplications.
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.NamespacedNames getSelectedApplications() {
if (backupScopeCase_ == 14) {
return (com.google.cloud.gkebackup.v1.NamespacedNames) backupScope_;
}
return com.google.cloud.gkebackup.v1.NamespacedNames.getDefaultInstance();
}
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.NamespacedNamesOrBuilder getSelectedApplicationsOrBuilder() {
if (backupScopeCase_ == 14) {
return (com.google.cloud.gkebackup.v1.NamespacedNames) backupScope_;
}
return com.google.cloud.gkebackup.v1.NamespacedNames.getDefaultInstance();
}
public static final int CONTAINS_VOLUME_DATA_FIELD_NUMBER = 15;
private boolean containsVolumeData_ = false;
/**
*
*
*
* Output only. Whether or not the Backup contains volume data. Controlled by
* the parent BackupPlan's
* [include_volume_data][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.include_volume_data]
* value.
*
*
* bool contains_volume_data = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The containsVolumeData.
*/
@java.lang.Override
public boolean getContainsVolumeData() {
return containsVolumeData_;
}
public static final int CONTAINS_SECRETS_FIELD_NUMBER = 16;
private boolean containsSecrets_ = false;
/**
*
*
*
* Output only. Whether or not the Backup contains Kubernetes Secrets.
* Controlled by the parent BackupPlan's
* [include_secrets][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.include_secrets]
* value.
*
*
* bool contains_secrets = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The containsSecrets.
*/
@java.lang.Override
public boolean getContainsSecrets() {
return containsSecrets_;
}
public static final int CLUSTER_METADATA_FIELD_NUMBER = 17;
private com.google.cloud.gkebackup.v1.Backup.ClusterMetadata clusterMetadata_;
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the clusterMetadata field is set.
*/
@java.lang.Override
public boolean hasClusterMetadata() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The clusterMetadata.
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup.ClusterMetadata getClusterMetadata() {
return clusterMetadata_ == null
? com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.getDefaultInstance()
: clusterMetadata_;
}
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup.ClusterMetadataOrBuilder
getClusterMetadataOrBuilder() {
return clusterMetadata_ == null
? com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.getDefaultInstance()
: clusterMetadata_;
}
public static final int STATE_FIELD_NUMBER = 18;
private int state_ = 0;
/**
*
*
*
* Output only. Current state of the Backup
*
*
*
* .google.cloud.gkebackup.v1.Backup.State state = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The enum numeric value on the wire for state.
*/
@java.lang.Override
public int getStateValue() {
return state_;
}
/**
*
*
*
* Output only. Current state of the Backup
*
*
*
* .google.cloud.gkebackup.v1.Backup.State state = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The state.
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup.State getState() {
com.google.cloud.gkebackup.v1.Backup.State result =
com.google.cloud.gkebackup.v1.Backup.State.forNumber(state_);
return result == null ? com.google.cloud.gkebackup.v1.Backup.State.UNRECOGNIZED : result;
}
public static final int STATE_REASON_FIELD_NUMBER = 19;
@SuppressWarnings("serial")
private volatile java.lang.Object stateReason_ = "";
/**
*
*
*
* Output only. Human-readable description of why the backup is in the current
* `state`.
*
*
* string state_reason = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The stateReason.
*/
@java.lang.Override
public java.lang.String getStateReason() {
java.lang.Object ref = stateReason_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stateReason_ = s;
return s;
}
}
/**
*
*
*
* Output only. Human-readable description of why the backup is in the current
* `state`.
*
*
* string state_reason = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for stateReason.
*/
@java.lang.Override
public com.google.protobuf.ByteString getStateReasonBytes() {
java.lang.Object ref = stateReason_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
stateReason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMPLETE_TIME_FIELD_NUMBER = 20;
private com.google.protobuf.Timestamp completeTime_;
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the completeTime field is set.
*/
@java.lang.Override
public boolean hasCompleteTime() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The completeTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getCompleteTime() {
return completeTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: completeTime_;
}
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getCompleteTimeOrBuilder() {
return completeTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: completeTime_;
}
public static final int RESOURCE_COUNT_FIELD_NUMBER = 21;
private int resourceCount_ = 0;
/**
*
*
*
* Output only. The total number of Kubernetes resources included in the
* Backup.
*
*
* int32 resource_count = 21 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The resourceCount.
*/
@java.lang.Override
public int getResourceCount() {
return resourceCount_;
}
public static final int VOLUME_COUNT_FIELD_NUMBER = 22;
private int volumeCount_ = 0;
/**
*
*
*
* Output only. The total number of volume backups contained in the Backup.
*
*
* int32 volume_count = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The volumeCount.
*/
@java.lang.Override
public int getVolumeCount() {
return volumeCount_;
}
public static final int SIZE_BYTES_FIELD_NUMBER = 23;
private long sizeBytes_ = 0L;
/**
*
*
*
* Output only. The total size of the Backup in bytes = config backup size +
* sum(volume backup sizes)
*
*
* int64 size_bytes = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The sizeBytes.
*/
@java.lang.Override
public long getSizeBytes() {
return sizeBytes_;
}
public static final int ETAG_FIELD_NUMBER = 24;
@SuppressWarnings("serial")
private volatile java.lang.Object etag_ = "";
/**
*
*
*
* Output only. `etag` is used for optimistic concurrency control as a way to
* help prevent simultaneous updates of a backup from overwriting each other.
* It is strongly suggested that systems make use of the `etag` in the
* read-modify-write cycle to perform backup updates in order to avoid
* race conditions: An `etag` is returned in the response to `GetBackup`,
* and systems are expected to put that etag in the request to
* `UpdateBackup` or `DeleteBackup` to ensure that their change will be
* applied to the same version of the resource.
*
*
* string etag = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The etag.
*/
@java.lang.Override
public java.lang.String getEtag() {
java.lang.Object ref = etag_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
etag_ = s;
return s;
}
}
/**
*
*
*
* Output only. `etag` is used for optimistic concurrency control as a way to
* help prevent simultaneous updates of a backup from overwriting each other.
* It is strongly suggested that systems make use of the `etag` in the
* read-modify-write cycle to perform backup updates in order to avoid
* race conditions: An `etag` is returned in the response to `GetBackup`,
* and systems are expected to put that etag in the request to
* `UpdateBackup` or `DeleteBackup` to ensure that their change will be
* applied to the same version of the resource.
*
*
* string etag = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for etag.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEtagBytes() {
java.lang.Object ref = etag_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
etag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 25;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
*
*
*
* Optional. User specified descriptive string for this Backup.
*
*
* string description = 25 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
*
*
* Optional. User specified descriptive string for this Backup.
*
*
* string description = 25 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int POD_COUNT_FIELD_NUMBER = 26;
private int podCount_ = 0;
/**
*
*
*
* Output only. The total number of Kubernetes Pods contained in the Backup.
*
*
* int32 pod_count = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The podCount.
*/
@java.lang.Override
public int getPodCount() {
return podCount_;
}
public static final int CONFIG_BACKUP_SIZE_BYTES_FIELD_NUMBER = 27;
private long configBackupSizeBytes_ = 0L;
/**
*
*
*
* Output only. The size of the config backup in bytes.
*
*
* int64 config_backup_size_bytes = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The configBackupSizeBytes.
*/
@java.lang.Override
public long getConfigBackupSizeBytes() {
return configBackupSizeBytes_;
}
public static final int PERMISSIVE_MODE_FIELD_NUMBER = 28;
private boolean permissiveMode_ = false;
/**
*
*
*
* Output only. If false, Backup will fail when Backup for GKE detects
* Kubernetes configuration that is non-standard or
* requires additional setup to restore.
*
* Inherited from the parent BackupPlan's
* [permissive_mode][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.permissive_mode]
* value.
*
*
* bool permissive_mode = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The permissiveMode.
*/
@java.lang.Override
public boolean getPermissiveMode() {
return permissiveMode_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(uid_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, uid_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(3, getCreateTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(4, getUpdateTime());
}
if (manual_ != false) {
output.writeBool(5, manual_);
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetLabels(), LabelsDefaultEntryHolder.defaultEntry, 6);
if (deleteLockDays_ != 0) {
output.writeInt32(7, deleteLockDays_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(8, getDeleteLockExpireTime());
}
if (retainDays_ != 0) {
output.writeInt32(9, retainDays_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(10, getRetainExpireTime());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(11, getEncryptionKey());
}
if (backupScopeCase_ == 12) {
output.writeBool(12, (boolean) ((java.lang.Boolean) backupScope_));
}
if (backupScopeCase_ == 13) {
output.writeMessage(13, (com.google.cloud.gkebackup.v1.Namespaces) backupScope_);
}
if (backupScopeCase_ == 14) {
output.writeMessage(14, (com.google.cloud.gkebackup.v1.NamespacedNames) backupScope_);
}
if (containsVolumeData_ != false) {
output.writeBool(15, containsVolumeData_);
}
if (containsSecrets_ != false) {
output.writeBool(16, containsSecrets_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(17, getClusterMetadata());
}
if (state_ != com.google.cloud.gkebackup.v1.Backup.State.STATE_UNSPECIFIED.getNumber()) {
output.writeEnum(18, state_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stateReason_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 19, stateReason_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(20, getCompleteTime());
}
if (resourceCount_ != 0) {
output.writeInt32(21, resourceCount_);
}
if (volumeCount_ != 0) {
output.writeInt32(22, volumeCount_);
}
if (sizeBytes_ != 0L) {
output.writeInt64(23, sizeBytes_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(etag_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 24, etag_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 25, description_);
}
if (podCount_ != 0) {
output.writeInt32(26, podCount_);
}
if (configBackupSizeBytes_ != 0L) {
output.writeInt64(27, configBackupSizeBytes_);
}
if (permissiveMode_ != false) {
output.writeBool(28, permissiveMode_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(uid_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, uid_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(3, getCreateTime());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(4, getUpdateTime());
}
if (manual_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(5, manual_);
}
for (java.util.Map.Entry entry :
internalGetLabels().getMap().entrySet()) {
com.google.protobuf.MapEntry labels__ =
LabelsDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(6, labels__);
}
if (deleteLockDays_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(7, deleteLockDays_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(8, getDeleteLockExpireTime());
}
if (retainDays_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(9, retainDays_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(10, getRetainExpireTime());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(11, getEncryptionKey());
}
if (backupScopeCase_ == 12) {
size +=
com.google.protobuf.CodedOutputStream.computeBoolSize(
12, (boolean) ((java.lang.Boolean) backupScope_));
}
if (backupScopeCase_ == 13) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
13, (com.google.cloud.gkebackup.v1.Namespaces) backupScope_);
}
if (backupScopeCase_ == 14) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
14, (com.google.cloud.gkebackup.v1.NamespacedNames) backupScope_);
}
if (containsVolumeData_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(15, containsVolumeData_);
}
if (containsSecrets_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(16, containsSecrets_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(17, getClusterMetadata());
}
if (state_ != com.google.cloud.gkebackup.v1.Backup.State.STATE_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(18, state_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(stateReason_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(19, stateReason_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(20, getCompleteTime());
}
if (resourceCount_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(21, resourceCount_);
}
if (volumeCount_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(22, volumeCount_);
}
if (sizeBytes_ != 0L) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(23, sizeBytes_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(etag_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(24, etag_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(25, description_);
}
if (podCount_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(26, podCount_);
}
if (configBackupSizeBytes_ != 0L) {
size += com.google.protobuf.CodedOutputStream.computeInt64Size(27, configBackupSizeBytes_);
}
if (permissiveMode_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(28, permissiveMode_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.gkebackup.v1.Backup)) {
return super.equals(obj);
}
com.google.cloud.gkebackup.v1.Backup other = (com.google.cloud.gkebackup.v1.Backup) obj;
if (!getName().equals(other.getName())) return false;
if (!getUid().equals(other.getUid())) return false;
if (hasCreateTime() != other.hasCreateTime()) return false;
if (hasCreateTime()) {
if (!getCreateTime().equals(other.getCreateTime())) return false;
}
if (hasUpdateTime() != other.hasUpdateTime()) return false;
if (hasUpdateTime()) {
if (!getUpdateTime().equals(other.getUpdateTime())) return false;
}
if (getManual() != other.getManual()) return false;
if (!internalGetLabels().equals(other.internalGetLabels())) return false;
if (getDeleteLockDays() != other.getDeleteLockDays()) return false;
if (hasDeleteLockExpireTime() != other.hasDeleteLockExpireTime()) return false;
if (hasDeleteLockExpireTime()) {
if (!getDeleteLockExpireTime().equals(other.getDeleteLockExpireTime())) return false;
}
if (getRetainDays() != other.getRetainDays()) return false;
if (hasRetainExpireTime() != other.hasRetainExpireTime()) return false;
if (hasRetainExpireTime()) {
if (!getRetainExpireTime().equals(other.getRetainExpireTime())) return false;
}
if (hasEncryptionKey() != other.hasEncryptionKey()) return false;
if (hasEncryptionKey()) {
if (!getEncryptionKey().equals(other.getEncryptionKey())) return false;
}
if (getContainsVolumeData() != other.getContainsVolumeData()) return false;
if (getContainsSecrets() != other.getContainsSecrets()) return false;
if (hasClusterMetadata() != other.hasClusterMetadata()) return false;
if (hasClusterMetadata()) {
if (!getClusterMetadata().equals(other.getClusterMetadata())) return false;
}
if (state_ != other.state_) return false;
if (!getStateReason().equals(other.getStateReason())) return false;
if (hasCompleteTime() != other.hasCompleteTime()) return false;
if (hasCompleteTime()) {
if (!getCompleteTime().equals(other.getCompleteTime())) return false;
}
if (getResourceCount() != other.getResourceCount()) return false;
if (getVolumeCount() != other.getVolumeCount()) return false;
if (getSizeBytes() != other.getSizeBytes()) return false;
if (!getEtag().equals(other.getEtag())) return false;
if (!getDescription().equals(other.getDescription())) return false;
if (getPodCount() != other.getPodCount()) return false;
if (getConfigBackupSizeBytes() != other.getConfigBackupSizeBytes()) return false;
if (getPermissiveMode() != other.getPermissiveMode()) return false;
if (!getBackupScopeCase().equals(other.getBackupScopeCase())) return false;
switch (backupScopeCase_) {
case 12:
if (getAllNamespaces() != other.getAllNamespaces()) return false;
break;
case 13:
if (!getSelectedNamespaces().equals(other.getSelectedNamespaces())) return false;
break;
case 14:
if (!getSelectedApplications().equals(other.getSelectedApplications())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + UID_FIELD_NUMBER;
hash = (53 * hash) + getUid().hashCode();
if (hasCreateTime()) {
hash = (37 * hash) + CREATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getCreateTime().hashCode();
}
if (hasUpdateTime()) {
hash = (37 * hash) + UPDATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getUpdateTime().hashCode();
}
hash = (37 * hash) + MANUAL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getManual());
if (!internalGetLabels().getMap().isEmpty()) {
hash = (37 * hash) + LABELS_FIELD_NUMBER;
hash = (53 * hash) + internalGetLabels().hashCode();
}
hash = (37 * hash) + DELETE_LOCK_DAYS_FIELD_NUMBER;
hash = (53 * hash) + getDeleteLockDays();
if (hasDeleteLockExpireTime()) {
hash = (37 * hash) + DELETE_LOCK_EXPIRE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getDeleteLockExpireTime().hashCode();
}
hash = (37 * hash) + RETAIN_DAYS_FIELD_NUMBER;
hash = (53 * hash) + getRetainDays();
if (hasRetainExpireTime()) {
hash = (37 * hash) + RETAIN_EXPIRE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getRetainExpireTime().hashCode();
}
if (hasEncryptionKey()) {
hash = (37 * hash) + ENCRYPTION_KEY_FIELD_NUMBER;
hash = (53 * hash) + getEncryptionKey().hashCode();
}
hash = (37 * hash) + CONTAINS_VOLUME_DATA_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getContainsVolumeData());
hash = (37 * hash) + CONTAINS_SECRETS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getContainsSecrets());
if (hasClusterMetadata()) {
hash = (37 * hash) + CLUSTER_METADATA_FIELD_NUMBER;
hash = (53 * hash) + getClusterMetadata().hashCode();
}
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + state_;
hash = (37 * hash) + STATE_REASON_FIELD_NUMBER;
hash = (53 * hash) + getStateReason().hashCode();
if (hasCompleteTime()) {
hash = (37 * hash) + COMPLETE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getCompleteTime().hashCode();
}
hash = (37 * hash) + RESOURCE_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getResourceCount();
hash = (37 * hash) + VOLUME_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getVolumeCount();
hash = (37 * hash) + SIZE_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getSizeBytes());
hash = (37 * hash) + ETAG_FIELD_NUMBER;
hash = (53 * hash) + getEtag().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + POD_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getPodCount();
hash = (37 * hash) + CONFIG_BACKUP_SIZE_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(getConfigBackupSizeBytes());
hash = (37 * hash) + PERMISSIVE_MODE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getPermissiveMode());
switch (backupScopeCase_) {
case 12:
hash = (37 * hash) + ALL_NAMESPACES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getAllNamespaces());
break;
case 13:
hash = (37 * hash) + SELECTED_NAMESPACES_FIELD_NUMBER;
hash = (53 * hash) + getSelectedNamespaces().hashCode();
break;
case 14:
hash = (37 * hash) + SELECTED_APPLICATIONS_FIELD_NUMBER;
hash = (53 * hash) + getSelectedApplications().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.gkebackup.v1.Backup parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.gkebackup.v1.Backup parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.gkebackup.v1.Backup parseFrom(com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.gkebackup.v1.Backup parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.gkebackup.v1.Backup parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.gkebackup.v1.Backup parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.gkebackup.v1.Backup parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.gkebackup.v1.Backup parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.gkebackup.v1.Backup parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.gkebackup.v1.Backup parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.gkebackup.v1.Backup parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.gkebackup.v1.Backup parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.gkebackup.v1.Backup prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Represents a request to perform a single point-in-time capture of
* some portion of the state of a GKE cluster, the record of the backup
* operation itself, and an anchor for the underlying artifacts that
* comprise the Backup (the config backup and VolumeBackups).
*
*
* Protobuf type {@code google.cloud.gkebackup.v1.Backup}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.gkebackup.v1.Backup)
com.google.cloud.gkebackup.v1.BackupOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 6:
return internalGetLabels();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 6:
return internalGetMutableLabels();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.gkebackup.v1.Backup.class,
com.google.cloud.gkebackup.v1.Backup.Builder.class);
}
// Construct using com.google.cloud.gkebackup.v1.Backup.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getCreateTimeFieldBuilder();
getUpdateTimeFieldBuilder();
getDeleteLockExpireTimeFieldBuilder();
getRetainExpireTimeFieldBuilder();
getEncryptionKeyFieldBuilder();
getClusterMetadataFieldBuilder();
getCompleteTimeFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
name_ = "";
uid_ = "";
createTime_ = null;
if (createTimeBuilder_ != null) {
createTimeBuilder_.dispose();
createTimeBuilder_ = null;
}
updateTime_ = null;
if (updateTimeBuilder_ != null) {
updateTimeBuilder_.dispose();
updateTimeBuilder_ = null;
}
manual_ = false;
internalGetMutableLabels().clear();
deleteLockDays_ = 0;
deleteLockExpireTime_ = null;
if (deleteLockExpireTimeBuilder_ != null) {
deleteLockExpireTimeBuilder_.dispose();
deleteLockExpireTimeBuilder_ = null;
}
retainDays_ = 0;
retainExpireTime_ = null;
if (retainExpireTimeBuilder_ != null) {
retainExpireTimeBuilder_.dispose();
retainExpireTimeBuilder_ = null;
}
encryptionKey_ = null;
if (encryptionKeyBuilder_ != null) {
encryptionKeyBuilder_.dispose();
encryptionKeyBuilder_ = null;
}
if (selectedNamespacesBuilder_ != null) {
selectedNamespacesBuilder_.clear();
}
if (selectedApplicationsBuilder_ != null) {
selectedApplicationsBuilder_.clear();
}
containsVolumeData_ = false;
containsSecrets_ = false;
clusterMetadata_ = null;
if (clusterMetadataBuilder_ != null) {
clusterMetadataBuilder_.dispose();
clusterMetadataBuilder_ = null;
}
state_ = 0;
stateReason_ = "";
completeTime_ = null;
if (completeTimeBuilder_ != null) {
completeTimeBuilder_.dispose();
completeTimeBuilder_ = null;
}
resourceCount_ = 0;
volumeCount_ = 0;
sizeBytes_ = 0L;
etag_ = "";
description_ = "";
podCount_ = 0;
configBackupSizeBytes_ = 0L;
permissiveMode_ = false;
backupScopeCase_ = 0;
backupScope_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.gkebackup.v1.BackupProto
.internal_static_google_cloud_gkebackup_v1_Backup_descriptor;
}
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup getDefaultInstanceForType() {
return com.google.cloud.gkebackup.v1.Backup.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup build() {
com.google.cloud.gkebackup.v1.Backup result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup buildPartial() {
com.google.cloud.gkebackup.v1.Backup result = new com.google.cloud.gkebackup.v1.Backup(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.gkebackup.v1.Backup result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.uid_ = uid_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000004) != 0)) {
result.createTime_ = createTimeBuilder_ == null ? createTime_ : createTimeBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.updateTime_ = updateTimeBuilder_ == null ? updateTime_ : updateTimeBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.manual_ = manual_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.labels_ = internalGetLabels();
result.labels_.makeImmutable();
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.deleteLockDays_ = deleteLockDays_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.deleteLockExpireTime_ =
deleteLockExpireTimeBuilder_ == null
? deleteLockExpireTime_
: deleteLockExpireTimeBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.retainDays_ = retainDays_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.retainExpireTime_ =
retainExpireTimeBuilder_ == null ? retainExpireTime_ : retainExpireTimeBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.encryptionKey_ =
encryptionKeyBuilder_ == null ? encryptionKey_ : encryptionKeyBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.containsVolumeData_ = containsVolumeData_;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.containsSecrets_ = containsSecrets_;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.clusterMetadata_ =
clusterMetadataBuilder_ == null ? clusterMetadata_ : clusterMetadataBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.state_ = state_;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.stateReason_ = stateReason_;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.completeTime_ =
completeTimeBuilder_ == null ? completeTime_ : completeTimeBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.resourceCount_ = resourceCount_;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.volumeCount_ = volumeCount_;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.sizeBytes_ = sizeBytes_;
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.etag_ = etag_;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
result.description_ = description_;
}
if (((from_bitField0_ & 0x02000000) != 0)) {
result.podCount_ = podCount_;
}
if (((from_bitField0_ & 0x04000000) != 0)) {
result.configBackupSizeBytes_ = configBackupSizeBytes_;
}
if (((from_bitField0_ & 0x08000000) != 0)) {
result.permissiveMode_ = permissiveMode_;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartialOneofs(com.google.cloud.gkebackup.v1.Backup result) {
result.backupScopeCase_ = backupScopeCase_;
result.backupScope_ = this.backupScope_;
if (backupScopeCase_ == 13 && selectedNamespacesBuilder_ != null) {
result.backupScope_ = selectedNamespacesBuilder_.build();
}
if (backupScopeCase_ == 14 && selectedApplicationsBuilder_ != null) {
result.backupScope_ = selectedApplicationsBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.gkebackup.v1.Backup) {
return mergeFrom((com.google.cloud.gkebackup.v1.Backup) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.gkebackup.v1.Backup other) {
if (other == com.google.cloud.gkebackup.v1.Backup.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getUid().isEmpty()) {
uid_ = other.uid_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.hasCreateTime()) {
mergeCreateTime(other.getCreateTime());
}
if (other.hasUpdateTime()) {
mergeUpdateTime(other.getUpdateTime());
}
if (other.getManual() != false) {
setManual(other.getManual());
}
internalGetMutableLabels().mergeFrom(other.internalGetLabels());
bitField0_ |= 0x00000020;
if (other.getDeleteLockDays() != 0) {
setDeleteLockDays(other.getDeleteLockDays());
}
if (other.hasDeleteLockExpireTime()) {
mergeDeleteLockExpireTime(other.getDeleteLockExpireTime());
}
if (other.getRetainDays() != 0) {
setRetainDays(other.getRetainDays());
}
if (other.hasRetainExpireTime()) {
mergeRetainExpireTime(other.getRetainExpireTime());
}
if (other.hasEncryptionKey()) {
mergeEncryptionKey(other.getEncryptionKey());
}
if (other.getContainsVolumeData() != false) {
setContainsVolumeData(other.getContainsVolumeData());
}
if (other.getContainsSecrets() != false) {
setContainsSecrets(other.getContainsSecrets());
}
if (other.hasClusterMetadata()) {
mergeClusterMetadata(other.getClusterMetadata());
}
if (other.state_ != 0) {
setStateValue(other.getStateValue());
}
if (!other.getStateReason().isEmpty()) {
stateReason_ = other.stateReason_;
bitField0_ |= 0x00040000;
onChanged();
}
if (other.hasCompleteTime()) {
mergeCompleteTime(other.getCompleteTime());
}
if (other.getResourceCount() != 0) {
setResourceCount(other.getResourceCount());
}
if (other.getVolumeCount() != 0) {
setVolumeCount(other.getVolumeCount());
}
if (other.getSizeBytes() != 0L) {
setSizeBytes(other.getSizeBytes());
}
if (!other.getEtag().isEmpty()) {
etag_ = other.etag_;
bitField0_ |= 0x00800000;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
bitField0_ |= 0x01000000;
onChanged();
}
if (other.getPodCount() != 0) {
setPodCount(other.getPodCount());
}
if (other.getConfigBackupSizeBytes() != 0L) {
setConfigBackupSizeBytes(other.getConfigBackupSizeBytes());
}
if (other.getPermissiveMode() != false) {
setPermissiveMode(other.getPermissiveMode());
}
switch (other.getBackupScopeCase()) {
case ALL_NAMESPACES:
{
setAllNamespaces(other.getAllNamespaces());
break;
}
case SELECTED_NAMESPACES:
{
mergeSelectedNamespaces(other.getSelectedNamespaces());
break;
}
case SELECTED_APPLICATIONS:
{
mergeSelectedApplications(other.getSelectedApplications());
break;
}
case BACKUPSCOPE_NOT_SET:
{
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
uid_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26:
{
input.readMessage(getCreateTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 34:
{
input.readMessage(getUpdateTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 40:
{
manual_ = input.readBool();
bitField0_ |= 0x00000010;
break;
} // case 40
case 50:
{
com.google.protobuf.MapEntry labels__ =
input.readMessage(
LabelsDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableLabels()
.getMutableMap()
.put(labels__.getKey(), labels__.getValue());
bitField0_ |= 0x00000020;
break;
} // case 50
case 56:
{
deleteLockDays_ = input.readInt32();
bitField0_ |= 0x00000040;
break;
} // case 56
case 66:
{
input.readMessage(
getDeleteLockExpireTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 66
case 72:
{
retainDays_ = input.readInt32();
bitField0_ |= 0x00000100;
break;
} // case 72
case 82:
{
input.readMessage(
getRetainExpireTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000200;
break;
} // case 82
case 90:
{
input.readMessage(getEncryptionKeyFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000400;
break;
} // case 90
case 96:
{
backupScope_ = input.readBool();
backupScopeCase_ = 12;
break;
} // case 96
case 106:
{
input.readMessage(
getSelectedNamespacesFieldBuilder().getBuilder(), extensionRegistry);
backupScopeCase_ = 13;
break;
} // case 106
case 114:
{
input.readMessage(
getSelectedApplicationsFieldBuilder().getBuilder(), extensionRegistry);
backupScopeCase_ = 14;
break;
} // case 114
case 120:
{
containsVolumeData_ = input.readBool();
bitField0_ |= 0x00004000;
break;
} // case 120
case 128:
{
containsSecrets_ = input.readBool();
bitField0_ |= 0x00008000;
break;
} // case 128
case 138:
{
input.readMessage(getClusterMetadataFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00010000;
break;
} // case 138
case 144:
{
state_ = input.readEnum();
bitField0_ |= 0x00020000;
break;
} // case 144
case 154:
{
stateReason_ = input.readStringRequireUtf8();
bitField0_ |= 0x00040000;
break;
} // case 154
case 162:
{
input.readMessage(getCompleteTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00080000;
break;
} // case 162
case 168:
{
resourceCount_ = input.readInt32();
bitField0_ |= 0x00100000;
break;
} // case 168
case 176:
{
volumeCount_ = input.readInt32();
bitField0_ |= 0x00200000;
break;
} // case 176
case 184:
{
sizeBytes_ = input.readInt64();
bitField0_ |= 0x00400000;
break;
} // case 184
case 194:
{
etag_ = input.readStringRequireUtf8();
bitField0_ |= 0x00800000;
break;
} // case 194
case 202:
{
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x01000000;
break;
} // case 202
case 208:
{
podCount_ = input.readInt32();
bitField0_ |= 0x02000000;
break;
} // case 208
case 216:
{
configBackupSizeBytes_ = input.readInt64();
bitField0_ |= 0x04000000;
break;
} // case 216
case 224:
{
permissiveMode_ = input.readBool();
bitField0_ |= 0x08000000;
break;
} // case 224
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int backupScopeCase_ = 0;
private java.lang.Object backupScope_;
public BackupScopeCase getBackupScopeCase() {
return BackupScopeCase.forNumber(backupScopeCase_);
}
public Builder clearBackupScope() {
backupScopeCase_ = 0;
backupScope_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.lang.Object name_ = "";
/**
*
*
*
* Output only. The fully qualified name of the Backup.
* `projects/*/locations/*/backupPlans/*/backups/*`
*
*
* string name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. The fully qualified name of the Backup.
* `projects/*/locations/*/backupPlans/*/backups/*`
*
*
* string name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. The fully qualified name of the Backup.
* `projects/*/locations/*/backupPlans/*/backups/*`
*
*
* string name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Output only. The fully qualified name of the Backup.
* `projects/*/locations/*/backupPlans/*/backups/*`
*
*
* string name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* Output only. The fully qualified name of the Backup.
* `projects/*/locations/*/backupPlans/*/backups/*`
*
*
* string name = 1 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object uid_ = "";
/**
*
*
*
* Output only. Server generated global unique identifier of
* [UUID4](https://en.wikipedia.org/wiki/Universally_unique_identifier)
*
*
* string uid = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The uid.
*/
public java.lang.String getUid() {
java.lang.Object ref = uid_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uid_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. Server generated global unique identifier of
* [UUID4](https://en.wikipedia.org/wiki/Universally_unique_identifier)
*
*
* string uid = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for uid.
*/
public com.google.protobuf.ByteString getUidBytes() {
java.lang.Object ref = uid_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
uid_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. Server generated global unique identifier of
* [UUID4](https://en.wikipedia.org/wiki/Universally_unique_identifier)
*
*
* string uid = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The uid to set.
* @return This builder for chaining.
*/
public Builder setUid(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uid_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Output only. Server generated global unique identifier of
* [UUID4](https://en.wikipedia.org/wiki/Universally_unique_identifier)
*
*
* string uid = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearUid() {
uid_ = getDefaultInstance().getUid();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* Output only. Server generated global unique identifier of
* [UUID4](https://en.wikipedia.org/wiki/Universally_unique_identifier)
*
*
* string uid = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The bytes for uid to set.
* @return This builder for chaining.
*/
public Builder setUidBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uid_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.Timestamp createTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
createTimeBuilder_;
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the createTime field is set.
*/
public boolean hasCreateTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The createTime.
*/
public com.google.protobuf.Timestamp getCreateTime() {
if (createTimeBuilder_ == null) {
return createTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: createTime_;
} else {
return createTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setCreateTime(com.google.protobuf.Timestamp value) {
if (createTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
createTime_ = value;
} else {
createTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setCreateTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (createTimeBuilder_ == null) {
createTime_ = builderForValue.build();
} else {
createTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeCreateTime(com.google.protobuf.Timestamp value) {
if (createTimeBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)
&& createTime_ != null
&& createTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getCreateTimeBuilder().mergeFrom(value);
} else {
createTime_ = value;
}
} else {
createTimeBuilder_.mergeFrom(value);
}
if (createTime_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearCreateTime() {
bitField0_ = (bitField0_ & ~0x00000004);
createTime_ = null;
if (createTimeBuilder_ != null) {
createTimeBuilder_.dispose();
createTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.Timestamp.Builder getCreateTimeBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getCreateTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder() {
if (createTimeBuilder_ != null) {
return createTimeBuilder_.getMessageOrBuilder();
} else {
return createTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: createTime_;
}
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was created.
*
*
*
* .google.protobuf.Timestamp create_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getCreateTimeFieldBuilder() {
if (createTimeBuilder_ == null) {
createTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getCreateTime(), getParentForChildren(), isClean());
createTime_ = null;
}
return createTimeBuilder_;
}
private com.google.protobuf.Timestamp updateTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
updateTimeBuilder_;
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the updateTime field is set.
*/
public boolean hasUpdateTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The updateTime.
*/
public com.google.protobuf.Timestamp getUpdateTime() {
if (updateTimeBuilder_ == null) {
return updateTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: updateTime_;
} else {
return updateTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setUpdateTime(com.google.protobuf.Timestamp value) {
if (updateTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
updateTime_ = value;
} else {
updateTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setUpdateTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (updateTimeBuilder_ == null) {
updateTime_ = builderForValue.build();
} else {
updateTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeUpdateTime(com.google.protobuf.Timestamp value) {
if (updateTimeBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& updateTime_ != null
&& updateTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getUpdateTimeBuilder().mergeFrom(value);
} else {
updateTime_ = value;
}
} else {
updateTimeBuilder_.mergeFrom(value);
}
if (updateTime_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearUpdateTime() {
bitField0_ = (bitField0_ & ~0x00000008);
updateTime_ = null;
if (updateTimeBuilder_ != null) {
updateTimeBuilder_.dispose();
updateTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.Timestamp.Builder getUpdateTimeBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getUpdateTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.TimestampOrBuilder getUpdateTimeOrBuilder() {
if (updateTimeBuilder_ != null) {
return updateTimeBuilder_.getMessageOrBuilder();
} else {
return updateTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: updateTime_;
}
}
/**
*
*
*
* Output only. The timestamp when this Backup resource was last updated.
*
*
*
* .google.protobuf.Timestamp update_time = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getUpdateTimeFieldBuilder() {
if (updateTimeBuilder_ == null) {
updateTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getUpdateTime(), getParentForChildren(), isClean());
updateTime_ = null;
}
return updateTimeBuilder_;
}
private boolean manual_;
/**
*
*
*
* Output only. This flag indicates whether this Backup resource was created
* manually by a user or via a schedule in the BackupPlan. A value of True
* means that the Backup was created manually.
*
*
* bool manual = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The manual.
*/
@java.lang.Override
public boolean getManual() {
return manual_;
}
/**
*
*
*
* Output only. This flag indicates whether this Backup resource was created
* manually by a user or via a schedule in the BackupPlan. A value of True
* means that the Backup was created manually.
*
*
* bool manual = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The manual to set.
* @return This builder for chaining.
*/
public Builder setManual(boolean value) {
manual_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Output only. This flag indicates whether this Backup resource was created
* manually by a user or via a schedule in the BackupPlan. A value of True
* means that the Backup was created manually.
*
*
* bool manual = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearManual() {
bitField0_ = (bitField0_ & ~0x00000010);
manual_ = false;
onChanged();
return this;
}
private com.google.protobuf.MapField labels_;
private com.google.protobuf.MapField internalGetLabels() {
if (labels_ == null) {
return com.google.protobuf.MapField.emptyMapField(LabelsDefaultEntryHolder.defaultEntry);
}
return labels_;
}
private com.google.protobuf.MapField
internalGetMutableLabels() {
if (labels_ == null) {
labels_ = com.google.protobuf.MapField.newMapField(LabelsDefaultEntryHolder.defaultEntry);
}
if (!labels_.isMutable()) {
labels_ = labels_.copy();
}
bitField0_ |= 0x00000020;
onChanged();
return labels_;
}
public int getLabelsCount() {
return internalGetLabels().getMap().size();
}
/**
*
*
*
* Optional. A set of custom labels supplied by user.
*
*
* map<string, string> labels = 6 [(.google.api.field_behavior) = OPTIONAL];
*/
@java.lang.Override
public boolean containsLabels(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetLabels().getMap().containsKey(key);
}
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getLabels() {
return getLabelsMap();
}
/**
*
*
*
* Optional. A set of custom labels supplied by user.
*
*
* map<string, string> labels = 6 [(.google.api.field_behavior) = OPTIONAL];
*/
@java.lang.Override
public java.util.Map getLabelsMap() {
return internalGetLabels().getMap();
}
/**
*
*
*
* Optional. A set of custom labels supplied by user.
*
*
* map<string, string> labels = 6 [(.google.api.field_behavior) = OPTIONAL];
*/
@java.lang.Override
public /* nullable */ java.lang.String getLabelsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Optional. A set of custom labels supplied by user.
*
*
* map<string, string> labels = 6 [(.google.api.field_behavior) = OPTIONAL];
*/
@java.lang.Override
public java.lang.String getLabelsOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map = internalGetLabels().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearLabels() {
bitField0_ = (bitField0_ & ~0x00000020);
internalGetMutableLabels().getMutableMap().clear();
return this;
}
/**
*
*
*
* Optional. A set of custom labels supplied by user.
*
*
* map<string, string> labels = 6 [(.google.api.field_behavior) = OPTIONAL];
*/
public Builder removeLabels(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableLabels().getMutableMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map getMutableLabels() {
bitField0_ |= 0x00000020;
return internalGetMutableLabels().getMutableMap();
}
/**
*
*
*
* Optional. A set of custom labels supplied by user.
*
*
* map<string, string> labels = 6 [(.google.api.field_behavior) = OPTIONAL];
*/
public Builder putLabels(java.lang.String key, java.lang.String value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableLabels().getMutableMap().put(key, value);
bitField0_ |= 0x00000020;
return this;
}
/**
*
*
*
* Optional. A set of custom labels supplied by user.
*
*
* map<string, string> labels = 6 [(.google.api.field_behavior) = OPTIONAL];
*/
public Builder putAllLabels(java.util.Map values) {
internalGetMutableLabels().getMutableMap().putAll(values);
bitField0_ |= 0x00000020;
return this;
}
private int deleteLockDays_;
/**
*
*
*
* Optional. Minimum age for this Backup (in days). If this field is set to a
* non-zero value, the Backup will be "locked" against deletion (either manual
* or automatic deletion) for the number of days provided (measured from the
* creation time of the Backup). MUST be an integer value between 0-90
* (inclusive).
*
* Defaults to parent BackupPlan's
* [backup_delete_lock_days][google.cloud.gkebackup.v1.BackupPlan.RetentionPolicy.backup_delete_lock_days]
* setting and may only be increased
* (either at creation time or in a subsequent update).
*
*
* int32 delete_lock_days = 7 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The deleteLockDays.
*/
@java.lang.Override
public int getDeleteLockDays() {
return deleteLockDays_;
}
/**
*
*
*
* Optional. Minimum age for this Backup (in days). If this field is set to a
* non-zero value, the Backup will be "locked" against deletion (either manual
* or automatic deletion) for the number of days provided (measured from the
* creation time of the Backup). MUST be an integer value between 0-90
* (inclusive).
*
* Defaults to parent BackupPlan's
* [backup_delete_lock_days][google.cloud.gkebackup.v1.BackupPlan.RetentionPolicy.backup_delete_lock_days]
* setting and may only be increased
* (either at creation time or in a subsequent update).
*
*
* int32 delete_lock_days = 7 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The deleteLockDays to set.
* @return This builder for chaining.
*/
public Builder setDeleteLockDays(int value) {
deleteLockDays_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Optional. Minimum age for this Backup (in days). If this field is set to a
* non-zero value, the Backup will be "locked" against deletion (either manual
* or automatic deletion) for the number of days provided (measured from the
* creation time of the Backup). MUST be an integer value between 0-90
* (inclusive).
*
* Defaults to parent BackupPlan's
* [backup_delete_lock_days][google.cloud.gkebackup.v1.BackupPlan.RetentionPolicy.backup_delete_lock_days]
* setting and may only be increased
* (either at creation time or in a subsequent update).
*
*
* int32 delete_lock_days = 7 [(.google.api.field_behavior) = OPTIONAL];
*
* @return This builder for chaining.
*/
public Builder clearDeleteLockDays() {
bitField0_ = (bitField0_ & ~0x00000040);
deleteLockDays_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Timestamp deleteLockExpireTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
deleteLockExpireTimeBuilder_;
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the deleteLockExpireTime field is set.
*/
public boolean hasDeleteLockExpireTime() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The deleteLockExpireTime.
*/
public com.google.protobuf.Timestamp getDeleteLockExpireTime() {
if (deleteLockExpireTimeBuilder_ == null) {
return deleteLockExpireTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: deleteLockExpireTime_;
} else {
return deleteLockExpireTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setDeleteLockExpireTime(com.google.protobuf.Timestamp value) {
if (deleteLockExpireTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
deleteLockExpireTime_ = value;
} else {
deleteLockExpireTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setDeleteLockExpireTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (deleteLockExpireTimeBuilder_ == null) {
deleteLockExpireTime_ = builderForValue.build();
} else {
deleteLockExpireTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeDeleteLockExpireTime(com.google.protobuf.Timestamp value) {
if (deleteLockExpireTimeBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)
&& deleteLockExpireTime_ != null
&& deleteLockExpireTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getDeleteLockExpireTimeBuilder().mergeFrom(value);
} else {
deleteLockExpireTime_ = value;
}
} else {
deleteLockExpireTimeBuilder_.mergeFrom(value);
}
if (deleteLockExpireTime_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearDeleteLockExpireTime() {
bitField0_ = (bitField0_ & ~0x00000080);
deleteLockExpireTime_ = null;
if (deleteLockExpireTimeBuilder_ != null) {
deleteLockExpireTimeBuilder_.dispose();
deleteLockExpireTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.Timestamp.Builder getDeleteLockExpireTimeBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getDeleteLockExpireTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.TimestampOrBuilder getDeleteLockExpireTimeOrBuilder() {
if (deleteLockExpireTimeBuilder_ != null) {
return deleteLockExpireTimeBuilder_.getMessageOrBuilder();
} else {
return deleteLockExpireTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: deleteLockExpireTime_;
}
}
/**
*
*
*
* Output only. The time at which an existing delete lock will expire for this
* backup (calculated from create_time +
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days]).
*
*
*
* .google.protobuf.Timestamp delete_lock_expire_time = 8 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getDeleteLockExpireTimeFieldBuilder() {
if (deleteLockExpireTimeBuilder_ == null) {
deleteLockExpireTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getDeleteLockExpireTime(), getParentForChildren(), isClean());
deleteLockExpireTime_ = null;
}
return deleteLockExpireTimeBuilder_;
}
private int retainDays_;
/**
*
*
*
* Optional. The age (in days) after which this Backup will be automatically
* deleted. Must be an integer value >= 0:
*
* - If 0, no automatic deletion will occur for this Backup.
* - If not 0, this must be >=
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days] and
* <= 365.
*
* Once a Backup is created, this value may only be increased.
*
* Defaults to the parent BackupPlan's
* [backup_retain_days][google.cloud.gkebackup.v1.BackupPlan.RetentionPolicy.backup_retain_days]
* value.
*
*
* int32 retain_days = 9 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The retainDays.
*/
@java.lang.Override
public int getRetainDays() {
return retainDays_;
}
/**
*
*
*
* Optional. The age (in days) after which this Backup will be automatically
* deleted. Must be an integer value >= 0:
*
* - If 0, no automatic deletion will occur for this Backup.
* - If not 0, this must be >=
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days] and
* <= 365.
*
* Once a Backup is created, this value may only be increased.
*
* Defaults to the parent BackupPlan's
* [backup_retain_days][google.cloud.gkebackup.v1.BackupPlan.RetentionPolicy.backup_retain_days]
* value.
*
*
* int32 retain_days = 9 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The retainDays to set.
* @return This builder for chaining.
*/
public Builder setRetainDays(int value) {
retainDays_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Optional. The age (in days) after which this Backup will be automatically
* deleted. Must be an integer value >= 0:
*
* - If 0, no automatic deletion will occur for this Backup.
* - If not 0, this must be >=
* [delete_lock_days][google.cloud.gkebackup.v1.Backup.delete_lock_days] and
* <= 365.
*
* Once a Backup is created, this value may only be increased.
*
* Defaults to the parent BackupPlan's
* [backup_retain_days][google.cloud.gkebackup.v1.BackupPlan.RetentionPolicy.backup_retain_days]
* value.
*
*
* int32 retain_days = 9 [(.google.api.field_behavior) = OPTIONAL];
*
* @return This builder for chaining.
*/
public Builder clearRetainDays() {
bitField0_ = (bitField0_ & ~0x00000100);
retainDays_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Timestamp retainExpireTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
retainExpireTimeBuilder_;
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the retainExpireTime field is set.
*/
public boolean hasRetainExpireTime() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The retainExpireTime.
*/
public com.google.protobuf.Timestamp getRetainExpireTime() {
if (retainExpireTimeBuilder_ == null) {
return retainExpireTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: retainExpireTime_;
} else {
return retainExpireTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setRetainExpireTime(com.google.protobuf.Timestamp value) {
if (retainExpireTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
retainExpireTime_ = value;
} else {
retainExpireTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setRetainExpireTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (retainExpireTimeBuilder_ == null) {
retainExpireTime_ = builderForValue.build();
} else {
retainExpireTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeRetainExpireTime(com.google.protobuf.Timestamp value) {
if (retainExpireTimeBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)
&& retainExpireTime_ != null
&& retainExpireTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getRetainExpireTimeBuilder().mergeFrom(value);
} else {
retainExpireTime_ = value;
}
} else {
retainExpireTimeBuilder_.mergeFrom(value);
}
if (retainExpireTime_ != null) {
bitField0_ |= 0x00000200;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearRetainExpireTime() {
bitField0_ = (bitField0_ & ~0x00000200);
retainExpireTime_ = null;
if (retainExpireTimeBuilder_ != null) {
retainExpireTimeBuilder_.dispose();
retainExpireTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.Timestamp.Builder getRetainExpireTimeBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getRetainExpireTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.TimestampOrBuilder getRetainExpireTimeOrBuilder() {
if (retainExpireTimeBuilder_ != null) {
return retainExpireTimeBuilder_.getMessageOrBuilder();
} else {
return retainExpireTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: retainExpireTime_;
}
}
/**
*
*
*
* Output only. The time at which this Backup will be automatically deleted
* (calculated from create_time +
* [retain_days][google.cloud.gkebackup.v1.Backup.retain_days]).
*
*
*
* .google.protobuf.Timestamp retain_expire_time = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getRetainExpireTimeFieldBuilder() {
if (retainExpireTimeBuilder_ == null) {
retainExpireTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getRetainExpireTime(), getParentForChildren(), isClean());
retainExpireTime_ = null;
}
return retainExpireTimeBuilder_;
}
private com.google.cloud.gkebackup.v1.EncryptionKey encryptionKey_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.EncryptionKey,
com.google.cloud.gkebackup.v1.EncryptionKey.Builder,
com.google.cloud.gkebackup.v1.EncryptionKeyOrBuilder>
encryptionKeyBuilder_;
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the encryptionKey field is set.
*/
public boolean hasEncryptionKey() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The encryptionKey.
*/
public com.google.cloud.gkebackup.v1.EncryptionKey getEncryptionKey() {
if (encryptionKeyBuilder_ == null) {
return encryptionKey_ == null
? com.google.cloud.gkebackup.v1.EncryptionKey.getDefaultInstance()
: encryptionKey_;
} else {
return encryptionKeyBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setEncryptionKey(com.google.cloud.gkebackup.v1.EncryptionKey value) {
if (encryptionKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
encryptionKey_ = value;
} else {
encryptionKeyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setEncryptionKey(
com.google.cloud.gkebackup.v1.EncryptionKey.Builder builderForValue) {
if (encryptionKeyBuilder_ == null) {
encryptionKey_ = builderForValue.build();
} else {
encryptionKeyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeEncryptionKey(com.google.cloud.gkebackup.v1.EncryptionKey value) {
if (encryptionKeyBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0)
&& encryptionKey_ != null
&& encryptionKey_ != com.google.cloud.gkebackup.v1.EncryptionKey.getDefaultInstance()) {
getEncryptionKeyBuilder().mergeFrom(value);
} else {
encryptionKey_ = value;
}
} else {
encryptionKeyBuilder_.mergeFrom(value);
}
if (encryptionKey_ != null) {
bitField0_ |= 0x00000400;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearEncryptionKey() {
bitField0_ = (bitField0_ & ~0x00000400);
encryptionKey_ = null;
if (encryptionKeyBuilder_ != null) {
encryptionKeyBuilder_.dispose();
encryptionKeyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.cloud.gkebackup.v1.EncryptionKey.Builder getEncryptionKeyBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getEncryptionKeyFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.cloud.gkebackup.v1.EncryptionKeyOrBuilder getEncryptionKeyOrBuilder() {
if (encryptionKeyBuilder_ != null) {
return encryptionKeyBuilder_.getMessageOrBuilder();
} else {
return encryptionKey_ == null
? com.google.cloud.gkebackup.v1.EncryptionKey.getDefaultInstance()
: encryptionKey_;
}
}
/**
*
*
*
* Output only. The customer managed encryption key that was used to encrypt
* the Backup's artifacts. Inherited from the parent BackupPlan's
* [encryption_key][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.encryption_key]
* value.
*
*
*
* .google.cloud.gkebackup.v1.EncryptionKey encryption_key = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.EncryptionKey,
com.google.cloud.gkebackup.v1.EncryptionKey.Builder,
com.google.cloud.gkebackup.v1.EncryptionKeyOrBuilder>
getEncryptionKeyFieldBuilder() {
if (encryptionKeyBuilder_ == null) {
encryptionKeyBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.EncryptionKey,
com.google.cloud.gkebackup.v1.EncryptionKey.Builder,
com.google.cloud.gkebackup.v1.EncryptionKeyOrBuilder>(
getEncryptionKey(), getParentForChildren(), isClean());
encryptionKey_ = null;
}
return encryptionKeyBuilder_;
}
/**
*
*
*
* Output only. If True, all namespaces were included in the Backup.
*
*
* bool all_namespaces = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return Whether the allNamespaces field is set.
*/
public boolean hasAllNamespaces() {
return backupScopeCase_ == 12;
}
/**
*
*
*
* Output only. If True, all namespaces were included in the Backup.
*
*
* bool all_namespaces = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The allNamespaces.
*/
public boolean getAllNamespaces() {
if (backupScopeCase_ == 12) {
return (java.lang.Boolean) backupScope_;
}
return false;
}
/**
*
*
*
* Output only. If True, all namespaces were included in the Backup.
*
*
* bool all_namespaces = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The allNamespaces to set.
* @return This builder for chaining.
*/
public Builder setAllNamespaces(boolean value) {
backupScopeCase_ = 12;
backupScope_ = value;
onChanged();
return this;
}
/**
*
*
*
* Output only. If True, all namespaces were included in the Backup.
*
*
* bool all_namespaces = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearAllNamespaces() {
if (backupScopeCase_ == 12) {
backupScopeCase_ = 0;
backupScope_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.Namespaces,
com.google.cloud.gkebackup.v1.Namespaces.Builder,
com.google.cloud.gkebackup.v1.NamespacesOrBuilder>
selectedNamespacesBuilder_;
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the selectedNamespaces field is set.
*/
@java.lang.Override
public boolean hasSelectedNamespaces() {
return backupScopeCase_ == 13;
}
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The selectedNamespaces.
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.Namespaces getSelectedNamespaces() {
if (selectedNamespacesBuilder_ == null) {
if (backupScopeCase_ == 13) {
return (com.google.cloud.gkebackup.v1.Namespaces) backupScope_;
}
return com.google.cloud.gkebackup.v1.Namespaces.getDefaultInstance();
} else {
if (backupScopeCase_ == 13) {
return selectedNamespacesBuilder_.getMessage();
}
return com.google.cloud.gkebackup.v1.Namespaces.getDefaultInstance();
}
}
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setSelectedNamespaces(com.google.cloud.gkebackup.v1.Namespaces value) {
if (selectedNamespacesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
backupScope_ = value;
onChanged();
} else {
selectedNamespacesBuilder_.setMessage(value);
}
backupScopeCase_ = 13;
return this;
}
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setSelectedNamespaces(
com.google.cloud.gkebackup.v1.Namespaces.Builder builderForValue) {
if (selectedNamespacesBuilder_ == null) {
backupScope_ = builderForValue.build();
onChanged();
} else {
selectedNamespacesBuilder_.setMessage(builderForValue.build());
}
backupScopeCase_ = 13;
return this;
}
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeSelectedNamespaces(com.google.cloud.gkebackup.v1.Namespaces value) {
if (selectedNamespacesBuilder_ == null) {
if (backupScopeCase_ == 13
&& backupScope_ != com.google.cloud.gkebackup.v1.Namespaces.getDefaultInstance()) {
backupScope_ =
com.google.cloud.gkebackup.v1.Namespaces.newBuilder(
(com.google.cloud.gkebackup.v1.Namespaces) backupScope_)
.mergeFrom(value)
.buildPartial();
} else {
backupScope_ = value;
}
onChanged();
} else {
if (backupScopeCase_ == 13) {
selectedNamespacesBuilder_.mergeFrom(value);
} else {
selectedNamespacesBuilder_.setMessage(value);
}
}
backupScopeCase_ = 13;
return this;
}
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearSelectedNamespaces() {
if (selectedNamespacesBuilder_ == null) {
if (backupScopeCase_ == 13) {
backupScopeCase_ = 0;
backupScope_ = null;
onChanged();
}
} else {
if (backupScopeCase_ == 13) {
backupScopeCase_ = 0;
backupScope_ = null;
}
selectedNamespacesBuilder_.clear();
}
return this;
}
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.cloud.gkebackup.v1.Namespaces.Builder getSelectedNamespacesBuilder() {
return getSelectedNamespacesFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.NamespacesOrBuilder getSelectedNamespacesOrBuilder() {
if ((backupScopeCase_ == 13) && (selectedNamespacesBuilder_ != null)) {
return selectedNamespacesBuilder_.getMessageOrBuilder();
} else {
if (backupScopeCase_ == 13) {
return (com.google.cloud.gkebackup.v1.Namespaces) backupScope_;
}
return com.google.cloud.gkebackup.v1.Namespaces.getDefaultInstance();
}
}
/**
*
*
*
* Output only. If set, the list of namespaces that were included in the
* Backup.
*
*
*
* .google.cloud.gkebackup.v1.Namespaces selected_namespaces = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.Namespaces,
com.google.cloud.gkebackup.v1.Namespaces.Builder,
com.google.cloud.gkebackup.v1.NamespacesOrBuilder>
getSelectedNamespacesFieldBuilder() {
if (selectedNamespacesBuilder_ == null) {
if (!(backupScopeCase_ == 13)) {
backupScope_ = com.google.cloud.gkebackup.v1.Namespaces.getDefaultInstance();
}
selectedNamespacesBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.Namespaces,
com.google.cloud.gkebackup.v1.Namespaces.Builder,
com.google.cloud.gkebackup.v1.NamespacesOrBuilder>(
(com.google.cloud.gkebackup.v1.Namespaces) backupScope_,
getParentForChildren(),
isClean());
backupScope_ = null;
}
backupScopeCase_ = 13;
onChanged();
return selectedNamespacesBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.NamespacedNames,
com.google.cloud.gkebackup.v1.NamespacedNames.Builder,
com.google.cloud.gkebackup.v1.NamespacedNamesOrBuilder>
selectedApplicationsBuilder_;
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the selectedApplications field is set.
*/
@java.lang.Override
public boolean hasSelectedApplications() {
return backupScopeCase_ == 14;
}
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The selectedApplications.
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.NamespacedNames getSelectedApplications() {
if (selectedApplicationsBuilder_ == null) {
if (backupScopeCase_ == 14) {
return (com.google.cloud.gkebackup.v1.NamespacedNames) backupScope_;
}
return com.google.cloud.gkebackup.v1.NamespacedNames.getDefaultInstance();
} else {
if (backupScopeCase_ == 14) {
return selectedApplicationsBuilder_.getMessage();
}
return com.google.cloud.gkebackup.v1.NamespacedNames.getDefaultInstance();
}
}
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setSelectedApplications(com.google.cloud.gkebackup.v1.NamespacedNames value) {
if (selectedApplicationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
backupScope_ = value;
onChanged();
} else {
selectedApplicationsBuilder_.setMessage(value);
}
backupScopeCase_ = 14;
return this;
}
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setSelectedApplications(
com.google.cloud.gkebackup.v1.NamespacedNames.Builder builderForValue) {
if (selectedApplicationsBuilder_ == null) {
backupScope_ = builderForValue.build();
onChanged();
} else {
selectedApplicationsBuilder_.setMessage(builderForValue.build());
}
backupScopeCase_ = 14;
return this;
}
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeSelectedApplications(com.google.cloud.gkebackup.v1.NamespacedNames value) {
if (selectedApplicationsBuilder_ == null) {
if (backupScopeCase_ == 14
&& backupScope_ != com.google.cloud.gkebackup.v1.NamespacedNames.getDefaultInstance()) {
backupScope_ =
com.google.cloud.gkebackup.v1.NamespacedNames.newBuilder(
(com.google.cloud.gkebackup.v1.NamespacedNames) backupScope_)
.mergeFrom(value)
.buildPartial();
} else {
backupScope_ = value;
}
onChanged();
} else {
if (backupScopeCase_ == 14) {
selectedApplicationsBuilder_.mergeFrom(value);
} else {
selectedApplicationsBuilder_.setMessage(value);
}
}
backupScopeCase_ = 14;
return this;
}
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearSelectedApplications() {
if (selectedApplicationsBuilder_ == null) {
if (backupScopeCase_ == 14) {
backupScopeCase_ = 0;
backupScope_ = null;
onChanged();
}
} else {
if (backupScopeCase_ == 14) {
backupScopeCase_ = 0;
backupScope_ = null;
}
selectedApplicationsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.cloud.gkebackup.v1.NamespacedNames.Builder getSelectedApplicationsBuilder() {
return getSelectedApplicationsFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.NamespacedNamesOrBuilder
getSelectedApplicationsOrBuilder() {
if ((backupScopeCase_ == 14) && (selectedApplicationsBuilder_ != null)) {
return selectedApplicationsBuilder_.getMessageOrBuilder();
} else {
if (backupScopeCase_ == 14) {
return (com.google.cloud.gkebackup.v1.NamespacedNames) backupScope_;
}
return com.google.cloud.gkebackup.v1.NamespacedNames.getDefaultInstance();
}
}
/**
*
*
*
* Output only. If set, the list of ProtectedApplications whose resources
* were included in the Backup.
*
*
*
* .google.cloud.gkebackup.v1.NamespacedNames selected_applications = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.NamespacedNames,
com.google.cloud.gkebackup.v1.NamespacedNames.Builder,
com.google.cloud.gkebackup.v1.NamespacedNamesOrBuilder>
getSelectedApplicationsFieldBuilder() {
if (selectedApplicationsBuilder_ == null) {
if (!(backupScopeCase_ == 14)) {
backupScope_ = com.google.cloud.gkebackup.v1.NamespacedNames.getDefaultInstance();
}
selectedApplicationsBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.NamespacedNames,
com.google.cloud.gkebackup.v1.NamespacedNames.Builder,
com.google.cloud.gkebackup.v1.NamespacedNamesOrBuilder>(
(com.google.cloud.gkebackup.v1.NamespacedNames) backupScope_,
getParentForChildren(),
isClean());
backupScope_ = null;
}
backupScopeCase_ = 14;
onChanged();
return selectedApplicationsBuilder_;
}
private boolean containsVolumeData_;
/**
*
*
*
* Output only. Whether or not the Backup contains volume data. Controlled by
* the parent BackupPlan's
* [include_volume_data][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.include_volume_data]
* value.
*
*
* bool contains_volume_data = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The containsVolumeData.
*/
@java.lang.Override
public boolean getContainsVolumeData() {
return containsVolumeData_;
}
/**
*
*
*
* Output only. Whether or not the Backup contains volume data. Controlled by
* the parent BackupPlan's
* [include_volume_data][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.include_volume_data]
* value.
*
*
* bool contains_volume_data = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The containsVolumeData to set.
* @return This builder for chaining.
*/
public Builder setContainsVolumeData(boolean value) {
containsVolumeData_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* Output only. Whether or not the Backup contains volume data. Controlled by
* the parent BackupPlan's
* [include_volume_data][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.include_volume_data]
* value.
*
*
* bool contains_volume_data = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearContainsVolumeData() {
bitField0_ = (bitField0_ & ~0x00004000);
containsVolumeData_ = false;
onChanged();
return this;
}
private boolean containsSecrets_;
/**
*
*
*
* Output only. Whether or not the Backup contains Kubernetes Secrets.
* Controlled by the parent BackupPlan's
* [include_secrets][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.include_secrets]
* value.
*
*
* bool contains_secrets = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The containsSecrets.
*/
@java.lang.Override
public boolean getContainsSecrets() {
return containsSecrets_;
}
/**
*
*
*
* Output only. Whether or not the Backup contains Kubernetes Secrets.
* Controlled by the parent BackupPlan's
* [include_secrets][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.include_secrets]
* value.
*
*
* bool contains_secrets = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The containsSecrets to set.
* @return This builder for chaining.
*/
public Builder setContainsSecrets(boolean value) {
containsSecrets_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Output only. Whether or not the Backup contains Kubernetes Secrets.
* Controlled by the parent BackupPlan's
* [include_secrets][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.include_secrets]
* value.
*
*
* bool contains_secrets = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearContainsSecrets() {
bitField0_ = (bitField0_ & ~0x00008000);
containsSecrets_ = false;
onChanged();
return this;
}
private com.google.cloud.gkebackup.v1.Backup.ClusterMetadata clusterMetadata_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata,
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.Builder,
com.google.cloud.gkebackup.v1.Backup.ClusterMetadataOrBuilder>
clusterMetadataBuilder_;
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the clusterMetadata field is set.
*/
public boolean hasClusterMetadata() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The clusterMetadata.
*/
public com.google.cloud.gkebackup.v1.Backup.ClusterMetadata getClusterMetadata() {
if (clusterMetadataBuilder_ == null) {
return clusterMetadata_ == null
? com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.getDefaultInstance()
: clusterMetadata_;
} else {
return clusterMetadataBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setClusterMetadata(com.google.cloud.gkebackup.v1.Backup.ClusterMetadata value) {
if (clusterMetadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
clusterMetadata_ = value;
} else {
clusterMetadataBuilder_.setMessage(value);
}
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setClusterMetadata(
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.Builder builderForValue) {
if (clusterMetadataBuilder_ == null) {
clusterMetadata_ = builderForValue.build();
} else {
clusterMetadataBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeClusterMetadata(
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata value) {
if (clusterMetadataBuilder_ == null) {
if (((bitField0_ & 0x00010000) != 0)
&& clusterMetadata_ != null
&& clusterMetadata_
!= com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.getDefaultInstance()) {
getClusterMetadataBuilder().mergeFrom(value);
} else {
clusterMetadata_ = value;
}
} else {
clusterMetadataBuilder_.mergeFrom(value);
}
if (clusterMetadata_ != null) {
bitField0_ |= 0x00010000;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearClusterMetadata() {
bitField0_ = (bitField0_ & ~0x00010000);
clusterMetadata_ = null;
if (clusterMetadataBuilder_ != null) {
clusterMetadataBuilder_.dispose();
clusterMetadataBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.Builder
getClusterMetadataBuilder() {
bitField0_ |= 0x00010000;
onChanged();
return getClusterMetadataFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.cloud.gkebackup.v1.Backup.ClusterMetadataOrBuilder
getClusterMetadataOrBuilder() {
if (clusterMetadataBuilder_ != null) {
return clusterMetadataBuilder_.getMessageOrBuilder();
} else {
return clusterMetadata_ == null
? com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.getDefaultInstance()
: clusterMetadata_;
}
}
/**
*
*
*
* Output only. Information about the GKE cluster from which this Backup was
* created.
*
*
*
* .google.cloud.gkebackup.v1.Backup.ClusterMetadata cluster_metadata = 17 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata,
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.Builder,
com.google.cloud.gkebackup.v1.Backup.ClusterMetadataOrBuilder>
getClusterMetadataFieldBuilder() {
if (clusterMetadataBuilder_ == null) {
clusterMetadataBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata,
com.google.cloud.gkebackup.v1.Backup.ClusterMetadata.Builder,
com.google.cloud.gkebackup.v1.Backup.ClusterMetadataOrBuilder>(
getClusterMetadata(), getParentForChildren(), isClean());
clusterMetadata_ = null;
}
return clusterMetadataBuilder_;
}
private int state_ = 0;
/**
*
*
*
* Output only. Current state of the Backup
*
*
*
* .google.cloud.gkebackup.v1.Backup.State state = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The enum numeric value on the wire for state.
*/
@java.lang.Override
public int getStateValue() {
return state_;
}
/**
*
*
*
* Output only. Current state of the Backup
*
*
*
* .google.cloud.gkebackup.v1.Backup.State state = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @param value The enum numeric value on the wire for state to set.
* @return This builder for chaining.
*/
public Builder setStateValue(int value) {
state_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
*
*
* Output only. Current state of the Backup
*
*
*
* .google.cloud.gkebackup.v1.Backup.State state = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The state.
*/
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup.State getState() {
com.google.cloud.gkebackup.v1.Backup.State result =
com.google.cloud.gkebackup.v1.Backup.State.forNumber(state_);
return result == null ? com.google.cloud.gkebackup.v1.Backup.State.UNRECOGNIZED : result;
}
/**
*
*
*
* Output only. Current state of the Backup
*
*
*
* .google.cloud.gkebackup.v1.Backup.State state = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @param value The state to set.
* @return This builder for chaining.
*/
public Builder setState(com.google.cloud.gkebackup.v1.Backup.State value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00020000;
state_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
*
* Output only. Current state of the Backup
*
*
*
* .google.cloud.gkebackup.v1.Backup.State state = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return This builder for chaining.
*/
public Builder clearState() {
bitField0_ = (bitField0_ & ~0x00020000);
state_ = 0;
onChanged();
return this;
}
private java.lang.Object stateReason_ = "";
/**
*
*
*
* Output only. Human-readable description of why the backup is in the current
* `state`.
*
*
* string state_reason = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The stateReason.
*/
public java.lang.String getStateReason() {
java.lang.Object ref = stateReason_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
stateReason_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. Human-readable description of why the backup is in the current
* `state`.
*
*
* string state_reason = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for stateReason.
*/
public com.google.protobuf.ByteString getStateReasonBytes() {
java.lang.Object ref = stateReason_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
stateReason_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. Human-readable description of why the backup is in the current
* `state`.
*
*
* string state_reason = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The stateReason to set.
* @return This builder for chaining.
*/
public Builder setStateReason(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
stateReason_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
*
*
* Output only. Human-readable description of why the backup is in the current
* `state`.
*
*
* string state_reason = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearStateReason() {
stateReason_ = getDefaultInstance().getStateReason();
bitField0_ = (bitField0_ & ~0x00040000);
onChanged();
return this;
}
/**
*
*
*
* Output only. Human-readable description of why the backup is in the current
* `state`.
*
*
* string state_reason = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The bytes for stateReason to set.
* @return This builder for chaining.
*/
public Builder setStateReasonBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
stateReason_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
private com.google.protobuf.Timestamp completeTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
completeTimeBuilder_;
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the completeTime field is set.
*/
public boolean hasCompleteTime() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The completeTime.
*/
public com.google.protobuf.Timestamp getCompleteTime() {
if (completeTimeBuilder_ == null) {
return completeTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: completeTime_;
} else {
return completeTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setCompleteTime(com.google.protobuf.Timestamp value) {
if (completeTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
completeTime_ = value;
} else {
completeTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setCompleteTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (completeTimeBuilder_ == null) {
completeTime_ = builderForValue.build();
} else {
completeTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeCompleteTime(com.google.protobuf.Timestamp value) {
if (completeTimeBuilder_ == null) {
if (((bitField0_ & 0x00080000) != 0)
&& completeTime_ != null
&& completeTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getCompleteTimeBuilder().mergeFrom(value);
} else {
completeTime_ = value;
}
} else {
completeTimeBuilder_.mergeFrom(value);
}
if (completeTime_ != null) {
bitField0_ |= 0x00080000;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearCompleteTime() {
bitField0_ = (bitField0_ & ~0x00080000);
completeTime_ = null;
if (completeTimeBuilder_ != null) {
completeTimeBuilder_.dispose();
completeTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.Timestamp.Builder getCompleteTimeBuilder() {
bitField0_ |= 0x00080000;
onChanged();
return getCompleteTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.TimestampOrBuilder getCompleteTimeOrBuilder() {
if (completeTimeBuilder_ != null) {
return completeTimeBuilder_.getMessageOrBuilder();
} else {
return completeTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: completeTime_;
}
}
/**
*
*
*
* Output only. Completion time of the Backup
*
*
*
* .google.protobuf.Timestamp complete_time = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getCompleteTimeFieldBuilder() {
if (completeTimeBuilder_ == null) {
completeTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getCompleteTime(), getParentForChildren(), isClean());
completeTime_ = null;
}
return completeTimeBuilder_;
}
private int resourceCount_;
/**
*
*
*
* Output only. The total number of Kubernetes resources included in the
* Backup.
*
*
* int32 resource_count = 21 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The resourceCount.
*/
@java.lang.Override
public int getResourceCount() {
return resourceCount_;
}
/**
*
*
*
* Output only. The total number of Kubernetes resources included in the
* Backup.
*
*
* int32 resource_count = 21 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The resourceCount to set.
* @return This builder for chaining.
*/
public Builder setResourceCount(int value) {
resourceCount_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
*
*
* Output only. The total number of Kubernetes resources included in the
* Backup.
*
*
* int32 resource_count = 21 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearResourceCount() {
bitField0_ = (bitField0_ & ~0x00100000);
resourceCount_ = 0;
onChanged();
return this;
}
private int volumeCount_;
/**
*
*
*
* Output only. The total number of volume backups contained in the Backup.
*
*
* int32 volume_count = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The volumeCount.
*/
@java.lang.Override
public int getVolumeCount() {
return volumeCount_;
}
/**
*
*
*
* Output only. The total number of volume backups contained in the Backup.
*
*
* int32 volume_count = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The volumeCount to set.
* @return This builder for chaining.
*/
public Builder setVolumeCount(int value) {
volumeCount_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
*
*
* Output only. The total number of volume backups contained in the Backup.
*
*
* int32 volume_count = 22 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearVolumeCount() {
bitField0_ = (bitField0_ & ~0x00200000);
volumeCount_ = 0;
onChanged();
return this;
}
private long sizeBytes_;
/**
*
*
*
* Output only. The total size of the Backup in bytes = config backup size +
* sum(volume backup sizes)
*
*
* int64 size_bytes = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The sizeBytes.
*/
@java.lang.Override
public long getSizeBytes() {
return sizeBytes_;
}
/**
*
*
*
* Output only. The total size of the Backup in bytes = config backup size +
* sum(volume backup sizes)
*
*
* int64 size_bytes = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The sizeBytes to set.
* @return This builder for chaining.
*/
public Builder setSizeBytes(long value) {
sizeBytes_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
*
*
* Output only. The total size of the Backup in bytes = config backup size +
* sum(volume backup sizes)
*
*
* int64 size_bytes = 23 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearSizeBytes() {
bitField0_ = (bitField0_ & ~0x00400000);
sizeBytes_ = 0L;
onChanged();
return this;
}
private java.lang.Object etag_ = "";
/**
*
*
*
* Output only. `etag` is used for optimistic concurrency control as a way to
* help prevent simultaneous updates of a backup from overwriting each other.
* It is strongly suggested that systems make use of the `etag` in the
* read-modify-write cycle to perform backup updates in order to avoid
* race conditions: An `etag` is returned in the response to `GetBackup`,
* and systems are expected to put that etag in the request to
* `UpdateBackup` or `DeleteBackup` to ensure that their change will be
* applied to the same version of the resource.
*
*
* string etag = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The etag.
*/
public java.lang.String getEtag() {
java.lang.Object ref = etag_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
etag_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. `etag` is used for optimistic concurrency control as a way to
* help prevent simultaneous updates of a backup from overwriting each other.
* It is strongly suggested that systems make use of the `etag` in the
* read-modify-write cycle to perform backup updates in order to avoid
* race conditions: An `etag` is returned in the response to `GetBackup`,
* and systems are expected to put that etag in the request to
* `UpdateBackup` or `DeleteBackup` to ensure that their change will be
* applied to the same version of the resource.
*
*
* string etag = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for etag.
*/
public com.google.protobuf.ByteString getEtagBytes() {
java.lang.Object ref = etag_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
etag_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. `etag` is used for optimistic concurrency control as a way to
* help prevent simultaneous updates of a backup from overwriting each other.
* It is strongly suggested that systems make use of the `etag` in the
* read-modify-write cycle to perform backup updates in order to avoid
* race conditions: An `etag` is returned in the response to `GetBackup`,
* and systems are expected to put that etag in the request to
* `UpdateBackup` or `DeleteBackup` to ensure that their change will be
* applied to the same version of the resource.
*
*
* string etag = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The etag to set.
* @return This builder for chaining.
*/
public Builder setEtag(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
etag_ = value;
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
*
*
* Output only. `etag` is used for optimistic concurrency control as a way to
* help prevent simultaneous updates of a backup from overwriting each other.
* It is strongly suggested that systems make use of the `etag` in the
* read-modify-write cycle to perform backup updates in order to avoid
* race conditions: An `etag` is returned in the response to `GetBackup`,
* and systems are expected to put that etag in the request to
* `UpdateBackup` or `DeleteBackup` to ensure that their change will be
* applied to the same version of the resource.
*
*
* string etag = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearEtag() {
etag_ = getDefaultInstance().getEtag();
bitField0_ = (bitField0_ & ~0x00800000);
onChanged();
return this;
}
/**
*
*
*
* Output only. `etag` is used for optimistic concurrency control as a way to
* help prevent simultaneous updates of a backup from overwriting each other.
* It is strongly suggested that systems make use of the `etag` in the
* read-modify-write cycle to perform backup updates in order to avoid
* race conditions: An `etag` is returned in the response to `GetBackup`,
* and systems are expected to put that etag in the request to
* `UpdateBackup` or `DeleteBackup` to ensure that their change will be
* applied to the same version of the resource.
*
*
* string etag = 24 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The bytes for etag to set.
* @return This builder for chaining.
*/
public Builder setEtagBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
etag_ = value;
bitField0_ |= 0x00800000;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
*
*
* Optional. User specified descriptive string for this Backup.
*
*
* string description = 25 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Optional. User specified descriptive string for this Backup.
*
*
* string description = 25 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for description.
*/
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Optional. User specified descriptive string for this Backup.
*
*
* string description = 25 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
*
*
*
* Optional. User specified descriptive string for this Backup.
*
*
* string description = 25 [(.google.api.field_behavior) = OPTIONAL];
*
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x01000000);
onChanged();
return this;
}
/**
*
*
*
* Optional. User specified descriptive string for this Backup.
*
*
* string description = 25 [(.google.api.field_behavior) = OPTIONAL];
*
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
private int podCount_;
/**
*
*
*
* Output only. The total number of Kubernetes Pods contained in the Backup.
*
*
* int32 pod_count = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The podCount.
*/
@java.lang.Override
public int getPodCount() {
return podCount_;
}
/**
*
*
*
* Output only. The total number of Kubernetes Pods contained in the Backup.
*
*
* int32 pod_count = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The podCount to set.
* @return This builder for chaining.
*/
public Builder setPodCount(int value) {
podCount_ = value;
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
*
*
* Output only. The total number of Kubernetes Pods contained in the Backup.
*
*
* int32 pod_count = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearPodCount() {
bitField0_ = (bitField0_ & ~0x02000000);
podCount_ = 0;
onChanged();
return this;
}
private long configBackupSizeBytes_;
/**
*
*
*
* Output only. The size of the config backup in bytes.
*
*
* int64 config_backup_size_bytes = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The configBackupSizeBytes.
*/
@java.lang.Override
public long getConfigBackupSizeBytes() {
return configBackupSizeBytes_;
}
/**
*
*
*
* Output only. The size of the config backup in bytes.
*
*
* int64 config_backup_size_bytes = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @param value The configBackupSizeBytes to set.
* @return This builder for chaining.
*/
public Builder setConfigBackupSizeBytes(long value) {
configBackupSizeBytes_ = value;
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
*
*
*
* Output only. The size of the config backup in bytes.
*
*
* int64 config_backup_size_bytes = 27 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return This builder for chaining.
*/
public Builder clearConfigBackupSizeBytes() {
bitField0_ = (bitField0_ & ~0x04000000);
configBackupSizeBytes_ = 0L;
onChanged();
return this;
}
private boolean permissiveMode_;
/**
*
*
*
* Output only. If false, Backup will fail when Backup for GKE detects
* Kubernetes configuration that is non-standard or
* requires additional setup to restore.
*
* Inherited from the parent BackupPlan's
* [permissive_mode][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.permissive_mode]
* value.
*
*
* bool permissive_mode = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The permissiveMode.
*/
@java.lang.Override
public boolean getPermissiveMode() {
return permissiveMode_;
}
/**
*
*
*
* Output only. If false, Backup will fail when Backup for GKE detects
* Kubernetes configuration that is non-standard or
* requires additional setup to restore.
*
* Inherited from the parent BackupPlan's
* [permissive_mode][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.permissive_mode]
* value.
*
*
* bool permissive_mode = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The permissiveMode to set.
* @return This builder for chaining.
*/
public Builder setPermissiveMode(boolean value) {
permissiveMode_ = value;
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
*
*
* Output only. If false, Backup will fail when Backup for GKE detects
* Kubernetes configuration that is non-standard or
* requires additional setup to restore.
*
* Inherited from the parent BackupPlan's
* [permissive_mode][google.cloud.gkebackup.v1.BackupPlan.BackupConfig.permissive_mode]
* value.
*
*
* bool permissive_mode = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearPermissiveMode() {
bitField0_ = (bitField0_ & ~0x08000000);
permissiveMode_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.gkebackup.v1.Backup)
}
// @@protoc_insertion_point(class_scope:google.cloud.gkebackup.v1.Backup)
private static final com.google.cloud.gkebackup.v1.Backup DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.gkebackup.v1.Backup();
}
public static com.google.cloud.gkebackup.v1.Backup getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Backup parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.gkebackup.v1.Backup getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy