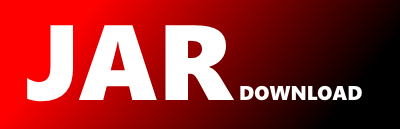
com.google.cloud.video.livestream.v1.ChannelOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-live-stream-v1 Show documentation
Show all versions of proto-google-cloud-live-stream-v1 Show documentation
Proto library for google-cloud-live-stream
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/video/livestream/v1/resources.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.video.livestream.v1;
public interface ChannelOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.video.livestream.v1.Channel)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* The resource name of the channel, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
*
*
* string name = 1;
*
* @return The name.
*/
java.lang.String getName();
/**
*
*
*
* The resource name of the channel, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
*
*
* string name = 1;
*
* @return The bytes for name.
*/
com.google.protobuf.ByteString getNameBytes();
/**
*
*
*
* Output only. The creation time.
*
*
* .google.protobuf.Timestamp create_time = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the createTime field is set.
*/
boolean hasCreateTime();
/**
*
*
*
* Output only. The creation time.
*
*
* .google.protobuf.Timestamp create_time = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The createTime.
*/
com.google.protobuf.Timestamp getCreateTime();
/**
*
*
*
* Output only. The creation time.
*
*
* .google.protobuf.Timestamp create_time = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder();
/**
*
*
*
* Output only. The update time.
*
*
* .google.protobuf.Timestamp update_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the updateTime field is set.
*/
boolean hasUpdateTime();
/**
*
*
*
* Output only. The update time.
*
*
* .google.protobuf.Timestamp update_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The updateTime.
*/
com.google.protobuf.Timestamp getUpdateTime();
/**
*
*
*
* Output only. The update time.
*
*
* .google.protobuf.Timestamp update_time = 3 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.protobuf.TimestampOrBuilder getUpdateTimeOrBuilder();
/**
*
*
*
* User-defined key/value metadata.
*
*
* map<string, string> labels = 4;
*/
int getLabelsCount();
/**
*
*
*
* User-defined key/value metadata.
*
*
* map<string, string> labels = 4;
*/
boolean containsLabels(java.lang.String key);
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Deprecated
java.util.Map getLabels();
/**
*
*
*
* User-defined key/value metadata.
*
*
* map<string, string> labels = 4;
*/
java.util.Map getLabelsMap();
/**
*
*
*
* User-defined key/value metadata.
*
*
* map<string, string> labels = 4;
*/
/* nullable */
java.lang.String getLabelsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
*
*
*
* User-defined key/value metadata.
*
*
* map<string, string> labels = 4;
*/
java.lang.String getLabelsOrThrow(java.lang.String key);
/**
*
*
*
* A list of input attachments that this channel uses.
* One channel can have multiple inputs as the input sources. Only one
* input can be selected as the input source at one time.
*
*
* repeated .google.cloud.video.livestream.v1.InputAttachment input_attachments = 16;
*/
java.util.List getInputAttachmentsList();
/**
*
*
*
* A list of input attachments that this channel uses.
* One channel can have multiple inputs as the input sources. Only one
* input can be selected as the input source at one time.
*
*
* repeated .google.cloud.video.livestream.v1.InputAttachment input_attachments = 16;
*/
com.google.cloud.video.livestream.v1.InputAttachment getInputAttachments(int index);
/**
*
*
*
* A list of input attachments that this channel uses.
* One channel can have multiple inputs as the input sources. Only one
* input can be selected as the input source at one time.
*
*
* repeated .google.cloud.video.livestream.v1.InputAttachment input_attachments = 16;
*/
int getInputAttachmentsCount();
/**
*
*
*
* A list of input attachments that this channel uses.
* One channel can have multiple inputs as the input sources. Only one
* input can be selected as the input source at one time.
*
*
* repeated .google.cloud.video.livestream.v1.InputAttachment input_attachments = 16;
*/
java.util.List extends com.google.cloud.video.livestream.v1.InputAttachmentOrBuilder>
getInputAttachmentsOrBuilderList();
/**
*
*
*
* A list of input attachments that this channel uses.
* One channel can have multiple inputs as the input sources. Only one
* input can be selected as the input source at one time.
*
*
* repeated .google.cloud.video.livestream.v1.InputAttachment input_attachments = 16;
*/
com.google.cloud.video.livestream.v1.InputAttachmentOrBuilder getInputAttachmentsOrBuilder(
int index);
/**
*
*
*
* Output only. The
* [InputAttachment.key][google.cloud.video.livestream.v1.InputAttachment.key]
* that serves as the current input source. The first input in the
* [input_attachments][google.cloud.video.livestream.v1.Channel.input_attachments]
* is the initial input source.
*
*
* string active_input = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The activeInput.
*/
java.lang.String getActiveInput();
/**
*
*
*
* Output only. The
* [InputAttachment.key][google.cloud.video.livestream.v1.InputAttachment.key]
* that serves as the current input source. The first input in the
* [input_attachments][google.cloud.video.livestream.v1.Channel.input_attachments]
* is the initial input source.
*
*
* string active_input = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for activeInput.
*/
com.google.protobuf.ByteString getActiveInputBytes();
/**
*
*
*
* Required. Information about the output (that is, the Cloud Storage bucket
* to store the generated live stream).
*
*
*
* .google.cloud.video.livestream.v1.Channel.Output output = 9 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the output field is set.
*/
boolean hasOutput();
/**
*
*
*
* Required. Information about the output (that is, the Cloud Storage bucket
* to store the generated live stream).
*
*
*
* .google.cloud.video.livestream.v1.Channel.Output output = 9 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The output.
*/
com.google.cloud.video.livestream.v1.Channel.Output getOutput();
/**
*
*
*
* Required. Information about the output (that is, the Cloud Storage bucket
* to store the generated live stream).
*
*
*
* .google.cloud.video.livestream.v1.Channel.Output output = 9 [(.google.api.field_behavior) = REQUIRED];
*
*/
com.google.cloud.video.livestream.v1.Channel.OutputOrBuilder getOutputOrBuilder();
/**
*
*
*
* List of elementary streams.
*
*
* repeated .google.cloud.video.livestream.v1.ElementaryStream elementary_streams = 10;
*
*/
java.util.List getElementaryStreamsList();
/**
*
*
*
* List of elementary streams.
*
*
* repeated .google.cloud.video.livestream.v1.ElementaryStream elementary_streams = 10;
*
*/
com.google.cloud.video.livestream.v1.ElementaryStream getElementaryStreams(int index);
/**
*
*
*
* List of elementary streams.
*
*
* repeated .google.cloud.video.livestream.v1.ElementaryStream elementary_streams = 10;
*
*/
int getElementaryStreamsCount();
/**
*
*
*
* List of elementary streams.
*
*
* repeated .google.cloud.video.livestream.v1.ElementaryStream elementary_streams = 10;
*
*/
java.util.List extends com.google.cloud.video.livestream.v1.ElementaryStreamOrBuilder>
getElementaryStreamsOrBuilderList();
/**
*
*
*
* List of elementary streams.
*
*
* repeated .google.cloud.video.livestream.v1.ElementaryStream elementary_streams = 10;
*
*/
com.google.cloud.video.livestream.v1.ElementaryStreamOrBuilder getElementaryStreamsOrBuilder(
int index);
/**
*
*
*
* List of multiplexing settings for output streams.
*
*
* repeated .google.cloud.video.livestream.v1.MuxStream mux_streams = 11;
*/
java.util.List getMuxStreamsList();
/**
*
*
*
* List of multiplexing settings for output streams.
*
*
* repeated .google.cloud.video.livestream.v1.MuxStream mux_streams = 11;
*/
com.google.cloud.video.livestream.v1.MuxStream getMuxStreams(int index);
/**
*
*
*
* List of multiplexing settings for output streams.
*
*
* repeated .google.cloud.video.livestream.v1.MuxStream mux_streams = 11;
*/
int getMuxStreamsCount();
/**
*
*
*
* List of multiplexing settings for output streams.
*
*
* repeated .google.cloud.video.livestream.v1.MuxStream mux_streams = 11;
*/
java.util.List extends com.google.cloud.video.livestream.v1.MuxStreamOrBuilder>
getMuxStreamsOrBuilderList();
/**
*
*
*
* List of multiplexing settings for output streams.
*
*
* repeated .google.cloud.video.livestream.v1.MuxStream mux_streams = 11;
*/
com.google.cloud.video.livestream.v1.MuxStreamOrBuilder getMuxStreamsOrBuilder(int index);
/**
*
*
*
* List of output manifests.
*
*
* repeated .google.cloud.video.livestream.v1.Manifest manifests = 12;
*/
java.util.List getManifestsList();
/**
*
*
*
* List of output manifests.
*
*
* repeated .google.cloud.video.livestream.v1.Manifest manifests = 12;
*/
com.google.cloud.video.livestream.v1.Manifest getManifests(int index);
/**
*
*
*
* List of output manifests.
*
*
* repeated .google.cloud.video.livestream.v1.Manifest manifests = 12;
*/
int getManifestsCount();
/**
*
*
*
* List of output manifests.
*
*
* repeated .google.cloud.video.livestream.v1.Manifest manifests = 12;
*/
java.util.List extends com.google.cloud.video.livestream.v1.ManifestOrBuilder>
getManifestsOrBuilderList();
/**
*
*
*
* List of output manifests.
*
*
* repeated .google.cloud.video.livestream.v1.Manifest manifests = 12;
*/
com.google.cloud.video.livestream.v1.ManifestOrBuilder getManifestsOrBuilder(int index);
/**
*
*
*
* List of output sprite sheets.
*
*
* repeated .google.cloud.video.livestream.v1.SpriteSheet sprite_sheets = 13;
*/
java.util.List getSpriteSheetsList();
/**
*
*
*
* List of output sprite sheets.
*
*
* repeated .google.cloud.video.livestream.v1.SpriteSheet sprite_sheets = 13;
*/
com.google.cloud.video.livestream.v1.SpriteSheet getSpriteSheets(int index);
/**
*
*
*
* List of output sprite sheets.
*
*
* repeated .google.cloud.video.livestream.v1.SpriteSheet sprite_sheets = 13;
*/
int getSpriteSheetsCount();
/**
*
*
*
* List of output sprite sheets.
*
*
* repeated .google.cloud.video.livestream.v1.SpriteSheet sprite_sheets = 13;
*/
java.util.List extends com.google.cloud.video.livestream.v1.SpriteSheetOrBuilder>
getSpriteSheetsOrBuilderList();
/**
*
*
*
* List of output sprite sheets.
*
*
* repeated .google.cloud.video.livestream.v1.SpriteSheet sprite_sheets = 13;
*/
com.google.cloud.video.livestream.v1.SpriteSheetOrBuilder getSpriteSheetsOrBuilder(int index);
/**
*
*
*
* Output only. State of the streaming operation.
*
*
*
* .google.cloud.video.livestream.v1.Channel.StreamingState streaming_state = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The enum numeric value on the wire for streamingState.
*/
int getStreamingStateValue();
/**
*
*
*
* Output only. State of the streaming operation.
*
*
*
* .google.cloud.video.livestream.v1.Channel.StreamingState streaming_state = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The streamingState.
*/
com.google.cloud.video.livestream.v1.Channel.StreamingState getStreamingState();
/**
*
*
*
* Output only. A description of the reason for the streaming error. This
* property is always present when
* [streaming_state][google.cloud.video.livestream.v1.Channel.streaming_state]
* is
* [STREAMING_ERROR][google.cloud.video.livestream.v1.Channel.StreamingState.STREAMING_ERROR].
*
*
* .google.rpc.Status streaming_error = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the streamingError field is set.
*/
boolean hasStreamingError();
/**
*
*
*
* Output only. A description of the reason for the streaming error. This
* property is always present when
* [streaming_state][google.cloud.video.livestream.v1.Channel.streaming_state]
* is
* [STREAMING_ERROR][google.cloud.video.livestream.v1.Channel.StreamingState.STREAMING_ERROR].
*
*
* .google.rpc.Status streaming_error = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The streamingError.
*/
com.google.rpc.Status getStreamingError();
/**
*
*
*
* Output only. A description of the reason for the streaming error. This
* property is always present when
* [streaming_state][google.cloud.video.livestream.v1.Channel.streaming_state]
* is
* [STREAMING_ERROR][google.cloud.video.livestream.v1.Channel.StreamingState.STREAMING_ERROR].
*
*
* .google.rpc.Status streaming_error = 18 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.rpc.StatusOrBuilder getStreamingErrorOrBuilder();
/**
*
*
*
* Configuration of platform logs for this channel.
*
*
* .google.cloud.video.livestream.v1.LogConfig log_config = 19;
*
* @return Whether the logConfig field is set.
*/
boolean hasLogConfig();
/**
*
*
*
* Configuration of platform logs for this channel.
*
*
* .google.cloud.video.livestream.v1.LogConfig log_config = 19;
*
* @return The logConfig.
*/
com.google.cloud.video.livestream.v1.LogConfig getLogConfig();
/**
*
*
*
* Configuration of platform logs for this channel.
*
*
* .google.cloud.video.livestream.v1.LogConfig log_config = 19;
*/
com.google.cloud.video.livestream.v1.LogConfigOrBuilder getLogConfigOrBuilder();
/**
*
*
*
* Configuration of timecode for this channel.
*
*
* .google.cloud.video.livestream.v1.TimecodeConfig timecode_config = 21;
*
* @return Whether the timecodeConfig field is set.
*/
boolean hasTimecodeConfig();
/**
*
*
*
* Configuration of timecode for this channel.
*
*
* .google.cloud.video.livestream.v1.TimecodeConfig timecode_config = 21;
*
* @return The timecodeConfig.
*/
com.google.cloud.video.livestream.v1.TimecodeConfig getTimecodeConfig();
/**
*
*
*
* Configuration of timecode for this channel.
*
*
* .google.cloud.video.livestream.v1.TimecodeConfig timecode_config = 21;
*/
com.google.cloud.video.livestream.v1.TimecodeConfigOrBuilder getTimecodeConfigOrBuilder();
/**
*
*
*
* Encryption configurations for this channel. Each configuration has an ID
* which is referred to by each MuxStream to indicate which configuration is
* used for that output.
*
*
* repeated .google.cloud.video.livestream.v1.Encryption encryptions = 24;
*/
java.util.List getEncryptionsList();
/**
*
*
*
* Encryption configurations for this channel. Each configuration has an ID
* which is referred to by each MuxStream to indicate which configuration is
* used for that output.
*
*
* repeated .google.cloud.video.livestream.v1.Encryption encryptions = 24;
*/
com.google.cloud.video.livestream.v1.Encryption getEncryptions(int index);
/**
*
*
*
* Encryption configurations for this channel. Each configuration has an ID
* which is referred to by each MuxStream to indicate which configuration is
* used for that output.
*
*
* repeated .google.cloud.video.livestream.v1.Encryption encryptions = 24;
*/
int getEncryptionsCount();
/**
*
*
*
* Encryption configurations for this channel. Each configuration has an ID
* which is referred to by each MuxStream to indicate which configuration is
* used for that output.
*
*
* repeated .google.cloud.video.livestream.v1.Encryption encryptions = 24;
*/
java.util.List extends com.google.cloud.video.livestream.v1.EncryptionOrBuilder>
getEncryptionsOrBuilderList();
/**
*
*
*
* Encryption configurations for this channel. Each configuration has an ID
* which is referred to by each MuxStream to indicate which configuration is
* used for that output.
*
*
* repeated .google.cloud.video.livestream.v1.Encryption encryptions = 24;
*/
com.google.cloud.video.livestream.v1.EncryptionOrBuilder getEncryptionsOrBuilder(int index);
/**
*
*
*
* The configuration for input sources defined in
* [input_attachments][google.cloud.video.livestream.v1.Channel.input_attachments].
*
*
* .google.cloud.video.livestream.v1.InputConfig input_config = 25;
*
* @return Whether the inputConfig field is set.
*/
boolean hasInputConfig();
/**
*
*
*
* The configuration for input sources defined in
* [input_attachments][google.cloud.video.livestream.v1.Channel.input_attachments].
*
*
* .google.cloud.video.livestream.v1.InputConfig input_config = 25;
*
* @return The inputConfig.
*/
com.google.cloud.video.livestream.v1.InputConfig getInputConfig();
/**
*
*
*
* The configuration for input sources defined in
* [input_attachments][google.cloud.video.livestream.v1.Channel.input_attachments].
*
*
* .google.cloud.video.livestream.v1.InputConfig input_config = 25;
*/
com.google.cloud.video.livestream.v1.InputConfigOrBuilder getInputConfigOrBuilder();
/**
*
*
*
* Optional. Configuration for retention of output files for this channel.
*
*
*
* .google.cloud.video.livestream.v1.RetentionConfig retention_config = 26 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the retentionConfig field is set.
*/
boolean hasRetentionConfig();
/**
*
*
*
* Optional. Configuration for retention of output files for this channel.
*
*
*
* .google.cloud.video.livestream.v1.RetentionConfig retention_config = 26 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The retentionConfig.
*/
com.google.cloud.video.livestream.v1.RetentionConfig getRetentionConfig();
/**
*
*
*
* Optional. Configuration for retention of output files for this channel.
*
*
*
* .google.cloud.video.livestream.v1.RetentionConfig retention_config = 26 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.video.livestream.v1.RetentionConfigOrBuilder getRetentionConfigOrBuilder();
/**
*
*
*
* Optional. List of static overlay images. Those images display over the
* output content for the whole duration of the live stream.
*
*
*
* repeated .google.cloud.video.livestream.v1.StaticOverlay static_overlays = 27 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List getStaticOverlaysList();
/**
*
*
*
* Optional. List of static overlay images. Those images display over the
* output content for the whole duration of the live stream.
*
*
*
* repeated .google.cloud.video.livestream.v1.StaticOverlay static_overlays = 27 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.video.livestream.v1.StaticOverlay getStaticOverlays(int index);
/**
*
*
*
* Optional. List of static overlay images. Those images display over the
* output content for the whole duration of the live stream.
*
*
*
* repeated .google.cloud.video.livestream.v1.StaticOverlay static_overlays = 27 [(.google.api.field_behavior) = OPTIONAL];
*
*/
int getStaticOverlaysCount();
/**
*
*
*
* Optional. List of static overlay images. Those images display over the
* output content for the whole duration of the live stream.
*
*
*
* repeated .google.cloud.video.livestream.v1.StaticOverlay static_overlays = 27 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List extends com.google.cloud.video.livestream.v1.StaticOverlayOrBuilder>
getStaticOverlaysOrBuilderList();
/**
*
*
*
* Optional. List of static overlay images. Those images display over the
* output content for the whole duration of the live stream.
*
*
*
* repeated .google.cloud.video.livestream.v1.StaticOverlay static_overlays = 27 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.video.livestream.v1.StaticOverlayOrBuilder getStaticOverlaysOrBuilder(int index);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy