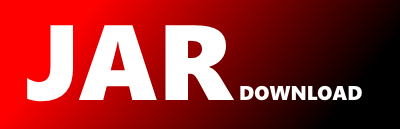
com.google.cloud.video.livestream.v1.VideoStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-live-stream-v1 Show documentation
Show all versions of proto-google-cloud-live-stream-v1 Show documentation
Proto library for google-cloud-live-stream
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/video/livestream/v1/outputs.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.video.livestream.v1;
/**
*
*
*
* Video stream resource.
*
*
* Protobuf type {@code google.cloud.video.livestream.v1.VideoStream}
*/
public final class VideoStream extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.video.livestream.v1.VideoStream)
VideoStreamOrBuilder {
private static final long serialVersionUID = 0L;
// Use VideoStream.newBuilder() to construct.
private VideoStream(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private VideoStream() {}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new VideoStream();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.video.livestream.v1.OutputsProto
.internal_static_google_cloud_video_livestream_v1_VideoStream_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.video.livestream.v1.OutputsProto
.internal_static_google_cloud_video_livestream_v1_VideoStream_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.video.livestream.v1.VideoStream.class,
com.google.cloud.video.livestream.v1.VideoStream.Builder.class);
}
public interface H264CodecSettingsOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.video.livestream.v1.VideoStream.H264CodecSettings)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Required. The width of the video in pixels. Must be an even integer.
* Valid range is [320, 1920].
*
*
* int32 width_pixels = 1;
*
* @return The widthPixels.
*/
int getWidthPixels();
/**
*
*
*
* Required. The height of the video in pixels. Must be an even integer.
* Valid range is [180, 1080].
*
*
* int32 height_pixels = 2;
*
* @return The heightPixels.
*/
int getHeightPixels();
/**
*
*
*
* Required. The target video frame rate in frames per second (FPS). Must be
* less than or equal to 60. Will default to the input frame rate if larger
* than the input frame rate. The API will generate an output FPS that is
* divisible by the input FPS, and smaller or equal to the target FPS. See
* [Calculating frame
* rate](https://cloud.google.com/transcoder/docs/concepts/frame-rate) for
* more information.
*
*
* double frame_rate = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @return The frameRate.
*/
double getFrameRate();
/**
*
*
*
* Required. The video bitrate in bits per second. Minimum value is 10,000.
*
* - For SD resolution (< 720p), must be <= 3,000,000 (3 Mbps).
* - For HD resolution (<= 1080p), must be <= 15,000,000 (15 Mbps).
*
*
* int32 bitrate_bps = 4 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bitrateBps.
*/
int getBitrateBps();
/**
*
*
*
* Specifies whether an open Group of Pictures (GOP) structure should be
* allowed or not. The default is `false`.
*
*
* bool allow_open_gop = 6;
*
* @return The allowOpenGop.
*/
boolean getAllowOpenGop();
/**
*
*
*
* Select the GOP size based on the specified frame count.
* If GOP frame count is set instead of GOP duration, GOP duration will be
* calculated by `gopFrameCount`/`frameRate`. The calculated GOP duration
* must satisfy the limitations on `gopDuration` as well.
* Valid range is [60, 600].
*
*
* int32 gop_frame_count = 7;
*
* @return Whether the gopFrameCount field is set.
*/
boolean hasGopFrameCount();
/**
*
*
*
* Select the GOP size based on the specified frame count.
* If GOP frame count is set instead of GOP duration, GOP duration will be
* calculated by `gopFrameCount`/`frameRate`. The calculated GOP duration
* must satisfy the limitations on `gopDuration` as well.
* Valid range is [60, 600].
*
*
* int32 gop_frame_count = 7;
*
* @return The gopFrameCount.
*/
int getGopFrameCount();
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*
* @return Whether the gopDuration field is set.
*/
boolean hasGopDuration();
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*
* @return The gopDuration.
*/
com.google.protobuf.Duration getGopDuration();
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*/
com.google.protobuf.DurationOrBuilder getGopDurationOrBuilder();
/**
*
*
*
* Size of the Video Buffering Verifier (VBV) buffer in bits. Must be
* greater than zero. The default is equal to
* [bitrate_bps][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.bitrate_bps].
*
*
* int32 vbv_size_bits = 9;
*
* @return The vbvSizeBits.
*/
int getVbvSizeBits();
/**
*
*
*
* Initial fullness of the Video Buffering Verifier (VBV) buffer in bits.
* Must be greater than zero. The default is equal to 90% of
* [vbv_size_bits][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.vbv_size_bits].
*
*
* int32 vbv_fullness_bits = 10;
*
* @return The vbvFullnessBits.
*/
int getVbvFullnessBits();
/**
*
*
*
* The entropy coder to use. The default is `cabac`.
*
* Supported entropy coders:
*
* - `cavlc`
* - `cabac`
*
*
* string entropy_coder = 11;
*
* @return The entropyCoder.
*/
java.lang.String getEntropyCoder();
/**
*
*
*
* The entropy coder to use. The default is `cabac`.
*
* Supported entropy coders:
*
* - `cavlc`
* - `cabac`
*
*
* string entropy_coder = 11;
*
* @return The bytes for entropyCoder.
*/
com.google.protobuf.ByteString getEntropyCoderBytes();
/**
*
*
*
* Allow B-pyramid for reference frame selection. This may not be supported
* on all decoders. The default is `false`.
*
*
* bool b_pyramid = 12;
*
* @return The bPyramid.
*/
boolean getBPyramid();
/**
*
*
*
* The number of consecutive B-frames. Must be greater than or equal to
* zero. Must be less than
* [gop_frame_count][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.gop_frame_count]
* if set. The default is 0.
*
*
* int32 b_frame_count = 13;
*
* @return The bFrameCount.
*/
int getBFrameCount();
/**
*
*
*
* Specify the intensity of the adaptive quantizer (AQ). Must be between 0
* and 1, where 0 disables the quantizer and 1 maximizes the quantizer. A
* higher value equals a lower bitrate but smoother image. The default is 0.
*
*
* double aq_strength = 14;
*
* @return The aqStrength.
*/
double getAqStrength();
/**
*
*
*
* Enforces the specified codec profile. The following profiles are
* supported:
*
* * `baseline`
* * `main` (default)
* * `high`
*
* The available options are [FFmpeg-compatible Profile
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Profile).
* Note that certain values for this field may cause the
* transcoder to override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string profile = 15;
*
* @return The profile.
*/
java.lang.String getProfile();
/**
*
*
*
* Enforces the specified codec profile. The following profiles are
* supported:
*
* * `baseline`
* * `main` (default)
* * `high`
*
* The available options are [FFmpeg-compatible Profile
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Profile).
* Note that certain values for this field may cause the
* transcoder to override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string profile = 15;
*
* @return The bytes for profile.
*/
com.google.protobuf.ByteString getProfileBytes();
/**
*
*
*
* Enforces the specified codec tune. The available options are
* [FFmpeg-compatible Encode
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Tune)
* Note that certain values for this field may cause the transcoder to
* override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string tune = 16;
*
* @return The tune.
*/
java.lang.String getTune();
/**
*
*
*
* Enforces the specified codec tune. The available options are
* [FFmpeg-compatible Encode
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Tune)
* Note that certain values for this field may cause the transcoder to
* override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string tune = 16;
*
* @return The bytes for tune.
*/
com.google.protobuf.ByteString getTuneBytes();
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.GopModeCase getGopModeCase();
}
/**
*
*
*
* H264 codec settings.
*
*
* Protobuf type {@code google.cloud.video.livestream.v1.VideoStream.H264CodecSettings}
*/
public static final class H264CodecSettings extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.video.livestream.v1.VideoStream.H264CodecSettings)
H264CodecSettingsOrBuilder {
private static final long serialVersionUID = 0L;
// Use H264CodecSettings.newBuilder() to construct.
private H264CodecSettings(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private H264CodecSettings() {
entropyCoder_ = "";
profile_ = "";
tune_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new H264CodecSettings();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.video.livestream.v1.OutputsProto
.internal_static_google_cloud_video_livestream_v1_VideoStream_H264CodecSettings_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.video.livestream.v1.OutputsProto
.internal_static_google_cloud_video_livestream_v1_VideoStream_H264CodecSettings_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.class,
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.Builder.class);
}
private int gopModeCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object gopMode_;
public enum GopModeCase
implements
com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
GOP_FRAME_COUNT(7),
GOP_DURATION(8),
GOPMODE_NOT_SET(0);
private final int value;
private GopModeCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static GopModeCase valueOf(int value) {
return forNumber(value);
}
public static GopModeCase forNumber(int value) {
switch (value) {
case 7:
return GOP_FRAME_COUNT;
case 8:
return GOP_DURATION;
case 0:
return GOPMODE_NOT_SET;
default:
return null;
}
}
public int getNumber() {
return this.value;
}
};
public GopModeCase getGopModeCase() {
return GopModeCase.forNumber(gopModeCase_);
}
public static final int WIDTH_PIXELS_FIELD_NUMBER = 1;
private int widthPixels_ = 0;
/**
*
*
*
* Required. The width of the video in pixels. Must be an even integer.
* Valid range is [320, 1920].
*
*
* int32 width_pixels = 1;
*
* @return The widthPixels.
*/
@java.lang.Override
public int getWidthPixels() {
return widthPixels_;
}
public static final int HEIGHT_PIXELS_FIELD_NUMBER = 2;
private int heightPixels_ = 0;
/**
*
*
*
* Required. The height of the video in pixels. Must be an even integer.
* Valid range is [180, 1080].
*
*
* int32 height_pixels = 2;
*
* @return The heightPixels.
*/
@java.lang.Override
public int getHeightPixels() {
return heightPixels_;
}
public static final int FRAME_RATE_FIELD_NUMBER = 3;
private double frameRate_ = 0D;
/**
*
*
*
* Required. The target video frame rate in frames per second (FPS). Must be
* less than or equal to 60. Will default to the input frame rate if larger
* than the input frame rate. The API will generate an output FPS that is
* divisible by the input FPS, and smaller or equal to the target FPS. See
* [Calculating frame
* rate](https://cloud.google.com/transcoder/docs/concepts/frame-rate) for
* more information.
*
*
* double frame_rate = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @return The frameRate.
*/
@java.lang.Override
public double getFrameRate() {
return frameRate_;
}
public static final int BITRATE_BPS_FIELD_NUMBER = 4;
private int bitrateBps_ = 0;
/**
*
*
*
* Required. The video bitrate in bits per second. Minimum value is 10,000.
*
* - For SD resolution (< 720p), must be <= 3,000,000 (3 Mbps).
* - For HD resolution (<= 1080p), must be <= 15,000,000 (15 Mbps).
*
*
* int32 bitrate_bps = 4 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bitrateBps.
*/
@java.lang.Override
public int getBitrateBps() {
return bitrateBps_;
}
public static final int ALLOW_OPEN_GOP_FIELD_NUMBER = 6;
private boolean allowOpenGop_ = false;
/**
*
*
*
* Specifies whether an open Group of Pictures (GOP) structure should be
* allowed or not. The default is `false`.
*
*
* bool allow_open_gop = 6;
*
* @return The allowOpenGop.
*/
@java.lang.Override
public boolean getAllowOpenGop() {
return allowOpenGop_;
}
public static final int GOP_FRAME_COUNT_FIELD_NUMBER = 7;
/**
*
*
*
* Select the GOP size based on the specified frame count.
* If GOP frame count is set instead of GOP duration, GOP duration will be
* calculated by `gopFrameCount`/`frameRate`. The calculated GOP duration
* must satisfy the limitations on `gopDuration` as well.
* Valid range is [60, 600].
*
*
* int32 gop_frame_count = 7;
*
* @return Whether the gopFrameCount field is set.
*/
@java.lang.Override
public boolean hasGopFrameCount() {
return gopModeCase_ == 7;
}
/**
*
*
*
* Select the GOP size based on the specified frame count.
* If GOP frame count is set instead of GOP duration, GOP duration will be
* calculated by `gopFrameCount`/`frameRate`. The calculated GOP duration
* must satisfy the limitations on `gopDuration` as well.
* Valid range is [60, 600].
*
*
* int32 gop_frame_count = 7;
*
* @return The gopFrameCount.
*/
@java.lang.Override
public int getGopFrameCount() {
if (gopModeCase_ == 7) {
return (java.lang.Integer) gopMode_;
}
return 0;
}
public static final int GOP_DURATION_FIELD_NUMBER = 8;
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*
* @return Whether the gopDuration field is set.
*/
@java.lang.Override
public boolean hasGopDuration() {
return gopModeCase_ == 8;
}
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*
* @return The gopDuration.
*/
@java.lang.Override
public com.google.protobuf.Duration getGopDuration() {
if (gopModeCase_ == 8) {
return (com.google.protobuf.Duration) gopMode_;
}
return com.google.protobuf.Duration.getDefaultInstance();
}
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getGopDurationOrBuilder() {
if (gopModeCase_ == 8) {
return (com.google.protobuf.Duration) gopMode_;
}
return com.google.protobuf.Duration.getDefaultInstance();
}
public static final int VBV_SIZE_BITS_FIELD_NUMBER = 9;
private int vbvSizeBits_ = 0;
/**
*
*
*
* Size of the Video Buffering Verifier (VBV) buffer in bits. Must be
* greater than zero. The default is equal to
* [bitrate_bps][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.bitrate_bps].
*
*
* int32 vbv_size_bits = 9;
*
* @return The vbvSizeBits.
*/
@java.lang.Override
public int getVbvSizeBits() {
return vbvSizeBits_;
}
public static final int VBV_FULLNESS_BITS_FIELD_NUMBER = 10;
private int vbvFullnessBits_ = 0;
/**
*
*
*
* Initial fullness of the Video Buffering Verifier (VBV) buffer in bits.
* Must be greater than zero. The default is equal to 90% of
* [vbv_size_bits][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.vbv_size_bits].
*
*
* int32 vbv_fullness_bits = 10;
*
* @return The vbvFullnessBits.
*/
@java.lang.Override
public int getVbvFullnessBits() {
return vbvFullnessBits_;
}
public static final int ENTROPY_CODER_FIELD_NUMBER = 11;
@SuppressWarnings("serial")
private volatile java.lang.Object entropyCoder_ = "";
/**
*
*
*
* The entropy coder to use. The default is `cabac`.
*
* Supported entropy coders:
*
* - `cavlc`
* - `cabac`
*
*
* string entropy_coder = 11;
*
* @return The entropyCoder.
*/
@java.lang.Override
public java.lang.String getEntropyCoder() {
java.lang.Object ref = entropyCoder_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
entropyCoder_ = s;
return s;
}
}
/**
*
*
*
* The entropy coder to use. The default is `cabac`.
*
* Supported entropy coders:
*
* - `cavlc`
* - `cabac`
*
*
* string entropy_coder = 11;
*
* @return The bytes for entropyCoder.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEntropyCoderBytes() {
java.lang.Object ref = entropyCoder_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
entropyCoder_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int B_PYRAMID_FIELD_NUMBER = 12;
private boolean bPyramid_ = false;
/**
*
*
*
* Allow B-pyramid for reference frame selection. This may not be supported
* on all decoders. The default is `false`.
*
*
* bool b_pyramid = 12;
*
* @return The bPyramid.
*/
@java.lang.Override
public boolean getBPyramid() {
return bPyramid_;
}
public static final int B_FRAME_COUNT_FIELD_NUMBER = 13;
private int bFrameCount_ = 0;
/**
*
*
*
* The number of consecutive B-frames. Must be greater than or equal to
* zero. Must be less than
* [gop_frame_count][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.gop_frame_count]
* if set. The default is 0.
*
*
* int32 b_frame_count = 13;
*
* @return The bFrameCount.
*/
@java.lang.Override
public int getBFrameCount() {
return bFrameCount_;
}
public static final int AQ_STRENGTH_FIELD_NUMBER = 14;
private double aqStrength_ = 0D;
/**
*
*
*
* Specify the intensity of the adaptive quantizer (AQ). Must be between 0
* and 1, where 0 disables the quantizer and 1 maximizes the quantizer. A
* higher value equals a lower bitrate but smoother image. The default is 0.
*
*
* double aq_strength = 14;
*
* @return The aqStrength.
*/
@java.lang.Override
public double getAqStrength() {
return aqStrength_;
}
public static final int PROFILE_FIELD_NUMBER = 15;
@SuppressWarnings("serial")
private volatile java.lang.Object profile_ = "";
/**
*
*
*
* Enforces the specified codec profile. The following profiles are
* supported:
*
* * `baseline`
* * `main` (default)
* * `high`
*
* The available options are [FFmpeg-compatible Profile
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Profile).
* Note that certain values for this field may cause the
* transcoder to override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string profile = 15;
*
* @return The profile.
*/
@java.lang.Override
public java.lang.String getProfile() {
java.lang.Object ref = profile_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
profile_ = s;
return s;
}
}
/**
*
*
*
* Enforces the specified codec profile. The following profiles are
* supported:
*
* * `baseline`
* * `main` (default)
* * `high`
*
* The available options are [FFmpeg-compatible Profile
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Profile).
* Note that certain values for this field may cause the
* transcoder to override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string profile = 15;
*
* @return The bytes for profile.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProfileBytes() {
java.lang.Object ref = profile_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
profile_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TUNE_FIELD_NUMBER = 16;
@SuppressWarnings("serial")
private volatile java.lang.Object tune_ = "";
/**
*
*
*
* Enforces the specified codec tune. The available options are
* [FFmpeg-compatible Encode
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Tune)
* Note that certain values for this field may cause the transcoder to
* override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string tune = 16;
*
* @return The tune.
*/
@java.lang.Override
public java.lang.String getTune() {
java.lang.Object ref = tune_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tune_ = s;
return s;
}
}
/**
*
*
*
* Enforces the specified codec tune. The available options are
* [FFmpeg-compatible Encode
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Tune)
* Note that certain values for this field may cause the transcoder to
* override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string tune = 16;
*
* @return The bytes for tune.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTuneBytes() {
java.lang.Object ref = tune_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
tune_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (widthPixels_ != 0) {
output.writeInt32(1, widthPixels_);
}
if (heightPixels_ != 0) {
output.writeInt32(2, heightPixels_);
}
if (java.lang.Double.doubleToRawLongBits(frameRate_) != 0) {
output.writeDouble(3, frameRate_);
}
if (bitrateBps_ != 0) {
output.writeInt32(4, bitrateBps_);
}
if (allowOpenGop_ != false) {
output.writeBool(6, allowOpenGop_);
}
if (gopModeCase_ == 7) {
output.writeInt32(7, (int) ((java.lang.Integer) gopMode_));
}
if (gopModeCase_ == 8) {
output.writeMessage(8, (com.google.protobuf.Duration) gopMode_);
}
if (vbvSizeBits_ != 0) {
output.writeInt32(9, vbvSizeBits_);
}
if (vbvFullnessBits_ != 0) {
output.writeInt32(10, vbvFullnessBits_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(entropyCoder_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, entropyCoder_);
}
if (bPyramid_ != false) {
output.writeBool(12, bPyramid_);
}
if (bFrameCount_ != 0) {
output.writeInt32(13, bFrameCount_);
}
if (java.lang.Double.doubleToRawLongBits(aqStrength_) != 0) {
output.writeDouble(14, aqStrength_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(profile_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, profile_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tune_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, tune_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (widthPixels_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(1, widthPixels_);
}
if (heightPixels_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(2, heightPixels_);
}
if (java.lang.Double.doubleToRawLongBits(frameRate_) != 0) {
size += com.google.protobuf.CodedOutputStream.computeDoubleSize(3, frameRate_);
}
if (bitrateBps_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(4, bitrateBps_);
}
if (allowOpenGop_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(6, allowOpenGop_);
}
if (gopModeCase_ == 7) {
size +=
com.google.protobuf.CodedOutputStream.computeInt32Size(
7, (int) ((java.lang.Integer) gopMode_));
}
if (gopModeCase_ == 8) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
8, (com.google.protobuf.Duration) gopMode_);
}
if (vbvSizeBits_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(9, vbvSizeBits_);
}
if (vbvFullnessBits_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(10, vbvFullnessBits_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(entropyCoder_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, entropyCoder_);
}
if (bPyramid_ != false) {
size += com.google.protobuf.CodedOutputStream.computeBoolSize(12, bPyramid_);
}
if (bFrameCount_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(13, bFrameCount_);
}
if (java.lang.Double.doubleToRawLongBits(aqStrength_) != 0) {
size += com.google.protobuf.CodedOutputStream.computeDoubleSize(14, aqStrength_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(profile_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, profile_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(tune_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, tune_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings)) {
return super.equals(obj);
}
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings other =
(com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings) obj;
if (getWidthPixels() != other.getWidthPixels()) return false;
if (getHeightPixels() != other.getHeightPixels()) return false;
if (java.lang.Double.doubleToLongBits(getFrameRate())
!= java.lang.Double.doubleToLongBits(other.getFrameRate())) return false;
if (getBitrateBps() != other.getBitrateBps()) return false;
if (getAllowOpenGop() != other.getAllowOpenGop()) return false;
if (getVbvSizeBits() != other.getVbvSizeBits()) return false;
if (getVbvFullnessBits() != other.getVbvFullnessBits()) return false;
if (!getEntropyCoder().equals(other.getEntropyCoder())) return false;
if (getBPyramid() != other.getBPyramid()) return false;
if (getBFrameCount() != other.getBFrameCount()) return false;
if (java.lang.Double.doubleToLongBits(getAqStrength())
!= java.lang.Double.doubleToLongBits(other.getAqStrength())) return false;
if (!getProfile().equals(other.getProfile())) return false;
if (!getTune().equals(other.getTune())) return false;
if (!getGopModeCase().equals(other.getGopModeCase())) return false;
switch (gopModeCase_) {
case 7:
if (getGopFrameCount() != other.getGopFrameCount()) return false;
break;
case 8:
if (!getGopDuration().equals(other.getGopDuration())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + WIDTH_PIXELS_FIELD_NUMBER;
hash = (53 * hash) + getWidthPixels();
hash = (37 * hash) + HEIGHT_PIXELS_FIELD_NUMBER;
hash = (53 * hash) + getHeightPixels();
hash = (37 * hash) + FRAME_RATE_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getFrameRate()));
hash = (37 * hash) + BITRATE_BPS_FIELD_NUMBER;
hash = (53 * hash) + getBitrateBps();
hash = (37 * hash) + ALLOW_OPEN_GOP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getAllowOpenGop());
hash = (37 * hash) + VBV_SIZE_BITS_FIELD_NUMBER;
hash = (53 * hash) + getVbvSizeBits();
hash = (37 * hash) + VBV_FULLNESS_BITS_FIELD_NUMBER;
hash = (53 * hash) + getVbvFullnessBits();
hash = (37 * hash) + ENTROPY_CODER_FIELD_NUMBER;
hash = (53 * hash) + getEntropyCoder().hashCode();
hash = (37 * hash) + B_PYRAMID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(getBPyramid());
hash = (37 * hash) + B_FRAME_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getBFrameCount();
hash = (37 * hash) + AQ_STRENGTH_FIELD_NUMBER;
hash =
(53 * hash)
+ com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getAqStrength()));
hash = (37 * hash) + PROFILE_FIELD_NUMBER;
hash = (53 * hash) + getProfile().hashCode();
hash = (37 * hash) + TUNE_FIELD_NUMBER;
hash = (53 * hash) + getTune().hashCode();
switch (gopModeCase_) {
case 7:
hash = (37 * hash) + GOP_FRAME_COUNT_FIELD_NUMBER;
hash = (53 * hash) + getGopFrameCount();
break;
case 8:
hash = (37 * hash) + GOP_DURATION_FIELD_NUMBER;
hash = (53 * hash) + getGopDuration().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings parseFrom(
byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
parseDelimitedFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* H264 codec settings.
*
*
* Protobuf type {@code google.cloud.video.livestream.v1.VideoStream.H264CodecSettings}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.video.livestream.v1.VideoStream.H264CodecSettings)
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettingsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.video.livestream.v1.OutputsProto
.internal_static_google_cloud_video_livestream_v1_VideoStream_H264CodecSettings_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.video.livestream.v1.OutputsProto
.internal_static_google_cloud_video_livestream_v1_VideoStream_H264CodecSettings_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.class,
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.Builder.class);
}
// Construct using
// com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
widthPixels_ = 0;
heightPixels_ = 0;
frameRate_ = 0D;
bitrateBps_ = 0;
allowOpenGop_ = false;
if (gopDurationBuilder_ != null) {
gopDurationBuilder_.clear();
}
vbvSizeBits_ = 0;
vbvFullnessBits_ = 0;
entropyCoder_ = "";
bPyramid_ = false;
bFrameCount_ = 0;
aqStrength_ = 0D;
profile_ = "";
tune_ = "";
gopModeCase_ = 0;
gopMode_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.video.livestream.v1.OutputsProto
.internal_static_google_cloud_video_livestream_v1_VideoStream_H264CodecSettings_descriptor;
}
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
getDefaultInstanceForType() {
return com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings build() {
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings buildPartial() {
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings result =
new com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.widthPixels_ = widthPixels_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.heightPixels_ = heightPixels_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.frameRate_ = frameRate_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.bitrateBps_ = bitrateBps_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.allowOpenGop_ = allowOpenGop_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.vbvSizeBits_ = vbvSizeBits_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.vbvFullnessBits_ = vbvFullnessBits_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.entropyCoder_ = entropyCoder_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.bPyramid_ = bPyramid_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.bFrameCount_ = bFrameCount_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.aqStrength_ = aqStrength_;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.profile_ = profile_;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.tune_ = tune_;
}
}
private void buildPartialOneofs(
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings result) {
result.gopModeCase_ = gopModeCase_;
result.gopMode_ = this.gopMode_;
if (gopModeCase_ == 8 && gopDurationBuilder_ != null) {
result.gopMode_ = gopDurationBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings) {
return mergeFrom(
(com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings other) {
if (other
== com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
.getDefaultInstance()) return this;
if (other.getWidthPixels() != 0) {
setWidthPixels(other.getWidthPixels());
}
if (other.getHeightPixels() != 0) {
setHeightPixels(other.getHeightPixels());
}
if (other.getFrameRate() != 0D) {
setFrameRate(other.getFrameRate());
}
if (other.getBitrateBps() != 0) {
setBitrateBps(other.getBitrateBps());
}
if (other.getAllowOpenGop() != false) {
setAllowOpenGop(other.getAllowOpenGop());
}
if (other.getVbvSizeBits() != 0) {
setVbvSizeBits(other.getVbvSizeBits());
}
if (other.getVbvFullnessBits() != 0) {
setVbvFullnessBits(other.getVbvFullnessBits());
}
if (!other.getEntropyCoder().isEmpty()) {
entropyCoder_ = other.entropyCoder_;
bitField0_ |= 0x00000200;
onChanged();
}
if (other.getBPyramid() != false) {
setBPyramid(other.getBPyramid());
}
if (other.getBFrameCount() != 0) {
setBFrameCount(other.getBFrameCount());
}
if (other.getAqStrength() != 0D) {
setAqStrength(other.getAqStrength());
}
if (!other.getProfile().isEmpty()) {
profile_ = other.profile_;
bitField0_ |= 0x00002000;
onChanged();
}
if (!other.getTune().isEmpty()) {
tune_ = other.tune_;
bitField0_ |= 0x00004000;
onChanged();
}
switch (other.getGopModeCase()) {
case GOP_FRAME_COUNT:
{
setGopFrameCount(other.getGopFrameCount());
break;
}
case GOP_DURATION:
{
mergeGopDuration(other.getGopDuration());
break;
}
case GOPMODE_NOT_SET:
{
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8:
{
widthPixels_ = input.readInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16:
{
heightPixels_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 25:
{
frameRate_ = input.readDouble();
bitField0_ |= 0x00000004;
break;
} // case 25
case 32:
{
bitrateBps_ = input.readInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
case 48:
{
allowOpenGop_ = input.readBool();
bitField0_ |= 0x00000010;
break;
} // case 48
case 56:
{
gopMode_ = input.readInt32();
gopModeCase_ = 7;
break;
} // case 56
case 66:
{
input.readMessage(getGopDurationFieldBuilder().getBuilder(), extensionRegistry);
gopModeCase_ = 8;
break;
} // case 66
case 72:
{
vbvSizeBits_ = input.readInt32();
bitField0_ |= 0x00000080;
break;
} // case 72
case 80:
{
vbvFullnessBits_ = input.readInt32();
bitField0_ |= 0x00000100;
break;
} // case 80
case 90:
{
entropyCoder_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case 90
case 96:
{
bPyramid_ = input.readBool();
bitField0_ |= 0x00000400;
break;
} // case 96
case 104:
{
bFrameCount_ = input.readInt32();
bitField0_ |= 0x00000800;
break;
} // case 104
case 113:
{
aqStrength_ = input.readDouble();
bitField0_ |= 0x00001000;
break;
} // case 113
case 122:
{
profile_ = input.readStringRequireUtf8();
bitField0_ |= 0x00002000;
break;
} // case 122
case 130:
{
tune_ = input.readStringRequireUtf8();
bitField0_ |= 0x00004000;
break;
} // case 130
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int gopModeCase_ = 0;
private java.lang.Object gopMode_;
public GopModeCase getGopModeCase() {
return GopModeCase.forNumber(gopModeCase_);
}
public Builder clearGopMode() {
gopModeCase_ = 0;
gopMode_ = null;
onChanged();
return this;
}
private int bitField0_;
private int widthPixels_;
/**
*
*
*
* Required. The width of the video in pixels. Must be an even integer.
* Valid range is [320, 1920].
*
*
* int32 width_pixels = 1;
*
* @return The widthPixels.
*/
@java.lang.Override
public int getWidthPixels() {
return widthPixels_;
}
/**
*
*
*
* Required. The width of the video in pixels. Must be an even integer.
* Valid range is [320, 1920].
*
*
* int32 width_pixels = 1;
*
* @param value The widthPixels to set.
* @return This builder for chaining.
*/
public Builder setWidthPixels(int value) {
widthPixels_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Required. The width of the video in pixels. Must be an even integer.
* Valid range is [320, 1920].
*
*
* int32 width_pixels = 1;
*
* @return This builder for chaining.
*/
public Builder clearWidthPixels() {
bitField0_ = (bitField0_ & ~0x00000001);
widthPixels_ = 0;
onChanged();
return this;
}
private int heightPixels_;
/**
*
*
*
* Required. The height of the video in pixels. Must be an even integer.
* Valid range is [180, 1080].
*
*
* int32 height_pixels = 2;
*
* @return The heightPixels.
*/
@java.lang.Override
public int getHeightPixels() {
return heightPixels_;
}
/**
*
*
*
* Required. The height of the video in pixels. Must be an even integer.
* Valid range is [180, 1080].
*
*
* int32 height_pixels = 2;
*
* @param value The heightPixels to set.
* @return This builder for chaining.
*/
public Builder setHeightPixels(int value) {
heightPixels_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Required. The height of the video in pixels. Must be an even integer.
* Valid range is [180, 1080].
*
*
* int32 height_pixels = 2;
*
* @return This builder for chaining.
*/
public Builder clearHeightPixels() {
bitField0_ = (bitField0_ & ~0x00000002);
heightPixels_ = 0;
onChanged();
return this;
}
private double frameRate_;
/**
*
*
*
* Required. The target video frame rate in frames per second (FPS). Must be
* less than or equal to 60. Will default to the input frame rate if larger
* than the input frame rate. The API will generate an output FPS that is
* divisible by the input FPS, and smaller or equal to the target FPS. See
* [Calculating frame
* rate](https://cloud.google.com/transcoder/docs/concepts/frame-rate) for
* more information.
*
*
* double frame_rate = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @return The frameRate.
*/
@java.lang.Override
public double getFrameRate() {
return frameRate_;
}
/**
*
*
*
* Required. The target video frame rate in frames per second (FPS). Must be
* less than or equal to 60. Will default to the input frame rate if larger
* than the input frame rate. The API will generate an output FPS that is
* divisible by the input FPS, and smaller or equal to the target FPS. See
* [Calculating frame
* rate](https://cloud.google.com/transcoder/docs/concepts/frame-rate) for
* more information.
*
*
* double frame_rate = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @param value The frameRate to set.
* @return This builder for chaining.
*/
public Builder setFrameRate(double value) {
frameRate_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Required. The target video frame rate in frames per second (FPS). Must be
* less than or equal to 60. Will default to the input frame rate if larger
* than the input frame rate. The API will generate an output FPS that is
* divisible by the input FPS, and smaller or equal to the target FPS. See
* [Calculating frame
* rate](https://cloud.google.com/transcoder/docs/concepts/frame-rate) for
* more information.
*
*
* double frame_rate = 3 [(.google.api.field_behavior) = REQUIRED];
*
* @return This builder for chaining.
*/
public Builder clearFrameRate() {
bitField0_ = (bitField0_ & ~0x00000004);
frameRate_ = 0D;
onChanged();
return this;
}
private int bitrateBps_;
/**
*
*
*
* Required. The video bitrate in bits per second. Minimum value is 10,000.
*
* - For SD resolution (< 720p), must be <= 3,000,000 (3 Mbps).
* - For HD resolution (<= 1080p), must be <= 15,000,000 (15 Mbps).
*
*
* int32 bitrate_bps = 4 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bitrateBps.
*/
@java.lang.Override
public int getBitrateBps() {
return bitrateBps_;
}
/**
*
*
*
* Required. The video bitrate in bits per second. Minimum value is 10,000.
*
* - For SD resolution (< 720p), must be <= 3,000,000 (3 Mbps).
* - For HD resolution (<= 1080p), must be <= 15,000,000 (15 Mbps).
*
*
* int32 bitrate_bps = 4 [(.google.api.field_behavior) = REQUIRED];
*
* @param value The bitrateBps to set.
* @return This builder for chaining.
*/
public Builder setBitrateBps(int value) {
bitrateBps_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Required. The video bitrate in bits per second. Minimum value is 10,000.
*
* - For SD resolution (< 720p), must be <= 3,000,000 (3 Mbps).
* - For HD resolution (<= 1080p), must be <= 15,000,000 (15 Mbps).
*
*
* int32 bitrate_bps = 4 [(.google.api.field_behavior) = REQUIRED];
*
* @return This builder for chaining.
*/
public Builder clearBitrateBps() {
bitField0_ = (bitField0_ & ~0x00000008);
bitrateBps_ = 0;
onChanged();
return this;
}
private boolean allowOpenGop_;
/**
*
*
*
* Specifies whether an open Group of Pictures (GOP) structure should be
* allowed or not. The default is `false`.
*
*
* bool allow_open_gop = 6;
*
* @return The allowOpenGop.
*/
@java.lang.Override
public boolean getAllowOpenGop() {
return allowOpenGop_;
}
/**
*
*
*
* Specifies whether an open Group of Pictures (GOP) structure should be
* allowed or not. The default is `false`.
*
*
* bool allow_open_gop = 6;
*
* @param value The allowOpenGop to set.
* @return This builder for chaining.
*/
public Builder setAllowOpenGop(boolean value) {
allowOpenGop_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Specifies whether an open Group of Pictures (GOP) structure should be
* allowed or not. The default is `false`.
*
*
* bool allow_open_gop = 6;
*
* @return This builder for chaining.
*/
public Builder clearAllowOpenGop() {
bitField0_ = (bitField0_ & ~0x00000010);
allowOpenGop_ = false;
onChanged();
return this;
}
/**
*
*
*
* Select the GOP size based on the specified frame count.
* If GOP frame count is set instead of GOP duration, GOP duration will be
* calculated by `gopFrameCount`/`frameRate`. The calculated GOP duration
* must satisfy the limitations on `gopDuration` as well.
* Valid range is [60, 600].
*
*
* int32 gop_frame_count = 7;
*
* @return Whether the gopFrameCount field is set.
*/
public boolean hasGopFrameCount() {
return gopModeCase_ == 7;
}
/**
*
*
*
* Select the GOP size based on the specified frame count.
* If GOP frame count is set instead of GOP duration, GOP duration will be
* calculated by `gopFrameCount`/`frameRate`. The calculated GOP duration
* must satisfy the limitations on `gopDuration` as well.
* Valid range is [60, 600].
*
*
* int32 gop_frame_count = 7;
*
* @return The gopFrameCount.
*/
public int getGopFrameCount() {
if (gopModeCase_ == 7) {
return (java.lang.Integer) gopMode_;
}
return 0;
}
/**
*
*
*
* Select the GOP size based on the specified frame count.
* If GOP frame count is set instead of GOP duration, GOP duration will be
* calculated by `gopFrameCount`/`frameRate`. The calculated GOP duration
* must satisfy the limitations on `gopDuration` as well.
* Valid range is [60, 600].
*
*
* int32 gop_frame_count = 7;
*
* @param value The gopFrameCount to set.
* @return This builder for chaining.
*/
public Builder setGopFrameCount(int value) {
gopModeCase_ = 7;
gopMode_ = value;
onChanged();
return this;
}
/**
*
*
*
* Select the GOP size based on the specified frame count.
* If GOP frame count is set instead of GOP duration, GOP duration will be
* calculated by `gopFrameCount`/`frameRate`. The calculated GOP duration
* must satisfy the limitations on `gopDuration` as well.
* Valid range is [60, 600].
*
*
* int32 gop_frame_count = 7;
*
* @return This builder for chaining.
*/
public Builder clearGopFrameCount() {
if (gopModeCase_ == 7) {
gopModeCase_ = 0;
gopMode_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
gopDurationBuilder_;
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*
* @return Whether the gopDuration field is set.
*/
@java.lang.Override
public boolean hasGopDuration() {
return gopModeCase_ == 8;
}
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*
* @return The gopDuration.
*/
@java.lang.Override
public com.google.protobuf.Duration getGopDuration() {
if (gopDurationBuilder_ == null) {
if (gopModeCase_ == 8) {
return (com.google.protobuf.Duration) gopMode_;
}
return com.google.protobuf.Duration.getDefaultInstance();
} else {
if (gopModeCase_ == 8) {
return gopDurationBuilder_.getMessage();
}
return com.google.protobuf.Duration.getDefaultInstance();
}
}
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*/
public Builder setGopDuration(com.google.protobuf.Duration value) {
if (gopDurationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
gopMode_ = value;
onChanged();
} else {
gopDurationBuilder_.setMessage(value);
}
gopModeCase_ = 8;
return this;
}
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*/
public Builder setGopDuration(com.google.protobuf.Duration.Builder builderForValue) {
if (gopDurationBuilder_ == null) {
gopMode_ = builderForValue.build();
onChanged();
} else {
gopDurationBuilder_.setMessage(builderForValue.build());
}
gopModeCase_ = 8;
return this;
}
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*/
public Builder mergeGopDuration(com.google.protobuf.Duration value) {
if (gopDurationBuilder_ == null) {
if (gopModeCase_ == 8 && gopMode_ != com.google.protobuf.Duration.getDefaultInstance()) {
gopMode_ =
com.google.protobuf.Duration.newBuilder((com.google.protobuf.Duration) gopMode_)
.mergeFrom(value)
.buildPartial();
} else {
gopMode_ = value;
}
onChanged();
} else {
if (gopModeCase_ == 8) {
gopDurationBuilder_.mergeFrom(value);
} else {
gopDurationBuilder_.setMessage(value);
}
}
gopModeCase_ = 8;
return this;
}
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*/
public Builder clearGopDuration() {
if (gopDurationBuilder_ == null) {
if (gopModeCase_ == 8) {
gopModeCase_ = 0;
gopMode_ = null;
onChanged();
}
} else {
if (gopModeCase_ == 8) {
gopModeCase_ = 0;
gopMode_ = null;
}
gopDurationBuilder_.clear();
}
return this;
}
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*/
public com.google.protobuf.Duration.Builder getGopDurationBuilder() {
return getGopDurationFieldBuilder().getBuilder();
}
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getGopDurationOrBuilder() {
if ((gopModeCase_ == 8) && (gopDurationBuilder_ != null)) {
return gopDurationBuilder_.getMessageOrBuilder();
} else {
if (gopModeCase_ == 8) {
return (com.google.protobuf.Duration) gopMode_;
}
return com.google.protobuf.Duration.getDefaultInstance();
}
}
/**
*
*
*
* Select the GOP size based on the specified duration. The default is
* `2s`. Note that `gopDuration` must be less than or equal to
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration],
* and
* [segment_duration][google.cloud.video.livestream.v1.SegmentSettings.segment_duration]
* must be divisible by `gopDuration`. Valid range is [2s, 20s].
*
* All video streams in the same channel must have the same GOP size.
*
*
* .google.protobuf.Duration gop_duration = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>
getGopDurationFieldBuilder() {
if (gopDurationBuilder_ == null) {
if (!(gopModeCase_ == 8)) {
gopMode_ = com.google.protobuf.Duration.getDefaultInstance();
}
gopDurationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration,
com.google.protobuf.Duration.Builder,
com.google.protobuf.DurationOrBuilder>(
(com.google.protobuf.Duration) gopMode_, getParentForChildren(), isClean());
gopMode_ = null;
}
gopModeCase_ = 8;
onChanged();
return gopDurationBuilder_;
}
private int vbvSizeBits_;
/**
*
*
*
* Size of the Video Buffering Verifier (VBV) buffer in bits. Must be
* greater than zero. The default is equal to
* [bitrate_bps][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.bitrate_bps].
*
*
* int32 vbv_size_bits = 9;
*
* @return The vbvSizeBits.
*/
@java.lang.Override
public int getVbvSizeBits() {
return vbvSizeBits_;
}
/**
*
*
*
* Size of the Video Buffering Verifier (VBV) buffer in bits. Must be
* greater than zero. The default is equal to
* [bitrate_bps][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.bitrate_bps].
*
*
* int32 vbv_size_bits = 9;
*
* @param value The vbvSizeBits to set.
* @return This builder for chaining.
*/
public Builder setVbvSizeBits(int value) {
vbvSizeBits_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* Size of the Video Buffering Verifier (VBV) buffer in bits. Must be
* greater than zero. The default is equal to
* [bitrate_bps][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.bitrate_bps].
*
*
* int32 vbv_size_bits = 9;
*
* @return This builder for chaining.
*/
public Builder clearVbvSizeBits() {
bitField0_ = (bitField0_ & ~0x00000080);
vbvSizeBits_ = 0;
onChanged();
return this;
}
private int vbvFullnessBits_;
/**
*
*
*
* Initial fullness of the Video Buffering Verifier (VBV) buffer in bits.
* Must be greater than zero. The default is equal to 90% of
* [vbv_size_bits][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.vbv_size_bits].
*
*
* int32 vbv_fullness_bits = 10;
*
* @return The vbvFullnessBits.
*/
@java.lang.Override
public int getVbvFullnessBits() {
return vbvFullnessBits_;
}
/**
*
*
*
* Initial fullness of the Video Buffering Verifier (VBV) buffer in bits.
* Must be greater than zero. The default is equal to 90% of
* [vbv_size_bits][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.vbv_size_bits].
*
*
* int32 vbv_fullness_bits = 10;
*
* @param value The vbvFullnessBits to set.
* @return This builder for chaining.
*/
public Builder setVbvFullnessBits(int value) {
vbvFullnessBits_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Initial fullness of the Video Buffering Verifier (VBV) buffer in bits.
* Must be greater than zero. The default is equal to 90% of
* [vbv_size_bits][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.vbv_size_bits].
*
*
* int32 vbv_fullness_bits = 10;
*
* @return This builder for chaining.
*/
public Builder clearVbvFullnessBits() {
bitField0_ = (bitField0_ & ~0x00000100);
vbvFullnessBits_ = 0;
onChanged();
return this;
}
private java.lang.Object entropyCoder_ = "";
/**
*
*
*
* The entropy coder to use. The default is `cabac`.
*
* Supported entropy coders:
*
* - `cavlc`
* - `cabac`
*
*
* string entropy_coder = 11;
*
* @return The entropyCoder.
*/
public java.lang.String getEntropyCoder() {
java.lang.Object ref = entropyCoder_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
entropyCoder_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The entropy coder to use. The default is `cabac`.
*
* Supported entropy coders:
*
* - `cavlc`
* - `cabac`
*
*
* string entropy_coder = 11;
*
* @return The bytes for entropyCoder.
*/
public com.google.protobuf.ByteString getEntropyCoderBytes() {
java.lang.Object ref = entropyCoder_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
entropyCoder_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The entropy coder to use. The default is `cabac`.
*
* Supported entropy coders:
*
* - `cavlc`
* - `cabac`
*
*
* string entropy_coder = 11;
*
* @param value The entropyCoder to set.
* @return This builder for chaining.
*/
public Builder setEntropyCoder(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
entropyCoder_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* The entropy coder to use. The default is `cabac`.
*
* Supported entropy coders:
*
* - `cavlc`
* - `cabac`
*
*
* string entropy_coder = 11;
*
* @return This builder for chaining.
*/
public Builder clearEntropyCoder() {
entropyCoder_ = getDefaultInstance().getEntropyCoder();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
*
*
*
* The entropy coder to use. The default is `cabac`.
*
* Supported entropy coders:
*
* - `cavlc`
* - `cabac`
*
*
* string entropy_coder = 11;
*
* @param value The bytes for entropyCoder to set.
* @return This builder for chaining.
*/
public Builder setEntropyCoderBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
entropyCoder_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
private boolean bPyramid_;
/**
*
*
*
* Allow B-pyramid for reference frame selection. This may not be supported
* on all decoders. The default is `false`.
*
*
* bool b_pyramid = 12;
*
* @return The bPyramid.
*/
@java.lang.Override
public boolean getBPyramid() {
return bPyramid_;
}
/**
*
*
*
* Allow B-pyramid for reference frame selection. This may not be supported
* on all decoders. The default is `false`.
*
*
* bool b_pyramid = 12;
*
* @param value The bPyramid to set.
* @return This builder for chaining.
*/
public Builder setBPyramid(boolean value) {
bPyramid_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Allow B-pyramid for reference frame selection. This may not be supported
* on all decoders. The default is `false`.
*
*
* bool b_pyramid = 12;
*
* @return This builder for chaining.
*/
public Builder clearBPyramid() {
bitField0_ = (bitField0_ & ~0x00000400);
bPyramid_ = false;
onChanged();
return this;
}
private int bFrameCount_;
/**
*
*
*
* The number of consecutive B-frames. Must be greater than or equal to
* zero. Must be less than
* [gop_frame_count][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.gop_frame_count]
* if set. The default is 0.
*
*
* int32 b_frame_count = 13;
*
* @return The bFrameCount.
*/
@java.lang.Override
public int getBFrameCount() {
return bFrameCount_;
}
/**
*
*
*
* The number of consecutive B-frames. Must be greater than or equal to
* zero. Must be less than
* [gop_frame_count][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.gop_frame_count]
* if set. The default is 0.
*
*
* int32 b_frame_count = 13;
*
* @param value The bFrameCount to set.
* @return This builder for chaining.
*/
public Builder setBFrameCount(int value) {
bFrameCount_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* The number of consecutive B-frames. Must be greater than or equal to
* zero. Must be less than
* [gop_frame_count][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.gop_frame_count]
* if set. The default is 0.
*
*
* int32 b_frame_count = 13;
*
* @return This builder for chaining.
*/
public Builder clearBFrameCount() {
bitField0_ = (bitField0_ & ~0x00000800);
bFrameCount_ = 0;
onChanged();
return this;
}
private double aqStrength_;
/**
*
*
*
* Specify the intensity of the adaptive quantizer (AQ). Must be between 0
* and 1, where 0 disables the quantizer and 1 maximizes the quantizer. A
* higher value equals a lower bitrate but smoother image. The default is 0.
*
*
* double aq_strength = 14;
*
* @return The aqStrength.
*/
@java.lang.Override
public double getAqStrength() {
return aqStrength_;
}
/**
*
*
*
* Specify the intensity of the adaptive quantizer (AQ). Must be between 0
* and 1, where 0 disables the quantizer and 1 maximizes the quantizer. A
* higher value equals a lower bitrate but smoother image. The default is 0.
*
*
* double aq_strength = 14;
*
* @param value The aqStrength to set.
* @return This builder for chaining.
*/
public Builder setAqStrength(double value) {
aqStrength_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* Specify the intensity of the adaptive quantizer (AQ). Must be between 0
* and 1, where 0 disables the quantizer and 1 maximizes the quantizer. A
* higher value equals a lower bitrate but smoother image. The default is 0.
*
*
* double aq_strength = 14;
*
* @return This builder for chaining.
*/
public Builder clearAqStrength() {
bitField0_ = (bitField0_ & ~0x00001000);
aqStrength_ = 0D;
onChanged();
return this;
}
private java.lang.Object profile_ = "";
/**
*
*
*
* Enforces the specified codec profile. The following profiles are
* supported:
*
* * `baseline`
* * `main` (default)
* * `high`
*
* The available options are [FFmpeg-compatible Profile
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Profile).
* Note that certain values for this field may cause the
* transcoder to override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string profile = 15;
*
* @return The profile.
*/
public java.lang.String getProfile() {
java.lang.Object ref = profile_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
profile_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Enforces the specified codec profile. The following profiles are
* supported:
*
* * `baseline`
* * `main` (default)
* * `high`
*
* The available options are [FFmpeg-compatible Profile
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Profile).
* Note that certain values for this field may cause the
* transcoder to override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string profile = 15;
*
* @return The bytes for profile.
*/
public com.google.protobuf.ByteString getProfileBytes() {
java.lang.Object ref = profile_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
profile_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Enforces the specified codec profile. The following profiles are
* supported:
*
* * `baseline`
* * `main` (default)
* * `high`
*
* The available options are [FFmpeg-compatible Profile
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Profile).
* Note that certain values for this field may cause the
* transcoder to override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string profile = 15;
*
* @param value The profile to set.
* @return This builder for chaining.
*/
public Builder setProfile(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
profile_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* Enforces the specified codec profile. The following profiles are
* supported:
*
* * `baseline`
* * `main` (default)
* * `high`
*
* The available options are [FFmpeg-compatible Profile
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Profile).
* Note that certain values for this field may cause the
* transcoder to override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string profile = 15;
*
* @return This builder for chaining.
*/
public Builder clearProfile() {
profile_ = getDefaultInstance().getProfile();
bitField0_ = (bitField0_ & ~0x00002000);
onChanged();
return this;
}
/**
*
*
*
* Enforces the specified codec profile. The following profiles are
* supported:
*
* * `baseline`
* * `main` (default)
* * `high`
*
* The available options are [FFmpeg-compatible Profile
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Profile).
* Note that certain values for this field may cause the
* transcoder to override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string profile = 15;
*
* @param value The bytes for profile to set.
* @return This builder for chaining.
*/
public Builder setProfileBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
profile_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
private java.lang.Object tune_ = "";
/**
*
*
*
* Enforces the specified codec tune. The available options are
* [FFmpeg-compatible Encode
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Tune)
* Note that certain values for this field may cause the transcoder to
* override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string tune = 16;
*
* @return The tune.
*/
public java.lang.String getTune() {
java.lang.Object ref = tune_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tune_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Enforces the specified codec tune. The available options are
* [FFmpeg-compatible Encode
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Tune)
* Note that certain values for this field may cause the transcoder to
* override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string tune = 16;
*
* @return The bytes for tune.
*/
public com.google.protobuf.ByteString getTuneBytes() {
java.lang.Object ref = tune_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
tune_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Enforces the specified codec tune. The available options are
* [FFmpeg-compatible Encode
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Tune)
* Note that certain values for this field may cause the transcoder to
* override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string tune = 16;
*
* @param value The tune to set.
* @return This builder for chaining.
*/
public Builder setTune(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tune_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* Enforces the specified codec tune. The available options are
* [FFmpeg-compatible Encode
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Tune)
* Note that certain values for this field may cause the transcoder to
* override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string tune = 16;
*
* @return This builder for chaining.
*/
public Builder clearTune() {
tune_ = getDefaultInstance().getTune();
bitField0_ = (bitField0_ & ~0x00004000);
onChanged();
return this;
}
/**
*
*
*
* Enforces the specified codec tune. The available options are
* [FFmpeg-compatible Encode
* Options](https://trac.ffmpeg.org/wiki/Encode/H.264#Tune)
* Note that certain values for this field may cause the transcoder to
* override other fields you set in the
* [H264CodecSettings][google.cloud.video.livestream.v1.VideoStream.H264CodecSettings]
* message.
*
*
* string tune = 16;
*
* @param value The bytes for tune to set.
* @return This builder for chaining.
*/
public Builder setTuneBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tune_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.video.livestream.v1.VideoStream.H264CodecSettings)
}
// @@protoc_insertion_point(class_scope:google.cloud.video.livestream.v1.VideoStream.H264CodecSettings)
private static final com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings();
}
public static com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public H264CodecSettings parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int codecSettingsCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object codecSettings_;
public enum CodecSettingsCase
implements
com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
H264(20),
CODECSETTINGS_NOT_SET(0);
private final int value;
private CodecSettingsCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CodecSettingsCase valueOf(int value) {
return forNumber(value);
}
public static CodecSettingsCase forNumber(int value) {
switch (value) {
case 20:
return H264;
case 0:
return CODECSETTINGS_NOT_SET;
default:
return null;
}
}
public int getNumber() {
return this.value;
}
};
public CodecSettingsCase getCodecSettingsCase() {
return CodecSettingsCase.forNumber(codecSettingsCase_);
}
public static final int H264_FIELD_NUMBER = 20;
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*
* @return Whether the h264 field is set.
*/
@java.lang.Override
public boolean hasH264() {
return codecSettingsCase_ == 20;
}
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*
* @return The h264.
*/
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings getH264() {
if (codecSettingsCase_ == 20) {
return (com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings) codecSettings_;
}
return com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.getDefaultInstance();
}
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*/
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettingsOrBuilder
getH264OrBuilder() {
if (codecSettingsCase_ == 20) {
return (com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings) codecSettings_;
}
return com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (codecSettingsCase_ == 20) {
output.writeMessage(
20, (com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings) codecSettings_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (codecSettingsCase_ == 20) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
20,
(com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings) codecSettings_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.video.livestream.v1.VideoStream)) {
return super.equals(obj);
}
com.google.cloud.video.livestream.v1.VideoStream other =
(com.google.cloud.video.livestream.v1.VideoStream) obj;
if (!getCodecSettingsCase().equals(other.getCodecSettingsCase())) return false;
switch (codecSettingsCase_) {
case 20:
if (!getH264().equals(other.getH264())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (codecSettingsCase_) {
case 20:
hash = (37 * hash) + H264_FIELD_NUMBER;
hash = (53 * hash) + getH264().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.video.livestream.v1.VideoStream parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.video.livestream.v1.VideoStream parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.video.livestream.v1.VideoStream parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.video.livestream.v1.VideoStream parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.video.livestream.v1.VideoStream parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.video.livestream.v1.VideoStream parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.video.livestream.v1.VideoStream parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.video.livestream.v1.VideoStream parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.video.livestream.v1.VideoStream parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.video.livestream.v1.VideoStream parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.video.livestream.v1.VideoStream parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.video.livestream.v1.VideoStream parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.video.livestream.v1.VideoStream prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Video stream resource.
*
*
* Protobuf type {@code google.cloud.video.livestream.v1.VideoStream}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.video.livestream.v1.VideoStream)
com.google.cloud.video.livestream.v1.VideoStreamOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.video.livestream.v1.OutputsProto
.internal_static_google_cloud_video_livestream_v1_VideoStream_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.video.livestream.v1.OutputsProto
.internal_static_google_cloud_video_livestream_v1_VideoStream_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.video.livestream.v1.VideoStream.class,
com.google.cloud.video.livestream.v1.VideoStream.Builder.class);
}
// Construct using com.google.cloud.video.livestream.v1.VideoStream.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (h264Builder_ != null) {
h264Builder_.clear();
}
codecSettingsCase_ = 0;
codecSettings_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.video.livestream.v1.OutputsProto
.internal_static_google_cloud_video_livestream_v1_VideoStream_descriptor;
}
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream getDefaultInstanceForType() {
return com.google.cloud.video.livestream.v1.VideoStream.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream build() {
com.google.cloud.video.livestream.v1.VideoStream result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream buildPartial() {
com.google.cloud.video.livestream.v1.VideoStream result =
new com.google.cloud.video.livestream.v1.VideoStream(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.video.livestream.v1.VideoStream result) {
int from_bitField0_ = bitField0_;
}
private void buildPartialOneofs(com.google.cloud.video.livestream.v1.VideoStream result) {
result.codecSettingsCase_ = codecSettingsCase_;
result.codecSettings_ = this.codecSettings_;
if (codecSettingsCase_ == 20 && h264Builder_ != null) {
result.codecSettings_ = h264Builder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.video.livestream.v1.VideoStream) {
return mergeFrom((com.google.cloud.video.livestream.v1.VideoStream) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.video.livestream.v1.VideoStream other) {
if (other == com.google.cloud.video.livestream.v1.VideoStream.getDefaultInstance())
return this;
switch (other.getCodecSettingsCase()) {
case H264:
{
mergeH264(other.getH264());
break;
}
case CODECSETTINGS_NOT_SET:
{
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 162:
{
input.readMessage(getH264FieldBuilder().getBuilder(), extensionRegistry);
codecSettingsCase_ = 20;
break;
} // case 162
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int codecSettingsCase_ = 0;
private java.lang.Object codecSettings_;
public CodecSettingsCase getCodecSettingsCase() {
return CodecSettingsCase.forNumber(codecSettingsCase_);
}
public Builder clearCodecSettings() {
codecSettingsCase_ = 0;
codecSettings_ = null;
onChanged();
return this;
}
private int bitField0_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings,
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.Builder,
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettingsOrBuilder>
h264Builder_;
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*
* @return Whether the h264 field is set.
*/
@java.lang.Override
public boolean hasH264() {
return codecSettingsCase_ == 20;
}
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*
* @return The h264.
*/
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings getH264() {
if (h264Builder_ == null) {
if (codecSettingsCase_ == 20) {
return (com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings)
codecSettings_;
}
return com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
.getDefaultInstance();
} else {
if (codecSettingsCase_ == 20) {
return h264Builder_.getMessage();
}
return com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
.getDefaultInstance();
}
}
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*/
public Builder setH264(
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings value) {
if (h264Builder_ == null) {
if (value == null) {
throw new NullPointerException();
}
codecSettings_ = value;
onChanged();
} else {
h264Builder_.setMessage(value);
}
codecSettingsCase_ = 20;
return this;
}
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*/
public Builder setH264(
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.Builder
builderForValue) {
if (h264Builder_ == null) {
codecSettings_ = builderForValue.build();
onChanged();
} else {
h264Builder_.setMessage(builderForValue.build());
}
codecSettingsCase_ = 20;
return this;
}
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*/
public Builder mergeH264(
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings value) {
if (h264Builder_ == null) {
if (codecSettingsCase_ == 20
&& codecSettings_
!= com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
.getDefaultInstance()) {
codecSettings_ =
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.newBuilder(
(com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings)
codecSettings_)
.mergeFrom(value)
.buildPartial();
} else {
codecSettings_ = value;
}
onChanged();
} else {
if (codecSettingsCase_ == 20) {
h264Builder_.mergeFrom(value);
} else {
h264Builder_.setMessage(value);
}
}
codecSettingsCase_ = 20;
return this;
}
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*/
public Builder clearH264() {
if (h264Builder_ == null) {
if (codecSettingsCase_ == 20) {
codecSettingsCase_ = 0;
codecSettings_ = null;
onChanged();
}
} else {
if (codecSettingsCase_ == 20) {
codecSettingsCase_ = 0;
codecSettings_ = null;
}
h264Builder_.clear();
}
return this;
}
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*/
public com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.Builder
getH264Builder() {
return getH264FieldBuilder().getBuilder();
}
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*/
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettingsOrBuilder
getH264OrBuilder() {
if ((codecSettingsCase_ == 20) && (h264Builder_ != null)) {
return h264Builder_.getMessageOrBuilder();
} else {
if (codecSettingsCase_ == 20) {
return (com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings)
codecSettings_;
}
return com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
.getDefaultInstance();
}
}
/**
*
*
*
* H264 codec settings.
*
*
* .google.cloud.video.livestream.v1.VideoStream.H264CodecSettings h264 = 20;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings,
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.Builder,
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettingsOrBuilder>
getH264FieldBuilder() {
if (h264Builder_ == null) {
if (!(codecSettingsCase_ == 20)) {
codecSettings_ =
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings
.getDefaultInstance();
}
h264Builder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings,
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings.Builder,
com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettingsOrBuilder>(
(com.google.cloud.video.livestream.v1.VideoStream.H264CodecSettings) codecSettings_,
getParentForChildren(),
isClean());
codecSettings_ = null;
}
codecSettingsCase_ = 20;
onChanged();
return h264Builder_;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.video.livestream.v1.VideoStream)
}
// @@protoc_insertion_point(class_scope:google.cloud.video.livestream.v1.VideoStream)
private static final com.google.cloud.video.livestream.v1.VideoStream DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.video.livestream.v1.VideoStream();
}
public static com.google.cloud.video.livestream.v1.VideoStream getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public VideoStream parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.video.livestream.v1.VideoStream getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy