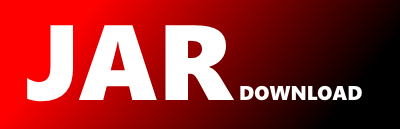
com.google.cloud.notebooks.v2.GceSetupOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-notebooks-v2 Show documentation
Show all versions of proto-google-cloud-notebooks-v2 Show documentation
Proto library for google-cloud-notebooks
/*
* Copyright 2023 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/notebooks/v2/gce_setup.proto
package com.google.cloud.notebooks.v2;
public interface GceSetupOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.notebooks.v2.GceSetup)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Optional. The machine type of the VM instance.
* https://cloud.google.com/compute/docs/machine-resource
*
*
* string machine_type = 1 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The machineType.
*/
java.lang.String getMachineType();
/**
*
*
*
* Optional. The machine type of the VM instance.
* https://cloud.google.com/compute/docs/machine-resource
*
*
* string machine_type = 1 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for machineType.
*/
com.google.protobuf.ByteString getMachineTypeBytes();
/**
*
*
*
* Optional. The hardware accelerators used on this instance. If you use
* accelerators, make sure that your configuration has
* [enough vCPUs and memory to support the `machine_type` you have
* selected](https://cloud.google.com/compute/docs/gpus/#gpus-list).
* Currently supports only one accelerator configuration.
*
*
*
* repeated .google.cloud.notebooks.v2.AcceleratorConfig accelerator_configs = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List getAcceleratorConfigsList();
/**
*
*
*
* Optional. The hardware accelerators used on this instance. If you use
* accelerators, make sure that your configuration has
* [enough vCPUs and memory to support the `machine_type` you have
* selected](https://cloud.google.com/compute/docs/gpus/#gpus-list).
* Currently supports only one accelerator configuration.
*
*
*
* repeated .google.cloud.notebooks.v2.AcceleratorConfig accelerator_configs = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.AcceleratorConfig getAcceleratorConfigs(int index);
/**
*
*
*
* Optional. The hardware accelerators used on this instance. If you use
* accelerators, make sure that your configuration has
* [enough vCPUs and memory to support the `machine_type` you have
* selected](https://cloud.google.com/compute/docs/gpus/#gpus-list).
* Currently supports only one accelerator configuration.
*
*
*
* repeated .google.cloud.notebooks.v2.AcceleratorConfig accelerator_configs = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
int getAcceleratorConfigsCount();
/**
*
*
*
* Optional. The hardware accelerators used on this instance. If you use
* accelerators, make sure that your configuration has
* [enough vCPUs and memory to support the `machine_type` you have
* selected](https://cloud.google.com/compute/docs/gpus/#gpus-list).
* Currently supports only one accelerator configuration.
*
*
*
* repeated .google.cloud.notebooks.v2.AcceleratorConfig accelerator_configs = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List extends com.google.cloud.notebooks.v2.AcceleratorConfigOrBuilder>
getAcceleratorConfigsOrBuilderList();
/**
*
*
*
* Optional. The hardware accelerators used on this instance. If you use
* accelerators, make sure that your configuration has
* [enough vCPUs and memory to support the `machine_type` you have
* selected](https://cloud.google.com/compute/docs/gpus/#gpus-list).
* Currently supports only one accelerator configuration.
*
*
*
* repeated .google.cloud.notebooks.v2.AcceleratorConfig accelerator_configs = 2 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.AcceleratorConfigOrBuilder getAcceleratorConfigsOrBuilder(
int index);
/**
*
*
*
* Optional. The service account that serves as an identity for the VM
* instance. Currently supports only one service account.
*
*
*
* repeated .google.cloud.notebooks.v2.ServiceAccount service_accounts = 3 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List getServiceAccountsList();
/**
*
*
*
* Optional. The service account that serves as an identity for the VM
* instance. Currently supports only one service account.
*
*
*
* repeated .google.cloud.notebooks.v2.ServiceAccount service_accounts = 3 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.ServiceAccount getServiceAccounts(int index);
/**
*
*
*
* Optional. The service account that serves as an identity for the VM
* instance. Currently supports only one service account.
*
*
*
* repeated .google.cloud.notebooks.v2.ServiceAccount service_accounts = 3 [(.google.api.field_behavior) = OPTIONAL];
*
*/
int getServiceAccountsCount();
/**
*
*
*
* Optional. The service account that serves as an identity for the VM
* instance. Currently supports only one service account.
*
*
*
* repeated .google.cloud.notebooks.v2.ServiceAccount service_accounts = 3 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List extends com.google.cloud.notebooks.v2.ServiceAccountOrBuilder>
getServiceAccountsOrBuilderList();
/**
*
*
*
* Optional. The service account that serves as an identity for the VM
* instance. Currently supports only one service account.
*
*
*
* repeated .google.cloud.notebooks.v2.ServiceAccount service_accounts = 3 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.ServiceAccountOrBuilder getServiceAccountsOrBuilder(int index);
/**
*
*
*
* Optional. Use a Compute Engine VM image to start the notebook instance.
*
*
*
* .google.cloud.notebooks.v2.VmImage vm_image = 4 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the vmImage field is set.
*/
boolean hasVmImage();
/**
*
*
*
* Optional. Use a Compute Engine VM image to start the notebook instance.
*
*
*
* .google.cloud.notebooks.v2.VmImage vm_image = 4 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The vmImage.
*/
com.google.cloud.notebooks.v2.VmImage getVmImage();
/**
*
*
*
* Optional. Use a Compute Engine VM image to start the notebook instance.
*
*
*
* .google.cloud.notebooks.v2.VmImage vm_image = 4 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.VmImageOrBuilder getVmImageOrBuilder();
/**
*
*
*
* Optional. Use a container image to start the notebook instance.
*
*
*
* .google.cloud.notebooks.v2.ContainerImage container_image = 5 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the containerImage field is set.
*/
boolean hasContainerImage();
/**
*
*
*
* Optional. Use a container image to start the notebook instance.
*
*
*
* .google.cloud.notebooks.v2.ContainerImage container_image = 5 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The containerImage.
*/
com.google.cloud.notebooks.v2.ContainerImage getContainerImage();
/**
*
*
*
* Optional. Use a container image to start the notebook instance.
*
*
*
* .google.cloud.notebooks.v2.ContainerImage container_image = 5 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.ContainerImageOrBuilder getContainerImageOrBuilder();
/**
*
*
*
* Optional. The boot disk for the VM.
*
*
*
* .google.cloud.notebooks.v2.BootDisk boot_disk = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the bootDisk field is set.
*/
boolean hasBootDisk();
/**
*
*
*
* Optional. The boot disk for the VM.
*
*
*
* .google.cloud.notebooks.v2.BootDisk boot_disk = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The bootDisk.
*/
com.google.cloud.notebooks.v2.BootDisk getBootDisk();
/**
*
*
*
* Optional. The boot disk for the VM.
*
*
*
* .google.cloud.notebooks.v2.BootDisk boot_disk = 6 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.BootDiskOrBuilder getBootDiskOrBuilder();
/**
*
*
*
* Optional. Data disks attached to the VM instance.
* Currently supports only one data disk.
*
*
*
* repeated .google.cloud.notebooks.v2.DataDisk data_disks = 7 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List getDataDisksList();
/**
*
*
*
* Optional. Data disks attached to the VM instance.
* Currently supports only one data disk.
*
*
*
* repeated .google.cloud.notebooks.v2.DataDisk data_disks = 7 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.DataDisk getDataDisks(int index);
/**
*
*
*
* Optional. Data disks attached to the VM instance.
* Currently supports only one data disk.
*
*
*
* repeated .google.cloud.notebooks.v2.DataDisk data_disks = 7 [(.google.api.field_behavior) = OPTIONAL];
*
*/
int getDataDisksCount();
/**
*
*
*
* Optional. Data disks attached to the VM instance.
* Currently supports only one data disk.
*
*
*
* repeated .google.cloud.notebooks.v2.DataDisk data_disks = 7 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List extends com.google.cloud.notebooks.v2.DataDiskOrBuilder>
getDataDisksOrBuilderList();
/**
*
*
*
* Optional. Data disks attached to the VM instance.
* Currently supports only one data disk.
*
*
*
* repeated .google.cloud.notebooks.v2.DataDisk data_disks = 7 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.DataDiskOrBuilder getDataDisksOrBuilder(int index);
/**
*
*
*
* Optional. Shielded VM configuration.
* [Images using supported Shielded VM
* features](https://cloud.google.com/compute/docs/instances/modifying-shielded-vm).
*
*
*
* .google.cloud.notebooks.v2.ShieldedInstanceConfig shielded_instance_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the shieldedInstanceConfig field is set.
*/
boolean hasShieldedInstanceConfig();
/**
*
*
*
* Optional. Shielded VM configuration.
* [Images using supported Shielded VM
* features](https://cloud.google.com/compute/docs/instances/modifying-shielded-vm).
*
*
*
* .google.cloud.notebooks.v2.ShieldedInstanceConfig shielded_instance_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The shieldedInstanceConfig.
*/
com.google.cloud.notebooks.v2.ShieldedInstanceConfig getShieldedInstanceConfig();
/**
*
*
*
* Optional. Shielded VM configuration.
* [Images using supported Shielded VM
* features](https://cloud.google.com/compute/docs/instances/modifying-shielded-vm).
*
*
*
* .google.cloud.notebooks.v2.ShieldedInstanceConfig shielded_instance_config = 8 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.ShieldedInstanceConfigOrBuilder
getShieldedInstanceConfigOrBuilder();
/**
*
*
*
* Optional. The network interfaces for the VM.
* Supports only one interface.
*
*
*
* repeated .google.cloud.notebooks.v2.NetworkInterface network_interfaces = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List getNetworkInterfacesList();
/**
*
*
*
* Optional. The network interfaces for the VM.
* Supports only one interface.
*
*
*
* repeated .google.cloud.notebooks.v2.NetworkInterface network_interfaces = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.NetworkInterface getNetworkInterfaces(int index);
/**
*
*
*
* Optional. The network interfaces for the VM.
* Supports only one interface.
*
*
*
* repeated .google.cloud.notebooks.v2.NetworkInterface network_interfaces = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
int getNetworkInterfacesCount();
/**
*
*
*
* Optional. The network interfaces for the VM.
* Supports only one interface.
*
*
*
* repeated .google.cloud.notebooks.v2.NetworkInterface network_interfaces = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.List extends com.google.cloud.notebooks.v2.NetworkInterfaceOrBuilder>
getNetworkInterfacesOrBuilderList();
/**
*
*
*
* Optional. The network interfaces for the VM.
* Supports only one interface.
*
*
*
* repeated .google.cloud.notebooks.v2.NetworkInterface network_interfaces = 9 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.NetworkInterfaceOrBuilder getNetworkInterfacesOrBuilder(int index);
/**
*
*
*
* Optional. If true, no external IP will be assigned to this VM instance.
*
*
* bool disable_public_ip = 10 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The disablePublicIp.
*/
boolean getDisablePublicIp();
/**
*
*
*
* Optional. The Compute Engine tags to add to runtime (see [Tagging
* instances](https://cloud.google.com/compute/docs/label-or-tag-resources#tags)).
*
*
* repeated string tags = 11 [(.google.api.field_behavior) = OPTIONAL];
*
* @return A list containing the tags.
*/
java.util.List getTagsList();
/**
*
*
*
* Optional. The Compute Engine tags to add to runtime (see [Tagging
* instances](https://cloud.google.com/compute/docs/label-or-tag-resources#tags)).
*
*
* repeated string tags = 11 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The count of tags.
*/
int getTagsCount();
/**
*
*
*
* Optional. The Compute Engine tags to add to runtime (see [Tagging
* instances](https://cloud.google.com/compute/docs/label-or-tag-resources#tags)).
*
*
* repeated string tags = 11 [(.google.api.field_behavior) = OPTIONAL];
*
* @param index The index of the element to return.
* @return The tags at the given index.
*/
java.lang.String getTags(int index);
/**
*
*
*
* Optional. The Compute Engine tags to add to runtime (see [Tagging
* instances](https://cloud.google.com/compute/docs/label-or-tag-resources#tags)).
*
*
* repeated string tags = 11 [(.google.api.field_behavior) = OPTIONAL];
*
* @param index The index of the value to return.
* @return The bytes of the tags at the given index.
*/
com.google.protobuf.ByteString getTagsBytes(int index);
/**
*
*
*
* Optional. Custom metadata to apply to this instance.
*
*
* map<string, string> metadata = 12 [(.google.api.field_behavior) = OPTIONAL];
*/
int getMetadataCount();
/**
*
*
*
* Optional. Custom metadata to apply to this instance.
*
*
* map<string, string> metadata = 12 [(.google.api.field_behavior) = OPTIONAL];
*/
boolean containsMetadata(java.lang.String key);
/** Use {@link #getMetadataMap()} instead. */
@java.lang.Deprecated
java.util.Map getMetadata();
/**
*
*
*
* Optional. Custom metadata to apply to this instance.
*
*
* map<string, string> metadata = 12 [(.google.api.field_behavior) = OPTIONAL];
*/
java.util.Map getMetadataMap();
/**
*
*
*
* Optional. Custom metadata to apply to this instance.
*
*
* map<string, string> metadata = 12 [(.google.api.field_behavior) = OPTIONAL];
*/
/* nullable */
java.lang.String getMetadataOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
*
*
*
* Optional. Custom metadata to apply to this instance.
*
*
* map<string, string> metadata = 12 [(.google.api.field_behavior) = OPTIONAL];
*/
java.lang.String getMetadataOrThrow(java.lang.String key);
/**
*
*
*
* Optional. Flag to enable ip forwarding or not, default false/off.
* https://cloud.google.com/vpc/docs/using-routes#canipforward
*
*
* bool enable_ip_forwarding = 13 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The enableIpForwarding.
*/
boolean getEnableIpForwarding();
/**
*
*
*
* Optional. Configuration for GPU drivers.
*
*
*
* .google.cloud.notebooks.v2.GPUDriverConfig gpu_driver_config = 14 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the gpuDriverConfig field is set.
*/
boolean hasGpuDriverConfig();
/**
*
*
*
* Optional. Configuration for GPU drivers.
*
*
*
* .google.cloud.notebooks.v2.GPUDriverConfig gpu_driver_config = 14 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The gpuDriverConfig.
*/
com.google.cloud.notebooks.v2.GPUDriverConfig getGpuDriverConfig();
/**
*
*
*
* Optional. Configuration for GPU drivers.
*
*
*
* .google.cloud.notebooks.v2.GPUDriverConfig gpu_driver_config = 14 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.notebooks.v2.GPUDriverConfigOrBuilder getGpuDriverConfigOrBuilder();
com.google.cloud.notebooks.v2.GceSetup.ImageCase getImageCase();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy