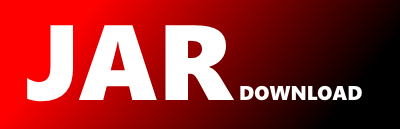
com.google.cloud.redis.v1beta1.InstanceOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-redis-v1beta1 Show documentation
Show all versions of proto-google-cloud-redis-v1beta1 Show documentation
PROTO library for proto-google-cloud-redis-v1beta1
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/redis/v1beta1/cloud_redis.proto
// Protobuf Java Version: 3.25.4
package com.google.cloud.redis.v1beta1;
public interface InstanceOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.redis.v1beta1.Instance)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Required. Unique name of the resource in this scope including project and
* location using the form:
* `projects/{project_id}/locations/{location_id}/instances/{instance_id}`
*
* Note: Redis instances are managed and addressed at regional level so
* location_id here refers to a GCP region; however, users may choose which
* specific zone (or collection of zones for cross-zone instances) an instance
* should be provisioned in. Refer to [location_id][google.cloud.redis.v1beta1.Instance.location_id] and
* [alternative_location_id][google.cloud.redis.v1beta1.Instance.alternative_location_id] fields for more details.
*
*
* string name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The name.
*/
java.lang.String getName();
/**
*
*
*
* Required. Unique name of the resource in this scope including project and
* location using the form:
* `projects/{project_id}/locations/{location_id}/instances/{instance_id}`
*
* Note: Redis instances are managed and addressed at regional level so
* location_id here refers to a GCP region; however, users may choose which
* specific zone (or collection of zones for cross-zone instances) an instance
* should be provisioned in. Refer to [location_id][google.cloud.redis.v1beta1.Instance.location_id] and
* [alternative_location_id][google.cloud.redis.v1beta1.Instance.alternative_location_id] fields for more details.
*
*
* string name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for name.
*/
com.google.protobuf.ByteString getNameBytes();
/**
*
*
*
* An arbitrary and optional user-provided name for the instance.
*
*
* string display_name = 2;
*
* @return The displayName.
*/
java.lang.String getDisplayName();
/**
*
*
*
* An arbitrary and optional user-provided name for the instance.
*
*
* string display_name = 2;
*
* @return The bytes for displayName.
*/
com.google.protobuf.ByteString getDisplayNameBytes();
/**
*
*
*
* Resource labels to represent user provided metadata
*
*
* map<string, string> labels = 3;
*/
int getLabelsCount();
/**
*
*
*
* Resource labels to represent user provided metadata
*
*
* map<string, string> labels = 3;
*/
boolean containsLabels(java.lang.String key);
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Deprecated
java.util.Map getLabels();
/**
*
*
*
* Resource labels to represent user provided metadata
*
*
* map<string, string> labels = 3;
*/
java.util.Map getLabelsMap();
/**
*
*
*
* Resource labels to represent user provided metadata
*
*
* map<string, string> labels = 3;
*/
/* nullable */
java.lang.String getLabelsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
*
*
*
* Resource labels to represent user provided metadata
*
*
* map<string, string> labels = 3;
*/
java.lang.String getLabelsOrThrow(java.lang.String key);
/**
*
*
*
* Optional. The zone where the instance will be provisioned. If not provided,
* the service will choose a zone from the specified region for the instance.
* For standard tier, additional nodes will be added across multiple zones for
* protection against zonal failures. If specified, at least one node will be
* provisioned in this zone.
*
*
* string location_id = 4 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The locationId.
*/
java.lang.String getLocationId();
/**
*
*
*
* Optional. The zone where the instance will be provisioned. If not provided,
* the service will choose a zone from the specified region for the instance.
* For standard tier, additional nodes will be added across multiple zones for
* protection against zonal failures. If specified, at least one node will be
* provisioned in this zone.
*
*
* string location_id = 4 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for locationId.
*/
com.google.protobuf.ByteString getLocationIdBytes();
/**
*
*
*
* Optional. If specified, at least one node will be provisioned in this zone
* in addition to the zone specified in location_id. Only applicable to
* standard tier. If provided, it must be a different zone from the one
* provided in [location_id]. Additional nodes beyond the first 2 will be
* placed in zones selected by the service.
*
*
* string alternative_location_id = 5 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The alternativeLocationId.
*/
java.lang.String getAlternativeLocationId();
/**
*
*
*
* Optional. If specified, at least one node will be provisioned in this zone
* in addition to the zone specified in location_id. Only applicable to
* standard tier. If provided, it must be a different zone from the one
* provided in [location_id]. Additional nodes beyond the first 2 will be
* placed in zones selected by the service.
*
*
* string alternative_location_id = 5 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for alternativeLocationId.
*/
com.google.protobuf.ByteString getAlternativeLocationIdBytes();
/**
*
*
*
* Optional. The version of Redis software.
* If not provided, latest supported version will be used. Currently, the
* supported values are:
*
* * `REDIS_3_2` for Redis 3.2 compatibility
* * `REDIS_4_0` for Redis 4.0 compatibility (default)
* * `REDIS_5_0` for Redis 5.0 compatibility
* * `REDIS_6_X` for Redis 6.x compatibility
*
*
* string redis_version = 7 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The redisVersion.
*/
java.lang.String getRedisVersion();
/**
*
*
*
* Optional. The version of Redis software.
* If not provided, latest supported version will be used. Currently, the
* supported values are:
*
* * `REDIS_3_2` for Redis 3.2 compatibility
* * `REDIS_4_0` for Redis 4.0 compatibility (default)
* * `REDIS_5_0` for Redis 5.0 compatibility
* * `REDIS_6_X` for Redis 6.x compatibility
*
*
* string redis_version = 7 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for redisVersion.
*/
com.google.protobuf.ByteString getRedisVersionBytes();
/**
*
*
*
* Optional. For DIRECT_PEERING mode, the CIDR range of internal addresses
* that are reserved for this instance. Range must
* be unique and non-overlapping with existing subnets in an authorized
* network. For PRIVATE_SERVICE_ACCESS mode, the name of one allocated IP
* address ranges associated with this private service access connection.
* If not provided, the service will choose an unused /29 block, for
* example, 10.0.0.0/29 or 192.168.0.0/29. For READ_REPLICAS_ENABLED
* the default block size is /28.
*
*
* string reserved_ip_range = 9 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The reservedIpRange.
*/
java.lang.String getReservedIpRange();
/**
*
*
*
* Optional. For DIRECT_PEERING mode, the CIDR range of internal addresses
* that are reserved for this instance. Range must
* be unique and non-overlapping with existing subnets in an authorized
* network. For PRIVATE_SERVICE_ACCESS mode, the name of one allocated IP
* address ranges associated with this private service access connection.
* If not provided, the service will choose an unused /29 block, for
* example, 10.0.0.0/29 or 192.168.0.0/29. For READ_REPLICAS_ENABLED
* the default block size is /28.
*
*
* string reserved_ip_range = 9 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for reservedIpRange.
*/
com.google.protobuf.ByteString getReservedIpRangeBytes();
/**
*
*
*
* Optional. Additional IP range for node placement. Required when enabling read
* replicas on an existing instance. For DIRECT_PEERING mode value must be a
* CIDR range of size /28, or "auto". For PRIVATE_SERVICE_ACCESS mode value
* must be the name of an allocated address range associated with the private
* service access connection, or "auto".
*
*
* string secondary_ip_range = 30 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The secondaryIpRange.
*/
java.lang.String getSecondaryIpRange();
/**
*
*
*
* Optional. Additional IP range for node placement. Required when enabling read
* replicas on an existing instance. For DIRECT_PEERING mode value must be a
* CIDR range of size /28, or "auto". For PRIVATE_SERVICE_ACCESS mode value
* must be the name of an allocated address range associated with the private
* service access connection, or "auto".
*
*
* string secondary_ip_range = 30 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for secondaryIpRange.
*/
com.google.protobuf.ByteString getSecondaryIpRangeBytes();
/**
*
*
*
* Output only. Hostname or IP address of the exposed Redis endpoint used by
* clients to connect to the service.
*
*
* string host = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The host.
*/
java.lang.String getHost();
/**
*
*
*
* Output only. Hostname or IP address of the exposed Redis endpoint used by
* clients to connect to the service.
*
*
* string host = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for host.
*/
com.google.protobuf.ByteString getHostBytes();
/**
*
*
*
* Output only. The port number of the exposed Redis endpoint.
*
*
* int32 port = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The port.
*/
int getPort();
/**
*
*
*
* Output only. The current zone where the Redis primary node is located. In
* basic tier, this will always be the same as [location_id]. In
* standard tier, this can be the zone of any node in the instance.
*
*
* string current_location_id = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The currentLocationId.
*/
java.lang.String getCurrentLocationId();
/**
*
*
*
* Output only. The current zone where the Redis primary node is located. In
* basic tier, this will always be the same as [location_id]. In
* standard tier, this can be the zone of any node in the instance.
*
*
* string current_location_id = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for currentLocationId.
*/
com.google.protobuf.ByteString getCurrentLocationIdBytes();
/**
*
*
*
* Output only. The time the instance was created.
*
*
* .google.protobuf.Timestamp create_time = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the createTime field is set.
*/
boolean hasCreateTime();
/**
*
*
*
* Output only. The time the instance was created.
*
*
* .google.protobuf.Timestamp create_time = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The createTime.
*/
com.google.protobuf.Timestamp getCreateTime();
/**
*
*
*
* Output only. The time the instance was created.
*
*
* .google.protobuf.Timestamp create_time = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder();
/**
*
*
*
* Output only. The current state of this instance.
*
*
*
* .google.cloud.redis.v1beta1.Instance.State state = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The enum numeric value on the wire for state.
*/
int getStateValue();
/**
*
*
*
* Output only. The current state of this instance.
*
*
*
* .google.cloud.redis.v1beta1.Instance.State state = 14 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The state.
*/
com.google.cloud.redis.v1beta1.Instance.State getState();
/**
*
*
*
* Output only. Additional information about the current status of this
* instance, if available.
*
*
* string status_message = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The statusMessage.
*/
java.lang.String getStatusMessage();
/**
*
*
*
* Output only. Additional information about the current status of this
* instance, if available.
*
*
* string status_message = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for statusMessage.
*/
com.google.protobuf.ByteString getStatusMessageBytes();
/**
*
*
*
* Optional. Redis configuration parameters, according to
* http://redis.io/topics/config. Currently, the only supported parameters
* are:
*
* Redis version 3.2 and newer:
*
* * maxmemory-policy
* * notify-keyspace-events
*
* Redis version 4.0 and newer:
*
* * activedefrag
* * lfu-decay-time
* * lfu-log-factor
* * maxmemory-gb
*
* Redis version 5.0 and newer:
*
* * stream-node-max-bytes
* * stream-node-max-entries
*
*
* map<string, string> redis_configs = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
int getRedisConfigsCount();
/**
*
*
*
* Optional. Redis configuration parameters, according to
* http://redis.io/topics/config. Currently, the only supported parameters
* are:
*
* Redis version 3.2 and newer:
*
* * maxmemory-policy
* * notify-keyspace-events
*
* Redis version 4.0 and newer:
*
* * activedefrag
* * lfu-decay-time
* * lfu-log-factor
* * maxmemory-gb
*
* Redis version 5.0 and newer:
*
* * stream-node-max-bytes
* * stream-node-max-entries
*
*
* map<string, string> redis_configs = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
boolean containsRedisConfigs(java.lang.String key);
/** Use {@link #getRedisConfigsMap()} instead. */
@java.lang.Deprecated
java.util.Map getRedisConfigs();
/**
*
*
*
* Optional. Redis configuration parameters, according to
* http://redis.io/topics/config. Currently, the only supported parameters
* are:
*
* Redis version 3.2 and newer:
*
* * maxmemory-policy
* * notify-keyspace-events
*
* Redis version 4.0 and newer:
*
* * activedefrag
* * lfu-decay-time
* * lfu-log-factor
* * maxmemory-gb
*
* Redis version 5.0 and newer:
*
* * stream-node-max-bytes
* * stream-node-max-entries
*
*
* map<string, string> redis_configs = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.util.Map getRedisConfigsMap();
/**
*
*
*
* Optional. Redis configuration parameters, according to
* http://redis.io/topics/config. Currently, the only supported parameters
* are:
*
* Redis version 3.2 and newer:
*
* * maxmemory-policy
* * notify-keyspace-events
*
* Redis version 4.0 and newer:
*
* * activedefrag
* * lfu-decay-time
* * lfu-log-factor
* * maxmemory-gb
*
* Redis version 5.0 and newer:
*
* * stream-node-max-bytes
* * stream-node-max-entries
*
*
* map<string, string> redis_configs = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
/* nullable */
java.lang.String getRedisConfigsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
*
*
*
* Optional. Redis configuration parameters, according to
* http://redis.io/topics/config. Currently, the only supported parameters
* are:
*
* Redis version 3.2 and newer:
*
* * maxmemory-policy
* * notify-keyspace-events
*
* Redis version 4.0 and newer:
*
* * activedefrag
* * lfu-decay-time
* * lfu-log-factor
* * maxmemory-gb
*
* Redis version 5.0 and newer:
*
* * stream-node-max-bytes
* * stream-node-max-entries
*
*
* map<string, string> redis_configs = 16 [(.google.api.field_behavior) = OPTIONAL];
*
*/
java.lang.String getRedisConfigsOrThrow(java.lang.String key);
/**
*
*
*
* Required. The service tier of the instance.
*
*
*
* .google.cloud.redis.v1beta1.Instance.Tier tier = 17 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The enum numeric value on the wire for tier.
*/
int getTierValue();
/**
*
*
*
* Required. The service tier of the instance.
*
*
*
* .google.cloud.redis.v1beta1.Instance.Tier tier = 17 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The tier.
*/
com.google.cloud.redis.v1beta1.Instance.Tier getTier();
/**
*
*
*
* Required. Redis memory size in GiB.
*
*
* int32 memory_size_gb = 18 [(.google.api.field_behavior) = REQUIRED];
*
* @return The memorySizeGb.
*/
int getMemorySizeGb();
/**
*
*
*
* Optional. The full name of the Google Compute Engine
* [network](https://cloud.google.com/vpc/docs/vpc) to which the
* instance is connected. If left unspecified, the `default` network
* will be used.
*
*
* string authorized_network = 20 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The authorizedNetwork.
*/
java.lang.String getAuthorizedNetwork();
/**
*
*
*
* Optional. The full name of the Google Compute Engine
* [network](https://cloud.google.com/vpc/docs/vpc) to which the
* instance is connected. If left unspecified, the `default` network
* will be used.
*
*
* string authorized_network = 20 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for authorizedNetwork.
*/
com.google.protobuf.ByteString getAuthorizedNetworkBytes();
/**
*
*
*
* Output only. Cloud IAM identity used by import / export operations to
* transfer data to/from Cloud Storage. Format is
* "serviceAccount:<service_account_email>". The value may change over time
* for a given instance so should be checked before each import/export
* operation.
*
*
* string persistence_iam_identity = 21 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The persistenceIamIdentity.
*/
java.lang.String getPersistenceIamIdentity();
/**
*
*
*
* Output only. Cloud IAM identity used by import / export operations to
* transfer data to/from Cloud Storage. Format is
* "serviceAccount:<service_account_email>". The value may change over time
* for a given instance so should be checked before each import/export
* operation.
*
*
* string persistence_iam_identity = 21 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for persistenceIamIdentity.
*/
com.google.protobuf.ByteString getPersistenceIamIdentityBytes();
/**
*
*
*
* Optional. The network connect mode of the Redis instance.
* If not provided, the connect mode defaults to DIRECT_PEERING.
*
*
*
* .google.cloud.redis.v1beta1.Instance.ConnectMode connect_mode = 22 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The enum numeric value on the wire for connectMode.
*/
int getConnectModeValue();
/**
*
*
*
* Optional. The network connect mode of the Redis instance.
* If not provided, the connect mode defaults to DIRECT_PEERING.
*
*
*
* .google.cloud.redis.v1beta1.Instance.ConnectMode connect_mode = 22 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The connectMode.
*/
com.google.cloud.redis.v1beta1.Instance.ConnectMode getConnectMode();
/**
*
*
*
* Optional. Indicates whether OSS Redis AUTH is enabled for the instance. If set to
* "true" AUTH is enabled on the instance. Default value is "false" meaning
* AUTH is disabled.
*
*
* bool auth_enabled = 23 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The authEnabled.
*/
boolean getAuthEnabled();
/**
*
*
*
* Output only. List of server CA certificates for the instance.
*
*
*
* repeated .google.cloud.redis.v1beta1.TlsCertificate server_ca_certs = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
java.util.List getServerCaCertsList();
/**
*
*
*
* Output only. List of server CA certificates for the instance.
*
*
*
* repeated .google.cloud.redis.v1beta1.TlsCertificate server_ca_certs = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.cloud.redis.v1beta1.TlsCertificate getServerCaCerts(int index);
/**
*
*
*
* Output only. List of server CA certificates for the instance.
*
*
*
* repeated .google.cloud.redis.v1beta1.TlsCertificate server_ca_certs = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
int getServerCaCertsCount();
/**
*
*
*
* Output only. List of server CA certificates for the instance.
*
*
*
* repeated .google.cloud.redis.v1beta1.TlsCertificate server_ca_certs = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
java.util.List extends com.google.cloud.redis.v1beta1.TlsCertificateOrBuilder>
getServerCaCertsOrBuilderList();
/**
*
*
*
* Output only. List of server CA certificates for the instance.
*
*
*
* repeated .google.cloud.redis.v1beta1.TlsCertificate server_ca_certs = 25 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.cloud.redis.v1beta1.TlsCertificateOrBuilder getServerCaCertsOrBuilder(int index);
/**
*
*
*
* Optional. The TLS mode of the Redis instance.
* If not provided, TLS is disabled for the instance.
*
*
*
* .google.cloud.redis.v1beta1.Instance.TransitEncryptionMode transit_encryption_mode = 26 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The enum numeric value on the wire for transitEncryptionMode.
*/
int getTransitEncryptionModeValue();
/**
*
*
*
* Optional. The TLS mode of the Redis instance.
* If not provided, TLS is disabled for the instance.
*
*
*
* .google.cloud.redis.v1beta1.Instance.TransitEncryptionMode transit_encryption_mode = 26 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The transitEncryptionMode.
*/
com.google.cloud.redis.v1beta1.Instance.TransitEncryptionMode getTransitEncryptionMode();
/**
*
*
*
* Optional. The maintenance policy for the instance. If not provided,
* maintenance events can be performed at any time.
*
*
*
* .google.cloud.redis.v1beta1.MaintenancePolicy maintenance_policy = 27 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the maintenancePolicy field is set.
*/
boolean hasMaintenancePolicy();
/**
*
*
*
* Optional. The maintenance policy for the instance. If not provided,
* maintenance events can be performed at any time.
*
*
*
* .google.cloud.redis.v1beta1.MaintenancePolicy maintenance_policy = 27 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The maintenancePolicy.
*/
com.google.cloud.redis.v1beta1.MaintenancePolicy getMaintenancePolicy();
/**
*
*
*
* Optional. The maintenance policy for the instance. If not provided,
* maintenance events can be performed at any time.
*
*
*
* .google.cloud.redis.v1beta1.MaintenancePolicy maintenance_policy = 27 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.redis.v1beta1.MaintenancePolicyOrBuilder getMaintenancePolicyOrBuilder();
/**
*
*
*
* Output only. Date and time of upcoming maintenance events which have been
* scheduled.
*
*
*
* .google.cloud.redis.v1beta1.MaintenanceSchedule maintenance_schedule = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the maintenanceSchedule field is set.
*/
boolean hasMaintenanceSchedule();
/**
*
*
*
* Output only. Date and time of upcoming maintenance events which have been
* scheduled.
*
*
*
* .google.cloud.redis.v1beta1.MaintenanceSchedule maintenance_schedule = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The maintenanceSchedule.
*/
com.google.cloud.redis.v1beta1.MaintenanceSchedule getMaintenanceSchedule();
/**
*
*
*
* Output only. Date and time of upcoming maintenance events which have been
* scheduled.
*
*
*
* .google.cloud.redis.v1beta1.MaintenanceSchedule maintenance_schedule = 28 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.cloud.redis.v1beta1.MaintenanceScheduleOrBuilder getMaintenanceScheduleOrBuilder();
/**
*
*
*
* Optional. The number of replica nodes. The valid range for the Standard Tier with
* read replicas enabled is [1-5] and defaults to 2. If read replicas are not
* enabled for a Standard Tier instance, the only valid value is 1 and the
* default is 1. The valid value for basic tier is 0 and the default is also
* 0.
*
*
* int32 replica_count = 31 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The replicaCount.
*/
int getReplicaCount();
/**
*
*
*
* Output only. Info per node.
*
*
*
* repeated .google.cloud.redis.v1beta1.NodeInfo nodes = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
java.util.List getNodesList();
/**
*
*
*
* Output only. Info per node.
*
*
*
* repeated .google.cloud.redis.v1beta1.NodeInfo nodes = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.cloud.redis.v1beta1.NodeInfo getNodes(int index);
/**
*
*
*
* Output only. Info per node.
*
*
*
* repeated .google.cloud.redis.v1beta1.NodeInfo nodes = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
int getNodesCount();
/**
*
*
*
* Output only. Info per node.
*
*
*
* repeated .google.cloud.redis.v1beta1.NodeInfo nodes = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
java.util.List extends com.google.cloud.redis.v1beta1.NodeInfoOrBuilder>
getNodesOrBuilderList();
/**
*
*
*
* Output only. Info per node.
*
*
*
* repeated .google.cloud.redis.v1beta1.NodeInfo nodes = 32 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.cloud.redis.v1beta1.NodeInfoOrBuilder getNodesOrBuilder(int index);
/**
*
*
*
* Output only. Hostname or IP address of the exposed readonly Redis
* endpoint. Standard tier only. Targets all healthy replica nodes in
* instance. Replication is asynchronous and replica nodes will exhibit some
* lag behind the primary. Write requests must target 'host'.
*
*
* string read_endpoint = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The readEndpoint.
*/
java.lang.String getReadEndpoint();
/**
*
*
*
* Output only. Hostname or IP address of the exposed readonly Redis
* endpoint. Standard tier only. Targets all healthy replica nodes in
* instance. Replication is asynchronous and replica nodes will exhibit some
* lag behind the primary. Write requests must target 'host'.
*
*
* string read_endpoint = 33 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for readEndpoint.
*/
com.google.protobuf.ByteString getReadEndpointBytes();
/**
*
*
*
* Output only. The port number of the exposed readonly redis
* endpoint. Standard tier only. Write requests should target 'port'.
*
*
* int32 read_endpoint_port = 34 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The readEndpointPort.
*/
int getReadEndpointPort();
/**
*
*
*
* Optional. Read replicas mode for the instance. Defaults to READ_REPLICAS_DISABLED.
*
*
*
* .google.cloud.redis.v1beta1.Instance.ReadReplicasMode read_replicas_mode = 35 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The enum numeric value on the wire for readReplicasMode.
*/
int getReadReplicasModeValue();
/**
*
*
*
* Optional. Read replicas mode for the instance. Defaults to READ_REPLICAS_DISABLED.
*
*
*
* .google.cloud.redis.v1beta1.Instance.ReadReplicasMode read_replicas_mode = 35 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The readReplicasMode.
*/
com.google.cloud.redis.v1beta1.Instance.ReadReplicasMode getReadReplicasMode();
/**
*
*
*
* Optional. Persistence configuration parameters
*
*
*
* .google.cloud.redis.v1beta1.PersistenceConfig persistence_config = 37 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the persistenceConfig field is set.
*/
boolean hasPersistenceConfig();
/**
*
*
*
* Optional. Persistence configuration parameters
*
*
*
* .google.cloud.redis.v1beta1.PersistenceConfig persistence_config = 37 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The persistenceConfig.
*/
com.google.cloud.redis.v1beta1.PersistenceConfig getPersistenceConfig();
/**
*
*
*
* Optional. Persistence configuration parameters
*
*
*
* .google.cloud.redis.v1beta1.PersistenceConfig persistence_config = 37 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.redis.v1beta1.PersistenceConfigOrBuilder getPersistenceConfigOrBuilder();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy