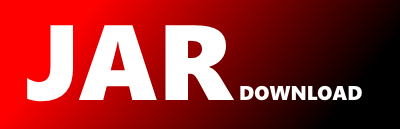
com.google.cloud.retail.v2alpha.CatalogAttributeOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Proto library for google-cloud-retail
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/retail/v2alpha/catalog.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.retail.v2alpha;
public interface CatalogAttributeOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.CatalogAttribute)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Required. Attribute name.
* For example: `color`, `brands`, `attributes.custom_attribute`, such as
* `attributes.xyz`.
* To be indexable, the attribute name can contain only alpha-numeric
* characters and underscores. For example, an attribute named
* `attributes.abc_xyz` can be indexed, but an attribute named
* `attributes.abc-xyz` cannot be indexed.
*
* If the attribute key starts with `attributes.`, then the attribute is a
* custom attribute. Attributes such as `brands`, `patterns`, and `title` are
* built-in and called system attributes.
*
*
* string key = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The key.
*/
java.lang.String getKey();
/**
*
*
*
* Required. Attribute name.
* For example: `color`, `brands`, `attributes.custom_attribute`, such as
* `attributes.xyz`.
* To be indexable, the attribute name can contain only alpha-numeric
* characters and underscores. For example, an attribute named
* `attributes.abc_xyz` can be indexed, but an attribute named
* `attributes.abc-xyz` cannot be indexed.
*
* If the attribute key starts with `attributes.`, then the attribute is a
* custom attribute. Attributes such as `brands`, `patterns`, and `title` are
* built-in and called system attributes.
*
*
* string key = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for key.
*/
com.google.protobuf.ByteString getKeyBytes();
/**
*
*
*
* Output only. Indicates whether this attribute has been used by any
* products. `True` if at least one
* [Product][google.cloud.retail.v2alpha.Product] is using this attribute in
* [Product.attributes][google.cloud.retail.v2alpha.Product.attributes].
* Otherwise, this field is `False`.
*
* [CatalogAttribute][google.cloud.retail.v2alpha.CatalogAttribute] can be
* pre-loaded by using
* [CatalogService.AddCatalogAttribute][google.cloud.retail.v2alpha.CatalogService.AddCatalogAttribute],
* [CatalogService.ImportCatalogAttributes][google.cloud.retail.v2alpha.CatalogService.ImportCatalogAttributes],
* or
* [CatalogService.UpdateAttributesConfig][google.cloud.retail.v2alpha.CatalogService.UpdateAttributesConfig]
* APIs. This field is `False` for pre-loaded
* [CatalogAttribute][google.cloud.retail.v2alpha.CatalogAttribute]s.
*
* Only pre-loaded [catalog
* attributes][google.cloud.retail.v2alpha.CatalogAttribute] that are neither
* in use by products nor predefined can be deleted. [Catalog
* attributes][google.cloud.retail.v2alpha.CatalogAttribute] that are
* either in use by products or are predefined attributes cannot be deleted;
* however, their configuration properties will reset to default values upon
* removal request.
*
* After catalog changes, it takes about 10 minutes for this field to update.
*
*
* bool in_use = 9 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The inUse.
*/
boolean getInUse();
/**
*
*
*
* Output only. The type of this attribute. This is derived from the attribute
* in [Product.attributes][google.cloud.retail.v2alpha.Product.attributes].
*
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.AttributeType type = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The enum numeric value on the wire for type.
*/
int getTypeValue();
/**
*
*
*
* Output only. The type of this attribute. This is derived from the attribute
* in [Product.attributes][google.cloud.retail.v2alpha.Product.attributes].
*
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.AttributeType type = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The type.
*/
com.google.cloud.retail.v2alpha.CatalogAttribute.AttributeType getType();
/**
*
*
*
* When
* [AttributesConfig.attribute_config_level][google.cloud.retail.v2alpha.AttributesConfig.attribute_config_level]
* is CATALOG_LEVEL_ATTRIBUTE_CONFIG, if INDEXABLE_ENABLED attribute values
* are indexed so that it can be filtered, faceted, or boosted in
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search].
*
* Must be specified when
* [AttributesConfig.attribute_config_level][google.cloud.retail.v2alpha.AttributesConfig.attribute_config_level]
* is CATALOG_LEVEL_ATTRIBUTE_CONFIG, otherwise throws INVALID_FORMAT error.
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.IndexableOption indexable_option = 5;
*
*
* @return The enum numeric value on the wire for indexableOption.
*/
int getIndexableOptionValue();
/**
*
*
*
* When
* [AttributesConfig.attribute_config_level][google.cloud.retail.v2alpha.AttributesConfig.attribute_config_level]
* is CATALOG_LEVEL_ATTRIBUTE_CONFIG, if INDEXABLE_ENABLED attribute values
* are indexed so that it can be filtered, faceted, or boosted in
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search].
*
* Must be specified when
* [AttributesConfig.attribute_config_level][google.cloud.retail.v2alpha.AttributesConfig.attribute_config_level]
* is CATALOG_LEVEL_ATTRIBUTE_CONFIG, otherwise throws INVALID_FORMAT error.
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.IndexableOption indexable_option = 5;
*
*
* @return The indexableOption.
*/
com.google.cloud.retail.v2alpha.CatalogAttribute.IndexableOption getIndexableOption();
/**
*
*
*
* If DYNAMIC_FACETABLE_ENABLED, attribute values are available for dynamic
* facet. Could only be DYNAMIC_FACETABLE_DISABLED if
* [CatalogAttribute.indexable_option][google.cloud.retail.v2alpha.CatalogAttribute.indexable_option]
* is INDEXABLE_DISABLED. Otherwise, an INVALID_ARGUMENT error is returned.
*
* Must be specified, otherwise throws INVALID_FORMAT error.
*
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.DynamicFacetableOption dynamic_facetable_option = 6;
*
*
* @return The enum numeric value on the wire for dynamicFacetableOption.
*/
int getDynamicFacetableOptionValue();
/**
*
*
*
* If DYNAMIC_FACETABLE_ENABLED, attribute values are available for dynamic
* facet. Could only be DYNAMIC_FACETABLE_DISABLED if
* [CatalogAttribute.indexable_option][google.cloud.retail.v2alpha.CatalogAttribute.indexable_option]
* is INDEXABLE_DISABLED. Otherwise, an INVALID_ARGUMENT error is returned.
*
* Must be specified, otherwise throws INVALID_FORMAT error.
*
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.DynamicFacetableOption dynamic_facetable_option = 6;
*
*
* @return The dynamicFacetableOption.
*/
com.google.cloud.retail.v2alpha.CatalogAttribute.DynamicFacetableOption
getDynamicFacetableOption();
/**
*
*
*
* When
* [AttributesConfig.attribute_config_level][google.cloud.retail.v2alpha.AttributesConfig.attribute_config_level]
* is CATALOG_LEVEL_ATTRIBUTE_CONFIG, if SEARCHABLE_ENABLED, attribute values
* are searchable by text queries in
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search].
*
* If SEARCHABLE_ENABLED but attribute type is numerical, attribute values
* will not be searchable by text queries in
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search],
* as there are no text values associated to numerical attributes.
*
* Must be specified, when
* [AttributesConfig.attribute_config_level][google.cloud.retail.v2alpha.AttributesConfig.attribute_config_level]
* is CATALOG_LEVEL_ATTRIBUTE_CONFIG, otherwise throws INVALID_FORMAT error.
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.SearchableOption searchable_option = 7;
*
*
* @return The enum numeric value on the wire for searchableOption.
*/
int getSearchableOptionValue();
/**
*
*
*
* When
* [AttributesConfig.attribute_config_level][google.cloud.retail.v2alpha.AttributesConfig.attribute_config_level]
* is CATALOG_LEVEL_ATTRIBUTE_CONFIG, if SEARCHABLE_ENABLED, attribute values
* are searchable by text queries in
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search].
*
* If SEARCHABLE_ENABLED but attribute type is numerical, attribute values
* will not be searchable by text queries in
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search],
* as there are no text values associated to numerical attributes.
*
* Must be specified, when
* [AttributesConfig.attribute_config_level][google.cloud.retail.v2alpha.AttributesConfig.attribute_config_level]
* is CATALOG_LEVEL_ATTRIBUTE_CONFIG, otherwise throws INVALID_FORMAT error.
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.SearchableOption searchable_option = 7;
*
*
* @return The searchableOption.
*/
com.google.cloud.retail.v2alpha.CatalogAttribute.SearchableOption getSearchableOption();
/**
*
*
*
* When
* [AttributesConfig.attribute_config_level][google.cloud.retail.v2alpha.AttributesConfig.attribute_config_level]
* is CATALOG_LEVEL_ATTRIBUTE_CONFIG, if RECOMMENDATIONS_FILTERING_ENABLED,
* attribute values are filterable for recommendations.
* This option works for categorical features only,
* does not work for numerical features, inventory filtering.
*
*
*
* .google.cloud.retail.v2alpha.RecommendationsFilteringOption recommendations_filtering_option = 8;
*
*
* @return The enum numeric value on the wire for recommendationsFilteringOption.
*/
int getRecommendationsFilteringOptionValue();
/**
*
*
*
* When
* [AttributesConfig.attribute_config_level][google.cloud.retail.v2alpha.AttributesConfig.attribute_config_level]
* is CATALOG_LEVEL_ATTRIBUTE_CONFIG, if RECOMMENDATIONS_FILTERING_ENABLED,
* attribute values are filterable for recommendations.
* This option works for categorical features only,
* does not work for numerical features, inventory filtering.
*
*
*
* .google.cloud.retail.v2alpha.RecommendationsFilteringOption recommendations_filtering_option = 8;
*
*
* @return The recommendationsFilteringOption.
*/
com.google.cloud.retail.v2alpha.RecommendationsFilteringOption
getRecommendationsFilteringOption();
/**
*
*
*
* If EXACT_SEARCHABLE_ENABLED, attribute values will be exact searchable.
* This property only applies to textual custom attributes and requires
* indexable set to enabled to enable exact-searchable. If unset, the server
* behavior defaults to
* [EXACT_SEARCHABLE_DISABLED][google.cloud.retail.v2alpha.CatalogAttribute.ExactSearchableOption.EXACT_SEARCHABLE_DISABLED].
*
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.ExactSearchableOption exact_searchable_option = 11;
*
*
* @return The enum numeric value on the wire for exactSearchableOption.
*/
int getExactSearchableOptionValue();
/**
*
*
*
* If EXACT_SEARCHABLE_ENABLED, attribute values will be exact searchable.
* This property only applies to textual custom attributes and requires
* indexable set to enabled to enable exact-searchable. If unset, the server
* behavior defaults to
* [EXACT_SEARCHABLE_DISABLED][google.cloud.retail.v2alpha.CatalogAttribute.ExactSearchableOption.EXACT_SEARCHABLE_DISABLED].
*
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.ExactSearchableOption exact_searchable_option = 11;
*
*
* @return The exactSearchableOption.
*/
com.google.cloud.retail.v2alpha.CatalogAttribute.ExactSearchableOption getExactSearchableOption();
/**
*
*
*
* If RETRIEVABLE_ENABLED, attribute values are retrievable in the search
* results. If unset, the server behavior defaults to
* [RETRIEVABLE_DISABLED][google.cloud.retail.v2alpha.CatalogAttribute.RetrievableOption.RETRIEVABLE_DISABLED].
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.RetrievableOption retrievable_option = 12;
*
*
* @return The enum numeric value on the wire for retrievableOption.
*/
int getRetrievableOptionValue();
/**
*
*
*
* If RETRIEVABLE_ENABLED, attribute values are retrievable in the search
* results. If unset, the server behavior defaults to
* [RETRIEVABLE_DISABLED][google.cloud.retail.v2alpha.CatalogAttribute.RetrievableOption.RETRIEVABLE_DISABLED].
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.RetrievableOption retrievable_option = 12;
*
*
* @return The retrievableOption.
*/
com.google.cloud.retail.v2alpha.CatalogAttribute.RetrievableOption getRetrievableOption();
/**
*
*
*
* Contains facet options.
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.FacetConfig facet_config = 13;
*
* @return Whether the facetConfig field is set.
*/
boolean hasFacetConfig();
/**
*
*
*
* Contains facet options.
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.FacetConfig facet_config = 13;
*
* @return The facetConfig.
*/
com.google.cloud.retail.v2alpha.CatalogAttribute.FacetConfig getFacetConfig();
/**
*
*
*
* Contains facet options.
*
*
* .google.cloud.retail.v2alpha.CatalogAttribute.FacetConfig facet_config = 13;
*/
com.google.cloud.retail.v2alpha.CatalogAttribute.FacetConfigOrBuilder getFacetConfigOrBuilder();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy