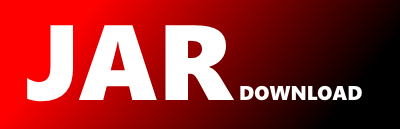
com.google.cloud.retail.v2alpha.ModelOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Proto library for google-cloud-retail
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/retail/v2alpha/model.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.retail.v2alpha;
public interface ModelOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Model)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Optional. The page optimization config.
*
*
*
* .google.cloud.retail.v2alpha.Model.PageOptimizationConfig page_optimization_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the pageOptimizationConfig field is set.
*/
boolean hasPageOptimizationConfig();
/**
*
*
*
* Optional. The page optimization config.
*
*
*
* .google.cloud.retail.v2alpha.Model.PageOptimizationConfig page_optimization_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The pageOptimizationConfig.
*/
com.google.cloud.retail.v2alpha.Model.PageOptimizationConfig getPageOptimizationConfig();
/**
*
*
*
* Optional. The page optimization config.
*
*
*
* .google.cloud.retail.v2alpha.Model.PageOptimizationConfig page_optimization_config = 17 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.retail.v2alpha.Model.PageOptimizationConfigOrBuilder
getPageOptimizationConfigOrBuilder();
/**
*
*
*
* Required. The fully qualified resource name of the model.
*
* Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
* catalog_id has char limit of 50.
* recommendation_model_id has char limit of 40.
*
*
* string name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The name.
*/
java.lang.String getName();
/**
*
*
*
* Required. The fully qualified resource name of the model.
*
* Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
* catalog_id has char limit of 50.
* recommendation_model_id has char limit of 40.
*
*
* string name = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for name.
*/
com.google.protobuf.ByteString getNameBytes();
/**
*
*
*
* Required. The display name of the model.
*
* Should be human readable, used to display Recommendation Models in the
* Retail Cloud Console Dashboard. UTF-8 encoded string with limit of 1024
* characters.
*
*
* string display_name = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return The displayName.
*/
java.lang.String getDisplayName();
/**
*
*
*
* Required. The display name of the model.
*
* Should be human readable, used to display Recommendation Models in the
* Retail Cloud Console Dashboard. UTF-8 encoded string with limit of 1024
* characters.
*
*
* string display_name = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for displayName.
*/
com.google.protobuf.ByteString getDisplayNameBytes();
/**
*
*
*
* Optional. The training state that the model is in (e.g.
* `TRAINING` or `PAUSED`).
*
* Since part of the cost of running the service
* is frequency of training - this can be used to determine when to train
* model in order to control cost. If not specified: the default value for
* `CreateModel` method is `TRAINING`. The default value for
* `UpdateModel` method is to keep the state the same as before.
*
*
*
* .google.cloud.retail.v2alpha.Model.TrainingState training_state = 3 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The enum numeric value on the wire for trainingState.
*/
int getTrainingStateValue();
/**
*
*
*
* Optional. The training state that the model is in (e.g.
* `TRAINING` or `PAUSED`).
*
* Since part of the cost of running the service
* is frequency of training - this can be used to determine when to train
* model in order to control cost. If not specified: the default value for
* `CreateModel` method is `TRAINING`. The default value for
* `UpdateModel` method is to keep the state the same as before.
*
*
*
* .google.cloud.retail.v2alpha.Model.TrainingState training_state = 3 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The trainingState.
*/
com.google.cloud.retail.v2alpha.Model.TrainingState getTrainingState();
/**
*
*
*
* Output only. The serving state of the model: `ACTIVE`, `NOT_ACTIVE`.
*
*
*
* .google.cloud.retail.v2alpha.Model.ServingState serving_state = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The enum numeric value on the wire for servingState.
*/
int getServingStateValue();
/**
*
*
*
* Output only. The serving state of the model: `ACTIVE`, `NOT_ACTIVE`.
*
*
*
* .google.cloud.retail.v2alpha.Model.ServingState serving_state = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The servingState.
*/
com.google.cloud.retail.v2alpha.Model.ServingState getServingState();
/**
*
*
*
* Output only. Timestamp the Recommendation Model was created at.
*
*
* .google.protobuf.Timestamp create_time = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the createTime field is set.
*/
boolean hasCreateTime();
/**
*
*
*
* Output only. Timestamp the Recommendation Model was created at.
*
*
* .google.protobuf.Timestamp create_time = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The createTime.
*/
com.google.protobuf.Timestamp getCreateTime();
/**
*
*
*
* Output only. Timestamp the Recommendation Model was created at.
*
*
* .google.protobuf.Timestamp create_time = 5 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder();
/**
*
*
*
* Output only. Timestamp the Recommendation Model was last updated. E.g.
* if a Recommendation Model was paused - this would be the time the pause was
* initiated.
*
*
* .google.protobuf.Timestamp update_time = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the updateTime field is set.
*/
boolean hasUpdateTime();
/**
*
*
*
* Output only. Timestamp the Recommendation Model was last updated. E.g.
* if a Recommendation Model was paused - this would be the time the pause was
* initiated.
*
*
* .google.protobuf.Timestamp update_time = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The updateTime.
*/
com.google.protobuf.Timestamp getUpdateTime();
/**
*
*
*
* Output only. Timestamp the Recommendation Model was last updated. E.g.
* if a Recommendation Model was paused - this would be the time the pause was
* initiated.
*
*
* .google.protobuf.Timestamp update_time = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.protobuf.TimestampOrBuilder getUpdateTimeOrBuilder();
/**
*
*
*
* Required. The type of model e.g. `home-page`.
*
* Currently supported values: `recommended-for-you`, `others-you-may-like`,
* `frequently-bought-together`, `page-optimization`, `similar-items`,
* `buy-it-again`, `on-sale-items`, and `recently-viewed`(readonly value).
*
*
* This field together with
* [optimization_objective][google.cloud.retail.v2alpha.Model.optimization_objective]
* describe model metadata to use to control model training and serving.
* See https://cloud.google.com/retail/docs/models
* for more details on what the model metadata control and which combination
* of parameters are valid. For invalid combinations of parameters (e.g. type
* = `frequently-bought-together` and optimization_objective = `ctr`), you
* receive an error 400 if you try to create/update a recommendation with
* this set of knobs.
*
*
* string type = 7 [(.google.api.field_behavior) = REQUIRED];
*
* @return The type.
*/
java.lang.String getType();
/**
*
*
*
* Required. The type of model e.g. `home-page`.
*
* Currently supported values: `recommended-for-you`, `others-you-may-like`,
* `frequently-bought-together`, `page-optimization`, `similar-items`,
* `buy-it-again`, `on-sale-items`, and `recently-viewed`(readonly value).
*
*
* This field together with
* [optimization_objective][google.cloud.retail.v2alpha.Model.optimization_objective]
* describe model metadata to use to control model training and serving.
* See https://cloud.google.com/retail/docs/models
* for more details on what the model metadata control and which combination
* of parameters are valid. For invalid combinations of parameters (e.g. type
* = `frequently-bought-together` and optimization_objective = `ctr`), you
* receive an error 400 if you try to create/update a recommendation with
* this set of knobs.
*
*
* string type = 7 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for type.
*/
com.google.protobuf.ByteString getTypeBytes();
/**
*
*
*
* Optional. The optimization objective e.g. `cvr`.
*
* Currently supported
* values: `ctr`, `cvr`, `revenue-per-order`.
*
* If not specified, we choose default based on model type.
* Default depends on type of recommendation:
*
* `recommended-for-you` => `ctr`
*
* `others-you-may-like` => `ctr`
*
* `frequently-bought-together` => `revenue_per_order`
*
* This field together with
* [optimization_objective][google.cloud.retail.v2alpha.Model.type]
* describe model metadata to use to control model training and serving.
* See https://cloud.google.com/retail/docs/models
* for more details on what the model metadata control and which combination
* of parameters are valid. For invalid combinations of parameters (e.g. type
* = `frequently-bought-together` and optimization_objective = `ctr`), you
* receive an error 400 if you try to create/update a recommendation with
* this set of knobs.
*
*
* string optimization_objective = 8 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The optimizationObjective.
*/
java.lang.String getOptimizationObjective();
/**
*
*
*
* Optional. The optimization objective e.g. `cvr`.
*
* Currently supported
* values: `ctr`, `cvr`, `revenue-per-order`.
*
* If not specified, we choose default based on model type.
* Default depends on type of recommendation:
*
* `recommended-for-you` => `ctr`
*
* `others-you-may-like` => `ctr`
*
* `frequently-bought-together` => `revenue_per_order`
*
* This field together with
* [optimization_objective][google.cloud.retail.v2alpha.Model.type]
* describe model metadata to use to control model training and serving.
* See https://cloud.google.com/retail/docs/models
* for more details on what the model metadata control and which combination
* of parameters are valid. For invalid combinations of parameters (e.g. type
* = `frequently-bought-together` and optimization_objective = `ctr`), you
* receive an error 400 if you try to create/update a recommendation with
* this set of knobs.
*
*
* string optimization_objective = 8 [(.google.api.field_behavior) = OPTIONAL];
*
* @return The bytes for optimizationObjective.
*/
com.google.protobuf.ByteString getOptimizationObjectiveBytes();
/**
*
*
*
* Optional. The state of periodic tuning.
*
* The period we use is 3 months - to do a
* one-off tune earlier use the `TuneModel` method. Default value
* is `PERIODIC_TUNING_ENABLED`.
*
*
*
* .google.cloud.retail.v2alpha.Model.PeriodicTuningState periodic_tuning_state = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The enum numeric value on the wire for periodicTuningState.
*/
int getPeriodicTuningStateValue();
/**
*
*
*
* Optional. The state of periodic tuning.
*
* The period we use is 3 months - to do a
* one-off tune earlier use the `TuneModel` method. Default value
* is `PERIODIC_TUNING_ENABLED`.
*
*
*
* .google.cloud.retail.v2alpha.Model.PeriodicTuningState periodic_tuning_state = 11 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The periodicTuningState.
*/
com.google.cloud.retail.v2alpha.Model.PeriodicTuningState getPeriodicTuningState();
/**
*
*
*
* Output only. The timestamp when the latest successful tune finished.
*
*
*
* .google.protobuf.Timestamp last_tune_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the lastTuneTime field is set.
*/
boolean hasLastTuneTime();
/**
*
*
*
* Output only. The timestamp when the latest successful tune finished.
*
*
*
* .google.protobuf.Timestamp last_tune_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The lastTuneTime.
*/
com.google.protobuf.Timestamp getLastTuneTime();
/**
*
*
*
* Output only. The timestamp when the latest successful tune finished.
*
*
*
* .google.protobuf.Timestamp last_tune_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.protobuf.TimestampOrBuilder getLastTuneTimeOrBuilder();
/**
*
*
*
* Output only. The tune operation associated with the model.
*
* Can be used to determine if there is an ongoing tune for this
* recommendation. Empty field implies no tune is goig on.
*
*
* string tuning_operation = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The tuningOperation.
*/
java.lang.String getTuningOperation();
/**
*
*
*
* Output only. The tune operation associated with the model.
*
* Can be used to determine if there is an ongoing tune for this
* recommendation. Empty field implies no tune is goig on.
*
*
* string tuning_operation = 15 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for tuningOperation.
*/
com.google.protobuf.ByteString getTuningOperationBytes();
/**
*
*
*
* Output only. The state of data requirements for this model: `DATA_OK` and
* `DATA_ERROR`.
*
* Recommendation model cannot be trained if the data is in
* `DATA_ERROR` state. Recommendation model can have `DATA_ERROR` state even
* if serving state is `ACTIVE`: models were trained successfully before, but
* cannot be refreshed because model no longer has sufficient
* data for training.
*
*
*
* .google.cloud.retail.v2alpha.Model.DataState data_state = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The enum numeric value on the wire for dataState.
*/
int getDataStateValue();
/**
*
*
*
* Output only. The state of data requirements for this model: `DATA_OK` and
* `DATA_ERROR`.
*
* Recommendation model cannot be trained if the data is in
* `DATA_ERROR` state. Recommendation model can have `DATA_ERROR` state even
* if serving state is `ACTIVE`: models were trained successfully before, but
* cannot be refreshed because model no longer has sufficient
* data for training.
*
*
*
* .google.cloud.retail.v2alpha.Model.DataState data_state = 16 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The dataState.
*/
com.google.cloud.retail.v2alpha.Model.DataState getDataState();
/**
*
*
*
* Optional. If `RECOMMENDATIONS_FILTERING_ENABLED`, recommendation filtering
* by attributes is enabled for the model.
*
*
*
* .google.cloud.retail.v2alpha.RecommendationsFilteringOption filtering_option = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The enum numeric value on the wire for filteringOption.
*/
int getFilteringOptionValue();
/**
*
*
*
* Optional. If `RECOMMENDATIONS_FILTERING_ENABLED`, recommendation filtering
* by attributes is enabled for the model.
*
*
*
* .google.cloud.retail.v2alpha.RecommendationsFilteringOption filtering_option = 18 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The filteringOption.
*/
com.google.cloud.retail.v2alpha.RecommendationsFilteringOption getFilteringOption();
/**
*
*
*
* Output only. The list of valid serving configs associated with the
* PageOptimizationConfig.
*
*
*
* repeated .google.cloud.retail.v2alpha.Model.ServingConfigList serving_config_lists = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
java.util.List
getServingConfigListsList();
/**
*
*
*
* Output only. The list of valid serving configs associated with the
* PageOptimizationConfig.
*
*
*
* repeated .google.cloud.retail.v2alpha.Model.ServingConfigList serving_config_lists = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.cloud.retail.v2alpha.Model.ServingConfigList getServingConfigLists(int index);
/**
*
*
*
* Output only. The list of valid serving configs associated with the
* PageOptimizationConfig.
*
*
*
* repeated .google.cloud.retail.v2alpha.Model.ServingConfigList serving_config_lists = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
int getServingConfigListsCount();
/**
*
*
*
* Output only. The list of valid serving configs associated with the
* PageOptimizationConfig.
*
*
*
* repeated .google.cloud.retail.v2alpha.Model.ServingConfigList serving_config_lists = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
java.util.List extends com.google.cloud.retail.v2alpha.Model.ServingConfigListOrBuilder>
getServingConfigListsOrBuilderList();
/**
*
*
*
* Output only. The list of valid serving configs associated with the
* PageOptimizationConfig.
*
*
*
* repeated .google.cloud.retail.v2alpha.Model.ServingConfigList serving_config_lists = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.cloud.retail.v2alpha.Model.ServingConfigListOrBuilder getServingConfigListsOrBuilder(
int index);
/**
*
*
*
* Optional. Additional model features config.
*
*
*
* .google.cloud.retail.v2alpha.Model.ModelFeaturesConfig model_features_config = 22 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the modelFeaturesConfig field is set.
*/
boolean hasModelFeaturesConfig();
/**
*
*
*
* Optional. Additional model features config.
*
*
*
* .google.cloud.retail.v2alpha.Model.ModelFeaturesConfig model_features_config = 22 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The modelFeaturesConfig.
*/
com.google.cloud.retail.v2alpha.Model.ModelFeaturesConfig getModelFeaturesConfig();
/**
*
*
*
* Optional. Additional model features config.
*
*
*
* .google.cloud.retail.v2alpha.Model.ModelFeaturesConfig model_features_config = 22 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.retail.v2alpha.Model.ModelFeaturesConfigOrBuilder
getModelFeaturesConfigOrBuilder();
com.google.cloud.retail.v2alpha.Model.TrainingConfigCase getTrainingConfigCase();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy