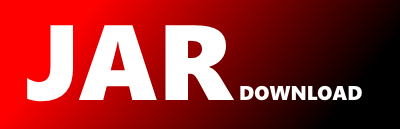
com.google.cloud.retail.v2alpha.Rule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Proto library for google-cloud-retail
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/retail/v2alpha/common.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.retail.v2alpha;
/**
*
*
*
* A rule is a condition-action pair
*
* * A condition defines when a rule is to be triggered.
* * An action specifies what occurs on that trigger.
* Currently rules only work for [controls][google.cloud.retail.v2alpha.Control]
* with
* [SOLUTION_TYPE_SEARCH][google.cloud.retail.v2alpha.SolutionType.SOLUTION_TYPE_SEARCH].
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule}
*/
public final class Rule extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule)
RuleOrBuilder {
private static final long serialVersionUID = 0L;
// Use Rule.newBuilder() to construct.
private Rule(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Rule() {}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Rule();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.class,
com.google.cloud.retail.v2alpha.Rule.Builder.class);
}
public interface BoostActionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Rule.BoostAction)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Strength of the condition boost, which must be in [-1, 1]. Negative
* boost means demotion. Default is 0.0.
*
* Setting to 1.0 gives the item a big promotion. However, it does not
* necessarily mean that the boosted item will be the top result at all
* times, nor that other items will be excluded. Results could still be
* shown even when none of them matches the condition. And results that
* are significantly more relevant to the search query can still trump
* your heavily favored but irrelevant items.
*
* Setting to -1.0 gives the item a big demotion. However, results that
* are deeply relevant might still be shown. The item will have an
* upstream battle to get a fairly high ranking, but it is not blocked out
* completely.
*
* Setting to 0.0 means no boost applied. The boosting condition is
* ignored.
*
*
* float boost = 1;
*
* @return The boost.
*/
float getBoost();
/**
*
*
*
* The filter can have a max size of 5000 characters.
* An expression which specifies which products to apply an action to.
* The syntax and supported fields are the same as a filter expression. See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for detail syntax and limitations.
*
* Examples:
*
* * To boost products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string products_filter = 2;
*
* @return The productsFilter.
*/
java.lang.String getProductsFilter();
/**
*
*
*
* The filter can have a max size of 5000 characters.
* An expression which specifies which products to apply an action to.
* The syntax and supported fields are the same as a filter expression. See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for detail syntax and limitations.
*
* Examples:
*
* * To boost products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string products_filter = 2;
*
* @return The bytes for productsFilter.
*/
com.google.protobuf.ByteString getProductsFilterBytes();
}
/**
*
*
*
* A boost action to apply to results matching condition specified above.
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.BoostAction}
*/
public static final class BoostAction extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule.BoostAction)
BoostActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use BoostAction.newBuilder() to construct.
private BoostAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private BoostAction() {
productsFilter_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new BoostAction();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_BoostAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_BoostAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.BoostAction.class,
com.google.cloud.retail.v2alpha.Rule.BoostAction.Builder.class);
}
public static final int BOOST_FIELD_NUMBER = 1;
private float boost_ = 0F;
/**
*
*
*
* Strength of the condition boost, which must be in [-1, 1]. Negative
* boost means demotion. Default is 0.0.
*
* Setting to 1.0 gives the item a big promotion. However, it does not
* necessarily mean that the boosted item will be the top result at all
* times, nor that other items will be excluded. Results could still be
* shown even when none of them matches the condition. And results that
* are significantly more relevant to the search query can still trump
* your heavily favored but irrelevant items.
*
* Setting to -1.0 gives the item a big demotion. However, results that
* are deeply relevant might still be shown. The item will have an
* upstream battle to get a fairly high ranking, but it is not blocked out
* completely.
*
* Setting to 0.0 means no boost applied. The boosting condition is
* ignored.
*
*
* float boost = 1;
*
* @return The boost.
*/
@java.lang.Override
public float getBoost() {
return boost_;
}
public static final int PRODUCTS_FILTER_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object productsFilter_ = "";
/**
*
*
*
* The filter can have a max size of 5000 characters.
* An expression which specifies which products to apply an action to.
* The syntax and supported fields are the same as a filter expression. See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for detail syntax and limitations.
*
* Examples:
*
* * To boost products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string products_filter = 2;
*
* @return The productsFilter.
*/
@java.lang.Override
public java.lang.String getProductsFilter() {
java.lang.Object ref = productsFilter_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
productsFilter_ = s;
return s;
}
}
/**
*
*
*
* The filter can have a max size of 5000 characters.
* An expression which specifies which products to apply an action to.
* The syntax and supported fields are the same as a filter expression. See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for detail syntax and limitations.
*
* Examples:
*
* * To boost products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string products_filter = 2;
*
* @return The bytes for productsFilter.
*/
@java.lang.Override
public com.google.protobuf.ByteString getProductsFilterBytes() {
java.lang.Object ref = productsFilter_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
productsFilter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (java.lang.Float.floatToRawIntBits(boost_) != 0) {
output.writeFloat(1, boost_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(productsFilter_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, productsFilter_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (java.lang.Float.floatToRawIntBits(boost_) != 0) {
size += com.google.protobuf.CodedOutputStream.computeFloatSize(1, boost_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(productsFilter_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, productsFilter_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.Rule.BoostAction)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule.BoostAction other =
(com.google.cloud.retail.v2alpha.Rule.BoostAction) obj;
if (java.lang.Float.floatToIntBits(getBoost())
!= java.lang.Float.floatToIntBits(other.getBoost())) return false;
if (!getProductsFilter().equals(other.getProductsFilter())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + BOOST_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(getBoost());
hash = (37 * hash) + PRODUCTS_FILTER_FIELD_NUMBER;
hash = (53 * hash) + getProductsFilter().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.retail.v2alpha.Rule.BoostAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* A boost action to apply to results matching condition specified above.
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.BoostAction}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule.BoostAction)
com.google.cloud.retail.v2alpha.Rule.BoostActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_BoostAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_BoostAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.BoostAction.class,
com.google.cloud.retail.v2alpha.Rule.BoostAction.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.Rule.BoostAction.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
boost_ = 0F;
productsFilter_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_BoostAction_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.BoostAction getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.BoostAction.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.BoostAction build() {
com.google.cloud.retail.v2alpha.Rule.BoostAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.BoostAction buildPartial() {
com.google.cloud.retail.v2alpha.Rule.BoostAction result =
new com.google.cloud.retail.v2alpha.Rule.BoostAction(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.retail.v2alpha.Rule.BoostAction result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.boost_ = boost_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.productsFilter_ = productsFilter_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.Rule.BoostAction) {
return mergeFrom((com.google.cloud.retail.v2alpha.Rule.BoostAction) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.Rule.BoostAction other) {
if (other == com.google.cloud.retail.v2alpha.Rule.BoostAction.getDefaultInstance())
return this;
if (other.getBoost() != 0F) {
setBoost(other.getBoost());
}
if (!other.getProductsFilter().isEmpty()) {
productsFilter_ = other.productsFilter_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 13:
{
boost_ = input.readFloat();
bitField0_ |= 0x00000001;
break;
} // case 13
case 18:
{
productsFilter_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private float boost_;
/**
*
*
*
* Strength of the condition boost, which must be in [-1, 1]. Negative
* boost means demotion. Default is 0.0.
*
* Setting to 1.0 gives the item a big promotion. However, it does not
* necessarily mean that the boosted item will be the top result at all
* times, nor that other items will be excluded. Results could still be
* shown even when none of them matches the condition. And results that
* are significantly more relevant to the search query can still trump
* your heavily favored but irrelevant items.
*
* Setting to -1.0 gives the item a big demotion. However, results that
* are deeply relevant might still be shown. The item will have an
* upstream battle to get a fairly high ranking, but it is not blocked out
* completely.
*
* Setting to 0.0 means no boost applied. The boosting condition is
* ignored.
*
*
* float boost = 1;
*
* @return The boost.
*/
@java.lang.Override
public float getBoost() {
return boost_;
}
/**
*
*
*
* Strength of the condition boost, which must be in [-1, 1]. Negative
* boost means demotion. Default is 0.0.
*
* Setting to 1.0 gives the item a big promotion. However, it does not
* necessarily mean that the boosted item will be the top result at all
* times, nor that other items will be excluded. Results could still be
* shown even when none of them matches the condition. And results that
* are significantly more relevant to the search query can still trump
* your heavily favored but irrelevant items.
*
* Setting to -1.0 gives the item a big demotion. However, results that
* are deeply relevant might still be shown. The item will have an
* upstream battle to get a fairly high ranking, but it is not blocked out
* completely.
*
* Setting to 0.0 means no boost applied. The boosting condition is
* ignored.
*
*
* float boost = 1;
*
* @param value The boost to set.
* @return This builder for chaining.
*/
public Builder setBoost(float value) {
boost_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Strength of the condition boost, which must be in [-1, 1]. Negative
* boost means demotion. Default is 0.0.
*
* Setting to 1.0 gives the item a big promotion. However, it does not
* necessarily mean that the boosted item will be the top result at all
* times, nor that other items will be excluded. Results could still be
* shown even when none of them matches the condition. And results that
* are significantly more relevant to the search query can still trump
* your heavily favored but irrelevant items.
*
* Setting to -1.0 gives the item a big demotion. However, results that
* are deeply relevant might still be shown. The item will have an
* upstream battle to get a fairly high ranking, but it is not blocked out
* completely.
*
* Setting to 0.0 means no boost applied. The boosting condition is
* ignored.
*
*
* float boost = 1;
*
* @return This builder for chaining.
*/
public Builder clearBoost() {
bitField0_ = (bitField0_ & ~0x00000001);
boost_ = 0F;
onChanged();
return this;
}
private java.lang.Object productsFilter_ = "";
/**
*
*
*
* The filter can have a max size of 5000 characters.
* An expression which specifies which products to apply an action to.
* The syntax and supported fields are the same as a filter expression. See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for detail syntax and limitations.
*
* Examples:
*
* * To boost products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string products_filter = 2;
*
* @return The productsFilter.
*/
public java.lang.String getProductsFilter() {
java.lang.Object ref = productsFilter_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
productsFilter_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The filter can have a max size of 5000 characters.
* An expression which specifies which products to apply an action to.
* The syntax and supported fields are the same as a filter expression. See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for detail syntax and limitations.
*
* Examples:
*
* * To boost products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string products_filter = 2;
*
* @return The bytes for productsFilter.
*/
public com.google.protobuf.ByteString getProductsFilterBytes() {
java.lang.Object ref = productsFilter_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
productsFilter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The filter can have a max size of 5000 characters.
* An expression which specifies which products to apply an action to.
* The syntax and supported fields are the same as a filter expression. See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for detail syntax and limitations.
*
* Examples:
*
* * To boost products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string products_filter = 2;
*
* @param value The productsFilter to set.
* @return This builder for chaining.
*/
public Builder setProductsFilter(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
productsFilter_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* The filter can have a max size of 5000 characters.
* An expression which specifies which products to apply an action to.
* The syntax and supported fields are the same as a filter expression. See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for detail syntax and limitations.
*
* Examples:
*
* * To boost products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string products_filter = 2;
*
* @return This builder for chaining.
*/
public Builder clearProductsFilter() {
productsFilter_ = getDefaultInstance().getProductsFilter();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* The filter can have a max size of 5000 characters.
* An expression which specifies which products to apply an action to.
* The syntax and supported fields are the same as a filter expression. See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for detail syntax and limitations.
*
* Examples:
*
* * To boost products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string products_filter = 2;
*
* @param value The bytes for productsFilter to set.
* @return This builder for chaining.
*/
public Builder setProductsFilterBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
productsFilter_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule.BoostAction)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule.BoostAction)
private static final com.google.cloud.retail.v2alpha.Rule.BoostAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.Rule.BoostAction();
}
public static com.google.cloud.retail.v2alpha.Rule.BoostAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BoostAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.BoostAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FilterActionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Rule.FilterAction)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* A filter to apply on the matching condition results. Supported features:
*
* * [filter][google.cloud.retail.v2alpha.Rule.FilterAction.filter] must be
* set.
* * Filter syntax is identical to
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter].
* For more
* information, see [Filter](/retail/docs/filter-and-order#filter).
* * To filter products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string filter = 1;
*
* @return The filter.
*/
java.lang.String getFilter();
/**
*
*
*
* A filter to apply on the matching condition results. Supported features:
*
* * [filter][google.cloud.retail.v2alpha.Rule.FilterAction.filter] must be
* set.
* * Filter syntax is identical to
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter].
* For more
* information, see [Filter](/retail/docs/filter-and-order#filter).
* * To filter products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string filter = 1;
*
* @return The bytes for filter.
*/
com.google.protobuf.ByteString getFilterBytes();
}
/**
*
*
*
* * Rule Condition:
* - No
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* provided is a global match.
* - 1 or more
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* provided are combined with OR operator.
*
* * Action Input: The request query and filter that are applied to the
* retrieved products, in addition to any filters already provided with the
* SearchRequest. The AND operator is used to combine the query's existing
* filters with the filter rule(s). NOTE: May result in 0 results when
* filters conflict.
*
* * Action Result: Filters the returned objects to be ONLY those that passed
* the filter.
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.FilterAction}
*/
public static final class FilterAction extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule.FilterAction)
FilterActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use FilterAction.newBuilder() to construct.
private FilterAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FilterAction() {
filter_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new FilterAction();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_FilterAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_FilterAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.FilterAction.class,
com.google.cloud.retail.v2alpha.Rule.FilterAction.Builder.class);
}
public static final int FILTER_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object filter_ = "";
/**
*
*
*
* A filter to apply on the matching condition results. Supported features:
*
* * [filter][google.cloud.retail.v2alpha.Rule.FilterAction.filter] must be
* set.
* * Filter syntax is identical to
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter].
* For more
* information, see [Filter](/retail/docs/filter-and-order#filter).
* * To filter products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string filter = 1;
*
* @return The filter.
*/
@java.lang.Override
public java.lang.String getFilter() {
java.lang.Object ref = filter_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
filter_ = s;
return s;
}
}
/**
*
*
*
* A filter to apply on the matching condition results. Supported features:
*
* * [filter][google.cloud.retail.v2alpha.Rule.FilterAction.filter] must be
* set.
* * Filter syntax is identical to
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter].
* For more
* information, see [Filter](/retail/docs/filter-and-order#filter).
* * To filter products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string filter = 1;
*
* @return The bytes for filter.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFilterBytes() {
java.lang.Object ref = filter_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
filter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(filter_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, filter_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(filter_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, filter_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.Rule.FilterAction)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule.FilterAction other =
(com.google.cloud.retail.v2alpha.Rule.FilterAction) obj;
if (!getFilter().equals(other.getFilter())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FILTER_FIELD_NUMBER;
hash = (53 * hash) + getFilter().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.retail.v2alpha.Rule.FilterAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* * Rule Condition:
* - No
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* provided is a global match.
* - 1 or more
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* provided are combined with OR operator.
*
* * Action Input: The request query and filter that are applied to the
* retrieved products, in addition to any filters already provided with the
* SearchRequest. The AND operator is used to combine the query's existing
* filters with the filter rule(s). NOTE: May result in 0 results when
* filters conflict.
*
* * Action Result: Filters the returned objects to be ONLY those that passed
* the filter.
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.FilterAction}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule.FilterAction)
com.google.cloud.retail.v2alpha.Rule.FilterActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_FilterAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_FilterAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.FilterAction.class,
com.google.cloud.retail.v2alpha.Rule.FilterAction.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.Rule.FilterAction.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
filter_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_FilterAction_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.FilterAction getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.FilterAction.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.FilterAction build() {
com.google.cloud.retail.v2alpha.Rule.FilterAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.FilterAction buildPartial() {
com.google.cloud.retail.v2alpha.Rule.FilterAction result =
new com.google.cloud.retail.v2alpha.Rule.FilterAction(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.retail.v2alpha.Rule.FilterAction result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.filter_ = filter_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.Rule.FilterAction) {
return mergeFrom((com.google.cloud.retail.v2alpha.Rule.FilterAction) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.Rule.FilterAction other) {
if (other == com.google.cloud.retail.v2alpha.Rule.FilterAction.getDefaultInstance())
return this;
if (!other.getFilter().isEmpty()) {
filter_ = other.filter_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
filter_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object filter_ = "";
/**
*
*
*
* A filter to apply on the matching condition results. Supported features:
*
* * [filter][google.cloud.retail.v2alpha.Rule.FilterAction.filter] must be
* set.
* * Filter syntax is identical to
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter].
* For more
* information, see [Filter](/retail/docs/filter-and-order#filter).
* * To filter products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string filter = 1;
*
* @return The filter.
*/
public java.lang.String getFilter() {
java.lang.Object ref = filter_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
filter_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* A filter to apply on the matching condition results. Supported features:
*
* * [filter][google.cloud.retail.v2alpha.Rule.FilterAction.filter] must be
* set.
* * Filter syntax is identical to
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter].
* For more
* information, see [Filter](/retail/docs/filter-and-order#filter).
* * To filter products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string filter = 1;
*
* @return The bytes for filter.
*/
public com.google.protobuf.ByteString getFilterBytes() {
java.lang.Object ref = filter_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
filter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* A filter to apply on the matching condition results. Supported features:
*
* * [filter][google.cloud.retail.v2alpha.Rule.FilterAction.filter] must be
* set.
* * Filter syntax is identical to
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter].
* For more
* information, see [Filter](/retail/docs/filter-and-order#filter).
* * To filter products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string filter = 1;
*
* @param value The filter to set.
* @return This builder for chaining.
*/
public Builder setFilter(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
filter_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* A filter to apply on the matching condition results. Supported features:
*
* * [filter][google.cloud.retail.v2alpha.Rule.FilterAction.filter] must be
* set.
* * Filter syntax is identical to
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter].
* For more
* information, see [Filter](/retail/docs/filter-and-order#filter).
* * To filter products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string filter = 1;
*
* @return This builder for chaining.
*/
public Builder clearFilter() {
filter_ = getDefaultInstance().getFilter();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* A filter to apply on the matching condition results. Supported features:
*
* * [filter][google.cloud.retail.v2alpha.Rule.FilterAction.filter] must be
* set.
* * Filter syntax is identical to
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter].
* For more
* information, see [Filter](/retail/docs/filter-and-order#filter).
* * To filter products with product ID "product_1" or "product_2", and
* color
* "Red" or "Blue":<br>
* *(id: ANY("product_1", "product_2"))<br>*
* *AND<br>*
* *(colorFamilies: ANY("Red", "Blue"))<br>*
*
*
* string filter = 1;
*
* @param value The bytes for filter to set.
* @return This builder for chaining.
*/
public Builder setFilterBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
filter_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule.FilterAction)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule.FilterAction)
private static final com.google.cloud.retail.v2alpha.Rule.FilterAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.Rule.FilterAction();
}
public static com.google.cloud.retail.v2alpha.Rule.FilterAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FilterAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.FilterAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RedirectActionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Rule.RedirectAction)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* URL must have length equal or less than 2000 characters.
*
*
* string redirect_uri = 1;
*
* @return The redirectUri.
*/
java.lang.String getRedirectUri();
/**
*
*
*
* URL must have length equal or less than 2000 characters.
*
*
* string redirect_uri = 1;
*
* @return The bytes for redirectUri.
*/
com.google.protobuf.ByteString getRedirectUriBytes();
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
* * Rule Condition:
* Must specify
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms].
* * Action Input: Request Query
* * Action Result: Redirects shopper to provided uri.
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.RedirectAction}
*/
public static final class RedirectAction extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule.RedirectAction)
RedirectActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use RedirectAction.newBuilder() to construct.
private RedirectAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RedirectAction() {
redirectUri_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new RedirectAction();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_RedirectAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_RedirectAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.RedirectAction.class,
com.google.cloud.retail.v2alpha.Rule.RedirectAction.Builder.class);
}
public static final int REDIRECT_URI_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object redirectUri_ = "";
/**
*
*
*
* URL must have length equal or less than 2000 characters.
*
*
* string redirect_uri = 1;
*
* @return The redirectUri.
*/
@java.lang.Override
public java.lang.String getRedirectUri() {
java.lang.Object ref = redirectUri_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
redirectUri_ = s;
return s;
}
}
/**
*
*
*
* URL must have length equal or less than 2000 characters.
*
*
* string redirect_uri = 1;
*
* @return The bytes for redirectUri.
*/
@java.lang.Override
public com.google.protobuf.ByteString getRedirectUriBytes() {
java.lang.Object ref = redirectUri_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
redirectUri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(redirectUri_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, redirectUri_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(redirectUri_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, redirectUri_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.Rule.RedirectAction)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule.RedirectAction other =
(com.google.cloud.retail.v2alpha.Rule.RedirectAction) obj;
if (!getRedirectUri().equals(other.getRedirectUri())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + REDIRECT_URI_FIELD_NUMBER;
hash = (53 * hash) + getRedirectUri().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.retail.v2alpha.Rule.RedirectAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
* * Rule Condition:
* Must specify
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms].
* * Action Input: Request Query
* * Action Result: Redirects shopper to provided uri.
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.RedirectAction}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule.RedirectAction)
com.google.cloud.retail.v2alpha.Rule.RedirectActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_RedirectAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_RedirectAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.RedirectAction.class,
com.google.cloud.retail.v2alpha.Rule.RedirectAction.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.Rule.RedirectAction.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
redirectUri_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_RedirectAction_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RedirectAction getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.RedirectAction.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RedirectAction build() {
com.google.cloud.retail.v2alpha.Rule.RedirectAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RedirectAction buildPartial() {
com.google.cloud.retail.v2alpha.Rule.RedirectAction result =
new com.google.cloud.retail.v2alpha.Rule.RedirectAction(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.retail.v2alpha.Rule.RedirectAction result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.redirectUri_ = redirectUri_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.Rule.RedirectAction) {
return mergeFrom((com.google.cloud.retail.v2alpha.Rule.RedirectAction) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.Rule.RedirectAction other) {
if (other == com.google.cloud.retail.v2alpha.Rule.RedirectAction.getDefaultInstance())
return this;
if (!other.getRedirectUri().isEmpty()) {
redirectUri_ = other.redirectUri_;
bitField0_ |= 0x00000001;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
redirectUri_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object redirectUri_ = "";
/**
*
*
*
* URL must have length equal or less than 2000 characters.
*
*
* string redirect_uri = 1;
*
* @return The redirectUri.
*/
public java.lang.String getRedirectUri() {
java.lang.Object ref = redirectUri_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
redirectUri_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* URL must have length equal or less than 2000 characters.
*
*
* string redirect_uri = 1;
*
* @return The bytes for redirectUri.
*/
public com.google.protobuf.ByteString getRedirectUriBytes() {
java.lang.Object ref = redirectUri_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
redirectUri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* URL must have length equal or less than 2000 characters.
*
*
* string redirect_uri = 1;
*
* @param value The redirectUri to set.
* @return This builder for chaining.
*/
public Builder setRedirectUri(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
redirectUri_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* URL must have length equal or less than 2000 characters.
*
*
* string redirect_uri = 1;
*
* @return This builder for chaining.
*/
public Builder clearRedirectUri() {
redirectUri_ = getDefaultInstance().getRedirectUri();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* URL must have length equal or less than 2000 characters.
*
*
* string redirect_uri = 1;
*
* @param value The bytes for redirectUri to set.
* @return This builder for chaining.
*/
public Builder setRedirectUriBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
redirectUri_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule.RedirectAction)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule.RedirectAction)
private static final com.google.cloud.retail.v2alpha.Rule.RedirectAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.Rule.RedirectAction();
}
public static com.google.cloud.retail.v2alpha.Rule.RedirectAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RedirectAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RedirectAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TwowaySynonymsActionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @return A list containing the synonyms.
*/
java.util.List getSynonymsList();
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @return The count of synonyms.
*/
int getSynonymsCount();
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @param index The index of the element to return.
* @return The synonyms at the given index.
*/
java.lang.String getSynonyms(int index);
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @param index The index of the value to return.
* @return The bytes of the synonyms at the given index.
*/
com.google.protobuf.ByteString getSynonymsBytes(int index);
}
/**
*
*
*
* Creates a set of terms that will be treated as synonyms of each other.
* Example: synonyms of "sneakers" and "shoes":
*
* * "sneakers" will use a synonym of "shoes".
* * "shoes" will use a synonym of "sneakers".
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction}
*/
public static final class TwowaySynonymsAction extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction)
TwowaySynonymsActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use TwowaySynonymsAction.newBuilder() to construct.
private TwowaySynonymsAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TwowaySynonymsAction() {
synonyms_ = com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new TwowaySynonymsAction();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_TwowaySynonymsAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_TwowaySynonymsAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.class,
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.Builder.class);
}
public static final int SYNONYMS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList synonyms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @return A list containing the synonyms.
*/
public com.google.protobuf.ProtocolStringList getSynonymsList() {
return synonyms_;
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @return The count of synonyms.
*/
public int getSynonymsCount() {
return synonyms_.size();
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @param index The index of the element to return.
* @return The synonyms at the given index.
*/
public java.lang.String getSynonyms(int index) {
return synonyms_.get(index);
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @param index The index of the value to return.
* @return The bytes of the synonyms at the given index.
*/
public com.google.protobuf.ByteString getSynonymsBytes(int index) {
return synonyms_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
for (int i = 0; i < synonyms_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, synonyms_.getRaw(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < synonyms_.size(); i++) {
dataSize += computeStringSizeNoTag(synonyms_.getRaw(i));
}
size += dataSize;
size += 1 * getSynonymsList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction other =
(com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction) obj;
if (!getSynonymsList().equals(other.getSynonymsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getSynonymsCount() > 0) {
hash = (37 * hash) + SYNONYMS_FIELD_NUMBER;
hash = (53 * hash) + getSynonymsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Creates a set of terms that will be treated as synonyms of each other.
* Example: synonyms of "sneakers" and "shoes":
*
* * "sneakers" will use a synonym of "shoes".
* * "shoes" will use a synonym of "sneakers".
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction)
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_TwowaySynonymsAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_TwowaySynonymsAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.class,
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
synonyms_ = com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_TwowaySynonymsAction_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction build() {
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction buildPartial() {
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction result =
new com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
synonyms_.makeImmutable();
result.synonyms_ = synonyms_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction) {
return mergeFrom((com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction other) {
if (other == com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.getDefaultInstance())
return this;
if (!other.synonyms_.isEmpty()) {
if (synonyms_.isEmpty()) {
synonyms_ = other.synonyms_;
bitField0_ |= 0x00000001;
} else {
ensureSynonymsIsMutable();
synonyms_.addAll(other.synonyms_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
java.lang.String s = input.readStringRequireUtf8();
ensureSynonymsIsMutable();
synonyms_.add(s);
break;
} // case 10
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList synonyms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureSynonymsIsMutable() {
if (!synonyms_.isModifiable()) {
synonyms_ = new com.google.protobuf.LazyStringArrayList(synonyms_);
}
bitField0_ |= 0x00000001;
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @return A list containing the synonyms.
*/
public com.google.protobuf.ProtocolStringList getSynonymsList() {
synonyms_.makeImmutable();
return synonyms_;
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @return The count of synonyms.
*/
public int getSynonymsCount() {
return synonyms_.size();
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @param index The index of the element to return.
* @return The synonyms at the given index.
*/
public java.lang.String getSynonyms(int index) {
return synonyms_.get(index);
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @param index The index of the value to return.
* @return The bytes of the synonyms at the given index.
*/
public com.google.protobuf.ByteString getSynonymsBytes(int index) {
return synonyms_.getByteString(index);
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @param index The index to set the value at.
* @param value The synonyms to set.
* @return This builder for chaining.
*/
public Builder setSynonyms(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSynonymsIsMutable();
synonyms_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @param value The synonyms to add.
* @return This builder for chaining.
*/
public Builder addSynonyms(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSynonymsIsMutable();
synonyms_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @param values The synonyms to add.
* @return This builder for chaining.
*/
public Builder addAllSynonyms(java.lang.Iterable values) {
ensureSynonymsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, synonyms_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @return This builder for chaining.
*/
public Builder clearSynonyms() {
synonyms_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
;
onChanged();
return this;
}
/**
*
*
*
* Defines a set of synonyms.
* Can specify up to 100 synonyms.
* Must specify at least 2 synonyms.
*
*
* repeated string synonyms = 1;
*
* @param value The bytes of the synonyms to add.
* @return This builder for chaining.
*/
public Builder addSynonymsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSynonymsIsMutable();
synonyms_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction)
private static final com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction();
}
public static com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TwowaySynonymsAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OnewaySynonymsActionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @return A list containing the queryTerms.
*/
java.util.List getQueryTermsList();
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @return The count of queryTerms.
*/
int getQueryTermsCount();
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @param index The index of the element to return.
* @return The queryTerms at the given index.
*/
java.lang.String getQueryTerms(int index);
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @param index The index of the value to return.
* @return The bytes of the queryTerms at the given index.
*/
com.google.protobuf.ByteString getQueryTermsBytes(int index);
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @return A list containing the synonyms.
*/
java.util.List getSynonymsList();
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @return The count of synonyms.
*/
int getSynonymsCount();
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @param index The index of the element to return.
* @return The synonyms at the given index.
*/
java.lang.String getSynonyms(int index);
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @param index The index of the value to return.
* @return The bytes of the synonyms at the given index.
*/
com.google.protobuf.ByteString getSynonymsBytes(int index);
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @return A list containing the onewayTerms.
*/
java.util.List getOnewayTermsList();
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @return The count of onewayTerms.
*/
int getOnewayTermsCount();
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @param index The index of the element to return.
* @return The onewayTerms at the given index.
*/
java.lang.String getOnewayTerms(int index);
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @param index The index of the value to return.
* @return The bytes of the onewayTerms at the given index.
*/
com.google.protobuf.ByteString getOnewayTermsBytes(int index);
}
/**
*
*
*
* Maps a set of terms to a set of synonyms.
* Set of synonyms will be treated as synonyms of each query term only.
* `query_terms` will not be treated as synonyms of each other.
* Example: "sneakers" will use a synonym of "shoes".
* "shoes" will not use a synonym of "sneakers".
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction}
*/
public static final class OnewaySynonymsAction extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction)
OnewaySynonymsActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use OnewaySynonymsAction.newBuilder() to construct.
private OnewaySynonymsAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OnewaySynonymsAction() {
queryTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
synonyms_ = com.google.protobuf.LazyStringArrayList.emptyList();
onewayTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new OnewaySynonymsAction();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_OnewaySynonymsAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_OnewaySynonymsAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.class,
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.Builder.class);
}
public static final int QUERY_TERMS_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList queryTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @return A list containing the queryTerms.
*/
public com.google.protobuf.ProtocolStringList getQueryTermsList() {
return queryTerms_;
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @return The count of queryTerms.
*/
public int getQueryTermsCount() {
return queryTerms_.size();
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @param index The index of the element to return.
* @return The queryTerms at the given index.
*/
public java.lang.String getQueryTerms(int index) {
return queryTerms_.get(index);
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @param index The index of the value to return.
* @return The bytes of the queryTerms at the given index.
*/
public com.google.protobuf.ByteString getQueryTermsBytes(int index) {
return queryTerms_.getByteString(index);
}
public static final int SYNONYMS_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList synonyms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @return A list containing the synonyms.
*/
public com.google.protobuf.ProtocolStringList getSynonymsList() {
return synonyms_;
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @return The count of synonyms.
*/
public int getSynonymsCount() {
return synonyms_.size();
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @param index The index of the element to return.
* @return The synonyms at the given index.
*/
public java.lang.String getSynonyms(int index) {
return synonyms_.get(index);
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @param index The index of the value to return.
* @return The bytes of the synonyms at the given index.
*/
public com.google.protobuf.ByteString getSynonymsBytes(int index) {
return synonyms_.getByteString(index);
}
public static final int ONEWAY_TERMS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList onewayTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @return A list containing the onewayTerms.
*/
public com.google.protobuf.ProtocolStringList getOnewayTermsList() {
return onewayTerms_;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @return The count of onewayTerms.
*/
public int getOnewayTermsCount() {
return onewayTerms_.size();
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @param index The index of the element to return.
* @return The onewayTerms at the given index.
*/
public java.lang.String getOnewayTerms(int index) {
return onewayTerms_.get(index);
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @param index The index of the value to return.
* @return The bytes of the onewayTerms at the given index.
*/
public com.google.protobuf.ByteString getOnewayTermsBytes(int index) {
return onewayTerms_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
for (int i = 0; i < onewayTerms_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, onewayTerms_.getRaw(i));
}
for (int i = 0; i < queryTerms_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, queryTerms_.getRaw(i));
}
for (int i = 0; i < synonyms_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, synonyms_.getRaw(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < onewayTerms_.size(); i++) {
dataSize += computeStringSizeNoTag(onewayTerms_.getRaw(i));
}
size += dataSize;
size += 1 * getOnewayTermsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < queryTerms_.size(); i++) {
dataSize += computeStringSizeNoTag(queryTerms_.getRaw(i));
}
size += dataSize;
size += 1 * getQueryTermsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < synonyms_.size(); i++) {
dataSize += computeStringSizeNoTag(synonyms_.getRaw(i));
}
size += dataSize;
size += 1 * getSynonymsList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction other =
(com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction) obj;
if (!getQueryTermsList().equals(other.getQueryTermsList())) return false;
if (!getSynonymsList().equals(other.getSynonymsList())) return false;
if (!getOnewayTermsList().equals(other.getOnewayTermsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getQueryTermsCount() > 0) {
hash = (37 * hash) + QUERY_TERMS_FIELD_NUMBER;
hash = (53 * hash) + getQueryTermsList().hashCode();
}
if (getSynonymsCount() > 0) {
hash = (37 * hash) + SYNONYMS_FIELD_NUMBER;
hash = (53 * hash) + getSynonymsList().hashCode();
}
if (getOnewayTermsCount() > 0) {
hash = (37 * hash) + ONEWAY_TERMS_FIELD_NUMBER;
hash = (53 * hash) + getOnewayTermsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Maps a set of terms to a set of synonyms.
* Set of synonyms will be treated as synonyms of each query term only.
* `query_terms` will not be treated as synonyms of each other.
* Example: "sneakers" will use a synonym of "shoes".
* "shoes" will not use a synonym of "sneakers".
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction)
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_OnewaySynonymsAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_OnewaySynonymsAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.class,
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
queryTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
synonyms_ = com.google.protobuf.LazyStringArrayList.emptyList();
onewayTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_OnewaySynonymsAction_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction build() {
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction buildPartial() {
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction result =
new com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
queryTerms_.makeImmutable();
result.queryTerms_ = queryTerms_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
synonyms_.makeImmutable();
result.synonyms_ = synonyms_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
onewayTerms_.makeImmutable();
result.onewayTerms_ = onewayTerms_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction) {
return mergeFrom((com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction other) {
if (other == com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.getDefaultInstance())
return this;
if (!other.queryTerms_.isEmpty()) {
if (queryTerms_.isEmpty()) {
queryTerms_ = other.queryTerms_;
bitField0_ |= 0x00000001;
} else {
ensureQueryTermsIsMutable();
queryTerms_.addAll(other.queryTerms_);
}
onChanged();
}
if (!other.synonyms_.isEmpty()) {
if (synonyms_.isEmpty()) {
synonyms_ = other.synonyms_;
bitField0_ |= 0x00000002;
} else {
ensureSynonymsIsMutable();
synonyms_.addAll(other.synonyms_);
}
onChanged();
}
if (!other.onewayTerms_.isEmpty()) {
if (onewayTerms_.isEmpty()) {
onewayTerms_ = other.onewayTerms_;
bitField0_ |= 0x00000004;
} else {
ensureOnewayTermsIsMutable();
onewayTerms_.addAll(other.onewayTerms_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 18:
{
java.lang.String s = input.readStringRequireUtf8();
ensureOnewayTermsIsMutable();
onewayTerms_.add(s);
break;
} // case 18
case 26:
{
java.lang.String s = input.readStringRequireUtf8();
ensureQueryTermsIsMutable();
queryTerms_.add(s);
break;
} // case 26
case 34:
{
java.lang.String s = input.readStringRequireUtf8();
ensureSynonymsIsMutable();
synonyms_.add(s);
break;
} // case 34
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList queryTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureQueryTermsIsMutable() {
if (!queryTerms_.isModifiable()) {
queryTerms_ = new com.google.protobuf.LazyStringArrayList(queryTerms_);
}
bitField0_ |= 0x00000001;
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @return A list containing the queryTerms.
*/
public com.google.protobuf.ProtocolStringList getQueryTermsList() {
queryTerms_.makeImmutable();
return queryTerms_;
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @return The count of queryTerms.
*/
public int getQueryTermsCount() {
return queryTerms_.size();
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @param index The index of the element to return.
* @return The queryTerms at the given index.
*/
public java.lang.String getQueryTerms(int index) {
return queryTerms_.get(index);
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @param index The index of the value to return.
* @return The bytes of the queryTerms at the given index.
*/
public com.google.protobuf.ByteString getQueryTermsBytes(int index) {
return queryTerms_.getByteString(index);
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @param index The index to set the value at.
* @param value The queryTerms to set.
* @return This builder for chaining.
*/
public Builder setQueryTerms(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryTermsIsMutable();
queryTerms_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @param value The queryTerms to add.
* @return This builder for chaining.
*/
public Builder addQueryTerms(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryTermsIsMutable();
queryTerms_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @param values The queryTerms to add.
* @return This builder for chaining.
*/
public Builder addAllQueryTerms(java.lang.Iterable values) {
ensureQueryTermsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, queryTerms_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @return This builder for chaining.
*/
public Builder clearQueryTerms() {
queryTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will treat synonyms as their synonyms.
* Not themselves synonyms of the synonyms.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 3;
*
* @param value The bytes of the queryTerms to add.
* @return This builder for chaining.
*/
public Builder addQueryTermsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureQueryTermsIsMutable();
queryTerms_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList synonyms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureSynonymsIsMutable() {
if (!synonyms_.isModifiable()) {
synonyms_ = new com.google.protobuf.LazyStringArrayList(synonyms_);
}
bitField0_ |= 0x00000002;
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @return A list containing the synonyms.
*/
public com.google.protobuf.ProtocolStringList getSynonymsList() {
synonyms_.makeImmutable();
return synonyms_;
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @return The count of synonyms.
*/
public int getSynonymsCount() {
return synonyms_.size();
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @param index The index of the element to return.
* @return The synonyms at the given index.
*/
public java.lang.String getSynonyms(int index) {
return synonyms_.get(index);
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @param index The index of the value to return.
* @return The bytes of the synonyms at the given index.
*/
public com.google.protobuf.ByteString getSynonymsBytes(int index) {
return synonyms_.getByteString(index);
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @param index The index to set the value at.
* @param value The synonyms to set.
* @return This builder for chaining.
*/
public Builder setSynonyms(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSynonymsIsMutable();
synonyms_.set(index, value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @param value The synonyms to add.
* @return This builder for chaining.
*/
public Builder addSynonyms(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureSynonymsIsMutable();
synonyms_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @param values The synonyms to add.
* @return This builder for chaining.
*/
public Builder addAllSynonyms(java.lang.Iterable values) {
ensureSynonymsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, synonyms_);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @return This builder for chaining.
*/
public Builder clearSynonyms() {
synonyms_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
;
onChanged();
return this;
}
/**
*
*
*
* Defines a set of synonyms.
* Cannot contain duplicates.
* Can specify up to 100 synonyms.
*
*
* repeated string synonyms = 4;
*
* @param value The bytes of the synonyms to add.
* @return This builder for chaining.
*/
public Builder addSynonymsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureSynonymsIsMutable();
synonyms_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList onewayTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureOnewayTermsIsMutable() {
if (!onewayTerms_.isModifiable()) {
onewayTerms_ = new com.google.protobuf.LazyStringArrayList(onewayTerms_);
}
bitField0_ |= 0x00000004;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @return A list containing the onewayTerms.
*/
public com.google.protobuf.ProtocolStringList getOnewayTermsList() {
onewayTerms_.makeImmutable();
return onewayTerms_;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @return The count of onewayTerms.
*/
public int getOnewayTermsCount() {
return onewayTerms_.size();
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @param index The index of the element to return.
* @return The onewayTerms at the given index.
*/
public java.lang.String getOnewayTerms(int index) {
return onewayTerms_.get(index);
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @param index The index of the value to return.
* @return The bytes of the onewayTerms at the given index.
*/
public com.google.protobuf.ByteString getOnewayTermsBytes(int index) {
return onewayTerms_.getByteString(index);
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @param index The index to set the value at.
* @param value The onewayTerms to set.
* @return This builder for chaining.
*/
public Builder setOnewayTerms(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureOnewayTermsIsMutable();
onewayTerms_.set(index, value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @param value The onewayTerms to add.
* @return This builder for chaining.
*/
public Builder addOnewayTerms(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureOnewayTermsIsMutable();
onewayTerms_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @param values The onewayTerms to add.
* @return This builder for chaining.
*/
public Builder addAllOnewayTerms(java.lang.Iterable values) {
ensureOnewayTermsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, onewayTerms_);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @return This builder for chaining.
*/
public Builder clearOnewayTerms() {
onewayTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
;
onChanged();
return this;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string oneway_terms = 2;
*
* @param value The bytes of the onewayTerms to add.
* @return This builder for chaining.
*/
public Builder addOnewayTermsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureOnewayTermsIsMutable();
onewayTerms_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction)
private static final com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction();
}
public static com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OnewaySynonymsAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DoNotAssociateActionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Rule.DoNotAssociateAction)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return A list containing the queryTerms.
*/
java.util.List getQueryTermsList();
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return The count of queryTerms.
*/
int getQueryTermsCount();
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the element to return.
* @return The queryTerms at the given index.
*/
java.lang.String getQueryTerms(int index);
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the value to return.
* @return The bytes of the queryTerms at the given index.
*/
com.google.protobuf.ByteString getQueryTermsBytes(int index);
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @return A list containing the doNotAssociateTerms.
*/
java.util.List getDoNotAssociateTermsList();
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @return The count of doNotAssociateTerms.
*/
int getDoNotAssociateTermsCount();
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @param index The index of the element to return.
* @return The doNotAssociateTerms at the given index.
*/
java.lang.String getDoNotAssociateTerms(int index);
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @param index The index of the value to return.
* @return The bytes of the doNotAssociateTerms at the given index.
*/
com.google.protobuf.ByteString getDoNotAssociateTermsBytes(int index);
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @return A list containing the terms.
*/
java.util.List getTermsList();
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @return The count of terms.
*/
int getTermsCount();
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @param index The index of the element to return.
* @return The terms at the given index.
*/
java.lang.String getTerms(int index);
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @param index The index of the value to return.
* @return The bytes of the terms at the given index.
*/
com.google.protobuf.ByteString getTermsBytes(int index);
}
/**
*
*
*
* Prevents `query_term` from being associated with specified terms during
* search.
* Example: Don't associate "gShoe" and "cheap".
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.DoNotAssociateAction}
*/
public static final class DoNotAssociateAction extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule.DoNotAssociateAction)
DoNotAssociateActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use DoNotAssociateAction.newBuilder() to construct.
private DoNotAssociateAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DoNotAssociateAction() {
queryTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
doNotAssociateTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
terms_ = com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new DoNotAssociateAction();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_DoNotAssociateAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_DoNotAssociateAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.class,
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.Builder.class);
}
public static final int QUERY_TERMS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList queryTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return A list containing the queryTerms.
*/
public com.google.protobuf.ProtocolStringList getQueryTermsList() {
return queryTerms_;
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return The count of queryTerms.
*/
public int getQueryTermsCount() {
return queryTerms_.size();
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the element to return.
* @return The queryTerms at the given index.
*/
public java.lang.String getQueryTerms(int index) {
return queryTerms_.get(index);
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the value to return.
* @return The bytes of the queryTerms at the given index.
*/
public com.google.protobuf.ByteString getQueryTermsBytes(int index) {
return queryTerms_.getByteString(index);
}
public static final int DO_NOT_ASSOCIATE_TERMS_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList doNotAssociateTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @return A list containing the doNotAssociateTerms.
*/
public com.google.protobuf.ProtocolStringList getDoNotAssociateTermsList() {
return doNotAssociateTerms_;
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @return The count of doNotAssociateTerms.
*/
public int getDoNotAssociateTermsCount() {
return doNotAssociateTerms_.size();
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @param index The index of the element to return.
* @return The doNotAssociateTerms at the given index.
*/
public java.lang.String getDoNotAssociateTerms(int index) {
return doNotAssociateTerms_.get(index);
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @param index The index of the value to return.
* @return The bytes of the doNotAssociateTerms at the given index.
*/
public com.google.protobuf.ByteString getDoNotAssociateTermsBytes(int index) {
return doNotAssociateTerms_.getByteString(index);
}
public static final int TERMS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList terms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @return A list containing the terms.
*/
public com.google.protobuf.ProtocolStringList getTermsList() {
return terms_;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @return The count of terms.
*/
public int getTermsCount() {
return terms_.size();
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @param index The index of the element to return.
* @return The terms at the given index.
*/
public java.lang.String getTerms(int index) {
return terms_.get(index);
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @param index The index of the value to return.
* @return The bytes of the terms at the given index.
*/
public com.google.protobuf.ByteString getTermsBytes(int index) {
return terms_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
for (int i = 0; i < terms_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, terms_.getRaw(i));
}
for (int i = 0; i < queryTerms_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, queryTerms_.getRaw(i));
}
for (int i = 0; i < doNotAssociateTerms_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(
output, 3, doNotAssociateTerms_.getRaw(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < terms_.size(); i++) {
dataSize += computeStringSizeNoTag(terms_.getRaw(i));
}
size += dataSize;
size += 1 * getTermsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < queryTerms_.size(); i++) {
dataSize += computeStringSizeNoTag(queryTerms_.getRaw(i));
}
size += dataSize;
size += 1 * getQueryTermsList().size();
}
{
int dataSize = 0;
for (int i = 0; i < doNotAssociateTerms_.size(); i++) {
dataSize += computeStringSizeNoTag(doNotAssociateTerms_.getRaw(i));
}
size += dataSize;
size += 1 * getDoNotAssociateTermsList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction other =
(com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction) obj;
if (!getQueryTermsList().equals(other.getQueryTermsList())) return false;
if (!getDoNotAssociateTermsList().equals(other.getDoNotAssociateTermsList())) return false;
if (!getTermsList().equals(other.getTermsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getQueryTermsCount() > 0) {
hash = (37 * hash) + QUERY_TERMS_FIELD_NUMBER;
hash = (53 * hash) + getQueryTermsList().hashCode();
}
if (getDoNotAssociateTermsCount() > 0) {
hash = (37 * hash) + DO_NOT_ASSOCIATE_TERMS_FIELD_NUMBER;
hash = (53 * hash) + getDoNotAssociateTermsList().hashCode();
}
if (getTermsCount() > 0) {
hash = (37 * hash) + TERMS_FIELD_NUMBER;
hash = (53 * hash) + getTermsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Prevents `query_term` from being associated with specified terms during
* search.
* Example: Don't associate "gShoe" and "cheap".
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.DoNotAssociateAction}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule.DoNotAssociateAction)
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_DoNotAssociateAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_DoNotAssociateAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.class,
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
queryTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
doNotAssociateTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
terms_ = com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_DoNotAssociateAction_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction build() {
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction buildPartial() {
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction result =
new com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
queryTerms_.makeImmutable();
result.queryTerms_ = queryTerms_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
doNotAssociateTerms_.makeImmutable();
result.doNotAssociateTerms_ = doNotAssociateTerms_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
terms_.makeImmutable();
result.terms_ = terms_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction) {
return mergeFrom((com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction other) {
if (other == com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.getDefaultInstance())
return this;
if (!other.queryTerms_.isEmpty()) {
if (queryTerms_.isEmpty()) {
queryTerms_ = other.queryTerms_;
bitField0_ |= 0x00000001;
} else {
ensureQueryTermsIsMutable();
queryTerms_.addAll(other.queryTerms_);
}
onChanged();
}
if (!other.doNotAssociateTerms_.isEmpty()) {
if (doNotAssociateTerms_.isEmpty()) {
doNotAssociateTerms_ = other.doNotAssociateTerms_;
bitField0_ |= 0x00000002;
} else {
ensureDoNotAssociateTermsIsMutable();
doNotAssociateTerms_.addAll(other.doNotAssociateTerms_);
}
onChanged();
}
if (!other.terms_.isEmpty()) {
if (terms_.isEmpty()) {
terms_ = other.terms_;
bitField0_ |= 0x00000004;
} else {
ensureTermsIsMutable();
terms_.addAll(other.terms_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
java.lang.String s = input.readStringRequireUtf8();
ensureTermsIsMutable();
terms_.add(s);
break;
} // case 10
case 18:
{
java.lang.String s = input.readStringRequireUtf8();
ensureQueryTermsIsMutable();
queryTerms_.add(s);
break;
} // case 18
case 26:
{
java.lang.String s = input.readStringRequireUtf8();
ensureDoNotAssociateTermsIsMutable();
doNotAssociateTerms_.add(s);
break;
} // case 26
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList queryTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureQueryTermsIsMutable() {
if (!queryTerms_.isModifiable()) {
queryTerms_ = new com.google.protobuf.LazyStringArrayList(queryTerms_);
}
bitField0_ |= 0x00000001;
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return A list containing the queryTerms.
*/
public com.google.protobuf.ProtocolStringList getQueryTermsList() {
queryTerms_.makeImmutable();
return queryTerms_;
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return The count of queryTerms.
*/
public int getQueryTermsCount() {
return queryTerms_.size();
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the element to return.
* @return The queryTerms at the given index.
*/
public java.lang.String getQueryTerms(int index) {
return queryTerms_.get(index);
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the value to return.
* @return The bytes of the queryTerms at the given index.
*/
public com.google.protobuf.ByteString getQueryTermsBytes(int index) {
return queryTerms_.getByteString(index);
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index to set the value at.
* @param value The queryTerms to set.
* @return This builder for chaining.
*/
public Builder setQueryTerms(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryTermsIsMutable();
queryTerms_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param value The queryTerms to add.
* @return This builder for chaining.
*/
public Builder addQueryTerms(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryTermsIsMutable();
queryTerms_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param values The queryTerms to add.
* @return This builder for chaining.
*/
public Builder addAllQueryTerms(java.lang.Iterable values) {
ensureQueryTermsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, queryTerms_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return This builder for chaining.
*/
public Builder clearQueryTerms() {
queryTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will not consider do_not_associate_terms for search if in search query.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param value The bytes of the queryTerms to add.
* @return This builder for chaining.
*/
public Builder addQueryTermsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureQueryTermsIsMutable();
queryTerms_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList doNotAssociateTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureDoNotAssociateTermsIsMutable() {
if (!doNotAssociateTerms_.isModifiable()) {
doNotAssociateTerms_ = new com.google.protobuf.LazyStringArrayList(doNotAssociateTerms_);
}
bitField0_ |= 0x00000002;
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @return A list containing the doNotAssociateTerms.
*/
public com.google.protobuf.ProtocolStringList getDoNotAssociateTermsList() {
doNotAssociateTerms_.makeImmutable();
return doNotAssociateTerms_;
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @return The count of doNotAssociateTerms.
*/
public int getDoNotAssociateTermsCount() {
return doNotAssociateTerms_.size();
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @param index The index of the element to return.
* @return The doNotAssociateTerms at the given index.
*/
public java.lang.String getDoNotAssociateTerms(int index) {
return doNotAssociateTerms_.get(index);
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @param index The index of the value to return.
* @return The bytes of the doNotAssociateTerms at the given index.
*/
public com.google.protobuf.ByteString getDoNotAssociateTermsBytes(int index) {
return doNotAssociateTerms_.getByteString(index);
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @param index The index to set the value at.
* @param value The doNotAssociateTerms to set.
* @return This builder for chaining.
*/
public Builder setDoNotAssociateTerms(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureDoNotAssociateTermsIsMutable();
doNotAssociateTerms_.set(index, value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @param value The doNotAssociateTerms to add.
* @return This builder for chaining.
*/
public Builder addDoNotAssociateTerms(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureDoNotAssociateTermsIsMutable();
doNotAssociateTerms_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @param values The doNotAssociateTerms to add.
* @return This builder for chaining.
*/
public Builder addAllDoNotAssociateTerms(java.lang.Iterable values) {
ensureDoNotAssociateTermsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, doNotAssociateTerms_);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @return This builder for chaining.
*/
public Builder clearDoNotAssociateTerms() {
doNotAssociateTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
;
onChanged();
return this;
}
/**
*
*
*
* Cannot contain duplicates or the query term.
* Can specify up to 100 terms.
*
*
* repeated string do_not_associate_terms = 3;
*
* @param value The bytes of the doNotAssociateTerms to add.
* @return This builder for chaining.
*/
public Builder addDoNotAssociateTermsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureDoNotAssociateTermsIsMutable();
doNotAssociateTerms_.add(value);
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList terms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureTermsIsMutable() {
if (!terms_.isModifiable()) {
terms_ = new com.google.protobuf.LazyStringArrayList(terms_);
}
bitField0_ |= 0x00000004;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @return A list containing the terms.
*/
public com.google.protobuf.ProtocolStringList getTermsList() {
terms_.makeImmutable();
return terms_;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @return The count of terms.
*/
public int getTermsCount() {
return terms_.size();
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @param index The index of the element to return.
* @return The terms at the given index.
*/
public java.lang.String getTerms(int index) {
return terms_.get(index);
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @param index The index of the value to return.
* @return The bytes of the terms at the given index.
*/
public com.google.protobuf.ByteString getTermsBytes(int index) {
return terms_.getByteString(index);
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @param index The index to set the value at.
* @param value The terms to set.
* @return This builder for chaining.
*/
public Builder setTerms(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTermsIsMutable();
terms_.set(index, value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @param value The terms to add.
* @return This builder for chaining.
*/
public Builder addTerms(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureTermsIsMutable();
terms_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @param values The terms to add.
* @return This builder for chaining.
*/
public Builder addAllTerms(java.lang.Iterable values) {
ensureTermsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, terms_);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @return This builder for chaining.
*/
public Builder clearTerms() {
terms_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
;
onChanged();
return this;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* repeated string terms = 1;
*
* @param value The bytes of the terms to add.
* @return This builder for chaining.
*/
public Builder addTermsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureTermsIsMutable();
terms_.add(value);
bitField0_ |= 0x00000004;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule.DoNotAssociateAction)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule.DoNotAssociateAction)
private static final com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction();
}
public static com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DoNotAssociateAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ReplacementActionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Rule.ReplacementAction)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return A list containing the queryTerms.
*/
java.util.List getQueryTermsList();
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return The count of queryTerms.
*/
int getQueryTermsCount();
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the element to return.
* @return The queryTerms at the given index.
*/
java.lang.String getQueryTerms(int index);
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the value to return.
* @return The bytes of the queryTerms at the given index.
*/
com.google.protobuf.ByteString getQueryTermsBytes(int index);
/**
*
*
*
* Term that will be used for replacement.
*
*
* string replacement_term = 3;
*
* @return The replacementTerm.
*/
java.lang.String getReplacementTerm();
/**
*
*
*
* Term that will be used for replacement.
*
*
* string replacement_term = 3;
*
* @return The bytes for replacementTerm.
*/
com.google.protobuf.ByteString getReplacementTermBytes();
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* string term = 1;
*
* @return The term.
*/
java.lang.String getTerm();
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* string term = 1;
*
* @return The bytes for term.
*/
com.google.protobuf.ByteString getTermBytes();
}
/**
*
*
*
* Replaces a term in the query. Multiple replacement candidates can be
* specified. All `query_terms` will be replaced with the replacement term.
* Example: Replace "gShoe" with "google shoe".
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.ReplacementAction}
*/
public static final class ReplacementAction extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule.ReplacementAction)
ReplacementActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use ReplacementAction.newBuilder() to construct.
private ReplacementAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ReplacementAction() {
queryTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
replacementTerm_ = "";
term_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new ReplacementAction();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ReplacementAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ReplacementAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.ReplacementAction.class,
com.google.cloud.retail.v2alpha.Rule.ReplacementAction.Builder.class);
}
public static final int QUERY_TERMS_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList queryTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return A list containing the queryTerms.
*/
public com.google.protobuf.ProtocolStringList getQueryTermsList() {
return queryTerms_;
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return The count of queryTerms.
*/
public int getQueryTermsCount() {
return queryTerms_.size();
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the element to return.
* @return The queryTerms at the given index.
*/
public java.lang.String getQueryTerms(int index) {
return queryTerms_.get(index);
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the value to return.
* @return The bytes of the queryTerms at the given index.
*/
public com.google.protobuf.ByteString getQueryTermsBytes(int index) {
return queryTerms_.getByteString(index);
}
public static final int REPLACEMENT_TERM_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object replacementTerm_ = "";
/**
*
*
*
* Term that will be used for replacement.
*
*
* string replacement_term = 3;
*
* @return The replacementTerm.
*/
@java.lang.Override
public java.lang.String getReplacementTerm() {
java.lang.Object ref = replacementTerm_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
replacementTerm_ = s;
return s;
}
}
/**
*
*
*
* Term that will be used for replacement.
*
*
* string replacement_term = 3;
*
* @return The bytes for replacementTerm.
*/
@java.lang.Override
public com.google.protobuf.ByteString getReplacementTermBytes() {
java.lang.Object ref = replacementTerm_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
replacementTerm_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TERM_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object term_ = "";
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* string term = 1;
*
* @return The term.
*/
@java.lang.Override
public java.lang.String getTerm() {
java.lang.Object ref = term_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
term_ = s;
return s;
}
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* string term = 1;
*
* @return The bytes for term.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTermBytes() {
java.lang.Object ref = term_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
term_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(term_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, term_);
}
for (int i = 0; i < queryTerms_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, queryTerms_.getRaw(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(replacementTerm_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, replacementTerm_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(term_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, term_);
}
{
int dataSize = 0;
for (int i = 0; i < queryTerms_.size(); i++) {
dataSize += computeStringSizeNoTag(queryTerms_.getRaw(i));
}
size += dataSize;
size += 1 * getQueryTermsList().size();
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(replacementTerm_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, replacementTerm_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.Rule.ReplacementAction)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule.ReplacementAction other =
(com.google.cloud.retail.v2alpha.Rule.ReplacementAction) obj;
if (!getQueryTermsList().equals(other.getQueryTermsList())) return false;
if (!getReplacementTerm().equals(other.getReplacementTerm())) return false;
if (!getTerm().equals(other.getTerm())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getQueryTermsCount() > 0) {
hash = (37 * hash) + QUERY_TERMS_FIELD_NUMBER;
hash = (53 * hash) + getQueryTermsList().hashCode();
}
hash = (37 * hash) + REPLACEMENT_TERM_FIELD_NUMBER;
hash = (53 * hash) + getReplacementTerm().hashCode();
hash = (37 * hash) + TERM_FIELD_NUMBER;
hash = (53 * hash) + getTerm().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.retail.v2alpha.Rule.ReplacementAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Replaces a term in the query. Multiple replacement candidates can be
* specified. All `query_terms` will be replaced with the replacement term.
* Example: Replace "gShoe" with "google shoe".
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.ReplacementAction}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule.ReplacementAction)
com.google.cloud.retail.v2alpha.Rule.ReplacementActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ReplacementAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ReplacementAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.ReplacementAction.class,
com.google.cloud.retail.v2alpha.Rule.ReplacementAction.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.Rule.ReplacementAction.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
queryTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
replacementTerm_ = "";
term_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ReplacementAction_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ReplacementAction getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.ReplacementAction.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ReplacementAction build() {
com.google.cloud.retail.v2alpha.Rule.ReplacementAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ReplacementAction buildPartial() {
com.google.cloud.retail.v2alpha.Rule.ReplacementAction result =
new com.google.cloud.retail.v2alpha.Rule.ReplacementAction(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.retail.v2alpha.Rule.ReplacementAction result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
queryTerms_.makeImmutable();
result.queryTerms_ = queryTerms_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.replacementTerm_ = replacementTerm_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.term_ = term_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.Rule.ReplacementAction) {
return mergeFrom((com.google.cloud.retail.v2alpha.Rule.ReplacementAction) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.Rule.ReplacementAction other) {
if (other == com.google.cloud.retail.v2alpha.Rule.ReplacementAction.getDefaultInstance())
return this;
if (!other.queryTerms_.isEmpty()) {
if (queryTerms_.isEmpty()) {
queryTerms_ = other.queryTerms_;
bitField0_ |= 0x00000001;
} else {
ensureQueryTermsIsMutable();
queryTerms_.addAll(other.queryTerms_);
}
onChanged();
}
if (!other.getReplacementTerm().isEmpty()) {
replacementTerm_ = other.replacementTerm_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.getTerm().isEmpty()) {
term_ = other.term_;
bitField0_ |= 0x00000004;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
term_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 10
case 18:
{
java.lang.String s = input.readStringRequireUtf8();
ensureQueryTermsIsMutable();
queryTerms_.add(s);
break;
} // case 18
case 26:
{
replacementTerm_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 26
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList queryTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureQueryTermsIsMutable() {
if (!queryTerms_.isModifiable()) {
queryTerms_ = new com.google.protobuf.LazyStringArrayList(queryTerms_);
}
bitField0_ |= 0x00000001;
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return A list containing the queryTerms.
*/
public com.google.protobuf.ProtocolStringList getQueryTermsList() {
queryTerms_.makeImmutable();
return queryTerms_;
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return The count of queryTerms.
*/
public int getQueryTermsCount() {
return queryTerms_.size();
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the element to return.
* @return The queryTerms at the given index.
*/
public java.lang.String getQueryTerms(int index) {
return queryTerms_.get(index);
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index of the value to return.
* @return The bytes of the queryTerms at the given index.
*/
public com.google.protobuf.ByteString getQueryTermsBytes(int index) {
return queryTerms_.getByteString(index);
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param index The index to set the value at.
* @param value The queryTerms to set.
* @return This builder for chaining.
*/
public Builder setQueryTerms(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryTermsIsMutable();
queryTerms_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param value The queryTerms to add.
* @return This builder for chaining.
*/
public Builder addQueryTerms(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureQueryTermsIsMutable();
queryTerms_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param values The queryTerms to add.
* @return This builder for chaining.
*/
public Builder addAllQueryTerms(java.lang.Iterable values) {
ensureQueryTermsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, queryTerms_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @return This builder for chaining.
*/
public Builder clearQueryTerms() {
queryTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
;
onChanged();
return this;
}
/**
*
*
*
* Terms from the search query.
* Will be replaced by replacement term.
* Can specify up to 100 terms.
*
*
* repeated string query_terms = 2;
*
* @param value The bytes of the queryTerms to add.
* @return This builder for chaining.
*/
public Builder addQueryTermsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureQueryTermsIsMutable();
queryTerms_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object replacementTerm_ = "";
/**
*
*
*
* Term that will be used for replacement.
*
*
* string replacement_term = 3;
*
* @return The replacementTerm.
*/
public java.lang.String getReplacementTerm() {
java.lang.Object ref = replacementTerm_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
replacementTerm_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Term that will be used for replacement.
*
*
* string replacement_term = 3;
*
* @return The bytes for replacementTerm.
*/
public com.google.protobuf.ByteString getReplacementTermBytes() {
java.lang.Object ref = replacementTerm_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
replacementTerm_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Term that will be used for replacement.
*
*
* string replacement_term = 3;
*
* @param value The replacementTerm to set.
* @return This builder for chaining.
*/
public Builder setReplacementTerm(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
replacementTerm_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Term that will be used for replacement.
*
*
* string replacement_term = 3;
*
* @return This builder for chaining.
*/
public Builder clearReplacementTerm() {
replacementTerm_ = getDefaultInstance().getReplacementTerm();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* Term that will be used for replacement.
*
*
* string replacement_term = 3;
*
* @param value The bytes for replacementTerm to set.
* @return This builder for chaining.
*/
public Builder setReplacementTermBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
replacementTerm_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object term_ = "";
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* string term = 1;
*
* @return The term.
*/
public java.lang.String getTerm() {
java.lang.Object ref = term_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
term_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* string term = 1;
*
* @return The bytes for term.
*/
public com.google.protobuf.ByteString getTermBytes() {
java.lang.Object ref = term_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
term_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* string term = 1;
*
* @param value The term to set.
* @return This builder for chaining.
*/
public Builder setTerm(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
term_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* string term = 1;
*
* @return This builder for chaining.
*/
public Builder clearTerm() {
term_ = getDefaultInstance().getTerm();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* Will be [deprecated = true] post migration;
*
*
* string term = 1;
*
* @param value The bytes for term to set.
* @return This builder for chaining.
*/
public Builder setTermBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
term_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule.ReplacementAction)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule.ReplacementAction)
private static final com.google.cloud.retail.v2alpha.Rule.ReplacementAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.Rule.ReplacementAction();
}
public static com.google.cloud.retail.v2alpha.Rule.ReplacementAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ReplacementAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ReplacementAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface IgnoreActionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Rule.IgnoreAction)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @return A list containing the ignoreTerms.
*/
java.util.List getIgnoreTermsList();
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @return The count of ignoreTerms.
*/
int getIgnoreTermsCount();
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @param index The index of the element to return.
* @return The ignoreTerms at the given index.
*/
java.lang.String getIgnoreTerms(int index);
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @param index The index of the value to return.
* @return The bytes of the ignoreTerms at the given index.
*/
com.google.protobuf.ByteString getIgnoreTermsBytes(int index);
}
/**
*
*
*
* Prevents a term in the query from being used in search.
* Example: Don't search for "shoddy".
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.IgnoreAction}
*/
public static final class IgnoreAction extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule.IgnoreAction)
IgnoreActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use IgnoreAction.newBuilder() to construct.
private IgnoreAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private IgnoreAction() {
ignoreTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new IgnoreAction();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_IgnoreAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_IgnoreAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.IgnoreAction.class,
com.google.cloud.retail.v2alpha.Rule.IgnoreAction.Builder.class);
}
public static final int IGNORE_TERMS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList ignoreTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @return A list containing the ignoreTerms.
*/
public com.google.protobuf.ProtocolStringList getIgnoreTermsList() {
return ignoreTerms_;
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @return The count of ignoreTerms.
*/
public int getIgnoreTermsCount() {
return ignoreTerms_.size();
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @param index The index of the element to return.
* @return The ignoreTerms at the given index.
*/
public java.lang.String getIgnoreTerms(int index) {
return ignoreTerms_.get(index);
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @param index The index of the value to return.
* @return The bytes of the ignoreTerms at the given index.
*/
public com.google.protobuf.ByteString getIgnoreTermsBytes(int index) {
return ignoreTerms_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
for (int i = 0; i < ignoreTerms_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, ignoreTerms_.getRaw(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < ignoreTerms_.size(); i++) {
dataSize += computeStringSizeNoTag(ignoreTerms_.getRaw(i));
}
size += dataSize;
size += 1 * getIgnoreTermsList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.Rule.IgnoreAction)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule.IgnoreAction other =
(com.google.cloud.retail.v2alpha.Rule.IgnoreAction) obj;
if (!getIgnoreTermsList().equals(other.getIgnoreTermsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getIgnoreTermsCount() > 0) {
hash = (37 * hash) + IGNORE_TERMS_FIELD_NUMBER;
hash = (53 * hash) + getIgnoreTermsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.retail.v2alpha.Rule.IgnoreAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Prevents a term in the query from being used in search.
* Example: Don't search for "shoddy".
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.IgnoreAction}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule.IgnoreAction)
com.google.cloud.retail.v2alpha.Rule.IgnoreActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_IgnoreAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_IgnoreAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.IgnoreAction.class,
com.google.cloud.retail.v2alpha.Rule.IgnoreAction.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.Rule.IgnoreAction.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
ignoreTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_IgnoreAction_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.IgnoreAction getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.IgnoreAction.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.IgnoreAction build() {
com.google.cloud.retail.v2alpha.Rule.IgnoreAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.IgnoreAction buildPartial() {
com.google.cloud.retail.v2alpha.Rule.IgnoreAction result =
new com.google.cloud.retail.v2alpha.Rule.IgnoreAction(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.retail.v2alpha.Rule.IgnoreAction result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
ignoreTerms_.makeImmutable();
result.ignoreTerms_ = ignoreTerms_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.Rule.IgnoreAction) {
return mergeFrom((com.google.cloud.retail.v2alpha.Rule.IgnoreAction) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.Rule.IgnoreAction other) {
if (other == com.google.cloud.retail.v2alpha.Rule.IgnoreAction.getDefaultInstance())
return this;
if (!other.ignoreTerms_.isEmpty()) {
if (ignoreTerms_.isEmpty()) {
ignoreTerms_ = other.ignoreTerms_;
bitField0_ |= 0x00000001;
} else {
ensureIgnoreTermsIsMutable();
ignoreTerms_.addAll(other.ignoreTerms_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
java.lang.String s = input.readStringRequireUtf8();
ensureIgnoreTermsIsMutable();
ignoreTerms_.add(s);
break;
} // case 10
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList ignoreTerms_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureIgnoreTermsIsMutable() {
if (!ignoreTerms_.isModifiable()) {
ignoreTerms_ = new com.google.protobuf.LazyStringArrayList(ignoreTerms_);
}
bitField0_ |= 0x00000001;
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @return A list containing the ignoreTerms.
*/
public com.google.protobuf.ProtocolStringList getIgnoreTermsList() {
ignoreTerms_.makeImmutable();
return ignoreTerms_;
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @return The count of ignoreTerms.
*/
public int getIgnoreTermsCount() {
return ignoreTerms_.size();
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @param index The index of the element to return.
* @return The ignoreTerms at the given index.
*/
public java.lang.String getIgnoreTerms(int index) {
return ignoreTerms_.get(index);
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @param index The index of the value to return.
* @return The bytes of the ignoreTerms at the given index.
*/
public com.google.protobuf.ByteString getIgnoreTermsBytes(int index) {
return ignoreTerms_.getByteString(index);
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @param index The index to set the value at.
* @param value The ignoreTerms to set.
* @return This builder for chaining.
*/
public Builder setIgnoreTerms(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIgnoreTermsIsMutable();
ignoreTerms_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @param value The ignoreTerms to add.
* @return This builder for chaining.
*/
public Builder addIgnoreTerms(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIgnoreTermsIsMutable();
ignoreTerms_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @param values The ignoreTerms to add.
* @return This builder for chaining.
*/
public Builder addAllIgnoreTerms(java.lang.Iterable values) {
ensureIgnoreTermsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, ignoreTerms_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @return This builder for chaining.
*/
public Builder clearIgnoreTerms() {
ignoreTerms_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
;
onChanged();
return this;
}
/**
*
*
*
* Terms to ignore in the search query.
*
*
* repeated string ignore_terms = 1;
*
* @param value The bytes of the ignoreTerms to add.
* @return This builder for chaining.
*/
public Builder addIgnoreTermsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureIgnoreTermsIsMutable();
ignoreTerms_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule.IgnoreAction)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule.IgnoreAction)
private static final com.google.cloud.retail.v2alpha.Rule.IgnoreAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.Rule.IgnoreAction();
}
public static com.google.cloud.retail.v2alpha.Rule.IgnoreAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IgnoreAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.IgnoreAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ForceReturnFacetActionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
java.util.List<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment>
getFacetPositionAdjustmentsList();
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
getFacetPositionAdjustments(int index);
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
int getFacetPositionAdjustmentsCount();
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
java.util.List<
? extends
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustmentOrBuilder>
getFacetPositionAdjustmentsOrBuilderList();
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustmentOrBuilder
getFacetPositionAdjustmentsOrBuilder(int index);
}
/**
*
*
*
* Force returns an attribute/facet in the request around a certain position
* or above.
*
* * Rule Condition:
* Must specify non-empty
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* (for search only) or
* [Condition.page_categories][google.cloud.retail.v2alpha.Condition.page_categories]
* (for browse only), but can't specify both.
*
* * Action Inputs: attribute name, position
*
* * Action Result: Will force return a facet key around a certain position
* or above if the condition is satisfied.
*
* Example: Suppose the query is "shoes", the
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* is "shoes", the
* [ForceReturnFacetAction.FacetPositionAdjustment.attribute_name][google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment.attribute_name]
* is "size" and the
* [ForceReturnFacetAction.FacetPositionAdjustment.position][google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment.position]
* is 8.
*
* Two cases: a) The facet key "size" is not already in the top 8 slots, then
* the facet "size" will appear at a position close to 8. b) The facet key
* "size" in among the top 8 positions in the request, then it will stay at
* its current rank.
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction}
*/
public static final class ForceReturnFacetAction extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction)
ForceReturnFacetActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use ForceReturnFacetAction.newBuilder() to construct.
private ForceReturnFacetAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ForceReturnFacetAction() {
facetPositionAdjustments_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new ForceReturnFacetAction();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ForceReturnFacetAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ForceReturnFacetAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.class,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.Builder.class);
}
public interface FacetPositionAdjustmentOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* The attribute name to force return as a facet. Each attribute name
* should be a valid attribute name, be non-empty and contain at most 80
* characters long.
*
*
* string attribute_name = 1;
*
* @return The attributeName.
*/
java.lang.String getAttributeName();
/**
*
*
*
* The attribute name to force return as a facet. Each attribute name
* should be a valid attribute name, be non-empty and contain at most 80
* characters long.
*
*
* string attribute_name = 1;
*
* @return The bytes for attributeName.
*/
com.google.protobuf.ByteString getAttributeNameBytes();
/**
*
*
*
* This is the position in the request as explained above. It should be
* strictly positive be at most 100.
*
*
* int32 position = 2;
*
* @return The position.
*/
int getPosition();
}
/**
*
*
*
* Each facet position adjustment consists of a single attribute name (i.e.
* facet key) along with a specified position.
*
*
* Protobuf type {@code
* google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment}
*/
public static final class FacetPositionAdjustment extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment)
FacetPositionAdjustmentOrBuilder {
private static final long serialVersionUID = 0L;
// Use FacetPositionAdjustment.newBuilder() to construct.
private FacetPositionAdjustment(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FacetPositionAdjustment() {
attributeName_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new FacetPositionAdjustment();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ForceReturnFacetAction_FacetPositionAdjustment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ForceReturnFacetAction_FacetPositionAdjustment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.class,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.Builder.class);
}
public static final int ATTRIBUTE_NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object attributeName_ = "";
/**
*
*
*
* The attribute name to force return as a facet. Each attribute name
* should be a valid attribute name, be non-empty and contain at most 80
* characters long.
*
*
* string attribute_name = 1;
*
* @return The attributeName.
*/
@java.lang.Override
public java.lang.String getAttributeName() {
java.lang.Object ref = attributeName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
attributeName_ = s;
return s;
}
}
/**
*
*
*
* The attribute name to force return as a facet. Each attribute name
* should be a valid attribute name, be non-empty and contain at most 80
* characters long.
*
*
* string attribute_name = 1;
*
* @return The bytes for attributeName.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAttributeNameBytes() {
java.lang.Object ref = attributeName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
attributeName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int POSITION_FIELD_NUMBER = 2;
private int position_ = 0;
/**
*
*
*
* This is the position in the request as explained above. It should be
* strictly positive be at most 100.
*
*
* int32 position = 2;
*
* @return The position.
*/
@java.lang.Override
public int getPosition() {
return position_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(attributeName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, attributeName_);
}
if (position_ != 0) {
output.writeInt32(2, position_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(attributeName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, attributeName_);
}
if (position_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(2, position_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj
instanceof
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment other =
(com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment)
obj;
if (!getAttributeName().equals(other.getAttributeName())) return false;
if (getPosition() != other.getPosition()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ATTRIBUTE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getAttributeName().hashCode();
hash = (37 * hash) + POSITION_FIELD_NUMBER;
hash = (53 * hash) + getPosition();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseFrom(com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseFrom(byte[] data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseFrom(byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseDelimitedFrom(java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseFrom(com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Each facet position adjustment consists of a single attribute name (i.e.
* facet key) along with a specified position.
*
*
* Protobuf type {@code
* google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment)
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustmentOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ForceReturnFacetAction_FacetPositionAdjustment_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ForceReturnFacetAction_FacetPositionAdjustment_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment.class,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment.Builder.class);
}
// Construct using
// com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
attributeName_ = "";
position_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ForceReturnFacetAction_FacetPositionAdjustment_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
build() {
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
buildPartial() {
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
result =
new com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.attributeName_ = attributeName_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.position_ = position_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other
instanceof
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment) {
return mergeFrom(
(com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment)
other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
other) {
if (other
== com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.getDefaultInstance()) return this;
if (!other.getAttributeName().isEmpty()) {
attributeName_ = other.attributeName_;
bitField0_ |= 0x00000001;
onChanged();
}
if (other.getPosition() != 0) {
setPosition(other.getPosition());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
attributeName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 16:
{
position_ = input.readInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object attributeName_ = "";
/**
*
*
*
* The attribute name to force return as a facet. Each attribute name
* should be a valid attribute name, be non-empty and contain at most 80
* characters long.
*
*
* string attribute_name = 1;
*
* @return The attributeName.
*/
public java.lang.String getAttributeName() {
java.lang.Object ref = attributeName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
attributeName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The attribute name to force return as a facet. Each attribute name
* should be a valid attribute name, be non-empty and contain at most 80
* characters long.
*
*
* string attribute_name = 1;
*
* @return The bytes for attributeName.
*/
public com.google.protobuf.ByteString getAttributeNameBytes() {
java.lang.Object ref = attributeName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
attributeName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The attribute name to force return as a facet. Each attribute name
* should be a valid attribute name, be non-empty and contain at most 80
* characters long.
*
*
* string attribute_name = 1;
*
* @param value The attributeName to set.
* @return This builder for chaining.
*/
public Builder setAttributeName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
attributeName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The attribute name to force return as a facet. Each attribute name
* should be a valid attribute name, be non-empty and contain at most 80
* characters long.
*
*
* string attribute_name = 1;
*
* @return This builder for chaining.
*/
public Builder clearAttributeName() {
attributeName_ = getDefaultInstance().getAttributeName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* The attribute name to force return as a facet. Each attribute name
* should be a valid attribute name, be non-empty and contain at most 80
* characters long.
*
*
* string attribute_name = 1;
*
* @param value The bytes for attributeName to set.
* @return This builder for chaining.
*/
public Builder setAttributeNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
attributeName_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private int position_;
/**
*
*
*
* This is the position in the request as explained above. It should be
* strictly positive be at most 100.
*
*
* int32 position = 2;
*
* @return The position.
*/
@java.lang.Override
public int getPosition() {
return position_;
}
/**
*
*
*
* This is the position in the request as explained above. It should be
* strictly positive be at most 100.
*
*
* int32 position = 2;
*
* @param value The position to set.
* @return This builder for chaining.
*/
public Builder setPosition(int value) {
position_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* This is the position in the request as explained above. It should be
* strictly positive be at most 100.
*
*
* int32 position = 2;
*
* @return This builder for chaining.
*/
public Builder clearPosition() {
bitField0_ = (bitField0_ & ~0x00000002);
position_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment)
private static final com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE =
new com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment();
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public FacetPositionAdjustment parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int FACET_POSITION_ADJUSTMENTS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment>
facetPositionAdjustments_;
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
@java.lang.Override
public java.util.List<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment>
getFacetPositionAdjustmentsList() {
return facetPositionAdjustments_;
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
@java.lang.Override
public java.util.List<
? extends
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustmentOrBuilder>
getFacetPositionAdjustmentsOrBuilderList() {
return facetPositionAdjustments_;
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
@java.lang.Override
public int getFacetPositionAdjustmentsCount() {
return facetPositionAdjustments_.size();
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
getFacetPositionAdjustments(int index) {
return facetPositionAdjustments_.get(index);
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustmentOrBuilder
getFacetPositionAdjustmentsOrBuilder(int index) {
return facetPositionAdjustments_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
for (int i = 0; i < facetPositionAdjustments_.size(); i++) {
output.writeMessage(1, facetPositionAdjustments_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < facetPositionAdjustments_.size(); i++) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
1, facetPositionAdjustments_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction other =
(com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction) obj;
if (!getFacetPositionAdjustmentsList().equals(other.getFacetPositionAdjustmentsList()))
return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getFacetPositionAdjustmentsCount() > 0) {
hash = (37 * hash) + FACET_POSITION_ADJUSTMENTS_FIELD_NUMBER;
hash = (53 * hash) + getFacetPositionAdjustmentsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Force returns an attribute/facet in the request around a certain position
* or above.
*
* * Rule Condition:
* Must specify non-empty
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* (for search only) or
* [Condition.page_categories][google.cloud.retail.v2alpha.Condition.page_categories]
* (for browse only), but can't specify both.
*
* * Action Inputs: attribute name, position
*
* * Action Result: Will force return a facet key around a certain position
* or above if the condition is satisfied.
*
* Example: Suppose the query is "shoes", the
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* is "shoes", the
* [ForceReturnFacetAction.FacetPositionAdjustment.attribute_name][google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment.attribute_name]
* is "size" and the
* [ForceReturnFacetAction.FacetPositionAdjustment.position][google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment.position]
* is 8.
*
* Two cases: a) The facet key "size" is not already in the top 8 slots, then
* the facet "size" will appear at a position close to 8. b) The facet key
* "size" in among the top 8 positions in the request, then it will stay at
* its current rank.
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction)
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ForceReturnFacetAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ForceReturnFacetAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.class,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (facetPositionAdjustmentsBuilder_ == null) {
facetPositionAdjustments_ = java.util.Collections.emptyList();
} else {
facetPositionAdjustments_ = null;
facetPositionAdjustmentsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_ForceReturnFacetAction_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction build() {
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction buildPartial() {
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction result =
new com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction result) {
if (facetPositionAdjustmentsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
facetPositionAdjustments_ =
java.util.Collections.unmodifiableList(facetPositionAdjustments_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.facetPositionAdjustments_ = facetPositionAdjustments_;
} else {
result.facetPositionAdjustments_ = facetPositionAdjustmentsBuilder_.build();
}
}
private void buildPartial0(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction) {
return mergeFrom((com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction other) {
if (other
== com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.getDefaultInstance())
return this;
if (facetPositionAdjustmentsBuilder_ == null) {
if (!other.facetPositionAdjustments_.isEmpty()) {
if (facetPositionAdjustments_.isEmpty()) {
facetPositionAdjustments_ = other.facetPositionAdjustments_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFacetPositionAdjustmentsIsMutable();
facetPositionAdjustments_.addAll(other.facetPositionAdjustments_);
}
onChanged();
}
} else {
if (!other.facetPositionAdjustments_.isEmpty()) {
if (facetPositionAdjustmentsBuilder_.isEmpty()) {
facetPositionAdjustmentsBuilder_.dispose();
facetPositionAdjustmentsBuilder_ = null;
facetPositionAdjustments_ = other.facetPositionAdjustments_;
bitField0_ = (bitField0_ & ~0x00000001);
facetPositionAdjustmentsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getFacetPositionAdjustmentsFieldBuilder()
: null;
} else {
facetPositionAdjustmentsBuilder_.addAllMessages(other.facetPositionAdjustments_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment
m =
input.readMessage(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment.parser(),
extensionRegistry);
if (facetPositionAdjustmentsBuilder_ == null) {
ensureFacetPositionAdjustmentsIsMutable();
facetPositionAdjustments_.add(m);
} else {
facetPositionAdjustmentsBuilder_.addMessage(m);
}
break;
} // case 10
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment>
facetPositionAdjustments_ = java.util.Collections.emptyList();
private void ensureFacetPositionAdjustmentsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
facetPositionAdjustments_ =
new java.util.ArrayList<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment>(facetPositionAdjustments_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.Builder,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustmentOrBuilder>
facetPositionAdjustmentsBuilder_;
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public java.util.List<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment>
getFacetPositionAdjustmentsList() {
if (facetPositionAdjustmentsBuilder_ == null) {
return java.util.Collections.unmodifiableList(facetPositionAdjustments_);
} else {
return facetPositionAdjustmentsBuilder_.getMessageList();
}
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public int getFacetPositionAdjustmentsCount() {
if (facetPositionAdjustmentsBuilder_ == null) {
return facetPositionAdjustments_.size();
} else {
return facetPositionAdjustmentsBuilder_.getCount();
}
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
getFacetPositionAdjustments(int index) {
if (facetPositionAdjustmentsBuilder_ == null) {
return facetPositionAdjustments_.get(index);
} else {
return facetPositionAdjustmentsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public Builder setFacetPositionAdjustments(
int index,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
value) {
if (facetPositionAdjustmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFacetPositionAdjustmentsIsMutable();
facetPositionAdjustments_.set(index, value);
onChanged();
} else {
facetPositionAdjustmentsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public Builder setFacetPositionAdjustments(
int index,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.Builder
builderForValue) {
if (facetPositionAdjustmentsBuilder_ == null) {
ensureFacetPositionAdjustmentsIsMutable();
facetPositionAdjustments_.set(index, builderForValue.build());
onChanged();
} else {
facetPositionAdjustmentsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public Builder addFacetPositionAdjustments(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
value) {
if (facetPositionAdjustmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFacetPositionAdjustmentsIsMutable();
facetPositionAdjustments_.add(value);
onChanged();
} else {
facetPositionAdjustmentsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public Builder addFacetPositionAdjustments(
int index,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
value) {
if (facetPositionAdjustmentsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFacetPositionAdjustmentsIsMutable();
facetPositionAdjustments_.add(index, value);
onChanged();
} else {
facetPositionAdjustmentsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public Builder addFacetPositionAdjustments(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.Builder
builderForValue) {
if (facetPositionAdjustmentsBuilder_ == null) {
ensureFacetPositionAdjustmentsIsMutable();
facetPositionAdjustments_.add(builderForValue.build());
onChanged();
} else {
facetPositionAdjustmentsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public Builder addFacetPositionAdjustments(
int index,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.Builder
builderForValue) {
if (facetPositionAdjustmentsBuilder_ == null) {
ensureFacetPositionAdjustmentsIsMutable();
facetPositionAdjustments_.add(index, builderForValue.build());
onChanged();
} else {
facetPositionAdjustmentsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public Builder addAllFacetPositionAdjustments(
java.lang.Iterable<
? extends
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment>
values) {
if (facetPositionAdjustmentsBuilder_ == null) {
ensureFacetPositionAdjustmentsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, facetPositionAdjustments_);
onChanged();
} else {
facetPositionAdjustmentsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public Builder clearFacetPositionAdjustments() {
if (facetPositionAdjustmentsBuilder_ == null) {
facetPositionAdjustments_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
facetPositionAdjustmentsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public Builder removeFacetPositionAdjustments(int index) {
if (facetPositionAdjustmentsBuilder_ == null) {
ensureFacetPositionAdjustmentsIsMutable();
facetPositionAdjustments_.remove(index);
onChanged();
} else {
facetPositionAdjustmentsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.Builder
getFacetPositionAdjustmentsBuilder(int index) {
return getFacetPositionAdjustmentsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustmentOrBuilder
getFacetPositionAdjustmentsOrBuilder(int index) {
if (facetPositionAdjustmentsBuilder_ == null) {
return facetPositionAdjustments_.get(index);
} else {
return facetPositionAdjustmentsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public java.util.List<
? extends
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustmentOrBuilder>
getFacetPositionAdjustmentsOrBuilderList() {
if (facetPositionAdjustmentsBuilder_ != null) {
return facetPositionAdjustmentsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(facetPositionAdjustments_);
}
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.Builder
addFacetPositionAdjustmentsBuilder() {
return getFacetPositionAdjustmentsFieldBuilder()
.addBuilder(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.getDefaultInstance());
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.Builder
addFacetPositionAdjustmentsBuilder(int index) {
return getFacetPositionAdjustmentsFieldBuilder()
.addBuilder(
index,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.getDefaultInstance());
}
/**
*
*
*
* Each instance corresponds to a force return attribute for the given
* condition. There can't be more 15 instances here.
*
*
*
* repeated .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment facet_position_adjustments = 1;
*
*/
public java.util.List<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.Builder>
getFacetPositionAdjustmentsBuilderList() {
return getFacetPositionAdjustmentsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.FacetPositionAdjustment
.Builder,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustmentOrBuilder>
getFacetPositionAdjustmentsFieldBuilder() {
if (facetPositionAdjustmentsBuilder_ == null) {
facetPositionAdjustmentsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustment.Builder,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.FacetPositionAdjustmentOrBuilder>(
facetPositionAdjustments_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
facetPositionAdjustments_ = null;
}
return facetPositionAdjustmentsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction)
private static final com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction();
}
public static com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ForceReturnFacetAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RemoveFacetActionOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.Rule.RemoveFacetAction)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @return A list containing the attributeNames.
*/
java.util.List getAttributeNamesList();
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @return The count of attributeNames.
*/
int getAttributeNamesCount();
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @param index The index of the element to return.
* @return The attributeNames at the given index.
*/
java.lang.String getAttributeNames(int index);
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @param index The index of the value to return.
* @return The bytes of the attributeNames at the given index.
*/
com.google.protobuf.ByteString getAttributeNamesBytes(int index);
}
/**
*
*
*
* Removes an attribute/facet in the request if is present.
*
* * Rule Condition:
* Must specify non-empty
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* (for search only) or
* [Condition.page_categories][google.cloud.retail.v2alpha.Condition.page_categories]
* (for browse only), but can't specify both.
*
* * Action Input: attribute name
*
* * Action Result: Will remove the attribute (as a facet) from the request
* if it is present.
*
* Example: Suppose the query is "shoes", the
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* is "shoes" and the attribute name "size", then facet key "size" will be
* removed from the request (if it is present).
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.RemoveFacetAction}
*/
public static final class RemoveFacetAction extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.Rule.RemoveFacetAction)
RemoveFacetActionOrBuilder {
private static final long serialVersionUID = 0L;
// Use RemoveFacetAction.newBuilder() to construct.
private RemoveFacetAction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RemoveFacetAction() {
attributeNames_ = com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new RemoveFacetAction();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_RemoveFacetAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_RemoveFacetAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.class,
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.Builder.class);
}
public static final int ATTRIBUTE_NAMES_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList attributeNames_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @return A list containing the attributeNames.
*/
public com.google.protobuf.ProtocolStringList getAttributeNamesList() {
return attributeNames_;
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @return The count of attributeNames.
*/
public int getAttributeNamesCount() {
return attributeNames_.size();
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @param index The index of the element to return.
* @return The attributeNames at the given index.
*/
public java.lang.String getAttributeNames(int index) {
return attributeNames_.get(index);
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @param index The index of the value to return.
* @return The bytes of the attributeNames at the given index.
*/
public com.google.protobuf.ByteString getAttributeNamesBytes(int index) {
return attributeNames_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
for (int i = 0; i < attributeNames_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, attributeNames_.getRaw(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < attributeNames_.size(); i++) {
dataSize += computeStringSizeNoTag(attributeNames_.getRaw(i));
}
size += dataSize;
size += 1 * getAttributeNamesList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction other =
(com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction) obj;
if (!getAttributeNamesList().equals(other.getAttributeNamesList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getAttributeNamesCount() > 0) {
hash = (37 * hash) + ATTRIBUTE_NAMES_FIELD_NUMBER;
hash = (53 * hash) + getAttributeNamesList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseFrom(
java.nio.ByteBuffer data) throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Removes an attribute/facet in the request if is present.
*
* * Rule Condition:
* Must specify non-empty
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* (for search only) or
* [Condition.page_categories][google.cloud.retail.v2alpha.Condition.page_categories]
* (for browse only), but can't specify both.
*
* * Action Input: attribute name
*
* * Action Result: Will remove the attribute (as a facet) from the request
* if it is present.
*
* Example: Suppose the query is "shoes", the
* [Condition.query_terms][google.cloud.retail.v2alpha.Condition.query_terms]
* is "shoes" and the attribute name "size", then facet key "size" will be
* removed from the request (if it is present).
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule.RemoveFacetAction}
*/
public static final class Builder
extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule.RemoveFacetAction)
com.google.cloud.retail.v2alpha.Rule.RemoveFacetActionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_RemoveFacetAction_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_RemoveFacetAction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.class,
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.newBuilder()
private Builder() {}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
attributeNames_ = com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_RemoveFacetAction_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction build() {
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction buildPartial() {
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction result =
new com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
attributeNames_.makeImmutable();
result.attributeNames_ = attributeNames_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index,
java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction) {
return mergeFrom((com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction other) {
if (other == com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.getDefaultInstance())
return this;
if (!other.attributeNames_.isEmpty()) {
if (attributeNames_.isEmpty()) {
attributeNames_ = other.attributeNames_;
bitField0_ |= 0x00000001;
} else {
ensureAttributeNamesIsMutable();
attributeNames_.addAll(other.attributeNames_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
java.lang.String s = input.readStringRequireUtf8();
ensureAttributeNamesIsMutable();
attributeNames_.add(s);
break;
} // case 10
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList attributeNames_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureAttributeNamesIsMutable() {
if (!attributeNames_.isModifiable()) {
attributeNames_ = new com.google.protobuf.LazyStringArrayList(attributeNames_);
}
bitField0_ |= 0x00000001;
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @return A list containing the attributeNames.
*/
public com.google.protobuf.ProtocolStringList getAttributeNamesList() {
attributeNames_.makeImmutable();
return attributeNames_;
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @return The count of attributeNames.
*/
public int getAttributeNamesCount() {
return attributeNames_.size();
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @param index The index of the element to return.
* @return The attributeNames at the given index.
*/
public java.lang.String getAttributeNames(int index) {
return attributeNames_.get(index);
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @param index The index of the value to return.
* @return The bytes of the attributeNames at the given index.
*/
public com.google.protobuf.ByteString getAttributeNamesBytes(int index) {
return attributeNames_.getByteString(index);
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @param index The index to set the value at.
* @param value The attributeNames to set.
* @return This builder for chaining.
*/
public Builder setAttributeNames(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttributeNamesIsMutable();
attributeNames_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @param value The attributeNames to add.
* @return This builder for chaining.
*/
public Builder addAttributeNames(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureAttributeNamesIsMutable();
attributeNames_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @param values The attributeNames to add.
* @return This builder for chaining.
*/
public Builder addAllAttributeNames(java.lang.Iterable values) {
ensureAttributeNamesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, attributeNames_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @return This builder for chaining.
*/
public Builder clearAttributeNames() {
attributeNames_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
;
onChanged();
return this;
}
/**
*
*
*
* The attribute names (i.e. facet keys) to remove from the dynamic facets
* (if present in the request). There can't be more 3 attribute names.
* Each attribute name should be a valid attribute name, be non-empty and
* contain at most 80 characters.
*
*
* repeated string attribute_names = 1;
*
* @param value The bytes of the attributeNames to add.
* @return This builder for chaining.
*/
public Builder addAttributeNamesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureAttributeNamesIsMutable();
attributeNames_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule.RemoveFacetAction)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule.RemoveFacetAction)
private static final com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction();
}
public static com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RemoveFacetAction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException()
.setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
private int actionCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object action_;
public enum ActionCase
implements
com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
BOOST_ACTION(2),
REDIRECT_ACTION(3),
ONEWAY_SYNONYMS_ACTION(6),
DO_NOT_ASSOCIATE_ACTION(7),
REPLACEMENT_ACTION(8),
IGNORE_ACTION(9),
FILTER_ACTION(10),
TWOWAY_SYNONYMS_ACTION(11),
FORCE_RETURN_FACET_ACTION(12),
REMOVE_FACET_ACTION(13),
ACTION_NOT_SET(0);
private final int value;
private ActionCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ActionCase valueOf(int value) {
return forNumber(value);
}
public static ActionCase forNumber(int value) {
switch (value) {
case 2:
return BOOST_ACTION;
case 3:
return REDIRECT_ACTION;
case 6:
return ONEWAY_SYNONYMS_ACTION;
case 7:
return DO_NOT_ASSOCIATE_ACTION;
case 8:
return REPLACEMENT_ACTION;
case 9:
return IGNORE_ACTION;
case 10:
return FILTER_ACTION;
case 11:
return TWOWAY_SYNONYMS_ACTION;
case 12:
return FORCE_RETURN_FACET_ACTION;
case 13:
return REMOVE_FACET_ACTION;
case 0:
return ACTION_NOT_SET;
default:
return null;
}
}
public int getNumber() {
return this.value;
}
};
public ActionCase getActionCase() {
return ActionCase.forNumber(actionCase_);
}
public static final int BOOST_ACTION_FIELD_NUMBER = 2;
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*
* @return Whether the boostAction field is set.
*/
@java.lang.Override
public boolean hasBoostAction() {
return actionCase_ == 2;
}
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*
* @return The boostAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.BoostAction getBoostAction() {
if (actionCase_ == 2) {
return (com.google.cloud.retail.v2alpha.Rule.BoostAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.BoostAction.getDefaultInstance();
}
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.BoostActionOrBuilder getBoostActionOrBuilder() {
if (actionCase_ == 2) {
return (com.google.cloud.retail.v2alpha.Rule.BoostAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.BoostAction.getDefaultInstance();
}
public static final int REDIRECT_ACTION_FIELD_NUMBER = 3;
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*
* @return Whether the redirectAction field is set.
*/
@java.lang.Override
public boolean hasRedirectAction() {
return actionCase_ == 3;
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*
* @return The redirectAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RedirectAction getRedirectAction() {
if (actionCase_ == 3) {
return (com.google.cloud.retail.v2alpha.Rule.RedirectAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.RedirectAction.getDefaultInstance();
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RedirectActionOrBuilder getRedirectActionOrBuilder() {
if (actionCase_ == 3) {
return (com.google.cloud.retail.v2alpha.Rule.RedirectAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.RedirectAction.getDefaultInstance();
}
public static final int ONEWAY_SYNONYMS_ACTION_FIELD_NUMBER = 6;
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*
* @return Whether the onewaySynonymsAction field is set.
*/
@java.lang.Override
public boolean hasOnewaySynonymsAction() {
return actionCase_ == 6;
}
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*
* @return The onewaySynonymsAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction getOnewaySynonymsAction() {
if (actionCase_ == 6) {
return (com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.getDefaultInstance();
}
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsActionOrBuilder
getOnewaySynonymsActionOrBuilder() {
if (actionCase_ == 6) {
return (com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.getDefaultInstance();
}
public static final int DO_NOT_ASSOCIATE_ACTION_FIELD_NUMBER = 7;
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*
* @return Whether the doNotAssociateAction field is set.
*/
@java.lang.Override
public boolean hasDoNotAssociateAction() {
return actionCase_ == 7;
}
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*
* @return The doNotAssociateAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction getDoNotAssociateAction() {
if (actionCase_ == 7) {
return (com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.getDefaultInstance();
}
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.DoNotAssociateActionOrBuilder
getDoNotAssociateActionOrBuilder() {
if (actionCase_ == 7) {
return (com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.getDefaultInstance();
}
public static final int REPLACEMENT_ACTION_FIELD_NUMBER = 8;
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*
* @return Whether the replacementAction field is set.
*/
@java.lang.Override
public boolean hasReplacementAction() {
return actionCase_ == 8;
}
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*
* @return The replacementAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ReplacementAction getReplacementAction() {
if (actionCase_ == 8) {
return (com.google.cloud.retail.v2alpha.Rule.ReplacementAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.ReplacementAction.getDefaultInstance();
}
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ReplacementActionOrBuilder
getReplacementActionOrBuilder() {
if (actionCase_ == 8) {
return (com.google.cloud.retail.v2alpha.Rule.ReplacementAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.ReplacementAction.getDefaultInstance();
}
public static final int IGNORE_ACTION_FIELD_NUMBER = 9;
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*
* @return Whether the ignoreAction field is set.
*/
@java.lang.Override
public boolean hasIgnoreAction() {
return actionCase_ == 9;
}
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*
* @return The ignoreAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.IgnoreAction getIgnoreAction() {
if (actionCase_ == 9) {
return (com.google.cloud.retail.v2alpha.Rule.IgnoreAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.IgnoreAction.getDefaultInstance();
}
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.IgnoreActionOrBuilder getIgnoreActionOrBuilder() {
if (actionCase_ == 9) {
return (com.google.cloud.retail.v2alpha.Rule.IgnoreAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.IgnoreAction.getDefaultInstance();
}
public static final int FILTER_ACTION_FIELD_NUMBER = 10;
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*
* @return Whether the filterAction field is set.
*/
@java.lang.Override
public boolean hasFilterAction() {
return actionCase_ == 10;
}
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*
* @return The filterAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.FilterAction getFilterAction() {
if (actionCase_ == 10) {
return (com.google.cloud.retail.v2alpha.Rule.FilterAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.FilterAction.getDefaultInstance();
}
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.FilterActionOrBuilder getFilterActionOrBuilder() {
if (actionCase_ == 10) {
return (com.google.cloud.retail.v2alpha.Rule.FilterAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.FilterAction.getDefaultInstance();
}
public static final int TWOWAY_SYNONYMS_ACTION_FIELD_NUMBER = 11;
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*
* @return Whether the twowaySynonymsAction field is set.
*/
@java.lang.Override
public boolean hasTwowaySynonymsAction() {
return actionCase_ == 11;
}
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*
* @return The twowaySynonymsAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction getTwowaySynonymsAction() {
if (actionCase_ == 11) {
return (com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.getDefaultInstance();
}
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsActionOrBuilder
getTwowaySynonymsActionOrBuilder() {
if (actionCase_ == 11) {
return (com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.getDefaultInstance();
}
public static final int FORCE_RETURN_FACET_ACTION_FIELD_NUMBER = 12;
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*
* @return Whether the forceReturnFacetAction field is set.
*/
@java.lang.Override
public boolean hasForceReturnFacetAction() {
return actionCase_ == 12;
}
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*
* @return The forceReturnFacetAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction getForceReturnFacetAction() {
if (actionCase_ == 12) {
return (com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.getDefaultInstance();
}
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetActionOrBuilder
getForceReturnFacetActionOrBuilder() {
if (actionCase_ == 12) {
return (com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.getDefaultInstance();
}
public static final int REMOVE_FACET_ACTION_FIELD_NUMBER = 13;
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*
* @return Whether the removeFacetAction field is set.
*/
@java.lang.Override
public boolean hasRemoveFacetAction() {
return actionCase_ == 13;
}
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*
* @return The removeFacetAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction getRemoveFacetAction() {
if (actionCase_ == 13) {
return (com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.getDefaultInstance();
}
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RemoveFacetActionOrBuilder
getRemoveFacetActionOrBuilder() {
if (actionCase_ == 13) {
return (com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.getDefaultInstance();
}
public static final int CONDITION_FIELD_NUMBER = 1;
private com.google.cloud.retail.v2alpha.Condition condition_;
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the condition field is set.
*/
@java.lang.Override
public boolean hasCondition() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The condition.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Condition getCondition() {
return condition_ == null
? com.google.cloud.retail.v2alpha.Condition.getDefaultInstance()
: condition_;
}
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.ConditionOrBuilder getConditionOrBuilder() {
return condition_ == null
? com.google.cloud.retail.v2alpha.Condition.getDefaultInstance()
: condition_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getCondition());
}
if (actionCase_ == 2) {
output.writeMessage(2, (com.google.cloud.retail.v2alpha.Rule.BoostAction) action_);
}
if (actionCase_ == 3) {
output.writeMessage(3, (com.google.cloud.retail.v2alpha.Rule.RedirectAction) action_);
}
if (actionCase_ == 6) {
output.writeMessage(6, (com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction) action_);
}
if (actionCase_ == 7) {
output.writeMessage(7, (com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction) action_);
}
if (actionCase_ == 8) {
output.writeMessage(8, (com.google.cloud.retail.v2alpha.Rule.ReplacementAction) action_);
}
if (actionCase_ == 9) {
output.writeMessage(9, (com.google.cloud.retail.v2alpha.Rule.IgnoreAction) action_);
}
if (actionCase_ == 10) {
output.writeMessage(10, (com.google.cloud.retail.v2alpha.Rule.FilterAction) action_);
}
if (actionCase_ == 11) {
output.writeMessage(11, (com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction) action_);
}
if (actionCase_ == 12) {
output.writeMessage(
12, (com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction) action_);
}
if (actionCase_ == 13) {
output.writeMessage(13, (com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction) action_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(1, getCondition());
}
if (actionCase_ == 2) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
2, (com.google.cloud.retail.v2alpha.Rule.BoostAction) action_);
}
if (actionCase_ == 3) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
3, (com.google.cloud.retail.v2alpha.Rule.RedirectAction) action_);
}
if (actionCase_ == 6) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
6, (com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction) action_);
}
if (actionCase_ == 7) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
7, (com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction) action_);
}
if (actionCase_ == 8) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
8, (com.google.cloud.retail.v2alpha.Rule.ReplacementAction) action_);
}
if (actionCase_ == 9) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
9, (com.google.cloud.retail.v2alpha.Rule.IgnoreAction) action_);
}
if (actionCase_ == 10) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
10, (com.google.cloud.retail.v2alpha.Rule.FilterAction) action_);
}
if (actionCase_ == 11) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
11, (com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction) action_);
}
if (actionCase_ == 12) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
12, (com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction) action_);
}
if (actionCase_ == 13) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(
13, (com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction) action_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.Rule)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.Rule other = (com.google.cloud.retail.v2alpha.Rule) obj;
if (hasCondition() != other.hasCondition()) return false;
if (hasCondition()) {
if (!getCondition().equals(other.getCondition())) return false;
}
if (!getActionCase().equals(other.getActionCase())) return false;
switch (actionCase_) {
case 2:
if (!getBoostAction().equals(other.getBoostAction())) return false;
break;
case 3:
if (!getRedirectAction().equals(other.getRedirectAction())) return false;
break;
case 6:
if (!getOnewaySynonymsAction().equals(other.getOnewaySynonymsAction())) return false;
break;
case 7:
if (!getDoNotAssociateAction().equals(other.getDoNotAssociateAction())) return false;
break;
case 8:
if (!getReplacementAction().equals(other.getReplacementAction())) return false;
break;
case 9:
if (!getIgnoreAction().equals(other.getIgnoreAction())) return false;
break;
case 10:
if (!getFilterAction().equals(other.getFilterAction())) return false;
break;
case 11:
if (!getTwowaySynonymsAction().equals(other.getTwowaySynonymsAction())) return false;
break;
case 12:
if (!getForceReturnFacetAction().equals(other.getForceReturnFacetAction())) return false;
break;
case 13:
if (!getRemoveFacetAction().equals(other.getRemoveFacetAction())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasCondition()) {
hash = (37 * hash) + CONDITION_FIELD_NUMBER;
hash = (53 * hash) + getCondition().hashCode();
}
switch (actionCase_) {
case 2:
hash = (37 * hash) + BOOST_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getBoostAction().hashCode();
break;
case 3:
hash = (37 * hash) + REDIRECT_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getRedirectAction().hashCode();
break;
case 6:
hash = (37 * hash) + ONEWAY_SYNONYMS_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getOnewaySynonymsAction().hashCode();
break;
case 7:
hash = (37 * hash) + DO_NOT_ASSOCIATE_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getDoNotAssociateAction().hashCode();
break;
case 8:
hash = (37 * hash) + REPLACEMENT_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getReplacementAction().hashCode();
break;
case 9:
hash = (37 * hash) + IGNORE_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getIgnoreAction().hashCode();
break;
case 10:
hash = (37 * hash) + FILTER_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getFilterAction().hashCode();
break;
case 11:
hash = (37 * hash) + TWOWAY_SYNONYMS_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getTwowaySynonymsAction().hashCode();
break;
case 12:
hash = (37 * hash) + FORCE_RETURN_FACET_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getForceReturnFacetAction().hashCode();
break;
case 13:
hash = (37 * hash) + REMOVE_FACET_ACTION_FIELD_NUMBER;
hash = (53 * hash) + getRemoveFacetAction().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.Rule parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule parseFrom(com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.Rule parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.Rule parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.Rule parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.retail.v2alpha.Rule prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* A rule is a condition-action pair
*
* * A condition defines when a rule is to be triggered.
* * An action specifies what occurs on that trigger.
* Currently rules only work for [controls][google.cloud.retail.v2alpha.Control]
* with
* [SOLUTION_TYPE_SEARCH][google.cloud.retail.v2alpha.SolutionType.SOLUTION_TYPE_SEARCH].
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.Rule}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.Rule)
com.google.cloud.retail.v2alpha.RuleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.Rule.class,
com.google.cloud.retail.v2alpha.Rule.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.Rule.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getConditionFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (boostActionBuilder_ != null) {
boostActionBuilder_.clear();
}
if (redirectActionBuilder_ != null) {
redirectActionBuilder_.clear();
}
if (onewaySynonymsActionBuilder_ != null) {
onewaySynonymsActionBuilder_.clear();
}
if (doNotAssociateActionBuilder_ != null) {
doNotAssociateActionBuilder_.clear();
}
if (replacementActionBuilder_ != null) {
replacementActionBuilder_.clear();
}
if (ignoreActionBuilder_ != null) {
ignoreActionBuilder_.clear();
}
if (filterActionBuilder_ != null) {
filterActionBuilder_.clear();
}
if (twowaySynonymsActionBuilder_ != null) {
twowaySynonymsActionBuilder_.clear();
}
if (forceReturnFacetActionBuilder_ != null) {
forceReturnFacetActionBuilder_.clear();
}
if (removeFacetActionBuilder_ != null) {
removeFacetActionBuilder_.clear();
}
condition_ = null;
if (conditionBuilder_ != null) {
conditionBuilder_.dispose();
conditionBuilder_ = null;
}
actionCase_ = 0;
action_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.CommonProto
.internal_static_google_cloud_retail_v2alpha_Rule_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.Rule.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule build() {
com.google.cloud.retail.v2alpha.Rule result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule buildPartial() {
com.google.cloud.retail.v2alpha.Rule result = new com.google.cloud.retail.v2alpha.Rule(this);
if (bitField0_ != 0) {
buildPartial0(result);
}
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartial0(com.google.cloud.retail.v2alpha.Rule result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000400) != 0)) {
result.condition_ = conditionBuilder_ == null ? condition_ : conditionBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartialOneofs(com.google.cloud.retail.v2alpha.Rule result) {
result.actionCase_ = actionCase_;
result.action_ = this.action_;
if (actionCase_ == 2 && boostActionBuilder_ != null) {
result.action_ = boostActionBuilder_.build();
}
if (actionCase_ == 3 && redirectActionBuilder_ != null) {
result.action_ = redirectActionBuilder_.build();
}
if (actionCase_ == 6 && onewaySynonymsActionBuilder_ != null) {
result.action_ = onewaySynonymsActionBuilder_.build();
}
if (actionCase_ == 7 && doNotAssociateActionBuilder_ != null) {
result.action_ = doNotAssociateActionBuilder_.build();
}
if (actionCase_ == 8 && replacementActionBuilder_ != null) {
result.action_ = replacementActionBuilder_.build();
}
if (actionCase_ == 9 && ignoreActionBuilder_ != null) {
result.action_ = ignoreActionBuilder_.build();
}
if (actionCase_ == 10 && filterActionBuilder_ != null) {
result.action_ = filterActionBuilder_.build();
}
if (actionCase_ == 11 && twowaySynonymsActionBuilder_ != null) {
result.action_ = twowaySynonymsActionBuilder_.build();
}
if (actionCase_ == 12 && forceReturnFacetActionBuilder_ != null) {
result.action_ = forceReturnFacetActionBuilder_.build();
}
if (actionCase_ == 13 && removeFacetActionBuilder_ != null) {
result.action_ = removeFacetActionBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.Rule) {
return mergeFrom((com.google.cloud.retail.v2alpha.Rule) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.Rule other) {
if (other == com.google.cloud.retail.v2alpha.Rule.getDefaultInstance()) return this;
if (other.hasCondition()) {
mergeCondition(other.getCondition());
}
switch (other.getActionCase()) {
case BOOST_ACTION:
{
mergeBoostAction(other.getBoostAction());
break;
}
case REDIRECT_ACTION:
{
mergeRedirectAction(other.getRedirectAction());
break;
}
case ONEWAY_SYNONYMS_ACTION:
{
mergeOnewaySynonymsAction(other.getOnewaySynonymsAction());
break;
}
case DO_NOT_ASSOCIATE_ACTION:
{
mergeDoNotAssociateAction(other.getDoNotAssociateAction());
break;
}
case REPLACEMENT_ACTION:
{
mergeReplacementAction(other.getReplacementAction());
break;
}
case IGNORE_ACTION:
{
mergeIgnoreAction(other.getIgnoreAction());
break;
}
case FILTER_ACTION:
{
mergeFilterAction(other.getFilterAction());
break;
}
case TWOWAY_SYNONYMS_ACTION:
{
mergeTwowaySynonymsAction(other.getTwowaySynonymsAction());
break;
}
case FORCE_RETURN_FACET_ACTION:
{
mergeForceReturnFacetAction(other.getForceReturnFacetAction());
break;
}
case REMOVE_FACET_ACTION:
{
mergeRemoveFacetAction(other.getRemoveFacetAction());
break;
}
case ACTION_NOT_SET:
{
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
input.readMessage(getConditionFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000400;
break;
} // case 10
case 18:
{
input.readMessage(getBoostActionFieldBuilder().getBuilder(), extensionRegistry);
actionCase_ = 2;
break;
} // case 18
case 26:
{
input.readMessage(getRedirectActionFieldBuilder().getBuilder(), extensionRegistry);
actionCase_ = 3;
break;
} // case 26
case 50:
{
input.readMessage(
getOnewaySynonymsActionFieldBuilder().getBuilder(), extensionRegistry);
actionCase_ = 6;
break;
} // case 50
case 58:
{
input.readMessage(
getDoNotAssociateActionFieldBuilder().getBuilder(), extensionRegistry);
actionCase_ = 7;
break;
} // case 58
case 66:
{
input.readMessage(
getReplacementActionFieldBuilder().getBuilder(), extensionRegistry);
actionCase_ = 8;
break;
} // case 66
case 74:
{
input.readMessage(getIgnoreActionFieldBuilder().getBuilder(), extensionRegistry);
actionCase_ = 9;
break;
} // case 74
case 82:
{
input.readMessage(getFilterActionFieldBuilder().getBuilder(), extensionRegistry);
actionCase_ = 10;
break;
} // case 82
case 90:
{
input.readMessage(
getTwowaySynonymsActionFieldBuilder().getBuilder(), extensionRegistry);
actionCase_ = 11;
break;
} // case 90
case 98:
{
input.readMessage(
getForceReturnFacetActionFieldBuilder().getBuilder(), extensionRegistry);
actionCase_ = 12;
break;
} // case 98
case 106:
{
input.readMessage(
getRemoveFacetActionFieldBuilder().getBuilder(), extensionRegistry);
actionCase_ = 13;
break;
} // case 106
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int actionCase_ = 0;
private java.lang.Object action_;
public ActionCase getActionCase() {
return ActionCase.forNumber(actionCase_);
}
public Builder clearAction() {
actionCase_ = 0;
action_ = null;
onChanged();
return this;
}
private int bitField0_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.BoostAction,
com.google.cloud.retail.v2alpha.Rule.BoostAction.Builder,
com.google.cloud.retail.v2alpha.Rule.BoostActionOrBuilder>
boostActionBuilder_;
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*
* @return Whether the boostAction field is set.
*/
@java.lang.Override
public boolean hasBoostAction() {
return actionCase_ == 2;
}
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*
* @return The boostAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.BoostAction getBoostAction() {
if (boostActionBuilder_ == null) {
if (actionCase_ == 2) {
return (com.google.cloud.retail.v2alpha.Rule.BoostAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.BoostAction.getDefaultInstance();
} else {
if (actionCase_ == 2) {
return boostActionBuilder_.getMessage();
}
return com.google.cloud.retail.v2alpha.Rule.BoostAction.getDefaultInstance();
}
}
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*/
public Builder setBoostAction(com.google.cloud.retail.v2alpha.Rule.BoostAction value) {
if (boostActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
boostActionBuilder_.setMessage(value);
}
actionCase_ = 2;
return this;
}
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*/
public Builder setBoostAction(
com.google.cloud.retail.v2alpha.Rule.BoostAction.Builder builderForValue) {
if (boostActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
boostActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 2;
return this;
}
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*/
public Builder mergeBoostAction(com.google.cloud.retail.v2alpha.Rule.BoostAction value) {
if (boostActionBuilder_ == null) {
if (actionCase_ == 2
&& action_ != com.google.cloud.retail.v2alpha.Rule.BoostAction.getDefaultInstance()) {
action_ =
com.google.cloud.retail.v2alpha.Rule.BoostAction.newBuilder(
(com.google.cloud.retail.v2alpha.Rule.BoostAction) action_)
.mergeFrom(value)
.buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 2) {
boostActionBuilder_.mergeFrom(value);
} else {
boostActionBuilder_.setMessage(value);
}
}
actionCase_ = 2;
return this;
}
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*/
public Builder clearBoostAction() {
if (boostActionBuilder_ == null) {
if (actionCase_ == 2) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 2) {
actionCase_ = 0;
action_ = null;
}
boostActionBuilder_.clear();
}
return this;
}
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*/
public com.google.cloud.retail.v2alpha.Rule.BoostAction.Builder getBoostActionBuilder() {
return getBoostActionFieldBuilder().getBuilder();
}
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.BoostActionOrBuilder getBoostActionOrBuilder() {
if ((actionCase_ == 2) && (boostActionBuilder_ != null)) {
return boostActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 2) {
return (com.google.cloud.retail.v2alpha.Rule.BoostAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.BoostAction.getDefaultInstance();
}
}
/**
*
*
*
* A boost action.
*
*
* .google.cloud.retail.v2alpha.Rule.BoostAction boost_action = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.BoostAction,
com.google.cloud.retail.v2alpha.Rule.BoostAction.Builder,
com.google.cloud.retail.v2alpha.Rule.BoostActionOrBuilder>
getBoostActionFieldBuilder() {
if (boostActionBuilder_ == null) {
if (!(actionCase_ == 2)) {
action_ = com.google.cloud.retail.v2alpha.Rule.BoostAction.getDefaultInstance();
}
boostActionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.BoostAction,
com.google.cloud.retail.v2alpha.Rule.BoostAction.Builder,
com.google.cloud.retail.v2alpha.Rule.BoostActionOrBuilder>(
(com.google.cloud.retail.v2alpha.Rule.BoostAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 2;
onChanged();
return boostActionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.RedirectAction,
com.google.cloud.retail.v2alpha.Rule.RedirectAction.Builder,
com.google.cloud.retail.v2alpha.Rule.RedirectActionOrBuilder>
redirectActionBuilder_;
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*
* @return Whether the redirectAction field is set.
*/
@java.lang.Override
public boolean hasRedirectAction() {
return actionCase_ == 3;
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*
* @return The redirectAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RedirectAction getRedirectAction() {
if (redirectActionBuilder_ == null) {
if (actionCase_ == 3) {
return (com.google.cloud.retail.v2alpha.Rule.RedirectAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.RedirectAction.getDefaultInstance();
} else {
if (actionCase_ == 3) {
return redirectActionBuilder_.getMessage();
}
return com.google.cloud.retail.v2alpha.Rule.RedirectAction.getDefaultInstance();
}
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*/
public Builder setRedirectAction(com.google.cloud.retail.v2alpha.Rule.RedirectAction value) {
if (redirectActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
redirectActionBuilder_.setMessage(value);
}
actionCase_ = 3;
return this;
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*/
public Builder setRedirectAction(
com.google.cloud.retail.v2alpha.Rule.RedirectAction.Builder builderForValue) {
if (redirectActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
redirectActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 3;
return this;
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*/
public Builder mergeRedirectAction(com.google.cloud.retail.v2alpha.Rule.RedirectAction value) {
if (redirectActionBuilder_ == null) {
if (actionCase_ == 3
&& action_
!= com.google.cloud.retail.v2alpha.Rule.RedirectAction.getDefaultInstance()) {
action_ =
com.google.cloud.retail.v2alpha.Rule.RedirectAction.newBuilder(
(com.google.cloud.retail.v2alpha.Rule.RedirectAction) action_)
.mergeFrom(value)
.buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 3) {
redirectActionBuilder_.mergeFrom(value);
} else {
redirectActionBuilder_.setMessage(value);
}
}
actionCase_ = 3;
return this;
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*/
public Builder clearRedirectAction() {
if (redirectActionBuilder_ == null) {
if (actionCase_ == 3) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 3) {
actionCase_ = 0;
action_ = null;
}
redirectActionBuilder_.clear();
}
return this;
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*/
public com.google.cloud.retail.v2alpha.Rule.RedirectAction.Builder getRedirectActionBuilder() {
return getRedirectActionFieldBuilder().getBuilder();
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RedirectActionOrBuilder
getRedirectActionOrBuilder() {
if ((actionCase_ == 3) && (redirectActionBuilder_ != null)) {
return redirectActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 3) {
return (com.google.cloud.retail.v2alpha.Rule.RedirectAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.RedirectAction.getDefaultInstance();
}
}
/**
*
*
*
* Redirects a shopper to a specific page.
*
*
* .google.cloud.retail.v2alpha.Rule.RedirectAction redirect_action = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.RedirectAction,
com.google.cloud.retail.v2alpha.Rule.RedirectAction.Builder,
com.google.cloud.retail.v2alpha.Rule.RedirectActionOrBuilder>
getRedirectActionFieldBuilder() {
if (redirectActionBuilder_ == null) {
if (!(actionCase_ == 3)) {
action_ = com.google.cloud.retail.v2alpha.Rule.RedirectAction.getDefaultInstance();
}
redirectActionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.RedirectAction,
com.google.cloud.retail.v2alpha.Rule.RedirectAction.Builder,
com.google.cloud.retail.v2alpha.Rule.RedirectActionOrBuilder>(
(com.google.cloud.retail.v2alpha.Rule.RedirectAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 3;
onChanged();
return redirectActionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction,
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.Builder,
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsActionOrBuilder>
onewaySynonymsActionBuilder_;
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*
*
* @return Whether the onewaySynonymsAction field is set.
*/
@java.lang.Override
public boolean hasOnewaySynonymsAction() {
return actionCase_ == 6;
}
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*
*
* @return The onewaySynonymsAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction getOnewaySynonymsAction() {
if (onewaySynonymsActionBuilder_ == null) {
if (actionCase_ == 6) {
return (com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.getDefaultInstance();
} else {
if (actionCase_ == 6) {
return onewaySynonymsActionBuilder_.getMessage();
}
return com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.getDefaultInstance();
}
}
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*
*/
public Builder setOnewaySynonymsAction(
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction value) {
if (onewaySynonymsActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
onewaySynonymsActionBuilder_.setMessage(value);
}
actionCase_ = 6;
return this;
}
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*
*/
public Builder setOnewaySynonymsAction(
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.Builder builderForValue) {
if (onewaySynonymsActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
onewaySynonymsActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 6;
return this;
}
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*
*/
public Builder mergeOnewaySynonymsAction(
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction value) {
if (onewaySynonymsActionBuilder_ == null) {
if (actionCase_ == 6
&& action_
!= com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.getDefaultInstance()) {
action_ =
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.newBuilder(
(com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction) action_)
.mergeFrom(value)
.buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 6) {
onewaySynonymsActionBuilder_.mergeFrom(value);
} else {
onewaySynonymsActionBuilder_.setMessage(value);
}
}
actionCase_ = 6;
return this;
}
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*
*/
public Builder clearOnewaySynonymsAction() {
if (onewaySynonymsActionBuilder_ == null) {
if (actionCase_ == 6) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 6) {
actionCase_ = 0;
action_ = null;
}
onewaySynonymsActionBuilder_.clear();
}
return this;
}
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*
*/
public com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.Builder
getOnewaySynonymsActionBuilder() {
return getOnewaySynonymsActionFieldBuilder().getBuilder();
}
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsActionOrBuilder
getOnewaySynonymsActionOrBuilder() {
if ((actionCase_ == 6) && (onewaySynonymsActionBuilder_ != null)) {
return onewaySynonymsActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 6) {
return (com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.getDefaultInstance();
}
}
/**
*
*
*
* Treats specific term as a synonym with a group of terms.
* Group of terms will not be treated as synonyms with the specific term.
*
*
* .google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction oneway_synonyms_action = 6;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction,
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.Builder,
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsActionOrBuilder>
getOnewaySynonymsActionFieldBuilder() {
if (onewaySynonymsActionBuilder_ == null) {
if (!(actionCase_ == 6)) {
action_ = com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.getDefaultInstance();
}
onewaySynonymsActionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction,
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction.Builder,
com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsActionOrBuilder>(
(com.google.cloud.retail.v2alpha.Rule.OnewaySynonymsAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 6;
onChanged();
return onewaySynonymsActionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction,
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.Builder,
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateActionOrBuilder>
doNotAssociateActionBuilder_;
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*
* @return Whether the doNotAssociateAction field is set.
*/
@java.lang.Override
public boolean hasDoNotAssociateAction() {
return actionCase_ == 7;
}
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*
* @return The doNotAssociateAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction getDoNotAssociateAction() {
if (doNotAssociateActionBuilder_ == null) {
if (actionCase_ == 7) {
return (com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.getDefaultInstance();
} else {
if (actionCase_ == 7) {
return doNotAssociateActionBuilder_.getMessage();
}
return com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.getDefaultInstance();
}
}
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*/
public Builder setDoNotAssociateAction(
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction value) {
if (doNotAssociateActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
doNotAssociateActionBuilder_.setMessage(value);
}
actionCase_ = 7;
return this;
}
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*/
public Builder setDoNotAssociateAction(
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.Builder builderForValue) {
if (doNotAssociateActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
doNotAssociateActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 7;
return this;
}
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*/
public Builder mergeDoNotAssociateAction(
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction value) {
if (doNotAssociateActionBuilder_ == null) {
if (actionCase_ == 7
&& action_
!= com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.getDefaultInstance()) {
action_ =
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.newBuilder(
(com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction) action_)
.mergeFrom(value)
.buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 7) {
doNotAssociateActionBuilder_.mergeFrom(value);
} else {
doNotAssociateActionBuilder_.setMessage(value);
}
}
actionCase_ = 7;
return this;
}
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*/
public Builder clearDoNotAssociateAction() {
if (doNotAssociateActionBuilder_ == null) {
if (actionCase_ == 7) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 7) {
actionCase_ = 0;
action_ = null;
}
doNotAssociateActionBuilder_.clear();
}
return this;
}
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*/
public com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.Builder
getDoNotAssociateActionBuilder() {
return getDoNotAssociateActionFieldBuilder().getBuilder();
}
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.DoNotAssociateActionOrBuilder
getDoNotAssociateActionOrBuilder() {
if ((actionCase_ == 7) && (doNotAssociateActionBuilder_ != null)) {
return doNotAssociateActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 7) {
return (com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.getDefaultInstance();
}
}
/**
*
*
*
* Prevents term from being associated with other terms.
*
*
* .google.cloud.retail.v2alpha.Rule.DoNotAssociateAction do_not_associate_action = 7;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction,
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.Builder,
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateActionOrBuilder>
getDoNotAssociateActionFieldBuilder() {
if (doNotAssociateActionBuilder_ == null) {
if (!(actionCase_ == 7)) {
action_ = com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.getDefaultInstance();
}
doNotAssociateActionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction,
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction.Builder,
com.google.cloud.retail.v2alpha.Rule.DoNotAssociateActionOrBuilder>(
(com.google.cloud.retail.v2alpha.Rule.DoNotAssociateAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 7;
onChanged();
return doNotAssociateActionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.ReplacementAction,
com.google.cloud.retail.v2alpha.Rule.ReplacementAction.Builder,
com.google.cloud.retail.v2alpha.Rule.ReplacementActionOrBuilder>
replacementActionBuilder_;
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*
* @return Whether the replacementAction field is set.
*/
@java.lang.Override
public boolean hasReplacementAction() {
return actionCase_ == 8;
}
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*
* @return The replacementAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ReplacementAction getReplacementAction() {
if (replacementActionBuilder_ == null) {
if (actionCase_ == 8) {
return (com.google.cloud.retail.v2alpha.Rule.ReplacementAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.ReplacementAction.getDefaultInstance();
} else {
if (actionCase_ == 8) {
return replacementActionBuilder_.getMessage();
}
return com.google.cloud.retail.v2alpha.Rule.ReplacementAction.getDefaultInstance();
}
}
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*/
public Builder setReplacementAction(
com.google.cloud.retail.v2alpha.Rule.ReplacementAction value) {
if (replacementActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
replacementActionBuilder_.setMessage(value);
}
actionCase_ = 8;
return this;
}
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*/
public Builder setReplacementAction(
com.google.cloud.retail.v2alpha.Rule.ReplacementAction.Builder builderForValue) {
if (replacementActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
replacementActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 8;
return this;
}
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*/
public Builder mergeReplacementAction(
com.google.cloud.retail.v2alpha.Rule.ReplacementAction value) {
if (replacementActionBuilder_ == null) {
if (actionCase_ == 8
&& action_
!= com.google.cloud.retail.v2alpha.Rule.ReplacementAction.getDefaultInstance()) {
action_ =
com.google.cloud.retail.v2alpha.Rule.ReplacementAction.newBuilder(
(com.google.cloud.retail.v2alpha.Rule.ReplacementAction) action_)
.mergeFrom(value)
.buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 8) {
replacementActionBuilder_.mergeFrom(value);
} else {
replacementActionBuilder_.setMessage(value);
}
}
actionCase_ = 8;
return this;
}
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*/
public Builder clearReplacementAction() {
if (replacementActionBuilder_ == null) {
if (actionCase_ == 8) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 8) {
actionCase_ = 0;
action_ = null;
}
replacementActionBuilder_.clear();
}
return this;
}
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*/
public com.google.cloud.retail.v2alpha.Rule.ReplacementAction.Builder
getReplacementActionBuilder() {
return getReplacementActionFieldBuilder().getBuilder();
}
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ReplacementActionOrBuilder
getReplacementActionOrBuilder() {
if ((actionCase_ == 8) && (replacementActionBuilder_ != null)) {
return replacementActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 8) {
return (com.google.cloud.retail.v2alpha.Rule.ReplacementAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.ReplacementAction.getDefaultInstance();
}
}
/**
*
*
*
* Replaces specific terms in the query.
*
*
* .google.cloud.retail.v2alpha.Rule.ReplacementAction replacement_action = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.ReplacementAction,
com.google.cloud.retail.v2alpha.Rule.ReplacementAction.Builder,
com.google.cloud.retail.v2alpha.Rule.ReplacementActionOrBuilder>
getReplacementActionFieldBuilder() {
if (replacementActionBuilder_ == null) {
if (!(actionCase_ == 8)) {
action_ = com.google.cloud.retail.v2alpha.Rule.ReplacementAction.getDefaultInstance();
}
replacementActionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.ReplacementAction,
com.google.cloud.retail.v2alpha.Rule.ReplacementAction.Builder,
com.google.cloud.retail.v2alpha.Rule.ReplacementActionOrBuilder>(
(com.google.cloud.retail.v2alpha.Rule.ReplacementAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 8;
onChanged();
return replacementActionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.IgnoreAction,
com.google.cloud.retail.v2alpha.Rule.IgnoreAction.Builder,
com.google.cloud.retail.v2alpha.Rule.IgnoreActionOrBuilder>
ignoreActionBuilder_;
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*
* @return Whether the ignoreAction field is set.
*/
@java.lang.Override
public boolean hasIgnoreAction() {
return actionCase_ == 9;
}
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*
* @return The ignoreAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.IgnoreAction getIgnoreAction() {
if (ignoreActionBuilder_ == null) {
if (actionCase_ == 9) {
return (com.google.cloud.retail.v2alpha.Rule.IgnoreAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.IgnoreAction.getDefaultInstance();
} else {
if (actionCase_ == 9) {
return ignoreActionBuilder_.getMessage();
}
return com.google.cloud.retail.v2alpha.Rule.IgnoreAction.getDefaultInstance();
}
}
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*/
public Builder setIgnoreAction(com.google.cloud.retail.v2alpha.Rule.IgnoreAction value) {
if (ignoreActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
ignoreActionBuilder_.setMessage(value);
}
actionCase_ = 9;
return this;
}
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*/
public Builder setIgnoreAction(
com.google.cloud.retail.v2alpha.Rule.IgnoreAction.Builder builderForValue) {
if (ignoreActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
ignoreActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 9;
return this;
}
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*/
public Builder mergeIgnoreAction(com.google.cloud.retail.v2alpha.Rule.IgnoreAction value) {
if (ignoreActionBuilder_ == null) {
if (actionCase_ == 9
&& action_ != com.google.cloud.retail.v2alpha.Rule.IgnoreAction.getDefaultInstance()) {
action_ =
com.google.cloud.retail.v2alpha.Rule.IgnoreAction.newBuilder(
(com.google.cloud.retail.v2alpha.Rule.IgnoreAction) action_)
.mergeFrom(value)
.buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 9) {
ignoreActionBuilder_.mergeFrom(value);
} else {
ignoreActionBuilder_.setMessage(value);
}
}
actionCase_ = 9;
return this;
}
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*/
public Builder clearIgnoreAction() {
if (ignoreActionBuilder_ == null) {
if (actionCase_ == 9) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 9) {
actionCase_ = 0;
action_ = null;
}
ignoreActionBuilder_.clear();
}
return this;
}
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*/
public com.google.cloud.retail.v2alpha.Rule.IgnoreAction.Builder getIgnoreActionBuilder() {
return getIgnoreActionFieldBuilder().getBuilder();
}
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.IgnoreActionOrBuilder getIgnoreActionOrBuilder() {
if ((actionCase_ == 9) && (ignoreActionBuilder_ != null)) {
return ignoreActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 9) {
return (com.google.cloud.retail.v2alpha.Rule.IgnoreAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.IgnoreAction.getDefaultInstance();
}
}
/**
*
*
*
* Ignores specific terms from query during search.
*
*
* .google.cloud.retail.v2alpha.Rule.IgnoreAction ignore_action = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.IgnoreAction,
com.google.cloud.retail.v2alpha.Rule.IgnoreAction.Builder,
com.google.cloud.retail.v2alpha.Rule.IgnoreActionOrBuilder>
getIgnoreActionFieldBuilder() {
if (ignoreActionBuilder_ == null) {
if (!(actionCase_ == 9)) {
action_ = com.google.cloud.retail.v2alpha.Rule.IgnoreAction.getDefaultInstance();
}
ignoreActionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.IgnoreAction,
com.google.cloud.retail.v2alpha.Rule.IgnoreAction.Builder,
com.google.cloud.retail.v2alpha.Rule.IgnoreActionOrBuilder>(
(com.google.cloud.retail.v2alpha.Rule.IgnoreAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 9;
onChanged();
return ignoreActionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.FilterAction,
com.google.cloud.retail.v2alpha.Rule.FilterAction.Builder,
com.google.cloud.retail.v2alpha.Rule.FilterActionOrBuilder>
filterActionBuilder_;
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*
* @return Whether the filterAction field is set.
*/
@java.lang.Override
public boolean hasFilterAction() {
return actionCase_ == 10;
}
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*
* @return The filterAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.FilterAction getFilterAction() {
if (filterActionBuilder_ == null) {
if (actionCase_ == 10) {
return (com.google.cloud.retail.v2alpha.Rule.FilterAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.FilterAction.getDefaultInstance();
} else {
if (actionCase_ == 10) {
return filterActionBuilder_.getMessage();
}
return com.google.cloud.retail.v2alpha.Rule.FilterAction.getDefaultInstance();
}
}
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*/
public Builder setFilterAction(com.google.cloud.retail.v2alpha.Rule.FilterAction value) {
if (filterActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
filterActionBuilder_.setMessage(value);
}
actionCase_ = 10;
return this;
}
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*/
public Builder setFilterAction(
com.google.cloud.retail.v2alpha.Rule.FilterAction.Builder builderForValue) {
if (filterActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
filterActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 10;
return this;
}
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*/
public Builder mergeFilterAction(com.google.cloud.retail.v2alpha.Rule.FilterAction value) {
if (filterActionBuilder_ == null) {
if (actionCase_ == 10
&& action_ != com.google.cloud.retail.v2alpha.Rule.FilterAction.getDefaultInstance()) {
action_ =
com.google.cloud.retail.v2alpha.Rule.FilterAction.newBuilder(
(com.google.cloud.retail.v2alpha.Rule.FilterAction) action_)
.mergeFrom(value)
.buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 10) {
filterActionBuilder_.mergeFrom(value);
} else {
filterActionBuilder_.setMessage(value);
}
}
actionCase_ = 10;
return this;
}
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*/
public Builder clearFilterAction() {
if (filterActionBuilder_ == null) {
if (actionCase_ == 10) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 10) {
actionCase_ = 0;
action_ = null;
}
filterActionBuilder_.clear();
}
return this;
}
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*/
public com.google.cloud.retail.v2alpha.Rule.FilterAction.Builder getFilterActionBuilder() {
return getFilterActionFieldBuilder().getBuilder();
}
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.FilterActionOrBuilder getFilterActionOrBuilder() {
if ((actionCase_ == 10) && (filterActionBuilder_ != null)) {
return filterActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 10) {
return (com.google.cloud.retail.v2alpha.Rule.FilterAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.FilterAction.getDefaultInstance();
}
}
/**
*
*
*
* Filters results.
*
*
* .google.cloud.retail.v2alpha.Rule.FilterAction filter_action = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.FilterAction,
com.google.cloud.retail.v2alpha.Rule.FilterAction.Builder,
com.google.cloud.retail.v2alpha.Rule.FilterActionOrBuilder>
getFilterActionFieldBuilder() {
if (filterActionBuilder_ == null) {
if (!(actionCase_ == 10)) {
action_ = com.google.cloud.retail.v2alpha.Rule.FilterAction.getDefaultInstance();
}
filterActionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.FilterAction,
com.google.cloud.retail.v2alpha.Rule.FilterAction.Builder,
com.google.cloud.retail.v2alpha.Rule.FilterActionOrBuilder>(
(com.google.cloud.retail.v2alpha.Rule.FilterAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 10;
onChanged();
return filterActionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction,
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.Builder,
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsActionOrBuilder>
twowaySynonymsActionBuilder_;
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*
* @return Whether the twowaySynonymsAction field is set.
*/
@java.lang.Override
public boolean hasTwowaySynonymsAction() {
return actionCase_ == 11;
}
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*
* @return The twowaySynonymsAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction getTwowaySynonymsAction() {
if (twowaySynonymsActionBuilder_ == null) {
if (actionCase_ == 11) {
return (com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.getDefaultInstance();
} else {
if (actionCase_ == 11) {
return twowaySynonymsActionBuilder_.getMessage();
}
return com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.getDefaultInstance();
}
}
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*/
public Builder setTwowaySynonymsAction(
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction value) {
if (twowaySynonymsActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
twowaySynonymsActionBuilder_.setMessage(value);
}
actionCase_ = 11;
return this;
}
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*/
public Builder setTwowaySynonymsAction(
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.Builder builderForValue) {
if (twowaySynonymsActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
twowaySynonymsActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 11;
return this;
}
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*/
public Builder mergeTwowaySynonymsAction(
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction value) {
if (twowaySynonymsActionBuilder_ == null) {
if (actionCase_ == 11
&& action_
!= com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.getDefaultInstance()) {
action_ =
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.newBuilder(
(com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction) action_)
.mergeFrom(value)
.buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 11) {
twowaySynonymsActionBuilder_.mergeFrom(value);
} else {
twowaySynonymsActionBuilder_.setMessage(value);
}
}
actionCase_ = 11;
return this;
}
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*/
public Builder clearTwowaySynonymsAction() {
if (twowaySynonymsActionBuilder_ == null) {
if (actionCase_ == 11) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 11) {
actionCase_ = 0;
action_ = null;
}
twowaySynonymsActionBuilder_.clear();
}
return this;
}
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*/
public com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.Builder
getTwowaySynonymsActionBuilder() {
return getTwowaySynonymsActionFieldBuilder().getBuilder();
}
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsActionOrBuilder
getTwowaySynonymsActionOrBuilder() {
if ((actionCase_ == 11) && (twowaySynonymsActionBuilder_ != null)) {
return twowaySynonymsActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 11) {
return (com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.getDefaultInstance();
}
}
/**
*
*
*
* Treats a set of terms as synonyms of one another.
*
*
* .google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction twoway_synonyms_action = 11;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction,
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.Builder,
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsActionOrBuilder>
getTwowaySynonymsActionFieldBuilder() {
if (twowaySynonymsActionBuilder_ == null) {
if (!(actionCase_ == 11)) {
action_ = com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.getDefaultInstance();
}
twowaySynonymsActionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction,
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction.Builder,
com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsActionOrBuilder>(
(com.google.cloud.retail.v2alpha.Rule.TwowaySynonymsAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 11;
onChanged();
return twowaySynonymsActionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.Builder,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetActionOrBuilder>
forceReturnFacetActionBuilder_;
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*
* @return Whether the forceReturnFacetAction field is set.
*/
@java.lang.Override
public boolean hasForceReturnFacetAction() {
return actionCase_ == 12;
}
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*
* @return The forceReturnFacetAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction getForceReturnFacetAction() {
if (forceReturnFacetActionBuilder_ == null) {
if (actionCase_ == 12) {
return (com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.getDefaultInstance();
} else {
if (actionCase_ == 12) {
return forceReturnFacetActionBuilder_.getMessage();
}
return com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.getDefaultInstance();
}
}
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*/
public Builder setForceReturnFacetAction(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction value) {
if (forceReturnFacetActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
forceReturnFacetActionBuilder_.setMessage(value);
}
actionCase_ = 12;
return this;
}
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*/
public Builder setForceReturnFacetAction(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.Builder builderForValue) {
if (forceReturnFacetActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
forceReturnFacetActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 12;
return this;
}
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*/
public Builder mergeForceReturnFacetAction(
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction value) {
if (forceReturnFacetActionBuilder_ == null) {
if (actionCase_ == 12
&& action_
!= com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction
.getDefaultInstance()) {
action_ =
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.newBuilder(
(com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction) action_)
.mergeFrom(value)
.buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 12) {
forceReturnFacetActionBuilder_.mergeFrom(value);
} else {
forceReturnFacetActionBuilder_.setMessage(value);
}
}
actionCase_ = 12;
return this;
}
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*/
public Builder clearForceReturnFacetAction() {
if (forceReturnFacetActionBuilder_ == null) {
if (actionCase_ == 12) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 12) {
actionCase_ = 0;
action_ = null;
}
forceReturnFacetActionBuilder_.clear();
}
return this;
}
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*/
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.Builder
getForceReturnFacetActionBuilder() {
return getForceReturnFacetActionFieldBuilder().getBuilder();
}
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetActionOrBuilder
getForceReturnFacetActionOrBuilder() {
if ((actionCase_ == 12) && (forceReturnFacetActionBuilder_ != null)) {
return forceReturnFacetActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 12) {
return (com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.getDefaultInstance();
}
}
/**
*
*
*
* Force returns an attribute as a facet in the request.
*
*
*
* .google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction force_return_facet_action = 12;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.Builder,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetActionOrBuilder>
getForceReturnFacetActionFieldBuilder() {
if (forceReturnFacetActionBuilder_ == null) {
if (!(actionCase_ == 12)) {
action_ =
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.getDefaultInstance();
}
forceReturnFacetActionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction.Builder,
com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetActionOrBuilder>(
(com.google.cloud.retail.v2alpha.Rule.ForceReturnFacetAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 12;
onChanged();
return forceReturnFacetActionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction,
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.Builder,
com.google.cloud.retail.v2alpha.Rule.RemoveFacetActionOrBuilder>
removeFacetActionBuilder_;
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*
* @return Whether the removeFacetAction field is set.
*/
@java.lang.Override
public boolean hasRemoveFacetAction() {
return actionCase_ == 13;
}
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*
* @return The removeFacetAction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction getRemoveFacetAction() {
if (removeFacetActionBuilder_ == null) {
if (actionCase_ == 13) {
return (com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.getDefaultInstance();
} else {
if (actionCase_ == 13) {
return removeFacetActionBuilder_.getMessage();
}
return com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.getDefaultInstance();
}
}
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*/
public Builder setRemoveFacetAction(
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction value) {
if (removeFacetActionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
action_ = value;
onChanged();
} else {
removeFacetActionBuilder_.setMessage(value);
}
actionCase_ = 13;
return this;
}
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*/
public Builder setRemoveFacetAction(
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.Builder builderForValue) {
if (removeFacetActionBuilder_ == null) {
action_ = builderForValue.build();
onChanged();
} else {
removeFacetActionBuilder_.setMessage(builderForValue.build());
}
actionCase_ = 13;
return this;
}
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*/
public Builder mergeRemoveFacetAction(
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction value) {
if (removeFacetActionBuilder_ == null) {
if (actionCase_ == 13
&& action_
!= com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.getDefaultInstance()) {
action_ =
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.newBuilder(
(com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction) action_)
.mergeFrom(value)
.buildPartial();
} else {
action_ = value;
}
onChanged();
} else {
if (actionCase_ == 13) {
removeFacetActionBuilder_.mergeFrom(value);
} else {
removeFacetActionBuilder_.setMessage(value);
}
}
actionCase_ = 13;
return this;
}
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*/
public Builder clearRemoveFacetAction() {
if (removeFacetActionBuilder_ == null) {
if (actionCase_ == 13) {
actionCase_ = 0;
action_ = null;
onChanged();
}
} else {
if (actionCase_ == 13) {
actionCase_ = 0;
action_ = null;
}
removeFacetActionBuilder_.clear();
}
return this;
}
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*/
public com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.Builder
getRemoveFacetActionBuilder() {
return getRemoveFacetActionFieldBuilder().getBuilder();
}
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule.RemoveFacetActionOrBuilder
getRemoveFacetActionOrBuilder() {
if ((actionCase_ == 13) && (removeFacetActionBuilder_ != null)) {
return removeFacetActionBuilder_.getMessageOrBuilder();
} else {
if (actionCase_ == 13) {
return (com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction) action_;
}
return com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.getDefaultInstance();
}
}
/**
*
*
*
* Remove an attribute as a facet in the request (if present).
*
*
* .google.cloud.retail.v2alpha.Rule.RemoveFacetAction remove_facet_action = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction,
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.Builder,
com.google.cloud.retail.v2alpha.Rule.RemoveFacetActionOrBuilder>
getRemoveFacetActionFieldBuilder() {
if (removeFacetActionBuilder_ == null) {
if (!(actionCase_ == 13)) {
action_ = com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.getDefaultInstance();
}
removeFacetActionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction,
com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction.Builder,
com.google.cloud.retail.v2alpha.Rule.RemoveFacetActionOrBuilder>(
(com.google.cloud.retail.v2alpha.Rule.RemoveFacetAction) action_,
getParentForChildren(),
isClean());
action_ = null;
}
actionCase_ = 13;
onChanged();
return removeFacetActionBuilder_;
}
private com.google.cloud.retail.v2alpha.Condition condition_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Condition,
com.google.cloud.retail.v2alpha.Condition.Builder,
com.google.cloud.retail.v2alpha.ConditionOrBuilder>
conditionBuilder_;
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return Whether the condition field is set.
*/
public boolean hasCondition() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*
* @return The condition.
*/
public com.google.cloud.retail.v2alpha.Condition getCondition() {
if (conditionBuilder_ == null) {
return condition_ == null
? com.google.cloud.retail.v2alpha.Condition.getDefaultInstance()
: condition_;
} else {
return conditionBuilder_.getMessage();
}
}
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setCondition(com.google.cloud.retail.v2alpha.Condition value) {
if (conditionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
condition_ = value;
} else {
conditionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder setCondition(com.google.cloud.retail.v2alpha.Condition.Builder builderForValue) {
if (conditionBuilder_ == null) {
condition_ = builderForValue.build();
} else {
conditionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder mergeCondition(com.google.cloud.retail.v2alpha.Condition value) {
if (conditionBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0)
&& condition_ != null
&& condition_ != com.google.cloud.retail.v2alpha.Condition.getDefaultInstance()) {
getConditionBuilder().mergeFrom(value);
} else {
condition_ = value;
}
} else {
conditionBuilder_.mergeFrom(value);
}
if (condition_ != null) {
bitField0_ |= 0x00000400;
onChanged();
}
return this;
}
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public Builder clearCondition() {
bitField0_ = (bitField0_ & ~0x00000400);
condition_ = null;
if (conditionBuilder_ != null) {
conditionBuilder_.dispose();
conditionBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.cloud.retail.v2alpha.Condition.Builder getConditionBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getConditionFieldBuilder().getBuilder();
}
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
public com.google.cloud.retail.v2alpha.ConditionOrBuilder getConditionOrBuilder() {
if (conditionBuilder_ != null) {
return conditionBuilder_.getMessageOrBuilder();
} else {
return condition_ == null
? com.google.cloud.retail.v2alpha.Condition.getDefaultInstance()
: condition_;
}
}
/**
*
*
*
* Required. The condition that triggers the rule.
* If the condition is empty, the rule will always apply.
*
*
*
* .google.cloud.retail.v2alpha.Condition condition = 1 [(.google.api.field_behavior) = REQUIRED];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Condition,
com.google.cloud.retail.v2alpha.Condition.Builder,
com.google.cloud.retail.v2alpha.ConditionOrBuilder>
getConditionFieldBuilder() {
if (conditionBuilder_ == null) {
conditionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.Condition,
com.google.cloud.retail.v2alpha.Condition.Builder,
com.google.cloud.retail.v2alpha.ConditionOrBuilder>(
getCondition(), getParentForChildren(), isClean());
condition_ = null;
}
return conditionBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.Rule)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.Rule)
private static final com.google.cloud.retail.v2alpha.Rule DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.Rule();
}
public static com.google.cloud.retail.v2alpha.Rule getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Rule parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.Rule getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy