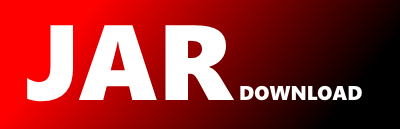
com.google.cloud.retail.v2alpha.SearchRequestOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Proto library for google-cloud-retail
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/retail/v2alpha/search_service.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.retail.v2alpha;
public interface SearchRequestOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.cloud.retail.v2alpha.SearchRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Required. The resource name of the Retail Search serving config, such as
* `projects/*/locations/global/catalogs/default_catalog/servingConfigs/default_serving_config`
* or the name of the legacy placement resource, such as
* `projects/*/locations/global/catalogs/default_catalog/placements/default_search`.
* This field is used to identify the serving config name and the set
* of models that are used to make the search.
*
*
* string placement = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The placement.
*/
java.lang.String getPlacement();
/**
*
*
*
* Required. The resource name of the Retail Search serving config, such as
* `projects/*/locations/global/catalogs/default_catalog/servingConfigs/default_serving_config`
* or the name of the legacy placement resource, such as
* `projects/*/locations/global/catalogs/default_catalog/placements/default_search`.
* This field is used to identify the serving config name and the set
* of models that are used to make the search.
*
*
* string placement = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for placement.
*/
com.google.protobuf.ByteString getPlacementBytes();
/**
*
*
*
* The branch resource name, such as
* `projects/*/locations/global/catalogs/default_catalog/branches/0`.
*
* Use "default_branch" as the branch ID or leave this field empty, to search
* products under the default branch.
*
*
* string branch = 2 [(.google.api.resource_reference) = { ... }
*
* @return The branch.
*/
java.lang.String getBranch();
/**
*
*
*
* The branch resource name, such as
* `projects/*/locations/global/catalogs/default_catalog/branches/0`.
*
* Use "default_branch" as the branch ID or leave this field empty, to search
* products under the default branch.
*
*
* string branch = 2 [(.google.api.resource_reference) = { ... }
*
* @return The bytes for branch.
*/
com.google.protobuf.ByteString getBranchBytes();
/**
*
*
*
* Raw search query.
*
* If this field is empty, the request is considered a category browsing
* request and returned results are based on
* [filter][google.cloud.retail.v2alpha.SearchRequest.filter] and
* [page_categories][google.cloud.retail.v2alpha.SearchRequest.page_categories].
*
*
* string query = 3;
*
* @return The query.
*/
java.lang.String getQuery();
/**
*
*
*
* Raw search query.
*
* If this field is empty, the request is considered a category browsing
* request and returned results are based on
* [filter][google.cloud.retail.v2alpha.SearchRequest.filter] and
* [page_categories][google.cloud.retail.v2alpha.SearchRequest.page_categories].
*
*
* string query = 3;
*
* @return The bytes for query.
*/
com.google.protobuf.ByteString getQueryBytes();
/**
*
*
*
* Required. A unique identifier for tracking visitors. For example, this
* could be implemented with an HTTP cookie, which should be able to uniquely
* identify a visitor on a single device. This unique identifier should not
* change if the visitor logs in or out of the website.
*
* This should be the same identifier as
* [UserEvent.visitor_id][google.cloud.retail.v2alpha.UserEvent.visitor_id].
*
* The field must be a UTF-8 encoded string with a length limit of 128
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string visitor_id = 4 [(.google.api.field_behavior) = REQUIRED];
*
* @return The visitorId.
*/
java.lang.String getVisitorId();
/**
*
*
*
* Required. A unique identifier for tracking visitors. For example, this
* could be implemented with an HTTP cookie, which should be able to uniquely
* identify a visitor on a single device. This unique identifier should not
* change if the visitor logs in or out of the website.
*
* This should be the same identifier as
* [UserEvent.visitor_id][google.cloud.retail.v2alpha.UserEvent.visitor_id].
*
* The field must be a UTF-8 encoded string with a length limit of 128
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string visitor_id = 4 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for visitorId.
*/
com.google.protobuf.ByteString getVisitorIdBytes();
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 5;
*
* @return Whether the userInfo field is set.
*/
boolean hasUserInfo();
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 5;
*
* @return The userInfo.
*/
com.google.cloud.retail.v2alpha.UserInfo getUserInfo();
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 5;
*/
com.google.cloud.retail.v2alpha.UserInfoOrBuilder getUserInfoOrBuilder();
/**
*
*
*
* Maximum number of [Product][google.cloud.retail.v2alpha.Product]s to
* return. If unspecified, defaults to a reasonable value. The maximum allowed
* value is 120. Values above 120 will be coerced to 120.
*
* If this field is negative, an INVALID_ARGUMENT is returned.
*
*
* int32 page_size = 7;
*
* @return The pageSize.
*/
int getPageSize();
/**
*
*
*
* A page token
* [SearchResponse.next_page_token][google.cloud.retail.v2alpha.SearchResponse.next_page_token],
* received from a previous
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search]
* call. Provide this to retrieve the subsequent page.
*
* When paginating, all other parameters provided to
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search]
* must match the call that provided the page token. Otherwise, an
* INVALID_ARGUMENT error is returned.
*
*
* string page_token = 8;
*
* @return The pageToken.
*/
java.lang.String getPageToken();
/**
*
*
*
* A page token
* [SearchResponse.next_page_token][google.cloud.retail.v2alpha.SearchResponse.next_page_token],
* received from a previous
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search]
* call. Provide this to retrieve the subsequent page.
*
* When paginating, all other parameters provided to
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search]
* must match the call that provided the page token. Otherwise, an
* INVALID_ARGUMENT error is returned.
*
*
* string page_token = 8;
*
* @return The bytes for pageToken.
*/
com.google.protobuf.ByteString getPageTokenBytes();
/**
*
*
*
* A 0-indexed integer that specifies the current offset (that is, starting
* result location, amongst the
* [Product][google.cloud.retail.v2alpha.Product]s deemed by the API as
* relevant) in search results. This field is only considered if
* [page_token][google.cloud.retail.v2alpha.SearchRequest.page_token] is
* unset.
*
* If this field is negative, an INVALID_ARGUMENT is returned.
*
*
* int32 offset = 9;
*
* @return The offset.
*/
int getOffset();
/**
*
*
*
* The filter syntax consists of an expression language for constructing a
* predicate from one or more fields of the products being filtered. Filter
* expression is case-sensitive. For more information, see
* [Filter](https://cloud.google.com/retail/docs/filter-and-order#filter).
*
* If this field is unrecognizable, an INVALID_ARGUMENT is returned.
*
*
* string filter = 10;
*
* @return The filter.
*/
java.lang.String getFilter();
/**
*
*
*
* The filter syntax consists of an expression language for constructing a
* predicate from one or more fields of the products being filtered. Filter
* expression is case-sensitive. For more information, see
* [Filter](https://cloud.google.com/retail/docs/filter-and-order#filter).
*
* If this field is unrecognizable, an INVALID_ARGUMENT is returned.
*
*
* string filter = 10;
*
* @return The bytes for filter.
*/
com.google.protobuf.ByteString getFilterBytes();
/**
*
*
*
* The default filter that is applied when a user performs a search without
* checking any filters on the search page.
*
* The filter applied to every search request when quality improvement such as
* query expansion is needed. In the case a query does not have a sufficient
* amount of results this filter will be used to determine whether or not to
* enable the query expansion flow. The original filter will still be used for
* the query expanded search.
* This field is strongly recommended to achieve high search quality.
*
* For more information about filter syntax, see
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter].
*
*
* string canonical_filter = 28;
*
* @return The canonicalFilter.
*/
java.lang.String getCanonicalFilter();
/**
*
*
*
* The default filter that is applied when a user performs a search without
* checking any filters on the search page.
*
* The filter applied to every search request when quality improvement such as
* query expansion is needed. In the case a query does not have a sufficient
* amount of results this filter will be used to determine whether or not to
* enable the query expansion flow. The original filter will still be used for
* the query expanded search.
* This field is strongly recommended to achieve high search quality.
*
* For more information about filter syntax, see
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter].
*
*
* string canonical_filter = 28;
*
* @return The bytes for canonicalFilter.
*/
com.google.protobuf.ByteString getCanonicalFilterBytes();
/**
*
*
*
* The order in which products are returned. Products can be ordered by
* a field in an [Product][google.cloud.retail.v2alpha.Product] object. Leave
* it unset if ordered by relevance. OrderBy expression is case-sensitive. For
* more information, see
* [Order](https://cloud.google.com/retail/docs/filter-and-order#order).
*
* If this field is unrecognizable, an INVALID_ARGUMENT is returned.
*
*
* string order_by = 11;
*
* @return The orderBy.
*/
java.lang.String getOrderBy();
/**
*
*
*
* The order in which products are returned. Products can be ordered by
* a field in an [Product][google.cloud.retail.v2alpha.Product] object. Leave
* it unset if ordered by relevance. OrderBy expression is case-sensitive. For
* more information, see
* [Order](https://cloud.google.com/retail/docs/filter-and-order#order).
*
* If this field is unrecognizable, an INVALID_ARGUMENT is returned.
*
*
* string order_by = 11;
*
* @return The bytes for orderBy.
*/
com.google.protobuf.ByteString getOrderByBytes();
/**
*
*
*
* Facet specifications for faceted search. If empty, no facets are returned.
*
* A maximum of 200 values are allowed. Otherwise, an INVALID_ARGUMENT error
* is returned.
*
*
* repeated .google.cloud.retail.v2alpha.SearchRequest.FacetSpec facet_specs = 12;
*/
java.util.List getFacetSpecsList();
/**
*
*
*
* Facet specifications for faceted search. If empty, no facets are returned.
*
* A maximum of 200 values are allowed. Otherwise, an INVALID_ARGUMENT error
* is returned.
*
*
* repeated .google.cloud.retail.v2alpha.SearchRequest.FacetSpec facet_specs = 12;
*/
com.google.cloud.retail.v2alpha.SearchRequest.FacetSpec getFacetSpecs(int index);
/**
*
*
*
* Facet specifications for faceted search. If empty, no facets are returned.
*
* A maximum of 200 values are allowed. Otherwise, an INVALID_ARGUMENT error
* is returned.
*
*
* repeated .google.cloud.retail.v2alpha.SearchRequest.FacetSpec facet_specs = 12;
*/
int getFacetSpecsCount();
/**
*
*
*
* Facet specifications for faceted search. If empty, no facets are returned.
*
* A maximum of 200 values are allowed. Otherwise, an INVALID_ARGUMENT error
* is returned.
*
*
* repeated .google.cloud.retail.v2alpha.SearchRequest.FacetSpec facet_specs = 12;
*/
java.util.List extends com.google.cloud.retail.v2alpha.SearchRequest.FacetSpecOrBuilder>
getFacetSpecsOrBuilderList();
/**
*
*
*
* Facet specifications for faceted search. If empty, no facets are returned.
*
* A maximum of 200 values are allowed. Otherwise, an INVALID_ARGUMENT error
* is returned.
*
*
* repeated .google.cloud.retail.v2alpha.SearchRequest.FacetSpec facet_specs = 12;
*/
com.google.cloud.retail.v2alpha.SearchRequest.FacetSpecOrBuilder getFacetSpecsOrBuilder(
int index);
/**
*
*
*
* Deprecated. Refer to https://cloud.google.com/retail/docs/configs#dynamic
* to enable dynamic facets. Do not set this field.
*
* The specification for dynamically generated facets. Notice that only
* textual facets can be dynamically generated.
*
*
*
* .google.cloud.retail.v2alpha.SearchRequest.DynamicFacetSpec dynamic_facet_spec = 21 [deprecated = true];
*
*
* @deprecated google.cloud.retail.v2alpha.SearchRequest.dynamic_facet_spec is deprecated. See
* google/cloud/retail/v2alpha/search_service.proto;l=721
* @return Whether the dynamicFacetSpec field is set.
*/
@java.lang.Deprecated
boolean hasDynamicFacetSpec();
/**
*
*
*
* Deprecated. Refer to https://cloud.google.com/retail/docs/configs#dynamic
* to enable dynamic facets. Do not set this field.
*
* The specification for dynamically generated facets. Notice that only
* textual facets can be dynamically generated.
*
*
*
* .google.cloud.retail.v2alpha.SearchRequest.DynamicFacetSpec dynamic_facet_spec = 21 [deprecated = true];
*
*
* @deprecated google.cloud.retail.v2alpha.SearchRequest.dynamic_facet_spec is deprecated. See
* google/cloud/retail/v2alpha/search_service.proto;l=721
* @return The dynamicFacetSpec.
*/
@java.lang.Deprecated
com.google.cloud.retail.v2alpha.SearchRequest.DynamicFacetSpec getDynamicFacetSpec();
/**
*
*
*
* Deprecated. Refer to https://cloud.google.com/retail/docs/configs#dynamic
* to enable dynamic facets. Do not set this field.
*
* The specification for dynamically generated facets. Notice that only
* textual facets can be dynamically generated.
*
*
*
* .google.cloud.retail.v2alpha.SearchRequest.DynamicFacetSpec dynamic_facet_spec = 21 [deprecated = true];
*
*/
@java.lang.Deprecated
com.google.cloud.retail.v2alpha.SearchRequest.DynamicFacetSpecOrBuilder
getDynamicFacetSpecOrBuilder();
/**
*
*
*
* Boost specification to boost certain products. For more information, see
* [Boost results](https://cloud.google.com/retail/docs/boosting).
*
* Notice that if both
* [ServingConfig.boost_control_ids][google.cloud.retail.v2alpha.ServingConfig.boost_control_ids]
* and
* [SearchRequest.boost_spec][google.cloud.retail.v2alpha.SearchRequest.boost_spec]
* are set, the boost conditions from both places are evaluated. If a search
* request matches multiple boost conditions, the final boost score is equal
* to the sum of the boost scores from all matched boost conditions.
*
*
* .google.cloud.retail.v2alpha.SearchRequest.BoostSpec boost_spec = 13;
*
* @return Whether the boostSpec field is set.
*/
boolean hasBoostSpec();
/**
*
*
*
* Boost specification to boost certain products. For more information, see
* [Boost results](https://cloud.google.com/retail/docs/boosting).
*
* Notice that if both
* [ServingConfig.boost_control_ids][google.cloud.retail.v2alpha.ServingConfig.boost_control_ids]
* and
* [SearchRequest.boost_spec][google.cloud.retail.v2alpha.SearchRequest.boost_spec]
* are set, the boost conditions from both places are evaluated. If a search
* request matches multiple boost conditions, the final boost score is equal
* to the sum of the boost scores from all matched boost conditions.
*
*
* .google.cloud.retail.v2alpha.SearchRequest.BoostSpec boost_spec = 13;
*
* @return The boostSpec.
*/
com.google.cloud.retail.v2alpha.SearchRequest.BoostSpec getBoostSpec();
/**
*
*
*
* Boost specification to boost certain products. For more information, see
* [Boost results](https://cloud.google.com/retail/docs/boosting).
*
* Notice that if both
* [ServingConfig.boost_control_ids][google.cloud.retail.v2alpha.ServingConfig.boost_control_ids]
* and
* [SearchRequest.boost_spec][google.cloud.retail.v2alpha.SearchRequest.boost_spec]
* are set, the boost conditions from both places are evaluated. If a search
* request matches multiple boost conditions, the final boost score is equal
* to the sum of the boost scores from all matched boost conditions.
*
*
* .google.cloud.retail.v2alpha.SearchRequest.BoostSpec boost_spec = 13;
*/
com.google.cloud.retail.v2alpha.SearchRequest.BoostSpecOrBuilder getBoostSpecOrBuilder();
/**
*
*
*
* The query expansion specification that specifies the conditions under which
* query expansion occurs. For more information, see [Query
* expansion](https://cloud.google.com/retail/docs/result-size#query_expansion).
*
*
* .google.cloud.retail.v2alpha.SearchRequest.QueryExpansionSpec query_expansion_spec = 14;
*
*
* @return Whether the queryExpansionSpec field is set.
*/
boolean hasQueryExpansionSpec();
/**
*
*
*
* The query expansion specification that specifies the conditions under which
* query expansion occurs. For more information, see [Query
* expansion](https://cloud.google.com/retail/docs/result-size#query_expansion).
*
*
* .google.cloud.retail.v2alpha.SearchRequest.QueryExpansionSpec query_expansion_spec = 14;
*
*
* @return The queryExpansionSpec.
*/
com.google.cloud.retail.v2alpha.SearchRequest.QueryExpansionSpec getQueryExpansionSpec();
/**
*
*
*
* The query expansion specification that specifies the conditions under which
* query expansion occurs. For more information, see [Query
* expansion](https://cloud.google.com/retail/docs/result-size#query_expansion).
*
*
* .google.cloud.retail.v2alpha.SearchRequest.QueryExpansionSpec query_expansion_spec = 14;
*
*/
com.google.cloud.retail.v2alpha.SearchRequest.QueryExpansionSpecOrBuilder
getQueryExpansionSpecOrBuilder();
/**
*
*
*
* The relevance threshold of the search results.
*
* Defaults to
* [RelevanceThreshold.HIGH][google.cloud.retail.v2alpha.SearchRequest.RelevanceThreshold.HIGH],
* which means only the most relevant results are shown, and the least number
* of results are returned. For more information, see [Adjust result
* size](https://cloud.google.com/retail/docs/result-size#relevance_thresholding).
*
*
* .google.cloud.retail.v2alpha.SearchRequest.RelevanceThreshold relevance_threshold = 15;
*
*
* @return The enum numeric value on the wire for relevanceThreshold.
*/
int getRelevanceThresholdValue();
/**
*
*
*
* The relevance threshold of the search results.
*
* Defaults to
* [RelevanceThreshold.HIGH][google.cloud.retail.v2alpha.SearchRequest.RelevanceThreshold.HIGH],
* which means only the most relevant results are shown, and the least number
* of results are returned. For more information, see [Adjust result
* size](https://cloud.google.com/retail/docs/result-size#relevance_thresholding).
*
*
* .google.cloud.retail.v2alpha.SearchRequest.RelevanceThreshold relevance_threshold = 15;
*
*
* @return The relevanceThreshold.
*/
com.google.cloud.retail.v2alpha.SearchRequest.RelevanceThreshold getRelevanceThreshold();
/**
*
*
*
* The keys to fetch and rollup the matching
* [variant][google.cloud.retail.v2alpha.Product.Type.VARIANT]
* [Product][google.cloud.retail.v2alpha.Product]s attributes,
* [FulfillmentInfo][google.cloud.retail.v2alpha.FulfillmentInfo] or
* [LocalInventory][google.cloud.retail.v2alpha.LocalInventory]s attributes.
* The attributes from all the matching
* [variant][google.cloud.retail.v2alpha.Product.Type.VARIANT]
* [Product][google.cloud.retail.v2alpha.Product]s or
* [LocalInventory][google.cloud.retail.v2alpha.LocalInventory]s are merged
* and de-duplicated. Notice that rollup attributes will lead to extra query
* latency. Maximum number of keys is 30.
*
* For [FulfillmentInfo][google.cloud.retail.v2alpha.FulfillmentInfo], a
* fulfillment type and a fulfillment ID must be provided in the format of
* "fulfillmentType.fulfillmentId". E.g., in "pickupInStore.store123",
* "pickupInStore" is fulfillment type and "store123" is the store ID.
*
* Supported keys are:
*
* * colorFamilies
* * price
* * originalPrice
* * discount
* * variantId
* * inventory(place_id,price)
* * inventory(place_id,original_price)
* * inventory(place_id,attributes.key), where key is any key in the
* [Product.local_inventories.attributes][google.cloud.retail.v2alpha.LocalInventory.attributes]
* map.
* * attributes.key, where key is any key in the
* [Product.attributes][google.cloud.retail.v2alpha.Product.attributes] map.
* * pickupInStore.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "pickup-in-store".
* * shipToStore.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "ship-to-store".
* * sameDayDelivery.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "same-day-delivery".
* * nextDayDelivery.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "next-day-delivery".
* * customFulfillment1.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-1".
* * customFulfillment2.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-2".
* * customFulfillment3.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-3".
* * customFulfillment4.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-4".
* * customFulfillment5.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-5".
*
* If this field is set to an invalid value other than these, an
* INVALID_ARGUMENT error is returned.
*
*
* repeated string variant_rollup_keys = 17;
*
* @return A list containing the variantRollupKeys.
*/
java.util.List getVariantRollupKeysList();
/**
*
*
*
* The keys to fetch and rollup the matching
* [variant][google.cloud.retail.v2alpha.Product.Type.VARIANT]
* [Product][google.cloud.retail.v2alpha.Product]s attributes,
* [FulfillmentInfo][google.cloud.retail.v2alpha.FulfillmentInfo] or
* [LocalInventory][google.cloud.retail.v2alpha.LocalInventory]s attributes.
* The attributes from all the matching
* [variant][google.cloud.retail.v2alpha.Product.Type.VARIANT]
* [Product][google.cloud.retail.v2alpha.Product]s or
* [LocalInventory][google.cloud.retail.v2alpha.LocalInventory]s are merged
* and de-duplicated. Notice that rollup attributes will lead to extra query
* latency. Maximum number of keys is 30.
*
* For [FulfillmentInfo][google.cloud.retail.v2alpha.FulfillmentInfo], a
* fulfillment type and a fulfillment ID must be provided in the format of
* "fulfillmentType.fulfillmentId". E.g., in "pickupInStore.store123",
* "pickupInStore" is fulfillment type and "store123" is the store ID.
*
* Supported keys are:
*
* * colorFamilies
* * price
* * originalPrice
* * discount
* * variantId
* * inventory(place_id,price)
* * inventory(place_id,original_price)
* * inventory(place_id,attributes.key), where key is any key in the
* [Product.local_inventories.attributes][google.cloud.retail.v2alpha.LocalInventory.attributes]
* map.
* * attributes.key, where key is any key in the
* [Product.attributes][google.cloud.retail.v2alpha.Product.attributes] map.
* * pickupInStore.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "pickup-in-store".
* * shipToStore.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "ship-to-store".
* * sameDayDelivery.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "same-day-delivery".
* * nextDayDelivery.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "next-day-delivery".
* * customFulfillment1.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-1".
* * customFulfillment2.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-2".
* * customFulfillment3.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-3".
* * customFulfillment4.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-4".
* * customFulfillment5.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-5".
*
* If this field is set to an invalid value other than these, an
* INVALID_ARGUMENT error is returned.
*
*
* repeated string variant_rollup_keys = 17;
*
* @return The count of variantRollupKeys.
*/
int getVariantRollupKeysCount();
/**
*
*
*
* The keys to fetch and rollup the matching
* [variant][google.cloud.retail.v2alpha.Product.Type.VARIANT]
* [Product][google.cloud.retail.v2alpha.Product]s attributes,
* [FulfillmentInfo][google.cloud.retail.v2alpha.FulfillmentInfo] or
* [LocalInventory][google.cloud.retail.v2alpha.LocalInventory]s attributes.
* The attributes from all the matching
* [variant][google.cloud.retail.v2alpha.Product.Type.VARIANT]
* [Product][google.cloud.retail.v2alpha.Product]s or
* [LocalInventory][google.cloud.retail.v2alpha.LocalInventory]s are merged
* and de-duplicated. Notice that rollup attributes will lead to extra query
* latency. Maximum number of keys is 30.
*
* For [FulfillmentInfo][google.cloud.retail.v2alpha.FulfillmentInfo], a
* fulfillment type and a fulfillment ID must be provided in the format of
* "fulfillmentType.fulfillmentId". E.g., in "pickupInStore.store123",
* "pickupInStore" is fulfillment type and "store123" is the store ID.
*
* Supported keys are:
*
* * colorFamilies
* * price
* * originalPrice
* * discount
* * variantId
* * inventory(place_id,price)
* * inventory(place_id,original_price)
* * inventory(place_id,attributes.key), where key is any key in the
* [Product.local_inventories.attributes][google.cloud.retail.v2alpha.LocalInventory.attributes]
* map.
* * attributes.key, where key is any key in the
* [Product.attributes][google.cloud.retail.v2alpha.Product.attributes] map.
* * pickupInStore.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "pickup-in-store".
* * shipToStore.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "ship-to-store".
* * sameDayDelivery.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "same-day-delivery".
* * nextDayDelivery.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "next-day-delivery".
* * customFulfillment1.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-1".
* * customFulfillment2.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-2".
* * customFulfillment3.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-3".
* * customFulfillment4.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-4".
* * customFulfillment5.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-5".
*
* If this field is set to an invalid value other than these, an
* INVALID_ARGUMENT error is returned.
*
*
* repeated string variant_rollup_keys = 17;
*
* @param index The index of the element to return.
* @return The variantRollupKeys at the given index.
*/
java.lang.String getVariantRollupKeys(int index);
/**
*
*
*
* The keys to fetch and rollup the matching
* [variant][google.cloud.retail.v2alpha.Product.Type.VARIANT]
* [Product][google.cloud.retail.v2alpha.Product]s attributes,
* [FulfillmentInfo][google.cloud.retail.v2alpha.FulfillmentInfo] or
* [LocalInventory][google.cloud.retail.v2alpha.LocalInventory]s attributes.
* The attributes from all the matching
* [variant][google.cloud.retail.v2alpha.Product.Type.VARIANT]
* [Product][google.cloud.retail.v2alpha.Product]s or
* [LocalInventory][google.cloud.retail.v2alpha.LocalInventory]s are merged
* and de-duplicated. Notice that rollup attributes will lead to extra query
* latency. Maximum number of keys is 30.
*
* For [FulfillmentInfo][google.cloud.retail.v2alpha.FulfillmentInfo], a
* fulfillment type and a fulfillment ID must be provided in the format of
* "fulfillmentType.fulfillmentId". E.g., in "pickupInStore.store123",
* "pickupInStore" is fulfillment type and "store123" is the store ID.
*
* Supported keys are:
*
* * colorFamilies
* * price
* * originalPrice
* * discount
* * variantId
* * inventory(place_id,price)
* * inventory(place_id,original_price)
* * inventory(place_id,attributes.key), where key is any key in the
* [Product.local_inventories.attributes][google.cloud.retail.v2alpha.LocalInventory.attributes]
* map.
* * attributes.key, where key is any key in the
* [Product.attributes][google.cloud.retail.v2alpha.Product.attributes] map.
* * pickupInStore.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "pickup-in-store".
* * shipToStore.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "ship-to-store".
* * sameDayDelivery.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "same-day-delivery".
* * nextDayDelivery.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "next-day-delivery".
* * customFulfillment1.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-1".
* * customFulfillment2.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-2".
* * customFulfillment3.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-3".
* * customFulfillment4.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-4".
* * customFulfillment5.id, where id is any
* [FulfillmentInfo.place_ids][google.cloud.retail.v2alpha.FulfillmentInfo.place_ids]
* for
* [FulfillmentInfo.type][google.cloud.retail.v2alpha.FulfillmentInfo.type]
* "custom-type-5".
*
* If this field is set to an invalid value other than these, an
* INVALID_ARGUMENT error is returned.
*
*
* repeated string variant_rollup_keys = 17;
*
* @param index The index of the value to return.
* @return The bytes of the variantRollupKeys at the given index.
*/
com.google.protobuf.ByteString getVariantRollupKeysBytes(int index);
/**
*
*
*
* The categories associated with a category page. Must be set for category
* navigation queries to achieve good search quality. The format should be
* the same as
* [UserEvent.page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories];
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
*
* repeated string page_categories = 23;
*
* @return A list containing the pageCategories.
*/
java.util.List getPageCategoriesList();
/**
*
*
*
* The categories associated with a category page. Must be set for category
* navigation queries to achieve good search quality. The format should be
* the same as
* [UserEvent.page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories];
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
*
* repeated string page_categories = 23;
*
* @return The count of pageCategories.
*/
int getPageCategoriesCount();
/**
*
*
*
* The categories associated with a category page. Must be set for category
* navigation queries to achieve good search quality. The format should be
* the same as
* [UserEvent.page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories];
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
*
* repeated string page_categories = 23;
*
* @param index The index of the element to return.
* @return The pageCategories at the given index.
*/
java.lang.String getPageCategories(int index);
/**
*
*
*
* The categories associated with a category page. Must be set for category
* navigation queries to achieve good search quality. The format should be
* the same as
* [UserEvent.page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories];
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
*
* repeated string page_categories = 23;
*
* @param index The index of the value to return.
* @return The bytes of the pageCategories at the given index.
*/
com.google.protobuf.ByteString getPageCategoriesBytes(int index);
/**
*
*
*
* The search mode of the search request. If not specified, a single search
* request triggers both product search and faceted search.
*
*
* .google.cloud.retail.v2alpha.SearchRequest.SearchMode search_mode = 31;
*
* @return The enum numeric value on the wire for searchMode.
*/
int getSearchModeValue();
/**
*
*
*
* The search mode of the search request. If not specified, a single search
* request triggers both product search and faceted search.
*
*
* .google.cloud.retail.v2alpha.SearchRequest.SearchMode search_mode = 31;
*
* @return The searchMode.
*/
com.google.cloud.retail.v2alpha.SearchRequest.SearchMode getSearchMode();
/**
*
*
*
* The specification for personalization.
*
* Notice that if both
* [ServingConfig.personalization_spec][google.cloud.retail.v2alpha.ServingConfig.personalization_spec]
* and
* [SearchRequest.personalization_spec][google.cloud.retail.v2alpha.SearchRequest.personalization_spec]
* are set.
* [SearchRequest.personalization_spec][google.cloud.retail.v2alpha.SearchRequest.personalization_spec]
* will override
* [ServingConfig.personalization_spec][google.cloud.retail.v2alpha.ServingConfig.personalization_spec].
*
*
* .google.cloud.retail.v2alpha.SearchRequest.PersonalizationSpec personalization_spec = 32;
*
*
* @return Whether the personalizationSpec field is set.
*/
boolean hasPersonalizationSpec();
/**
*
*
*
* The specification for personalization.
*
* Notice that if both
* [ServingConfig.personalization_spec][google.cloud.retail.v2alpha.ServingConfig.personalization_spec]
* and
* [SearchRequest.personalization_spec][google.cloud.retail.v2alpha.SearchRequest.personalization_spec]
* are set.
* [SearchRequest.personalization_spec][google.cloud.retail.v2alpha.SearchRequest.personalization_spec]
* will override
* [ServingConfig.personalization_spec][google.cloud.retail.v2alpha.ServingConfig.personalization_spec].
*
*
* .google.cloud.retail.v2alpha.SearchRequest.PersonalizationSpec personalization_spec = 32;
*
*
* @return The personalizationSpec.
*/
com.google.cloud.retail.v2alpha.SearchRequest.PersonalizationSpec getPersonalizationSpec();
/**
*
*
*
* The specification for personalization.
*
* Notice that if both
* [ServingConfig.personalization_spec][google.cloud.retail.v2alpha.ServingConfig.personalization_spec]
* and
* [SearchRequest.personalization_spec][google.cloud.retail.v2alpha.SearchRequest.personalization_spec]
* are set.
* [SearchRequest.personalization_spec][google.cloud.retail.v2alpha.SearchRequest.personalization_spec]
* will override
* [ServingConfig.personalization_spec][google.cloud.retail.v2alpha.ServingConfig.personalization_spec].
*
*
* .google.cloud.retail.v2alpha.SearchRequest.PersonalizationSpec personalization_spec = 32;
*
*/
com.google.cloud.retail.v2alpha.SearchRequest.PersonalizationSpecOrBuilder
getPersonalizationSpecOrBuilder();
/**
*
*
*
* The labels applied to a resource must meet the following requirements:
*
* * Each resource can have multiple labels, up to a maximum of 64.
* * Each label must be a key-value pair.
* * Keys have a minimum length of 1 character and a maximum length of 63
* characters and cannot be empty. Values can be empty and have a maximum
* length of 63 characters.
* * Keys and values can contain only lowercase letters, numeric characters,
* underscores, and dashes. All characters must use UTF-8 encoding, and
* international characters are allowed.
* * The key portion of a label must be unique. However, you can use the same
* key with multiple resources.
* * Keys must start with a lowercase letter or international character.
*
* For more information, see [Requirements for
* labels](https://cloud.google.com/resource-manager/docs/creating-managing-labels#requirements)
* in the Resource Manager documentation.
*
*
* map<string, string> labels = 34;
*/
int getLabelsCount();
/**
*
*
*
* The labels applied to a resource must meet the following requirements:
*
* * Each resource can have multiple labels, up to a maximum of 64.
* * Each label must be a key-value pair.
* * Keys have a minimum length of 1 character and a maximum length of 63
* characters and cannot be empty. Values can be empty and have a maximum
* length of 63 characters.
* * Keys and values can contain only lowercase letters, numeric characters,
* underscores, and dashes. All characters must use UTF-8 encoding, and
* international characters are allowed.
* * The key portion of a label must be unique. However, you can use the same
* key with multiple resources.
* * Keys must start with a lowercase letter or international character.
*
* For more information, see [Requirements for
* labels](https://cloud.google.com/resource-manager/docs/creating-managing-labels#requirements)
* in the Resource Manager documentation.
*
*
* map<string, string> labels = 34;
*/
boolean containsLabels(java.lang.String key);
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Deprecated
java.util.Map getLabels();
/**
*
*
*
* The labels applied to a resource must meet the following requirements:
*
* * Each resource can have multiple labels, up to a maximum of 64.
* * Each label must be a key-value pair.
* * Keys have a minimum length of 1 character and a maximum length of 63
* characters and cannot be empty. Values can be empty and have a maximum
* length of 63 characters.
* * Keys and values can contain only lowercase letters, numeric characters,
* underscores, and dashes. All characters must use UTF-8 encoding, and
* international characters are allowed.
* * The key portion of a label must be unique. However, you can use the same
* key with multiple resources.
* * Keys must start with a lowercase letter or international character.
*
* For more information, see [Requirements for
* labels](https://cloud.google.com/resource-manager/docs/creating-managing-labels#requirements)
* in the Resource Manager documentation.
*
*
* map<string, string> labels = 34;
*/
java.util.Map getLabelsMap();
/**
*
*
*
* The labels applied to a resource must meet the following requirements:
*
* * Each resource can have multiple labels, up to a maximum of 64.
* * Each label must be a key-value pair.
* * Keys have a minimum length of 1 character and a maximum length of 63
* characters and cannot be empty. Values can be empty and have a maximum
* length of 63 characters.
* * Keys and values can contain only lowercase letters, numeric characters,
* underscores, and dashes. All characters must use UTF-8 encoding, and
* international characters are allowed.
* * The key portion of a label must be unique. However, you can use the same
* key with multiple resources.
* * Keys must start with a lowercase letter or international character.
*
* For more information, see [Requirements for
* labels](https://cloud.google.com/resource-manager/docs/creating-managing-labels#requirements)
* in the Resource Manager documentation.
*
*
* map<string, string> labels = 34;
*/
/* nullable */
java.lang.String getLabelsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
*
*
*
* The labels applied to a resource must meet the following requirements:
*
* * Each resource can have multiple labels, up to a maximum of 64.
* * Each label must be a key-value pair.
* * Keys have a minimum length of 1 character and a maximum length of 63
* characters and cannot be empty. Values can be empty and have a maximum
* length of 63 characters.
* * Keys and values can contain only lowercase letters, numeric characters,
* underscores, and dashes. All characters must use UTF-8 encoding, and
* international characters are allowed.
* * The key portion of a label must be unique. However, you can use the same
* key with multiple resources.
* * Keys must start with a lowercase letter or international character.
*
* For more information, see [Requirements for
* labels](https://cloud.google.com/resource-manager/docs/creating-managing-labels#requirements)
* in the Resource Manager documentation.
*
*
* map<string, string> labels = 34;
*/
java.lang.String getLabelsOrThrow(java.lang.String key);
/**
*
*
*
* The spell correction specification that specifies the mode under
* which spell correction will take effect.
*
*
*
* optional .google.cloud.retail.v2alpha.SearchRequest.SpellCorrectionSpec spell_correction_spec = 35;
*
*
* @return Whether the spellCorrectionSpec field is set.
*/
boolean hasSpellCorrectionSpec();
/**
*
*
*
* The spell correction specification that specifies the mode under
* which spell correction will take effect.
*
*
*
* optional .google.cloud.retail.v2alpha.SearchRequest.SpellCorrectionSpec spell_correction_spec = 35;
*
*
* @return The spellCorrectionSpec.
*/
com.google.cloud.retail.v2alpha.SearchRequest.SpellCorrectionSpec getSpellCorrectionSpec();
/**
*
*
*
* The spell correction specification that specifies the mode under
* which spell correction will take effect.
*
*
*
* optional .google.cloud.retail.v2alpha.SearchRequest.SpellCorrectionSpec spell_correction_spec = 35;
*
*/
com.google.cloud.retail.v2alpha.SearchRequest.SpellCorrectionSpecOrBuilder
getSpellCorrectionSpecOrBuilder();
/**
*
*
*
* The entity for customers that may run multiple different entities, domains,
* sites or regions, for example, `Google US`, `Google Ads`, `Waymo`,
* `google.com`, `youtube.com`, etc.
* If this is set, it should be exactly matched with
* [UserEvent.entity][google.cloud.retail.v2alpha.UserEvent.entity] to get
* search results boosted by entity.
*
*
* string entity = 38;
*
* @return The entity.
*/
java.lang.String getEntity();
/**
*
*
*
* The entity for customers that may run multiple different entities, domains,
* sites or regions, for example, `Google US`, `Google Ads`, `Waymo`,
* `google.com`, `youtube.com`, etc.
* If this is set, it should be exactly matched with
* [UserEvent.entity][google.cloud.retail.v2alpha.UserEvent.entity] to get
* search results boosted by entity.
*
*
* string entity = 38;
*
* @return The bytes for entity.
*/
com.google.protobuf.ByteString getEntityBytes();
/**
*
*
*
* Optional. This field specifies all conversational related parameters
* addition to traditional retail search.
*
*
*
* .google.cloud.retail.v2alpha.SearchRequest.ConversationalSearchSpec conversational_search_spec = 40 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the conversationalSearchSpec field is set.
*/
boolean hasConversationalSearchSpec();
/**
*
*
*
* Optional. This field specifies all conversational related parameters
* addition to traditional retail search.
*
*
*
* .google.cloud.retail.v2alpha.SearchRequest.ConversationalSearchSpec conversational_search_spec = 40 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The conversationalSearchSpec.
*/
com.google.cloud.retail.v2alpha.SearchRequest.ConversationalSearchSpec
getConversationalSearchSpec();
/**
*
*
*
* Optional. This field specifies all conversational related parameters
* addition to traditional retail search.
*
*
*
* .google.cloud.retail.v2alpha.SearchRequest.ConversationalSearchSpec conversational_search_spec = 40 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.retail.v2alpha.SearchRequest.ConversationalSearchSpecOrBuilder
getConversationalSearchSpecOrBuilder();
/**
*
*
*
* Optional. This field specifies tile navigation related parameters.
*
*
*
* .google.cloud.retail.v2alpha.SearchRequest.TileNavigationSpec tile_navigation_spec = 41 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return Whether the tileNavigationSpec field is set.
*/
boolean hasTileNavigationSpec();
/**
*
*
*
* Optional. This field specifies tile navigation related parameters.
*
*
*
* .google.cloud.retail.v2alpha.SearchRequest.TileNavigationSpec tile_navigation_spec = 41 [(.google.api.field_behavior) = OPTIONAL];
*
*
* @return The tileNavigationSpec.
*/
com.google.cloud.retail.v2alpha.SearchRequest.TileNavigationSpec getTileNavigationSpec();
/**
*
*
*
* Optional. This field specifies tile navigation related parameters.
*
*
*
* .google.cloud.retail.v2alpha.SearchRequest.TileNavigationSpec tile_navigation_spec = 41 [(.google.api.field_behavior) = OPTIONAL];
*
*/
com.google.cloud.retail.v2alpha.SearchRequest.TileNavigationSpecOrBuilder
getTileNavigationSpecOrBuilder();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy