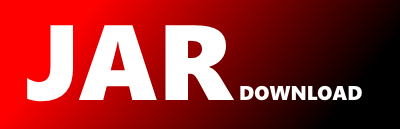
com.google.cloud.retail.v2alpha.UserEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Proto library for google-cloud-retail
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/retail/v2alpha/user_event.proto
// Protobuf Java Version: 3.25.5
package com.google.cloud.retail.v2alpha;
/**
*
*
*
* UserEvent captures all metadata information Retail API needs to know about
* how end users interact with customers' website.
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.UserEvent}
*/
public final class UserEvent extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.retail.v2alpha.UserEvent)
UserEventOrBuilder {
private static final long serialVersionUID = 0L;
// Use UserEvent.newBuilder() to construct.
private UserEvent(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private UserEvent() {
eventType_ = "";
visitorId_ = "";
sessionId_ = "";
experimentIds_ = com.google.protobuf.LazyStringArrayList.emptyList();
attributionToken_ = "";
productDetails_ = java.util.Collections.emptyList();
cartId_ = "";
searchQuery_ = "";
filter_ = "";
orderBy_ = "";
pageCategories_ = com.google.protobuf.LazyStringArrayList.emptyList();
uri_ = "";
referrerUri_ = "";
pageViewId_ = "";
entity_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new UserEvent();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.UserEventProto
.internal_static_google_cloud_retail_v2alpha_UserEvent_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 7:
return internalGetAttributes();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.UserEventProto
.internal_static_google_cloud_retail_v2alpha_UserEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.UserEvent.class,
com.google.cloud.retail.v2alpha.UserEvent.Builder.class);
}
private int bitField0_;
public static final int EVENT_TYPE_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object eventType_ = "";
/**
*
*
*
* Required. User event type. Allowed values are:
*
* * `add-to-cart`: Products being added to cart.
* * `remove-from-cart`: Products being removed from cart.
* * `category-page-view`: Special pages such as sale or promotion pages
* viewed.
* * `detail-page-view`: Products detail page viewed.
* * `home-page-view`: Homepage viewed.
* * `promotion-offered`: Promotion is offered to a user.
* * `promotion-not-offered`: Promotion is not offered to a user.
* * `purchase-complete`: User finishing a purchase.
* * `search`: Product search.
* * `shopping-cart-page-view`: User viewing a shopping cart.
*
*
* string event_type = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The eventType.
*/
@java.lang.Override
public java.lang.String getEventType() {
java.lang.Object ref = eventType_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
eventType_ = s;
return s;
}
}
/**
*
*
*
* Required. User event type. Allowed values are:
*
* * `add-to-cart`: Products being added to cart.
* * `remove-from-cart`: Products being removed from cart.
* * `category-page-view`: Special pages such as sale or promotion pages
* viewed.
* * `detail-page-view`: Products detail page viewed.
* * `home-page-view`: Homepage viewed.
* * `promotion-offered`: Promotion is offered to a user.
* * `promotion-not-offered`: Promotion is not offered to a user.
* * `purchase-complete`: User finishing a purchase.
* * `search`: Product search.
* * `shopping-cart-page-view`: User viewing a shopping cart.
*
*
* string event_type = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for eventType.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEventTypeBytes() {
java.lang.Object ref = eventType_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
eventType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VISITOR_ID_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object visitorId_ = "";
/**
*
*
*
* Required. A unique identifier for tracking visitors.
*
* For example, this could be implemented with an HTTP cookie, which should be
* able to uniquely identify a visitor on a single device. This unique
* identifier should not change if the visitor log in/out of the website.
*
* Don't set the field to the same fixed ID for different users. This mixes
* the event history of those users together, which results in degraded model
* quality.
*
* The field must be a UTF-8 encoded string with a length limit of 128
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* The field should not contain PII or user-data. We recommend to use Google
* Analytics [Client
* ID](https://developers.google.com/analytics/devguides/collection/analyticsjs/field-reference#clientId)
* for this field.
*
*
* string visitor_id = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return The visitorId.
*/
@java.lang.Override
public java.lang.String getVisitorId() {
java.lang.Object ref = visitorId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
visitorId_ = s;
return s;
}
}
/**
*
*
*
* Required. A unique identifier for tracking visitors.
*
* For example, this could be implemented with an HTTP cookie, which should be
* able to uniquely identify a visitor on a single device. This unique
* identifier should not change if the visitor log in/out of the website.
*
* Don't set the field to the same fixed ID for different users. This mixes
* the event history of those users together, which results in degraded model
* quality.
*
* The field must be a UTF-8 encoded string with a length limit of 128
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* The field should not contain PII or user-data. We recommend to use Google
* Analytics [Client
* ID](https://developers.google.com/analytics/devguides/collection/analyticsjs/field-reference#clientId)
* for this field.
*
*
* string visitor_id = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for visitorId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getVisitorIdBytes() {
java.lang.Object ref = visitorId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
visitorId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SESSION_ID_FIELD_NUMBER = 21;
@SuppressWarnings("serial")
private volatile java.lang.Object sessionId_ = "";
/**
*
*
*
* A unique identifier for tracking a visitor session with a length limit of
* 128 bytes. A session is an aggregation of an end user behavior in a time
* span.
*
* A general guideline to populate the sesion_id:
* 1. If user has no activity for 30 min, a new session_id should be assigned.
* 2. The session_id should be unique across users, suggest use uuid or add
* visitor_id as prefix.
*
*
* string session_id = 21;
*
* @return The sessionId.
*/
@java.lang.Override
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
}
}
/**
*
*
*
* A unique identifier for tracking a visitor session with a length limit of
* 128 bytes. A session is an aggregation of an end user behavior in a time
* span.
*
* A general guideline to populate the sesion_id:
* 1. If user has no activity for 30 min, a new session_id should be assigned.
* 2. The session_id should be unique across users, suggest use uuid or add
* visitor_id as prefix.
*
*
* string session_id = 21;
*
* @return The bytes for sessionId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EVENT_TIME_FIELD_NUMBER = 3;
private com.google.protobuf.Timestamp eventTime_;
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*
* @return Whether the eventTime field is set.
*/
@java.lang.Override
public boolean hasEventTime() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*
* @return The eventTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getEventTime() {
return eventTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : eventTime_;
}
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getEventTimeOrBuilder() {
return eventTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : eventTime_;
}
public static final int EXPERIMENT_IDS_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList experimentIds_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @return A list containing the experimentIds.
*/
public com.google.protobuf.ProtocolStringList getExperimentIdsList() {
return experimentIds_;
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @return The count of experimentIds.
*/
public int getExperimentIdsCount() {
return experimentIds_.size();
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @param index The index of the element to return.
* @return The experimentIds at the given index.
*/
public java.lang.String getExperimentIds(int index) {
return experimentIds_.get(index);
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @param index The index of the value to return.
* @return The bytes of the experimentIds at the given index.
*/
public com.google.protobuf.ByteString getExperimentIdsBytes(int index) {
return experimentIds_.getByteString(index);
}
public static final int ATTRIBUTION_TOKEN_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object attributionToken_ = "";
/**
*
*
*
* Highly recommended for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* This field enables accurate attribution of recommendation model
* performance.
*
* The value must be a valid
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* The value must be a valid
* [SearchResponse.attribution_token][google.cloud.retail.v2alpha.SearchResponse.attribution_token]
* for user events that are the result of
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search].
*
* This token enables us to accurately attribute page view or purchase back to
* the event and the particular predict response containing this
* clicked/purchased product. If user clicks on product K in the
* recommendation results, pass
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* as a URL parameter to product K's page. When recording events on product
* K's page, log the
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* to this field.
*
*
* string attribution_token = 5;
*
* @return The attributionToken.
*/
@java.lang.Override
public java.lang.String getAttributionToken() {
java.lang.Object ref = attributionToken_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
attributionToken_ = s;
return s;
}
}
/**
*
*
*
* Highly recommended for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* This field enables accurate attribution of recommendation model
* performance.
*
* The value must be a valid
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* The value must be a valid
* [SearchResponse.attribution_token][google.cloud.retail.v2alpha.SearchResponse.attribution_token]
* for user events that are the result of
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search].
*
* This token enables us to accurately attribute page view or purchase back to
* the event and the particular predict response containing this
* clicked/purchased product. If user clicks on product K in the
* recommendation results, pass
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* as a URL parameter to product K's page. When recording events on product
* K's page, log the
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* to this field.
*
*
* string attribution_token = 5;
*
* @return The bytes for attributionToken.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAttributionTokenBytes() {
java.lang.Object ref = attributionToken_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
attributionToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PRODUCT_DETAILS_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private java.util.List productDetails_;
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
@java.lang.Override
public java.util.List getProductDetailsList() {
return productDetails_;
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.retail.v2alpha.ProductDetailOrBuilder>
getProductDetailsOrBuilderList() {
return productDetails_;
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
@java.lang.Override
public int getProductDetailsCount() {
return productDetails_.size();
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.ProductDetail getProductDetails(int index) {
return productDetails_.get(index);
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.ProductDetailOrBuilder getProductDetailsOrBuilder(
int index) {
return productDetails_.get(index);
}
public static final int COMPLETION_DETAIL_FIELD_NUMBER = 22;
private com.google.cloud.retail.v2alpha.CompletionDetail completionDetail_;
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*
* @return Whether the completionDetail field is set.
*/
@java.lang.Override
public boolean hasCompletionDetail() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*
* @return The completionDetail.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.CompletionDetail getCompletionDetail() {
return completionDetail_ == null
? com.google.cloud.retail.v2alpha.CompletionDetail.getDefaultInstance()
: completionDetail_;
}
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.CompletionDetailOrBuilder getCompletionDetailOrBuilder() {
return completionDetail_ == null
? com.google.cloud.retail.v2alpha.CompletionDetail.getDefaultInstance()
: completionDetail_;
}
public static final int ATTRIBUTES_FIELD_NUMBER = 7;
private static final class AttributesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.retail.v2alpha.CustomAttribute>
defaultEntry =
com.google.protobuf.MapEntry
.
newDefaultInstance(
com.google.cloud.retail.v2alpha.UserEventProto
.internal_static_google_cloud_retail_v2alpha_UserEvent_AttributesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.cloud.retail.v2alpha.CustomAttribute.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.cloud.retail.v2alpha.CustomAttribute>
attributes_;
private com.google.protobuf.MapField<
java.lang.String, com.google.cloud.retail.v2alpha.CustomAttribute>
internalGetAttributes() {
if (attributes_ == null) {
return com.google.protobuf.MapField.emptyMapField(AttributesDefaultEntryHolder.defaultEntry);
}
return attributes_;
}
public int getAttributesCount() {
return internalGetAttributes().getMap().size();
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
@java.lang.Override
public boolean containsAttributes(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetAttributes().getMap().containsKey(key);
}
/** Use {@link #getAttributesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getAttributes() {
return getAttributesMap();
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
@java.lang.Override
public java.util.Map
getAttributesMap() {
return internalGetAttributes().getMap();
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
@java.lang.Override
public /* nullable */ com.google.cloud.retail.v2alpha.CustomAttribute getAttributesOrDefault(
java.lang.String key,
/* nullable */
com.google.cloud.retail.v2alpha.CustomAttribute defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetAttributes().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.CustomAttribute getAttributesOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetAttributes().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int CART_ID_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private volatile java.lang.Object cartId_ = "";
/**
*
*
*
* The ID or name of the associated shopping cart. This ID is used
* to associate multiple items added or present in the cart before purchase.
*
* This can only be set for `add-to-cart`, `purchase-complete`, or
* `shopping-cart-page-view` events.
*
*
* string cart_id = 8;
*
* @return The cartId.
*/
@java.lang.Override
public java.lang.String getCartId() {
java.lang.Object ref = cartId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cartId_ = s;
return s;
}
}
/**
*
*
*
* The ID or name of the associated shopping cart. This ID is used
* to associate multiple items added or present in the cart before purchase.
*
* This can only be set for `add-to-cart`, `purchase-complete`, or
* `shopping-cart-page-view` events.
*
*
* string cart_id = 8;
*
* @return The bytes for cartId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCartIdBytes() {
java.lang.Object ref = cartId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
cartId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PURCHASE_TRANSACTION_FIELD_NUMBER = 9;
private com.google.cloud.retail.v2alpha.PurchaseTransaction purchaseTransaction_;
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*
* @return Whether the purchaseTransaction field is set.
*/
@java.lang.Override
public boolean hasPurchaseTransaction() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*
* @return The purchaseTransaction.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.PurchaseTransaction getPurchaseTransaction() {
return purchaseTransaction_ == null
? com.google.cloud.retail.v2alpha.PurchaseTransaction.getDefaultInstance()
: purchaseTransaction_;
}
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.PurchaseTransactionOrBuilder
getPurchaseTransactionOrBuilder() {
return purchaseTransaction_ == null
? com.google.cloud.retail.v2alpha.PurchaseTransaction.getDefaultInstance()
: purchaseTransaction_;
}
public static final int SEARCH_QUERY_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private volatile java.lang.Object searchQuery_ = "";
/**
*
*
*
* The user's search query.
*
* See [SearchRequest.query][google.cloud.retail.v2alpha.SearchRequest.query]
* for definition.
*
* The value must be a UTF-8 encoded string with a length limit of 5,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string search_query = 10;
*
* @return The searchQuery.
*/
@java.lang.Override
public java.lang.String getSearchQuery() {
java.lang.Object ref = searchQuery_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
searchQuery_ = s;
return s;
}
}
/**
*
*
*
* The user's search query.
*
* See [SearchRequest.query][google.cloud.retail.v2alpha.SearchRequest.query]
* for definition.
*
* The value must be a UTF-8 encoded string with a length limit of 5,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string search_query = 10;
*
* @return The bytes for searchQuery.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSearchQueryBytes() {
java.lang.Object ref = searchQuery_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
searchQuery_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FILTER_FIELD_NUMBER = 16;
@SuppressWarnings("serial")
private volatile java.lang.Object filter_ = "";
/**
*
*
*
* The filter syntax consists of an expression language for constructing a
* predicate from one or more fields of the products being filtered.
*
* See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string filter = 16;
*
* @return The filter.
*/
@java.lang.Override
public java.lang.String getFilter() {
java.lang.Object ref = filter_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
filter_ = s;
return s;
}
}
/**
*
*
*
* The filter syntax consists of an expression language for constructing a
* predicate from one or more fields of the products being filtered.
*
* See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string filter = 16;
*
* @return The bytes for filter.
*/
@java.lang.Override
public com.google.protobuf.ByteString getFilterBytes() {
java.lang.Object ref = filter_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
filter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ORDER_BY_FIELD_NUMBER = 17;
@SuppressWarnings("serial")
private volatile java.lang.Object orderBy_ = "";
/**
*
*
*
* The order in which products are returned.
*
* See
* [SearchRequest.order_by][google.cloud.retail.v2alpha.SearchRequest.order_by]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* This can only be set for `search` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string order_by = 17;
*
* @return The orderBy.
*/
@java.lang.Override
public java.lang.String getOrderBy() {
java.lang.Object ref = orderBy_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
orderBy_ = s;
return s;
}
}
/**
*
*
*
* The order in which products are returned.
*
* See
* [SearchRequest.order_by][google.cloud.retail.v2alpha.SearchRequest.order_by]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* This can only be set for `search` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string order_by = 17;
*
* @return The bytes for orderBy.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOrderByBytes() {
java.lang.Object ref = orderBy_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
orderBy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int OFFSET_FIELD_NUMBER = 18;
private int offset_ = 0;
/**
*
*
*
* An integer that specifies the current offset for pagination (the 0-indexed
* starting location, amongst the products deemed by the API as relevant).
*
* See
* [SearchRequest.offset][google.cloud.retail.v2alpha.SearchRequest.offset]
* for definition.
*
* If this field is negative, an INVALID_ARGUMENT is returned.
*
* This can only be set for `search` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* int32 offset = 18;
*
* @return The offset.
*/
@java.lang.Override
public int getOffset() {
return offset_;
}
public static final int PAGE_CATEGORIES_FIELD_NUMBER = 11;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList pageCategories_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @return A list containing the pageCategories.
*/
public com.google.protobuf.ProtocolStringList getPageCategoriesList() {
return pageCategories_;
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @return The count of pageCategories.
*/
public int getPageCategoriesCount() {
return pageCategories_.size();
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @param index The index of the element to return.
* @return The pageCategories at the given index.
*/
public java.lang.String getPageCategories(int index) {
return pageCategories_.get(index);
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @param index The index of the value to return.
* @return The bytes of the pageCategories at the given index.
*/
public com.google.protobuf.ByteString getPageCategoriesBytes(int index) {
return pageCategories_.getByteString(index);
}
public static final int USER_INFO_FIELD_NUMBER = 12;
private com.google.cloud.retail.v2alpha.UserInfo userInfo_;
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*
* @return Whether the userInfo field is set.
*/
@java.lang.Override
public boolean hasUserInfo() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*
* @return The userInfo.
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.UserInfo getUserInfo() {
return userInfo_ == null
? com.google.cloud.retail.v2alpha.UserInfo.getDefaultInstance()
: userInfo_;
}
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.UserInfoOrBuilder getUserInfoOrBuilder() {
return userInfo_ == null
? com.google.cloud.retail.v2alpha.UserInfo.getDefaultInstance()
: userInfo_;
}
public static final int URI_FIELD_NUMBER = 13;
@SuppressWarnings("serial")
private volatile java.lang.Object uri_ = "";
/**
*
*
*
* Complete URL (window.location.href) of the user's current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically. Maximum length 5,000
* characters.
*
*
* string uri = 13;
*
* @return The uri.
*/
@java.lang.Override
public java.lang.String getUri() {
java.lang.Object ref = uri_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uri_ = s;
return s;
}
}
/**
*
*
*
* Complete URL (window.location.href) of the user's current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically. Maximum length 5,000
* characters.
*
*
* string uri = 13;
*
* @return The bytes for uri.
*/
@java.lang.Override
public com.google.protobuf.ByteString getUriBytes() {
java.lang.Object ref = uri_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
uri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int REFERRER_URI_FIELD_NUMBER = 14;
@SuppressWarnings("serial")
private volatile java.lang.Object referrerUri_ = "";
/**
*
*
*
* The referrer URL of the current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string referrer_uri = 14;
*
* @return The referrerUri.
*/
@java.lang.Override
public java.lang.String getReferrerUri() {
java.lang.Object ref = referrerUri_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
referrerUri_ = s;
return s;
}
}
/**
*
*
*
* The referrer URL of the current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string referrer_uri = 14;
*
* @return The bytes for referrerUri.
*/
@java.lang.Override
public com.google.protobuf.ByteString getReferrerUriBytes() {
java.lang.Object ref = referrerUri_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
referrerUri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAGE_VIEW_ID_FIELD_NUMBER = 15;
@SuppressWarnings("serial")
private volatile java.lang.Object pageViewId_ = "";
/**
*
*
*
* A unique ID of a web page view.
*
* This should be kept the same for all user events triggered from the same
* pageview. For example, an item detail page view could trigger multiple
* events as the user is browsing the page. The `pageViewId` property should
* be kept the same for all these events so that they can be grouped together
* properly.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string page_view_id = 15;
*
* @return The pageViewId.
*/
@java.lang.Override
public java.lang.String getPageViewId() {
java.lang.Object ref = pageViewId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
pageViewId_ = s;
return s;
}
}
/**
*
*
*
* A unique ID of a web page view.
*
* This should be kept the same for all user events triggered from the same
* pageview. For example, an item detail page view could trigger multiple
* events as the user is browsing the page. The `pageViewId` property should
* be kept the same for all these events so that they can be grouped together
* properly.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string page_view_id = 15;
*
* @return The bytes for pageViewId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getPageViewIdBytes() {
java.lang.Object ref = pageViewId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
pageViewId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ENTITY_FIELD_NUMBER = 23;
@SuppressWarnings("serial")
private volatile java.lang.Object entity_ = "";
/**
*
*
*
* The entity for customers that may run multiple different entities, domains,
* sites or regions, for example, `Google US`, `Google Ads`, `Waymo`,
* `google.com`, `youtube.com`, etc.
* We recommend that you set this field to get better per-entity search,
* completion, and prediction results.
*
*
* string entity = 23;
*
* @return The entity.
*/
@java.lang.Override
public java.lang.String getEntity() {
java.lang.Object ref = entity_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
entity_ = s;
return s;
}
}
/**
*
*
*
* The entity for customers that may run multiple different entities, domains,
* sites or regions, for example, `Google US`, `Google Ads`, `Waymo`,
* `google.com`, `youtube.com`, etc.
* We recommend that you set this field to get better per-entity search,
* completion, and prediction results.
*
*
* string entity = 23;
*
* @return The bytes for entity.
*/
@java.lang.Override
public com.google.protobuf.ByteString getEntityBytes() {
java.lang.Object ref = entity_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
entity_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(eventType_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, eventType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(visitorId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, visitorId_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(3, getEventTime());
}
for (int i = 0; i < experimentIds_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, experimentIds_.getRaw(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(attributionToken_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, attributionToken_);
}
for (int i = 0; i < productDetails_.size(); i++) {
output.writeMessage(6, productDetails_.get(i));
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetAttributes(), AttributesDefaultEntryHolder.defaultEntry, 7);
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(cartId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, cartId_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(9, getPurchaseTransaction());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(searchQuery_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 10, searchQuery_);
}
for (int i = 0; i < pageCategories_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, pageCategories_.getRaw(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(12, getUserInfo());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(uri_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 13, uri_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(referrerUri_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 14, referrerUri_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(pageViewId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 15, pageViewId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(filter_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 16, filter_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(orderBy_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 17, orderBy_);
}
if (offset_ != 0) {
output.writeInt32(18, offset_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sessionId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 21, sessionId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(22, getCompletionDetail());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(entity_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 23, entity_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(eventType_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, eventType_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(visitorId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, visitorId_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(3, getEventTime());
}
{
int dataSize = 0;
for (int i = 0; i < experimentIds_.size(); i++) {
dataSize += computeStringSizeNoTag(experimentIds_.getRaw(i));
}
size += dataSize;
size += 1 * getExperimentIdsList().size();
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(attributionToken_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, attributionToken_);
}
for (int i = 0; i < productDetails_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(6, productDetails_.get(i));
}
for (java.util.Map.Entry
entry : internalGetAttributes().getMap().entrySet()) {
com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.retail.v2alpha.CustomAttribute>
attributes__ =
AttributesDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(7, attributes__);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(cartId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, cartId_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(9, getPurchaseTransaction());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(searchQuery_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(10, searchQuery_);
}
{
int dataSize = 0;
for (int i = 0; i < pageCategories_.size(); i++) {
dataSize += computeStringSizeNoTag(pageCategories_.getRaw(i));
}
size += dataSize;
size += 1 * getPageCategoriesList().size();
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(12, getUserInfo());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(uri_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(13, uri_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(referrerUri_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(14, referrerUri_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(pageViewId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(15, pageViewId_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(filter_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(16, filter_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(orderBy_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(17, orderBy_);
}
if (offset_ != 0) {
size += com.google.protobuf.CodedOutputStream.computeInt32Size(18, offset_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(sessionId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(21, sessionId_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(22, getCompletionDetail());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(entity_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(23, entity_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.retail.v2alpha.UserEvent)) {
return super.equals(obj);
}
com.google.cloud.retail.v2alpha.UserEvent other =
(com.google.cloud.retail.v2alpha.UserEvent) obj;
if (!getEventType().equals(other.getEventType())) return false;
if (!getVisitorId().equals(other.getVisitorId())) return false;
if (!getSessionId().equals(other.getSessionId())) return false;
if (hasEventTime() != other.hasEventTime()) return false;
if (hasEventTime()) {
if (!getEventTime().equals(other.getEventTime())) return false;
}
if (!getExperimentIdsList().equals(other.getExperimentIdsList())) return false;
if (!getAttributionToken().equals(other.getAttributionToken())) return false;
if (!getProductDetailsList().equals(other.getProductDetailsList())) return false;
if (hasCompletionDetail() != other.hasCompletionDetail()) return false;
if (hasCompletionDetail()) {
if (!getCompletionDetail().equals(other.getCompletionDetail())) return false;
}
if (!internalGetAttributes().equals(other.internalGetAttributes())) return false;
if (!getCartId().equals(other.getCartId())) return false;
if (hasPurchaseTransaction() != other.hasPurchaseTransaction()) return false;
if (hasPurchaseTransaction()) {
if (!getPurchaseTransaction().equals(other.getPurchaseTransaction())) return false;
}
if (!getSearchQuery().equals(other.getSearchQuery())) return false;
if (!getFilter().equals(other.getFilter())) return false;
if (!getOrderBy().equals(other.getOrderBy())) return false;
if (getOffset() != other.getOffset()) return false;
if (!getPageCategoriesList().equals(other.getPageCategoriesList())) return false;
if (hasUserInfo() != other.hasUserInfo()) return false;
if (hasUserInfo()) {
if (!getUserInfo().equals(other.getUserInfo())) return false;
}
if (!getUri().equals(other.getUri())) return false;
if (!getReferrerUri().equals(other.getReferrerUri())) return false;
if (!getPageViewId().equals(other.getPageViewId())) return false;
if (!getEntity().equals(other.getEntity())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + EVENT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + getEventType().hashCode();
hash = (37 * hash) + VISITOR_ID_FIELD_NUMBER;
hash = (53 * hash) + getVisitorId().hashCode();
hash = (37 * hash) + SESSION_ID_FIELD_NUMBER;
hash = (53 * hash) + getSessionId().hashCode();
if (hasEventTime()) {
hash = (37 * hash) + EVENT_TIME_FIELD_NUMBER;
hash = (53 * hash) + getEventTime().hashCode();
}
if (getExperimentIdsCount() > 0) {
hash = (37 * hash) + EXPERIMENT_IDS_FIELD_NUMBER;
hash = (53 * hash) + getExperimentIdsList().hashCode();
}
hash = (37 * hash) + ATTRIBUTION_TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getAttributionToken().hashCode();
if (getProductDetailsCount() > 0) {
hash = (37 * hash) + PRODUCT_DETAILS_FIELD_NUMBER;
hash = (53 * hash) + getProductDetailsList().hashCode();
}
if (hasCompletionDetail()) {
hash = (37 * hash) + COMPLETION_DETAIL_FIELD_NUMBER;
hash = (53 * hash) + getCompletionDetail().hashCode();
}
if (!internalGetAttributes().getMap().isEmpty()) {
hash = (37 * hash) + ATTRIBUTES_FIELD_NUMBER;
hash = (53 * hash) + internalGetAttributes().hashCode();
}
hash = (37 * hash) + CART_ID_FIELD_NUMBER;
hash = (53 * hash) + getCartId().hashCode();
if (hasPurchaseTransaction()) {
hash = (37 * hash) + PURCHASE_TRANSACTION_FIELD_NUMBER;
hash = (53 * hash) + getPurchaseTransaction().hashCode();
}
hash = (37 * hash) + SEARCH_QUERY_FIELD_NUMBER;
hash = (53 * hash) + getSearchQuery().hashCode();
hash = (37 * hash) + FILTER_FIELD_NUMBER;
hash = (53 * hash) + getFilter().hashCode();
hash = (37 * hash) + ORDER_BY_FIELD_NUMBER;
hash = (53 * hash) + getOrderBy().hashCode();
hash = (37 * hash) + OFFSET_FIELD_NUMBER;
hash = (53 * hash) + getOffset();
if (getPageCategoriesCount() > 0) {
hash = (37 * hash) + PAGE_CATEGORIES_FIELD_NUMBER;
hash = (53 * hash) + getPageCategoriesList().hashCode();
}
if (hasUserInfo()) {
hash = (37 * hash) + USER_INFO_FIELD_NUMBER;
hash = (53 * hash) + getUserInfo().hashCode();
}
hash = (37 * hash) + URI_FIELD_NUMBER;
hash = (53 * hash) + getUri().hashCode();
hash = (37 * hash) + REFERRER_URI_FIELD_NUMBER;
hash = (53 * hash) + getReferrerUri().hashCode();
hash = (37 * hash) + PAGE_VIEW_ID_FIELD_NUMBER;
hash = (53 * hash) + getPageViewId().hashCode();
hash = (37 * hash) + ENTITY_FIELD_NUMBER;
hash = (53 * hash) + getEntity().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.retail.v2alpha.UserEvent parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.UserEvent parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.UserEvent parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.UserEvent parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.UserEvent parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.retail.v2alpha.UserEvent parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.UserEvent parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.UserEvent parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.UserEvent parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.UserEvent parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.retail.v2alpha.UserEvent parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.retail.v2alpha.UserEvent parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.retail.v2alpha.UserEvent prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* UserEvent captures all metadata information Retail API needs to know about
* how end users interact with customers' website.
*
*
* Protobuf type {@code google.cloud.retail.v2alpha.UserEvent}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.retail.v2alpha.UserEvent)
com.google.cloud.retail.v2alpha.UserEventOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.retail.v2alpha.UserEventProto
.internal_static_google_cloud_retail_v2alpha_UserEvent_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 7:
return internalGetAttributes();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 7:
return internalGetMutableAttributes();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.retail.v2alpha.UserEventProto
.internal_static_google_cloud_retail_v2alpha_UserEvent_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.retail.v2alpha.UserEvent.class,
com.google.cloud.retail.v2alpha.UserEvent.Builder.class);
}
// Construct using com.google.cloud.retail.v2alpha.UserEvent.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getEventTimeFieldBuilder();
getProductDetailsFieldBuilder();
getCompletionDetailFieldBuilder();
getPurchaseTransactionFieldBuilder();
getUserInfoFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
eventType_ = "";
visitorId_ = "";
sessionId_ = "";
eventTime_ = null;
if (eventTimeBuilder_ != null) {
eventTimeBuilder_.dispose();
eventTimeBuilder_ = null;
}
experimentIds_ = com.google.protobuf.LazyStringArrayList.emptyList();
attributionToken_ = "";
if (productDetailsBuilder_ == null) {
productDetails_ = java.util.Collections.emptyList();
} else {
productDetails_ = null;
productDetailsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
completionDetail_ = null;
if (completionDetailBuilder_ != null) {
completionDetailBuilder_.dispose();
completionDetailBuilder_ = null;
}
internalGetMutableAttributes().clear();
cartId_ = "";
purchaseTransaction_ = null;
if (purchaseTransactionBuilder_ != null) {
purchaseTransactionBuilder_.dispose();
purchaseTransactionBuilder_ = null;
}
searchQuery_ = "";
filter_ = "";
orderBy_ = "";
offset_ = 0;
pageCategories_ = com.google.protobuf.LazyStringArrayList.emptyList();
userInfo_ = null;
if (userInfoBuilder_ != null) {
userInfoBuilder_.dispose();
userInfoBuilder_ = null;
}
uri_ = "";
referrerUri_ = "";
pageViewId_ = "";
entity_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.retail.v2alpha.UserEventProto
.internal_static_google_cloud_retail_v2alpha_UserEvent_descriptor;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.UserEvent getDefaultInstanceForType() {
return com.google.cloud.retail.v2alpha.UserEvent.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.UserEvent build() {
com.google.cloud.retail.v2alpha.UserEvent result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.UserEvent buildPartial() {
com.google.cloud.retail.v2alpha.UserEvent result =
new com.google.cloud.retail.v2alpha.UserEvent(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.cloud.retail.v2alpha.UserEvent result) {
if (productDetailsBuilder_ == null) {
if (((bitField0_ & 0x00000040) != 0)) {
productDetails_ = java.util.Collections.unmodifiableList(productDetails_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.productDetails_ = productDetails_;
} else {
result.productDetails_ = productDetailsBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.retail.v2alpha.UserEvent result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.eventType_ = eventType_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.visitorId_ = visitorId_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.sessionId_ = sessionId_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000008) != 0)) {
result.eventTime_ = eventTimeBuilder_ == null ? eventTime_ : eventTimeBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
experimentIds_.makeImmutable();
result.experimentIds_ = experimentIds_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.attributionToken_ = attributionToken_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.completionDetail_ =
completionDetailBuilder_ == null ? completionDetail_ : completionDetailBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.attributes_ =
internalGetAttributes().build(AttributesDefaultEntryHolder.defaultEntry);
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.cartId_ = cartId_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.purchaseTransaction_ =
purchaseTransactionBuilder_ == null
? purchaseTransaction_
: purchaseTransactionBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.searchQuery_ = searchQuery_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.filter_ = filter_;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.orderBy_ = orderBy_;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.offset_ = offset_;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
pageCategories_.makeImmutable();
result.pageCategories_ = pageCategories_;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.userInfo_ = userInfoBuilder_ == null ? userInfo_ : userInfoBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.uri_ = uri_;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.referrerUri_ = referrerUri_;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.pageViewId_ = pageViewId_;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.entity_ = entity_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.retail.v2alpha.UserEvent) {
return mergeFrom((com.google.cloud.retail.v2alpha.UserEvent) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.retail.v2alpha.UserEvent other) {
if (other == com.google.cloud.retail.v2alpha.UserEvent.getDefaultInstance()) return this;
if (!other.getEventType().isEmpty()) {
eventType_ = other.eventType_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getVisitorId().isEmpty()) {
visitorId_ = other.visitorId_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.getSessionId().isEmpty()) {
sessionId_ = other.sessionId_;
bitField0_ |= 0x00000004;
onChanged();
}
if (other.hasEventTime()) {
mergeEventTime(other.getEventTime());
}
if (!other.experimentIds_.isEmpty()) {
if (experimentIds_.isEmpty()) {
experimentIds_ = other.experimentIds_;
bitField0_ |= 0x00000010;
} else {
ensureExperimentIdsIsMutable();
experimentIds_.addAll(other.experimentIds_);
}
onChanged();
}
if (!other.getAttributionToken().isEmpty()) {
attributionToken_ = other.attributionToken_;
bitField0_ |= 0x00000020;
onChanged();
}
if (productDetailsBuilder_ == null) {
if (!other.productDetails_.isEmpty()) {
if (productDetails_.isEmpty()) {
productDetails_ = other.productDetails_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureProductDetailsIsMutable();
productDetails_.addAll(other.productDetails_);
}
onChanged();
}
} else {
if (!other.productDetails_.isEmpty()) {
if (productDetailsBuilder_.isEmpty()) {
productDetailsBuilder_.dispose();
productDetailsBuilder_ = null;
productDetails_ = other.productDetails_;
bitField0_ = (bitField0_ & ~0x00000040);
productDetailsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getProductDetailsFieldBuilder()
: null;
} else {
productDetailsBuilder_.addAllMessages(other.productDetails_);
}
}
}
if (other.hasCompletionDetail()) {
mergeCompletionDetail(other.getCompletionDetail());
}
internalGetMutableAttributes().mergeFrom(other.internalGetAttributes());
bitField0_ |= 0x00000100;
if (!other.getCartId().isEmpty()) {
cartId_ = other.cartId_;
bitField0_ |= 0x00000200;
onChanged();
}
if (other.hasPurchaseTransaction()) {
mergePurchaseTransaction(other.getPurchaseTransaction());
}
if (!other.getSearchQuery().isEmpty()) {
searchQuery_ = other.searchQuery_;
bitField0_ |= 0x00000800;
onChanged();
}
if (!other.getFilter().isEmpty()) {
filter_ = other.filter_;
bitField0_ |= 0x00001000;
onChanged();
}
if (!other.getOrderBy().isEmpty()) {
orderBy_ = other.orderBy_;
bitField0_ |= 0x00002000;
onChanged();
}
if (other.getOffset() != 0) {
setOffset(other.getOffset());
}
if (!other.pageCategories_.isEmpty()) {
if (pageCategories_.isEmpty()) {
pageCategories_ = other.pageCategories_;
bitField0_ |= 0x00008000;
} else {
ensurePageCategoriesIsMutable();
pageCategories_.addAll(other.pageCategories_);
}
onChanged();
}
if (other.hasUserInfo()) {
mergeUserInfo(other.getUserInfo());
}
if (!other.getUri().isEmpty()) {
uri_ = other.uri_;
bitField0_ |= 0x00020000;
onChanged();
}
if (!other.getReferrerUri().isEmpty()) {
referrerUri_ = other.referrerUri_;
bitField0_ |= 0x00040000;
onChanged();
}
if (!other.getPageViewId().isEmpty()) {
pageViewId_ = other.pageViewId_;
bitField0_ |= 0x00080000;
onChanged();
}
if (!other.getEntity().isEmpty()) {
entity_ = other.entity_;
bitField0_ |= 0x00100000;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
eventType_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
visitorId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26:
{
input.readMessage(getEventTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 26
case 34:
{
java.lang.String s = input.readStringRequireUtf8();
ensureExperimentIdsIsMutable();
experimentIds_.add(s);
break;
} // case 34
case 42:
{
attributionToken_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 42
case 50:
{
com.google.cloud.retail.v2alpha.ProductDetail m =
input.readMessage(
com.google.cloud.retail.v2alpha.ProductDetail.parser(), extensionRegistry);
if (productDetailsBuilder_ == null) {
ensureProductDetailsIsMutable();
productDetails_.add(m);
} else {
productDetailsBuilder_.addMessage(m);
}
break;
} // case 50
case 58:
{
com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.retail.v2alpha.CustomAttribute>
attributes__ =
input.readMessage(
AttributesDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableAttributes()
.ensureBuilderMap()
.put(attributes__.getKey(), attributes__.getValue());
bitField0_ |= 0x00000100;
break;
} // case 58
case 66:
{
cartId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case 66
case 74:
{
input.readMessage(
getPurchaseTransactionFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000400;
break;
} // case 74
case 82:
{
searchQuery_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000800;
break;
} // case 82
case 90:
{
java.lang.String s = input.readStringRequireUtf8();
ensurePageCategoriesIsMutable();
pageCategories_.add(s);
break;
} // case 90
case 98:
{
input.readMessage(getUserInfoFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00010000;
break;
} // case 98
case 106:
{
uri_ = input.readStringRequireUtf8();
bitField0_ |= 0x00020000;
break;
} // case 106
case 114:
{
referrerUri_ = input.readStringRequireUtf8();
bitField0_ |= 0x00040000;
break;
} // case 114
case 122:
{
pageViewId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00080000;
break;
} // case 122
case 130:
{
filter_ = input.readStringRequireUtf8();
bitField0_ |= 0x00001000;
break;
} // case 130
case 138:
{
orderBy_ = input.readStringRequireUtf8();
bitField0_ |= 0x00002000;
break;
} // case 138
case 144:
{
offset_ = input.readInt32();
bitField0_ |= 0x00004000;
break;
} // case 144
case 170:
{
sessionId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 170
case 178:
{
input.readMessage(
getCompletionDetailFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000080;
break;
} // case 178
case 186:
{
entity_ = input.readStringRequireUtf8();
bitField0_ |= 0x00100000;
break;
} // case 186
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object eventType_ = "";
/**
*
*
*
* Required. User event type. Allowed values are:
*
* * `add-to-cart`: Products being added to cart.
* * `remove-from-cart`: Products being removed from cart.
* * `category-page-view`: Special pages such as sale or promotion pages
* viewed.
* * `detail-page-view`: Products detail page viewed.
* * `home-page-view`: Homepage viewed.
* * `promotion-offered`: Promotion is offered to a user.
* * `promotion-not-offered`: Promotion is not offered to a user.
* * `purchase-complete`: User finishing a purchase.
* * `search`: Product search.
* * `shopping-cart-page-view`: User viewing a shopping cart.
*
*
* string event_type = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The eventType.
*/
public java.lang.String getEventType() {
java.lang.Object ref = eventType_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
eventType_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Required. User event type. Allowed values are:
*
* * `add-to-cart`: Products being added to cart.
* * `remove-from-cart`: Products being removed from cart.
* * `category-page-view`: Special pages such as sale or promotion pages
* viewed.
* * `detail-page-view`: Products detail page viewed.
* * `home-page-view`: Homepage viewed.
* * `promotion-offered`: Promotion is offered to a user.
* * `promotion-not-offered`: Promotion is not offered to a user.
* * `purchase-complete`: User finishing a purchase.
* * `search`: Product search.
* * `shopping-cart-page-view`: User viewing a shopping cart.
*
*
* string event_type = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for eventType.
*/
public com.google.protobuf.ByteString getEventTypeBytes() {
java.lang.Object ref = eventType_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
eventType_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Required. User event type. Allowed values are:
*
* * `add-to-cart`: Products being added to cart.
* * `remove-from-cart`: Products being removed from cart.
* * `category-page-view`: Special pages such as sale or promotion pages
* viewed.
* * `detail-page-view`: Products detail page viewed.
* * `home-page-view`: Homepage viewed.
* * `promotion-offered`: Promotion is offered to a user.
* * `promotion-not-offered`: Promotion is not offered to a user.
* * `purchase-complete`: User finishing a purchase.
* * `search`: Product search.
* * `shopping-cart-page-view`: User viewing a shopping cart.
*
*
* string event_type = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @param value The eventType to set.
* @return This builder for chaining.
*/
public Builder setEventType(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
eventType_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Required. User event type. Allowed values are:
*
* * `add-to-cart`: Products being added to cart.
* * `remove-from-cart`: Products being removed from cart.
* * `category-page-view`: Special pages such as sale or promotion pages
* viewed.
* * `detail-page-view`: Products detail page viewed.
* * `home-page-view`: Homepage viewed.
* * `promotion-offered`: Promotion is offered to a user.
* * `promotion-not-offered`: Promotion is not offered to a user.
* * `purchase-complete`: User finishing a purchase.
* * `search`: Product search.
* * `shopping-cart-page-view`: User viewing a shopping cart.
*
*
* string event_type = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @return This builder for chaining.
*/
public Builder clearEventType() {
eventType_ = getDefaultInstance().getEventType();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* Required. User event type. Allowed values are:
*
* * `add-to-cart`: Products being added to cart.
* * `remove-from-cart`: Products being removed from cart.
* * `category-page-view`: Special pages such as sale or promotion pages
* viewed.
* * `detail-page-view`: Products detail page viewed.
* * `home-page-view`: Homepage viewed.
* * `promotion-offered`: Promotion is offered to a user.
* * `promotion-not-offered`: Promotion is not offered to a user.
* * `purchase-complete`: User finishing a purchase.
* * `search`: Product search.
* * `shopping-cart-page-view`: User viewing a shopping cart.
*
*
* string event_type = 1 [(.google.api.field_behavior) = REQUIRED];
*
* @param value The bytes for eventType to set.
* @return This builder for chaining.
*/
public Builder setEventTypeBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
eventType_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object visitorId_ = "";
/**
*
*
*
* Required. A unique identifier for tracking visitors.
*
* For example, this could be implemented with an HTTP cookie, which should be
* able to uniquely identify a visitor on a single device. This unique
* identifier should not change if the visitor log in/out of the website.
*
* Don't set the field to the same fixed ID for different users. This mixes
* the event history of those users together, which results in degraded model
* quality.
*
* The field must be a UTF-8 encoded string with a length limit of 128
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* The field should not contain PII or user-data. We recommend to use Google
* Analytics [Client
* ID](https://developers.google.com/analytics/devguides/collection/analyticsjs/field-reference#clientId)
* for this field.
*
*
* string visitor_id = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return The visitorId.
*/
public java.lang.String getVisitorId() {
java.lang.Object ref = visitorId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
visitorId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Required. A unique identifier for tracking visitors.
*
* For example, this could be implemented with an HTTP cookie, which should be
* able to uniquely identify a visitor on a single device. This unique
* identifier should not change if the visitor log in/out of the website.
*
* Don't set the field to the same fixed ID for different users. This mixes
* the event history of those users together, which results in degraded model
* quality.
*
* The field must be a UTF-8 encoded string with a length limit of 128
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* The field should not contain PII or user-data. We recommend to use Google
* Analytics [Client
* ID](https://developers.google.com/analytics/devguides/collection/analyticsjs/field-reference#clientId)
* for this field.
*
*
* string visitor_id = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return The bytes for visitorId.
*/
public com.google.protobuf.ByteString getVisitorIdBytes() {
java.lang.Object ref = visitorId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
visitorId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Required. A unique identifier for tracking visitors.
*
* For example, this could be implemented with an HTTP cookie, which should be
* able to uniquely identify a visitor on a single device. This unique
* identifier should not change if the visitor log in/out of the website.
*
* Don't set the field to the same fixed ID for different users. This mixes
* the event history of those users together, which results in degraded model
* quality.
*
* The field must be a UTF-8 encoded string with a length limit of 128
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* The field should not contain PII or user-data. We recommend to use Google
* Analytics [Client
* ID](https://developers.google.com/analytics/devguides/collection/analyticsjs/field-reference#clientId)
* for this field.
*
*
* string visitor_id = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @param value The visitorId to set.
* @return This builder for chaining.
*/
public Builder setVisitorId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
visitorId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Required. A unique identifier for tracking visitors.
*
* For example, this could be implemented with an HTTP cookie, which should be
* able to uniquely identify a visitor on a single device. This unique
* identifier should not change if the visitor log in/out of the website.
*
* Don't set the field to the same fixed ID for different users. This mixes
* the event history of those users together, which results in degraded model
* quality.
*
* The field must be a UTF-8 encoded string with a length limit of 128
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* The field should not contain PII or user-data. We recommend to use Google
* Analytics [Client
* ID](https://developers.google.com/analytics/devguides/collection/analyticsjs/field-reference#clientId)
* for this field.
*
*
* string visitor_id = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @return This builder for chaining.
*/
public Builder clearVisitorId() {
visitorId_ = getDefaultInstance().getVisitorId();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* Required. A unique identifier for tracking visitors.
*
* For example, this could be implemented with an HTTP cookie, which should be
* able to uniquely identify a visitor on a single device. This unique
* identifier should not change if the visitor log in/out of the website.
*
* Don't set the field to the same fixed ID for different users. This mixes
* the event history of those users together, which results in degraded model
* quality.
*
* The field must be a UTF-8 encoded string with a length limit of 128
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* The field should not contain PII or user-data. We recommend to use Google
* Analytics [Client
* ID](https://developers.google.com/analytics/devguides/collection/analyticsjs/field-reference#clientId)
* for this field.
*
*
* string visitor_id = 2 [(.google.api.field_behavior) = REQUIRED];
*
* @param value The bytes for visitorId to set.
* @return This builder for chaining.
*/
public Builder setVisitorIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
visitorId_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object sessionId_ = "";
/**
*
*
*
* A unique identifier for tracking a visitor session with a length limit of
* 128 bytes. A session is an aggregation of an end user behavior in a time
* span.
*
* A general guideline to populate the sesion_id:
* 1. If user has no activity for 30 min, a new session_id should be assigned.
* 2. The session_id should be unique across users, suggest use uuid or add
* visitor_id as prefix.
*
*
* string session_id = 21;
*
* @return The sessionId.
*/
public java.lang.String getSessionId() {
java.lang.Object ref = sessionId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
sessionId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* A unique identifier for tracking a visitor session with a length limit of
* 128 bytes. A session is an aggregation of an end user behavior in a time
* span.
*
* A general guideline to populate the sesion_id:
* 1. If user has no activity for 30 min, a new session_id should be assigned.
* 2. The session_id should be unique across users, suggest use uuid or add
* visitor_id as prefix.
*
*
* string session_id = 21;
*
* @return The bytes for sessionId.
*/
public com.google.protobuf.ByteString getSessionIdBytes() {
java.lang.Object ref = sessionId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
sessionId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* A unique identifier for tracking a visitor session with a length limit of
* 128 bytes. A session is an aggregation of an end user behavior in a time
* span.
*
* A general guideline to populate the sesion_id:
* 1. If user has no activity for 30 min, a new session_id should be assigned.
* 2. The session_id should be unique across users, suggest use uuid or add
* visitor_id as prefix.
*
*
* string session_id = 21;
*
* @param value The sessionId to set.
* @return This builder for chaining.
*/
public Builder setSessionId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
sessionId_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* A unique identifier for tracking a visitor session with a length limit of
* 128 bytes. A session is an aggregation of an end user behavior in a time
* span.
*
* A general guideline to populate the sesion_id:
* 1. If user has no activity for 30 min, a new session_id should be assigned.
* 2. The session_id should be unique across users, suggest use uuid or add
* visitor_id as prefix.
*
*
* string session_id = 21;
*
* @return This builder for chaining.
*/
public Builder clearSessionId() {
sessionId_ = getDefaultInstance().getSessionId();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* A unique identifier for tracking a visitor session with a length limit of
* 128 bytes. A session is an aggregation of an end user behavior in a time
* span.
*
* A general guideline to populate the sesion_id:
* 1. If user has no activity for 30 min, a new session_id should be assigned.
* 2. The session_id should be unique across users, suggest use uuid or add
* visitor_id as prefix.
*
*
* string session_id = 21;
*
* @param value The bytes for sessionId to set.
* @return This builder for chaining.
*/
public Builder setSessionIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
sessionId_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private com.google.protobuf.Timestamp eventTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
eventTimeBuilder_;
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*
* @return Whether the eventTime field is set.
*/
public boolean hasEventTime() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*
* @return The eventTime.
*/
public com.google.protobuf.Timestamp getEventTime() {
if (eventTimeBuilder_ == null) {
return eventTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : eventTime_;
} else {
return eventTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*/
public Builder setEventTime(com.google.protobuf.Timestamp value) {
if (eventTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
eventTime_ = value;
} else {
eventTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*/
public Builder setEventTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (eventTimeBuilder_ == null) {
eventTime_ = builderForValue.build();
} else {
eventTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*/
public Builder mergeEventTime(com.google.protobuf.Timestamp value) {
if (eventTimeBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)
&& eventTime_ != null
&& eventTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getEventTimeBuilder().mergeFrom(value);
} else {
eventTime_ = value;
}
} else {
eventTimeBuilder_.mergeFrom(value);
}
if (eventTime_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*/
public Builder clearEventTime() {
bitField0_ = (bitField0_ & ~0x00000008);
eventTime_ = null;
if (eventTimeBuilder_ != null) {
eventTimeBuilder_.dispose();
eventTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*/
public com.google.protobuf.Timestamp.Builder getEventTimeBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getEventTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*/
public com.google.protobuf.TimestampOrBuilder getEventTimeOrBuilder() {
if (eventTimeBuilder_ != null) {
return eventTimeBuilder_.getMessageOrBuilder();
} else {
return eventTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : eventTime_;
}
}
/**
*
*
*
* Only required for
* [UserEventService.ImportUserEvents][google.cloud.retail.v2alpha.UserEventService.ImportUserEvents]
* method. Timestamp of when the user event happened.
*
*
* .google.protobuf.Timestamp event_time = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getEventTimeFieldBuilder() {
if (eventTimeBuilder_ == null) {
eventTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getEventTime(), getParentForChildren(), isClean());
eventTime_ = null;
}
return eventTimeBuilder_;
}
private com.google.protobuf.LazyStringArrayList experimentIds_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureExperimentIdsIsMutable() {
if (!experimentIds_.isModifiable()) {
experimentIds_ = new com.google.protobuf.LazyStringArrayList(experimentIds_);
}
bitField0_ |= 0x00000010;
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @return A list containing the experimentIds.
*/
public com.google.protobuf.ProtocolStringList getExperimentIdsList() {
experimentIds_.makeImmutable();
return experimentIds_;
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @return The count of experimentIds.
*/
public int getExperimentIdsCount() {
return experimentIds_.size();
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @param index The index of the element to return.
* @return The experimentIds at the given index.
*/
public java.lang.String getExperimentIds(int index) {
return experimentIds_.get(index);
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @param index The index of the value to return.
* @return The bytes of the experimentIds at the given index.
*/
public com.google.protobuf.ByteString getExperimentIdsBytes(int index) {
return experimentIds_.getByteString(index);
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @param index The index to set the value at.
* @param value The experimentIds to set.
* @return This builder for chaining.
*/
public Builder setExperimentIds(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureExperimentIdsIsMutable();
experimentIds_.set(index, value);
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @param value The experimentIds to add.
* @return This builder for chaining.
*/
public Builder addExperimentIds(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureExperimentIdsIsMutable();
experimentIds_.add(value);
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @param values The experimentIds to add.
* @return This builder for chaining.
*/
public Builder addAllExperimentIds(java.lang.Iterable values) {
ensureExperimentIdsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, experimentIds_);
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @return This builder for chaining.
*/
public Builder clearExperimentIds() {
experimentIds_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
;
onChanged();
return this;
}
/**
*
*
*
* A list of identifiers for the independent experiment groups this user event
* belongs to. This is used to distinguish between user events associated with
* different experiment setups (e.g. using Retail API, using different
* recommendation models).
*
*
* repeated string experiment_ids = 4;
*
* @param value The bytes of the experimentIds to add.
* @return This builder for chaining.
*/
public Builder addExperimentIdsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureExperimentIdsIsMutable();
experimentIds_.add(value);
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private java.lang.Object attributionToken_ = "";
/**
*
*
*
* Highly recommended for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* This field enables accurate attribution of recommendation model
* performance.
*
* The value must be a valid
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* The value must be a valid
* [SearchResponse.attribution_token][google.cloud.retail.v2alpha.SearchResponse.attribution_token]
* for user events that are the result of
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search].
*
* This token enables us to accurately attribute page view or purchase back to
* the event and the particular predict response containing this
* clicked/purchased product. If user clicks on product K in the
* recommendation results, pass
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* as a URL parameter to product K's page. When recording events on product
* K's page, log the
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* to this field.
*
*
* string attribution_token = 5;
*
* @return The attributionToken.
*/
public java.lang.String getAttributionToken() {
java.lang.Object ref = attributionToken_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
attributionToken_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Highly recommended for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* This field enables accurate attribution of recommendation model
* performance.
*
* The value must be a valid
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* The value must be a valid
* [SearchResponse.attribution_token][google.cloud.retail.v2alpha.SearchResponse.attribution_token]
* for user events that are the result of
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search].
*
* This token enables us to accurately attribute page view or purchase back to
* the event and the particular predict response containing this
* clicked/purchased product. If user clicks on product K in the
* recommendation results, pass
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* as a URL parameter to product K's page. When recording events on product
* K's page, log the
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* to this field.
*
*
* string attribution_token = 5;
*
* @return The bytes for attributionToken.
*/
public com.google.protobuf.ByteString getAttributionTokenBytes() {
java.lang.Object ref = attributionToken_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
attributionToken_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Highly recommended for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* This field enables accurate attribution of recommendation model
* performance.
*
* The value must be a valid
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* The value must be a valid
* [SearchResponse.attribution_token][google.cloud.retail.v2alpha.SearchResponse.attribution_token]
* for user events that are the result of
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search].
*
* This token enables us to accurately attribute page view or purchase back to
* the event and the particular predict response containing this
* clicked/purchased product. If user clicks on product K in the
* recommendation results, pass
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* as a URL parameter to product K's page. When recording events on product
* K's page, log the
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* to this field.
*
*
* string attribution_token = 5;
*
* @param value The attributionToken to set.
* @return This builder for chaining.
*/
public Builder setAttributionToken(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
attributionToken_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Highly recommended for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* This field enables accurate attribution of recommendation model
* performance.
*
* The value must be a valid
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* The value must be a valid
* [SearchResponse.attribution_token][google.cloud.retail.v2alpha.SearchResponse.attribution_token]
* for user events that are the result of
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search].
*
* This token enables us to accurately attribute page view or purchase back to
* the event and the particular predict response containing this
* clicked/purchased product. If user clicks on product K in the
* recommendation results, pass
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* as a URL parameter to product K's page. When recording events on product
* K's page, log the
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* to this field.
*
*
* string attribution_token = 5;
*
* @return This builder for chaining.
*/
public Builder clearAttributionToken() {
attributionToken_ = getDefaultInstance().getAttributionToken();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
*
*
* Highly recommended for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* This field enables accurate attribution of recommendation model
* performance.
*
* The value must be a valid
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* for user events that are the result of
* [PredictionService.Predict][google.cloud.retail.v2alpha.PredictionService.Predict].
* The value must be a valid
* [SearchResponse.attribution_token][google.cloud.retail.v2alpha.SearchResponse.attribution_token]
* for user events that are the result of
* [SearchService.Search][google.cloud.retail.v2alpha.SearchService.Search].
*
* This token enables us to accurately attribute page view or purchase back to
* the event and the particular predict response containing this
* clicked/purchased product. If user clicks on product K in the
* recommendation results, pass
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* as a URL parameter to product K's page. When recording events on product
* K's page, log the
* [PredictResponse.attribution_token][google.cloud.retail.v2alpha.PredictResponse.attribution_token]
* to this field.
*
*
* string attribution_token = 5;
*
* @param value The bytes for attributionToken to set.
* @return This builder for chaining.
*/
public Builder setAttributionTokenBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
attributionToken_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.util.List productDetails_ =
java.util.Collections.emptyList();
private void ensureProductDetailsIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
productDetails_ =
new java.util.ArrayList(productDetails_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.retail.v2alpha.ProductDetail,
com.google.cloud.retail.v2alpha.ProductDetail.Builder,
com.google.cloud.retail.v2alpha.ProductDetailOrBuilder>
productDetailsBuilder_;
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public java.util.List getProductDetailsList() {
if (productDetailsBuilder_ == null) {
return java.util.Collections.unmodifiableList(productDetails_);
} else {
return productDetailsBuilder_.getMessageList();
}
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public int getProductDetailsCount() {
if (productDetailsBuilder_ == null) {
return productDetails_.size();
} else {
return productDetailsBuilder_.getCount();
}
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public com.google.cloud.retail.v2alpha.ProductDetail getProductDetails(int index) {
if (productDetailsBuilder_ == null) {
return productDetails_.get(index);
} else {
return productDetailsBuilder_.getMessage(index);
}
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public Builder setProductDetails(
int index, com.google.cloud.retail.v2alpha.ProductDetail value) {
if (productDetailsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureProductDetailsIsMutable();
productDetails_.set(index, value);
onChanged();
} else {
productDetailsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public Builder setProductDetails(
int index, com.google.cloud.retail.v2alpha.ProductDetail.Builder builderForValue) {
if (productDetailsBuilder_ == null) {
ensureProductDetailsIsMutable();
productDetails_.set(index, builderForValue.build());
onChanged();
} else {
productDetailsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public Builder addProductDetails(com.google.cloud.retail.v2alpha.ProductDetail value) {
if (productDetailsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureProductDetailsIsMutable();
productDetails_.add(value);
onChanged();
} else {
productDetailsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public Builder addProductDetails(
int index, com.google.cloud.retail.v2alpha.ProductDetail value) {
if (productDetailsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureProductDetailsIsMutable();
productDetails_.add(index, value);
onChanged();
} else {
productDetailsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public Builder addProductDetails(
com.google.cloud.retail.v2alpha.ProductDetail.Builder builderForValue) {
if (productDetailsBuilder_ == null) {
ensureProductDetailsIsMutable();
productDetails_.add(builderForValue.build());
onChanged();
} else {
productDetailsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public Builder addProductDetails(
int index, com.google.cloud.retail.v2alpha.ProductDetail.Builder builderForValue) {
if (productDetailsBuilder_ == null) {
ensureProductDetailsIsMutable();
productDetails_.add(index, builderForValue.build());
onChanged();
} else {
productDetailsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public Builder addAllProductDetails(
java.lang.Iterable extends com.google.cloud.retail.v2alpha.ProductDetail> values) {
if (productDetailsBuilder_ == null) {
ensureProductDetailsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, productDetails_);
onChanged();
} else {
productDetailsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public Builder clearProductDetails() {
if (productDetailsBuilder_ == null) {
productDetails_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
productDetailsBuilder_.clear();
}
return this;
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public Builder removeProductDetails(int index) {
if (productDetailsBuilder_ == null) {
ensureProductDetailsIsMutable();
productDetails_.remove(index);
onChanged();
} else {
productDetailsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public com.google.cloud.retail.v2alpha.ProductDetail.Builder getProductDetailsBuilder(
int index) {
return getProductDetailsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public com.google.cloud.retail.v2alpha.ProductDetailOrBuilder getProductDetailsOrBuilder(
int index) {
if (productDetailsBuilder_ == null) {
return productDetails_.get(index);
} else {
return productDetailsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public java.util.List extends com.google.cloud.retail.v2alpha.ProductDetailOrBuilder>
getProductDetailsOrBuilderList() {
if (productDetailsBuilder_ != null) {
return productDetailsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(productDetails_);
}
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public com.google.cloud.retail.v2alpha.ProductDetail.Builder addProductDetailsBuilder() {
return getProductDetailsFieldBuilder()
.addBuilder(com.google.cloud.retail.v2alpha.ProductDetail.getDefaultInstance());
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public com.google.cloud.retail.v2alpha.ProductDetail.Builder addProductDetailsBuilder(
int index) {
return getProductDetailsFieldBuilder()
.addBuilder(index, com.google.cloud.retail.v2alpha.ProductDetail.getDefaultInstance());
}
/**
*
*
*
* The main product details related to the event.
*
* This field is optional except for the following event types:
*
* * `add-to-cart`
* * `detail-page-view`
* * `purchase-complete`
*
* In a `search` event, this field represents the products returned to the end
* user on the current page (the end user may have not finished browsing the
* whole page yet). When a new page is returned to the end user, after
* pagination/filtering/ordering even for the same query, a new `search` event
* with different
* [product_details][google.cloud.retail.v2alpha.UserEvent.product_details] is
* desired. The end user may have not finished browsing the whole page yet.
*
*
* repeated .google.cloud.retail.v2alpha.ProductDetail product_details = 6;
*/
public java.util.List
getProductDetailsBuilderList() {
return getProductDetailsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.retail.v2alpha.ProductDetail,
com.google.cloud.retail.v2alpha.ProductDetail.Builder,
com.google.cloud.retail.v2alpha.ProductDetailOrBuilder>
getProductDetailsFieldBuilder() {
if (productDetailsBuilder_ == null) {
productDetailsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.retail.v2alpha.ProductDetail,
com.google.cloud.retail.v2alpha.ProductDetail.Builder,
com.google.cloud.retail.v2alpha.ProductDetailOrBuilder>(
productDetails_,
((bitField0_ & 0x00000040) != 0),
getParentForChildren(),
isClean());
productDetails_ = null;
}
return productDetailsBuilder_;
}
private com.google.cloud.retail.v2alpha.CompletionDetail completionDetail_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.CompletionDetail,
com.google.cloud.retail.v2alpha.CompletionDetail.Builder,
com.google.cloud.retail.v2alpha.CompletionDetailOrBuilder>
completionDetailBuilder_;
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*
* @return Whether the completionDetail field is set.
*/
public boolean hasCompletionDetail() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*
* @return The completionDetail.
*/
public com.google.cloud.retail.v2alpha.CompletionDetail getCompletionDetail() {
if (completionDetailBuilder_ == null) {
return completionDetail_ == null
? com.google.cloud.retail.v2alpha.CompletionDetail.getDefaultInstance()
: completionDetail_;
} else {
return completionDetailBuilder_.getMessage();
}
}
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*/
public Builder setCompletionDetail(com.google.cloud.retail.v2alpha.CompletionDetail value) {
if (completionDetailBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
completionDetail_ = value;
} else {
completionDetailBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*/
public Builder setCompletionDetail(
com.google.cloud.retail.v2alpha.CompletionDetail.Builder builderForValue) {
if (completionDetailBuilder_ == null) {
completionDetail_ = builderForValue.build();
} else {
completionDetailBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*/
public Builder mergeCompletionDetail(com.google.cloud.retail.v2alpha.CompletionDetail value) {
if (completionDetailBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)
&& completionDetail_ != null
&& completionDetail_
!= com.google.cloud.retail.v2alpha.CompletionDetail.getDefaultInstance()) {
getCompletionDetailBuilder().mergeFrom(value);
} else {
completionDetail_ = value;
}
} else {
completionDetailBuilder_.mergeFrom(value);
}
if (completionDetail_ != null) {
bitField0_ |= 0x00000080;
onChanged();
}
return this;
}
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*/
public Builder clearCompletionDetail() {
bitField0_ = (bitField0_ & ~0x00000080);
completionDetail_ = null;
if (completionDetailBuilder_ != null) {
completionDetailBuilder_.dispose();
completionDetailBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*/
public com.google.cloud.retail.v2alpha.CompletionDetail.Builder getCompletionDetailBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getCompletionDetailFieldBuilder().getBuilder();
}
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*/
public com.google.cloud.retail.v2alpha.CompletionDetailOrBuilder
getCompletionDetailOrBuilder() {
if (completionDetailBuilder_ != null) {
return completionDetailBuilder_.getMessageOrBuilder();
} else {
return completionDetail_ == null
? com.google.cloud.retail.v2alpha.CompletionDetail.getDefaultInstance()
: completionDetail_;
}
}
/**
*
*
*
* The main auto-completion details related to the event.
*
* This field should be set for `search` event when autocomplete function is
* enabled and the user clicks a suggestion for search.
*
*
* .google.cloud.retail.v2alpha.CompletionDetail completion_detail = 22;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.CompletionDetail,
com.google.cloud.retail.v2alpha.CompletionDetail.Builder,
com.google.cloud.retail.v2alpha.CompletionDetailOrBuilder>
getCompletionDetailFieldBuilder() {
if (completionDetailBuilder_ == null) {
completionDetailBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.CompletionDetail,
com.google.cloud.retail.v2alpha.CompletionDetail.Builder,
com.google.cloud.retail.v2alpha.CompletionDetailOrBuilder>(
getCompletionDetail(), getParentForChildren(), isClean());
completionDetail_ = null;
}
return completionDetailBuilder_;
}
private static final class AttributesConverter
implements com.google.protobuf.MapFieldBuilder.Converter<
java.lang.String,
com.google.cloud.retail.v2alpha.CustomAttributeOrBuilder,
com.google.cloud.retail.v2alpha.CustomAttribute> {
@java.lang.Override
public com.google.cloud.retail.v2alpha.CustomAttribute build(
com.google.cloud.retail.v2alpha.CustomAttributeOrBuilder val) {
if (val instanceof com.google.cloud.retail.v2alpha.CustomAttribute) {
return (com.google.cloud.retail.v2alpha.CustomAttribute) val;
}
return ((com.google.cloud.retail.v2alpha.CustomAttribute.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.retail.v2alpha.CustomAttribute>
defaultEntry() {
return AttributesDefaultEntryHolder.defaultEntry;
}
};
private static final AttributesConverter attributesConverter = new AttributesConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.retail.v2alpha.CustomAttributeOrBuilder,
com.google.cloud.retail.v2alpha.CustomAttribute,
com.google.cloud.retail.v2alpha.CustomAttribute.Builder>
attributes_;
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.retail.v2alpha.CustomAttributeOrBuilder,
com.google.cloud.retail.v2alpha.CustomAttribute,
com.google.cloud.retail.v2alpha.CustomAttribute.Builder>
internalGetAttributes() {
if (attributes_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(attributesConverter);
}
return attributes_;
}
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.retail.v2alpha.CustomAttributeOrBuilder,
com.google.cloud.retail.v2alpha.CustomAttribute,
com.google.cloud.retail.v2alpha.CustomAttribute.Builder>
internalGetMutableAttributes() {
if (attributes_ == null) {
attributes_ = new com.google.protobuf.MapFieldBuilder<>(attributesConverter);
}
bitField0_ |= 0x00000100;
onChanged();
return attributes_;
}
public int getAttributesCount() {
return internalGetAttributes().ensureBuilderMap().size();
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
@java.lang.Override
public boolean containsAttributes(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetAttributes().ensureBuilderMap().containsKey(key);
}
/** Use {@link #getAttributesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getAttributes() {
return getAttributesMap();
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
@java.lang.Override
public java.util.Map
getAttributesMap() {
return internalGetAttributes().getImmutableMap();
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
@java.lang.Override
public /* nullable */ com.google.cloud.retail.v2alpha.CustomAttribute getAttributesOrDefault(
java.lang.String key,
/* nullable */
com.google.cloud.retail.v2alpha.CustomAttribute defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetMutableAttributes().ensureBuilderMap();
return map.containsKey(key) ? attributesConverter.build(map.get(key)) : defaultValue;
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
@java.lang.Override
public com.google.cloud.retail.v2alpha.CustomAttribute getAttributesOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetMutableAttributes().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return attributesConverter.build(map.get(key));
}
public Builder clearAttributes() {
bitField0_ = (bitField0_ & ~0x00000100);
internalGetMutableAttributes().clear();
return this;
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
public Builder removeAttributes(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableAttributes().ensureBuilderMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map
getMutableAttributes() {
bitField0_ |= 0x00000100;
return internalGetMutableAttributes().ensureMessageMap();
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
public Builder putAttributes(
java.lang.String key, com.google.cloud.retail.v2alpha.CustomAttribute value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableAttributes().ensureBuilderMap().put(key, value);
bitField0_ |= 0x00000100;
return this;
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
public Builder putAllAttributes(
java.util.Map values) {
for (java.util.Map.Entry
e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableAttributes().ensureBuilderMap().putAll(values);
bitField0_ |= 0x00000100;
return this;
}
/**
*
*
*
* Extra user event features to include in the recommendation model.
*
* If you provide custom attributes for ingested user events, also include
* them in the user events that you associate with prediction requests. Custom
* attribute formatting must be consistent between imported events and events
* provided with prediction requests. This lets the Retail API use
* those custom attributes when training models and serving predictions, which
* helps improve recommendation quality.
*
* This field needs to pass all below criteria, otherwise an INVALID_ARGUMENT
* error is returned:
*
* * The key must be a UTF-8 encoded string with a length limit of 5,000
* characters.
* * For text attributes, at most 400 values are allowed. Empty values are not
* allowed. Each value must be a UTF-8 encoded string with a length limit of
* 256 characters.
* * For number attributes, at most 400 values are allowed.
*
* For product recommendations, an example of extra user information is
* traffic_channel, which is how a user arrives at the site. Users can arrive
* at the site by coming to the site directly, coming through Google
* search, or in other ways.
*
*
* map<string, .google.cloud.retail.v2alpha.CustomAttribute> attributes = 7;
*/
public com.google.cloud.retail.v2alpha.CustomAttribute.Builder putAttributesBuilderIfAbsent(
java.lang.String key) {
java.util.Map
builderMap = internalGetMutableAttributes().ensureBuilderMap();
com.google.cloud.retail.v2alpha.CustomAttributeOrBuilder entry = builderMap.get(key);
if (entry == null) {
entry = com.google.cloud.retail.v2alpha.CustomAttribute.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.cloud.retail.v2alpha.CustomAttribute) {
entry = ((com.google.cloud.retail.v2alpha.CustomAttribute) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.cloud.retail.v2alpha.CustomAttribute.Builder) entry;
}
private java.lang.Object cartId_ = "";
/**
*
*
*
* The ID or name of the associated shopping cart. This ID is used
* to associate multiple items added or present in the cart before purchase.
*
* This can only be set for `add-to-cart`, `purchase-complete`, or
* `shopping-cart-page-view` events.
*
*
* string cart_id = 8;
*
* @return The cartId.
*/
public java.lang.String getCartId() {
java.lang.Object ref = cartId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cartId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The ID or name of the associated shopping cart. This ID is used
* to associate multiple items added or present in the cart before purchase.
*
* This can only be set for `add-to-cart`, `purchase-complete`, or
* `shopping-cart-page-view` events.
*
*
* string cart_id = 8;
*
* @return The bytes for cartId.
*/
public com.google.protobuf.ByteString getCartIdBytes() {
java.lang.Object ref = cartId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
cartId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The ID or name of the associated shopping cart. This ID is used
* to associate multiple items added or present in the cart before purchase.
*
* This can only be set for `add-to-cart`, `purchase-complete`, or
* `shopping-cart-page-view` events.
*
*
* string cart_id = 8;
*
* @param value The cartId to set.
* @return This builder for chaining.
*/
public Builder setCartId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
cartId_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* The ID or name of the associated shopping cart. This ID is used
* to associate multiple items added or present in the cart before purchase.
*
* This can only be set for `add-to-cart`, `purchase-complete`, or
* `shopping-cart-page-view` events.
*
*
* string cart_id = 8;
*
* @return This builder for chaining.
*/
public Builder clearCartId() {
cartId_ = getDefaultInstance().getCartId();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
*
*
*
* The ID or name of the associated shopping cart. This ID is used
* to associate multiple items added or present in the cart before purchase.
*
* This can only be set for `add-to-cart`, `purchase-complete`, or
* `shopping-cart-page-view` events.
*
*
* string cart_id = 8;
*
* @param value The bytes for cartId to set.
* @return This builder for chaining.
*/
public Builder setCartIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
cartId_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
private com.google.cloud.retail.v2alpha.PurchaseTransaction purchaseTransaction_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.PurchaseTransaction,
com.google.cloud.retail.v2alpha.PurchaseTransaction.Builder,
com.google.cloud.retail.v2alpha.PurchaseTransactionOrBuilder>
purchaseTransactionBuilder_;
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*
* @return Whether the purchaseTransaction field is set.
*/
public boolean hasPurchaseTransaction() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*
* @return The purchaseTransaction.
*/
public com.google.cloud.retail.v2alpha.PurchaseTransaction getPurchaseTransaction() {
if (purchaseTransactionBuilder_ == null) {
return purchaseTransaction_ == null
? com.google.cloud.retail.v2alpha.PurchaseTransaction.getDefaultInstance()
: purchaseTransaction_;
} else {
return purchaseTransactionBuilder_.getMessage();
}
}
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*/
public Builder setPurchaseTransaction(
com.google.cloud.retail.v2alpha.PurchaseTransaction value) {
if (purchaseTransactionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
purchaseTransaction_ = value;
} else {
purchaseTransactionBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*/
public Builder setPurchaseTransaction(
com.google.cloud.retail.v2alpha.PurchaseTransaction.Builder builderForValue) {
if (purchaseTransactionBuilder_ == null) {
purchaseTransaction_ = builderForValue.build();
} else {
purchaseTransactionBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*/
public Builder mergePurchaseTransaction(
com.google.cloud.retail.v2alpha.PurchaseTransaction value) {
if (purchaseTransactionBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0)
&& purchaseTransaction_ != null
&& purchaseTransaction_
!= com.google.cloud.retail.v2alpha.PurchaseTransaction.getDefaultInstance()) {
getPurchaseTransactionBuilder().mergeFrom(value);
} else {
purchaseTransaction_ = value;
}
} else {
purchaseTransactionBuilder_.mergeFrom(value);
}
if (purchaseTransaction_ != null) {
bitField0_ |= 0x00000400;
onChanged();
}
return this;
}
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*/
public Builder clearPurchaseTransaction() {
bitField0_ = (bitField0_ & ~0x00000400);
purchaseTransaction_ = null;
if (purchaseTransactionBuilder_ != null) {
purchaseTransactionBuilder_.dispose();
purchaseTransactionBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*/
public com.google.cloud.retail.v2alpha.PurchaseTransaction.Builder
getPurchaseTransactionBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getPurchaseTransactionFieldBuilder().getBuilder();
}
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*/
public com.google.cloud.retail.v2alpha.PurchaseTransactionOrBuilder
getPurchaseTransactionOrBuilder() {
if (purchaseTransactionBuilder_ != null) {
return purchaseTransactionBuilder_.getMessageOrBuilder();
} else {
return purchaseTransaction_ == null
? com.google.cloud.retail.v2alpha.PurchaseTransaction.getDefaultInstance()
: purchaseTransaction_;
}
}
/**
*
*
*
* A transaction represents the entire purchase transaction.
*
* Required for `purchase-complete` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* .google.cloud.retail.v2alpha.PurchaseTransaction purchase_transaction = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.PurchaseTransaction,
com.google.cloud.retail.v2alpha.PurchaseTransaction.Builder,
com.google.cloud.retail.v2alpha.PurchaseTransactionOrBuilder>
getPurchaseTransactionFieldBuilder() {
if (purchaseTransactionBuilder_ == null) {
purchaseTransactionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.PurchaseTransaction,
com.google.cloud.retail.v2alpha.PurchaseTransaction.Builder,
com.google.cloud.retail.v2alpha.PurchaseTransactionOrBuilder>(
getPurchaseTransaction(), getParentForChildren(), isClean());
purchaseTransaction_ = null;
}
return purchaseTransactionBuilder_;
}
private java.lang.Object searchQuery_ = "";
/**
*
*
*
* The user's search query.
*
* See [SearchRequest.query][google.cloud.retail.v2alpha.SearchRequest.query]
* for definition.
*
* The value must be a UTF-8 encoded string with a length limit of 5,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string search_query = 10;
*
* @return The searchQuery.
*/
public java.lang.String getSearchQuery() {
java.lang.Object ref = searchQuery_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
searchQuery_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The user's search query.
*
* See [SearchRequest.query][google.cloud.retail.v2alpha.SearchRequest.query]
* for definition.
*
* The value must be a UTF-8 encoded string with a length limit of 5,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string search_query = 10;
*
* @return The bytes for searchQuery.
*/
public com.google.protobuf.ByteString getSearchQueryBytes() {
java.lang.Object ref = searchQuery_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
searchQuery_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The user's search query.
*
* See [SearchRequest.query][google.cloud.retail.v2alpha.SearchRequest.query]
* for definition.
*
* The value must be a UTF-8 encoded string with a length limit of 5,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string search_query = 10;
*
* @param value The searchQuery to set.
* @return This builder for chaining.
*/
public Builder setSearchQuery(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
searchQuery_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* The user's search query.
*
* See [SearchRequest.query][google.cloud.retail.v2alpha.SearchRequest.query]
* for definition.
*
* The value must be a UTF-8 encoded string with a length limit of 5,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string search_query = 10;
*
* @return This builder for chaining.
*/
public Builder clearSearchQuery() {
searchQuery_ = getDefaultInstance().getSearchQuery();
bitField0_ = (bitField0_ & ~0x00000800);
onChanged();
return this;
}
/**
*
*
*
* The user's search query.
*
* See [SearchRequest.query][google.cloud.retail.v2alpha.SearchRequest.query]
* for definition.
*
* The value must be a UTF-8 encoded string with a length limit of 5,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string search_query = 10;
*
* @param value The bytes for searchQuery to set.
* @return This builder for chaining.
*/
public Builder setSearchQueryBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
searchQuery_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
private java.lang.Object filter_ = "";
/**
*
*
*
* The filter syntax consists of an expression language for constructing a
* predicate from one or more fields of the products being filtered.
*
* See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string filter = 16;
*
* @return The filter.
*/
public java.lang.String getFilter() {
java.lang.Object ref = filter_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
filter_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The filter syntax consists of an expression language for constructing a
* predicate from one or more fields of the products being filtered.
*
* See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string filter = 16;
*
* @return The bytes for filter.
*/
public com.google.protobuf.ByteString getFilterBytes() {
java.lang.Object ref = filter_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
filter_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The filter syntax consists of an expression language for constructing a
* predicate from one or more fields of the products being filtered.
*
* See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string filter = 16;
*
* @param value The filter to set.
* @return This builder for chaining.
*/
public Builder setFilter(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
filter_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* The filter syntax consists of an expression language for constructing a
* predicate from one or more fields of the products being filtered.
*
* See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string filter = 16;
*
* @return This builder for chaining.
*/
public Builder clearFilter() {
filter_ = getDefaultInstance().getFilter();
bitField0_ = (bitField0_ & ~0x00001000);
onChanged();
return this;
}
/**
*
*
*
* The filter syntax consists of an expression language for constructing a
* predicate from one or more fields of the products being filtered.
*
* See
* [SearchRequest.filter][google.cloud.retail.v2alpha.SearchRequest.filter]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string filter = 16;
*
* @param value The bytes for filter to set.
* @return This builder for chaining.
*/
public Builder setFilterBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
filter_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
private java.lang.Object orderBy_ = "";
/**
*
*
*
* The order in which products are returned.
*
* See
* [SearchRequest.order_by][google.cloud.retail.v2alpha.SearchRequest.order_by]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* This can only be set for `search` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string order_by = 17;
*
* @return The orderBy.
*/
public java.lang.String getOrderBy() {
java.lang.Object ref = orderBy_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
orderBy_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The order in which products are returned.
*
* See
* [SearchRequest.order_by][google.cloud.retail.v2alpha.SearchRequest.order_by]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* This can only be set for `search` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string order_by = 17;
*
* @return The bytes for orderBy.
*/
public com.google.protobuf.ByteString getOrderByBytes() {
java.lang.Object ref = orderBy_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
orderBy_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The order in which products are returned.
*
* See
* [SearchRequest.order_by][google.cloud.retail.v2alpha.SearchRequest.order_by]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* This can only be set for `search` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string order_by = 17;
*
* @param value The orderBy to set.
* @return This builder for chaining.
*/
public Builder setOrderBy(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
orderBy_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* The order in which products are returned.
*
* See
* [SearchRequest.order_by][google.cloud.retail.v2alpha.SearchRequest.order_by]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* This can only be set for `search` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string order_by = 17;
*
* @return This builder for chaining.
*/
public Builder clearOrderBy() {
orderBy_ = getDefaultInstance().getOrderBy();
bitField0_ = (bitField0_ & ~0x00002000);
onChanged();
return this;
}
/**
*
*
*
* The order in which products are returned.
*
* See
* [SearchRequest.order_by][google.cloud.retail.v2alpha.SearchRequest.order_by]
* for definition and syntax.
*
* The value must be a UTF-8 encoded string with a length limit of 1,000
* characters. Otherwise, an INVALID_ARGUMENT error is returned.
*
* This can only be set for `search` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* string order_by = 17;
*
* @param value The bytes for orderBy to set.
* @return This builder for chaining.
*/
public Builder setOrderByBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
orderBy_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
private int offset_;
/**
*
*
*
* An integer that specifies the current offset for pagination (the 0-indexed
* starting location, amongst the products deemed by the API as relevant).
*
* See
* [SearchRequest.offset][google.cloud.retail.v2alpha.SearchRequest.offset]
* for definition.
*
* If this field is negative, an INVALID_ARGUMENT is returned.
*
* This can only be set for `search` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* int32 offset = 18;
*
* @return The offset.
*/
@java.lang.Override
public int getOffset() {
return offset_;
}
/**
*
*
*
* An integer that specifies the current offset for pagination (the 0-indexed
* starting location, amongst the products deemed by the API as relevant).
*
* See
* [SearchRequest.offset][google.cloud.retail.v2alpha.SearchRequest.offset]
* for definition.
*
* If this field is negative, an INVALID_ARGUMENT is returned.
*
* This can only be set for `search` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* int32 offset = 18;
*
* @param value The offset to set.
* @return This builder for chaining.
*/
public Builder setOffset(int value) {
offset_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* An integer that specifies the current offset for pagination (the 0-indexed
* starting location, amongst the products deemed by the API as relevant).
*
* See
* [SearchRequest.offset][google.cloud.retail.v2alpha.SearchRequest.offset]
* for definition.
*
* If this field is negative, an INVALID_ARGUMENT is returned.
*
* This can only be set for `search` events. Other event types should not set
* this field. Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* int32 offset = 18;
*
* @return This builder for chaining.
*/
public Builder clearOffset() {
bitField0_ = (bitField0_ & ~0x00004000);
offset_ = 0;
onChanged();
return this;
}
private com.google.protobuf.LazyStringArrayList pageCategories_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensurePageCategoriesIsMutable() {
if (!pageCategories_.isModifiable()) {
pageCategories_ = new com.google.protobuf.LazyStringArrayList(pageCategories_);
}
bitField0_ |= 0x00008000;
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @return A list containing the pageCategories.
*/
public com.google.protobuf.ProtocolStringList getPageCategoriesList() {
pageCategories_.makeImmutable();
return pageCategories_;
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @return The count of pageCategories.
*/
public int getPageCategoriesCount() {
return pageCategories_.size();
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @param index The index of the element to return.
* @return The pageCategories at the given index.
*/
public java.lang.String getPageCategories(int index) {
return pageCategories_.get(index);
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @param index The index of the value to return.
* @return The bytes of the pageCategories at the given index.
*/
public com.google.protobuf.ByteString getPageCategoriesBytes(int index) {
return pageCategories_.getByteString(index);
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @param index The index to set the value at.
* @param value The pageCategories to set.
* @return This builder for chaining.
*/
public Builder setPageCategories(int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensurePageCategoriesIsMutable();
pageCategories_.set(index, value);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @param value The pageCategories to add.
* @return This builder for chaining.
*/
public Builder addPageCategories(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensurePageCategoriesIsMutable();
pageCategories_.add(value);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @param values The pageCategories to add.
* @return This builder for chaining.
*/
public Builder addAllPageCategories(java.lang.Iterable values) {
ensurePageCategoriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, pageCategories_);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @return This builder for chaining.
*/
public Builder clearPageCategories() {
pageCategories_ = com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00008000);
;
onChanged();
return this;
}
/**
*
*
*
* The categories associated with a category page.
*
* To represent full path of category, use '>' sign to separate different
* hierarchies. If '>' is part of the category name, replace it with
* other character(s).
*
* Category pages include special pages such as sales or promotions. For
* instance, a special sale page may have the category hierarchy:
* "pageCategories" : ["Sales > 2017 Black Friday Deals"].
*
* Required for `category-page-view` events. At least one of
* [search_query][google.cloud.retail.v2alpha.UserEvent.search_query] or
* [page_categories][google.cloud.retail.v2alpha.UserEvent.page_categories] is
* required for `search` events. Other event types should not set this field.
* Otherwise, an INVALID_ARGUMENT error is returned.
*
*
* repeated string page_categories = 11;
*
* @param value The bytes of the pageCategories to add.
* @return This builder for chaining.
*/
public Builder addPageCategoriesBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensurePageCategoriesIsMutable();
pageCategories_.add(value);
bitField0_ |= 0x00008000;
onChanged();
return this;
}
private com.google.cloud.retail.v2alpha.UserInfo userInfo_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.UserInfo,
com.google.cloud.retail.v2alpha.UserInfo.Builder,
com.google.cloud.retail.v2alpha.UserInfoOrBuilder>
userInfoBuilder_;
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*
* @return Whether the userInfo field is set.
*/
public boolean hasUserInfo() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*
* @return The userInfo.
*/
public com.google.cloud.retail.v2alpha.UserInfo getUserInfo() {
if (userInfoBuilder_ == null) {
return userInfo_ == null
? com.google.cloud.retail.v2alpha.UserInfo.getDefaultInstance()
: userInfo_;
} else {
return userInfoBuilder_.getMessage();
}
}
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*/
public Builder setUserInfo(com.google.cloud.retail.v2alpha.UserInfo value) {
if (userInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
userInfo_ = value;
} else {
userInfoBuilder_.setMessage(value);
}
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*/
public Builder setUserInfo(com.google.cloud.retail.v2alpha.UserInfo.Builder builderForValue) {
if (userInfoBuilder_ == null) {
userInfo_ = builderForValue.build();
} else {
userInfoBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*/
public Builder mergeUserInfo(com.google.cloud.retail.v2alpha.UserInfo value) {
if (userInfoBuilder_ == null) {
if (((bitField0_ & 0x00010000) != 0)
&& userInfo_ != null
&& userInfo_ != com.google.cloud.retail.v2alpha.UserInfo.getDefaultInstance()) {
getUserInfoBuilder().mergeFrom(value);
} else {
userInfo_ = value;
}
} else {
userInfoBuilder_.mergeFrom(value);
}
if (userInfo_ != null) {
bitField0_ |= 0x00010000;
onChanged();
}
return this;
}
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*/
public Builder clearUserInfo() {
bitField0_ = (bitField0_ & ~0x00010000);
userInfo_ = null;
if (userInfoBuilder_ != null) {
userInfoBuilder_.dispose();
userInfoBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*/
public com.google.cloud.retail.v2alpha.UserInfo.Builder getUserInfoBuilder() {
bitField0_ |= 0x00010000;
onChanged();
return getUserInfoFieldBuilder().getBuilder();
}
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*/
public com.google.cloud.retail.v2alpha.UserInfoOrBuilder getUserInfoOrBuilder() {
if (userInfoBuilder_ != null) {
return userInfoBuilder_.getMessageOrBuilder();
} else {
return userInfo_ == null
? com.google.cloud.retail.v2alpha.UserInfo.getDefaultInstance()
: userInfo_;
}
}
/**
*
*
*
* User information.
*
*
* .google.cloud.retail.v2alpha.UserInfo user_info = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.UserInfo,
com.google.cloud.retail.v2alpha.UserInfo.Builder,
com.google.cloud.retail.v2alpha.UserInfoOrBuilder>
getUserInfoFieldBuilder() {
if (userInfoBuilder_ == null) {
userInfoBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.retail.v2alpha.UserInfo,
com.google.cloud.retail.v2alpha.UserInfo.Builder,
com.google.cloud.retail.v2alpha.UserInfoOrBuilder>(
getUserInfo(), getParentForChildren(), isClean());
userInfo_ = null;
}
return userInfoBuilder_;
}
private java.lang.Object uri_ = "";
/**
*
*
*
* Complete URL (window.location.href) of the user's current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically. Maximum length 5,000
* characters.
*
*
* string uri = 13;
*
* @return The uri.
*/
public java.lang.String getUri() {
java.lang.Object ref = uri_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
uri_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Complete URL (window.location.href) of the user's current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically. Maximum length 5,000
* characters.
*
*
* string uri = 13;
*
* @return The bytes for uri.
*/
public com.google.protobuf.ByteString getUriBytes() {
java.lang.Object ref = uri_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
uri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Complete URL (window.location.href) of the user's current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically. Maximum length 5,000
* characters.
*
*
* string uri = 13;
*
* @param value The uri to set.
* @return This builder for chaining.
*/
public Builder setUri(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
uri_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
*
*
* Complete URL (window.location.href) of the user's current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically. Maximum length 5,000
* characters.
*
*
* string uri = 13;
*
* @return This builder for chaining.
*/
public Builder clearUri() {
uri_ = getDefaultInstance().getUri();
bitField0_ = (bitField0_ & ~0x00020000);
onChanged();
return this;
}
/**
*
*
*
* Complete URL (window.location.href) of the user's current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically. Maximum length 5,000
* characters.
*
*
* string uri = 13;
*
* @param value The bytes for uri to set.
* @return This builder for chaining.
*/
public Builder setUriBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
uri_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
private java.lang.Object referrerUri_ = "";
/**
*
*
*
* The referrer URL of the current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string referrer_uri = 14;
*
* @return The referrerUri.
*/
public java.lang.String getReferrerUri() {
java.lang.Object ref = referrerUri_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
referrerUri_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The referrer URL of the current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string referrer_uri = 14;
*
* @return The bytes for referrerUri.
*/
public com.google.protobuf.ByteString getReferrerUriBytes() {
java.lang.Object ref = referrerUri_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
referrerUri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The referrer URL of the current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string referrer_uri = 14;
*
* @param value The referrerUri to set.
* @return This builder for chaining.
*/
public Builder setReferrerUri(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
referrerUri_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
*
*
* The referrer URL of the current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string referrer_uri = 14;
*
* @return This builder for chaining.
*/
public Builder clearReferrerUri() {
referrerUri_ = getDefaultInstance().getReferrerUri();
bitField0_ = (bitField0_ & ~0x00040000);
onChanged();
return this;
}
/**
*
*
*
* The referrer URL of the current page.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string referrer_uri = 14;
*
* @param value The bytes for referrerUri to set.
* @return This builder for chaining.
*/
public Builder setReferrerUriBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
referrerUri_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
private java.lang.Object pageViewId_ = "";
/**
*
*
*
* A unique ID of a web page view.
*
* This should be kept the same for all user events triggered from the same
* pageview. For example, an item detail page view could trigger multiple
* events as the user is browsing the page. The `pageViewId` property should
* be kept the same for all these events so that they can be grouped together
* properly.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string page_view_id = 15;
*
* @return The pageViewId.
*/
public java.lang.String getPageViewId() {
java.lang.Object ref = pageViewId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
pageViewId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* A unique ID of a web page view.
*
* This should be kept the same for all user events triggered from the same
* pageview. For example, an item detail page view could trigger multiple
* events as the user is browsing the page. The `pageViewId` property should
* be kept the same for all these events so that they can be grouped together
* properly.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string page_view_id = 15;
*
* @return The bytes for pageViewId.
*/
public com.google.protobuf.ByteString getPageViewIdBytes() {
java.lang.Object ref = pageViewId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
pageViewId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* A unique ID of a web page view.
*
* This should be kept the same for all user events triggered from the same
* pageview. For example, an item detail page view could trigger multiple
* events as the user is browsing the page. The `pageViewId` property should
* be kept the same for all these events so that they can be grouped together
* properly.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string page_view_id = 15;
*
* @param value The pageViewId to set.
* @return This builder for chaining.
*/
public Builder setPageViewId(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
pageViewId_ = value;
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
*
*
* A unique ID of a web page view.
*
* This should be kept the same for all user events triggered from the same
* pageview. For example, an item detail page view could trigger multiple
* events as the user is browsing the page. The `pageViewId` property should
* be kept the same for all these events so that they can be grouped together
* properly.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string page_view_id = 15;
*
* @return This builder for chaining.
*/
public Builder clearPageViewId() {
pageViewId_ = getDefaultInstance().getPageViewId();
bitField0_ = (bitField0_ & ~0x00080000);
onChanged();
return this;
}
/**
*
*
*
* A unique ID of a web page view.
*
* This should be kept the same for all user events triggered from the same
* pageview. For example, an item detail page view could trigger multiple
* events as the user is browsing the page. The `pageViewId` property should
* be kept the same for all these events so that they can be grouped together
* properly.
*
* When using the client side event reporting with JavaScript pixel and Google
* Tag Manager, this value is filled in automatically.
*
*
* string page_view_id = 15;
*
* @param value The bytes for pageViewId to set.
* @return This builder for chaining.
*/
public Builder setPageViewIdBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
pageViewId_ = value;
bitField0_ |= 0x00080000;
onChanged();
return this;
}
private java.lang.Object entity_ = "";
/**
*
*
*
* The entity for customers that may run multiple different entities, domains,
* sites or regions, for example, `Google US`, `Google Ads`, `Waymo`,
* `google.com`, `youtube.com`, etc.
* We recommend that you set this field to get better per-entity search,
* completion, and prediction results.
*
*
* string entity = 23;
*
* @return The entity.
*/
public java.lang.String getEntity() {
java.lang.Object ref = entity_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
entity_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The entity for customers that may run multiple different entities, domains,
* sites or regions, for example, `Google US`, `Google Ads`, `Waymo`,
* `google.com`, `youtube.com`, etc.
* We recommend that you set this field to get better per-entity search,
* completion, and prediction results.
*
*
* string entity = 23;
*
* @return The bytes for entity.
*/
public com.google.protobuf.ByteString getEntityBytes() {
java.lang.Object ref = entity_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
entity_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The entity for customers that may run multiple different entities, domains,
* sites or regions, for example, `Google US`, `Google Ads`, `Waymo`,
* `google.com`, `youtube.com`, etc.
* We recommend that you set this field to get better per-entity search,
* completion, and prediction results.
*
*
* string entity = 23;
*
* @param value The entity to set.
* @return This builder for chaining.
*/
public Builder setEntity(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
entity_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
*
*
* The entity for customers that may run multiple different entities, domains,
* sites or regions, for example, `Google US`, `Google Ads`, `Waymo`,
* `google.com`, `youtube.com`, etc.
* We recommend that you set this field to get better per-entity search,
* completion, and prediction results.
*
*
* string entity = 23;
*
* @return This builder for chaining.
*/
public Builder clearEntity() {
entity_ = getDefaultInstance().getEntity();
bitField0_ = (bitField0_ & ~0x00100000);
onChanged();
return this;
}
/**
*
*
*
* The entity for customers that may run multiple different entities, domains,
* sites or regions, for example, `Google US`, `Google Ads`, `Waymo`,
* `google.com`, `youtube.com`, etc.
* We recommend that you set this field to get better per-entity search,
* completion, and prediction results.
*
*
* string entity = 23;
*
* @param value The bytes for entity to set.
* @return This builder for chaining.
*/
public Builder setEntityBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
entity_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.retail.v2alpha.UserEvent)
}
// @@protoc_insertion_point(class_scope:google.cloud.retail.v2alpha.UserEvent)
private static final com.google.cloud.retail.v2alpha.UserEvent DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.retail.v2alpha.UserEvent();
}
public static com.google.cloud.retail.v2alpha.UserEvent getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public UserEvent parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.retail.v2alpha.UserEvent getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy