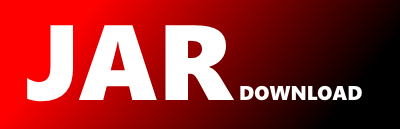
google.cloud.retail.v2alpha.generative_question_service.proto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Show all versions of proto-google-cloud-retail-v2alpha Show documentation
Proto library for google-cloud-retail
// Copyright 2024 Google LLC
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
syntax = "proto3";
package google.cloud.retail.v2alpha;
import "google/api/annotations.proto";
import "google/api/client.proto";
import "google/api/field_behavior.proto";
import "google/api/resource.proto";
import "google/cloud/retail/v2alpha/generative_question.proto";
import "google/protobuf/field_mask.proto";
option csharp_namespace = "Google.Cloud.Retail.V2Alpha";
option go_package = "cloud.google.com/go/retail/apiv2alpha/retailpb;retailpb";
option java_multiple_files = true;
option java_outer_classname = "GenerativeQuestionServiceProto";
option java_package = "com.google.cloud.retail.v2alpha";
option objc_class_prefix = "RETAIL";
option php_namespace = "Google\\Cloud\\Retail\\V2alpha";
option ruby_package = "Google::Cloud::Retail::V2alpha";
// Service for managing LLM generated questions in search serving.
service GenerativeQuestionService {
option (google.api.default_host) = "retail.googleapis.com";
option (google.api.oauth_scopes) =
"https://www.googleapis.com/auth/cloud-platform";
// Manages overal generative question feature state -- enables toggling
// feature on and off.
rpc UpdateGenerativeQuestionsFeatureConfig(
UpdateGenerativeQuestionsFeatureConfigRequest)
returns (GenerativeQuestionsFeatureConfig) {
option (google.api.http) = {
patch: "/v2alpha/{generative_questions_feature_config.catalog=projects/*/locations/*/catalogs/*}/generativeQuestionFeature"
body: "generative_questions_feature_config"
};
option (google.api.method_signature) =
"generative_questions_feature_config,update_mask";
}
// Manages overal generative question feature state -- enables toggling
// feature on and off.
rpc GetGenerativeQuestionsFeatureConfig(
GetGenerativeQuestionsFeatureConfigRequest)
returns (GenerativeQuestionsFeatureConfig) {
option (google.api.http) = {
get: "/v2alpha/{catalog=projects/*/locations/*/catalogs/*}/generativeQuestionFeature"
};
option (google.api.method_signature) = "catalog";
}
// Returns all questions for a given catalog.
rpc ListGenerativeQuestionConfigs(ListGenerativeQuestionConfigsRequest)
returns (ListGenerativeQuestionConfigsResponse) {
option (google.api.http) = {
get: "/v2alpha/{parent=projects/*/locations/*/catalogs/*}/generativeQuestions"
};
option (google.api.method_signature) = "parent";
}
// Allows management of individual questions.
rpc UpdateGenerativeQuestionConfig(UpdateGenerativeQuestionConfigRequest)
returns (GenerativeQuestionConfig) {
option (google.api.http) = {
patch: "/v2alpha/{generative_question_config.catalog=projects/*/locations/*/catalogs/*}/generativeQuestion"
body: "generative_question_config"
};
option (google.api.method_signature) =
"generative_question_config,update_mask";
}
// Allows management of multiple questions.
rpc BatchUpdateGenerativeQuestionConfigs(
BatchUpdateGenerativeQuestionConfigsRequest)
returns (BatchUpdateGenerativeQuestionConfigsResponse) {
option (google.api.http) = {
post: "/v2alpha/{parent=projects/*/locations/*/catalogs/*}/generativeQuestion:batchUpdate"
body: "*"
};
option (google.api.method_signature) = "parent,requests";
}
}
// Request for UpdateGenerativeQuestionsFeatureConfig method.
message UpdateGenerativeQuestionsFeatureConfigRequest {
// Required. The configuration managing the feature state.
GenerativeQuestionsFeatureConfig generative_questions_feature_config = 2
[(google.api.field_behavior) = REQUIRED];
// Optional. Indicates which fields in the provided
// [GenerativeQuestionsFeatureConfig][google.cloud.retail.v2alpha.GenerativeQuestionsFeatureConfig]
// to update. If not set or empty, all supported fields are updated.
google.protobuf.FieldMask update_mask = 4
[(google.api.field_behavior) = OPTIONAL];
}
// Request for GetGenerativeQuestionsFeatureConfig method.
message GetGenerativeQuestionsFeatureConfigRequest {
// Required. Resource name of the parent catalog.
// Format: projects/{project}/locations/{location}/catalogs/{catalog}
string catalog = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = { type: "retail.googleapis.com/Catalog" }
];
}
// Request for ListQuestions method.
message ListGenerativeQuestionConfigsRequest {
// Required. Resource name of the parent catalog.
// Format: projects/{project}/locations/{location}/catalogs/{catalog}
string parent = 1 [
(google.api.field_behavior) = REQUIRED,
(google.api.resource_reference) = { type: "retail.googleapis.com/Catalog" }
];
}
// Response for ListQuestions method.
message ListGenerativeQuestionConfigsResponse {
// All the questions for a given catalog.
repeated GenerativeQuestionConfig generative_question_configs = 1;
}
// Request for UpdateGenerativeQuestionConfig method.
message UpdateGenerativeQuestionConfigRequest {
// Required. The question to update.
GenerativeQuestionConfig generative_question_config = 3
[(google.api.field_behavior) = REQUIRED];
// Optional. Indicates which fields in the provided
// [GenerativeQuestionConfig][google.cloud.retail.v2alpha.GenerativeQuestionConfig]
// to update. The following are NOT supported:
//
// * [GenerativeQuestionConfig.frequency][google.cloud.retail.v2alpha.GenerativeQuestionConfig.frequency]
//
// If not set or empty, all supported fields are updated.
google.protobuf.FieldMask update_mask = 4
[(google.api.field_behavior) = OPTIONAL];
}
// Request for BatchUpdateGenerativeQuestionConfig method.
message BatchUpdateGenerativeQuestionConfigsRequest {
// Optional. Resource name of the parent catalog.
// Format: projects/{project}/locations/{location}/catalogs/{catalog}
string parent = 1 [
(google.api.field_behavior) = OPTIONAL,
(google.api.resource_reference) = { type: "retail.googleapis.com/Catalog" }
];
// Required. The updates question configs.
repeated UpdateGenerativeQuestionConfigRequest requests = 2
[(google.api.field_behavior) = REQUIRED];
}
// Aggregated response for UpdateGenerativeQuestionConfig method.
message BatchUpdateGenerativeQuestionConfigsResponse {
// Optional. The updates question configs.
repeated GenerativeQuestionConfig generative_question_configs = 1
[(google.api.field_behavior) = OPTIONAL];
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy