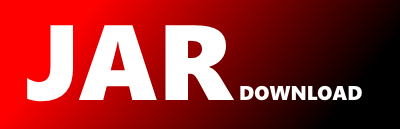
google.cloud.securitycenter.v1.vulnerability.proto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-securitycenter-v1 Show documentation
Show all versions of proto-google-cloud-securitycenter-v1 Show documentation
PROTO library for proto-google-cloud-securitycenter-v1
// Copyright 2023 Google LLC
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
syntax = "proto3";
package google.cloud.securitycenter.v1;
import "google/protobuf/timestamp.proto";
option csharp_namespace = "Google.Cloud.SecurityCenter.V1";
option go_package = "cloud.google.com/go/securitycenter/apiv1/securitycenterpb;securitycenterpb";
option java_multiple_files = true;
option java_outer_classname = "VulnerabilityProto";
option java_package = "com.google.cloud.securitycenter.v1";
option php_namespace = "Google\\Cloud\\SecurityCenter\\V1";
option ruby_package = "Google::Cloud::SecurityCenter::V1";
// Refers to common vulnerability fields e.g. cve, cvss, cwe etc.
message Vulnerability {
// CVE stands for Common Vulnerabilities and Exposures
// (https://cve.mitre.org/about/)
Cve cve = 1;
// The offending package is relevant to the finding.
Package offending_package = 2;
// The fixed package is relevant to the finding.
Package fixed_package = 3;
// The security bulletin is relevant to this finding.
SecurityBulletin security_bulletin = 4;
}
// CVE stands for Common Vulnerabilities and Exposures.
// Information from the [CVE
// record](https://www.cve.org/ResourcesSupport/Glossary) that describes this
// vulnerability.
message Cve {
// The possible values of impact of the vulnerability if it was to be
// exploited.
enum RiskRating {
// Invalid or empty value.
RISK_RATING_UNSPECIFIED = 0;
// Exploitation would have little to no security impact.
LOW = 1;
// Exploitation would enable attackers to perform activities, or could allow
// attackers to have a direct impact, but would require additional steps.
MEDIUM = 2;
// Exploitation would enable attackers to have a notable direct impact
// without needing to overcome any major mitigating factors.
HIGH = 3;
// Exploitation would fundamentally undermine the security of affected
// systems, enable actors to perform significant attacks with minimal
// effort, with little to no mitigating factors to overcome.
CRITICAL = 4;
}
// The possible values of exploitation activity of the vulnerability in the
// wild.
enum ExploitationActivity {
// Invalid or empty value.
EXPLOITATION_ACTIVITY_UNSPECIFIED = 0;
// Exploitation has been reported or confirmed to widely occur.
WIDE = 1;
// Limited reported or confirmed exploitation activities.
CONFIRMED = 2;
// Exploit is publicly available.
AVAILABLE = 3;
// No known exploitation activity, but has a high potential for
// exploitation.
ANTICIPATED = 4;
// No known exploitation activity.
NO_KNOWN = 5;
}
// The unique identifier for the vulnerability. e.g. CVE-2021-34527
string id = 1;
// Additional information about the CVE.
// e.g. https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2021-34527
repeated Reference references = 2;
// Describe Common Vulnerability Scoring System specified at
// https://www.first.org/cvss/v3.1/specification-document
Cvssv3 cvssv3 = 3;
// Whether upstream fix is available for the CVE.
bool upstream_fix_available = 4;
// The potential impact of the vulnerability if it was to be exploited.
RiskRating impact = 5;
// The exploitation activity of the vulnerability in the wild.
ExploitationActivity exploitation_activity = 6;
// Whether or not the vulnerability has been observed in the wild.
bool observed_in_the_wild = 7;
// Whether or not the vulnerability was zero day when the finding was
// published.
bool zero_day = 8;
}
// Additional Links
message Reference {
// Source of the reference e.g. NVD
string source = 1;
// Uri for the mentioned source e.g.
// https://cve.mitre.org/cgi-bin/cvename.cgi?name=CVE-2021-34527.
string uri = 2;
}
// Common Vulnerability Scoring System version 3.
message Cvssv3 {
// This metric reflects the context by which vulnerability exploitation is
// possible.
enum AttackVector {
// Invalid value.
ATTACK_VECTOR_UNSPECIFIED = 0;
// The vulnerable component is bound to the network stack and the set of
// possible attackers extends beyond the other options listed below, up to
// and including the entire Internet.
ATTACK_VECTOR_NETWORK = 1;
// The vulnerable component is bound to the network stack, but the attack is
// limited at the protocol level to a logically adjacent topology.
ATTACK_VECTOR_ADJACENT = 2;
// The vulnerable component is not bound to the network stack and the
// attacker's path is via read/write/execute capabilities.
ATTACK_VECTOR_LOCAL = 3;
// The attack requires the attacker to physically touch or manipulate the
// vulnerable component.
ATTACK_VECTOR_PHYSICAL = 4;
}
// This metric describes the conditions beyond the attacker's control that
// must exist in order to exploit the vulnerability.
enum AttackComplexity {
// Invalid value.
ATTACK_COMPLEXITY_UNSPECIFIED = 0;
// Specialized access conditions or extenuating circumstances do not exist.
// An attacker can expect repeatable success when attacking the vulnerable
// component.
ATTACK_COMPLEXITY_LOW = 1;
// A successful attack depends on conditions beyond the attacker's control.
// That is, a successful attack cannot be accomplished at will, but requires
// the attacker to invest in some measurable amount of effort in preparation
// or execution against the vulnerable component before a successful attack
// can be expected.
ATTACK_COMPLEXITY_HIGH = 2;
}
// This metric describes the level of privileges an attacker must possess
// before successfully exploiting the vulnerability.
enum PrivilegesRequired {
// Invalid value.
PRIVILEGES_REQUIRED_UNSPECIFIED = 0;
// The attacker is unauthorized prior to attack, and therefore does not
// require any access to settings or files of the vulnerable system to
// carry out an attack.
PRIVILEGES_REQUIRED_NONE = 1;
// The attacker requires privileges that provide basic user capabilities
// that could normally affect only settings and files owned by a user.
// Alternatively, an attacker with Low privileges has the ability to access
// only non-sensitive resources.
PRIVILEGES_REQUIRED_LOW = 2;
// The attacker requires privileges that provide significant (e.g.,
// administrative) control over the vulnerable component allowing access to
// component-wide settings and files.
PRIVILEGES_REQUIRED_HIGH = 3;
}
// This metric captures the requirement for a human user, other than the
// attacker, to participate in the successful compromise of the vulnerable
// component.
enum UserInteraction {
// Invalid value.
USER_INTERACTION_UNSPECIFIED = 0;
// The vulnerable system can be exploited without interaction from any user.
USER_INTERACTION_NONE = 1;
// Successful exploitation of this vulnerability requires a user to take
// some action before the vulnerability can be exploited.
USER_INTERACTION_REQUIRED = 2;
}
// The Scope metric captures whether a vulnerability in one vulnerable
// component impacts resources in components beyond its security scope.
enum Scope {
// Invalid value.
SCOPE_UNSPECIFIED = 0;
// An exploited vulnerability can only affect resources managed by the same
// security authority.
SCOPE_UNCHANGED = 1;
// An exploited vulnerability can affect resources beyond the security scope
// managed by the security authority of the vulnerable component.
SCOPE_CHANGED = 2;
}
// The Impact metrics capture the effects of a successfully exploited
// vulnerability on the component that suffers the worst outcome that is most
// directly and predictably associated with the attack.
enum Impact {
// Invalid value.
IMPACT_UNSPECIFIED = 0;
// High impact.
IMPACT_HIGH = 1;
// Low impact.
IMPACT_LOW = 2;
// No impact.
IMPACT_NONE = 3;
}
// The base score is a function of the base metric scores.
double base_score = 1;
// Base Metrics
// Represents the intrinsic characteristics of a vulnerability that are
// constant over time and across user environments.
// This metric reflects the context by which vulnerability exploitation is
// possible.
AttackVector attack_vector = 5;
// This metric describes the conditions beyond the attacker's control that
// must exist in order to exploit the vulnerability.
AttackComplexity attack_complexity = 6;
// This metric describes the level of privileges an attacker must possess
// before successfully exploiting the vulnerability.
PrivilegesRequired privileges_required = 7;
// This metric captures the requirement for a human user, other than the
// attacker, to participate in the successful compromise of the vulnerable
// component.
UserInteraction user_interaction = 8;
// The Scope metric captures whether a vulnerability in one vulnerable
// component impacts resources in components beyond its security scope.
Scope scope = 9;
// This metric measures the impact to the confidentiality of the information
// resources managed by a software component due to a successfully exploited
// vulnerability.
Impact confidentiality_impact = 10;
// This metric measures the impact to integrity of a successfully exploited
// vulnerability.
Impact integrity_impact = 11;
// This metric measures the impact to the availability of the impacted
// component resulting from a successfully exploited vulnerability.
Impact availability_impact = 12;
}
// Package is a generic definition of a package.
message Package {
// The name of the package where the vulnerability was detected.
string package_name = 1;
// The CPE URI where the vulnerability was detected.
string cpe_uri = 2;
// Type of package, for example, os, maven, or go.
string package_type = 3;
// The version of the package.
string package_version = 4;
}
// SecurityBulletin are notifications of vulnerabilities of Google products.
message SecurityBulletin {
// ID of the bulletin corresponding to the vulnerability.
string bulletin_id = 1;
// Submission time of this Security Bulletin.
google.protobuf.Timestamp submission_time = 2;
// This represents a version that the cluster receiving this notification
// should be upgraded to, based on its current version. For example, 1.15.0
string suggested_upgrade_version = 3;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy