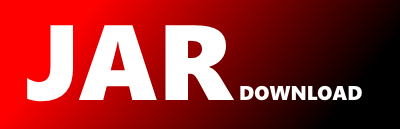
com.google.cloud.securitycenter.v2.Finding Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-securitycenter-v2 Show documentation
Show all versions of proto-google-cloud-securitycenter-v2 Show documentation
Proto library for google-cloud-securitycenter
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/cloud/securitycenter/v2/finding.proto
// Protobuf Java Version: 3.25.3
package com.google.cloud.securitycenter.v2;
/**
*
*
*
* Security Command Center finding.
*
* A finding is a record of assessment data like security, risk, health, or
* privacy, that is ingested into Security Command Center for presentation,
* notification, analysis, policy testing, and enforcement. For example, a
* cross-site scripting (XSS) vulnerability in an App Engine application is a
* finding.
*
*
* Protobuf type {@code google.cloud.securitycenter.v2.Finding}
*/
public final class Finding extends com.google.protobuf.GeneratedMessageV3
implements
// @@protoc_insertion_point(message_implements:google.cloud.securitycenter.v2.Finding)
FindingOrBuilder {
private static final long serialVersionUID = 0L;
// Use Finding.newBuilder() to construct.
private Finding(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Finding() {
name_ = "";
canonicalName_ = "";
parent_ = "";
resourceName_ = "";
state_ = 0;
category_ = "";
externalUri_ = "";
severity_ = 0;
mute_ = 0;
findingClass_ = 0;
connections_ = java.util.Collections.emptyList();
muteInitiator_ = "";
processes_ = java.util.Collections.emptyList();
compliances_ = java.util.Collections.emptyList();
parentDisplayName_ = "";
description_ = "";
iamBindings_ = java.util.Collections.emptyList();
nextSteps_ = "";
moduleName_ = "";
containers_ = java.util.Collections.emptyList();
files_ = java.util.Collections.emptyList();
orgPolicies_ = java.util.Collections.emptyList();
logEntries_ = java.util.Collections.emptyList();
loadBalancers_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(UnusedPrivateParameter unused) {
return new Finding();
}
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.securitycenter.v2.FindingProto
.internal_static_google_cloud_securitycenter_v2_Finding_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 9:
return internalGetSourceProperties();
case 20:
return internalGetExternalSystems();
case 26:
return internalGetContacts();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.securitycenter.v2.FindingProto
.internal_static_google_cloud_securitycenter_v2_Finding_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.securitycenter.v2.Finding.class,
com.google.cloud.securitycenter.v2.Finding.Builder.class);
}
/**
*
*
*
* The state of the finding.
*
*
* Protobuf enum {@code google.cloud.securitycenter.v2.Finding.State}
*/
public enum State implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* Unspecified state.
*
*
* STATE_UNSPECIFIED = 0;
*/
STATE_UNSPECIFIED(0),
/**
*
*
*
* The finding requires attention and has not been addressed yet.
*
*
* ACTIVE = 1;
*/
ACTIVE(1),
/**
*
*
*
* The finding has been fixed, triaged as a non-issue or otherwise addressed
* and is no longer active.
*
*
* INACTIVE = 2;
*/
INACTIVE(2),
UNRECOGNIZED(-1),
;
/**
*
*
*
* Unspecified state.
*
*
* STATE_UNSPECIFIED = 0;
*/
public static final int STATE_UNSPECIFIED_VALUE = 0;
/**
*
*
*
* The finding requires attention and has not been addressed yet.
*
*
* ACTIVE = 1;
*/
public static final int ACTIVE_VALUE = 1;
/**
*
*
*
* The finding has been fixed, triaged as a non-issue or otherwise addressed
* and is no longer active.
*
*
* INACTIVE = 2;
*/
public static final int INACTIVE_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static State valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static State forNumber(int value) {
switch (value) {
case 0:
return STATE_UNSPECIFIED;
case 1:
return ACTIVE;
case 2:
return INACTIVE;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public State findValueByNumber(int number) {
return State.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.securitycenter.v2.Finding.getDescriptor().getEnumTypes().get(0);
}
private static final State[] VALUES = values();
public static State valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private State(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.securitycenter.v2.Finding.State)
}
/**
*
*
*
* The severity of the finding.
*
*
* Protobuf enum {@code google.cloud.securitycenter.v2.Finding.Severity}
*/
public enum Severity implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* This value is used for findings when a source doesn't write a severity
* value.
*
*
* SEVERITY_UNSPECIFIED = 0;
*/
SEVERITY_UNSPECIFIED(0),
/**
*
*
*
* Vulnerability:
* A critical vulnerability is easily discoverable by an external actor,
* exploitable, and results in the direct ability to execute arbitrary code,
* exfiltrate data, and otherwise gain additional access and privileges to
* cloud resources and workloads. Examples include publicly accessible
* unprotected user data and public SSH access with weak or no
* passwords.
*
* Threat:
* Indicates a threat that is able to access, modify, or delete data or
* execute unauthorized code within existing resources.
*
*
* CRITICAL = 1;
*/
CRITICAL(1),
/**
*
*
*
* Vulnerability:
* A high risk vulnerability can be easily discovered and exploited in
* combination with other vulnerabilities in order to gain direct access and
* the ability to execute arbitrary code, exfiltrate data, and otherwise
* gain additional access and privileges to cloud resources and workloads.
* An example is a database with weak or no passwords that is only
* accessible internally. This database could easily be compromised by an
* actor that had access to the internal network.
*
* Threat:
* Indicates a threat that is able to create new computational resources in
* an environment but not able to access data or execute code in existing
* resources.
*
*
* HIGH = 2;
*/
HIGH(2),
/**
*
*
*
* Vulnerability:
* A medium risk vulnerability could be used by an actor to gain access to
* resources or privileges that enable them to eventually (through multiple
* steps or a complex exploit) gain access and the ability to execute
* arbitrary code or exfiltrate data. An example is a service account with
* access to more projects than it should have. If an actor gains access to
* the service account, they could potentially use that access to manipulate
* a project the service account was not intended to.
*
* Threat:
* Indicates a threat that is able to cause operational impact but may not
* access data or execute unauthorized code.
*
*
* MEDIUM = 3;
*/
MEDIUM(3),
/**
*
*
*
* Vulnerability:
* A low risk vulnerability hampers a security organization's ability to
* detect vulnerabilities or active threats in their deployment, or prevents
* the root cause investigation of security issues. An example is monitoring
* and logs being disabled for resource configurations and access.
*
* Threat:
* Indicates a threat that has obtained minimal access to an environment but
* is not able to access data, execute code, or create resources.
*
*
* LOW = 4;
*/
LOW(4),
UNRECOGNIZED(-1),
;
/**
*
*
*
* This value is used for findings when a source doesn't write a severity
* value.
*
*
* SEVERITY_UNSPECIFIED = 0;
*/
public static final int SEVERITY_UNSPECIFIED_VALUE = 0;
/**
*
*
*
* Vulnerability:
* A critical vulnerability is easily discoverable by an external actor,
* exploitable, and results in the direct ability to execute arbitrary code,
* exfiltrate data, and otherwise gain additional access and privileges to
* cloud resources and workloads. Examples include publicly accessible
* unprotected user data and public SSH access with weak or no
* passwords.
*
* Threat:
* Indicates a threat that is able to access, modify, or delete data or
* execute unauthorized code within existing resources.
*
*
* CRITICAL = 1;
*/
public static final int CRITICAL_VALUE = 1;
/**
*
*
*
* Vulnerability:
* A high risk vulnerability can be easily discovered and exploited in
* combination with other vulnerabilities in order to gain direct access and
* the ability to execute arbitrary code, exfiltrate data, and otherwise
* gain additional access and privileges to cloud resources and workloads.
* An example is a database with weak or no passwords that is only
* accessible internally. This database could easily be compromised by an
* actor that had access to the internal network.
*
* Threat:
* Indicates a threat that is able to create new computational resources in
* an environment but not able to access data or execute code in existing
* resources.
*
*
* HIGH = 2;
*/
public static final int HIGH_VALUE = 2;
/**
*
*
*
* Vulnerability:
* A medium risk vulnerability could be used by an actor to gain access to
* resources or privileges that enable them to eventually (through multiple
* steps or a complex exploit) gain access and the ability to execute
* arbitrary code or exfiltrate data. An example is a service account with
* access to more projects than it should have. If an actor gains access to
* the service account, they could potentially use that access to manipulate
* a project the service account was not intended to.
*
* Threat:
* Indicates a threat that is able to cause operational impact but may not
* access data or execute unauthorized code.
*
*
* MEDIUM = 3;
*/
public static final int MEDIUM_VALUE = 3;
/**
*
*
*
* Vulnerability:
* A low risk vulnerability hampers a security organization's ability to
* detect vulnerabilities or active threats in their deployment, or prevents
* the root cause investigation of security issues. An example is monitoring
* and logs being disabled for resource configurations and access.
*
* Threat:
* Indicates a threat that has obtained minimal access to an environment but
* is not able to access data, execute code, or create resources.
*
*
* LOW = 4;
*/
public static final int LOW_VALUE = 4;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Severity valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Severity forNumber(int value) {
switch (value) {
case 0:
return SEVERITY_UNSPECIFIED;
case 1:
return CRITICAL;
case 2:
return HIGH;
case 3:
return MEDIUM;
case 4:
return LOW;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Severity findValueByNumber(int number) {
return Severity.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.securitycenter.v2.Finding.getDescriptor().getEnumTypes().get(1);
}
private static final Severity[] VALUES = values();
public static Severity valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Severity(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.securitycenter.v2.Finding.Severity)
}
/**
*
*
*
* Mute state a finding can be in.
*
*
* Protobuf enum {@code google.cloud.securitycenter.v2.Finding.Mute}
*/
public enum Mute implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* Unspecified.
*
*
* MUTE_UNSPECIFIED = 0;
*/
MUTE_UNSPECIFIED(0),
/**
*
*
*
* Finding has been muted.
*
*
* MUTED = 1;
*/
MUTED(1),
/**
*
*
*
* Finding has been unmuted.
*
*
* UNMUTED = 2;
*/
UNMUTED(2),
/**
*
*
*
* Finding has never been muted/unmuted.
*
*
* UNDEFINED = 3;
*/
UNDEFINED(3),
UNRECOGNIZED(-1),
;
/**
*
*
*
* Unspecified.
*
*
* MUTE_UNSPECIFIED = 0;
*/
public static final int MUTE_UNSPECIFIED_VALUE = 0;
/**
*
*
*
* Finding has been muted.
*
*
* MUTED = 1;
*/
public static final int MUTED_VALUE = 1;
/**
*
*
*
* Finding has been unmuted.
*
*
* UNMUTED = 2;
*/
public static final int UNMUTED_VALUE = 2;
/**
*
*
*
* Finding has never been muted/unmuted.
*
*
* UNDEFINED = 3;
*/
public static final int UNDEFINED_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Mute valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Mute forNumber(int value) {
switch (value) {
case 0:
return MUTE_UNSPECIFIED;
case 1:
return MUTED;
case 2:
return UNMUTED;
case 3:
return UNDEFINED;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Mute findValueByNumber(int number) {
return Mute.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.securitycenter.v2.Finding.getDescriptor().getEnumTypes().get(2);
}
private static final Mute[] VALUES = values();
public static Mute valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Mute(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.securitycenter.v2.Finding.Mute)
}
/**
*
*
*
* Represents what kind of Finding it is.
*
*
* Protobuf enum {@code google.cloud.securitycenter.v2.Finding.FindingClass}
*/
public enum FindingClass implements com.google.protobuf.ProtocolMessageEnum {
/**
*
*
*
* Unspecified finding class.
*
*
* FINDING_CLASS_UNSPECIFIED = 0;
*/
FINDING_CLASS_UNSPECIFIED(0),
/**
*
*
*
* Describes unwanted or malicious activity.
*
*
* THREAT = 1;
*/
THREAT(1),
/**
*
*
*
* Describes a potential weakness in software that increases risk to
* Confidentiality & Integrity & Availability.
*
*
* VULNERABILITY = 2;
*/
VULNERABILITY(2),
/**
*
*
*
* Describes a potential weakness in cloud resource/asset configuration that
* increases risk.
*
*
* MISCONFIGURATION = 3;
*/
MISCONFIGURATION(3),
/**
*
*
*
* Describes a security observation that is for informational purposes.
*
*
* OBSERVATION = 4;
*/
OBSERVATION(4),
/**
*
*
*
* Describes an error that prevents some SCC functionality.
*
*
* SCC_ERROR = 5;
*/
SCC_ERROR(5),
/**
*
*
*
* Describes a potential security risk due to a change in the security
* posture.
*
*
* POSTURE_VIOLATION = 6;
*/
POSTURE_VIOLATION(6),
UNRECOGNIZED(-1),
;
/**
*
*
*
* Unspecified finding class.
*
*
* FINDING_CLASS_UNSPECIFIED = 0;
*/
public static final int FINDING_CLASS_UNSPECIFIED_VALUE = 0;
/**
*
*
*
* Describes unwanted or malicious activity.
*
*
* THREAT = 1;
*/
public static final int THREAT_VALUE = 1;
/**
*
*
*
* Describes a potential weakness in software that increases risk to
* Confidentiality & Integrity & Availability.
*
*
* VULNERABILITY = 2;
*/
public static final int VULNERABILITY_VALUE = 2;
/**
*
*
*
* Describes a potential weakness in cloud resource/asset configuration that
* increases risk.
*
*
* MISCONFIGURATION = 3;
*/
public static final int MISCONFIGURATION_VALUE = 3;
/**
*
*
*
* Describes a security observation that is for informational purposes.
*
*
* OBSERVATION = 4;
*/
public static final int OBSERVATION_VALUE = 4;
/**
*
*
*
* Describes an error that prevents some SCC functionality.
*
*
* SCC_ERROR = 5;
*/
public static final int SCC_ERROR_VALUE = 5;
/**
*
*
*
* Describes a potential security risk due to a change in the security
* posture.
*
*
* POSTURE_VIOLATION = 6;
*/
public static final int POSTURE_VIOLATION_VALUE = 6;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static FindingClass valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static FindingClass forNumber(int value) {
switch (value) {
case 0:
return FINDING_CLASS_UNSPECIFIED;
case 1:
return THREAT;
case 2:
return VULNERABILITY;
case 3:
return MISCONFIGURATION;
case 4:
return OBSERVATION;
case 5:
return SCC_ERROR;
case 6:
return POSTURE_VIOLATION;
default:
return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public FindingClass findValueByNumber(int number) {
return FindingClass.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor getDescriptor() {
return com.google.cloud.securitycenter.v2.Finding.getDescriptor().getEnumTypes().get(3);
}
private static final FindingClass[] VALUES = values();
public static FindingClass valueOf(com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException("EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private FindingClass(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:google.cloud.securitycenter.v2.Finding.FindingClass)
}
private int bitField0_;
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object name_ = "";
/**
*
*
*
* The [relative resource
* name](https://cloud.google.com/apis/design/resource_names#relative_resource_name)
* of the finding. The following list shows some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
*
* string name = 1;
*
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
*
*
* The [relative resource
* name](https://cloud.google.com/apis/design/resource_names#relative_resource_name)
* of the finding. The following list shows some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
*
* string name = 1;
*
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CANONICAL_NAME_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object canonicalName_ = "";
/**
*
*
*
* Output only. The canonical name of the finding. The following list shows
* some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
* The prefix is the closest CRM ancestor of the resource associated with the
* finding.
*
*
* string canonical_name = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The canonicalName.
*/
@java.lang.Override
public java.lang.String getCanonicalName() {
java.lang.Object ref = canonicalName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
canonicalName_ = s;
return s;
}
}
/**
*
*
*
* Output only. The canonical name of the finding. The following list shows
* some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
* The prefix is the closest CRM ancestor of the resource associated with the
* finding.
*
*
* string canonical_name = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for canonicalName.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCanonicalNameBytes() {
java.lang.Object ref = canonicalName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
canonicalName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PARENT_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object parent_ = "";
/**
*
*
*
* The relative resource name of the source and location the finding belongs
* to. See:
* https://cloud.google.com/apis/design/resource_names#relative_resource_name
* This field is immutable after creation time. The following list shows some
* examples:
*
* + `organizations/{organization_id}/sources/{source_id}`
* + `folders/{folders_id}/sources/{source_id}`
* + `projects/{projects_id}/sources/{source_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}`
* + `folders/{folders_id}/sources/{source_id}/locations/{location_id}`
* + `projects/{projects_id}/sources/{source_id}/locations/{location_id}`
*
*
* string parent = 3;
*
* @return The parent.
*/
@java.lang.Override
public java.lang.String getParent() {
java.lang.Object ref = parent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
parent_ = s;
return s;
}
}
/**
*
*
*
* The relative resource name of the source and location the finding belongs
* to. See:
* https://cloud.google.com/apis/design/resource_names#relative_resource_name
* This field is immutable after creation time. The following list shows some
* examples:
*
* + `organizations/{organization_id}/sources/{source_id}`
* + `folders/{folders_id}/sources/{source_id}`
* + `projects/{projects_id}/sources/{source_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}`
* + `folders/{folders_id}/sources/{source_id}/locations/{location_id}`
* + `projects/{projects_id}/sources/{source_id}/locations/{location_id}`
*
*
* string parent = 3;
*
* @return The bytes for parent.
*/
@java.lang.Override
public com.google.protobuf.ByteString getParentBytes() {
java.lang.Object ref = parent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
parent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RESOURCE_NAME_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object resourceName_ = "";
/**
*
*
*
* Immutable. For findings on Google Cloud resources, the full resource
* name of the Google Cloud resource this finding is for. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* When the finding is for a non-Google Cloud resource, the resourceName can
* be a customer or partner defined string.
*
*
* string resource_name = 4 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The resourceName.
*/
@java.lang.Override
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
}
}
/**
*
*
*
* Immutable. For findings on Google Cloud resources, the full resource
* name of the Google Cloud resource this finding is for. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* When the finding is for a non-Google Cloud resource, the resourceName can
* be a customer or partner defined string.
*
*
* string resource_name = 4 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The bytes for resourceName.
*/
@java.lang.Override
public com.google.protobuf.ByteString getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATE_FIELD_NUMBER = 6;
private int state_ = 0;
/**
*
*
*
* Output only. The state of the finding.
*
*
*
* .google.cloud.securitycenter.v2.Finding.State state = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The enum numeric value on the wire for state.
*/
@java.lang.Override
public int getStateValue() {
return state_;
}
/**
*
*
*
* Output only. The state of the finding.
*
*
*
* .google.cloud.securitycenter.v2.Finding.State state = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The state.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding.State getState() {
com.google.cloud.securitycenter.v2.Finding.State result =
com.google.cloud.securitycenter.v2.Finding.State.forNumber(state_);
return result == null ? com.google.cloud.securitycenter.v2.Finding.State.UNRECOGNIZED : result;
}
public static final int CATEGORY_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object category_ = "";
/**
*
*
*
* Immutable. The additional taxonomy group within findings from a given
* source. Example: "XSS_FLASH_INJECTION"
*
*
* string category = 7 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The category.
*/
@java.lang.Override
public java.lang.String getCategory() {
java.lang.Object ref = category_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
category_ = s;
return s;
}
}
/**
*
*
*
* Immutable. The additional taxonomy group within findings from a given
* source. Example: "XSS_FLASH_INJECTION"
*
*
* string category = 7 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The bytes for category.
*/
@java.lang.Override
public com.google.protobuf.ByteString getCategoryBytes() {
java.lang.Object ref = category_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
category_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXTERNAL_URI_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private volatile java.lang.Object externalUri_ = "";
/**
*
*
*
* The URI that, if available, points to a web page outside of Security
* Command Center where additional information about the finding can be found.
* This field is guaranteed to be either empty or a well formed URL.
*
*
* string external_uri = 8;
*
* @return The externalUri.
*/
@java.lang.Override
public java.lang.String getExternalUri() {
java.lang.Object ref = externalUri_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
externalUri_ = s;
return s;
}
}
/**
*
*
*
* The URI that, if available, points to a web page outside of Security
* Command Center where additional information about the finding can be found.
* This field is guaranteed to be either empty or a well formed URL.
*
*
* string external_uri = 8;
*
* @return The bytes for externalUri.
*/
@java.lang.Override
public com.google.protobuf.ByteString getExternalUriBytes() {
java.lang.Object ref = externalUri_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
externalUri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOURCE_PROPERTIES_FIELD_NUMBER = 9;
private static final class SourcePropertiesDefaultEntryHolder {
static final com.google.protobuf.MapEntry
defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.google.cloud.securitycenter.v2.FindingProto
.internal_static_google_cloud_securitycenter_v2_Finding_SourcePropertiesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.protobuf.Value.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField
sourceProperties_;
private com.google.protobuf.MapField
internalGetSourceProperties() {
if (sourceProperties_ == null) {
return com.google.protobuf.MapField.emptyMapField(
SourcePropertiesDefaultEntryHolder.defaultEntry);
}
return sourceProperties_;
}
public int getSourcePropertiesCount() {
return internalGetSourceProperties().getMap().size();
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
@java.lang.Override
public boolean containsSourceProperties(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetSourceProperties().getMap().containsKey(key);
}
/** Use {@link #getSourcePropertiesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getSourceProperties() {
return getSourcePropertiesMap();
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
@java.lang.Override
public java.util.Map getSourcePropertiesMap() {
return internalGetSourceProperties().getMap();
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
@java.lang.Override
public /* nullable */ com.google.protobuf.Value getSourcePropertiesOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetSourceProperties().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
@java.lang.Override
public com.google.protobuf.Value getSourcePropertiesOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetSourceProperties().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int SECURITY_MARKS_FIELD_NUMBER = 10;
private com.google.cloud.securitycenter.v2.SecurityMarks securityMarks_;
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the securityMarks field is set.
*/
@java.lang.Override
public boolean hasSecurityMarks() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The securityMarks.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.SecurityMarks getSecurityMarks() {
return securityMarks_ == null
? com.google.cloud.securitycenter.v2.SecurityMarks.getDefaultInstance()
: securityMarks_;
}
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.SecurityMarksOrBuilder getSecurityMarksOrBuilder() {
return securityMarks_ == null
? com.google.cloud.securitycenter.v2.SecurityMarks.getDefaultInstance()
: securityMarks_;
}
public static final int EVENT_TIME_FIELD_NUMBER = 11;
private com.google.protobuf.Timestamp eventTime_;
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*
* @return Whether the eventTime field is set.
*/
@java.lang.Override
public boolean hasEventTime() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*
* @return The eventTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getEventTime() {
return eventTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : eventTime_;
}
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getEventTimeOrBuilder() {
return eventTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : eventTime_;
}
public static final int CREATE_TIME_FIELD_NUMBER = 12;
private com.google.protobuf.Timestamp createTime_;
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the createTime field is set.
*/
@java.lang.Override
public boolean hasCreateTime() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The createTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getCreateTime() {
return createTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : createTime_;
}
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder() {
return createTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : createTime_;
}
public static final int SEVERITY_FIELD_NUMBER = 14;
private int severity_ = 0;
/**
*
*
*
* The severity of the finding. This field is managed by the source that
* writes the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.Severity severity = 14;
*
* @return The enum numeric value on the wire for severity.
*/
@java.lang.Override
public int getSeverityValue() {
return severity_;
}
/**
*
*
*
* The severity of the finding. This field is managed by the source that
* writes the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.Severity severity = 14;
*
* @return The severity.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding.Severity getSeverity() {
com.google.cloud.securitycenter.v2.Finding.Severity result =
com.google.cloud.securitycenter.v2.Finding.Severity.forNumber(severity_);
return result == null
? com.google.cloud.securitycenter.v2.Finding.Severity.UNRECOGNIZED
: result;
}
public static final int MUTE_FIELD_NUMBER = 15;
private int mute_ = 0;
/**
*
*
*
* Indicates the mute state of a finding (either muted, unmuted
* or undefined). Unlike other attributes of a finding, a finding provider
* shouldn't set the value of mute.
*
*
* .google.cloud.securitycenter.v2.Finding.Mute mute = 15;
*
* @return The enum numeric value on the wire for mute.
*/
@java.lang.Override
public int getMuteValue() {
return mute_;
}
/**
*
*
*
* Indicates the mute state of a finding (either muted, unmuted
* or undefined). Unlike other attributes of a finding, a finding provider
* shouldn't set the value of mute.
*
*
* .google.cloud.securitycenter.v2.Finding.Mute mute = 15;
*
* @return The mute.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding.Mute getMute() {
com.google.cloud.securitycenter.v2.Finding.Mute result =
com.google.cloud.securitycenter.v2.Finding.Mute.forNumber(mute_);
return result == null ? com.google.cloud.securitycenter.v2.Finding.Mute.UNRECOGNIZED : result;
}
public static final int FINDING_CLASS_FIELD_NUMBER = 16;
private int findingClass_ = 0;
/**
*
*
*
* The class of the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.FindingClass finding_class = 16;
*
* @return The enum numeric value on the wire for findingClass.
*/
@java.lang.Override
public int getFindingClassValue() {
return findingClass_;
}
/**
*
*
*
* The class of the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.FindingClass finding_class = 16;
*
* @return The findingClass.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding.FindingClass getFindingClass() {
com.google.cloud.securitycenter.v2.Finding.FindingClass result =
com.google.cloud.securitycenter.v2.Finding.FindingClass.forNumber(findingClass_);
return result == null
? com.google.cloud.securitycenter.v2.Finding.FindingClass.UNRECOGNIZED
: result;
}
public static final int INDICATOR_FIELD_NUMBER = 17;
private com.google.cloud.securitycenter.v2.Indicator indicator_;
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*
* @return Whether the indicator field is set.
*/
@java.lang.Override
public boolean hasIndicator() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*
* @return The indicator.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Indicator getIndicator() {
return indicator_ == null
? com.google.cloud.securitycenter.v2.Indicator.getDefaultInstance()
: indicator_;
}
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.IndicatorOrBuilder getIndicatorOrBuilder() {
return indicator_ == null
? com.google.cloud.securitycenter.v2.Indicator.getDefaultInstance()
: indicator_;
}
public static final int VULNERABILITY_FIELD_NUMBER = 18;
private com.google.cloud.securitycenter.v2.Vulnerability vulnerability_;
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*
* @return Whether the vulnerability field is set.
*/
@java.lang.Override
public boolean hasVulnerability() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*
* @return The vulnerability.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Vulnerability getVulnerability() {
return vulnerability_ == null
? com.google.cloud.securitycenter.v2.Vulnerability.getDefaultInstance()
: vulnerability_;
}
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.VulnerabilityOrBuilder getVulnerabilityOrBuilder() {
return vulnerability_ == null
? com.google.cloud.securitycenter.v2.Vulnerability.getDefaultInstance()
: vulnerability_;
}
public static final int MUTE_UPDATE_TIME_FIELD_NUMBER = 19;
private com.google.protobuf.Timestamp muteUpdateTime_;
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the muteUpdateTime field is set.
*/
@java.lang.Override
public boolean hasMuteUpdateTime() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The muteUpdateTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getMuteUpdateTime() {
return muteUpdateTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: muteUpdateTime_;
}
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getMuteUpdateTimeOrBuilder() {
return muteUpdateTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: muteUpdateTime_;
}
public static final int EXTERNAL_SYSTEMS_FIELD_NUMBER = 20;
private static final class ExternalSystemsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.securitycenter.v2.ExternalSystem>
defaultEntry =
com.google.protobuf.MapEntry
.
newDefaultInstance(
com.google.cloud.securitycenter.v2.FindingProto
.internal_static_google_cloud_securitycenter_v2_Finding_ExternalSystemsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.cloud.securitycenter.v2.ExternalSystem.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.cloud.securitycenter.v2.ExternalSystem>
externalSystems_;
private com.google.protobuf.MapField<
java.lang.String, com.google.cloud.securitycenter.v2.ExternalSystem>
internalGetExternalSystems() {
if (externalSystems_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ExternalSystemsDefaultEntryHolder.defaultEntry);
}
return externalSystems_;
}
public int getExternalSystemsCount() {
return internalGetExternalSystems().getMap().size();
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public boolean containsExternalSystems(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetExternalSystems().getMap().containsKey(key);
}
/** Use {@link #getExternalSystemsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getExternalSystems() {
return getExternalSystemsMap();
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public java.util.Map
getExternalSystemsMap() {
return internalGetExternalSystems().getMap();
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public /* nullable */ com.google.cloud.securitycenter.v2.ExternalSystem
getExternalSystemsOrDefault(
java.lang.String key,
/* nullable */
com.google.cloud.securitycenter.v2.ExternalSystem defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetExternalSystems().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.ExternalSystem getExternalSystemsOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetExternalSystems().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int MITRE_ATTACK_FIELD_NUMBER = 21;
private com.google.cloud.securitycenter.v2.MitreAttack mitreAttack_;
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*
* @return Whether the mitreAttack field is set.
*/
@java.lang.Override
public boolean hasMitreAttack() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*
* @return The mitreAttack.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.MitreAttack getMitreAttack() {
return mitreAttack_ == null
? com.google.cloud.securitycenter.v2.MitreAttack.getDefaultInstance()
: mitreAttack_;
}
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.MitreAttackOrBuilder getMitreAttackOrBuilder() {
return mitreAttack_ == null
? com.google.cloud.securitycenter.v2.MitreAttack.getDefaultInstance()
: mitreAttack_;
}
public static final int ACCESS_FIELD_NUMBER = 22;
private com.google.cloud.securitycenter.v2.Access access_;
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*
* @return Whether the access field is set.
*/
@java.lang.Override
public boolean hasAccess() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*
* @return The access.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Access getAccess() {
return access_ == null
? com.google.cloud.securitycenter.v2.Access.getDefaultInstance()
: access_;
}
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.AccessOrBuilder getAccessOrBuilder() {
return access_ == null
? com.google.cloud.securitycenter.v2.Access.getDefaultInstance()
: access_;
}
public static final int CONNECTIONS_FIELD_NUMBER = 23;
@SuppressWarnings("serial")
private java.util.List connections_;
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
@java.lang.Override
public java.util.List getConnectionsList() {
return connections_;
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.securitycenter.v2.ConnectionOrBuilder>
getConnectionsOrBuilderList() {
return connections_;
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
@java.lang.Override
public int getConnectionsCount() {
return connections_.size();
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Connection getConnections(int index) {
return connections_.get(index);
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.ConnectionOrBuilder getConnectionsOrBuilder(int index) {
return connections_.get(index);
}
public static final int MUTE_INITIATOR_FIELD_NUMBER = 24;
@SuppressWarnings("serial")
private volatile java.lang.Object muteInitiator_ = "";
/**
*
*
*
* Records additional information about the mute operation, for example, the
* [mute
* configuration](https://cloud.google.com/security-command-center/docs/how-to-mute-findings)
* that muted the finding and the user who muted the finding.
*
*
* string mute_initiator = 24;
*
* @return The muteInitiator.
*/
@java.lang.Override
public java.lang.String getMuteInitiator() {
java.lang.Object ref = muteInitiator_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
muteInitiator_ = s;
return s;
}
}
/**
*
*
*
* Records additional information about the mute operation, for example, the
* [mute
* configuration](https://cloud.google.com/security-command-center/docs/how-to-mute-findings)
* that muted the finding and the user who muted the finding.
*
*
* string mute_initiator = 24;
*
* @return The bytes for muteInitiator.
*/
@java.lang.Override
public com.google.protobuf.ByteString getMuteInitiatorBytes() {
java.lang.Object ref = muteInitiator_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
muteInitiator_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROCESSES_FIELD_NUMBER = 25;
@SuppressWarnings("serial")
private java.util.List processes_;
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
@java.lang.Override
public java.util.List getProcessesList() {
return processes_;
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.securitycenter.v2.ProcessOrBuilder>
getProcessesOrBuilderList() {
return processes_;
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
@java.lang.Override
public int getProcessesCount() {
return processes_.size();
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Process getProcesses(int index) {
return processes_.get(index);
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.ProcessOrBuilder getProcessesOrBuilder(int index) {
return processes_.get(index);
}
public static final int CONTACTS_FIELD_NUMBER = 26;
private static final class ContactsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.securitycenter.v2.ContactDetails>
defaultEntry =
com.google.protobuf.MapEntry
.
newDefaultInstance(
com.google.cloud.securitycenter.v2.FindingProto
.internal_static_google_cloud_securitycenter_v2_Finding_ContactsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.cloud.securitycenter.v2.ContactDetails.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.cloud.securitycenter.v2.ContactDetails>
contacts_;
private com.google.protobuf.MapField<
java.lang.String, com.google.cloud.securitycenter.v2.ContactDetails>
internalGetContacts() {
if (contacts_ == null) {
return com.google.protobuf.MapField.emptyMapField(ContactsDefaultEntryHolder.defaultEntry);
}
return contacts_;
}
public int getContactsCount() {
return internalGetContacts().getMap().size();
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public boolean containsContacts(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetContacts().getMap().containsKey(key);
}
/** Use {@link #getContactsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getContacts() {
return getContactsMap();
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public java.util.Map
getContactsMap() {
return internalGetContacts().getMap();
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public /* nullable */ com.google.cloud.securitycenter.v2.ContactDetails getContactsOrDefault(
java.lang.String key,
/* nullable */
com.google.cloud.securitycenter.v2.ContactDetails defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetContacts().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.ContactDetails getContactsOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetContacts().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int COMPLIANCES_FIELD_NUMBER = 27;
@SuppressWarnings("serial")
private java.util.List compliances_;
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
@java.lang.Override
public java.util.List getCompliancesList() {
return compliances_;
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.securitycenter.v2.ComplianceOrBuilder>
getCompliancesOrBuilderList() {
return compliances_;
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
@java.lang.Override
public int getCompliancesCount() {
return compliances_.size();
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Compliance getCompliances(int index) {
return compliances_.get(index);
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.ComplianceOrBuilder getCompliancesOrBuilder(int index) {
return compliances_.get(index);
}
public static final int PARENT_DISPLAY_NAME_FIELD_NUMBER = 29;
@SuppressWarnings("serial")
private volatile java.lang.Object parentDisplayName_ = "";
/**
*
*
*
* Output only. The human readable display name of the finding source such as
* "Event Threat Detection" or "Security Health Analytics".
*
*
* string parent_display_name = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The parentDisplayName.
*/
@java.lang.Override
public java.lang.String getParentDisplayName() {
java.lang.Object ref = parentDisplayName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
parentDisplayName_ = s;
return s;
}
}
/**
*
*
*
* Output only. The human readable display name of the finding source such as
* "Event Threat Detection" or "Security Health Analytics".
*
*
* string parent_display_name = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for parentDisplayName.
*/
@java.lang.Override
public com.google.protobuf.ByteString getParentDisplayNameBytes() {
java.lang.Object ref = parentDisplayName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
parentDisplayName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 30;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
*
*
*
* Contains more details about the finding.
*
*
* string description = 30;
*
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
*
*
* Contains more details about the finding.
*
*
* string description = 30;
*
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXFILTRATION_FIELD_NUMBER = 31;
private com.google.cloud.securitycenter.v2.Exfiltration exfiltration_;
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*
* @return Whether the exfiltration field is set.
*/
@java.lang.Override
public boolean hasExfiltration() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*
* @return The exfiltration.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Exfiltration getExfiltration() {
return exfiltration_ == null
? com.google.cloud.securitycenter.v2.Exfiltration.getDefaultInstance()
: exfiltration_;
}
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.ExfiltrationOrBuilder getExfiltrationOrBuilder() {
return exfiltration_ == null
? com.google.cloud.securitycenter.v2.Exfiltration.getDefaultInstance()
: exfiltration_;
}
public static final int IAM_BINDINGS_FIELD_NUMBER = 32;
@SuppressWarnings("serial")
private java.util.List iamBindings_;
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
@java.lang.Override
public java.util.List getIamBindingsList() {
return iamBindings_;
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.securitycenter.v2.IamBindingOrBuilder>
getIamBindingsOrBuilderList() {
return iamBindings_;
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
@java.lang.Override
public int getIamBindingsCount() {
return iamBindings_.size();
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.IamBinding getIamBindings(int index) {
return iamBindings_.get(index);
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.IamBindingOrBuilder getIamBindingsOrBuilder(int index) {
return iamBindings_.get(index);
}
public static final int NEXT_STEPS_FIELD_NUMBER = 33;
@SuppressWarnings("serial")
private volatile java.lang.Object nextSteps_ = "";
/**
*
*
*
* Steps to address the finding.
*
*
* string next_steps = 33;
*
* @return The nextSteps.
*/
@java.lang.Override
public java.lang.String getNextSteps() {
java.lang.Object ref = nextSteps_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nextSteps_ = s;
return s;
}
}
/**
*
*
*
* Steps to address the finding.
*
*
* string next_steps = 33;
*
* @return The bytes for nextSteps.
*/
@java.lang.Override
public com.google.protobuf.ByteString getNextStepsBytes() {
java.lang.Object ref = nextSteps_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
nextSteps_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MODULE_NAME_FIELD_NUMBER = 34;
@SuppressWarnings("serial")
private volatile java.lang.Object moduleName_ = "";
/**
*
*
*
* Unique identifier of the module which generated the finding.
* Example:
* folders/598186756061/securityHealthAnalyticsSettings/customModules/56799441161885
*
*
* string module_name = 34;
*
* @return The moduleName.
*/
@java.lang.Override
public java.lang.String getModuleName() {
java.lang.Object ref = moduleName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
moduleName_ = s;
return s;
}
}
/**
*
*
*
* Unique identifier of the module which generated the finding.
* Example:
* folders/598186756061/securityHealthAnalyticsSettings/customModules/56799441161885
*
*
* string module_name = 34;
*
* @return The bytes for moduleName.
*/
@java.lang.Override
public com.google.protobuf.ByteString getModuleNameBytes() {
java.lang.Object ref = moduleName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
moduleName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTAINERS_FIELD_NUMBER = 35;
@SuppressWarnings("serial")
private java.util.List containers_;
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
@java.lang.Override
public java.util.List getContainersList() {
return containers_;
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.securitycenter.v2.ContainerOrBuilder>
getContainersOrBuilderList() {
return containers_;
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
@java.lang.Override
public int getContainersCount() {
return containers_.size();
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Container getContainers(int index) {
return containers_.get(index);
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.ContainerOrBuilder getContainersOrBuilder(int index) {
return containers_.get(index);
}
public static final int KUBERNETES_FIELD_NUMBER = 36;
private com.google.cloud.securitycenter.v2.Kubernetes kubernetes_;
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*
* @return Whether the kubernetes field is set.
*/
@java.lang.Override
public boolean hasKubernetes() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*
* @return The kubernetes.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Kubernetes getKubernetes() {
return kubernetes_ == null
? com.google.cloud.securitycenter.v2.Kubernetes.getDefaultInstance()
: kubernetes_;
}
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.KubernetesOrBuilder getKubernetesOrBuilder() {
return kubernetes_ == null
? com.google.cloud.securitycenter.v2.Kubernetes.getDefaultInstance()
: kubernetes_;
}
public static final int DATABASE_FIELD_NUMBER = 37;
private com.google.cloud.securitycenter.v2.Database database_;
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*
* @return Whether the database field is set.
*/
@java.lang.Override
public boolean hasDatabase() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*
* @return The database.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Database getDatabase() {
return database_ == null
? com.google.cloud.securitycenter.v2.Database.getDefaultInstance()
: database_;
}
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.DatabaseOrBuilder getDatabaseOrBuilder() {
return database_ == null
? com.google.cloud.securitycenter.v2.Database.getDefaultInstance()
: database_;
}
public static final int ATTACK_EXPOSURE_FIELD_NUMBER = 38;
private com.google.cloud.securitycenter.v2.AttackExposure attackExposure_;
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*
* @return Whether the attackExposure field is set.
*/
@java.lang.Override
public boolean hasAttackExposure() {
return ((bitField0_ & 0x00000800) != 0);
}
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*
* @return The attackExposure.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.AttackExposure getAttackExposure() {
return attackExposure_ == null
? com.google.cloud.securitycenter.v2.AttackExposure.getDefaultInstance()
: attackExposure_;
}
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.AttackExposureOrBuilder getAttackExposureOrBuilder() {
return attackExposure_ == null
? com.google.cloud.securitycenter.v2.AttackExposure.getDefaultInstance()
: attackExposure_;
}
public static final int FILES_FIELD_NUMBER = 39;
@SuppressWarnings("serial")
private java.util.List files_;
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
@java.lang.Override
public java.util.List getFilesList() {
return files_;
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.securitycenter.v2.FileOrBuilder>
getFilesOrBuilderList() {
return files_;
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
@java.lang.Override
public int getFilesCount() {
return files_.size();
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.File getFiles(int index) {
return files_.get(index);
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.FileOrBuilder getFilesOrBuilder(int index) {
return files_.get(index);
}
public static final int CLOUD_DLP_INSPECTION_FIELD_NUMBER = 40;
private com.google.cloud.securitycenter.v2.CloudDlpInspection cloudDlpInspection_;
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*
* @return Whether the cloudDlpInspection field is set.
*/
@java.lang.Override
public boolean hasCloudDlpInspection() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*
* @return The cloudDlpInspection.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.CloudDlpInspection getCloudDlpInspection() {
return cloudDlpInspection_ == null
? com.google.cloud.securitycenter.v2.CloudDlpInspection.getDefaultInstance()
: cloudDlpInspection_;
}
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.CloudDlpInspectionOrBuilder
getCloudDlpInspectionOrBuilder() {
return cloudDlpInspection_ == null
? com.google.cloud.securitycenter.v2.CloudDlpInspection.getDefaultInstance()
: cloudDlpInspection_;
}
public static final int CLOUD_DLP_DATA_PROFILE_FIELD_NUMBER = 41;
private com.google.cloud.securitycenter.v2.CloudDlpDataProfile cloudDlpDataProfile_;
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*
* @return Whether the cloudDlpDataProfile field is set.
*/
@java.lang.Override
public boolean hasCloudDlpDataProfile() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*
* @return The cloudDlpDataProfile.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.CloudDlpDataProfile getCloudDlpDataProfile() {
return cloudDlpDataProfile_ == null
? com.google.cloud.securitycenter.v2.CloudDlpDataProfile.getDefaultInstance()
: cloudDlpDataProfile_;
}
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.CloudDlpDataProfileOrBuilder
getCloudDlpDataProfileOrBuilder() {
return cloudDlpDataProfile_ == null
? com.google.cloud.securitycenter.v2.CloudDlpDataProfile.getDefaultInstance()
: cloudDlpDataProfile_;
}
public static final int KERNEL_ROOTKIT_FIELD_NUMBER = 42;
private com.google.cloud.securitycenter.v2.KernelRootkit kernelRootkit_;
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*
* @return Whether the kernelRootkit field is set.
*/
@java.lang.Override
public boolean hasKernelRootkit() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*
* @return The kernelRootkit.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.KernelRootkit getKernelRootkit() {
return kernelRootkit_ == null
? com.google.cloud.securitycenter.v2.KernelRootkit.getDefaultInstance()
: kernelRootkit_;
}
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.KernelRootkitOrBuilder getKernelRootkitOrBuilder() {
return kernelRootkit_ == null
? com.google.cloud.securitycenter.v2.KernelRootkit.getDefaultInstance()
: kernelRootkit_;
}
public static final int ORG_POLICIES_FIELD_NUMBER = 43;
@SuppressWarnings("serial")
private java.util.List orgPolicies_;
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
@java.lang.Override
public java.util.List getOrgPoliciesList() {
return orgPolicies_;
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.securitycenter.v2.OrgPolicyOrBuilder>
getOrgPoliciesOrBuilderList() {
return orgPolicies_;
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
@java.lang.Override
public int getOrgPoliciesCount() {
return orgPolicies_.size();
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.OrgPolicy getOrgPolicies(int index) {
return orgPolicies_.get(index);
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.OrgPolicyOrBuilder getOrgPoliciesOrBuilder(int index) {
return orgPolicies_.get(index);
}
public static final int APPLICATION_FIELD_NUMBER = 45;
private com.google.cloud.securitycenter.v2.Application application_;
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*
* @return Whether the application field is set.
*/
@java.lang.Override
public boolean hasApplication() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*
* @return The application.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Application getApplication() {
return application_ == null
? com.google.cloud.securitycenter.v2.Application.getDefaultInstance()
: application_;
}
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.ApplicationOrBuilder getApplicationOrBuilder() {
return application_ == null
? com.google.cloud.securitycenter.v2.Application.getDefaultInstance()
: application_;
}
public static final int BACKUP_DISASTER_RECOVERY_FIELD_NUMBER = 47;
private com.google.cloud.securitycenter.v2.BackupDisasterRecovery backupDisasterRecovery_;
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*
* @return Whether the backupDisasterRecovery field is set.
*/
@java.lang.Override
public boolean hasBackupDisasterRecovery() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*
* @return The backupDisasterRecovery.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.BackupDisasterRecovery getBackupDisasterRecovery() {
return backupDisasterRecovery_ == null
? com.google.cloud.securitycenter.v2.BackupDisasterRecovery.getDefaultInstance()
: backupDisasterRecovery_;
}
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.BackupDisasterRecoveryOrBuilder
getBackupDisasterRecoveryOrBuilder() {
return backupDisasterRecovery_ == null
? com.google.cloud.securitycenter.v2.BackupDisasterRecovery.getDefaultInstance()
: backupDisasterRecovery_;
}
public static final int SECURITY_POSTURE_FIELD_NUMBER = 48;
private com.google.cloud.securitycenter.v2.SecurityPosture securityPosture_;
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*
* @return Whether the securityPosture field is set.
*/
@java.lang.Override
public boolean hasSecurityPosture() {
return ((bitField0_ & 0x00020000) != 0);
}
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*
* @return The securityPosture.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.SecurityPosture getSecurityPosture() {
return securityPosture_ == null
? com.google.cloud.securitycenter.v2.SecurityPosture.getDefaultInstance()
: securityPosture_;
}
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.SecurityPostureOrBuilder getSecurityPostureOrBuilder() {
return securityPosture_ == null
? com.google.cloud.securitycenter.v2.SecurityPosture.getDefaultInstance()
: securityPosture_;
}
public static final int LOG_ENTRIES_FIELD_NUMBER = 49;
@SuppressWarnings("serial")
private java.util.List logEntries_;
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
@java.lang.Override
public java.util.List getLogEntriesList() {
return logEntries_;
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.securitycenter.v2.LogEntryOrBuilder>
getLogEntriesOrBuilderList() {
return logEntries_;
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
@java.lang.Override
public int getLogEntriesCount() {
return logEntries_.size();
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.LogEntry getLogEntries(int index) {
return logEntries_.get(index);
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.LogEntryOrBuilder getLogEntriesOrBuilder(int index) {
return logEntries_.get(index);
}
public static final int LOAD_BALANCERS_FIELD_NUMBER = 50;
@SuppressWarnings("serial")
private java.util.List loadBalancers_;
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
@java.lang.Override
public java.util.List getLoadBalancersList() {
return loadBalancers_;
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
@java.lang.Override
public java.util.List extends com.google.cloud.securitycenter.v2.LoadBalancerOrBuilder>
getLoadBalancersOrBuilderList() {
return loadBalancers_;
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
@java.lang.Override
public int getLoadBalancersCount() {
return loadBalancers_.size();
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.LoadBalancer getLoadBalancers(int index) {
return loadBalancers_.get(index);
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.LoadBalancerOrBuilder getLoadBalancersOrBuilder(
int index) {
return loadBalancers_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output) throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(canonicalName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, canonicalName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(parent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, parent_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, resourceName_);
}
if (state_ != com.google.cloud.securitycenter.v2.Finding.State.STATE_UNSPECIFIED.getNumber()) {
output.writeEnum(6, state_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(category_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, category_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(externalUri_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 8, externalUri_);
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetSourceProperties(), SourcePropertiesDefaultEntryHolder.defaultEntry, 9);
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(10, getSecurityMarks());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(11, getEventTime());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(12, getCreateTime());
}
if (severity_
!= com.google.cloud.securitycenter.v2.Finding.Severity.SEVERITY_UNSPECIFIED.getNumber()) {
output.writeEnum(14, severity_);
}
if (mute_ != com.google.cloud.securitycenter.v2.Finding.Mute.MUTE_UNSPECIFIED.getNumber()) {
output.writeEnum(15, mute_);
}
if (findingClass_
!= com.google.cloud.securitycenter.v2.Finding.FindingClass.FINDING_CLASS_UNSPECIFIED
.getNumber()) {
output.writeEnum(16, findingClass_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(17, getIndicator());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(18, getVulnerability());
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(19, getMuteUpdateTime());
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetExternalSystems(), ExternalSystemsDefaultEntryHolder.defaultEntry, 20);
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(21, getMitreAttack());
}
if (((bitField0_ & 0x00000080) != 0)) {
output.writeMessage(22, getAccess());
}
for (int i = 0; i < connections_.size(); i++) {
output.writeMessage(23, connections_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(muteInitiator_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 24, muteInitiator_);
}
for (int i = 0; i < processes_.size(); i++) {
output.writeMessage(25, processes_.get(i));
}
com.google.protobuf.GeneratedMessageV3.serializeStringMapTo(
output, internalGetContacts(), ContactsDefaultEntryHolder.defaultEntry, 26);
for (int i = 0; i < compliances_.size(); i++) {
output.writeMessage(27, compliances_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(parentDisplayName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 29, parentDisplayName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 30, description_);
}
if (((bitField0_ & 0x00000100) != 0)) {
output.writeMessage(31, getExfiltration());
}
for (int i = 0; i < iamBindings_.size(); i++) {
output.writeMessage(32, iamBindings_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(nextSteps_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 33, nextSteps_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(moduleName_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 34, moduleName_);
}
for (int i = 0; i < containers_.size(); i++) {
output.writeMessage(35, containers_.get(i));
}
if (((bitField0_ & 0x00000200) != 0)) {
output.writeMessage(36, getKubernetes());
}
if (((bitField0_ & 0x00000400) != 0)) {
output.writeMessage(37, getDatabase());
}
if (((bitField0_ & 0x00000800) != 0)) {
output.writeMessage(38, getAttackExposure());
}
for (int i = 0; i < files_.size(); i++) {
output.writeMessage(39, files_.get(i));
}
if (((bitField0_ & 0x00001000) != 0)) {
output.writeMessage(40, getCloudDlpInspection());
}
if (((bitField0_ & 0x00002000) != 0)) {
output.writeMessage(41, getCloudDlpDataProfile());
}
if (((bitField0_ & 0x00004000) != 0)) {
output.writeMessage(42, getKernelRootkit());
}
for (int i = 0; i < orgPolicies_.size(); i++) {
output.writeMessage(43, orgPolicies_.get(i));
}
if (((bitField0_ & 0x00008000) != 0)) {
output.writeMessage(45, getApplication());
}
if (((bitField0_ & 0x00010000) != 0)) {
output.writeMessage(47, getBackupDisasterRecovery());
}
if (((bitField0_ & 0x00020000) != 0)) {
output.writeMessage(48, getSecurityPosture());
}
for (int i = 0; i < logEntries_.size(); i++) {
output.writeMessage(49, logEntries_.get(i));
}
for (int i = 0; i < loadBalancers_.size(); i++) {
output.writeMessage(50, loadBalancers_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(canonicalName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, canonicalName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(parent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, parent_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(resourceName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, resourceName_);
}
if (state_ != com.google.cloud.securitycenter.v2.Finding.State.STATE_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(6, state_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(category_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, category_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(externalUri_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(8, externalUri_);
}
for (java.util.Map.Entry entry :
internalGetSourceProperties().getMap().entrySet()) {
com.google.protobuf.MapEntry sourceProperties__ =
SourcePropertiesDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(9, sourceProperties__);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(10, getSecurityMarks());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(11, getEventTime());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(12, getCreateTime());
}
if (severity_
!= com.google.cloud.securitycenter.v2.Finding.Severity.SEVERITY_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(14, severity_);
}
if (mute_ != com.google.cloud.securitycenter.v2.Finding.Mute.MUTE_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(15, mute_);
}
if (findingClass_
!= com.google.cloud.securitycenter.v2.Finding.FindingClass.FINDING_CLASS_UNSPECIFIED
.getNumber()) {
size += com.google.protobuf.CodedOutputStream.computeEnumSize(16, findingClass_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(17, getIndicator());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(18, getVulnerability());
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(19, getMuteUpdateTime());
}
for (java.util.Map.Entry
entry : internalGetExternalSystems().getMap().entrySet()) {
com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.securitycenter.v2.ExternalSystem>
externalSystems__ =
ExternalSystemsDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(20, externalSystems__);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(21, getMitreAttack());
}
if (((bitField0_ & 0x00000080) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(22, getAccess());
}
for (int i = 0; i < connections_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(23, connections_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(muteInitiator_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(24, muteInitiator_);
}
for (int i = 0; i < processes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(25, processes_.get(i));
}
for (java.util.Map.Entry
entry : internalGetContacts().getMap().entrySet()) {
com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.securitycenter.v2.ContactDetails>
contacts__ =
ContactsDefaultEntryHolder.defaultEntry
.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream.computeMessageSize(26, contacts__);
}
for (int i = 0; i < compliances_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(27, compliances_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(parentDisplayName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(29, parentDisplayName_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(30, description_);
}
if (((bitField0_ & 0x00000100) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(31, getExfiltration());
}
for (int i = 0; i < iamBindings_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(32, iamBindings_.get(i));
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(nextSteps_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(33, nextSteps_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(moduleName_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(34, moduleName_);
}
for (int i = 0; i < containers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(35, containers_.get(i));
}
if (((bitField0_ & 0x00000200) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(36, getKubernetes());
}
if (((bitField0_ & 0x00000400) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(37, getDatabase());
}
if (((bitField0_ & 0x00000800) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(38, getAttackExposure());
}
for (int i = 0; i < files_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(39, files_.get(i));
}
if (((bitField0_ & 0x00001000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(40, getCloudDlpInspection());
}
if (((bitField0_ & 0x00002000) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(41, getCloudDlpDataProfile());
}
if (((bitField0_ & 0x00004000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(42, getKernelRootkit());
}
for (int i = 0; i < orgPolicies_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(43, orgPolicies_.get(i));
}
if (((bitField0_ & 0x00008000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(45, getApplication());
}
if (((bitField0_ & 0x00010000) != 0)) {
size +=
com.google.protobuf.CodedOutputStream.computeMessageSize(47, getBackupDisasterRecovery());
}
if (((bitField0_ & 0x00020000) != 0)) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(48, getSecurityPosture());
}
for (int i = 0; i < logEntries_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(49, logEntries_.get(i));
}
for (int i = 0; i < loadBalancers_.size(); i++) {
size += com.google.protobuf.CodedOutputStream.computeMessageSize(50, loadBalancers_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.cloud.securitycenter.v2.Finding)) {
return super.equals(obj);
}
com.google.cloud.securitycenter.v2.Finding other =
(com.google.cloud.securitycenter.v2.Finding) obj;
if (!getName().equals(other.getName())) return false;
if (!getCanonicalName().equals(other.getCanonicalName())) return false;
if (!getParent().equals(other.getParent())) return false;
if (!getResourceName().equals(other.getResourceName())) return false;
if (state_ != other.state_) return false;
if (!getCategory().equals(other.getCategory())) return false;
if (!getExternalUri().equals(other.getExternalUri())) return false;
if (!internalGetSourceProperties().equals(other.internalGetSourceProperties())) return false;
if (hasSecurityMarks() != other.hasSecurityMarks()) return false;
if (hasSecurityMarks()) {
if (!getSecurityMarks().equals(other.getSecurityMarks())) return false;
}
if (hasEventTime() != other.hasEventTime()) return false;
if (hasEventTime()) {
if (!getEventTime().equals(other.getEventTime())) return false;
}
if (hasCreateTime() != other.hasCreateTime()) return false;
if (hasCreateTime()) {
if (!getCreateTime().equals(other.getCreateTime())) return false;
}
if (severity_ != other.severity_) return false;
if (mute_ != other.mute_) return false;
if (findingClass_ != other.findingClass_) return false;
if (hasIndicator() != other.hasIndicator()) return false;
if (hasIndicator()) {
if (!getIndicator().equals(other.getIndicator())) return false;
}
if (hasVulnerability() != other.hasVulnerability()) return false;
if (hasVulnerability()) {
if (!getVulnerability().equals(other.getVulnerability())) return false;
}
if (hasMuteUpdateTime() != other.hasMuteUpdateTime()) return false;
if (hasMuteUpdateTime()) {
if (!getMuteUpdateTime().equals(other.getMuteUpdateTime())) return false;
}
if (!internalGetExternalSystems().equals(other.internalGetExternalSystems())) return false;
if (hasMitreAttack() != other.hasMitreAttack()) return false;
if (hasMitreAttack()) {
if (!getMitreAttack().equals(other.getMitreAttack())) return false;
}
if (hasAccess() != other.hasAccess()) return false;
if (hasAccess()) {
if (!getAccess().equals(other.getAccess())) return false;
}
if (!getConnectionsList().equals(other.getConnectionsList())) return false;
if (!getMuteInitiator().equals(other.getMuteInitiator())) return false;
if (!getProcessesList().equals(other.getProcessesList())) return false;
if (!internalGetContacts().equals(other.internalGetContacts())) return false;
if (!getCompliancesList().equals(other.getCompliancesList())) return false;
if (!getParentDisplayName().equals(other.getParentDisplayName())) return false;
if (!getDescription().equals(other.getDescription())) return false;
if (hasExfiltration() != other.hasExfiltration()) return false;
if (hasExfiltration()) {
if (!getExfiltration().equals(other.getExfiltration())) return false;
}
if (!getIamBindingsList().equals(other.getIamBindingsList())) return false;
if (!getNextSteps().equals(other.getNextSteps())) return false;
if (!getModuleName().equals(other.getModuleName())) return false;
if (!getContainersList().equals(other.getContainersList())) return false;
if (hasKubernetes() != other.hasKubernetes()) return false;
if (hasKubernetes()) {
if (!getKubernetes().equals(other.getKubernetes())) return false;
}
if (hasDatabase() != other.hasDatabase()) return false;
if (hasDatabase()) {
if (!getDatabase().equals(other.getDatabase())) return false;
}
if (hasAttackExposure() != other.hasAttackExposure()) return false;
if (hasAttackExposure()) {
if (!getAttackExposure().equals(other.getAttackExposure())) return false;
}
if (!getFilesList().equals(other.getFilesList())) return false;
if (hasCloudDlpInspection() != other.hasCloudDlpInspection()) return false;
if (hasCloudDlpInspection()) {
if (!getCloudDlpInspection().equals(other.getCloudDlpInspection())) return false;
}
if (hasCloudDlpDataProfile() != other.hasCloudDlpDataProfile()) return false;
if (hasCloudDlpDataProfile()) {
if (!getCloudDlpDataProfile().equals(other.getCloudDlpDataProfile())) return false;
}
if (hasKernelRootkit() != other.hasKernelRootkit()) return false;
if (hasKernelRootkit()) {
if (!getKernelRootkit().equals(other.getKernelRootkit())) return false;
}
if (!getOrgPoliciesList().equals(other.getOrgPoliciesList())) return false;
if (hasApplication() != other.hasApplication()) return false;
if (hasApplication()) {
if (!getApplication().equals(other.getApplication())) return false;
}
if (hasBackupDisasterRecovery() != other.hasBackupDisasterRecovery()) return false;
if (hasBackupDisasterRecovery()) {
if (!getBackupDisasterRecovery().equals(other.getBackupDisasterRecovery())) return false;
}
if (hasSecurityPosture() != other.hasSecurityPosture()) return false;
if (hasSecurityPosture()) {
if (!getSecurityPosture().equals(other.getSecurityPosture())) return false;
}
if (!getLogEntriesList().equals(other.getLogEntriesList())) return false;
if (!getLoadBalancersList().equals(other.getLoadBalancersList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + CANONICAL_NAME_FIELD_NUMBER;
hash = (53 * hash) + getCanonicalName().hashCode();
hash = (37 * hash) + PARENT_FIELD_NUMBER;
hash = (53 * hash) + getParent().hashCode();
hash = (37 * hash) + RESOURCE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getResourceName().hashCode();
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + state_;
hash = (37 * hash) + CATEGORY_FIELD_NUMBER;
hash = (53 * hash) + getCategory().hashCode();
hash = (37 * hash) + EXTERNAL_URI_FIELD_NUMBER;
hash = (53 * hash) + getExternalUri().hashCode();
if (!internalGetSourceProperties().getMap().isEmpty()) {
hash = (37 * hash) + SOURCE_PROPERTIES_FIELD_NUMBER;
hash = (53 * hash) + internalGetSourceProperties().hashCode();
}
if (hasSecurityMarks()) {
hash = (37 * hash) + SECURITY_MARKS_FIELD_NUMBER;
hash = (53 * hash) + getSecurityMarks().hashCode();
}
if (hasEventTime()) {
hash = (37 * hash) + EVENT_TIME_FIELD_NUMBER;
hash = (53 * hash) + getEventTime().hashCode();
}
if (hasCreateTime()) {
hash = (37 * hash) + CREATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getCreateTime().hashCode();
}
hash = (37 * hash) + SEVERITY_FIELD_NUMBER;
hash = (53 * hash) + severity_;
hash = (37 * hash) + MUTE_FIELD_NUMBER;
hash = (53 * hash) + mute_;
hash = (37 * hash) + FINDING_CLASS_FIELD_NUMBER;
hash = (53 * hash) + findingClass_;
if (hasIndicator()) {
hash = (37 * hash) + INDICATOR_FIELD_NUMBER;
hash = (53 * hash) + getIndicator().hashCode();
}
if (hasVulnerability()) {
hash = (37 * hash) + VULNERABILITY_FIELD_NUMBER;
hash = (53 * hash) + getVulnerability().hashCode();
}
if (hasMuteUpdateTime()) {
hash = (37 * hash) + MUTE_UPDATE_TIME_FIELD_NUMBER;
hash = (53 * hash) + getMuteUpdateTime().hashCode();
}
if (!internalGetExternalSystems().getMap().isEmpty()) {
hash = (37 * hash) + EXTERNAL_SYSTEMS_FIELD_NUMBER;
hash = (53 * hash) + internalGetExternalSystems().hashCode();
}
if (hasMitreAttack()) {
hash = (37 * hash) + MITRE_ATTACK_FIELD_NUMBER;
hash = (53 * hash) + getMitreAttack().hashCode();
}
if (hasAccess()) {
hash = (37 * hash) + ACCESS_FIELD_NUMBER;
hash = (53 * hash) + getAccess().hashCode();
}
if (getConnectionsCount() > 0) {
hash = (37 * hash) + CONNECTIONS_FIELD_NUMBER;
hash = (53 * hash) + getConnectionsList().hashCode();
}
hash = (37 * hash) + MUTE_INITIATOR_FIELD_NUMBER;
hash = (53 * hash) + getMuteInitiator().hashCode();
if (getProcessesCount() > 0) {
hash = (37 * hash) + PROCESSES_FIELD_NUMBER;
hash = (53 * hash) + getProcessesList().hashCode();
}
if (!internalGetContacts().getMap().isEmpty()) {
hash = (37 * hash) + CONTACTS_FIELD_NUMBER;
hash = (53 * hash) + internalGetContacts().hashCode();
}
if (getCompliancesCount() > 0) {
hash = (37 * hash) + COMPLIANCES_FIELD_NUMBER;
hash = (53 * hash) + getCompliancesList().hashCode();
}
hash = (37 * hash) + PARENT_DISPLAY_NAME_FIELD_NUMBER;
hash = (53 * hash) + getParentDisplayName().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
if (hasExfiltration()) {
hash = (37 * hash) + EXFILTRATION_FIELD_NUMBER;
hash = (53 * hash) + getExfiltration().hashCode();
}
if (getIamBindingsCount() > 0) {
hash = (37 * hash) + IAM_BINDINGS_FIELD_NUMBER;
hash = (53 * hash) + getIamBindingsList().hashCode();
}
hash = (37 * hash) + NEXT_STEPS_FIELD_NUMBER;
hash = (53 * hash) + getNextSteps().hashCode();
hash = (37 * hash) + MODULE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getModuleName().hashCode();
if (getContainersCount() > 0) {
hash = (37 * hash) + CONTAINERS_FIELD_NUMBER;
hash = (53 * hash) + getContainersList().hashCode();
}
if (hasKubernetes()) {
hash = (37 * hash) + KUBERNETES_FIELD_NUMBER;
hash = (53 * hash) + getKubernetes().hashCode();
}
if (hasDatabase()) {
hash = (37 * hash) + DATABASE_FIELD_NUMBER;
hash = (53 * hash) + getDatabase().hashCode();
}
if (hasAttackExposure()) {
hash = (37 * hash) + ATTACK_EXPOSURE_FIELD_NUMBER;
hash = (53 * hash) + getAttackExposure().hashCode();
}
if (getFilesCount() > 0) {
hash = (37 * hash) + FILES_FIELD_NUMBER;
hash = (53 * hash) + getFilesList().hashCode();
}
if (hasCloudDlpInspection()) {
hash = (37 * hash) + CLOUD_DLP_INSPECTION_FIELD_NUMBER;
hash = (53 * hash) + getCloudDlpInspection().hashCode();
}
if (hasCloudDlpDataProfile()) {
hash = (37 * hash) + CLOUD_DLP_DATA_PROFILE_FIELD_NUMBER;
hash = (53 * hash) + getCloudDlpDataProfile().hashCode();
}
if (hasKernelRootkit()) {
hash = (37 * hash) + KERNEL_ROOTKIT_FIELD_NUMBER;
hash = (53 * hash) + getKernelRootkit().hashCode();
}
if (getOrgPoliciesCount() > 0) {
hash = (37 * hash) + ORG_POLICIES_FIELD_NUMBER;
hash = (53 * hash) + getOrgPoliciesList().hashCode();
}
if (hasApplication()) {
hash = (37 * hash) + APPLICATION_FIELD_NUMBER;
hash = (53 * hash) + getApplication().hashCode();
}
if (hasBackupDisasterRecovery()) {
hash = (37 * hash) + BACKUP_DISASTER_RECOVERY_FIELD_NUMBER;
hash = (53 * hash) + getBackupDisasterRecovery().hashCode();
}
if (hasSecurityPosture()) {
hash = (37 * hash) + SECURITY_POSTURE_FIELD_NUMBER;
hash = (53 * hash) + getSecurityPosture().hashCode();
}
if (getLogEntriesCount() > 0) {
hash = (37 * hash) + LOG_ENTRIES_FIELD_NUMBER;
hash = (53 * hash) + getLogEntriesList().hashCode();
}
if (getLoadBalancersCount() > 0) {
hash = (37 * hash) + LOAD_BALANCERS_FIELD_NUMBER;
hash = (53 * hash) + getLoadBalancersList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.cloud.securitycenter.v2.Finding parseFrom(java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.securitycenter.v2.Finding parseFrom(
java.nio.ByteBuffer data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.securitycenter.v2.Finding parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.securitycenter.v2.Finding parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.securitycenter.v2.Finding parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.cloud.securitycenter.v2.Finding parseFrom(
byte[] data, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.cloud.securitycenter.v2.Finding parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.securitycenter.v2.Finding parseFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.securitycenter.v2.Finding parseDelimitedFrom(
java.io.InputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.cloud.securitycenter.v2.Finding parseDelimitedFrom(
java.io.InputStream input, com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseDelimitedWithIOException(
PARSER, input, extensionRegistry);
}
public static com.google.cloud.securitycenter.v2.Finding parseFrom(
com.google.protobuf.CodedInputStream input) throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(PARSER, input);
}
public static com.google.cloud.securitycenter.v2.Finding parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3.parseWithIOException(
PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() {
return newBuilder();
}
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.cloud.securitycenter.v2.Finding prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE ? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
*
*
* Security Command Center finding.
*
* A finding is a record of assessment data like security, risk, health, or
* privacy, that is ingested into Security Command Center for presentation,
* notification, analysis, policy testing, and enforcement. For example, a
* cross-site scripting (XSS) vulnerability in an App Engine application is a
* finding.
*
*
* Protobuf type {@code google.cloud.securitycenter.v2.Finding}
*/
public static final class Builder extends com.google.protobuf.GeneratedMessageV3.Builder
implements
// @@protoc_insertion_point(builder_implements:google.cloud.securitycenter.v2.Finding)
com.google.cloud.securitycenter.v2.FindingOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor getDescriptor() {
return com.google.cloud.securitycenter.v2.FindingProto
.internal_static_google_cloud_securitycenter_v2_Finding_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 9:
return internalGetSourceProperties();
case 20:
return internalGetExternalSystems();
case 26:
return internalGetContacts();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 9:
return internalGetMutableSourceProperties();
case 20:
return internalGetMutableExternalSystems();
case 26:
return internalGetMutableContacts();
default:
throw new RuntimeException("Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.cloud.securitycenter.v2.FindingProto
.internal_static_google_cloud_securitycenter_v2_Finding_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.cloud.securitycenter.v2.Finding.class,
com.google.cloud.securitycenter.v2.Finding.Builder.class);
}
// Construct using com.google.cloud.securitycenter.v2.Finding.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders) {
getSecurityMarksFieldBuilder();
getEventTimeFieldBuilder();
getCreateTimeFieldBuilder();
getIndicatorFieldBuilder();
getVulnerabilityFieldBuilder();
getMuteUpdateTimeFieldBuilder();
getMitreAttackFieldBuilder();
getAccessFieldBuilder();
getConnectionsFieldBuilder();
getProcessesFieldBuilder();
getCompliancesFieldBuilder();
getExfiltrationFieldBuilder();
getIamBindingsFieldBuilder();
getContainersFieldBuilder();
getKubernetesFieldBuilder();
getDatabaseFieldBuilder();
getAttackExposureFieldBuilder();
getFilesFieldBuilder();
getCloudDlpInspectionFieldBuilder();
getCloudDlpDataProfileFieldBuilder();
getKernelRootkitFieldBuilder();
getOrgPoliciesFieldBuilder();
getApplicationFieldBuilder();
getBackupDisasterRecoveryFieldBuilder();
getSecurityPostureFieldBuilder();
getLogEntriesFieldBuilder();
getLoadBalancersFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
bitField1_ = 0;
name_ = "";
canonicalName_ = "";
parent_ = "";
resourceName_ = "";
state_ = 0;
category_ = "";
externalUri_ = "";
internalGetMutableSourceProperties().clear();
securityMarks_ = null;
if (securityMarksBuilder_ != null) {
securityMarksBuilder_.dispose();
securityMarksBuilder_ = null;
}
eventTime_ = null;
if (eventTimeBuilder_ != null) {
eventTimeBuilder_.dispose();
eventTimeBuilder_ = null;
}
createTime_ = null;
if (createTimeBuilder_ != null) {
createTimeBuilder_.dispose();
createTimeBuilder_ = null;
}
severity_ = 0;
mute_ = 0;
findingClass_ = 0;
indicator_ = null;
if (indicatorBuilder_ != null) {
indicatorBuilder_.dispose();
indicatorBuilder_ = null;
}
vulnerability_ = null;
if (vulnerabilityBuilder_ != null) {
vulnerabilityBuilder_.dispose();
vulnerabilityBuilder_ = null;
}
muteUpdateTime_ = null;
if (muteUpdateTimeBuilder_ != null) {
muteUpdateTimeBuilder_.dispose();
muteUpdateTimeBuilder_ = null;
}
internalGetMutableExternalSystems().clear();
mitreAttack_ = null;
if (mitreAttackBuilder_ != null) {
mitreAttackBuilder_.dispose();
mitreAttackBuilder_ = null;
}
access_ = null;
if (accessBuilder_ != null) {
accessBuilder_.dispose();
accessBuilder_ = null;
}
if (connectionsBuilder_ == null) {
connections_ = java.util.Collections.emptyList();
} else {
connections_ = null;
connectionsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00100000);
muteInitiator_ = "";
if (processesBuilder_ == null) {
processes_ = java.util.Collections.emptyList();
} else {
processes_ = null;
processesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00400000);
internalGetMutableContacts().clear();
if (compliancesBuilder_ == null) {
compliances_ = java.util.Collections.emptyList();
} else {
compliances_ = null;
compliancesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x01000000);
parentDisplayName_ = "";
description_ = "";
exfiltration_ = null;
if (exfiltrationBuilder_ != null) {
exfiltrationBuilder_.dispose();
exfiltrationBuilder_ = null;
}
if (iamBindingsBuilder_ == null) {
iamBindings_ = java.util.Collections.emptyList();
} else {
iamBindings_ = null;
iamBindingsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x10000000);
nextSteps_ = "";
moduleName_ = "";
if (containersBuilder_ == null) {
containers_ = java.util.Collections.emptyList();
} else {
containers_ = null;
containersBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x80000000);
kubernetes_ = null;
if (kubernetesBuilder_ != null) {
kubernetesBuilder_.dispose();
kubernetesBuilder_ = null;
}
database_ = null;
if (databaseBuilder_ != null) {
databaseBuilder_.dispose();
databaseBuilder_ = null;
}
attackExposure_ = null;
if (attackExposureBuilder_ != null) {
attackExposureBuilder_.dispose();
attackExposureBuilder_ = null;
}
if (filesBuilder_ == null) {
files_ = java.util.Collections.emptyList();
} else {
files_ = null;
filesBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000008);
cloudDlpInspection_ = null;
if (cloudDlpInspectionBuilder_ != null) {
cloudDlpInspectionBuilder_.dispose();
cloudDlpInspectionBuilder_ = null;
}
cloudDlpDataProfile_ = null;
if (cloudDlpDataProfileBuilder_ != null) {
cloudDlpDataProfileBuilder_.dispose();
cloudDlpDataProfileBuilder_ = null;
}
kernelRootkit_ = null;
if (kernelRootkitBuilder_ != null) {
kernelRootkitBuilder_.dispose();
kernelRootkitBuilder_ = null;
}
if (orgPoliciesBuilder_ == null) {
orgPolicies_ = java.util.Collections.emptyList();
} else {
orgPolicies_ = null;
orgPoliciesBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000080);
application_ = null;
if (applicationBuilder_ != null) {
applicationBuilder_.dispose();
applicationBuilder_ = null;
}
backupDisasterRecovery_ = null;
if (backupDisasterRecoveryBuilder_ != null) {
backupDisasterRecoveryBuilder_.dispose();
backupDisasterRecoveryBuilder_ = null;
}
securityPosture_ = null;
if (securityPostureBuilder_ != null) {
securityPostureBuilder_.dispose();
securityPostureBuilder_ = null;
}
if (logEntriesBuilder_ == null) {
logEntries_ = java.util.Collections.emptyList();
} else {
logEntries_ = null;
logEntriesBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000800);
if (loadBalancersBuilder_ == null) {
loadBalancers_ = java.util.Collections.emptyList();
} else {
loadBalancers_ = null;
loadBalancersBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00001000);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor getDescriptorForType() {
return com.google.cloud.securitycenter.v2.FindingProto
.internal_static_google_cloud_securitycenter_v2_Finding_descriptor;
}
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding getDefaultInstanceForType() {
return com.google.cloud.securitycenter.v2.Finding.getDefaultInstance();
}
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding build() {
com.google.cloud.securitycenter.v2.Finding result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding buildPartial() {
com.google.cloud.securitycenter.v2.Finding result =
new com.google.cloud.securitycenter.v2.Finding(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) {
buildPartial0(result);
}
if (bitField1_ != 0) {
buildPartial1(result);
}
onBuilt();
return result;
}
private void buildPartialRepeatedFields(com.google.cloud.securitycenter.v2.Finding result) {
if (connectionsBuilder_ == null) {
if (((bitField0_ & 0x00100000) != 0)) {
connections_ = java.util.Collections.unmodifiableList(connections_);
bitField0_ = (bitField0_ & ~0x00100000);
}
result.connections_ = connections_;
} else {
result.connections_ = connectionsBuilder_.build();
}
if (processesBuilder_ == null) {
if (((bitField0_ & 0x00400000) != 0)) {
processes_ = java.util.Collections.unmodifiableList(processes_);
bitField0_ = (bitField0_ & ~0x00400000);
}
result.processes_ = processes_;
} else {
result.processes_ = processesBuilder_.build();
}
if (compliancesBuilder_ == null) {
if (((bitField0_ & 0x01000000) != 0)) {
compliances_ = java.util.Collections.unmodifiableList(compliances_);
bitField0_ = (bitField0_ & ~0x01000000);
}
result.compliances_ = compliances_;
} else {
result.compliances_ = compliancesBuilder_.build();
}
if (iamBindingsBuilder_ == null) {
if (((bitField0_ & 0x10000000) != 0)) {
iamBindings_ = java.util.Collections.unmodifiableList(iamBindings_);
bitField0_ = (bitField0_ & ~0x10000000);
}
result.iamBindings_ = iamBindings_;
} else {
result.iamBindings_ = iamBindingsBuilder_.build();
}
if (containersBuilder_ == null) {
if (((bitField0_ & 0x80000000) != 0)) {
containers_ = java.util.Collections.unmodifiableList(containers_);
bitField0_ = (bitField0_ & ~0x80000000);
}
result.containers_ = containers_;
} else {
result.containers_ = containersBuilder_.build();
}
if (filesBuilder_ == null) {
if (((bitField1_ & 0x00000008) != 0)) {
files_ = java.util.Collections.unmodifiableList(files_);
bitField1_ = (bitField1_ & ~0x00000008);
}
result.files_ = files_;
} else {
result.files_ = filesBuilder_.build();
}
if (orgPoliciesBuilder_ == null) {
if (((bitField1_ & 0x00000080) != 0)) {
orgPolicies_ = java.util.Collections.unmodifiableList(orgPolicies_);
bitField1_ = (bitField1_ & ~0x00000080);
}
result.orgPolicies_ = orgPolicies_;
} else {
result.orgPolicies_ = orgPoliciesBuilder_.build();
}
if (logEntriesBuilder_ == null) {
if (((bitField1_ & 0x00000800) != 0)) {
logEntries_ = java.util.Collections.unmodifiableList(logEntries_);
bitField1_ = (bitField1_ & ~0x00000800);
}
result.logEntries_ = logEntries_;
} else {
result.logEntries_ = logEntriesBuilder_.build();
}
if (loadBalancersBuilder_ == null) {
if (((bitField1_ & 0x00001000) != 0)) {
loadBalancers_ = java.util.Collections.unmodifiableList(loadBalancers_);
bitField1_ = (bitField1_ & ~0x00001000);
}
result.loadBalancers_ = loadBalancers_;
} else {
result.loadBalancers_ = loadBalancersBuilder_.build();
}
}
private void buildPartial0(com.google.cloud.securitycenter.v2.Finding result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.name_ = name_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.canonicalName_ = canonicalName_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.parent_ = parent_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.resourceName_ = resourceName_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.state_ = state_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.category_ = category_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.externalUri_ = externalUri_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.sourceProperties_ =
internalGetSourceProperties().build(SourcePropertiesDefaultEntryHolder.defaultEntry);
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000100) != 0)) {
result.securityMarks_ =
securityMarksBuilder_ == null ? securityMarks_ : securityMarksBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.eventTime_ = eventTimeBuilder_ == null ? eventTime_ : eventTimeBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.createTime_ = createTimeBuilder_ == null ? createTime_ : createTimeBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.severity_ = severity_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.mute_ = mute_;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.findingClass_ = findingClass_;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.indicator_ = indicatorBuilder_ == null ? indicator_ : indicatorBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.vulnerability_ =
vulnerabilityBuilder_ == null ? vulnerability_ : vulnerabilityBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.muteUpdateTime_ =
muteUpdateTimeBuilder_ == null ? muteUpdateTime_ : muteUpdateTimeBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.externalSystems_ =
internalGetExternalSystems().build(ExternalSystemsDefaultEntryHolder.defaultEntry);
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.mitreAttack_ =
mitreAttackBuilder_ == null ? mitreAttack_ : mitreAttackBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.access_ = accessBuilder_ == null ? access_ : accessBuilder_.build();
to_bitField0_ |= 0x00000080;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.muteInitiator_ = muteInitiator_;
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.contacts_ = internalGetContacts().build(ContactsDefaultEntryHolder.defaultEntry);
}
if (((from_bitField0_ & 0x02000000) != 0)) {
result.parentDisplayName_ = parentDisplayName_;
}
if (((from_bitField0_ & 0x04000000) != 0)) {
result.description_ = description_;
}
if (((from_bitField0_ & 0x08000000) != 0)) {
result.exfiltration_ =
exfiltrationBuilder_ == null ? exfiltration_ : exfiltrationBuilder_.build();
to_bitField0_ |= 0x00000100;
}
if (((from_bitField0_ & 0x20000000) != 0)) {
result.nextSteps_ = nextSteps_;
}
if (((from_bitField0_ & 0x40000000) != 0)) {
result.moduleName_ = moduleName_;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartial1(com.google.cloud.securitycenter.v2.Finding result) {
int from_bitField1_ = bitField1_;
int to_bitField0_ = 0;
if (((from_bitField1_ & 0x00000001) != 0)) {
result.kubernetes_ = kubernetesBuilder_ == null ? kubernetes_ : kubernetesBuilder_.build();
to_bitField0_ |= 0x00000200;
}
if (((from_bitField1_ & 0x00000002) != 0)) {
result.database_ = databaseBuilder_ == null ? database_ : databaseBuilder_.build();
to_bitField0_ |= 0x00000400;
}
if (((from_bitField1_ & 0x00000004) != 0)) {
result.attackExposure_ =
attackExposureBuilder_ == null ? attackExposure_ : attackExposureBuilder_.build();
to_bitField0_ |= 0x00000800;
}
if (((from_bitField1_ & 0x00000010) != 0)) {
result.cloudDlpInspection_ =
cloudDlpInspectionBuilder_ == null
? cloudDlpInspection_
: cloudDlpInspectionBuilder_.build();
to_bitField0_ |= 0x00001000;
}
if (((from_bitField1_ & 0x00000020) != 0)) {
result.cloudDlpDataProfile_ =
cloudDlpDataProfileBuilder_ == null
? cloudDlpDataProfile_
: cloudDlpDataProfileBuilder_.build();
to_bitField0_ |= 0x00002000;
}
if (((from_bitField1_ & 0x00000040) != 0)) {
result.kernelRootkit_ =
kernelRootkitBuilder_ == null ? kernelRootkit_ : kernelRootkitBuilder_.build();
to_bitField0_ |= 0x00004000;
}
if (((from_bitField1_ & 0x00000100) != 0)) {
result.application_ =
applicationBuilder_ == null ? application_ : applicationBuilder_.build();
to_bitField0_ |= 0x00008000;
}
if (((from_bitField1_ & 0x00000200) != 0)) {
result.backupDisasterRecovery_ =
backupDisasterRecoveryBuilder_ == null
? backupDisasterRecovery_
: backupDisasterRecoveryBuilder_.build();
to_bitField0_ |= 0x00010000;
}
if (((from_bitField1_ & 0x00000400) != 0)) {
result.securityPosture_ =
securityPostureBuilder_ == null ? securityPosture_ : securityPostureBuilder_.build();
to_bitField0_ |= 0x00020000;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field, java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.cloud.securitycenter.v2.Finding) {
return mergeFrom((com.google.cloud.securitycenter.v2.Finding) other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.cloud.securitycenter.v2.Finding other) {
if (other == com.google.cloud.securitycenter.v2.Finding.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getCanonicalName().isEmpty()) {
canonicalName_ = other.canonicalName_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.getParent().isEmpty()) {
parent_ = other.parent_;
bitField0_ |= 0x00000004;
onChanged();
}
if (!other.getResourceName().isEmpty()) {
resourceName_ = other.resourceName_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.state_ != 0) {
setStateValue(other.getStateValue());
}
if (!other.getCategory().isEmpty()) {
category_ = other.category_;
bitField0_ |= 0x00000020;
onChanged();
}
if (!other.getExternalUri().isEmpty()) {
externalUri_ = other.externalUri_;
bitField0_ |= 0x00000040;
onChanged();
}
internalGetMutableSourceProperties().mergeFrom(other.internalGetSourceProperties());
bitField0_ |= 0x00000080;
if (other.hasSecurityMarks()) {
mergeSecurityMarks(other.getSecurityMarks());
}
if (other.hasEventTime()) {
mergeEventTime(other.getEventTime());
}
if (other.hasCreateTime()) {
mergeCreateTime(other.getCreateTime());
}
if (other.severity_ != 0) {
setSeverityValue(other.getSeverityValue());
}
if (other.mute_ != 0) {
setMuteValue(other.getMuteValue());
}
if (other.findingClass_ != 0) {
setFindingClassValue(other.getFindingClassValue());
}
if (other.hasIndicator()) {
mergeIndicator(other.getIndicator());
}
if (other.hasVulnerability()) {
mergeVulnerability(other.getVulnerability());
}
if (other.hasMuteUpdateTime()) {
mergeMuteUpdateTime(other.getMuteUpdateTime());
}
internalGetMutableExternalSystems().mergeFrom(other.internalGetExternalSystems());
bitField0_ |= 0x00020000;
if (other.hasMitreAttack()) {
mergeMitreAttack(other.getMitreAttack());
}
if (other.hasAccess()) {
mergeAccess(other.getAccess());
}
if (connectionsBuilder_ == null) {
if (!other.connections_.isEmpty()) {
if (connections_.isEmpty()) {
connections_ = other.connections_;
bitField0_ = (bitField0_ & ~0x00100000);
} else {
ensureConnectionsIsMutable();
connections_.addAll(other.connections_);
}
onChanged();
}
} else {
if (!other.connections_.isEmpty()) {
if (connectionsBuilder_.isEmpty()) {
connectionsBuilder_.dispose();
connectionsBuilder_ = null;
connections_ = other.connections_;
bitField0_ = (bitField0_ & ~0x00100000);
connectionsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getConnectionsFieldBuilder()
: null;
} else {
connectionsBuilder_.addAllMessages(other.connections_);
}
}
}
if (!other.getMuteInitiator().isEmpty()) {
muteInitiator_ = other.muteInitiator_;
bitField0_ |= 0x00200000;
onChanged();
}
if (processesBuilder_ == null) {
if (!other.processes_.isEmpty()) {
if (processes_.isEmpty()) {
processes_ = other.processes_;
bitField0_ = (bitField0_ & ~0x00400000);
} else {
ensureProcessesIsMutable();
processes_.addAll(other.processes_);
}
onChanged();
}
} else {
if (!other.processes_.isEmpty()) {
if (processesBuilder_.isEmpty()) {
processesBuilder_.dispose();
processesBuilder_ = null;
processes_ = other.processes_;
bitField0_ = (bitField0_ & ~0x00400000);
processesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getProcessesFieldBuilder()
: null;
} else {
processesBuilder_.addAllMessages(other.processes_);
}
}
}
internalGetMutableContacts().mergeFrom(other.internalGetContacts());
bitField0_ |= 0x00800000;
if (compliancesBuilder_ == null) {
if (!other.compliances_.isEmpty()) {
if (compliances_.isEmpty()) {
compliances_ = other.compliances_;
bitField0_ = (bitField0_ & ~0x01000000);
} else {
ensureCompliancesIsMutable();
compliances_.addAll(other.compliances_);
}
onChanged();
}
} else {
if (!other.compliances_.isEmpty()) {
if (compliancesBuilder_.isEmpty()) {
compliancesBuilder_.dispose();
compliancesBuilder_ = null;
compliances_ = other.compliances_;
bitField0_ = (bitField0_ & ~0x01000000);
compliancesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getCompliancesFieldBuilder()
: null;
} else {
compliancesBuilder_.addAllMessages(other.compliances_);
}
}
}
if (!other.getParentDisplayName().isEmpty()) {
parentDisplayName_ = other.parentDisplayName_;
bitField0_ |= 0x02000000;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
bitField0_ |= 0x04000000;
onChanged();
}
if (other.hasExfiltration()) {
mergeExfiltration(other.getExfiltration());
}
if (iamBindingsBuilder_ == null) {
if (!other.iamBindings_.isEmpty()) {
if (iamBindings_.isEmpty()) {
iamBindings_ = other.iamBindings_;
bitField0_ = (bitField0_ & ~0x10000000);
} else {
ensureIamBindingsIsMutable();
iamBindings_.addAll(other.iamBindings_);
}
onChanged();
}
} else {
if (!other.iamBindings_.isEmpty()) {
if (iamBindingsBuilder_.isEmpty()) {
iamBindingsBuilder_.dispose();
iamBindingsBuilder_ = null;
iamBindings_ = other.iamBindings_;
bitField0_ = (bitField0_ & ~0x10000000);
iamBindingsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getIamBindingsFieldBuilder()
: null;
} else {
iamBindingsBuilder_.addAllMessages(other.iamBindings_);
}
}
}
if (!other.getNextSteps().isEmpty()) {
nextSteps_ = other.nextSteps_;
bitField0_ |= 0x20000000;
onChanged();
}
if (!other.getModuleName().isEmpty()) {
moduleName_ = other.moduleName_;
bitField0_ |= 0x40000000;
onChanged();
}
if (containersBuilder_ == null) {
if (!other.containers_.isEmpty()) {
if (containers_.isEmpty()) {
containers_ = other.containers_;
bitField0_ = (bitField0_ & ~0x80000000);
} else {
ensureContainersIsMutable();
containers_.addAll(other.containers_);
}
onChanged();
}
} else {
if (!other.containers_.isEmpty()) {
if (containersBuilder_.isEmpty()) {
containersBuilder_.dispose();
containersBuilder_ = null;
containers_ = other.containers_;
bitField0_ = (bitField0_ & ~0x80000000);
containersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getContainersFieldBuilder()
: null;
} else {
containersBuilder_.addAllMessages(other.containers_);
}
}
}
if (other.hasKubernetes()) {
mergeKubernetes(other.getKubernetes());
}
if (other.hasDatabase()) {
mergeDatabase(other.getDatabase());
}
if (other.hasAttackExposure()) {
mergeAttackExposure(other.getAttackExposure());
}
if (filesBuilder_ == null) {
if (!other.files_.isEmpty()) {
if (files_.isEmpty()) {
files_ = other.files_;
bitField1_ = (bitField1_ & ~0x00000008);
} else {
ensureFilesIsMutable();
files_.addAll(other.files_);
}
onChanged();
}
} else {
if (!other.files_.isEmpty()) {
if (filesBuilder_.isEmpty()) {
filesBuilder_.dispose();
filesBuilder_ = null;
files_ = other.files_;
bitField1_ = (bitField1_ & ~0x00000008);
filesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getFilesFieldBuilder()
: null;
} else {
filesBuilder_.addAllMessages(other.files_);
}
}
}
if (other.hasCloudDlpInspection()) {
mergeCloudDlpInspection(other.getCloudDlpInspection());
}
if (other.hasCloudDlpDataProfile()) {
mergeCloudDlpDataProfile(other.getCloudDlpDataProfile());
}
if (other.hasKernelRootkit()) {
mergeKernelRootkit(other.getKernelRootkit());
}
if (orgPoliciesBuilder_ == null) {
if (!other.orgPolicies_.isEmpty()) {
if (orgPolicies_.isEmpty()) {
orgPolicies_ = other.orgPolicies_;
bitField1_ = (bitField1_ & ~0x00000080);
} else {
ensureOrgPoliciesIsMutable();
orgPolicies_.addAll(other.orgPolicies_);
}
onChanged();
}
} else {
if (!other.orgPolicies_.isEmpty()) {
if (orgPoliciesBuilder_.isEmpty()) {
orgPoliciesBuilder_.dispose();
orgPoliciesBuilder_ = null;
orgPolicies_ = other.orgPolicies_;
bitField1_ = (bitField1_ & ~0x00000080);
orgPoliciesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getOrgPoliciesFieldBuilder()
: null;
} else {
orgPoliciesBuilder_.addAllMessages(other.orgPolicies_);
}
}
}
if (other.hasApplication()) {
mergeApplication(other.getApplication());
}
if (other.hasBackupDisasterRecovery()) {
mergeBackupDisasterRecovery(other.getBackupDisasterRecovery());
}
if (other.hasSecurityPosture()) {
mergeSecurityPosture(other.getSecurityPosture());
}
if (logEntriesBuilder_ == null) {
if (!other.logEntries_.isEmpty()) {
if (logEntries_.isEmpty()) {
logEntries_ = other.logEntries_;
bitField1_ = (bitField1_ & ~0x00000800);
} else {
ensureLogEntriesIsMutable();
logEntries_.addAll(other.logEntries_);
}
onChanged();
}
} else {
if (!other.logEntries_.isEmpty()) {
if (logEntriesBuilder_.isEmpty()) {
logEntriesBuilder_.dispose();
logEntriesBuilder_ = null;
logEntries_ = other.logEntries_;
bitField1_ = (bitField1_ & ~0x00000800);
logEntriesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getLogEntriesFieldBuilder()
: null;
} else {
logEntriesBuilder_.addAllMessages(other.logEntries_);
}
}
}
if (loadBalancersBuilder_ == null) {
if (!other.loadBalancers_.isEmpty()) {
if (loadBalancers_.isEmpty()) {
loadBalancers_ = other.loadBalancers_;
bitField1_ = (bitField1_ & ~0x00001000);
} else {
ensureLoadBalancersIsMutable();
loadBalancers_.addAll(other.loadBalancers_);
}
onChanged();
}
} else {
if (!other.loadBalancers_.isEmpty()) {
if (loadBalancersBuilder_.isEmpty()) {
loadBalancersBuilder_.dispose();
loadBalancersBuilder_ = null;
loadBalancers_ = other.loadBalancers_;
bitField1_ = (bitField1_ & ~0x00001000);
loadBalancersBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders
? getLoadBalancersFieldBuilder()
: null;
} else {
loadBalancersBuilder_.addAllMessages(other.loadBalancers_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10:
{
name_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18:
{
canonicalName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26:
{
parent_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34:
{
resourceName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 34
case 48:
{
state_ = input.readEnum();
bitField0_ |= 0x00000010;
break;
} // case 48
case 58:
{
category_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 58
case 66:
{
externalUri_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 66
case 74:
{
com.google.protobuf.MapEntry
sourceProperties__ =
input.readMessage(
SourcePropertiesDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableSourceProperties()
.ensureBuilderMap()
.put(sourceProperties__.getKey(), sourceProperties__.getValue());
bitField0_ |= 0x00000080;
break;
} // case 74
case 82:
{
input.readMessage(getSecurityMarksFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000100;
break;
} // case 82
case 90:
{
input.readMessage(getEventTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000200;
break;
} // case 90
case 98:
{
input.readMessage(getCreateTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00000400;
break;
} // case 98
case 112:
{
severity_ = input.readEnum();
bitField0_ |= 0x00000800;
break;
} // case 112
case 120:
{
mute_ = input.readEnum();
bitField0_ |= 0x00001000;
break;
} // case 120
case 128:
{
findingClass_ = input.readEnum();
bitField0_ |= 0x00002000;
break;
} // case 128
case 138:
{
input.readMessage(getIndicatorFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00004000;
break;
} // case 138
case 146:
{
input.readMessage(getVulnerabilityFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00008000;
break;
} // case 146
case 154:
{
input.readMessage(getMuteUpdateTimeFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00010000;
break;
} // case 154
case 162:
{
com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.securitycenter.v2.ExternalSystem>
externalSystems__ =
input.readMessage(
ExternalSystemsDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableExternalSystems()
.ensureBuilderMap()
.put(externalSystems__.getKey(), externalSystems__.getValue());
bitField0_ |= 0x00020000;
break;
} // case 162
case 170:
{
input.readMessage(getMitreAttackFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00040000;
break;
} // case 170
case 178:
{
input.readMessage(getAccessFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x00080000;
break;
} // case 178
case 186:
{
com.google.cloud.securitycenter.v2.Connection m =
input.readMessage(
com.google.cloud.securitycenter.v2.Connection.parser(), extensionRegistry);
if (connectionsBuilder_ == null) {
ensureConnectionsIsMutable();
connections_.add(m);
} else {
connectionsBuilder_.addMessage(m);
}
break;
} // case 186
case 194:
{
muteInitiator_ = input.readStringRequireUtf8();
bitField0_ |= 0x00200000;
break;
} // case 194
case 202:
{
com.google.cloud.securitycenter.v2.Process m =
input.readMessage(
com.google.cloud.securitycenter.v2.Process.parser(), extensionRegistry);
if (processesBuilder_ == null) {
ensureProcessesIsMutable();
processes_.add(m);
} else {
processesBuilder_.addMessage(m);
}
break;
} // case 202
case 210:
{
com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.securitycenter.v2.ContactDetails>
contacts__ =
input.readMessage(
ContactsDefaultEntryHolder.defaultEntry.getParserForType(),
extensionRegistry);
internalGetMutableContacts()
.ensureBuilderMap()
.put(contacts__.getKey(), contacts__.getValue());
bitField0_ |= 0x00800000;
break;
} // case 210
case 218:
{
com.google.cloud.securitycenter.v2.Compliance m =
input.readMessage(
com.google.cloud.securitycenter.v2.Compliance.parser(), extensionRegistry);
if (compliancesBuilder_ == null) {
ensureCompliancesIsMutable();
compliances_.add(m);
} else {
compliancesBuilder_.addMessage(m);
}
break;
} // case 218
case 234:
{
parentDisplayName_ = input.readStringRequireUtf8();
bitField0_ |= 0x02000000;
break;
} // case 234
case 242:
{
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x04000000;
break;
} // case 242
case 250:
{
input.readMessage(getExfiltrationFieldBuilder().getBuilder(), extensionRegistry);
bitField0_ |= 0x08000000;
break;
} // case 250
case 258:
{
com.google.cloud.securitycenter.v2.IamBinding m =
input.readMessage(
com.google.cloud.securitycenter.v2.IamBinding.parser(), extensionRegistry);
if (iamBindingsBuilder_ == null) {
ensureIamBindingsIsMutable();
iamBindings_.add(m);
} else {
iamBindingsBuilder_.addMessage(m);
}
break;
} // case 258
case 266:
{
nextSteps_ = input.readStringRequireUtf8();
bitField0_ |= 0x20000000;
break;
} // case 266
case 274:
{
moduleName_ = input.readStringRequireUtf8();
bitField0_ |= 0x40000000;
break;
} // case 274
case 282:
{
com.google.cloud.securitycenter.v2.Container m =
input.readMessage(
com.google.cloud.securitycenter.v2.Container.parser(), extensionRegistry);
if (containersBuilder_ == null) {
ensureContainersIsMutable();
containers_.add(m);
} else {
containersBuilder_.addMessage(m);
}
break;
} // case 282
case 290:
{
input.readMessage(getKubernetesFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000001;
break;
} // case 290
case 298:
{
input.readMessage(getDatabaseFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000002;
break;
} // case 298
case 306:
{
input.readMessage(getAttackExposureFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000004;
break;
} // case 306
case 314:
{
com.google.cloud.securitycenter.v2.File m =
input.readMessage(
com.google.cloud.securitycenter.v2.File.parser(), extensionRegistry);
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.add(m);
} else {
filesBuilder_.addMessage(m);
}
break;
} // case 314
case 322:
{
input.readMessage(
getCloudDlpInspectionFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000010;
break;
} // case 322
case 330:
{
input.readMessage(
getCloudDlpDataProfileFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000020;
break;
} // case 330
case 338:
{
input.readMessage(getKernelRootkitFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000040;
break;
} // case 338
case 346:
{
com.google.cloud.securitycenter.v2.OrgPolicy m =
input.readMessage(
com.google.cloud.securitycenter.v2.OrgPolicy.parser(), extensionRegistry);
if (orgPoliciesBuilder_ == null) {
ensureOrgPoliciesIsMutable();
orgPolicies_.add(m);
} else {
orgPoliciesBuilder_.addMessage(m);
}
break;
} // case 346
case 362:
{
input.readMessage(getApplicationFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000100;
break;
} // case 362
case 378:
{
input.readMessage(
getBackupDisasterRecoveryFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000200;
break;
} // case 378
case 386:
{
input.readMessage(getSecurityPostureFieldBuilder().getBuilder(), extensionRegistry);
bitField1_ |= 0x00000400;
break;
} // case 386
case 394:
{
com.google.cloud.securitycenter.v2.LogEntry m =
input.readMessage(
com.google.cloud.securitycenter.v2.LogEntry.parser(), extensionRegistry);
if (logEntriesBuilder_ == null) {
ensureLogEntriesIsMutable();
logEntries_.add(m);
} else {
logEntriesBuilder_.addMessage(m);
}
break;
} // case 394
case 402:
{
com.google.cloud.securitycenter.v2.LoadBalancer m =
input.readMessage(
com.google.cloud.securitycenter.v2.LoadBalancer.parser(),
extensionRegistry);
if (loadBalancersBuilder_ == null) {
ensureLoadBalancersIsMutable();
loadBalancers_.add(m);
} else {
loadBalancersBuilder_.addMessage(m);
}
break;
} // case 402
default:
{
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int bitField1_;
private java.lang.Object name_ = "";
/**
*
*
*
* The [relative resource
* name](https://cloud.google.com/apis/design/resource_names#relative_resource_name)
* of the finding. The following list shows some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
*
* string name = 1;
*
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The [relative resource
* name](https://cloud.google.com/apis/design/resource_names#relative_resource_name)
* of the finding. The following list shows some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
*
* string name = 1;
*
* @return The bytes for name.
*/
public com.google.protobuf.ByteString getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The [relative resource
* name](https://cloud.google.com/apis/design/resource_names#relative_resource_name)
* of the finding. The following list shows some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
*
* string name = 1;
*
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* The [relative resource
* name](https://cloud.google.com/apis/design/resource_names#relative_resource_name)
* of the finding. The following list shows some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
*
* string name = 1;
*
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
*
*
* The [relative resource
* name](https://cloud.google.com/apis/design/resource_names#relative_resource_name)
* of the finding. The following list shows some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
*
* string name = 1;
*
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object canonicalName_ = "";
/**
*
*
*
* Output only. The canonical name of the finding. The following list shows
* some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
* The prefix is the closest CRM ancestor of the resource associated with the
* finding.
*
*
* string canonical_name = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The canonicalName.
*/
public java.lang.String getCanonicalName() {
java.lang.Object ref = canonicalName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
canonicalName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. The canonical name of the finding. The following list shows
* some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
* The prefix is the closest CRM ancestor of the resource associated with the
* finding.
*
*
* string canonical_name = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for canonicalName.
*/
public com.google.protobuf.ByteString getCanonicalNameBytes() {
java.lang.Object ref = canonicalName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
canonicalName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. The canonical name of the finding. The following list shows
* some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
* The prefix is the closest CRM ancestor of the resource associated with the
* finding.
*
*
* string canonical_name = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The canonicalName to set.
* @return This builder for chaining.
*/
public Builder setCanonicalName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
canonicalName_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Output only. The canonical name of the finding. The following list shows
* some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
* The prefix is the closest CRM ancestor of the resource associated with the
* finding.
*
*
* string canonical_name = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearCanonicalName() {
canonicalName_ = getDefaultInstance().getCanonicalName();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
*
*
* Output only. The canonical name of the finding. The following list shows
* some examples:
*
* +
* `organizations/{organization_id}/sources/{source_id}/findings/{finding_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `folders/{folder_id}/sources/{source_id}/findings/{finding_id}`
* +
* `folders/{folder_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
* + `projects/{project_id}/sources/{source_id}/findings/{finding_id}`
* +
* `projects/{project_id}/sources/{source_id}/locations/{location_id}/findings/{finding_id}`
*
* The prefix is the closest CRM ancestor of the resource associated with the
* finding.
*
*
* string canonical_name = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The bytes for canonicalName to set.
* @return This builder for chaining.
*/
public Builder setCanonicalNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
canonicalName_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object parent_ = "";
/**
*
*
*
* The relative resource name of the source and location the finding belongs
* to. See:
* https://cloud.google.com/apis/design/resource_names#relative_resource_name
* This field is immutable after creation time. The following list shows some
* examples:
*
* + `organizations/{organization_id}/sources/{source_id}`
* + `folders/{folders_id}/sources/{source_id}`
* + `projects/{projects_id}/sources/{source_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}`
* + `folders/{folders_id}/sources/{source_id}/locations/{location_id}`
* + `projects/{projects_id}/sources/{source_id}/locations/{location_id}`
*
*
* string parent = 3;
*
* @return The parent.
*/
public java.lang.String getParent() {
java.lang.Object ref = parent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
parent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The relative resource name of the source and location the finding belongs
* to. See:
* https://cloud.google.com/apis/design/resource_names#relative_resource_name
* This field is immutable after creation time. The following list shows some
* examples:
*
* + `organizations/{organization_id}/sources/{source_id}`
* + `folders/{folders_id}/sources/{source_id}`
* + `projects/{projects_id}/sources/{source_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}`
* + `folders/{folders_id}/sources/{source_id}/locations/{location_id}`
* + `projects/{projects_id}/sources/{source_id}/locations/{location_id}`
*
*
* string parent = 3;
*
* @return The bytes for parent.
*/
public com.google.protobuf.ByteString getParentBytes() {
java.lang.Object ref = parent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
parent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The relative resource name of the source and location the finding belongs
* to. See:
* https://cloud.google.com/apis/design/resource_names#relative_resource_name
* This field is immutable after creation time. The following list shows some
* examples:
*
* + `organizations/{organization_id}/sources/{source_id}`
* + `folders/{folders_id}/sources/{source_id}`
* + `projects/{projects_id}/sources/{source_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}`
* + `folders/{folders_id}/sources/{source_id}/locations/{location_id}`
* + `projects/{projects_id}/sources/{source_id}/locations/{location_id}`
*
*
* string parent = 3;
*
* @param value The parent to set.
* @return This builder for chaining.
*/
public Builder setParent(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
parent_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* The relative resource name of the source and location the finding belongs
* to. See:
* https://cloud.google.com/apis/design/resource_names#relative_resource_name
* This field is immutable after creation time. The following list shows some
* examples:
*
* + `organizations/{organization_id}/sources/{source_id}`
* + `folders/{folders_id}/sources/{source_id}`
* + `projects/{projects_id}/sources/{source_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}`
* + `folders/{folders_id}/sources/{source_id}/locations/{location_id}`
* + `projects/{projects_id}/sources/{source_id}/locations/{location_id}`
*
*
* string parent = 3;
*
* @return This builder for chaining.
*/
public Builder clearParent() {
parent_ = getDefaultInstance().getParent();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
*
*
* The relative resource name of the source and location the finding belongs
* to. See:
* https://cloud.google.com/apis/design/resource_names#relative_resource_name
* This field is immutable after creation time. The following list shows some
* examples:
*
* + `organizations/{organization_id}/sources/{source_id}`
* + `folders/{folders_id}/sources/{source_id}`
* + `projects/{projects_id}/sources/{source_id}`
* +
* `organizations/{organization_id}/sources/{source_id}/locations/{location_id}`
* + `folders/{folders_id}/sources/{source_id}/locations/{location_id}`
* + `projects/{projects_id}/sources/{source_id}/locations/{location_id}`
*
*
* string parent = 3;
*
* @param value The bytes for parent to set.
* @return This builder for chaining.
*/
public Builder setParentBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
parent_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object resourceName_ = "";
/**
*
*
*
* Immutable. For findings on Google Cloud resources, the full resource
* name of the Google Cloud resource this finding is for. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* When the finding is for a non-Google Cloud resource, the resourceName can
* be a customer or partner defined string.
*
*
* string resource_name = 4 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The resourceName.
*/
public java.lang.String getResourceName() {
java.lang.Object ref = resourceName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
resourceName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Immutable. For findings on Google Cloud resources, the full resource
* name of the Google Cloud resource this finding is for. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* When the finding is for a non-Google Cloud resource, the resourceName can
* be a customer or partner defined string.
*
*
* string resource_name = 4 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The bytes for resourceName.
*/
public com.google.protobuf.ByteString getResourceNameBytes() {
java.lang.Object ref = resourceName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
resourceName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Immutable. For findings on Google Cloud resources, the full resource
* name of the Google Cloud resource this finding is for. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* When the finding is for a non-Google Cloud resource, the resourceName can
* be a customer or partner defined string.
*
*
* string resource_name = 4 [(.google.api.field_behavior) = IMMUTABLE];
*
* @param value The resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
resourceName_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
*
*
* Immutable. For findings on Google Cloud resources, the full resource
* name of the Google Cloud resource this finding is for. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* When the finding is for a non-Google Cloud resource, the resourceName can
* be a customer or partner defined string.
*
*
* string resource_name = 4 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return This builder for chaining.
*/
public Builder clearResourceName() {
resourceName_ = getDefaultInstance().getResourceName();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
*
*
* Immutable. For findings on Google Cloud resources, the full resource
* name of the Google Cloud resource this finding is for. See:
* https://cloud.google.com/apis/design/resource_names#full_resource_name
* When the finding is for a non-Google Cloud resource, the resourceName can
* be a customer or partner defined string.
*
*
* string resource_name = 4 [(.google.api.field_behavior) = IMMUTABLE];
*
* @param value The bytes for resourceName to set.
* @return This builder for chaining.
*/
public Builder setResourceNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
resourceName_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private int state_ = 0;
/**
*
*
*
* Output only. The state of the finding.
*
*
*
* .google.cloud.securitycenter.v2.Finding.State state = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The enum numeric value on the wire for state.
*/
@java.lang.Override
public int getStateValue() {
return state_;
}
/**
*
*
*
* Output only. The state of the finding.
*
*
*
* .google.cloud.securitycenter.v2.Finding.State state = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @param value The enum numeric value on the wire for state to set.
* @return This builder for chaining.
*/
public Builder setStateValue(int value) {
state_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Output only. The state of the finding.
*
*
*
* .google.cloud.securitycenter.v2.Finding.State state = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The state.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding.State getState() {
com.google.cloud.securitycenter.v2.Finding.State result =
com.google.cloud.securitycenter.v2.Finding.State.forNumber(state_);
return result == null
? com.google.cloud.securitycenter.v2.Finding.State.UNRECOGNIZED
: result;
}
/**
*
*
*
* Output only. The state of the finding.
*
*
*
* .google.cloud.securitycenter.v2.Finding.State state = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @param value The state to set.
* @return This builder for chaining.
*/
public Builder setState(com.google.cloud.securitycenter.v2.Finding.State value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
state_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
*
* Output only. The state of the finding.
*
*
*
* .google.cloud.securitycenter.v2.Finding.State state = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return This builder for chaining.
*/
public Builder clearState() {
bitField0_ = (bitField0_ & ~0x00000010);
state_ = 0;
onChanged();
return this;
}
private java.lang.Object category_ = "";
/**
*
*
*
* Immutable. The additional taxonomy group within findings from a given
* source. Example: "XSS_FLASH_INJECTION"
*
*
* string category = 7 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The category.
*/
public java.lang.String getCategory() {
java.lang.Object ref = category_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
category_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Immutable. The additional taxonomy group within findings from a given
* source. Example: "XSS_FLASH_INJECTION"
*
*
* string category = 7 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The bytes for category.
*/
public com.google.protobuf.ByteString getCategoryBytes() {
java.lang.Object ref = category_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
category_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Immutable. The additional taxonomy group within findings from a given
* source. Example: "XSS_FLASH_INJECTION"
*
*
* string category = 7 [(.google.api.field_behavior) = IMMUTABLE];
*
* @param value The category to set.
* @return This builder for chaining.
*/
public Builder setCategory(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
category_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Immutable. The additional taxonomy group within findings from a given
* source. Example: "XSS_FLASH_INJECTION"
*
*
* string category = 7 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return This builder for chaining.
*/
public Builder clearCategory() {
category_ = getDefaultInstance().getCategory();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
*
*
* Immutable. The additional taxonomy group within findings from a given
* source. Example: "XSS_FLASH_INJECTION"
*
*
* string category = 7 [(.google.api.field_behavior) = IMMUTABLE];
*
* @param value The bytes for category to set.
* @return This builder for chaining.
*/
public Builder setCategoryBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
category_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.lang.Object externalUri_ = "";
/**
*
*
*
* The URI that, if available, points to a web page outside of Security
* Command Center where additional information about the finding can be found.
* This field is guaranteed to be either empty or a well formed URL.
*
*
* string external_uri = 8;
*
* @return The externalUri.
*/
public java.lang.String getExternalUri() {
java.lang.Object ref = externalUri_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
externalUri_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* The URI that, if available, points to a web page outside of Security
* Command Center where additional information about the finding can be found.
* This field is guaranteed to be either empty or a well formed URL.
*
*
* string external_uri = 8;
*
* @return The bytes for externalUri.
*/
public com.google.protobuf.ByteString getExternalUriBytes() {
java.lang.Object ref = externalUri_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
externalUri_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* The URI that, if available, points to a web page outside of Security
* Command Center where additional information about the finding can be found.
* This field is guaranteed to be either empty or a well formed URL.
*
*
* string external_uri = 8;
*
* @param value The externalUri to set.
* @return This builder for chaining.
*/
public Builder setExternalUri(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
externalUri_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* The URI that, if available, points to a web page outside of Security
* Command Center where additional information about the finding can be found.
* This field is guaranteed to be either empty or a well formed URL.
*
*
* string external_uri = 8;
*
* @return This builder for chaining.
*/
public Builder clearExternalUri() {
externalUri_ = getDefaultInstance().getExternalUri();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
*
*
* The URI that, if available, points to a web page outside of Security
* Command Center where additional information about the finding can be found.
* This field is guaranteed to be either empty or a well formed URL.
*
*
* string external_uri = 8;
*
* @param value The bytes for externalUri to set.
* @return This builder for chaining.
*/
public Builder setExternalUriBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
externalUri_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private static final class SourcePropertiesConverter
implements com.google.protobuf.MapFieldBuilder.Converter<
java.lang.String, com.google.protobuf.ValueOrBuilder, com.google.protobuf.Value> {
@java.lang.Override
public com.google.protobuf.Value build(com.google.protobuf.ValueOrBuilder val) {
if (val instanceof com.google.protobuf.Value) {
return (com.google.protobuf.Value) val;
}
return ((com.google.protobuf.Value.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry
defaultEntry() {
return SourcePropertiesDefaultEntryHolder.defaultEntry;
}
};
private static final SourcePropertiesConverter sourcePropertiesConverter =
new SourcePropertiesConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.protobuf.ValueOrBuilder,
com.google.protobuf.Value,
com.google.protobuf.Value.Builder>
sourceProperties_;
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.protobuf.ValueOrBuilder,
com.google.protobuf.Value,
com.google.protobuf.Value.Builder>
internalGetSourceProperties() {
if (sourceProperties_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(sourcePropertiesConverter);
}
return sourceProperties_;
}
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.protobuf.ValueOrBuilder,
com.google.protobuf.Value,
com.google.protobuf.Value.Builder>
internalGetMutableSourceProperties() {
if (sourceProperties_ == null) {
sourceProperties_ = new com.google.protobuf.MapFieldBuilder<>(sourcePropertiesConverter);
}
bitField0_ |= 0x00000080;
onChanged();
return sourceProperties_;
}
public int getSourcePropertiesCount() {
return internalGetSourceProperties().ensureBuilderMap().size();
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
@java.lang.Override
public boolean containsSourceProperties(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetSourceProperties().ensureBuilderMap().containsKey(key);
}
/** Use {@link #getSourcePropertiesMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getSourceProperties() {
return getSourcePropertiesMap();
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
@java.lang.Override
public java.util.Map getSourcePropertiesMap() {
return internalGetSourceProperties().getImmutableMap();
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
@java.lang.Override
public /* nullable */ com.google.protobuf.Value getSourcePropertiesOrDefault(
java.lang.String key,
/* nullable */
com.google.protobuf.Value defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetMutableSourceProperties().ensureBuilderMap();
return map.containsKey(key) ? sourcePropertiesConverter.build(map.get(key)) : defaultValue;
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
@java.lang.Override
public com.google.protobuf.Value getSourcePropertiesOrThrow(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map map =
internalGetMutableSourceProperties().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return sourcePropertiesConverter.build(map.get(key));
}
public Builder clearSourceProperties() {
bitField0_ = (bitField0_ & ~0x00000080);
internalGetMutableSourceProperties().clear();
return this;
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
public Builder removeSourceProperties(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableSourceProperties().ensureBuilderMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map getMutableSourceProperties() {
bitField0_ |= 0x00000080;
return internalGetMutableSourceProperties().ensureMessageMap();
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
public Builder putSourceProperties(java.lang.String key, com.google.protobuf.Value value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableSourceProperties().ensureBuilderMap().put(key, value);
bitField0_ |= 0x00000080;
return this;
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
public Builder putAllSourceProperties(
java.util.Map values) {
for (java.util.Map.Entry e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableSourceProperties().ensureBuilderMap().putAll(values);
bitField0_ |= 0x00000080;
return this;
}
/**
*
*
*
* Source specific properties. These properties are managed by the source
* that writes the finding. The key names in the source_properties map must be
* between 1 and 255 characters, and must start with a letter and contain
* alphanumeric characters or underscores only.
*
*
* map<string, .google.protobuf.Value> source_properties = 9;
*/
public com.google.protobuf.Value.Builder putSourcePropertiesBuilderIfAbsent(
java.lang.String key) {
java.util.Map builderMap =
internalGetMutableSourceProperties().ensureBuilderMap();
com.google.protobuf.ValueOrBuilder entry = builderMap.get(key);
if (entry == null) {
entry = com.google.protobuf.Value.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.protobuf.Value) {
entry = ((com.google.protobuf.Value) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.protobuf.Value.Builder) entry;
}
private com.google.cloud.securitycenter.v2.SecurityMarks securityMarks_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.SecurityMarks,
com.google.cloud.securitycenter.v2.SecurityMarks.Builder,
com.google.cloud.securitycenter.v2.SecurityMarksOrBuilder>
securityMarksBuilder_;
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the securityMarks field is set.
*/
public boolean hasSecurityMarks() {
return ((bitField0_ & 0x00000100) != 0);
}
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The securityMarks.
*/
public com.google.cloud.securitycenter.v2.SecurityMarks getSecurityMarks() {
if (securityMarksBuilder_ == null) {
return securityMarks_ == null
? com.google.cloud.securitycenter.v2.SecurityMarks.getDefaultInstance()
: securityMarks_;
} else {
return securityMarksBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setSecurityMarks(com.google.cloud.securitycenter.v2.SecurityMarks value) {
if (securityMarksBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
securityMarks_ = value;
} else {
securityMarksBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setSecurityMarks(
com.google.cloud.securitycenter.v2.SecurityMarks.Builder builderForValue) {
if (securityMarksBuilder_ == null) {
securityMarks_ = builderForValue.build();
} else {
securityMarksBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeSecurityMarks(com.google.cloud.securitycenter.v2.SecurityMarks value) {
if (securityMarksBuilder_ == null) {
if (((bitField0_ & 0x00000100) != 0)
&& securityMarks_ != null
&& securityMarks_
!= com.google.cloud.securitycenter.v2.SecurityMarks.getDefaultInstance()) {
getSecurityMarksBuilder().mergeFrom(value);
} else {
securityMarks_ = value;
}
} else {
securityMarksBuilder_.mergeFrom(value);
}
if (securityMarks_ != null) {
bitField0_ |= 0x00000100;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearSecurityMarks() {
bitField0_ = (bitField0_ & ~0x00000100);
securityMarks_ = null;
if (securityMarksBuilder_ != null) {
securityMarksBuilder_.dispose();
securityMarksBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.cloud.securitycenter.v2.SecurityMarks.Builder getSecurityMarksBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getSecurityMarksFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.cloud.securitycenter.v2.SecurityMarksOrBuilder getSecurityMarksOrBuilder() {
if (securityMarksBuilder_ != null) {
return securityMarksBuilder_.getMessageOrBuilder();
} else {
return securityMarks_ == null
? com.google.cloud.securitycenter.v2.SecurityMarks.getDefaultInstance()
: securityMarks_;
}
}
/**
*
*
*
* Output only. User specified security marks. These marks are entirely
* managed by the user and come from the SecurityMarks resource that belongs
* to the finding.
*
*
*
* .google.cloud.securitycenter.v2.SecurityMarks security_marks = 10 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.SecurityMarks,
com.google.cloud.securitycenter.v2.SecurityMarks.Builder,
com.google.cloud.securitycenter.v2.SecurityMarksOrBuilder>
getSecurityMarksFieldBuilder() {
if (securityMarksBuilder_ == null) {
securityMarksBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.SecurityMarks,
com.google.cloud.securitycenter.v2.SecurityMarks.Builder,
com.google.cloud.securitycenter.v2.SecurityMarksOrBuilder>(
getSecurityMarks(), getParentForChildren(), isClean());
securityMarks_ = null;
}
return securityMarksBuilder_;
}
private com.google.protobuf.Timestamp eventTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
eventTimeBuilder_;
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*
* @return Whether the eventTime field is set.
*/
public boolean hasEventTime() {
return ((bitField0_ & 0x00000200) != 0);
}
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*
* @return The eventTime.
*/
public com.google.protobuf.Timestamp getEventTime() {
if (eventTimeBuilder_ == null) {
return eventTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : eventTime_;
} else {
return eventTimeBuilder_.getMessage();
}
}
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*/
public Builder setEventTime(com.google.protobuf.Timestamp value) {
if (eventTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
eventTime_ = value;
} else {
eventTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*/
public Builder setEventTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (eventTimeBuilder_ == null) {
eventTime_ = builderForValue.build();
} else {
eventTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*/
public Builder mergeEventTime(com.google.protobuf.Timestamp value) {
if (eventTimeBuilder_ == null) {
if (((bitField0_ & 0x00000200) != 0)
&& eventTime_ != null
&& eventTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getEventTimeBuilder().mergeFrom(value);
} else {
eventTime_ = value;
}
} else {
eventTimeBuilder_.mergeFrom(value);
}
if (eventTime_ != null) {
bitField0_ |= 0x00000200;
onChanged();
}
return this;
}
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*/
public Builder clearEventTime() {
bitField0_ = (bitField0_ & ~0x00000200);
eventTime_ = null;
if (eventTimeBuilder_ != null) {
eventTimeBuilder_.dispose();
eventTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*/
public com.google.protobuf.Timestamp.Builder getEventTimeBuilder() {
bitField0_ |= 0x00000200;
onChanged();
return getEventTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*/
public com.google.protobuf.TimestampOrBuilder getEventTimeOrBuilder() {
if (eventTimeBuilder_ != null) {
return eventTimeBuilder_.getMessageOrBuilder();
} else {
return eventTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : eventTime_;
}
}
/**
*
*
*
* The time the finding was first detected. If an existing finding is updated,
* then this is the time the update occurred.
* For example, if the finding represents an open firewall, this property
* captures the time the detector believes the firewall became open. The
* accuracy is determined by the detector. If the finding is later resolved,
* then this time reflects when the finding was resolved. This must not
* be set to a value greater than the current timestamp.
*
*
* .google.protobuf.Timestamp event_time = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getEventTimeFieldBuilder() {
if (eventTimeBuilder_ == null) {
eventTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getEventTime(), getParentForChildren(), isClean());
eventTime_ = null;
}
return eventTimeBuilder_;
}
private com.google.protobuf.Timestamp createTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
createTimeBuilder_;
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the createTime field is set.
*/
public boolean hasCreateTime() {
return ((bitField0_ & 0x00000400) != 0);
}
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The createTime.
*/
public com.google.protobuf.Timestamp getCreateTime() {
if (createTimeBuilder_ == null) {
return createTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: createTime_;
} else {
return createTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setCreateTime(com.google.protobuf.Timestamp value) {
if (createTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
createTime_ = value;
} else {
createTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setCreateTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (createTimeBuilder_ == null) {
createTime_ = builderForValue.build();
} else {
createTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeCreateTime(com.google.protobuf.Timestamp value) {
if (createTimeBuilder_ == null) {
if (((bitField0_ & 0x00000400) != 0)
&& createTime_ != null
&& createTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getCreateTimeBuilder().mergeFrom(value);
} else {
createTime_ = value;
}
} else {
createTimeBuilder_.mergeFrom(value);
}
if (createTime_ != null) {
bitField0_ |= 0x00000400;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearCreateTime() {
bitField0_ = (bitField0_ & ~0x00000400);
createTime_ = null;
if (createTimeBuilder_ != null) {
createTimeBuilder_.dispose();
createTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.Timestamp.Builder getCreateTimeBuilder() {
bitField0_ |= 0x00000400;
onChanged();
return getCreateTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder() {
if (createTimeBuilder_ != null) {
return createTimeBuilder_.getMessageOrBuilder();
} else {
return createTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: createTime_;
}
}
/**
*
*
*
* Output only. The time at which the finding was created in Security Command
* Center.
*
*
*
* .google.protobuf.Timestamp create_time = 12 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getCreateTimeFieldBuilder() {
if (createTimeBuilder_ == null) {
createTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getCreateTime(), getParentForChildren(), isClean());
createTime_ = null;
}
return createTimeBuilder_;
}
private int severity_ = 0;
/**
*
*
*
* The severity of the finding. This field is managed by the source that
* writes the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.Severity severity = 14;
*
* @return The enum numeric value on the wire for severity.
*/
@java.lang.Override
public int getSeverityValue() {
return severity_;
}
/**
*
*
*
* The severity of the finding. This field is managed by the source that
* writes the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.Severity severity = 14;
*
* @param value The enum numeric value on the wire for severity to set.
* @return This builder for chaining.
*/
public Builder setSeverityValue(int value) {
severity_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
*
*
* The severity of the finding. This field is managed by the source that
* writes the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.Severity severity = 14;
*
* @return The severity.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding.Severity getSeverity() {
com.google.cloud.securitycenter.v2.Finding.Severity result =
com.google.cloud.securitycenter.v2.Finding.Severity.forNumber(severity_);
return result == null
? com.google.cloud.securitycenter.v2.Finding.Severity.UNRECOGNIZED
: result;
}
/**
*
*
*
* The severity of the finding. This field is managed by the source that
* writes the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.Severity severity = 14;
*
* @param value The severity to set.
* @return This builder for chaining.
*/
public Builder setSeverity(com.google.cloud.securitycenter.v2.Finding.Severity value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000800;
severity_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
*
* The severity of the finding. This field is managed by the source that
* writes the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.Severity severity = 14;
*
* @return This builder for chaining.
*/
public Builder clearSeverity() {
bitField0_ = (bitField0_ & ~0x00000800);
severity_ = 0;
onChanged();
return this;
}
private int mute_ = 0;
/**
*
*
*
* Indicates the mute state of a finding (either muted, unmuted
* or undefined). Unlike other attributes of a finding, a finding provider
* shouldn't set the value of mute.
*
*
* .google.cloud.securitycenter.v2.Finding.Mute mute = 15;
*
* @return The enum numeric value on the wire for mute.
*/
@java.lang.Override
public int getMuteValue() {
return mute_;
}
/**
*
*
*
* Indicates the mute state of a finding (either muted, unmuted
* or undefined). Unlike other attributes of a finding, a finding provider
* shouldn't set the value of mute.
*
*
* .google.cloud.securitycenter.v2.Finding.Mute mute = 15;
*
* @param value The enum numeric value on the wire for mute to set.
* @return This builder for chaining.
*/
public Builder setMuteValue(int value) {
mute_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
*
*
* Indicates the mute state of a finding (either muted, unmuted
* or undefined). Unlike other attributes of a finding, a finding provider
* shouldn't set the value of mute.
*
*
* .google.cloud.securitycenter.v2.Finding.Mute mute = 15;
*
* @return The mute.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding.Mute getMute() {
com.google.cloud.securitycenter.v2.Finding.Mute result =
com.google.cloud.securitycenter.v2.Finding.Mute.forNumber(mute_);
return result == null ? com.google.cloud.securitycenter.v2.Finding.Mute.UNRECOGNIZED : result;
}
/**
*
*
*
* Indicates the mute state of a finding (either muted, unmuted
* or undefined). Unlike other attributes of a finding, a finding provider
* shouldn't set the value of mute.
*
*
* .google.cloud.securitycenter.v2.Finding.Mute mute = 15;
*
* @param value The mute to set.
* @return This builder for chaining.
*/
public Builder setMute(com.google.cloud.securitycenter.v2.Finding.Mute value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00001000;
mute_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
*
* Indicates the mute state of a finding (either muted, unmuted
* or undefined). Unlike other attributes of a finding, a finding provider
* shouldn't set the value of mute.
*
*
* .google.cloud.securitycenter.v2.Finding.Mute mute = 15;
*
* @return This builder for chaining.
*/
public Builder clearMute() {
bitField0_ = (bitField0_ & ~0x00001000);
mute_ = 0;
onChanged();
return this;
}
private int findingClass_ = 0;
/**
*
*
*
* The class of the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.FindingClass finding_class = 16;
*
* @return The enum numeric value on the wire for findingClass.
*/
@java.lang.Override
public int getFindingClassValue() {
return findingClass_;
}
/**
*
*
*
* The class of the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.FindingClass finding_class = 16;
*
* @param value The enum numeric value on the wire for findingClass to set.
* @return This builder for chaining.
*/
public Builder setFindingClassValue(int value) {
findingClass_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
*
*
* The class of the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.FindingClass finding_class = 16;
*
* @return The findingClass.
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding.FindingClass getFindingClass() {
com.google.cloud.securitycenter.v2.Finding.FindingClass result =
com.google.cloud.securitycenter.v2.Finding.FindingClass.forNumber(findingClass_);
return result == null
? com.google.cloud.securitycenter.v2.Finding.FindingClass.UNRECOGNIZED
: result;
}
/**
*
*
*
* The class of the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.FindingClass finding_class = 16;
*
* @param value The findingClass to set.
* @return This builder for chaining.
*/
public Builder setFindingClass(com.google.cloud.securitycenter.v2.Finding.FindingClass value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
findingClass_ = value.getNumber();
onChanged();
return this;
}
/**
*
*
*
* The class of the finding.
*
*
* .google.cloud.securitycenter.v2.Finding.FindingClass finding_class = 16;
*
* @return This builder for chaining.
*/
public Builder clearFindingClass() {
bitField0_ = (bitField0_ & ~0x00002000);
findingClass_ = 0;
onChanged();
return this;
}
private com.google.cloud.securitycenter.v2.Indicator indicator_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Indicator,
com.google.cloud.securitycenter.v2.Indicator.Builder,
com.google.cloud.securitycenter.v2.IndicatorOrBuilder>
indicatorBuilder_;
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*
* @return Whether the indicator field is set.
*/
public boolean hasIndicator() {
return ((bitField0_ & 0x00004000) != 0);
}
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*
* @return The indicator.
*/
public com.google.cloud.securitycenter.v2.Indicator getIndicator() {
if (indicatorBuilder_ == null) {
return indicator_ == null
? com.google.cloud.securitycenter.v2.Indicator.getDefaultInstance()
: indicator_;
} else {
return indicatorBuilder_.getMessage();
}
}
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*/
public Builder setIndicator(com.google.cloud.securitycenter.v2.Indicator value) {
if (indicatorBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
indicator_ = value;
} else {
indicatorBuilder_.setMessage(value);
}
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*/
public Builder setIndicator(
com.google.cloud.securitycenter.v2.Indicator.Builder builderForValue) {
if (indicatorBuilder_ == null) {
indicator_ = builderForValue.build();
} else {
indicatorBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*/
public Builder mergeIndicator(com.google.cloud.securitycenter.v2.Indicator value) {
if (indicatorBuilder_ == null) {
if (((bitField0_ & 0x00004000) != 0)
&& indicator_ != null
&& indicator_ != com.google.cloud.securitycenter.v2.Indicator.getDefaultInstance()) {
getIndicatorBuilder().mergeFrom(value);
} else {
indicator_ = value;
}
} else {
indicatorBuilder_.mergeFrom(value);
}
if (indicator_ != null) {
bitField0_ |= 0x00004000;
onChanged();
}
return this;
}
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*/
public Builder clearIndicator() {
bitField0_ = (bitField0_ & ~0x00004000);
indicator_ = null;
if (indicatorBuilder_ != null) {
indicatorBuilder_.dispose();
indicatorBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*/
public com.google.cloud.securitycenter.v2.Indicator.Builder getIndicatorBuilder() {
bitField0_ |= 0x00004000;
onChanged();
return getIndicatorFieldBuilder().getBuilder();
}
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*/
public com.google.cloud.securitycenter.v2.IndicatorOrBuilder getIndicatorOrBuilder() {
if (indicatorBuilder_ != null) {
return indicatorBuilder_.getMessageOrBuilder();
} else {
return indicator_ == null
? com.google.cloud.securitycenter.v2.Indicator.getDefaultInstance()
: indicator_;
}
}
/**
*
*
*
* Represents what's commonly known as an *indicator of compromise* (IoC) in
* computer forensics. This is an artifact observed on a network or in an
* operating system that, with high confidence, indicates a computer
* intrusion. For more information, see [Indicator of
* compromise](https://en.wikipedia.org/wiki/Indicator_of_compromise).
*
*
* .google.cloud.securitycenter.v2.Indicator indicator = 17;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Indicator,
com.google.cloud.securitycenter.v2.Indicator.Builder,
com.google.cloud.securitycenter.v2.IndicatorOrBuilder>
getIndicatorFieldBuilder() {
if (indicatorBuilder_ == null) {
indicatorBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Indicator,
com.google.cloud.securitycenter.v2.Indicator.Builder,
com.google.cloud.securitycenter.v2.IndicatorOrBuilder>(
getIndicator(), getParentForChildren(), isClean());
indicator_ = null;
}
return indicatorBuilder_;
}
private com.google.cloud.securitycenter.v2.Vulnerability vulnerability_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Vulnerability,
com.google.cloud.securitycenter.v2.Vulnerability.Builder,
com.google.cloud.securitycenter.v2.VulnerabilityOrBuilder>
vulnerabilityBuilder_;
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*
* @return Whether the vulnerability field is set.
*/
public boolean hasVulnerability() {
return ((bitField0_ & 0x00008000) != 0);
}
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*
* @return The vulnerability.
*/
public com.google.cloud.securitycenter.v2.Vulnerability getVulnerability() {
if (vulnerabilityBuilder_ == null) {
return vulnerability_ == null
? com.google.cloud.securitycenter.v2.Vulnerability.getDefaultInstance()
: vulnerability_;
} else {
return vulnerabilityBuilder_.getMessage();
}
}
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*/
public Builder setVulnerability(com.google.cloud.securitycenter.v2.Vulnerability value) {
if (vulnerabilityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
vulnerability_ = value;
} else {
vulnerabilityBuilder_.setMessage(value);
}
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*/
public Builder setVulnerability(
com.google.cloud.securitycenter.v2.Vulnerability.Builder builderForValue) {
if (vulnerabilityBuilder_ == null) {
vulnerability_ = builderForValue.build();
} else {
vulnerabilityBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*/
public Builder mergeVulnerability(com.google.cloud.securitycenter.v2.Vulnerability value) {
if (vulnerabilityBuilder_ == null) {
if (((bitField0_ & 0x00008000) != 0)
&& vulnerability_ != null
&& vulnerability_
!= com.google.cloud.securitycenter.v2.Vulnerability.getDefaultInstance()) {
getVulnerabilityBuilder().mergeFrom(value);
} else {
vulnerability_ = value;
}
} else {
vulnerabilityBuilder_.mergeFrom(value);
}
if (vulnerability_ != null) {
bitField0_ |= 0x00008000;
onChanged();
}
return this;
}
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*/
public Builder clearVulnerability() {
bitField0_ = (bitField0_ & ~0x00008000);
vulnerability_ = null;
if (vulnerabilityBuilder_ != null) {
vulnerabilityBuilder_.dispose();
vulnerabilityBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*/
public com.google.cloud.securitycenter.v2.Vulnerability.Builder getVulnerabilityBuilder() {
bitField0_ |= 0x00008000;
onChanged();
return getVulnerabilityFieldBuilder().getBuilder();
}
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*/
public com.google.cloud.securitycenter.v2.VulnerabilityOrBuilder getVulnerabilityOrBuilder() {
if (vulnerabilityBuilder_ != null) {
return vulnerabilityBuilder_.getMessageOrBuilder();
} else {
return vulnerability_ == null
? com.google.cloud.securitycenter.v2.Vulnerability.getDefaultInstance()
: vulnerability_;
}
}
/**
*
*
*
* Represents vulnerability-specific fields like CVE and CVSS scores.
* CVE stands for Common Vulnerabilities and Exposures
* (https://cve.mitre.org/about/)
*
*
* .google.cloud.securitycenter.v2.Vulnerability vulnerability = 18;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Vulnerability,
com.google.cloud.securitycenter.v2.Vulnerability.Builder,
com.google.cloud.securitycenter.v2.VulnerabilityOrBuilder>
getVulnerabilityFieldBuilder() {
if (vulnerabilityBuilder_ == null) {
vulnerabilityBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Vulnerability,
com.google.cloud.securitycenter.v2.Vulnerability.Builder,
com.google.cloud.securitycenter.v2.VulnerabilityOrBuilder>(
getVulnerability(), getParentForChildren(), isClean());
vulnerability_ = null;
}
return vulnerabilityBuilder_;
}
private com.google.protobuf.Timestamp muteUpdateTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
muteUpdateTimeBuilder_;
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the muteUpdateTime field is set.
*/
public boolean hasMuteUpdateTime() {
return ((bitField0_ & 0x00010000) != 0);
}
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The muteUpdateTime.
*/
public com.google.protobuf.Timestamp getMuteUpdateTime() {
if (muteUpdateTimeBuilder_ == null) {
return muteUpdateTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: muteUpdateTime_;
} else {
return muteUpdateTimeBuilder_.getMessage();
}
}
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setMuteUpdateTime(com.google.protobuf.Timestamp value) {
if (muteUpdateTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
muteUpdateTime_ = value;
} else {
muteUpdateTimeBuilder_.setMessage(value);
}
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder setMuteUpdateTime(com.google.protobuf.Timestamp.Builder builderForValue) {
if (muteUpdateTimeBuilder_ == null) {
muteUpdateTime_ = builderForValue.build();
} else {
muteUpdateTimeBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder mergeMuteUpdateTime(com.google.protobuf.Timestamp value) {
if (muteUpdateTimeBuilder_ == null) {
if (((bitField0_ & 0x00010000) != 0)
&& muteUpdateTime_ != null
&& muteUpdateTime_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
getMuteUpdateTimeBuilder().mergeFrom(value);
} else {
muteUpdateTime_ = value;
}
} else {
muteUpdateTimeBuilder_.mergeFrom(value);
}
if (muteUpdateTime_ != null) {
bitField0_ |= 0x00010000;
onChanged();
}
return this;
}
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder clearMuteUpdateTime() {
bitField0_ = (bitField0_ & ~0x00010000);
muteUpdateTime_ = null;
if (muteUpdateTimeBuilder_ != null) {
muteUpdateTimeBuilder_.dispose();
muteUpdateTimeBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.Timestamp.Builder getMuteUpdateTimeBuilder() {
bitField0_ |= 0x00010000;
onChanged();
return getMuteUpdateTimeFieldBuilder().getBuilder();
}
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.protobuf.TimestampOrBuilder getMuteUpdateTimeOrBuilder() {
if (muteUpdateTimeBuilder_ != null) {
return muteUpdateTimeBuilder_.getMessageOrBuilder();
} else {
return muteUpdateTime_ == null
? com.google.protobuf.Timestamp.getDefaultInstance()
: muteUpdateTime_;
}
}
/**
*
*
*
* Output only. The most recent time this finding was muted or unmuted.
*
*
*
* .google.protobuf.Timestamp mute_update_time = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>
getMuteUpdateTimeFieldBuilder() {
if (muteUpdateTimeBuilder_ == null) {
muteUpdateTimeBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp,
com.google.protobuf.Timestamp.Builder,
com.google.protobuf.TimestampOrBuilder>(
getMuteUpdateTime(), getParentForChildren(), isClean());
muteUpdateTime_ = null;
}
return muteUpdateTimeBuilder_;
}
private static final class ExternalSystemsConverter
implements com.google.protobuf.MapFieldBuilder.Converter<
java.lang.String,
com.google.cloud.securitycenter.v2.ExternalSystemOrBuilder,
com.google.cloud.securitycenter.v2.ExternalSystem> {
@java.lang.Override
public com.google.cloud.securitycenter.v2.ExternalSystem build(
com.google.cloud.securitycenter.v2.ExternalSystemOrBuilder val) {
if (val instanceof com.google.cloud.securitycenter.v2.ExternalSystem) {
return (com.google.cloud.securitycenter.v2.ExternalSystem) val;
}
return ((com.google.cloud.securitycenter.v2.ExternalSystem.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.securitycenter.v2.ExternalSystem>
defaultEntry() {
return ExternalSystemsDefaultEntryHolder.defaultEntry;
}
};
private static final ExternalSystemsConverter externalSystemsConverter =
new ExternalSystemsConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.securitycenter.v2.ExternalSystemOrBuilder,
com.google.cloud.securitycenter.v2.ExternalSystem,
com.google.cloud.securitycenter.v2.ExternalSystem.Builder>
externalSystems_;
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.securitycenter.v2.ExternalSystemOrBuilder,
com.google.cloud.securitycenter.v2.ExternalSystem,
com.google.cloud.securitycenter.v2.ExternalSystem.Builder>
internalGetExternalSystems() {
if (externalSystems_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(externalSystemsConverter);
}
return externalSystems_;
}
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.securitycenter.v2.ExternalSystemOrBuilder,
com.google.cloud.securitycenter.v2.ExternalSystem,
com.google.cloud.securitycenter.v2.ExternalSystem.Builder>
internalGetMutableExternalSystems() {
if (externalSystems_ == null) {
externalSystems_ = new com.google.protobuf.MapFieldBuilder<>(externalSystemsConverter);
}
bitField0_ |= 0x00020000;
onChanged();
return externalSystems_;
}
public int getExternalSystemsCount() {
return internalGetExternalSystems().ensureBuilderMap().size();
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public boolean containsExternalSystems(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetExternalSystems().ensureBuilderMap().containsKey(key);
}
/** Use {@link #getExternalSystemsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getExternalSystems() {
return getExternalSystemsMap();
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public java.util.Map
getExternalSystemsMap() {
return internalGetExternalSystems().getImmutableMap();
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public /* nullable */ com.google.cloud.securitycenter.v2.ExternalSystem
getExternalSystemsOrDefault(
java.lang.String key,
/* nullable */
com.google.cloud.securitycenter.v2.ExternalSystem defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetMutableExternalSystems().ensureBuilderMap();
return map.containsKey(key) ? externalSystemsConverter.build(map.get(key)) : defaultValue;
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.ExternalSystem getExternalSystemsOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetMutableExternalSystems().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return externalSystemsConverter.build(map.get(key));
}
public Builder clearExternalSystems() {
bitField0_ = (bitField0_ & ~0x00020000);
internalGetMutableExternalSystems().clear();
return this;
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder removeExternalSystems(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableExternalSystems().ensureBuilderMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map
getMutableExternalSystems() {
bitField0_ |= 0x00020000;
return internalGetMutableExternalSystems().ensureMessageMap();
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder putExternalSystems(
java.lang.String key, com.google.cloud.securitycenter.v2.ExternalSystem value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableExternalSystems().ensureBuilderMap().put(key, value);
bitField0_ |= 0x00020000;
return this;
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder putAllExternalSystems(
java.util.Map values) {
for (java.util.Map.Entry
e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableExternalSystems().ensureBuilderMap().putAll(values);
bitField0_ |= 0x00020000;
return this;
}
/**
*
*
*
* Output only. Third party SIEM/SOAR fields within SCC, contains external
* system information and external system finding fields.
*
*
*
* map<string, .google.cloud.securitycenter.v2.ExternalSystem> external_systems = 20 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.cloud.securitycenter.v2.ExternalSystem.Builder
putExternalSystemsBuilderIfAbsent(java.lang.String key) {
java.util.Map
builderMap = internalGetMutableExternalSystems().ensureBuilderMap();
com.google.cloud.securitycenter.v2.ExternalSystemOrBuilder entry = builderMap.get(key);
if (entry == null) {
entry = com.google.cloud.securitycenter.v2.ExternalSystem.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.cloud.securitycenter.v2.ExternalSystem) {
entry = ((com.google.cloud.securitycenter.v2.ExternalSystem) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.cloud.securitycenter.v2.ExternalSystem.Builder) entry;
}
private com.google.cloud.securitycenter.v2.MitreAttack mitreAttack_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.MitreAttack,
com.google.cloud.securitycenter.v2.MitreAttack.Builder,
com.google.cloud.securitycenter.v2.MitreAttackOrBuilder>
mitreAttackBuilder_;
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*
* @return Whether the mitreAttack field is set.
*/
public boolean hasMitreAttack() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*
* @return The mitreAttack.
*/
public com.google.cloud.securitycenter.v2.MitreAttack getMitreAttack() {
if (mitreAttackBuilder_ == null) {
return mitreAttack_ == null
? com.google.cloud.securitycenter.v2.MitreAttack.getDefaultInstance()
: mitreAttack_;
} else {
return mitreAttackBuilder_.getMessage();
}
}
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*/
public Builder setMitreAttack(com.google.cloud.securitycenter.v2.MitreAttack value) {
if (mitreAttackBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
mitreAttack_ = value;
} else {
mitreAttackBuilder_.setMessage(value);
}
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*/
public Builder setMitreAttack(
com.google.cloud.securitycenter.v2.MitreAttack.Builder builderForValue) {
if (mitreAttackBuilder_ == null) {
mitreAttack_ = builderForValue.build();
} else {
mitreAttackBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*/
public Builder mergeMitreAttack(com.google.cloud.securitycenter.v2.MitreAttack value) {
if (mitreAttackBuilder_ == null) {
if (((bitField0_ & 0x00040000) != 0)
&& mitreAttack_ != null
&& mitreAttack_
!= com.google.cloud.securitycenter.v2.MitreAttack.getDefaultInstance()) {
getMitreAttackBuilder().mergeFrom(value);
} else {
mitreAttack_ = value;
}
} else {
mitreAttackBuilder_.mergeFrom(value);
}
if (mitreAttack_ != null) {
bitField0_ |= 0x00040000;
onChanged();
}
return this;
}
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*/
public Builder clearMitreAttack() {
bitField0_ = (bitField0_ & ~0x00040000);
mitreAttack_ = null;
if (mitreAttackBuilder_ != null) {
mitreAttackBuilder_.dispose();
mitreAttackBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*/
public com.google.cloud.securitycenter.v2.MitreAttack.Builder getMitreAttackBuilder() {
bitField0_ |= 0x00040000;
onChanged();
return getMitreAttackFieldBuilder().getBuilder();
}
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*/
public com.google.cloud.securitycenter.v2.MitreAttackOrBuilder getMitreAttackOrBuilder() {
if (mitreAttackBuilder_ != null) {
return mitreAttackBuilder_.getMessageOrBuilder();
} else {
return mitreAttack_ == null
? com.google.cloud.securitycenter.v2.MitreAttack.getDefaultInstance()
: mitreAttack_;
}
}
/**
*
*
*
* MITRE ATT&CK tactics and techniques related to this finding.
* See: https://attack.mitre.org
*
*
* .google.cloud.securitycenter.v2.MitreAttack mitre_attack = 21;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.MitreAttack,
com.google.cloud.securitycenter.v2.MitreAttack.Builder,
com.google.cloud.securitycenter.v2.MitreAttackOrBuilder>
getMitreAttackFieldBuilder() {
if (mitreAttackBuilder_ == null) {
mitreAttackBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.MitreAttack,
com.google.cloud.securitycenter.v2.MitreAttack.Builder,
com.google.cloud.securitycenter.v2.MitreAttackOrBuilder>(
getMitreAttack(), getParentForChildren(), isClean());
mitreAttack_ = null;
}
return mitreAttackBuilder_;
}
private com.google.cloud.securitycenter.v2.Access access_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Access,
com.google.cloud.securitycenter.v2.Access.Builder,
com.google.cloud.securitycenter.v2.AccessOrBuilder>
accessBuilder_;
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*
* @return Whether the access field is set.
*/
public boolean hasAccess() {
return ((bitField0_ & 0x00080000) != 0);
}
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*
* @return The access.
*/
public com.google.cloud.securitycenter.v2.Access getAccess() {
if (accessBuilder_ == null) {
return access_ == null
? com.google.cloud.securitycenter.v2.Access.getDefaultInstance()
: access_;
} else {
return accessBuilder_.getMessage();
}
}
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*/
public Builder setAccess(com.google.cloud.securitycenter.v2.Access value) {
if (accessBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
access_ = value;
} else {
accessBuilder_.setMessage(value);
}
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*/
public Builder setAccess(com.google.cloud.securitycenter.v2.Access.Builder builderForValue) {
if (accessBuilder_ == null) {
access_ = builderForValue.build();
} else {
accessBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*/
public Builder mergeAccess(com.google.cloud.securitycenter.v2.Access value) {
if (accessBuilder_ == null) {
if (((bitField0_ & 0x00080000) != 0)
&& access_ != null
&& access_ != com.google.cloud.securitycenter.v2.Access.getDefaultInstance()) {
getAccessBuilder().mergeFrom(value);
} else {
access_ = value;
}
} else {
accessBuilder_.mergeFrom(value);
}
if (access_ != null) {
bitField0_ |= 0x00080000;
onChanged();
}
return this;
}
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*/
public Builder clearAccess() {
bitField0_ = (bitField0_ & ~0x00080000);
access_ = null;
if (accessBuilder_ != null) {
accessBuilder_.dispose();
accessBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*/
public com.google.cloud.securitycenter.v2.Access.Builder getAccessBuilder() {
bitField0_ |= 0x00080000;
onChanged();
return getAccessFieldBuilder().getBuilder();
}
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*/
public com.google.cloud.securitycenter.v2.AccessOrBuilder getAccessOrBuilder() {
if (accessBuilder_ != null) {
return accessBuilder_.getMessageOrBuilder();
} else {
return access_ == null
? com.google.cloud.securitycenter.v2.Access.getDefaultInstance()
: access_;
}
}
/**
*
*
*
* Access details associated with the finding, such as more information on the
* caller, which method was accessed, and from where.
*
*
* .google.cloud.securitycenter.v2.Access access = 22;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Access,
com.google.cloud.securitycenter.v2.Access.Builder,
com.google.cloud.securitycenter.v2.AccessOrBuilder>
getAccessFieldBuilder() {
if (accessBuilder_ == null) {
accessBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Access,
com.google.cloud.securitycenter.v2.Access.Builder,
com.google.cloud.securitycenter.v2.AccessOrBuilder>(
getAccess(), getParentForChildren(), isClean());
access_ = null;
}
return accessBuilder_;
}
private java.util.List connections_ =
java.util.Collections.emptyList();
private void ensureConnectionsIsMutable() {
if (!((bitField0_ & 0x00100000) != 0)) {
connections_ =
new java.util.ArrayList(connections_);
bitField0_ |= 0x00100000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Connection,
com.google.cloud.securitycenter.v2.Connection.Builder,
com.google.cloud.securitycenter.v2.ConnectionOrBuilder>
connectionsBuilder_;
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public java.util.List getConnectionsList() {
if (connectionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(connections_);
} else {
return connectionsBuilder_.getMessageList();
}
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public int getConnectionsCount() {
if (connectionsBuilder_ == null) {
return connections_.size();
} else {
return connectionsBuilder_.getCount();
}
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public com.google.cloud.securitycenter.v2.Connection getConnections(int index) {
if (connectionsBuilder_ == null) {
return connections_.get(index);
} else {
return connectionsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public Builder setConnections(int index, com.google.cloud.securitycenter.v2.Connection value) {
if (connectionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConnectionsIsMutable();
connections_.set(index, value);
onChanged();
} else {
connectionsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public Builder setConnections(
int index, com.google.cloud.securitycenter.v2.Connection.Builder builderForValue) {
if (connectionsBuilder_ == null) {
ensureConnectionsIsMutable();
connections_.set(index, builderForValue.build());
onChanged();
} else {
connectionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public Builder addConnections(com.google.cloud.securitycenter.v2.Connection value) {
if (connectionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConnectionsIsMutable();
connections_.add(value);
onChanged();
} else {
connectionsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public Builder addConnections(int index, com.google.cloud.securitycenter.v2.Connection value) {
if (connectionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConnectionsIsMutable();
connections_.add(index, value);
onChanged();
} else {
connectionsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public Builder addConnections(
com.google.cloud.securitycenter.v2.Connection.Builder builderForValue) {
if (connectionsBuilder_ == null) {
ensureConnectionsIsMutable();
connections_.add(builderForValue.build());
onChanged();
} else {
connectionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public Builder addConnections(
int index, com.google.cloud.securitycenter.v2.Connection.Builder builderForValue) {
if (connectionsBuilder_ == null) {
ensureConnectionsIsMutable();
connections_.add(index, builderForValue.build());
onChanged();
} else {
connectionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public Builder addAllConnections(
java.lang.Iterable extends com.google.cloud.securitycenter.v2.Connection> values) {
if (connectionsBuilder_ == null) {
ensureConnectionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, connections_);
onChanged();
} else {
connectionsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public Builder clearConnections() {
if (connectionsBuilder_ == null) {
connections_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00100000);
onChanged();
} else {
connectionsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public Builder removeConnections(int index) {
if (connectionsBuilder_ == null) {
ensureConnectionsIsMutable();
connections_.remove(index);
onChanged();
} else {
connectionsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public com.google.cloud.securitycenter.v2.Connection.Builder getConnectionsBuilder(int index) {
return getConnectionsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public com.google.cloud.securitycenter.v2.ConnectionOrBuilder getConnectionsOrBuilder(
int index) {
if (connectionsBuilder_ == null) {
return connections_.get(index);
} else {
return connectionsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public java.util.List extends com.google.cloud.securitycenter.v2.ConnectionOrBuilder>
getConnectionsOrBuilderList() {
if (connectionsBuilder_ != null) {
return connectionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(connections_);
}
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public com.google.cloud.securitycenter.v2.Connection.Builder addConnectionsBuilder() {
return getConnectionsFieldBuilder()
.addBuilder(com.google.cloud.securitycenter.v2.Connection.getDefaultInstance());
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public com.google.cloud.securitycenter.v2.Connection.Builder addConnectionsBuilder(int index) {
return getConnectionsFieldBuilder()
.addBuilder(index, com.google.cloud.securitycenter.v2.Connection.getDefaultInstance());
}
/**
*
*
*
* Contains information about the IP connection associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.Connection connections = 23;
*/
public java.util.List
getConnectionsBuilderList() {
return getConnectionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Connection,
com.google.cloud.securitycenter.v2.Connection.Builder,
com.google.cloud.securitycenter.v2.ConnectionOrBuilder>
getConnectionsFieldBuilder() {
if (connectionsBuilder_ == null) {
connectionsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Connection,
com.google.cloud.securitycenter.v2.Connection.Builder,
com.google.cloud.securitycenter.v2.ConnectionOrBuilder>(
connections_, ((bitField0_ & 0x00100000) != 0), getParentForChildren(), isClean());
connections_ = null;
}
return connectionsBuilder_;
}
private java.lang.Object muteInitiator_ = "";
/**
*
*
*
* Records additional information about the mute operation, for example, the
* [mute
* configuration](https://cloud.google.com/security-command-center/docs/how-to-mute-findings)
* that muted the finding and the user who muted the finding.
*
*
* string mute_initiator = 24;
*
* @return The muteInitiator.
*/
public java.lang.String getMuteInitiator() {
java.lang.Object ref = muteInitiator_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
muteInitiator_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Records additional information about the mute operation, for example, the
* [mute
* configuration](https://cloud.google.com/security-command-center/docs/how-to-mute-findings)
* that muted the finding and the user who muted the finding.
*
*
* string mute_initiator = 24;
*
* @return The bytes for muteInitiator.
*/
public com.google.protobuf.ByteString getMuteInitiatorBytes() {
java.lang.Object ref = muteInitiator_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
muteInitiator_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Records additional information about the mute operation, for example, the
* [mute
* configuration](https://cloud.google.com/security-command-center/docs/how-to-mute-findings)
* that muted the finding and the user who muted the finding.
*
*
* string mute_initiator = 24;
*
* @param value The muteInitiator to set.
* @return This builder for chaining.
*/
public Builder setMuteInitiator(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
muteInitiator_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
*
*
* Records additional information about the mute operation, for example, the
* [mute
* configuration](https://cloud.google.com/security-command-center/docs/how-to-mute-findings)
* that muted the finding and the user who muted the finding.
*
*
* string mute_initiator = 24;
*
* @return This builder for chaining.
*/
public Builder clearMuteInitiator() {
muteInitiator_ = getDefaultInstance().getMuteInitiator();
bitField0_ = (bitField0_ & ~0x00200000);
onChanged();
return this;
}
/**
*
*
*
* Records additional information about the mute operation, for example, the
* [mute
* configuration](https://cloud.google.com/security-command-center/docs/how-to-mute-findings)
* that muted the finding and the user who muted the finding.
*
*
* string mute_initiator = 24;
*
* @param value The bytes for muteInitiator to set.
* @return This builder for chaining.
*/
public Builder setMuteInitiatorBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
muteInitiator_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
private java.util.List processes_ =
java.util.Collections.emptyList();
private void ensureProcessesIsMutable() {
if (!((bitField0_ & 0x00400000) != 0)) {
processes_ =
new java.util.ArrayList(processes_);
bitField0_ |= 0x00400000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Process,
com.google.cloud.securitycenter.v2.Process.Builder,
com.google.cloud.securitycenter.v2.ProcessOrBuilder>
processesBuilder_;
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public java.util.List getProcessesList() {
if (processesBuilder_ == null) {
return java.util.Collections.unmodifiableList(processes_);
} else {
return processesBuilder_.getMessageList();
}
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public int getProcessesCount() {
if (processesBuilder_ == null) {
return processes_.size();
} else {
return processesBuilder_.getCount();
}
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public com.google.cloud.securitycenter.v2.Process getProcesses(int index) {
if (processesBuilder_ == null) {
return processes_.get(index);
} else {
return processesBuilder_.getMessage(index);
}
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public Builder setProcesses(int index, com.google.cloud.securitycenter.v2.Process value) {
if (processesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureProcessesIsMutable();
processes_.set(index, value);
onChanged();
} else {
processesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public Builder setProcesses(
int index, com.google.cloud.securitycenter.v2.Process.Builder builderForValue) {
if (processesBuilder_ == null) {
ensureProcessesIsMutable();
processes_.set(index, builderForValue.build());
onChanged();
} else {
processesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public Builder addProcesses(com.google.cloud.securitycenter.v2.Process value) {
if (processesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureProcessesIsMutable();
processes_.add(value);
onChanged();
} else {
processesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public Builder addProcesses(int index, com.google.cloud.securitycenter.v2.Process value) {
if (processesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureProcessesIsMutable();
processes_.add(index, value);
onChanged();
} else {
processesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public Builder addProcesses(
com.google.cloud.securitycenter.v2.Process.Builder builderForValue) {
if (processesBuilder_ == null) {
ensureProcessesIsMutable();
processes_.add(builderForValue.build());
onChanged();
} else {
processesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public Builder addProcesses(
int index, com.google.cloud.securitycenter.v2.Process.Builder builderForValue) {
if (processesBuilder_ == null) {
ensureProcessesIsMutable();
processes_.add(index, builderForValue.build());
onChanged();
} else {
processesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public Builder addAllProcesses(
java.lang.Iterable extends com.google.cloud.securitycenter.v2.Process> values) {
if (processesBuilder_ == null) {
ensureProcessesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, processes_);
onChanged();
} else {
processesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public Builder clearProcesses() {
if (processesBuilder_ == null) {
processes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00400000);
onChanged();
} else {
processesBuilder_.clear();
}
return this;
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public Builder removeProcesses(int index) {
if (processesBuilder_ == null) {
ensureProcessesIsMutable();
processes_.remove(index);
onChanged();
} else {
processesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public com.google.cloud.securitycenter.v2.Process.Builder getProcessesBuilder(int index) {
return getProcessesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public com.google.cloud.securitycenter.v2.ProcessOrBuilder getProcessesOrBuilder(int index) {
if (processesBuilder_ == null) {
return processes_.get(index);
} else {
return processesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public java.util.List extends com.google.cloud.securitycenter.v2.ProcessOrBuilder>
getProcessesOrBuilderList() {
if (processesBuilder_ != null) {
return processesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(processes_);
}
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public com.google.cloud.securitycenter.v2.Process.Builder addProcessesBuilder() {
return getProcessesFieldBuilder()
.addBuilder(com.google.cloud.securitycenter.v2.Process.getDefaultInstance());
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public com.google.cloud.securitycenter.v2.Process.Builder addProcessesBuilder(int index) {
return getProcessesFieldBuilder()
.addBuilder(index, com.google.cloud.securitycenter.v2.Process.getDefaultInstance());
}
/**
*
*
*
* Represents operating system processes associated with the Finding.
*
*
* repeated .google.cloud.securitycenter.v2.Process processes = 25;
*/
public java.util.List
getProcessesBuilderList() {
return getProcessesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Process,
com.google.cloud.securitycenter.v2.Process.Builder,
com.google.cloud.securitycenter.v2.ProcessOrBuilder>
getProcessesFieldBuilder() {
if (processesBuilder_ == null) {
processesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Process,
com.google.cloud.securitycenter.v2.Process.Builder,
com.google.cloud.securitycenter.v2.ProcessOrBuilder>(
processes_, ((bitField0_ & 0x00400000) != 0), getParentForChildren(), isClean());
processes_ = null;
}
return processesBuilder_;
}
private static final class ContactsConverter
implements com.google.protobuf.MapFieldBuilder.Converter<
java.lang.String,
com.google.cloud.securitycenter.v2.ContactDetailsOrBuilder,
com.google.cloud.securitycenter.v2.ContactDetails> {
@java.lang.Override
public com.google.cloud.securitycenter.v2.ContactDetails build(
com.google.cloud.securitycenter.v2.ContactDetailsOrBuilder val) {
if (val instanceof com.google.cloud.securitycenter.v2.ContactDetails) {
return (com.google.cloud.securitycenter.v2.ContactDetails) val;
}
return ((com.google.cloud.securitycenter.v2.ContactDetails.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry<
java.lang.String, com.google.cloud.securitycenter.v2.ContactDetails>
defaultEntry() {
return ContactsDefaultEntryHolder.defaultEntry;
}
};
private static final ContactsConverter contactsConverter = new ContactsConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.securitycenter.v2.ContactDetailsOrBuilder,
com.google.cloud.securitycenter.v2.ContactDetails,
com.google.cloud.securitycenter.v2.ContactDetails.Builder>
contacts_;
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.securitycenter.v2.ContactDetailsOrBuilder,
com.google.cloud.securitycenter.v2.ContactDetails,
com.google.cloud.securitycenter.v2.ContactDetails.Builder>
internalGetContacts() {
if (contacts_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(contactsConverter);
}
return contacts_;
}
private com.google.protobuf.MapFieldBuilder<
java.lang.String,
com.google.cloud.securitycenter.v2.ContactDetailsOrBuilder,
com.google.cloud.securitycenter.v2.ContactDetails,
com.google.cloud.securitycenter.v2.ContactDetails.Builder>
internalGetMutableContacts() {
if (contacts_ == null) {
contacts_ = new com.google.protobuf.MapFieldBuilder<>(contactsConverter);
}
bitField0_ |= 0x00800000;
onChanged();
return contacts_;
}
public int getContactsCount() {
return internalGetContacts().ensureBuilderMap().size();
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public boolean containsContacts(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
return internalGetContacts().ensureBuilderMap().containsKey(key);
}
/** Use {@link #getContactsMap()} instead. */
@java.lang.Override
@java.lang.Deprecated
public java.util.Map
getContacts() {
return getContactsMap();
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public java.util.Map
getContactsMap() {
return internalGetContacts().getImmutableMap();
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public /* nullable */ com.google.cloud.securitycenter.v2.ContactDetails getContactsOrDefault(
java.lang.String key,
/* nullable */
com.google.cloud.securitycenter.v2.ContactDetails defaultValue) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetMutableContacts().ensureBuilderMap();
return map.containsKey(key) ? contactsConverter.build(map.get(key)) : defaultValue;
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
@java.lang.Override
public com.google.cloud.securitycenter.v2.ContactDetails getContactsOrThrow(
java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
java.util.Map
map = internalGetMutableContacts().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return contactsConverter.build(map.get(key));
}
public Builder clearContacts() {
bitField0_ = (bitField0_ & ~0x00800000);
internalGetMutableContacts().clear();
return this;
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder removeContacts(java.lang.String key) {
if (key == null) {
throw new NullPointerException("map key");
}
internalGetMutableContacts().ensureBuilderMap().remove(key);
return this;
}
/** Use alternate mutation accessors instead. */
@java.lang.Deprecated
public java.util.Map
getMutableContacts() {
bitField0_ |= 0x00800000;
return internalGetMutableContacts().ensureMessageMap();
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder putContacts(
java.lang.String key, com.google.cloud.securitycenter.v2.ContactDetails value) {
if (key == null) {
throw new NullPointerException("map key");
}
if (value == null) {
throw new NullPointerException("map value");
}
internalGetMutableContacts().ensureBuilderMap().put(key, value);
bitField0_ |= 0x00800000;
return this;
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public Builder putAllContacts(
java.util.Map values) {
for (java.util.Map.Entry
e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableContacts().ensureBuilderMap().putAll(values);
bitField0_ |= 0x00800000;
return this;
}
/**
*
*
*
* Output only. Map containing the points of contact for the given finding.
* The key represents the type of contact, while the value contains a list of
* all the contacts that pertain. Please refer to:
* https://cloud.google.com/resource-manager/docs/managing-notification-contacts#notification-categories
*
* {
* "security": {
* "contacts": [
* {
* "email": "person1@company.com"
* },
* {
* "email": "person2@company.com"
* }
* ]
* }
* }
*
*
*
* map<string, .google.cloud.securitycenter.v2.ContactDetails> contacts = 26 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
public com.google.cloud.securitycenter.v2.ContactDetails.Builder putContactsBuilderIfAbsent(
java.lang.String key) {
java.util.Map
builderMap = internalGetMutableContacts().ensureBuilderMap();
com.google.cloud.securitycenter.v2.ContactDetailsOrBuilder entry = builderMap.get(key);
if (entry == null) {
entry = com.google.cloud.securitycenter.v2.ContactDetails.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.cloud.securitycenter.v2.ContactDetails) {
entry = ((com.google.cloud.securitycenter.v2.ContactDetails) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.cloud.securitycenter.v2.ContactDetails.Builder) entry;
}
private java.util.List compliances_ =
java.util.Collections.emptyList();
private void ensureCompliancesIsMutable() {
if (!((bitField0_ & 0x01000000) != 0)) {
compliances_ =
new java.util.ArrayList(compliances_);
bitField0_ |= 0x01000000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Compliance,
com.google.cloud.securitycenter.v2.Compliance.Builder,
com.google.cloud.securitycenter.v2.ComplianceOrBuilder>
compliancesBuilder_;
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public java.util.List getCompliancesList() {
if (compliancesBuilder_ == null) {
return java.util.Collections.unmodifiableList(compliances_);
} else {
return compliancesBuilder_.getMessageList();
}
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public int getCompliancesCount() {
if (compliancesBuilder_ == null) {
return compliances_.size();
} else {
return compliancesBuilder_.getCount();
}
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public com.google.cloud.securitycenter.v2.Compliance getCompliances(int index) {
if (compliancesBuilder_ == null) {
return compliances_.get(index);
} else {
return compliancesBuilder_.getMessage(index);
}
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public Builder setCompliances(int index, com.google.cloud.securitycenter.v2.Compliance value) {
if (compliancesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCompliancesIsMutable();
compliances_.set(index, value);
onChanged();
} else {
compliancesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public Builder setCompliances(
int index, com.google.cloud.securitycenter.v2.Compliance.Builder builderForValue) {
if (compliancesBuilder_ == null) {
ensureCompliancesIsMutable();
compliances_.set(index, builderForValue.build());
onChanged();
} else {
compliancesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public Builder addCompliances(com.google.cloud.securitycenter.v2.Compliance value) {
if (compliancesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCompliancesIsMutable();
compliances_.add(value);
onChanged();
} else {
compliancesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public Builder addCompliances(int index, com.google.cloud.securitycenter.v2.Compliance value) {
if (compliancesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureCompliancesIsMutable();
compliances_.add(index, value);
onChanged();
} else {
compliancesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public Builder addCompliances(
com.google.cloud.securitycenter.v2.Compliance.Builder builderForValue) {
if (compliancesBuilder_ == null) {
ensureCompliancesIsMutable();
compliances_.add(builderForValue.build());
onChanged();
} else {
compliancesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public Builder addCompliances(
int index, com.google.cloud.securitycenter.v2.Compliance.Builder builderForValue) {
if (compliancesBuilder_ == null) {
ensureCompliancesIsMutable();
compliances_.add(index, builderForValue.build());
onChanged();
} else {
compliancesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public Builder addAllCompliances(
java.lang.Iterable extends com.google.cloud.securitycenter.v2.Compliance> values) {
if (compliancesBuilder_ == null) {
ensureCompliancesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, compliances_);
onChanged();
} else {
compliancesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public Builder clearCompliances() {
if (compliancesBuilder_ == null) {
compliances_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x01000000);
onChanged();
} else {
compliancesBuilder_.clear();
}
return this;
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public Builder removeCompliances(int index) {
if (compliancesBuilder_ == null) {
ensureCompliancesIsMutable();
compliances_.remove(index);
onChanged();
} else {
compliancesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public com.google.cloud.securitycenter.v2.Compliance.Builder getCompliancesBuilder(int index) {
return getCompliancesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public com.google.cloud.securitycenter.v2.ComplianceOrBuilder getCompliancesOrBuilder(
int index) {
if (compliancesBuilder_ == null) {
return compliances_.get(index);
} else {
return compliancesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public java.util.List extends com.google.cloud.securitycenter.v2.ComplianceOrBuilder>
getCompliancesOrBuilderList() {
if (compliancesBuilder_ != null) {
return compliancesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(compliances_);
}
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public com.google.cloud.securitycenter.v2.Compliance.Builder addCompliancesBuilder() {
return getCompliancesFieldBuilder()
.addBuilder(com.google.cloud.securitycenter.v2.Compliance.getDefaultInstance());
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public com.google.cloud.securitycenter.v2.Compliance.Builder addCompliancesBuilder(int index) {
return getCompliancesFieldBuilder()
.addBuilder(index, com.google.cloud.securitycenter.v2.Compliance.getDefaultInstance());
}
/**
*
*
*
* Contains compliance information for security standards associated to the
* finding.
*
*
* repeated .google.cloud.securitycenter.v2.Compliance compliances = 27;
*/
public java.util.List
getCompliancesBuilderList() {
return getCompliancesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Compliance,
com.google.cloud.securitycenter.v2.Compliance.Builder,
com.google.cloud.securitycenter.v2.ComplianceOrBuilder>
getCompliancesFieldBuilder() {
if (compliancesBuilder_ == null) {
compliancesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Compliance,
com.google.cloud.securitycenter.v2.Compliance.Builder,
com.google.cloud.securitycenter.v2.ComplianceOrBuilder>(
compliances_, ((bitField0_ & 0x01000000) != 0), getParentForChildren(), isClean());
compliances_ = null;
}
return compliancesBuilder_;
}
private java.lang.Object parentDisplayName_ = "";
/**
*
*
*
* Output only. The human readable display name of the finding source such as
* "Event Threat Detection" or "Security Health Analytics".
*
*
* string parent_display_name = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The parentDisplayName.
*/
public java.lang.String getParentDisplayName() {
java.lang.Object ref = parentDisplayName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
parentDisplayName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Output only. The human readable display name of the finding source such as
* "Event Threat Detection" or "Security Health Analytics".
*
*
* string parent_display_name = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for parentDisplayName.
*/
public com.google.protobuf.ByteString getParentDisplayNameBytes() {
java.lang.Object ref = parentDisplayName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
parentDisplayName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Output only. The human readable display name of the finding source such as
* "Event Threat Detection" or "Security Health Analytics".
*
*
* string parent_display_name = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The parentDisplayName to set.
* @return This builder for chaining.
*/
public Builder setParentDisplayName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
parentDisplayName_ = value;
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
*
*
* Output only. The human readable display name of the finding source such as
* "Event Threat Detection" or "Security Health Analytics".
*
*
* string parent_display_name = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return This builder for chaining.
*/
public Builder clearParentDisplayName() {
parentDisplayName_ = getDefaultInstance().getParentDisplayName();
bitField0_ = (bitField0_ & ~0x02000000);
onChanged();
return this;
}
/**
*
*
*
* Output only. The human readable display name of the finding source such as
* "Event Threat Detection" or "Security Health Analytics".
*
*
* string parent_display_name = 29 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @param value The bytes for parentDisplayName to set.
* @return This builder for chaining.
*/
public Builder setParentDisplayNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
parentDisplayName_ = value;
bitField0_ |= 0x02000000;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
*
*
* Contains more details about the finding.
*
*
* string description = 30;
*
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Contains more details about the finding.
*
*
* string description = 30;
*
* @return The bytes for description.
*/
public com.google.protobuf.ByteString getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Contains more details about the finding.
*
*
* string description = 30;
*
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
*
*
*
* Contains more details about the finding.
*
*
* string description = 30;
*
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x04000000);
onChanged();
return this;
}
/**
*
*
*
* Contains more details about the finding.
*
*
* string description = 30;
*
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
bitField0_ |= 0x04000000;
onChanged();
return this;
}
private com.google.cloud.securitycenter.v2.Exfiltration exfiltration_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Exfiltration,
com.google.cloud.securitycenter.v2.Exfiltration.Builder,
com.google.cloud.securitycenter.v2.ExfiltrationOrBuilder>
exfiltrationBuilder_;
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*
* @return Whether the exfiltration field is set.
*/
public boolean hasExfiltration() {
return ((bitField0_ & 0x08000000) != 0);
}
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*
* @return The exfiltration.
*/
public com.google.cloud.securitycenter.v2.Exfiltration getExfiltration() {
if (exfiltrationBuilder_ == null) {
return exfiltration_ == null
? com.google.cloud.securitycenter.v2.Exfiltration.getDefaultInstance()
: exfiltration_;
} else {
return exfiltrationBuilder_.getMessage();
}
}
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*/
public Builder setExfiltration(com.google.cloud.securitycenter.v2.Exfiltration value) {
if (exfiltrationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
exfiltration_ = value;
} else {
exfiltrationBuilder_.setMessage(value);
}
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*/
public Builder setExfiltration(
com.google.cloud.securitycenter.v2.Exfiltration.Builder builderForValue) {
if (exfiltrationBuilder_ == null) {
exfiltration_ = builderForValue.build();
} else {
exfiltrationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*/
public Builder mergeExfiltration(com.google.cloud.securitycenter.v2.Exfiltration value) {
if (exfiltrationBuilder_ == null) {
if (((bitField0_ & 0x08000000) != 0)
&& exfiltration_ != null
&& exfiltration_
!= com.google.cloud.securitycenter.v2.Exfiltration.getDefaultInstance()) {
getExfiltrationBuilder().mergeFrom(value);
} else {
exfiltration_ = value;
}
} else {
exfiltrationBuilder_.mergeFrom(value);
}
if (exfiltration_ != null) {
bitField0_ |= 0x08000000;
onChanged();
}
return this;
}
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*/
public Builder clearExfiltration() {
bitField0_ = (bitField0_ & ~0x08000000);
exfiltration_ = null;
if (exfiltrationBuilder_ != null) {
exfiltrationBuilder_.dispose();
exfiltrationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*/
public com.google.cloud.securitycenter.v2.Exfiltration.Builder getExfiltrationBuilder() {
bitField0_ |= 0x08000000;
onChanged();
return getExfiltrationFieldBuilder().getBuilder();
}
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*/
public com.google.cloud.securitycenter.v2.ExfiltrationOrBuilder getExfiltrationOrBuilder() {
if (exfiltrationBuilder_ != null) {
return exfiltrationBuilder_.getMessageOrBuilder();
} else {
return exfiltration_ == null
? com.google.cloud.securitycenter.v2.Exfiltration.getDefaultInstance()
: exfiltration_;
}
}
/**
*
*
*
* Represents exfiltrations associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Exfiltration exfiltration = 31;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Exfiltration,
com.google.cloud.securitycenter.v2.Exfiltration.Builder,
com.google.cloud.securitycenter.v2.ExfiltrationOrBuilder>
getExfiltrationFieldBuilder() {
if (exfiltrationBuilder_ == null) {
exfiltrationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Exfiltration,
com.google.cloud.securitycenter.v2.Exfiltration.Builder,
com.google.cloud.securitycenter.v2.ExfiltrationOrBuilder>(
getExfiltration(), getParentForChildren(), isClean());
exfiltration_ = null;
}
return exfiltrationBuilder_;
}
private java.util.List iamBindings_ =
java.util.Collections.emptyList();
private void ensureIamBindingsIsMutable() {
if (!((bitField0_ & 0x10000000) != 0)) {
iamBindings_ =
new java.util.ArrayList(iamBindings_);
bitField0_ |= 0x10000000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.IamBinding,
com.google.cloud.securitycenter.v2.IamBinding.Builder,
com.google.cloud.securitycenter.v2.IamBindingOrBuilder>
iamBindingsBuilder_;
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public java.util.List getIamBindingsList() {
if (iamBindingsBuilder_ == null) {
return java.util.Collections.unmodifiableList(iamBindings_);
} else {
return iamBindingsBuilder_.getMessageList();
}
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public int getIamBindingsCount() {
if (iamBindingsBuilder_ == null) {
return iamBindings_.size();
} else {
return iamBindingsBuilder_.getCount();
}
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public com.google.cloud.securitycenter.v2.IamBinding getIamBindings(int index) {
if (iamBindingsBuilder_ == null) {
return iamBindings_.get(index);
} else {
return iamBindingsBuilder_.getMessage(index);
}
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public Builder setIamBindings(int index, com.google.cloud.securitycenter.v2.IamBinding value) {
if (iamBindingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIamBindingsIsMutable();
iamBindings_.set(index, value);
onChanged();
} else {
iamBindingsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public Builder setIamBindings(
int index, com.google.cloud.securitycenter.v2.IamBinding.Builder builderForValue) {
if (iamBindingsBuilder_ == null) {
ensureIamBindingsIsMutable();
iamBindings_.set(index, builderForValue.build());
onChanged();
} else {
iamBindingsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public Builder addIamBindings(com.google.cloud.securitycenter.v2.IamBinding value) {
if (iamBindingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIamBindingsIsMutable();
iamBindings_.add(value);
onChanged();
} else {
iamBindingsBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public Builder addIamBindings(int index, com.google.cloud.securitycenter.v2.IamBinding value) {
if (iamBindingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureIamBindingsIsMutable();
iamBindings_.add(index, value);
onChanged();
} else {
iamBindingsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public Builder addIamBindings(
com.google.cloud.securitycenter.v2.IamBinding.Builder builderForValue) {
if (iamBindingsBuilder_ == null) {
ensureIamBindingsIsMutable();
iamBindings_.add(builderForValue.build());
onChanged();
} else {
iamBindingsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public Builder addIamBindings(
int index, com.google.cloud.securitycenter.v2.IamBinding.Builder builderForValue) {
if (iamBindingsBuilder_ == null) {
ensureIamBindingsIsMutable();
iamBindings_.add(index, builderForValue.build());
onChanged();
} else {
iamBindingsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public Builder addAllIamBindings(
java.lang.Iterable extends com.google.cloud.securitycenter.v2.IamBinding> values) {
if (iamBindingsBuilder_ == null) {
ensureIamBindingsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, iamBindings_);
onChanged();
} else {
iamBindingsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public Builder clearIamBindings() {
if (iamBindingsBuilder_ == null) {
iamBindings_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x10000000);
onChanged();
} else {
iamBindingsBuilder_.clear();
}
return this;
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public Builder removeIamBindings(int index) {
if (iamBindingsBuilder_ == null) {
ensureIamBindingsIsMutable();
iamBindings_.remove(index);
onChanged();
} else {
iamBindingsBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public com.google.cloud.securitycenter.v2.IamBinding.Builder getIamBindingsBuilder(int index) {
return getIamBindingsFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public com.google.cloud.securitycenter.v2.IamBindingOrBuilder getIamBindingsOrBuilder(
int index) {
if (iamBindingsBuilder_ == null) {
return iamBindings_.get(index);
} else {
return iamBindingsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public java.util.List extends com.google.cloud.securitycenter.v2.IamBindingOrBuilder>
getIamBindingsOrBuilderList() {
if (iamBindingsBuilder_ != null) {
return iamBindingsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(iamBindings_);
}
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public com.google.cloud.securitycenter.v2.IamBinding.Builder addIamBindingsBuilder() {
return getIamBindingsFieldBuilder()
.addBuilder(com.google.cloud.securitycenter.v2.IamBinding.getDefaultInstance());
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public com.google.cloud.securitycenter.v2.IamBinding.Builder addIamBindingsBuilder(int index) {
return getIamBindingsFieldBuilder()
.addBuilder(index, com.google.cloud.securitycenter.v2.IamBinding.getDefaultInstance());
}
/**
*
*
*
* Represents IAM bindings associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.IamBinding iam_bindings = 32;
*/
public java.util.List
getIamBindingsBuilderList() {
return getIamBindingsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.IamBinding,
com.google.cloud.securitycenter.v2.IamBinding.Builder,
com.google.cloud.securitycenter.v2.IamBindingOrBuilder>
getIamBindingsFieldBuilder() {
if (iamBindingsBuilder_ == null) {
iamBindingsBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.IamBinding,
com.google.cloud.securitycenter.v2.IamBinding.Builder,
com.google.cloud.securitycenter.v2.IamBindingOrBuilder>(
iamBindings_, ((bitField0_ & 0x10000000) != 0), getParentForChildren(), isClean());
iamBindings_ = null;
}
return iamBindingsBuilder_;
}
private java.lang.Object nextSteps_ = "";
/**
*
*
*
* Steps to address the finding.
*
*
* string next_steps = 33;
*
* @return The nextSteps.
*/
public java.lang.String getNextSteps() {
java.lang.Object ref = nextSteps_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
nextSteps_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Steps to address the finding.
*
*
* string next_steps = 33;
*
* @return The bytes for nextSteps.
*/
public com.google.protobuf.ByteString getNextStepsBytes() {
java.lang.Object ref = nextSteps_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
nextSteps_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Steps to address the finding.
*
*
* string next_steps = 33;
*
* @param value The nextSteps to set.
* @return This builder for chaining.
*/
public Builder setNextSteps(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
nextSteps_ = value;
bitField0_ |= 0x20000000;
onChanged();
return this;
}
/**
*
*
*
* Steps to address the finding.
*
*
* string next_steps = 33;
*
* @return This builder for chaining.
*/
public Builder clearNextSteps() {
nextSteps_ = getDefaultInstance().getNextSteps();
bitField0_ = (bitField0_ & ~0x20000000);
onChanged();
return this;
}
/**
*
*
*
* Steps to address the finding.
*
*
* string next_steps = 33;
*
* @param value The bytes for nextSteps to set.
* @return This builder for chaining.
*/
public Builder setNextStepsBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
nextSteps_ = value;
bitField0_ |= 0x20000000;
onChanged();
return this;
}
private java.lang.Object moduleName_ = "";
/**
*
*
*
* Unique identifier of the module which generated the finding.
* Example:
* folders/598186756061/securityHealthAnalyticsSettings/customModules/56799441161885
*
*
* string module_name = 34;
*
* @return The moduleName.
*/
public java.lang.String getModuleName() {
java.lang.Object ref = moduleName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs = (com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
moduleName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
*
*
* Unique identifier of the module which generated the finding.
* Example:
* folders/598186756061/securityHealthAnalyticsSettings/customModules/56799441161885
*
*
* string module_name = 34;
*
* @return The bytes for moduleName.
*/
public com.google.protobuf.ByteString getModuleNameBytes() {
java.lang.Object ref = moduleName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8((java.lang.String) ref);
moduleName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
*
*
* Unique identifier of the module which generated the finding.
* Example:
* folders/598186756061/securityHealthAnalyticsSettings/customModules/56799441161885
*
*
* string module_name = 34;
*
* @param value The moduleName to set.
* @return This builder for chaining.
*/
public Builder setModuleName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
moduleName_ = value;
bitField0_ |= 0x40000000;
onChanged();
return this;
}
/**
*
*
*
* Unique identifier of the module which generated the finding.
* Example:
* folders/598186756061/securityHealthAnalyticsSettings/customModules/56799441161885
*
*
* string module_name = 34;
*
* @return This builder for chaining.
*/
public Builder clearModuleName() {
moduleName_ = getDefaultInstance().getModuleName();
bitField0_ = (bitField0_ & ~0x40000000);
onChanged();
return this;
}
/**
*
*
*
* Unique identifier of the module which generated the finding.
* Example:
* folders/598186756061/securityHealthAnalyticsSettings/customModules/56799441161885
*
*
* string module_name = 34;
*
* @param value The bytes for moduleName to set.
* @return This builder for chaining.
*/
public Builder setModuleNameBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
moduleName_ = value;
bitField0_ |= 0x40000000;
onChanged();
return this;
}
private java.util.List containers_ =
java.util.Collections.emptyList();
private void ensureContainersIsMutable() {
if (!((bitField0_ & 0x80000000) != 0)) {
containers_ =
new java.util.ArrayList(containers_);
bitField0_ |= 0x80000000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Container,
com.google.cloud.securitycenter.v2.Container.Builder,
com.google.cloud.securitycenter.v2.ContainerOrBuilder>
containersBuilder_;
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public java.util.List getContainersList() {
if (containersBuilder_ == null) {
return java.util.Collections.unmodifiableList(containers_);
} else {
return containersBuilder_.getMessageList();
}
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public int getContainersCount() {
if (containersBuilder_ == null) {
return containers_.size();
} else {
return containersBuilder_.getCount();
}
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public com.google.cloud.securitycenter.v2.Container getContainers(int index) {
if (containersBuilder_ == null) {
return containers_.get(index);
} else {
return containersBuilder_.getMessage(index);
}
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public Builder setContainers(int index, com.google.cloud.securitycenter.v2.Container value) {
if (containersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContainersIsMutable();
containers_.set(index, value);
onChanged();
} else {
containersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public Builder setContainers(
int index, com.google.cloud.securitycenter.v2.Container.Builder builderForValue) {
if (containersBuilder_ == null) {
ensureContainersIsMutable();
containers_.set(index, builderForValue.build());
onChanged();
} else {
containersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public Builder addContainers(com.google.cloud.securitycenter.v2.Container value) {
if (containersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContainersIsMutable();
containers_.add(value);
onChanged();
} else {
containersBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public Builder addContainers(int index, com.google.cloud.securitycenter.v2.Container value) {
if (containersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureContainersIsMutable();
containers_.add(index, value);
onChanged();
} else {
containersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public Builder addContainers(
com.google.cloud.securitycenter.v2.Container.Builder builderForValue) {
if (containersBuilder_ == null) {
ensureContainersIsMutable();
containers_.add(builderForValue.build());
onChanged();
} else {
containersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public Builder addContainers(
int index, com.google.cloud.securitycenter.v2.Container.Builder builderForValue) {
if (containersBuilder_ == null) {
ensureContainersIsMutable();
containers_.add(index, builderForValue.build());
onChanged();
} else {
containersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public Builder addAllContainers(
java.lang.Iterable extends com.google.cloud.securitycenter.v2.Container> values) {
if (containersBuilder_ == null) {
ensureContainersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, containers_);
onChanged();
} else {
containersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public Builder clearContainers() {
if (containersBuilder_ == null) {
containers_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x80000000);
onChanged();
} else {
containersBuilder_.clear();
}
return this;
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public Builder removeContainers(int index) {
if (containersBuilder_ == null) {
ensureContainersIsMutable();
containers_.remove(index);
onChanged();
} else {
containersBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public com.google.cloud.securitycenter.v2.Container.Builder getContainersBuilder(int index) {
return getContainersFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public com.google.cloud.securitycenter.v2.ContainerOrBuilder getContainersOrBuilder(int index) {
if (containersBuilder_ == null) {
return containers_.get(index);
} else {
return containersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public java.util.List extends com.google.cloud.securitycenter.v2.ContainerOrBuilder>
getContainersOrBuilderList() {
if (containersBuilder_ != null) {
return containersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(containers_);
}
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public com.google.cloud.securitycenter.v2.Container.Builder addContainersBuilder() {
return getContainersFieldBuilder()
.addBuilder(com.google.cloud.securitycenter.v2.Container.getDefaultInstance());
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public com.google.cloud.securitycenter.v2.Container.Builder addContainersBuilder(int index) {
return getContainersFieldBuilder()
.addBuilder(index, com.google.cloud.securitycenter.v2.Container.getDefaultInstance());
}
/**
*
*
*
* Containers associated with the finding. This field provides information for
* both Kubernetes and non-Kubernetes containers.
*
*
* repeated .google.cloud.securitycenter.v2.Container containers = 35;
*/
public java.util.List
getContainersBuilderList() {
return getContainersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Container,
com.google.cloud.securitycenter.v2.Container.Builder,
com.google.cloud.securitycenter.v2.ContainerOrBuilder>
getContainersFieldBuilder() {
if (containersBuilder_ == null) {
containersBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.Container,
com.google.cloud.securitycenter.v2.Container.Builder,
com.google.cloud.securitycenter.v2.ContainerOrBuilder>(
containers_, ((bitField0_ & 0x80000000) != 0), getParentForChildren(), isClean());
containers_ = null;
}
return containersBuilder_;
}
private com.google.cloud.securitycenter.v2.Kubernetes kubernetes_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Kubernetes,
com.google.cloud.securitycenter.v2.Kubernetes.Builder,
com.google.cloud.securitycenter.v2.KubernetesOrBuilder>
kubernetesBuilder_;
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*
* @return Whether the kubernetes field is set.
*/
public boolean hasKubernetes() {
return ((bitField1_ & 0x00000001) != 0);
}
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*
* @return The kubernetes.
*/
public com.google.cloud.securitycenter.v2.Kubernetes getKubernetes() {
if (kubernetesBuilder_ == null) {
return kubernetes_ == null
? com.google.cloud.securitycenter.v2.Kubernetes.getDefaultInstance()
: kubernetes_;
} else {
return kubernetesBuilder_.getMessage();
}
}
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*/
public Builder setKubernetes(com.google.cloud.securitycenter.v2.Kubernetes value) {
if (kubernetesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kubernetes_ = value;
} else {
kubernetesBuilder_.setMessage(value);
}
bitField1_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*/
public Builder setKubernetes(
com.google.cloud.securitycenter.v2.Kubernetes.Builder builderForValue) {
if (kubernetesBuilder_ == null) {
kubernetes_ = builderForValue.build();
} else {
kubernetesBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000001;
onChanged();
return this;
}
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*/
public Builder mergeKubernetes(com.google.cloud.securitycenter.v2.Kubernetes value) {
if (kubernetesBuilder_ == null) {
if (((bitField1_ & 0x00000001) != 0)
&& kubernetes_ != null
&& kubernetes_ != com.google.cloud.securitycenter.v2.Kubernetes.getDefaultInstance()) {
getKubernetesBuilder().mergeFrom(value);
} else {
kubernetes_ = value;
}
} else {
kubernetesBuilder_.mergeFrom(value);
}
if (kubernetes_ != null) {
bitField1_ |= 0x00000001;
onChanged();
}
return this;
}
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*/
public Builder clearKubernetes() {
bitField1_ = (bitField1_ & ~0x00000001);
kubernetes_ = null;
if (kubernetesBuilder_ != null) {
kubernetesBuilder_.dispose();
kubernetesBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*/
public com.google.cloud.securitycenter.v2.Kubernetes.Builder getKubernetesBuilder() {
bitField1_ |= 0x00000001;
onChanged();
return getKubernetesFieldBuilder().getBuilder();
}
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*/
public com.google.cloud.securitycenter.v2.KubernetesOrBuilder getKubernetesOrBuilder() {
if (kubernetesBuilder_ != null) {
return kubernetesBuilder_.getMessageOrBuilder();
} else {
return kubernetes_ == null
? com.google.cloud.securitycenter.v2.Kubernetes.getDefaultInstance()
: kubernetes_;
}
}
/**
*
*
*
* Kubernetes resources associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Kubernetes kubernetes = 36;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Kubernetes,
com.google.cloud.securitycenter.v2.Kubernetes.Builder,
com.google.cloud.securitycenter.v2.KubernetesOrBuilder>
getKubernetesFieldBuilder() {
if (kubernetesBuilder_ == null) {
kubernetesBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Kubernetes,
com.google.cloud.securitycenter.v2.Kubernetes.Builder,
com.google.cloud.securitycenter.v2.KubernetesOrBuilder>(
getKubernetes(), getParentForChildren(), isClean());
kubernetes_ = null;
}
return kubernetesBuilder_;
}
private com.google.cloud.securitycenter.v2.Database database_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Database,
com.google.cloud.securitycenter.v2.Database.Builder,
com.google.cloud.securitycenter.v2.DatabaseOrBuilder>
databaseBuilder_;
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*
* @return Whether the database field is set.
*/
public boolean hasDatabase() {
return ((bitField1_ & 0x00000002) != 0);
}
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*
* @return The database.
*/
public com.google.cloud.securitycenter.v2.Database getDatabase() {
if (databaseBuilder_ == null) {
return database_ == null
? com.google.cloud.securitycenter.v2.Database.getDefaultInstance()
: database_;
} else {
return databaseBuilder_.getMessage();
}
}
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*/
public Builder setDatabase(com.google.cloud.securitycenter.v2.Database value) {
if (databaseBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
database_ = value;
} else {
databaseBuilder_.setMessage(value);
}
bitField1_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*/
public Builder setDatabase(
com.google.cloud.securitycenter.v2.Database.Builder builderForValue) {
if (databaseBuilder_ == null) {
database_ = builderForValue.build();
} else {
databaseBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000002;
onChanged();
return this;
}
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*/
public Builder mergeDatabase(com.google.cloud.securitycenter.v2.Database value) {
if (databaseBuilder_ == null) {
if (((bitField1_ & 0x00000002) != 0)
&& database_ != null
&& database_ != com.google.cloud.securitycenter.v2.Database.getDefaultInstance()) {
getDatabaseBuilder().mergeFrom(value);
} else {
database_ = value;
}
} else {
databaseBuilder_.mergeFrom(value);
}
if (database_ != null) {
bitField1_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*/
public Builder clearDatabase() {
bitField1_ = (bitField1_ & ~0x00000002);
database_ = null;
if (databaseBuilder_ != null) {
databaseBuilder_.dispose();
databaseBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*/
public com.google.cloud.securitycenter.v2.Database.Builder getDatabaseBuilder() {
bitField1_ |= 0x00000002;
onChanged();
return getDatabaseFieldBuilder().getBuilder();
}
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*/
public com.google.cloud.securitycenter.v2.DatabaseOrBuilder getDatabaseOrBuilder() {
if (databaseBuilder_ != null) {
return databaseBuilder_.getMessageOrBuilder();
} else {
return database_ == null
? com.google.cloud.securitycenter.v2.Database.getDefaultInstance()
: database_;
}
}
/**
*
*
*
* Database associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Database database = 37;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Database,
com.google.cloud.securitycenter.v2.Database.Builder,
com.google.cloud.securitycenter.v2.DatabaseOrBuilder>
getDatabaseFieldBuilder() {
if (databaseBuilder_ == null) {
databaseBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Database,
com.google.cloud.securitycenter.v2.Database.Builder,
com.google.cloud.securitycenter.v2.DatabaseOrBuilder>(
getDatabase(), getParentForChildren(), isClean());
database_ = null;
}
return databaseBuilder_;
}
private com.google.cloud.securitycenter.v2.AttackExposure attackExposure_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.AttackExposure,
com.google.cloud.securitycenter.v2.AttackExposure.Builder,
com.google.cloud.securitycenter.v2.AttackExposureOrBuilder>
attackExposureBuilder_;
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*
* @return Whether the attackExposure field is set.
*/
public boolean hasAttackExposure() {
return ((bitField1_ & 0x00000004) != 0);
}
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*
* @return The attackExposure.
*/
public com.google.cloud.securitycenter.v2.AttackExposure getAttackExposure() {
if (attackExposureBuilder_ == null) {
return attackExposure_ == null
? com.google.cloud.securitycenter.v2.AttackExposure.getDefaultInstance()
: attackExposure_;
} else {
return attackExposureBuilder_.getMessage();
}
}
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*/
public Builder setAttackExposure(com.google.cloud.securitycenter.v2.AttackExposure value) {
if (attackExposureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
attackExposure_ = value;
} else {
attackExposureBuilder_.setMessage(value);
}
bitField1_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*/
public Builder setAttackExposure(
com.google.cloud.securitycenter.v2.AttackExposure.Builder builderForValue) {
if (attackExposureBuilder_ == null) {
attackExposure_ = builderForValue.build();
} else {
attackExposureBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000004;
onChanged();
return this;
}
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*/
public Builder mergeAttackExposure(com.google.cloud.securitycenter.v2.AttackExposure value) {
if (attackExposureBuilder_ == null) {
if (((bitField1_ & 0x00000004) != 0)
&& attackExposure_ != null
&& attackExposure_
!= com.google.cloud.securitycenter.v2.AttackExposure.getDefaultInstance()) {
getAttackExposureBuilder().mergeFrom(value);
} else {
attackExposure_ = value;
}
} else {
attackExposureBuilder_.mergeFrom(value);
}
if (attackExposure_ != null) {
bitField1_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*/
public Builder clearAttackExposure() {
bitField1_ = (bitField1_ & ~0x00000004);
attackExposure_ = null;
if (attackExposureBuilder_ != null) {
attackExposureBuilder_.dispose();
attackExposureBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*/
public com.google.cloud.securitycenter.v2.AttackExposure.Builder getAttackExposureBuilder() {
bitField1_ |= 0x00000004;
onChanged();
return getAttackExposureFieldBuilder().getBuilder();
}
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*/
public com.google.cloud.securitycenter.v2.AttackExposureOrBuilder getAttackExposureOrBuilder() {
if (attackExposureBuilder_ != null) {
return attackExposureBuilder_.getMessageOrBuilder();
} else {
return attackExposure_ == null
? com.google.cloud.securitycenter.v2.AttackExposure.getDefaultInstance()
: attackExposure_;
}
}
/**
*
*
*
* The results of an attack path simulation relevant to this finding.
*
*
* .google.cloud.securitycenter.v2.AttackExposure attack_exposure = 38;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.AttackExposure,
com.google.cloud.securitycenter.v2.AttackExposure.Builder,
com.google.cloud.securitycenter.v2.AttackExposureOrBuilder>
getAttackExposureFieldBuilder() {
if (attackExposureBuilder_ == null) {
attackExposureBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.AttackExposure,
com.google.cloud.securitycenter.v2.AttackExposure.Builder,
com.google.cloud.securitycenter.v2.AttackExposureOrBuilder>(
getAttackExposure(), getParentForChildren(), isClean());
attackExposure_ = null;
}
return attackExposureBuilder_;
}
private java.util.List files_ =
java.util.Collections.emptyList();
private void ensureFilesIsMutable() {
if (!((bitField1_ & 0x00000008) != 0)) {
files_ = new java.util.ArrayList(files_);
bitField1_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.File,
com.google.cloud.securitycenter.v2.File.Builder,
com.google.cloud.securitycenter.v2.FileOrBuilder>
filesBuilder_;
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public java.util.List getFilesList() {
if (filesBuilder_ == null) {
return java.util.Collections.unmodifiableList(files_);
} else {
return filesBuilder_.getMessageList();
}
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public int getFilesCount() {
if (filesBuilder_ == null) {
return files_.size();
} else {
return filesBuilder_.getCount();
}
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public com.google.cloud.securitycenter.v2.File getFiles(int index) {
if (filesBuilder_ == null) {
return files_.get(index);
} else {
return filesBuilder_.getMessage(index);
}
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public Builder setFiles(int index, com.google.cloud.securitycenter.v2.File value) {
if (filesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFilesIsMutable();
files_.set(index, value);
onChanged();
} else {
filesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public Builder setFiles(
int index, com.google.cloud.securitycenter.v2.File.Builder builderForValue) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.set(index, builderForValue.build());
onChanged();
} else {
filesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public Builder addFiles(com.google.cloud.securitycenter.v2.File value) {
if (filesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFilesIsMutable();
files_.add(value);
onChanged();
} else {
filesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public Builder addFiles(int index, com.google.cloud.securitycenter.v2.File value) {
if (filesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFilesIsMutable();
files_.add(index, value);
onChanged();
} else {
filesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public Builder addFiles(com.google.cloud.securitycenter.v2.File.Builder builderForValue) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.add(builderForValue.build());
onChanged();
} else {
filesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public Builder addFiles(
int index, com.google.cloud.securitycenter.v2.File.Builder builderForValue) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.add(index, builderForValue.build());
onChanged();
} else {
filesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public Builder addAllFiles(
java.lang.Iterable extends com.google.cloud.securitycenter.v2.File> values) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, files_);
onChanged();
} else {
filesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public Builder clearFiles() {
if (filesBuilder_ == null) {
files_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000008);
onChanged();
} else {
filesBuilder_.clear();
}
return this;
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public Builder removeFiles(int index) {
if (filesBuilder_ == null) {
ensureFilesIsMutable();
files_.remove(index);
onChanged();
} else {
filesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public com.google.cloud.securitycenter.v2.File.Builder getFilesBuilder(int index) {
return getFilesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public com.google.cloud.securitycenter.v2.FileOrBuilder getFilesOrBuilder(int index) {
if (filesBuilder_ == null) {
return files_.get(index);
} else {
return filesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public java.util.List extends com.google.cloud.securitycenter.v2.FileOrBuilder>
getFilesOrBuilderList() {
if (filesBuilder_ != null) {
return filesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(files_);
}
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public com.google.cloud.securitycenter.v2.File.Builder addFilesBuilder() {
return getFilesFieldBuilder()
.addBuilder(com.google.cloud.securitycenter.v2.File.getDefaultInstance());
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public com.google.cloud.securitycenter.v2.File.Builder addFilesBuilder(int index) {
return getFilesFieldBuilder()
.addBuilder(index, com.google.cloud.securitycenter.v2.File.getDefaultInstance());
}
/**
*
*
*
* File associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.File files = 39;
*/
public java.util.List getFilesBuilderList() {
return getFilesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.File,
com.google.cloud.securitycenter.v2.File.Builder,
com.google.cloud.securitycenter.v2.FileOrBuilder>
getFilesFieldBuilder() {
if (filesBuilder_ == null) {
filesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.File,
com.google.cloud.securitycenter.v2.File.Builder,
com.google.cloud.securitycenter.v2.FileOrBuilder>(
files_, ((bitField1_ & 0x00000008) != 0), getParentForChildren(), isClean());
files_ = null;
}
return filesBuilder_;
}
private com.google.cloud.securitycenter.v2.CloudDlpInspection cloudDlpInspection_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.CloudDlpInspection,
com.google.cloud.securitycenter.v2.CloudDlpInspection.Builder,
com.google.cloud.securitycenter.v2.CloudDlpInspectionOrBuilder>
cloudDlpInspectionBuilder_;
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*
* @return Whether the cloudDlpInspection field is set.
*/
public boolean hasCloudDlpInspection() {
return ((bitField1_ & 0x00000010) != 0);
}
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*
* @return The cloudDlpInspection.
*/
public com.google.cloud.securitycenter.v2.CloudDlpInspection getCloudDlpInspection() {
if (cloudDlpInspectionBuilder_ == null) {
return cloudDlpInspection_ == null
? com.google.cloud.securitycenter.v2.CloudDlpInspection.getDefaultInstance()
: cloudDlpInspection_;
} else {
return cloudDlpInspectionBuilder_.getMessage();
}
}
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*/
public Builder setCloudDlpInspection(
com.google.cloud.securitycenter.v2.CloudDlpInspection value) {
if (cloudDlpInspectionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cloudDlpInspection_ = value;
} else {
cloudDlpInspectionBuilder_.setMessage(value);
}
bitField1_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*/
public Builder setCloudDlpInspection(
com.google.cloud.securitycenter.v2.CloudDlpInspection.Builder builderForValue) {
if (cloudDlpInspectionBuilder_ == null) {
cloudDlpInspection_ = builderForValue.build();
} else {
cloudDlpInspectionBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000010;
onChanged();
return this;
}
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*/
public Builder mergeCloudDlpInspection(
com.google.cloud.securitycenter.v2.CloudDlpInspection value) {
if (cloudDlpInspectionBuilder_ == null) {
if (((bitField1_ & 0x00000010) != 0)
&& cloudDlpInspection_ != null
&& cloudDlpInspection_
!= com.google.cloud.securitycenter.v2.CloudDlpInspection.getDefaultInstance()) {
getCloudDlpInspectionBuilder().mergeFrom(value);
} else {
cloudDlpInspection_ = value;
}
} else {
cloudDlpInspectionBuilder_.mergeFrom(value);
}
if (cloudDlpInspection_ != null) {
bitField1_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*/
public Builder clearCloudDlpInspection() {
bitField1_ = (bitField1_ & ~0x00000010);
cloudDlpInspection_ = null;
if (cloudDlpInspectionBuilder_ != null) {
cloudDlpInspectionBuilder_.dispose();
cloudDlpInspectionBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*/
public com.google.cloud.securitycenter.v2.CloudDlpInspection.Builder
getCloudDlpInspectionBuilder() {
bitField1_ |= 0x00000010;
onChanged();
return getCloudDlpInspectionFieldBuilder().getBuilder();
}
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*/
public com.google.cloud.securitycenter.v2.CloudDlpInspectionOrBuilder
getCloudDlpInspectionOrBuilder() {
if (cloudDlpInspectionBuilder_ != null) {
return cloudDlpInspectionBuilder_.getMessageOrBuilder();
} else {
return cloudDlpInspection_ == null
? com.google.cloud.securitycenter.v2.CloudDlpInspection.getDefaultInstance()
: cloudDlpInspection_;
}
}
/**
*
*
*
* Cloud Data Loss Prevention (Cloud DLP) inspection results that are
* associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpInspection cloud_dlp_inspection = 40;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.CloudDlpInspection,
com.google.cloud.securitycenter.v2.CloudDlpInspection.Builder,
com.google.cloud.securitycenter.v2.CloudDlpInspectionOrBuilder>
getCloudDlpInspectionFieldBuilder() {
if (cloudDlpInspectionBuilder_ == null) {
cloudDlpInspectionBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.CloudDlpInspection,
com.google.cloud.securitycenter.v2.CloudDlpInspection.Builder,
com.google.cloud.securitycenter.v2.CloudDlpInspectionOrBuilder>(
getCloudDlpInspection(), getParentForChildren(), isClean());
cloudDlpInspection_ = null;
}
return cloudDlpInspectionBuilder_;
}
private com.google.cloud.securitycenter.v2.CloudDlpDataProfile cloudDlpDataProfile_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.CloudDlpDataProfile,
com.google.cloud.securitycenter.v2.CloudDlpDataProfile.Builder,
com.google.cloud.securitycenter.v2.CloudDlpDataProfileOrBuilder>
cloudDlpDataProfileBuilder_;
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*
* @return Whether the cloudDlpDataProfile field is set.
*/
public boolean hasCloudDlpDataProfile() {
return ((bitField1_ & 0x00000020) != 0);
}
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*
* @return The cloudDlpDataProfile.
*/
public com.google.cloud.securitycenter.v2.CloudDlpDataProfile getCloudDlpDataProfile() {
if (cloudDlpDataProfileBuilder_ == null) {
return cloudDlpDataProfile_ == null
? com.google.cloud.securitycenter.v2.CloudDlpDataProfile.getDefaultInstance()
: cloudDlpDataProfile_;
} else {
return cloudDlpDataProfileBuilder_.getMessage();
}
}
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*/
public Builder setCloudDlpDataProfile(
com.google.cloud.securitycenter.v2.CloudDlpDataProfile value) {
if (cloudDlpDataProfileBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
cloudDlpDataProfile_ = value;
} else {
cloudDlpDataProfileBuilder_.setMessage(value);
}
bitField1_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*/
public Builder setCloudDlpDataProfile(
com.google.cloud.securitycenter.v2.CloudDlpDataProfile.Builder builderForValue) {
if (cloudDlpDataProfileBuilder_ == null) {
cloudDlpDataProfile_ = builderForValue.build();
} else {
cloudDlpDataProfileBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000020;
onChanged();
return this;
}
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*/
public Builder mergeCloudDlpDataProfile(
com.google.cloud.securitycenter.v2.CloudDlpDataProfile value) {
if (cloudDlpDataProfileBuilder_ == null) {
if (((bitField1_ & 0x00000020) != 0)
&& cloudDlpDataProfile_ != null
&& cloudDlpDataProfile_
!= com.google.cloud.securitycenter.v2.CloudDlpDataProfile.getDefaultInstance()) {
getCloudDlpDataProfileBuilder().mergeFrom(value);
} else {
cloudDlpDataProfile_ = value;
}
} else {
cloudDlpDataProfileBuilder_.mergeFrom(value);
}
if (cloudDlpDataProfile_ != null) {
bitField1_ |= 0x00000020;
onChanged();
}
return this;
}
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*/
public Builder clearCloudDlpDataProfile() {
bitField1_ = (bitField1_ & ~0x00000020);
cloudDlpDataProfile_ = null;
if (cloudDlpDataProfileBuilder_ != null) {
cloudDlpDataProfileBuilder_.dispose();
cloudDlpDataProfileBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*/
public com.google.cloud.securitycenter.v2.CloudDlpDataProfile.Builder
getCloudDlpDataProfileBuilder() {
bitField1_ |= 0x00000020;
onChanged();
return getCloudDlpDataProfileFieldBuilder().getBuilder();
}
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*/
public com.google.cloud.securitycenter.v2.CloudDlpDataProfileOrBuilder
getCloudDlpDataProfileOrBuilder() {
if (cloudDlpDataProfileBuilder_ != null) {
return cloudDlpDataProfileBuilder_.getMessageOrBuilder();
} else {
return cloudDlpDataProfile_ == null
? com.google.cloud.securitycenter.v2.CloudDlpDataProfile.getDefaultInstance()
: cloudDlpDataProfile_;
}
}
/**
*
*
*
* Cloud DLP data profile that is associated with the finding.
*
*
* .google.cloud.securitycenter.v2.CloudDlpDataProfile cloud_dlp_data_profile = 41;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.CloudDlpDataProfile,
com.google.cloud.securitycenter.v2.CloudDlpDataProfile.Builder,
com.google.cloud.securitycenter.v2.CloudDlpDataProfileOrBuilder>
getCloudDlpDataProfileFieldBuilder() {
if (cloudDlpDataProfileBuilder_ == null) {
cloudDlpDataProfileBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.CloudDlpDataProfile,
com.google.cloud.securitycenter.v2.CloudDlpDataProfile.Builder,
com.google.cloud.securitycenter.v2.CloudDlpDataProfileOrBuilder>(
getCloudDlpDataProfile(), getParentForChildren(), isClean());
cloudDlpDataProfile_ = null;
}
return cloudDlpDataProfileBuilder_;
}
private com.google.cloud.securitycenter.v2.KernelRootkit kernelRootkit_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.KernelRootkit,
com.google.cloud.securitycenter.v2.KernelRootkit.Builder,
com.google.cloud.securitycenter.v2.KernelRootkitOrBuilder>
kernelRootkitBuilder_;
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*
* @return Whether the kernelRootkit field is set.
*/
public boolean hasKernelRootkit() {
return ((bitField1_ & 0x00000040) != 0);
}
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*
* @return The kernelRootkit.
*/
public com.google.cloud.securitycenter.v2.KernelRootkit getKernelRootkit() {
if (kernelRootkitBuilder_ == null) {
return kernelRootkit_ == null
? com.google.cloud.securitycenter.v2.KernelRootkit.getDefaultInstance()
: kernelRootkit_;
} else {
return kernelRootkitBuilder_.getMessage();
}
}
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*/
public Builder setKernelRootkit(com.google.cloud.securitycenter.v2.KernelRootkit value) {
if (kernelRootkitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kernelRootkit_ = value;
} else {
kernelRootkitBuilder_.setMessage(value);
}
bitField1_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*/
public Builder setKernelRootkit(
com.google.cloud.securitycenter.v2.KernelRootkit.Builder builderForValue) {
if (kernelRootkitBuilder_ == null) {
kernelRootkit_ = builderForValue.build();
} else {
kernelRootkitBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000040;
onChanged();
return this;
}
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*/
public Builder mergeKernelRootkit(com.google.cloud.securitycenter.v2.KernelRootkit value) {
if (kernelRootkitBuilder_ == null) {
if (((bitField1_ & 0x00000040) != 0)
&& kernelRootkit_ != null
&& kernelRootkit_
!= com.google.cloud.securitycenter.v2.KernelRootkit.getDefaultInstance()) {
getKernelRootkitBuilder().mergeFrom(value);
} else {
kernelRootkit_ = value;
}
} else {
kernelRootkitBuilder_.mergeFrom(value);
}
if (kernelRootkit_ != null) {
bitField1_ |= 0x00000040;
onChanged();
}
return this;
}
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*/
public Builder clearKernelRootkit() {
bitField1_ = (bitField1_ & ~0x00000040);
kernelRootkit_ = null;
if (kernelRootkitBuilder_ != null) {
kernelRootkitBuilder_.dispose();
kernelRootkitBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*/
public com.google.cloud.securitycenter.v2.KernelRootkit.Builder getKernelRootkitBuilder() {
bitField1_ |= 0x00000040;
onChanged();
return getKernelRootkitFieldBuilder().getBuilder();
}
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*/
public com.google.cloud.securitycenter.v2.KernelRootkitOrBuilder getKernelRootkitOrBuilder() {
if (kernelRootkitBuilder_ != null) {
return kernelRootkitBuilder_.getMessageOrBuilder();
} else {
return kernelRootkit_ == null
? com.google.cloud.securitycenter.v2.KernelRootkit.getDefaultInstance()
: kernelRootkit_;
}
}
/**
*
*
*
* Signature of the kernel rootkit.
*
*
* .google.cloud.securitycenter.v2.KernelRootkit kernel_rootkit = 42;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.KernelRootkit,
com.google.cloud.securitycenter.v2.KernelRootkit.Builder,
com.google.cloud.securitycenter.v2.KernelRootkitOrBuilder>
getKernelRootkitFieldBuilder() {
if (kernelRootkitBuilder_ == null) {
kernelRootkitBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.KernelRootkit,
com.google.cloud.securitycenter.v2.KernelRootkit.Builder,
com.google.cloud.securitycenter.v2.KernelRootkitOrBuilder>(
getKernelRootkit(), getParentForChildren(), isClean());
kernelRootkit_ = null;
}
return kernelRootkitBuilder_;
}
private java.util.List orgPolicies_ =
java.util.Collections.emptyList();
private void ensureOrgPoliciesIsMutable() {
if (!((bitField1_ & 0x00000080) != 0)) {
orgPolicies_ =
new java.util.ArrayList(orgPolicies_);
bitField1_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.OrgPolicy,
com.google.cloud.securitycenter.v2.OrgPolicy.Builder,
com.google.cloud.securitycenter.v2.OrgPolicyOrBuilder>
orgPoliciesBuilder_;
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public java.util.List getOrgPoliciesList() {
if (orgPoliciesBuilder_ == null) {
return java.util.Collections.unmodifiableList(orgPolicies_);
} else {
return orgPoliciesBuilder_.getMessageList();
}
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public int getOrgPoliciesCount() {
if (orgPoliciesBuilder_ == null) {
return orgPolicies_.size();
} else {
return orgPoliciesBuilder_.getCount();
}
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public com.google.cloud.securitycenter.v2.OrgPolicy getOrgPolicies(int index) {
if (orgPoliciesBuilder_ == null) {
return orgPolicies_.get(index);
} else {
return orgPoliciesBuilder_.getMessage(index);
}
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public Builder setOrgPolicies(int index, com.google.cloud.securitycenter.v2.OrgPolicy value) {
if (orgPoliciesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrgPoliciesIsMutable();
orgPolicies_.set(index, value);
onChanged();
} else {
orgPoliciesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public Builder setOrgPolicies(
int index, com.google.cloud.securitycenter.v2.OrgPolicy.Builder builderForValue) {
if (orgPoliciesBuilder_ == null) {
ensureOrgPoliciesIsMutable();
orgPolicies_.set(index, builderForValue.build());
onChanged();
} else {
orgPoliciesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public Builder addOrgPolicies(com.google.cloud.securitycenter.v2.OrgPolicy value) {
if (orgPoliciesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrgPoliciesIsMutable();
orgPolicies_.add(value);
onChanged();
} else {
orgPoliciesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public Builder addOrgPolicies(int index, com.google.cloud.securitycenter.v2.OrgPolicy value) {
if (orgPoliciesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureOrgPoliciesIsMutable();
orgPolicies_.add(index, value);
onChanged();
} else {
orgPoliciesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public Builder addOrgPolicies(
com.google.cloud.securitycenter.v2.OrgPolicy.Builder builderForValue) {
if (orgPoliciesBuilder_ == null) {
ensureOrgPoliciesIsMutable();
orgPolicies_.add(builderForValue.build());
onChanged();
} else {
orgPoliciesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public Builder addOrgPolicies(
int index, com.google.cloud.securitycenter.v2.OrgPolicy.Builder builderForValue) {
if (orgPoliciesBuilder_ == null) {
ensureOrgPoliciesIsMutable();
orgPolicies_.add(index, builderForValue.build());
onChanged();
} else {
orgPoliciesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public Builder addAllOrgPolicies(
java.lang.Iterable extends com.google.cloud.securitycenter.v2.OrgPolicy> values) {
if (orgPoliciesBuilder_ == null) {
ensureOrgPoliciesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, orgPolicies_);
onChanged();
} else {
orgPoliciesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public Builder clearOrgPolicies() {
if (orgPoliciesBuilder_ == null) {
orgPolicies_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000080);
onChanged();
} else {
orgPoliciesBuilder_.clear();
}
return this;
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public Builder removeOrgPolicies(int index) {
if (orgPoliciesBuilder_ == null) {
ensureOrgPoliciesIsMutable();
orgPolicies_.remove(index);
onChanged();
} else {
orgPoliciesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public com.google.cloud.securitycenter.v2.OrgPolicy.Builder getOrgPoliciesBuilder(int index) {
return getOrgPoliciesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public com.google.cloud.securitycenter.v2.OrgPolicyOrBuilder getOrgPoliciesOrBuilder(
int index) {
if (orgPoliciesBuilder_ == null) {
return orgPolicies_.get(index);
} else {
return orgPoliciesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public java.util.List extends com.google.cloud.securitycenter.v2.OrgPolicyOrBuilder>
getOrgPoliciesOrBuilderList() {
if (orgPoliciesBuilder_ != null) {
return orgPoliciesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(orgPolicies_);
}
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public com.google.cloud.securitycenter.v2.OrgPolicy.Builder addOrgPoliciesBuilder() {
return getOrgPoliciesFieldBuilder()
.addBuilder(com.google.cloud.securitycenter.v2.OrgPolicy.getDefaultInstance());
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public com.google.cloud.securitycenter.v2.OrgPolicy.Builder addOrgPoliciesBuilder(int index) {
return getOrgPoliciesFieldBuilder()
.addBuilder(index, com.google.cloud.securitycenter.v2.OrgPolicy.getDefaultInstance());
}
/**
*
*
*
* Contains information about the org policies associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.OrgPolicy org_policies = 43;
*/
public java.util.List
getOrgPoliciesBuilderList() {
return getOrgPoliciesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.OrgPolicy,
com.google.cloud.securitycenter.v2.OrgPolicy.Builder,
com.google.cloud.securitycenter.v2.OrgPolicyOrBuilder>
getOrgPoliciesFieldBuilder() {
if (orgPoliciesBuilder_ == null) {
orgPoliciesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.OrgPolicy,
com.google.cloud.securitycenter.v2.OrgPolicy.Builder,
com.google.cloud.securitycenter.v2.OrgPolicyOrBuilder>(
orgPolicies_, ((bitField1_ & 0x00000080) != 0), getParentForChildren(), isClean());
orgPolicies_ = null;
}
return orgPoliciesBuilder_;
}
private com.google.cloud.securitycenter.v2.Application application_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Application,
com.google.cloud.securitycenter.v2.Application.Builder,
com.google.cloud.securitycenter.v2.ApplicationOrBuilder>
applicationBuilder_;
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*
* @return Whether the application field is set.
*/
public boolean hasApplication() {
return ((bitField1_ & 0x00000100) != 0);
}
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*
* @return The application.
*/
public com.google.cloud.securitycenter.v2.Application getApplication() {
if (applicationBuilder_ == null) {
return application_ == null
? com.google.cloud.securitycenter.v2.Application.getDefaultInstance()
: application_;
} else {
return applicationBuilder_.getMessage();
}
}
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*/
public Builder setApplication(com.google.cloud.securitycenter.v2.Application value) {
if (applicationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
application_ = value;
} else {
applicationBuilder_.setMessage(value);
}
bitField1_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*/
public Builder setApplication(
com.google.cloud.securitycenter.v2.Application.Builder builderForValue) {
if (applicationBuilder_ == null) {
application_ = builderForValue.build();
} else {
applicationBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000100;
onChanged();
return this;
}
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*/
public Builder mergeApplication(com.google.cloud.securitycenter.v2.Application value) {
if (applicationBuilder_ == null) {
if (((bitField1_ & 0x00000100) != 0)
&& application_ != null
&& application_
!= com.google.cloud.securitycenter.v2.Application.getDefaultInstance()) {
getApplicationBuilder().mergeFrom(value);
} else {
application_ = value;
}
} else {
applicationBuilder_.mergeFrom(value);
}
if (application_ != null) {
bitField1_ |= 0x00000100;
onChanged();
}
return this;
}
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*/
public Builder clearApplication() {
bitField1_ = (bitField1_ & ~0x00000100);
application_ = null;
if (applicationBuilder_ != null) {
applicationBuilder_.dispose();
applicationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*/
public com.google.cloud.securitycenter.v2.Application.Builder getApplicationBuilder() {
bitField1_ |= 0x00000100;
onChanged();
return getApplicationFieldBuilder().getBuilder();
}
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*/
public com.google.cloud.securitycenter.v2.ApplicationOrBuilder getApplicationOrBuilder() {
if (applicationBuilder_ != null) {
return applicationBuilder_.getMessageOrBuilder();
} else {
return application_ == null
? com.google.cloud.securitycenter.v2.Application.getDefaultInstance()
: application_;
}
}
/**
*
*
*
* Represents an application associated with the finding.
*
*
* .google.cloud.securitycenter.v2.Application application = 45;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Application,
com.google.cloud.securitycenter.v2.Application.Builder,
com.google.cloud.securitycenter.v2.ApplicationOrBuilder>
getApplicationFieldBuilder() {
if (applicationBuilder_ == null) {
applicationBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.Application,
com.google.cloud.securitycenter.v2.Application.Builder,
com.google.cloud.securitycenter.v2.ApplicationOrBuilder>(
getApplication(), getParentForChildren(), isClean());
application_ = null;
}
return applicationBuilder_;
}
private com.google.cloud.securitycenter.v2.BackupDisasterRecovery backupDisasterRecovery_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.BackupDisasterRecovery,
com.google.cloud.securitycenter.v2.BackupDisasterRecovery.Builder,
com.google.cloud.securitycenter.v2.BackupDisasterRecoveryOrBuilder>
backupDisasterRecoveryBuilder_;
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*
* @return Whether the backupDisasterRecovery field is set.
*/
public boolean hasBackupDisasterRecovery() {
return ((bitField1_ & 0x00000200) != 0);
}
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*
* @return The backupDisasterRecovery.
*/
public com.google.cloud.securitycenter.v2.BackupDisasterRecovery getBackupDisasterRecovery() {
if (backupDisasterRecoveryBuilder_ == null) {
return backupDisasterRecovery_ == null
? com.google.cloud.securitycenter.v2.BackupDisasterRecovery.getDefaultInstance()
: backupDisasterRecovery_;
} else {
return backupDisasterRecoveryBuilder_.getMessage();
}
}
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*/
public Builder setBackupDisasterRecovery(
com.google.cloud.securitycenter.v2.BackupDisasterRecovery value) {
if (backupDisasterRecoveryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
backupDisasterRecovery_ = value;
} else {
backupDisasterRecoveryBuilder_.setMessage(value);
}
bitField1_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*/
public Builder setBackupDisasterRecovery(
com.google.cloud.securitycenter.v2.BackupDisasterRecovery.Builder builderForValue) {
if (backupDisasterRecoveryBuilder_ == null) {
backupDisasterRecovery_ = builderForValue.build();
} else {
backupDisasterRecoveryBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000200;
onChanged();
return this;
}
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*/
public Builder mergeBackupDisasterRecovery(
com.google.cloud.securitycenter.v2.BackupDisasterRecovery value) {
if (backupDisasterRecoveryBuilder_ == null) {
if (((bitField1_ & 0x00000200) != 0)
&& backupDisasterRecovery_ != null
&& backupDisasterRecovery_
!= com.google.cloud.securitycenter.v2.BackupDisasterRecovery.getDefaultInstance()) {
getBackupDisasterRecoveryBuilder().mergeFrom(value);
} else {
backupDisasterRecovery_ = value;
}
} else {
backupDisasterRecoveryBuilder_.mergeFrom(value);
}
if (backupDisasterRecovery_ != null) {
bitField1_ |= 0x00000200;
onChanged();
}
return this;
}
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*/
public Builder clearBackupDisasterRecovery() {
bitField1_ = (bitField1_ & ~0x00000200);
backupDisasterRecovery_ = null;
if (backupDisasterRecoveryBuilder_ != null) {
backupDisasterRecoveryBuilder_.dispose();
backupDisasterRecoveryBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*/
public com.google.cloud.securitycenter.v2.BackupDisasterRecovery.Builder
getBackupDisasterRecoveryBuilder() {
bitField1_ |= 0x00000200;
onChanged();
return getBackupDisasterRecoveryFieldBuilder().getBuilder();
}
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*/
public com.google.cloud.securitycenter.v2.BackupDisasterRecoveryOrBuilder
getBackupDisasterRecoveryOrBuilder() {
if (backupDisasterRecoveryBuilder_ != null) {
return backupDisasterRecoveryBuilder_.getMessageOrBuilder();
} else {
return backupDisasterRecovery_ == null
? com.google.cloud.securitycenter.v2.BackupDisasterRecovery.getDefaultInstance()
: backupDisasterRecovery_;
}
}
/**
*
*
*
* Fields related to Backup and DR findings.
*
*
* .google.cloud.securitycenter.v2.BackupDisasterRecovery backup_disaster_recovery = 47;
*
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.BackupDisasterRecovery,
com.google.cloud.securitycenter.v2.BackupDisasterRecovery.Builder,
com.google.cloud.securitycenter.v2.BackupDisasterRecoveryOrBuilder>
getBackupDisasterRecoveryFieldBuilder() {
if (backupDisasterRecoveryBuilder_ == null) {
backupDisasterRecoveryBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.BackupDisasterRecovery,
com.google.cloud.securitycenter.v2.BackupDisasterRecovery.Builder,
com.google.cloud.securitycenter.v2.BackupDisasterRecoveryOrBuilder>(
getBackupDisasterRecovery(), getParentForChildren(), isClean());
backupDisasterRecovery_ = null;
}
return backupDisasterRecoveryBuilder_;
}
private com.google.cloud.securitycenter.v2.SecurityPosture securityPosture_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.SecurityPosture,
com.google.cloud.securitycenter.v2.SecurityPosture.Builder,
com.google.cloud.securitycenter.v2.SecurityPostureOrBuilder>
securityPostureBuilder_;
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*
* @return Whether the securityPosture field is set.
*/
public boolean hasSecurityPosture() {
return ((bitField1_ & 0x00000400) != 0);
}
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*
* @return The securityPosture.
*/
public com.google.cloud.securitycenter.v2.SecurityPosture getSecurityPosture() {
if (securityPostureBuilder_ == null) {
return securityPosture_ == null
? com.google.cloud.securitycenter.v2.SecurityPosture.getDefaultInstance()
: securityPosture_;
} else {
return securityPostureBuilder_.getMessage();
}
}
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*/
public Builder setSecurityPosture(com.google.cloud.securitycenter.v2.SecurityPosture value) {
if (securityPostureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
securityPosture_ = value;
} else {
securityPostureBuilder_.setMessage(value);
}
bitField1_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*/
public Builder setSecurityPosture(
com.google.cloud.securitycenter.v2.SecurityPosture.Builder builderForValue) {
if (securityPostureBuilder_ == null) {
securityPosture_ = builderForValue.build();
} else {
securityPostureBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000400;
onChanged();
return this;
}
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*/
public Builder mergeSecurityPosture(com.google.cloud.securitycenter.v2.SecurityPosture value) {
if (securityPostureBuilder_ == null) {
if (((bitField1_ & 0x00000400) != 0)
&& securityPosture_ != null
&& securityPosture_
!= com.google.cloud.securitycenter.v2.SecurityPosture.getDefaultInstance()) {
getSecurityPostureBuilder().mergeFrom(value);
} else {
securityPosture_ = value;
}
} else {
securityPostureBuilder_.mergeFrom(value);
}
if (securityPosture_ != null) {
bitField1_ |= 0x00000400;
onChanged();
}
return this;
}
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*/
public Builder clearSecurityPosture() {
bitField1_ = (bitField1_ & ~0x00000400);
securityPosture_ = null;
if (securityPostureBuilder_ != null) {
securityPostureBuilder_.dispose();
securityPostureBuilder_ = null;
}
onChanged();
return this;
}
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*/
public com.google.cloud.securitycenter.v2.SecurityPosture.Builder getSecurityPostureBuilder() {
bitField1_ |= 0x00000400;
onChanged();
return getSecurityPostureFieldBuilder().getBuilder();
}
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*/
public com.google.cloud.securitycenter.v2.SecurityPostureOrBuilder
getSecurityPostureOrBuilder() {
if (securityPostureBuilder_ != null) {
return securityPostureBuilder_.getMessageOrBuilder();
} else {
return securityPosture_ == null
? com.google.cloud.securitycenter.v2.SecurityPosture.getDefaultInstance()
: securityPosture_;
}
}
/**
*
*
*
* The security posture associated with the finding.
*
*
* .google.cloud.securitycenter.v2.SecurityPosture security_posture = 48;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.SecurityPosture,
com.google.cloud.securitycenter.v2.SecurityPosture.Builder,
com.google.cloud.securitycenter.v2.SecurityPostureOrBuilder>
getSecurityPostureFieldBuilder() {
if (securityPostureBuilder_ == null) {
securityPostureBuilder_ =
new com.google.protobuf.SingleFieldBuilderV3<
com.google.cloud.securitycenter.v2.SecurityPosture,
com.google.cloud.securitycenter.v2.SecurityPosture.Builder,
com.google.cloud.securitycenter.v2.SecurityPostureOrBuilder>(
getSecurityPosture(), getParentForChildren(), isClean());
securityPosture_ = null;
}
return securityPostureBuilder_;
}
private java.util.List logEntries_ =
java.util.Collections.emptyList();
private void ensureLogEntriesIsMutable() {
if (!((bitField1_ & 0x00000800) != 0)) {
logEntries_ =
new java.util.ArrayList(logEntries_);
bitField1_ |= 0x00000800;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.LogEntry,
com.google.cloud.securitycenter.v2.LogEntry.Builder,
com.google.cloud.securitycenter.v2.LogEntryOrBuilder>
logEntriesBuilder_;
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public java.util.List getLogEntriesList() {
if (logEntriesBuilder_ == null) {
return java.util.Collections.unmodifiableList(logEntries_);
} else {
return logEntriesBuilder_.getMessageList();
}
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public int getLogEntriesCount() {
if (logEntriesBuilder_ == null) {
return logEntries_.size();
} else {
return logEntriesBuilder_.getCount();
}
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public com.google.cloud.securitycenter.v2.LogEntry getLogEntries(int index) {
if (logEntriesBuilder_ == null) {
return logEntries_.get(index);
} else {
return logEntriesBuilder_.getMessage(index);
}
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public Builder setLogEntries(int index, com.google.cloud.securitycenter.v2.LogEntry value) {
if (logEntriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogEntriesIsMutable();
logEntries_.set(index, value);
onChanged();
} else {
logEntriesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public Builder setLogEntries(
int index, com.google.cloud.securitycenter.v2.LogEntry.Builder builderForValue) {
if (logEntriesBuilder_ == null) {
ensureLogEntriesIsMutable();
logEntries_.set(index, builderForValue.build());
onChanged();
} else {
logEntriesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public Builder addLogEntries(com.google.cloud.securitycenter.v2.LogEntry value) {
if (logEntriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogEntriesIsMutable();
logEntries_.add(value);
onChanged();
} else {
logEntriesBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public Builder addLogEntries(int index, com.google.cloud.securitycenter.v2.LogEntry value) {
if (logEntriesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogEntriesIsMutable();
logEntries_.add(index, value);
onChanged();
} else {
logEntriesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public Builder addLogEntries(
com.google.cloud.securitycenter.v2.LogEntry.Builder builderForValue) {
if (logEntriesBuilder_ == null) {
ensureLogEntriesIsMutable();
logEntries_.add(builderForValue.build());
onChanged();
} else {
logEntriesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public Builder addLogEntries(
int index, com.google.cloud.securitycenter.v2.LogEntry.Builder builderForValue) {
if (logEntriesBuilder_ == null) {
ensureLogEntriesIsMutable();
logEntries_.add(index, builderForValue.build());
onChanged();
} else {
logEntriesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public Builder addAllLogEntries(
java.lang.Iterable extends com.google.cloud.securitycenter.v2.LogEntry> values) {
if (logEntriesBuilder_ == null) {
ensureLogEntriesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, logEntries_);
onChanged();
} else {
logEntriesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public Builder clearLogEntries() {
if (logEntriesBuilder_ == null) {
logEntries_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000800);
onChanged();
} else {
logEntriesBuilder_.clear();
}
return this;
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public Builder removeLogEntries(int index) {
if (logEntriesBuilder_ == null) {
ensureLogEntriesIsMutable();
logEntries_.remove(index);
onChanged();
} else {
logEntriesBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public com.google.cloud.securitycenter.v2.LogEntry.Builder getLogEntriesBuilder(int index) {
return getLogEntriesFieldBuilder().getBuilder(index);
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public com.google.cloud.securitycenter.v2.LogEntryOrBuilder getLogEntriesOrBuilder(int index) {
if (logEntriesBuilder_ == null) {
return logEntries_.get(index);
} else {
return logEntriesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public java.util.List extends com.google.cloud.securitycenter.v2.LogEntryOrBuilder>
getLogEntriesOrBuilderList() {
if (logEntriesBuilder_ != null) {
return logEntriesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(logEntries_);
}
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public com.google.cloud.securitycenter.v2.LogEntry.Builder addLogEntriesBuilder() {
return getLogEntriesFieldBuilder()
.addBuilder(com.google.cloud.securitycenter.v2.LogEntry.getDefaultInstance());
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public com.google.cloud.securitycenter.v2.LogEntry.Builder addLogEntriesBuilder(int index) {
return getLogEntriesFieldBuilder()
.addBuilder(index, com.google.cloud.securitycenter.v2.LogEntry.getDefaultInstance());
}
/**
*
*
*
* Log entries that are relevant to the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LogEntry log_entries = 49;
*/
public java.util.List
getLogEntriesBuilderList() {
return getLogEntriesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.LogEntry,
com.google.cloud.securitycenter.v2.LogEntry.Builder,
com.google.cloud.securitycenter.v2.LogEntryOrBuilder>
getLogEntriesFieldBuilder() {
if (logEntriesBuilder_ == null) {
logEntriesBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.LogEntry,
com.google.cloud.securitycenter.v2.LogEntry.Builder,
com.google.cloud.securitycenter.v2.LogEntryOrBuilder>(
logEntries_, ((bitField1_ & 0x00000800) != 0), getParentForChildren(), isClean());
logEntries_ = null;
}
return logEntriesBuilder_;
}
private java.util.List loadBalancers_ =
java.util.Collections.emptyList();
private void ensureLoadBalancersIsMutable() {
if (!((bitField1_ & 0x00001000) != 0)) {
loadBalancers_ =
new java.util.ArrayList(
loadBalancers_);
bitField1_ |= 0x00001000;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.LoadBalancer,
com.google.cloud.securitycenter.v2.LoadBalancer.Builder,
com.google.cloud.securitycenter.v2.LoadBalancerOrBuilder>
loadBalancersBuilder_;
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public java.util.List getLoadBalancersList() {
if (loadBalancersBuilder_ == null) {
return java.util.Collections.unmodifiableList(loadBalancers_);
} else {
return loadBalancersBuilder_.getMessageList();
}
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public int getLoadBalancersCount() {
if (loadBalancersBuilder_ == null) {
return loadBalancers_.size();
} else {
return loadBalancersBuilder_.getCount();
}
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public com.google.cloud.securitycenter.v2.LoadBalancer getLoadBalancers(int index) {
if (loadBalancersBuilder_ == null) {
return loadBalancers_.get(index);
} else {
return loadBalancersBuilder_.getMessage(index);
}
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public Builder setLoadBalancers(
int index, com.google.cloud.securitycenter.v2.LoadBalancer value) {
if (loadBalancersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLoadBalancersIsMutable();
loadBalancers_.set(index, value);
onChanged();
} else {
loadBalancersBuilder_.setMessage(index, value);
}
return this;
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public Builder setLoadBalancers(
int index, com.google.cloud.securitycenter.v2.LoadBalancer.Builder builderForValue) {
if (loadBalancersBuilder_ == null) {
ensureLoadBalancersIsMutable();
loadBalancers_.set(index, builderForValue.build());
onChanged();
} else {
loadBalancersBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public Builder addLoadBalancers(com.google.cloud.securitycenter.v2.LoadBalancer value) {
if (loadBalancersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLoadBalancersIsMutable();
loadBalancers_.add(value);
onChanged();
} else {
loadBalancersBuilder_.addMessage(value);
}
return this;
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public Builder addLoadBalancers(
int index, com.google.cloud.securitycenter.v2.LoadBalancer value) {
if (loadBalancersBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLoadBalancersIsMutable();
loadBalancers_.add(index, value);
onChanged();
} else {
loadBalancersBuilder_.addMessage(index, value);
}
return this;
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public Builder addLoadBalancers(
com.google.cloud.securitycenter.v2.LoadBalancer.Builder builderForValue) {
if (loadBalancersBuilder_ == null) {
ensureLoadBalancersIsMutable();
loadBalancers_.add(builderForValue.build());
onChanged();
} else {
loadBalancersBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public Builder addLoadBalancers(
int index, com.google.cloud.securitycenter.v2.LoadBalancer.Builder builderForValue) {
if (loadBalancersBuilder_ == null) {
ensureLoadBalancersIsMutable();
loadBalancers_.add(index, builderForValue.build());
onChanged();
} else {
loadBalancersBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public Builder addAllLoadBalancers(
java.lang.Iterable extends com.google.cloud.securitycenter.v2.LoadBalancer> values) {
if (loadBalancersBuilder_ == null) {
ensureLoadBalancersIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(values, loadBalancers_);
onChanged();
} else {
loadBalancersBuilder_.addAllMessages(values);
}
return this;
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public Builder clearLoadBalancers() {
if (loadBalancersBuilder_ == null) {
loadBalancers_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00001000);
onChanged();
} else {
loadBalancersBuilder_.clear();
}
return this;
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public Builder removeLoadBalancers(int index) {
if (loadBalancersBuilder_ == null) {
ensureLoadBalancersIsMutable();
loadBalancers_.remove(index);
onChanged();
} else {
loadBalancersBuilder_.remove(index);
}
return this;
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public com.google.cloud.securitycenter.v2.LoadBalancer.Builder getLoadBalancersBuilder(
int index) {
return getLoadBalancersFieldBuilder().getBuilder(index);
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public com.google.cloud.securitycenter.v2.LoadBalancerOrBuilder getLoadBalancersOrBuilder(
int index) {
if (loadBalancersBuilder_ == null) {
return loadBalancers_.get(index);
} else {
return loadBalancersBuilder_.getMessageOrBuilder(index);
}
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public java.util.List extends com.google.cloud.securitycenter.v2.LoadBalancerOrBuilder>
getLoadBalancersOrBuilderList() {
if (loadBalancersBuilder_ != null) {
return loadBalancersBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(loadBalancers_);
}
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public com.google.cloud.securitycenter.v2.LoadBalancer.Builder addLoadBalancersBuilder() {
return getLoadBalancersFieldBuilder()
.addBuilder(com.google.cloud.securitycenter.v2.LoadBalancer.getDefaultInstance());
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public com.google.cloud.securitycenter.v2.LoadBalancer.Builder addLoadBalancersBuilder(
int index) {
return getLoadBalancersFieldBuilder()
.addBuilder(index, com.google.cloud.securitycenter.v2.LoadBalancer.getDefaultInstance());
}
/**
*
*
*
* The load balancers associated with the finding.
*
*
* repeated .google.cloud.securitycenter.v2.LoadBalancer load_balancers = 50;
*/
public java.util.List
getLoadBalancersBuilderList() {
return getLoadBalancersFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.LoadBalancer,
com.google.cloud.securitycenter.v2.LoadBalancer.Builder,
com.google.cloud.securitycenter.v2.LoadBalancerOrBuilder>
getLoadBalancersFieldBuilder() {
if (loadBalancersBuilder_ == null) {
loadBalancersBuilder_ =
new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.cloud.securitycenter.v2.LoadBalancer,
com.google.cloud.securitycenter.v2.LoadBalancer.Builder,
com.google.cloud.securitycenter.v2.LoadBalancerOrBuilder>(
loadBalancers_,
((bitField1_ & 0x00001000) != 0),
getParentForChildren(),
isClean());
loadBalancers_ = null;
}
return loadBalancersBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.cloud.securitycenter.v2.Finding)
}
// @@protoc_insertion_point(class_scope:google.cloud.securitycenter.v2.Finding)
private static final com.google.cloud.securitycenter.v2.Finding DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.cloud.securitycenter.v2.Finding();
}
public static com.google.cloud.securitycenter.v2.Finding getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Finding parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.cloud.securitycenter.v2.Finding getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy