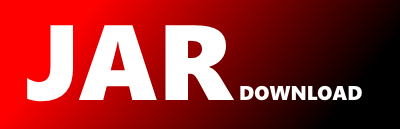
com.google.api.servicecontrol.v1.QuotaOperationOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-service-control-v1 Show documentation
Show all versions of proto-google-cloud-service-control-v1 Show documentation
Proto library for google-cloud-service-control
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/api/servicecontrol/v1/quota_controller.proto
// Protobuf Java Version: 3.25.5
package com.google.api.servicecontrol.v1;
public interface QuotaOperationOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.api.servicecontrol.v1.QuotaOperation)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Identity of the operation. This is expected to be unique within the scope
* of the service that generated the operation, and guarantees idempotency in
* case of retries.
*
* In order to ensure best performance and latency in the Quota backends,
* operation_ids are optimally associated with time, so that related
* operations can be accessed fast in storage. For this reason, the
* recommended token for services that intend to operate at a high QPS is
* Unix time in nanos + UUID
*
*
* string operation_id = 1;
*
* @return The operationId.
*/
java.lang.String getOperationId();
/**
*
*
*
* Identity of the operation. This is expected to be unique within the scope
* of the service that generated the operation, and guarantees idempotency in
* case of retries.
*
* In order to ensure best performance and latency in the Quota backends,
* operation_ids are optimally associated with time, so that related
* operations can be accessed fast in storage. For this reason, the
* recommended token for services that intend to operate at a high QPS is
* Unix time in nanos + UUID
*
*
* string operation_id = 1;
*
* @return The bytes for operationId.
*/
com.google.protobuf.ByteString getOperationIdBytes();
/**
*
*
*
* Fully qualified name of the API method for which this quota operation is
* requested. This name is used for matching quota rules or metric rules and
* billing status rules defined in service configuration.
*
* This field should not be set if any of the following is true:
* (1) the quota operation is performed on non-API resources.
* (2) quota_metrics is set because the caller is doing quota override.
*
*
* Example of an RPC method name:
* google.example.library.v1.LibraryService.CreateShelf
*
*
* string method_name = 2;
*
* @return The methodName.
*/
java.lang.String getMethodName();
/**
*
*
*
* Fully qualified name of the API method for which this quota operation is
* requested. This name is used for matching quota rules or metric rules and
* billing status rules defined in service configuration.
*
* This field should not be set if any of the following is true:
* (1) the quota operation is performed on non-API resources.
* (2) quota_metrics is set because the caller is doing quota override.
*
*
* Example of an RPC method name:
* google.example.library.v1.LibraryService.CreateShelf
*
*
* string method_name = 2;
*
* @return The bytes for methodName.
*/
com.google.protobuf.ByteString getMethodNameBytes();
/**
*
*
*
* Identity of the consumer for whom this quota operation is being performed.
*
* This can be in one of the following formats:
* project:<project_id>,
* project_number:<project_number>,
* api_key:<api_key>.
*
*
* string consumer_id = 3;
*
* @return The consumerId.
*/
java.lang.String getConsumerId();
/**
*
*
*
* Identity of the consumer for whom this quota operation is being performed.
*
* This can be in one of the following formats:
* project:<project_id>,
* project_number:<project_number>,
* api_key:<api_key>.
*
*
* string consumer_id = 3;
*
* @return The bytes for consumerId.
*/
com.google.protobuf.ByteString getConsumerIdBytes();
/**
*
*
*
* Labels describing the operation.
*
*
* map<string, string> labels = 4;
*/
int getLabelsCount();
/**
*
*
*
* Labels describing the operation.
*
*
* map<string, string> labels = 4;
*/
boolean containsLabels(java.lang.String key);
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Deprecated
java.util.Map getLabels();
/**
*
*
*
* Labels describing the operation.
*
*
* map<string, string> labels = 4;
*/
java.util.Map getLabelsMap();
/**
*
*
*
* Labels describing the operation.
*
*
* map<string, string> labels = 4;
*/
/* nullable */
java.lang.String getLabelsOrDefault(
java.lang.String key,
/* nullable */
java.lang.String defaultValue);
/**
*
*
*
* Labels describing the operation.
*
*
* map<string, string> labels = 4;
*/
java.lang.String getLabelsOrThrow(java.lang.String key);
/**
*
*
*
* Represents information about this operation. Each MetricValueSet
* corresponds to a metric defined in the service configuration.
* The data type used in the MetricValueSet must agree with
* the data type specified in the metric definition.
*
* Within a single operation, it is not allowed to have more than one
* MetricValue instances that have the same metric names and identical
* label value combinations. If a request has such duplicated MetricValue
* instances, the entire request is rejected with
* an invalid argument error.
*
* This field is mutually exclusive with method_name.
*
*
* repeated .google.api.servicecontrol.v1.MetricValueSet quota_metrics = 5;
*/
java.util.List getQuotaMetricsList();
/**
*
*
*
* Represents information about this operation. Each MetricValueSet
* corresponds to a metric defined in the service configuration.
* The data type used in the MetricValueSet must agree with
* the data type specified in the metric definition.
*
* Within a single operation, it is not allowed to have more than one
* MetricValue instances that have the same metric names and identical
* label value combinations. If a request has such duplicated MetricValue
* instances, the entire request is rejected with
* an invalid argument error.
*
* This field is mutually exclusive with method_name.
*
*
* repeated .google.api.servicecontrol.v1.MetricValueSet quota_metrics = 5;
*/
com.google.api.servicecontrol.v1.MetricValueSet getQuotaMetrics(int index);
/**
*
*
*
* Represents information about this operation. Each MetricValueSet
* corresponds to a metric defined in the service configuration.
* The data type used in the MetricValueSet must agree with
* the data type specified in the metric definition.
*
* Within a single operation, it is not allowed to have more than one
* MetricValue instances that have the same metric names and identical
* label value combinations. If a request has such duplicated MetricValue
* instances, the entire request is rejected with
* an invalid argument error.
*
* This field is mutually exclusive with method_name.
*
*
* repeated .google.api.servicecontrol.v1.MetricValueSet quota_metrics = 5;
*/
int getQuotaMetricsCount();
/**
*
*
*
* Represents information about this operation. Each MetricValueSet
* corresponds to a metric defined in the service configuration.
* The data type used in the MetricValueSet must agree with
* the data type specified in the metric definition.
*
* Within a single operation, it is not allowed to have more than one
* MetricValue instances that have the same metric names and identical
* label value combinations. If a request has such duplicated MetricValue
* instances, the entire request is rejected with
* an invalid argument error.
*
* This field is mutually exclusive with method_name.
*
*
* repeated .google.api.servicecontrol.v1.MetricValueSet quota_metrics = 5;
*/
java.util.List extends com.google.api.servicecontrol.v1.MetricValueSetOrBuilder>
getQuotaMetricsOrBuilderList();
/**
*
*
*
* Represents information about this operation. Each MetricValueSet
* corresponds to a metric defined in the service configuration.
* The data type used in the MetricValueSet must agree with
* the data type specified in the metric definition.
*
* Within a single operation, it is not allowed to have more than one
* MetricValue instances that have the same metric names and identical
* label value combinations. If a request has such duplicated MetricValue
* instances, the entire request is rejected with
* an invalid argument error.
*
* This field is mutually exclusive with method_name.
*
*
* repeated .google.api.servicecontrol.v1.MetricValueSet quota_metrics = 5;
*/
com.google.api.servicecontrol.v1.MetricValueSetOrBuilder getQuotaMetricsOrBuilder(int index);
/**
*
*
*
* Quota mode for this operation.
*
*
* .google.api.servicecontrol.v1.QuotaOperation.QuotaMode quota_mode = 6;
*
* @return The enum numeric value on the wire for quotaMode.
*/
int getQuotaModeValue();
/**
*
*
*
* Quota mode for this operation.
*
*
* .google.api.servicecontrol.v1.QuotaOperation.QuotaMode quota_mode = 6;
*
* @return The quotaMode.
*/
com.google.api.servicecontrol.v1.QuotaOperation.QuotaMode getQuotaMode();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy