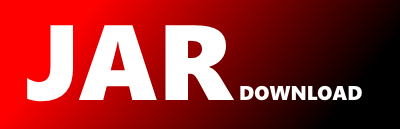
com.google.storage.v2.BucketOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto-google-cloud-storage-v2 Show documentation
Show all versions of proto-google-cloud-storage-v2 Show documentation
PROTO library for proto-google-cloud-storage-v2
/*
* Copyright 2020 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/storage/v2/storage.proto
package com.google.storage.v2;
public interface BucketOrBuilder
extends
// @@protoc_insertion_point(interface_extends:google.storage.v2.Bucket)
com.google.protobuf.MessageOrBuilder {
/**
*
*
*
* Immutable. The name of the bucket.
* Global buckets will be of the format `projects/{project}/buckets/{bucket}`.
* Other sorts of buckets in the future are not guaranteed to follow this
* pattern.
* For globally unique bucket names, a `_` may be substituted for the project
* ID.
*
*
* string name = 1 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The name.
*/
java.lang.String getName();
/**
*
*
*
* Immutable. The name of the bucket.
* Global buckets will be of the format `projects/{project}/buckets/{bucket}`.
* Other sorts of buckets in the future are not guaranteed to follow this
* pattern.
* For globally unique bucket names, a `_` may be substituted for the project
* ID.
*
*
* string name = 1 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The bytes for name.
*/
com.google.protobuf.ByteString getNameBytes();
/**
*
*
*
* Output only. The user-chosen part of the bucket name. The `{bucket}` portion of the
* `name` field. For globally unique buckets, this is equal to the "bucket
* name" of other Cloud Storage APIs. Example: "pub".
*
*
* string bucket_id = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bucketId.
*/
java.lang.String getBucketId();
/**
*
*
*
* Output only. The user-chosen part of the bucket name. The `{bucket}` portion of the
* `name` field. For globally unique buckets, this is equal to the "bucket
* name" of other Cloud Storage APIs. Example: "pub".
*
*
* string bucket_id = 2 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for bucketId.
*/
com.google.protobuf.ByteString getBucketIdBytes();
/**
*
*
*
* Immutable. The project which owns this bucket.
* Format: projects/{project_number}
* Example: `projects/123456`.
*
*
*
* string project = 3 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
*
*
* @return The project.
*/
java.lang.String getProject();
/**
*
*
*
* Immutable. The project which owns this bucket.
* Format: projects/{project_number}
* Example: `projects/123456`.
*
*
*
* string project = 3 [(.google.api.field_behavior) = IMMUTABLE, (.google.api.resource_reference) = { ... }
*
*
* @return The bytes for project.
*/
com.google.protobuf.ByteString getProjectBytes();
/**
*
*
*
* Output only. The metadata generation of this bucket.
* Attempting to set or update this field will result in a
* [FieldViolation][google.rpc.BadRequest.FieldViolation].
*
*
* int64 metageneration = 4 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The metageneration.
*/
long getMetageneration();
/**
*
*
*
* Immutable. The location of the bucket. Object data for objects in the bucket resides
* in physical storage within this region. Defaults to `US`. See the
* [https://developers.google.com/storage/docs/concepts-techniques#specifyinglocations"][developer's
* guide] for the authoritative list. Attempting to update this field after
* the bucket is created will result in an error.
*
*
* string location = 5 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The location.
*/
java.lang.String getLocation();
/**
*
*
*
* Immutable. The location of the bucket. Object data for objects in the bucket resides
* in physical storage within this region. Defaults to `US`. See the
* [https://developers.google.com/storage/docs/concepts-techniques#specifyinglocations"][developer's
* guide] for the authoritative list. Attempting to update this field after
* the bucket is created will result in an error.
*
*
* string location = 5 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The bytes for location.
*/
com.google.protobuf.ByteString getLocationBytes();
/**
*
*
*
* Output only. The location type of the bucket (region, dual-region, multi-region, etc).
*
*
* string location_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The locationType.
*/
java.lang.String getLocationType();
/**
*
*
*
* Output only. The location type of the bucket (region, dual-region, multi-region, etc).
*
*
* string location_type = 6 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The bytes for locationType.
*/
com.google.protobuf.ByteString getLocationTypeBytes();
/**
*
*
*
* The bucket's default storage class, used whenever no storageClass is
* specified for a newly-created object. This defines how objects in the
* bucket are stored and determines the SLA and the cost of storage.
* If this value is not specified when the bucket is created, it will default
* to `STANDARD`. For more information, see
* https://developers.google.com/storage/docs/storage-classes.
*
*
* string storage_class = 7;
*
* @return The storageClass.
*/
java.lang.String getStorageClass();
/**
*
*
*
* The bucket's default storage class, used whenever no storageClass is
* specified for a newly-created object. This defines how objects in the
* bucket are stored and determines the SLA and the cost of storage.
* If this value is not specified when the bucket is created, it will default
* to `STANDARD`. For more information, see
* https://developers.google.com/storage/docs/storage-classes.
*
*
* string storage_class = 7;
*
* @return The bytes for storageClass.
*/
com.google.protobuf.ByteString getStorageClassBytes();
/**
*
*
*
* Access controls on the bucket.
* If iamConfig.uniformBucketLevelAccess is enabled on this bucket,
* requests to set, read, or modify acl is an error.
*
*
* repeated .google.storage.v2.BucketAccessControl acl = 8;
*/
java.util.List getAclList();
/**
*
*
*
* Access controls on the bucket.
* If iamConfig.uniformBucketLevelAccess is enabled on this bucket,
* requests to set, read, or modify acl is an error.
*
*
* repeated .google.storage.v2.BucketAccessControl acl = 8;
*/
com.google.storage.v2.BucketAccessControl getAcl(int index);
/**
*
*
*
* Access controls on the bucket.
* If iamConfig.uniformBucketLevelAccess is enabled on this bucket,
* requests to set, read, or modify acl is an error.
*
*
* repeated .google.storage.v2.BucketAccessControl acl = 8;
*/
int getAclCount();
/**
*
*
*
* Access controls on the bucket.
* If iamConfig.uniformBucketLevelAccess is enabled on this bucket,
* requests to set, read, or modify acl is an error.
*
*
* repeated .google.storage.v2.BucketAccessControl acl = 8;
*/
java.util.List extends com.google.storage.v2.BucketAccessControlOrBuilder>
getAclOrBuilderList();
/**
*
*
*
* Access controls on the bucket.
* If iamConfig.uniformBucketLevelAccess is enabled on this bucket,
* requests to set, read, or modify acl is an error.
*
*
* repeated .google.storage.v2.BucketAccessControl acl = 8;
*/
com.google.storage.v2.BucketAccessControlOrBuilder getAclOrBuilder(int index);
/**
*
*
*
* Default access controls to apply to new objects when no ACL is provided.
* If iamConfig.uniformBucketLevelAccess is enabled on this bucket,
* requests to set, read, or modify acl is an error.
*
*
* repeated .google.storage.v2.ObjectAccessControl default_object_acl = 9;
*/
java.util.List getDefaultObjectAclList();
/**
*
*
*
* Default access controls to apply to new objects when no ACL is provided.
* If iamConfig.uniformBucketLevelAccess is enabled on this bucket,
* requests to set, read, or modify acl is an error.
*
*
* repeated .google.storage.v2.ObjectAccessControl default_object_acl = 9;
*/
com.google.storage.v2.ObjectAccessControl getDefaultObjectAcl(int index);
/**
*
*
*
* Default access controls to apply to new objects when no ACL is provided.
* If iamConfig.uniformBucketLevelAccess is enabled on this bucket,
* requests to set, read, or modify acl is an error.
*
*
* repeated .google.storage.v2.ObjectAccessControl default_object_acl = 9;
*/
int getDefaultObjectAclCount();
/**
*
*
*
* Default access controls to apply to new objects when no ACL is provided.
* If iamConfig.uniformBucketLevelAccess is enabled on this bucket,
* requests to set, read, or modify acl is an error.
*
*
* repeated .google.storage.v2.ObjectAccessControl default_object_acl = 9;
*/
java.util.List extends com.google.storage.v2.ObjectAccessControlOrBuilder>
getDefaultObjectAclOrBuilderList();
/**
*
*
*
* Default access controls to apply to new objects when no ACL is provided.
* If iamConfig.uniformBucketLevelAccess is enabled on this bucket,
* requests to set, read, or modify acl is an error.
*
*
* repeated .google.storage.v2.ObjectAccessControl default_object_acl = 9;
*/
com.google.storage.v2.ObjectAccessControlOrBuilder getDefaultObjectAclOrBuilder(int index);
/**
*
*
*
* The bucket's lifecycle config. See
* [https://developers.google.com/storage/docs/lifecycle]Lifecycle Management]
* for more information.
*
*
* .google.storage.v2.Bucket.Lifecycle lifecycle = 10;
*
* @return Whether the lifecycle field is set.
*/
boolean hasLifecycle();
/**
*
*
*
* The bucket's lifecycle config. See
* [https://developers.google.com/storage/docs/lifecycle]Lifecycle Management]
* for more information.
*
*
* .google.storage.v2.Bucket.Lifecycle lifecycle = 10;
*
* @return The lifecycle.
*/
com.google.storage.v2.Bucket.Lifecycle getLifecycle();
/**
*
*
*
* The bucket's lifecycle config. See
* [https://developers.google.com/storage/docs/lifecycle]Lifecycle Management]
* for more information.
*
*
* .google.storage.v2.Bucket.Lifecycle lifecycle = 10;
*/
com.google.storage.v2.Bucket.LifecycleOrBuilder getLifecycleOrBuilder();
/**
*
*
*
* Output only. The creation time of the bucket in
* [https://tools.ietf.org/html/rfc3339][RFC 3339] format.
* Attempting to set or update this field will result in a
* [FieldViolation][google.rpc.BadRequest.FieldViolation].
*
*
* .google.protobuf.Timestamp create_time = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the createTime field is set.
*/
boolean hasCreateTime();
/**
*
*
*
* Output only. The creation time of the bucket in
* [https://tools.ietf.org/html/rfc3339][RFC 3339] format.
* Attempting to set or update this field will result in a
* [FieldViolation][google.rpc.BadRequest.FieldViolation].
*
*
* .google.protobuf.Timestamp create_time = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The createTime.
*/
com.google.protobuf.Timestamp getCreateTime();
/**
*
*
*
* Output only. The creation time of the bucket in
* [https://tools.ietf.org/html/rfc3339][RFC 3339] format.
* Attempting to set or update this field will result in a
* [FieldViolation][google.rpc.BadRequest.FieldViolation].
*
*
* .google.protobuf.Timestamp create_time = 11 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.protobuf.TimestampOrBuilder getCreateTimeOrBuilder();
/**
*
*
*
* The bucket's [https://www.w3.org/TR/cors/][Cross-Origin Resource Sharing]
* (CORS) config.
*
*
* repeated .google.storage.v2.Bucket.Cors cors = 12;
*/
java.util.List getCorsList();
/**
*
*
*
* The bucket's [https://www.w3.org/TR/cors/][Cross-Origin Resource Sharing]
* (CORS) config.
*
*
* repeated .google.storage.v2.Bucket.Cors cors = 12;
*/
com.google.storage.v2.Bucket.Cors getCors(int index);
/**
*
*
*
* The bucket's [https://www.w3.org/TR/cors/][Cross-Origin Resource Sharing]
* (CORS) config.
*
*
* repeated .google.storage.v2.Bucket.Cors cors = 12;
*/
int getCorsCount();
/**
*
*
*
* The bucket's [https://www.w3.org/TR/cors/][Cross-Origin Resource Sharing]
* (CORS) config.
*
*
* repeated .google.storage.v2.Bucket.Cors cors = 12;
*/
java.util.List extends com.google.storage.v2.Bucket.CorsOrBuilder> getCorsOrBuilderList();
/**
*
*
*
* The bucket's [https://www.w3.org/TR/cors/][Cross-Origin Resource Sharing]
* (CORS) config.
*
*
* repeated .google.storage.v2.Bucket.Cors cors = 12;
*/
com.google.storage.v2.Bucket.CorsOrBuilder getCorsOrBuilder(int index);
/**
*
*
*
* Output only. The modification time of the bucket.
* Attempting to set or update this field will result in a
* [FieldViolation][google.rpc.BadRequest.FieldViolation].
*
*
* .google.protobuf.Timestamp update_time = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return Whether the updateTime field is set.
*/
boolean hasUpdateTime();
/**
*
*
*
* Output only. The modification time of the bucket.
* Attempting to set or update this field will result in a
* [FieldViolation][google.rpc.BadRequest.FieldViolation].
*
*
* .google.protobuf.Timestamp update_time = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*
* @return The updateTime.
*/
com.google.protobuf.Timestamp getUpdateTime();
/**
*
*
*
* Output only. The modification time of the bucket.
* Attempting to set or update this field will result in a
* [FieldViolation][google.rpc.BadRequest.FieldViolation].
*
*
* .google.protobuf.Timestamp update_time = 13 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
*/
com.google.protobuf.TimestampOrBuilder getUpdateTimeOrBuilder();
/**
*
*
*
* The default value for event-based hold on newly created objects in this
* bucket. Event-based hold is a way to retain objects indefinitely until an
* event occurs, signified by the
* hold's release. After being released, such objects will be subject to
* bucket-level retention (if any). One sample use case of this flag is for
* banks to hold loan documents for at least 3 years after loan is paid in
* full. Here, bucket-level retention is 3 years and the event is loan being
* paid in full. In this example, these objects will be held intact for any
* number of years until the event has occurred (event-based hold on the
* object is released) and then 3 more years after that. That means retention
* duration of the objects begins from the moment event-based hold
* transitioned from true to false. Objects under event-based hold cannot be
* deleted, overwritten or archived until the hold is removed.
*
*
* bool default_event_based_hold = 14;
*
* @return The defaultEventBasedHold.
*/
boolean getDefaultEventBasedHold();
/**
*
*
*
* User-provided labels, in key/value pairs.
*
*
* map<string, string> labels = 15;
*/
int getLabelsCount();
/**
*
*
*
* User-provided labels, in key/value pairs.
*
*
* map<string, string> labels = 15;
*/
boolean containsLabels(java.lang.String key);
/** Use {@link #getLabelsMap()} instead. */
@java.lang.Deprecated
java.util.Map getLabels();
/**
*
*
*
* User-provided labels, in key/value pairs.
*
*
* map<string, string> labels = 15;
*/
java.util.Map getLabelsMap();
/**
*
*
*
* User-provided labels, in key/value pairs.
*
*
* map<string, string> labels = 15;
*/
java.lang.String getLabelsOrDefault(java.lang.String key, java.lang.String defaultValue);
/**
*
*
*
* User-provided labels, in key/value pairs.
*
*
* map<string, string> labels = 15;
*/
java.lang.String getLabelsOrThrow(java.lang.String key);
/**
*
*
*
* The bucket's website config, controlling how the service behaves
* when accessing bucket contents as a web site. See the
* [https://cloud.google.com/storage/docs/static-website][Static Website
* Examples] for more information.
*
*
* .google.storage.v2.Bucket.Website website = 16;
*
* @return Whether the website field is set.
*/
boolean hasWebsite();
/**
*
*
*
* The bucket's website config, controlling how the service behaves
* when accessing bucket contents as a web site. See the
* [https://cloud.google.com/storage/docs/static-website][Static Website
* Examples] for more information.
*
*
* .google.storage.v2.Bucket.Website website = 16;
*
* @return The website.
*/
com.google.storage.v2.Bucket.Website getWebsite();
/**
*
*
*
* The bucket's website config, controlling how the service behaves
* when accessing bucket contents as a web site. See the
* [https://cloud.google.com/storage/docs/static-website][Static Website
* Examples] for more information.
*
*
* .google.storage.v2.Bucket.Website website = 16;
*/
com.google.storage.v2.Bucket.WebsiteOrBuilder getWebsiteOrBuilder();
/**
*
*
*
* The bucket's versioning config.
*
*
* .google.storage.v2.Bucket.Versioning versioning = 17;
*
* @return Whether the versioning field is set.
*/
boolean hasVersioning();
/**
*
*
*
* The bucket's versioning config.
*
*
* .google.storage.v2.Bucket.Versioning versioning = 17;
*
* @return The versioning.
*/
com.google.storage.v2.Bucket.Versioning getVersioning();
/**
*
*
*
* The bucket's versioning config.
*
*
* .google.storage.v2.Bucket.Versioning versioning = 17;
*/
com.google.storage.v2.Bucket.VersioningOrBuilder getVersioningOrBuilder();
/**
*
*
*
* The bucket's logging config, which defines the destination bucket
* and name prefix (if any) for the current bucket's logs.
*
*
* .google.storage.v2.Bucket.Logging logging = 18;
*
* @return Whether the logging field is set.
*/
boolean hasLogging();
/**
*
*
*
* The bucket's logging config, which defines the destination bucket
* and name prefix (if any) for the current bucket's logs.
*
*
* .google.storage.v2.Bucket.Logging logging = 18;
*
* @return The logging.
*/
com.google.storage.v2.Bucket.Logging getLogging();
/**
*
*
*
* The bucket's logging config, which defines the destination bucket
* and name prefix (if any) for the current bucket's logs.
*
*
* .google.storage.v2.Bucket.Logging logging = 18;
*/
com.google.storage.v2.Bucket.LoggingOrBuilder getLoggingOrBuilder();
/**
*
*
*
* Output only. The owner of the bucket. This is always the project team's owner group.
*
*
* .google.storage.v2.Owner owner = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return Whether the owner field is set.
*/
boolean hasOwner();
/**
*
*
*
* Output only. The owner of the bucket. This is always the project team's owner group.
*
*
* .google.storage.v2.Owner owner = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*
* @return The owner.
*/
com.google.storage.v2.Owner getOwner();
/**
*
*
*
* Output only. The owner of the bucket. This is always the project team's owner group.
*
*
* .google.storage.v2.Owner owner = 19 [(.google.api.field_behavior) = OUTPUT_ONLY];
*/
com.google.storage.v2.OwnerOrBuilder getOwnerOrBuilder();
/**
*
*
*
* Encryption config for a bucket.
*
*
* .google.storage.v2.Bucket.Encryption encryption = 20;
*
* @return Whether the encryption field is set.
*/
boolean hasEncryption();
/**
*
*
*
* Encryption config for a bucket.
*
*
* .google.storage.v2.Bucket.Encryption encryption = 20;
*
* @return The encryption.
*/
com.google.storage.v2.Bucket.Encryption getEncryption();
/**
*
*
*
* Encryption config for a bucket.
*
*
* .google.storage.v2.Bucket.Encryption encryption = 20;
*/
com.google.storage.v2.Bucket.EncryptionOrBuilder getEncryptionOrBuilder();
/**
*
*
*
* The bucket's billing config.
*
*
* .google.storage.v2.Bucket.Billing billing = 21;
*
* @return Whether the billing field is set.
*/
boolean hasBilling();
/**
*
*
*
* The bucket's billing config.
*
*
* .google.storage.v2.Bucket.Billing billing = 21;
*
* @return The billing.
*/
com.google.storage.v2.Bucket.Billing getBilling();
/**
*
*
*
* The bucket's billing config.
*
*
* .google.storage.v2.Bucket.Billing billing = 21;
*/
com.google.storage.v2.Bucket.BillingOrBuilder getBillingOrBuilder();
/**
*
*
*
* The bucket's retention policy. The retention policy enforces a minimum
* retention time for all objects contained in the bucket, based on their
* creation time. Any attempt to overwrite or delete objects younger than the
* retention period will result in a PERMISSION_DENIED error. An unlocked
* retention policy can be modified or removed from the bucket via a
* storage.buckets.update operation. A locked retention policy cannot be
* removed or shortened in duration for the lifetime of the bucket.
* Attempting to remove or decrease period of a locked retention policy will
* result in a PERMISSION_DENIED error.
*
*
* .google.storage.v2.Bucket.RetentionPolicy retention_policy = 22;
*
* @return Whether the retentionPolicy field is set.
*/
boolean hasRetentionPolicy();
/**
*
*
*
* The bucket's retention policy. The retention policy enforces a minimum
* retention time for all objects contained in the bucket, based on their
* creation time. Any attempt to overwrite or delete objects younger than the
* retention period will result in a PERMISSION_DENIED error. An unlocked
* retention policy can be modified or removed from the bucket via a
* storage.buckets.update operation. A locked retention policy cannot be
* removed or shortened in duration for the lifetime of the bucket.
* Attempting to remove or decrease period of a locked retention policy will
* result in a PERMISSION_DENIED error.
*
*
* .google.storage.v2.Bucket.RetentionPolicy retention_policy = 22;
*
* @return The retentionPolicy.
*/
com.google.storage.v2.Bucket.RetentionPolicy getRetentionPolicy();
/**
*
*
*
* The bucket's retention policy. The retention policy enforces a minimum
* retention time for all objects contained in the bucket, based on their
* creation time. Any attempt to overwrite or delete objects younger than the
* retention period will result in a PERMISSION_DENIED error. An unlocked
* retention policy can be modified or removed from the bucket via a
* storage.buckets.update operation. A locked retention policy cannot be
* removed or shortened in duration for the lifetime of the bucket.
* Attempting to remove or decrease period of a locked retention policy will
* result in a PERMISSION_DENIED error.
*
*
* .google.storage.v2.Bucket.RetentionPolicy retention_policy = 22;
*/
com.google.storage.v2.Bucket.RetentionPolicyOrBuilder getRetentionPolicyOrBuilder();
/**
*
*
*
* The bucket's IAM config.
*
*
* .google.storage.v2.Bucket.IamConfig iam_config = 23;
*
* @return Whether the iamConfig field is set.
*/
boolean hasIamConfig();
/**
*
*
*
* The bucket's IAM config.
*
*
* .google.storage.v2.Bucket.IamConfig iam_config = 23;
*
* @return The iamConfig.
*/
com.google.storage.v2.Bucket.IamConfig getIamConfig();
/**
*
*
*
* The bucket's IAM config.
*
*
* .google.storage.v2.Bucket.IamConfig iam_config = 23;
*/
com.google.storage.v2.Bucket.IamConfigOrBuilder getIamConfigOrBuilder();
/**
*
*
*
* Immutable. The zone or zones from which the bucket is intended to use zonal quota.
* Requests for data from outside the specified affinities are still allowed
* but won't be able to use zonal quota. The values are case-insensitive.
* Attempting to update this field after bucket is created will result in an
* error.
*
*
* repeated string zone_affinity = 24 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return A list containing the zoneAffinity.
*/
java.util.List getZoneAffinityList();
/**
*
*
*
* Immutable. The zone or zones from which the bucket is intended to use zonal quota.
* Requests for data from outside the specified affinities are still allowed
* but won't be able to use zonal quota. The values are case-insensitive.
* Attempting to update this field after bucket is created will result in an
* error.
*
*
* repeated string zone_affinity = 24 [(.google.api.field_behavior) = IMMUTABLE];
*
* @return The count of zoneAffinity.
*/
int getZoneAffinityCount();
/**
*
*
*
* Immutable. The zone or zones from which the bucket is intended to use zonal quota.
* Requests for data from outside the specified affinities are still allowed
* but won't be able to use zonal quota. The values are case-insensitive.
* Attempting to update this field after bucket is created will result in an
* error.
*
*
* repeated string zone_affinity = 24 [(.google.api.field_behavior) = IMMUTABLE];
*
* @param index The index of the element to return.
* @return The zoneAffinity at the given index.
*/
java.lang.String getZoneAffinity(int index);
/**
*
*
*
* Immutable. The zone or zones from which the bucket is intended to use zonal quota.
* Requests for data from outside the specified affinities are still allowed
* but won't be able to use zonal quota. The values are case-insensitive.
* Attempting to update this field after bucket is created will result in an
* error.
*
*
* repeated string zone_affinity = 24 [(.google.api.field_behavior) = IMMUTABLE];
*
* @param index The index of the value to return.
* @return The bytes of the zoneAffinity at the given index.
*/
com.google.protobuf.ByteString getZoneAffinityBytes(int index);
/**
*
*
*
* Reserved for future use.
*
*
* bool satisfies_pzs = 25;
*
* @return The satisfiesPzs.
*/
boolean getSatisfiesPzs();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy