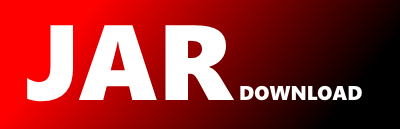
com.google.api.tools.framework.snippet.Context Maven / Gradle / Ivy
/*
* Copyright (C) 2016 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.api.tools.framework.snippet;
import com.google.api.tools.framework.snippet.SnippetSet.SnippetKey;
import com.google.common.base.Preconditions;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Maps;
import com.google.common.collect.Multimap;
import com.google.common.collect.Queues;
import java.util.Collection;
import java.util.Deque;
import java.util.Iterator;
import java.util.Map;
import javax.annotation.Nullable;
/**
* Represents a context for snippet evaluation. The context holds snippet
* definitions and global and local variables.
*/
public class Context {
private static final Map BUILTIN =
ImmutableMap.builder()
.put("BREAK", Doc.BREAK)
.put("SOFT_BREAK", Doc.SOFT_BREAK)
.put("EMPTY", Doc.EMPTY)
.put("TRUE", Values.TRUE)
.put("FALSE", Values.FALSE)
.put("COMMA_BREAK", Doc.text(",").add(Doc.BREAK))
.build();
private final Map snippets;
private final Multimap snippetsByName;
private final ImmutableMap globals;
private final Deque
© 2015 - 2024 Weber Informatics LLC | Privacy Policy