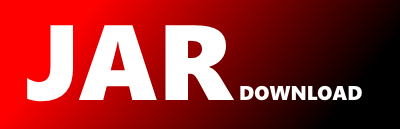
com.google.api.generator.gapic.protoparser.GapicLroRetrySettingsParser Maven / Gradle / Ivy
// Copyright 2020 Google LLC
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
package com.google.api.generator.gapic.protoparser;
import com.google.api.generator.gapic.model.GapicLroRetrySettings;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.base.Strings;
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import org.yaml.snakeyaml.LoaderOptions;
import org.yaml.snakeyaml.Yaml;
import org.yaml.snakeyaml.constructor.SafeConstructor;
public class GapicLroRetrySettingsParser {
private static final String YAML_KEY_INTERFACES = "interfaces";
private static final String YAML_KEY_NAME = "name";
private static final String YAML_KEY_METHODS = "methods";
private static final String YAML_KEY_LONG_RUNNING = "long_running";
private static final String YAML_KEY_INITIAL_POLL_DELAY_MILLIS = "initial_poll_delay_millis";
private static final String YAML_KEY_POLL_DELAY_MULTIPLIER = "poll_delay_multiplier";
private static final String YAML_KEY_MAX_POLL_DELAY_MILLIS = "max_poll_delay_millis";
private static final String YAML_KEY_TOTAL_POLL_TIMEOUT_MILLIS = "total_poll_timeout_millis";
public static Optional> parse(
Optional gapicYamlConfigFilePathOpt) {
return gapicYamlConfigFilePathOpt.isPresent()
? parse(gapicYamlConfigFilePathOpt.get())
: Optional.empty();
}
@VisibleForTesting
static Optional> parse(String gapicYamlConfigFilePath) {
if (Strings.isNullOrEmpty(gapicYamlConfigFilePath)
|| !(new File(gapicYamlConfigFilePath)).exists()) {
return Optional.empty();
}
String fileContents = null;
try {
fileContents =
new String(
Files.readAllBytes(Paths.get(gapicYamlConfigFilePath)), StandardCharsets.UTF_8);
} catch (IOException e) {
return Optional.empty();
}
Yaml yaml = new Yaml(new SafeConstructor(new LoaderOptions()));
Map yamlMap = yaml.load(fileContents);
return parseFromMap(yamlMap);
}
private static Optional> parseFromMap(Map yamlMap) {
if (!yamlMap.containsKey(YAML_KEY_INTERFACES)) {
return Optional.empty();
}
List settings = new ArrayList<>();
for (Map serviceYamlConfig :
(List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy