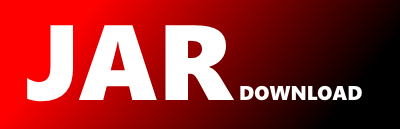
com.google.gapic.metadata.GapicMetadata Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: gapic_metadata.proto
// Protobuf Java Version: 3.25.4
package com.google.gapic.metadata;
/**
*
* Metadata about a GAPIC library for a specific combination of API, version, and
* computer language.
*
*
* Protobuf type {@code google.gapic.metadata.GapicMetadata}
*/
public final class GapicMetadata extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.gapic.metadata.GapicMetadata)
GapicMetadataOrBuilder {
private static final long serialVersionUID = 0L;
// Use GapicMetadata.newBuilder() to construct.
private GapicMetadata(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GapicMetadata() {
schema_ = "";
comment_ = "";
language_ = "";
protoPackage_ = "";
libraryPackage_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GapicMetadata();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 6:
return internalGetServices();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.gapic.metadata.GapicMetadata.class, com.google.gapic.metadata.GapicMetadata.Builder.class);
}
public interface ServiceForTransportOrBuilder extends
// @@protoc_insertion_point(interface_extends:google.gapic.metadata.GapicMetadata.ServiceForTransport)
com.google.protobuf.MessageOrBuilder {
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
int getClientsCount();
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
boolean containsClients(
java.lang.String key);
/**
* Use {@link #getClientsMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getClients();
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
java.util.Map
getClientsMap();
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
/* nullable */
com.google.gapic.metadata.GapicMetadata.ServiceAsClient getClientsOrDefault(
java.lang.String key,
/* nullable */
com.google.gapic.metadata.GapicMetadata.ServiceAsClient defaultValue);
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
com.google.gapic.metadata.GapicMetadata.ServiceAsClient getClientsOrThrow(
java.lang.String key);
}
/**
*
* A map from a transport name to ServiceAsClient, which allows
* listing information about the client objects that implement the
* parent RPC service for the specified transport.
*
* The key name is the transport, lower-cased with no separators
* (e.g. "grpc", "rest").
*
*
* Protobuf type {@code google.gapic.metadata.GapicMetadata.ServiceForTransport}
*/
public static final class ServiceForTransport extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.gapic.metadata.GapicMetadata.ServiceForTransport)
ServiceForTransportOrBuilder {
private static final long serialVersionUID = 0L;
// Use ServiceForTransport.newBuilder() to construct.
private ServiceForTransport(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ServiceForTransport() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ServiceForTransport();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceForTransport_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 1:
return internalGetClients();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceForTransport_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.gapic.metadata.GapicMetadata.ServiceForTransport.class, com.google.gapic.metadata.GapicMetadata.ServiceForTransport.Builder.class);
}
public static final int CLIENTS_FIELD_NUMBER = 1;
private static final class ClientsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.gapic.metadata.GapicMetadata.ServiceAsClient> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceForTransport_ClientsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.gapic.metadata.GapicMetadata.ServiceAsClient.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.gapic.metadata.GapicMetadata.ServiceAsClient> clients_;
private com.google.protobuf.MapField
internalGetClients() {
if (clients_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ClientsDefaultEntryHolder.defaultEntry);
}
return clients_;
}
public int getClientsCount() {
return internalGetClients().getMap().size();
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
@java.lang.Override
public boolean containsClients(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetClients().getMap().containsKey(key);
}
/**
* Use {@link #getClientsMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getClients() {
return getClientsMap();
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
@java.lang.Override
public java.util.Map getClientsMap() {
return internalGetClients().getMap();
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
@java.lang.Override
public /* nullable */
com.google.gapic.metadata.GapicMetadata.ServiceAsClient getClientsOrDefault(
java.lang.String key,
/* nullable */
com.google.gapic.metadata.GapicMetadata.ServiceAsClient defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetClients().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceAsClient getClientsOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetClients().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetClients(),
ClientsDefaultEntryHolder.defaultEntry,
1);
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (java.util.Map.Entry entry
: internalGetClients().getMap().entrySet()) {
com.google.protobuf.MapEntry
clients__ = ClientsDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, clients__);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.gapic.metadata.GapicMetadata.ServiceForTransport)) {
return super.equals(obj);
}
com.google.gapic.metadata.GapicMetadata.ServiceForTransport other = (com.google.gapic.metadata.GapicMetadata.ServiceForTransport) obj;
if (!internalGetClients().equals(
other.internalGetClients())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (!internalGetClients().getMap().isEmpty()) {
hash = (37 * hash) + CLIENTS_FIELD_NUMBER;
hash = (53 * hash) + internalGetClients().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.gapic.metadata.GapicMetadata.ServiceForTransport prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A map from a transport name to ServiceAsClient, which allows
* listing information about the client objects that implement the
* parent RPC service for the specified transport.
*
* The key name is the transport, lower-cased with no separators
* (e.g. "grpc", "rest").
*
*
* Protobuf type {@code google.gapic.metadata.GapicMetadata.ServiceForTransport}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.gapic.metadata.GapicMetadata.ServiceForTransport)
com.google.gapic.metadata.GapicMetadata.ServiceForTransportOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceForTransport_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 1:
return internalGetClients();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 1:
return internalGetMutableClients();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceForTransport_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.gapic.metadata.GapicMetadata.ServiceForTransport.class, com.google.gapic.metadata.GapicMetadata.ServiceForTransport.Builder.class);
}
// Construct using com.google.gapic.metadata.GapicMetadata.ServiceForTransport.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
internalGetMutableClients().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceForTransport_descriptor;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceForTransport getDefaultInstanceForType() {
return com.google.gapic.metadata.GapicMetadata.ServiceForTransport.getDefaultInstance();
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceForTransport build() {
com.google.gapic.metadata.GapicMetadata.ServiceForTransport result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceForTransport buildPartial() {
com.google.gapic.metadata.GapicMetadata.ServiceForTransport result = new com.google.gapic.metadata.GapicMetadata.ServiceForTransport(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.gapic.metadata.GapicMetadata.ServiceForTransport result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.clients_ = internalGetClients().build(ClientsDefaultEntryHolder.defaultEntry);
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.gapic.metadata.GapicMetadata.ServiceForTransport) {
return mergeFrom((com.google.gapic.metadata.GapicMetadata.ServiceForTransport)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.gapic.metadata.GapicMetadata.ServiceForTransport other) {
if (other == com.google.gapic.metadata.GapicMetadata.ServiceForTransport.getDefaultInstance()) return this;
internalGetMutableClients().mergeFrom(
other.internalGetClients());
bitField0_ |= 0x00000001;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.protobuf.MapEntry
clients__ = input.readMessage(
ClientsDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableClients().ensureBuilderMap().put(
clients__.getKey(), clients__.getValue());
bitField0_ |= 0x00000001;
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private static final class ClientsConverter implements com.google.protobuf.MapFieldBuilder.Converter {
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceAsClient build(com.google.gapic.metadata.GapicMetadata.ServiceAsClientOrBuilder val) {
if (val instanceof com.google.gapic.metadata.GapicMetadata.ServiceAsClient) { return (com.google.gapic.metadata.GapicMetadata.ServiceAsClient) val; }
return ((com.google.gapic.metadata.GapicMetadata.ServiceAsClient.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry defaultEntry() {
return ClientsDefaultEntryHolder.defaultEntry;
}
};
private static final ClientsConverter clientsConverter = new ClientsConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String, com.google.gapic.metadata.GapicMetadata.ServiceAsClientOrBuilder, com.google.gapic.metadata.GapicMetadata.ServiceAsClient, com.google.gapic.metadata.GapicMetadata.ServiceAsClient.Builder> clients_;
private com.google.protobuf.MapFieldBuilder
internalGetClients() {
if (clients_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(clientsConverter);
}
return clients_;
}
private com.google.protobuf.MapFieldBuilder
internalGetMutableClients() {
if (clients_ == null) {
clients_ = new com.google.protobuf.MapFieldBuilder<>(clientsConverter);
}
bitField0_ |= 0x00000001;
onChanged();
return clients_;
}
public int getClientsCount() {
return internalGetClients().ensureBuilderMap().size();
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
@java.lang.Override
public boolean containsClients(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetClients().ensureBuilderMap().containsKey(key);
}
/**
* Use {@link #getClientsMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getClients() {
return getClientsMap();
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
@java.lang.Override
public java.util.Map getClientsMap() {
return internalGetClients().getImmutableMap();
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
@java.lang.Override
public /* nullable */
com.google.gapic.metadata.GapicMetadata.ServiceAsClient getClientsOrDefault(
java.lang.String key,
/* nullable */
com.google.gapic.metadata.GapicMetadata.ServiceAsClient defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map = internalGetMutableClients().ensureBuilderMap();
return map.containsKey(key) ? clientsConverter.build(map.get(key)) : defaultValue;
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceAsClient getClientsOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map = internalGetMutableClients().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return clientsConverter.build(map.get(key));
}
public Builder clearClients() {
bitField0_ = (bitField0_ & ~0x00000001);
internalGetMutableClients().clear();
return this;
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
public Builder removeClients(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableClients().ensureBuilderMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableClients() {
bitField0_ |= 0x00000001;
return internalGetMutableClients().ensureMessageMap();
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
public Builder putClients(
java.lang.String key,
com.google.gapic.metadata.GapicMetadata.ServiceAsClient value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableClients().ensureBuilderMap()
.put(key, value);
bitField0_ |= 0x00000001;
return this;
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
public Builder putAllClients(
java.util.Map values) {
for (java.util.Map.Entry e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableClients().ensureBuilderMap()
.putAll(values);
bitField0_ |= 0x00000001;
return this;
}
/**
* map<string, .google.gapic.metadata.GapicMetadata.ServiceAsClient> clients = 1;
*/
public com.google.gapic.metadata.GapicMetadata.ServiceAsClient.Builder putClientsBuilderIfAbsent(
java.lang.String key) {
java.util.Map builderMap = internalGetMutableClients().ensureBuilderMap();
com.google.gapic.metadata.GapicMetadata.ServiceAsClientOrBuilder entry = builderMap.get(key);
if (entry == null) {
entry = com.google.gapic.metadata.GapicMetadata.ServiceAsClient.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.gapic.metadata.GapicMetadata.ServiceAsClient) {
entry = ((com.google.gapic.metadata.GapicMetadata.ServiceAsClient) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.gapic.metadata.GapicMetadata.ServiceAsClient.Builder) entry;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.gapic.metadata.GapicMetadata.ServiceForTransport)
}
// @@protoc_insertion_point(class_scope:google.gapic.metadata.GapicMetadata.ServiceForTransport)
private static final com.google.gapic.metadata.GapicMetadata.ServiceForTransport DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.gapic.metadata.GapicMetadata.ServiceForTransport();
}
public static com.google.gapic.metadata.GapicMetadata.ServiceForTransport getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ServiceForTransport parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceForTransport getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ServiceAsClientOrBuilder extends
// @@protoc_insertion_point(interface_extends:google.gapic.metadata.GapicMetadata.ServiceAsClient)
com.google.protobuf.MessageOrBuilder {
/**
*
* The name of the library client formatted as it appears in the source code
*
*
* string library_client = 1;
* @return The libraryClient.
*/
java.lang.String getLibraryClient();
/**
*
* The name of the library client formatted as it appears in the source code
*
*
* string library_client = 1;
* @return The bytes for libraryClient.
*/
com.google.protobuf.ByteString
getLibraryClientBytes();
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
int getRpcsCount();
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
boolean containsRpcs(
java.lang.String key);
/**
* Use {@link #getRpcsMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getRpcs();
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
java.util.Map
getRpcsMap();
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
/* nullable */
com.google.gapic.metadata.GapicMetadata.MethodList getRpcsOrDefault(
java.lang.String key,
/* nullable */
com.google.gapic.metadata.GapicMetadata.MethodList defaultValue);
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
com.google.gapic.metadata.GapicMetadata.MethodList getRpcsOrThrow(
java.lang.String key);
}
/**
*
* Information about a specific client implementing a proto-defined service.
*
*
* Protobuf type {@code google.gapic.metadata.GapicMetadata.ServiceAsClient}
*/
public static final class ServiceAsClient extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.gapic.metadata.GapicMetadata.ServiceAsClient)
ServiceAsClientOrBuilder {
private static final long serialVersionUID = 0L;
// Use ServiceAsClient.newBuilder() to construct.
private ServiceAsClient(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ServiceAsClient() {
libraryClient_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ServiceAsClient();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceAsClient_descriptor;
}
@SuppressWarnings({"rawtypes"})
@java.lang.Override
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 2:
return internalGetRpcs();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceAsClient_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.gapic.metadata.GapicMetadata.ServiceAsClient.class, com.google.gapic.metadata.GapicMetadata.ServiceAsClient.Builder.class);
}
public static final int LIBRARY_CLIENT_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object libraryClient_ = "";
/**
*
* The name of the library client formatted as it appears in the source code
*
*
* string library_client = 1;
* @return The libraryClient.
*/
@java.lang.Override
public java.lang.String getLibraryClient() {
java.lang.Object ref = libraryClient_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
libraryClient_ = s;
return s;
}
}
/**
*
* The name of the library client formatted as it appears in the source code
*
*
* string library_client = 1;
* @return The bytes for libraryClient.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLibraryClientBytes() {
java.lang.Object ref = libraryClient_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
libraryClient_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RPCS_FIELD_NUMBER = 2;
private static final class RpcsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.gapic.metadata.GapicMetadata.MethodList> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceAsClient_RpcsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.gapic.metadata.GapicMetadata.MethodList.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.gapic.metadata.GapicMetadata.MethodList> rpcs_;
private com.google.protobuf.MapField
internalGetRpcs() {
if (rpcs_ == null) {
return com.google.protobuf.MapField.emptyMapField(
RpcsDefaultEntryHolder.defaultEntry);
}
return rpcs_;
}
public int getRpcsCount() {
return internalGetRpcs().getMap().size();
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
@java.lang.Override
public boolean containsRpcs(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetRpcs().getMap().containsKey(key);
}
/**
* Use {@link #getRpcsMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getRpcs() {
return getRpcsMap();
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
@java.lang.Override
public java.util.Map getRpcsMap() {
return internalGetRpcs().getMap();
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
@java.lang.Override
public /* nullable */
com.google.gapic.metadata.GapicMetadata.MethodList getRpcsOrDefault(
java.lang.String key,
/* nullable */
com.google.gapic.metadata.GapicMetadata.MethodList defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetRpcs().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.MethodList getRpcsOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetRpcs().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(libraryClient_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, libraryClient_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetRpcs(),
RpcsDefaultEntryHolder.defaultEntry,
2);
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(libraryClient_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, libraryClient_);
}
for (java.util.Map.Entry entry
: internalGetRpcs().getMap().entrySet()) {
com.google.protobuf.MapEntry
rpcs__ = RpcsDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, rpcs__);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.gapic.metadata.GapicMetadata.ServiceAsClient)) {
return super.equals(obj);
}
com.google.gapic.metadata.GapicMetadata.ServiceAsClient other = (com.google.gapic.metadata.GapicMetadata.ServiceAsClient) obj;
if (!getLibraryClient()
.equals(other.getLibraryClient())) return false;
if (!internalGetRpcs().equals(
other.internalGetRpcs())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + LIBRARY_CLIENT_FIELD_NUMBER;
hash = (53 * hash) + getLibraryClient().hashCode();
if (!internalGetRpcs().getMap().isEmpty()) {
hash = (37 * hash) + RPCS_FIELD_NUMBER;
hash = (53 * hash) + internalGetRpcs().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.gapic.metadata.GapicMetadata.ServiceAsClient prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Information about a specific client implementing a proto-defined service.
*
*
* Protobuf type {@code google.gapic.metadata.GapicMetadata.ServiceAsClient}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.gapic.metadata.GapicMetadata.ServiceAsClient)
com.google.gapic.metadata.GapicMetadata.ServiceAsClientOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceAsClient_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 2:
return internalGetRpcs();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 2:
return internalGetMutableRpcs();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceAsClient_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.gapic.metadata.GapicMetadata.ServiceAsClient.class, com.google.gapic.metadata.GapicMetadata.ServiceAsClient.Builder.class);
}
// Construct using com.google.gapic.metadata.GapicMetadata.ServiceAsClient.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
libraryClient_ = "";
internalGetMutableRpcs().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServiceAsClient_descriptor;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceAsClient getDefaultInstanceForType() {
return com.google.gapic.metadata.GapicMetadata.ServiceAsClient.getDefaultInstance();
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceAsClient build() {
com.google.gapic.metadata.GapicMetadata.ServiceAsClient result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceAsClient buildPartial() {
com.google.gapic.metadata.GapicMetadata.ServiceAsClient result = new com.google.gapic.metadata.GapicMetadata.ServiceAsClient(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.gapic.metadata.GapicMetadata.ServiceAsClient result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.libraryClient_ = libraryClient_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.rpcs_ = internalGetRpcs().build(RpcsDefaultEntryHolder.defaultEntry);
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.gapic.metadata.GapicMetadata.ServiceAsClient) {
return mergeFrom((com.google.gapic.metadata.GapicMetadata.ServiceAsClient)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.gapic.metadata.GapicMetadata.ServiceAsClient other) {
if (other == com.google.gapic.metadata.GapicMetadata.ServiceAsClient.getDefaultInstance()) return this;
if (!other.getLibraryClient().isEmpty()) {
libraryClient_ = other.libraryClient_;
bitField0_ |= 0x00000001;
onChanged();
}
internalGetMutableRpcs().mergeFrom(
other.internalGetRpcs());
bitField0_ |= 0x00000002;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
libraryClient_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
com.google.protobuf.MapEntry
rpcs__ = input.readMessage(
RpcsDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableRpcs().ensureBuilderMap().put(
rpcs__.getKey(), rpcs__.getValue());
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object libraryClient_ = "";
/**
*
* The name of the library client formatted as it appears in the source code
*
*
* string library_client = 1;
* @return The libraryClient.
*/
public java.lang.String getLibraryClient() {
java.lang.Object ref = libraryClient_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
libraryClient_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the library client formatted as it appears in the source code
*
*
* string library_client = 1;
* @return The bytes for libraryClient.
*/
public com.google.protobuf.ByteString
getLibraryClientBytes() {
java.lang.Object ref = libraryClient_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
libraryClient_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the library client formatted as it appears in the source code
*
*
* string library_client = 1;
* @param value The libraryClient to set.
* @return This builder for chaining.
*/
public Builder setLibraryClient(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
libraryClient_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The name of the library client formatted as it appears in the source code
*
*
* string library_client = 1;
* @return This builder for chaining.
*/
public Builder clearLibraryClient() {
libraryClient_ = getDefaultInstance().getLibraryClient();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* The name of the library client formatted as it appears in the source code
*
*
* string library_client = 1;
* @param value The bytes for libraryClient to set.
* @return This builder for chaining.
*/
public Builder setLibraryClientBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
libraryClient_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private static final class RpcsConverter implements com.google.protobuf.MapFieldBuilder.Converter {
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.MethodList build(com.google.gapic.metadata.GapicMetadata.MethodListOrBuilder val) {
if (val instanceof com.google.gapic.metadata.GapicMetadata.MethodList) { return (com.google.gapic.metadata.GapicMetadata.MethodList) val; }
return ((com.google.gapic.metadata.GapicMetadata.MethodList.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry defaultEntry() {
return RpcsDefaultEntryHolder.defaultEntry;
}
};
private static final RpcsConverter rpcsConverter = new RpcsConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String, com.google.gapic.metadata.GapicMetadata.MethodListOrBuilder, com.google.gapic.metadata.GapicMetadata.MethodList, com.google.gapic.metadata.GapicMetadata.MethodList.Builder> rpcs_;
private com.google.protobuf.MapFieldBuilder
internalGetRpcs() {
if (rpcs_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(rpcsConverter);
}
return rpcs_;
}
private com.google.protobuf.MapFieldBuilder
internalGetMutableRpcs() {
if (rpcs_ == null) {
rpcs_ = new com.google.protobuf.MapFieldBuilder<>(rpcsConverter);
}
bitField0_ |= 0x00000002;
onChanged();
return rpcs_;
}
public int getRpcsCount() {
return internalGetRpcs().ensureBuilderMap().size();
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
@java.lang.Override
public boolean containsRpcs(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetRpcs().ensureBuilderMap().containsKey(key);
}
/**
* Use {@link #getRpcsMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getRpcs() {
return getRpcsMap();
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
@java.lang.Override
public java.util.Map getRpcsMap() {
return internalGetRpcs().getImmutableMap();
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
@java.lang.Override
public /* nullable */
com.google.gapic.metadata.GapicMetadata.MethodList getRpcsOrDefault(
java.lang.String key,
/* nullable */
com.google.gapic.metadata.GapicMetadata.MethodList defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map = internalGetMutableRpcs().ensureBuilderMap();
return map.containsKey(key) ? rpcsConverter.build(map.get(key)) : defaultValue;
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.MethodList getRpcsOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map = internalGetMutableRpcs().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return rpcsConverter.build(map.get(key));
}
public Builder clearRpcs() {
bitField0_ = (bitField0_ & ~0x00000002);
internalGetMutableRpcs().clear();
return this;
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
public Builder removeRpcs(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableRpcs().ensureBuilderMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableRpcs() {
bitField0_ |= 0x00000002;
return internalGetMutableRpcs().ensureMessageMap();
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
public Builder putRpcs(
java.lang.String key,
com.google.gapic.metadata.GapicMetadata.MethodList value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableRpcs().ensureBuilderMap()
.put(key, value);
bitField0_ |= 0x00000002;
return this;
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
public Builder putAllRpcs(
java.util.Map values) {
for (java.util.Map.Entry e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableRpcs().ensureBuilderMap()
.putAll(values);
bitField0_ |= 0x00000002;
return this;
}
/**
*
* A mapping from each proto-defined RPC name to the the list of
* methods in library_client that implement it. There can be more
* than one library_client method for each RPC. RPCs with no
* library_client methods need not be included.
*
* The key name is the name of the RPC as defined and formated in
* the proto file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.MethodList> rpcs = 2;
*/
public com.google.gapic.metadata.GapicMetadata.MethodList.Builder putRpcsBuilderIfAbsent(
java.lang.String key) {
java.util.Map builderMap = internalGetMutableRpcs().ensureBuilderMap();
com.google.gapic.metadata.GapicMetadata.MethodListOrBuilder entry = builderMap.get(key);
if (entry == null) {
entry = com.google.gapic.metadata.GapicMetadata.MethodList.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.gapic.metadata.GapicMetadata.MethodList) {
entry = ((com.google.gapic.metadata.GapicMetadata.MethodList) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.gapic.metadata.GapicMetadata.MethodList.Builder) entry;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.gapic.metadata.GapicMetadata.ServiceAsClient)
}
// @@protoc_insertion_point(class_scope:google.gapic.metadata.GapicMetadata.ServiceAsClient)
private static final com.google.gapic.metadata.GapicMetadata.ServiceAsClient DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.gapic.metadata.GapicMetadata.ServiceAsClient();
}
public static com.google.gapic.metadata.GapicMetadata.ServiceAsClient getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ServiceAsClient parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceAsClient getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MethodListOrBuilder extends
// @@protoc_insertion_point(interface_extends:google.gapic.metadata.GapicMetadata.MethodList)
com.google.protobuf.MessageOrBuilder {
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @return A list containing the methods.
*/
java.util.List
getMethodsList();
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @return The count of methods.
*/
int getMethodsCount();
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @param index The index of the element to return.
* @return The methods at the given index.
*/
java.lang.String getMethods(int index);
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @param index The index of the value to return.
* @return The bytes of the methods at the given index.
*/
com.google.protobuf.ByteString
getMethodsBytes(int index);
}
/**
*
* List of GAPIC client methods implementing the proto-defined RPC
* for the transport and service specified in the containing
* structures.
*
*
* Protobuf type {@code google.gapic.metadata.GapicMetadata.MethodList}
*/
public static final class MethodList extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:google.gapic.metadata.GapicMetadata.MethodList)
MethodListOrBuilder {
private static final long serialVersionUID = 0L;
// Use MethodList.newBuilder() to construct.
private MethodList(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MethodList() {
methods_ =
com.google.protobuf.LazyStringArrayList.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MethodList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_MethodList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_MethodList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.gapic.metadata.GapicMetadata.MethodList.class, com.google.gapic.metadata.GapicMetadata.MethodList.Builder.class);
}
public static final int METHODS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList methods_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @return A list containing the methods.
*/
public com.google.protobuf.ProtocolStringList
getMethodsList() {
return methods_;
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @return The count of methods.
*/
public int getMethodsCount() {
return methods_.size();
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @param index The index of the element to return.
* @return The methods at the given index.
*/
public java.lang.String getMethods(int index) {
return methods_.get(index);
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @param index The index of the value to return.
* @return The bytes of the methods at the given index.
*/
public com.google.protobuf.ByteString
getMethodsBytes(int index) {
return methods_.getByteString(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < methods_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, methods_.getRaw(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < methods_.size(); i++) {
dataSize += computeStringSizeNoTag(methods_.getRaw(i));
}
size += dataSize;
size += 1 * getMethodsList().size();
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.gapic.metadata.GapicMetadata.MethodList)) {
return super.equals(obj);
}
com.google.gapic.metadata.GapicMetadata.MethodList other = (com.google.gapic.metadata.GapicMetadata.MethodList) obj;
if (!getMethodsList()
.equals(other.getMethodsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getMethodsCount() > 0) {
hash = (37 * hash) + METHODS_FIELD_NUMBER;
hash = (53 * hash) + getMethodsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata.MethodList parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.gapic.metadata.GapicMetadata.MethodList prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* List of GAPIC client methods implementing the proto-defined RPC
* for the transport and service specified in the containing
* structures.
*
*
* Protobuf type {@code google.gapic.metadata.GapicMetadata.MethodList}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.gapic.metadata.GapicMetadata.MethodList)
com.google.gapic.metadata.GapicMetadata.MethodListOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_MethodList_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_MethodList_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.gapic.metadata.GapicMetadata.MethodList.class, com.google.gapic.metadata.GapicMetadata.MethodList.Builder.class);
}
// Construct using com.google.gapic.metadata.GapicMetadata.MethodList.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
methods_ =
com.google.protobuf.LazyStringArrayList.emptyList();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_MethodList_descriptor;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.MethodList getDefaultInstanceForType() {
return com.google.gapic.metadata.GapicMetadata.MethodList.getDefaultInstance();
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.MethodList build() {
com.google.gapic.metadata.GapicMetadata.MethodList result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.MethodList buildPartial() {
com.google.gapic.metadata.GapicMetadata.MethodList result = new com.google.gapic.metadata.GapicMetadata.MethodList(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.gapic.metadata.GapicMetadata.MethodList result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
methods_.makeImmutable();
result.methods_ = methods_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.gapic.metadata.GapicMetadata.MethodList) {
return mergeFrom((com.google.gapic.metadata.GapicMetadata.MethodList)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.gapic.metadata.GapicMetadata.MethodList other) {
if (other == com.google.gapic.metadata.GapicMetadata.MethodList.getDefaultInstance()) return this;
if (!other.methods_.isEmpty()) {
if (methods_.isEmpty()) {
methods_ = other.methods_;
bitField0_ |= 0x00000001;
} else {
ensureMethodsIsMutable();
methods_.addAll(other.methods_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
ensureMethodsIsMutable();
methods_.add(s);
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringArrayList methods_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureMethodsIsMutable() {
if (!methods_.isModifiable()) {
methods_ = new com.google.protobuf.LazyStringArrayList(methods_);
}
bitField0_ |= 0x00000001;
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @return A list containing the methods.
*/
public com.google.protobuf.ProtocolStringList
getMethodsList() {
methods_.makeImmutable();
return methods_;
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @return The count of methods.
*/
public int getMethodsCount() {
return methods_.size();
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @param index The index of the element to return.
* @return The methods at the given index.
*/
public java.lang.String getMethods(int index) {
return methods_.get(index);
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @param index The index of the value to return.
* @return The bytes of the methods at the given index.
*/
public com.google.protobuf.ByteString
getMethodsBytes(int index) {
return methods_.getByteString(index);
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @param index The index to set the value at.
* @param value The methods to set.
* @return This builder for chaining.
*/
public Builder setMethods(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureMethodsIsMutable();
methods_.set(index, value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @param value The methods to add.
* @return This builder for chaining.
*/
public Builder addMethods(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureMethodsIsMutable();
methods_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @param values The methods to add.
* @return This builder for chaining.
*/
public Builder addAllMethods(
java.lang.Iterable values) {
ensureMethodsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, methods_);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @return This builder for chaining.
*/
public Builder clearMethods() {
methods_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);;
onChanged();
return this;
}
/**
*
* List of methods for a specific proto-service client in the
* GAPIC. These names should be formatted as they appear in the
* source code.
*
*
* repeated string methods = 1;
* @param value The bytes of the methods to add.
* @return This builder for chaining.
*/
public Builder addMethodsBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureMethodsIsMutable();
methods_.add(value);
bitField0_ |= 0x00000001;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.gapic.metadata.GapicMetadata.MethodList)
}
// @@protoc_insertion_point(class_scope:google.gapic.metadata.GapicMetadata.MethodList)
private static final com.google.gapic.metadata.GapicMetadata.MethodList DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.gapic.metadata.GapicMetadata.MethodList();
}
public static com.google.gapic.metadata.GapicMetadata.MethodList getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MethodList parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.MethodList getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int SCHEMA_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object schema_ = "";
/**
*
* Schema version of this proto. Current value: 1.0
*
*
* string schema = 1;
* @return The schema.
*/
@java.lang.Override
public java.lang.String getSchema() {
java.lang.Object ref = schema_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schema_ = s;
return s;
}
}
/**
*
* Schema version of this proto. Current value: 1.0
*
*
* string schema = 1;
* @return The bytes for schema.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSchemaBytes() {
java.lang.Object ref = schema_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schema_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMMENT_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object comment_ = "";
/**
*
* Any human-readable comments to be included in this file.
*
*
* string comment = 2;
* @return The comment.
*/
@java.lang.Override
public java.lang.String getComment() {
java.lang.Object ref = comment_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
comment_ = s;
return s;
}
}
/**
*
* Any human-readable comments to be included in this file.
*
*
* string comment = 2;
* @return The bytes for comment.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCommentBytes() {
java.lang.Object ref = comment_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
comment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LANGUAGE_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object language_ = "";
/**
*
* Computer language of this generated language. This must be
* spelled out as it spoken in English, with no capitalization or
* separators (e.g. "csharp", "nodejs").
*
*
* string language = 3;
* @return The language.
*/
@java.lang.Override
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
language_ = s;
return s;
}
}
/**
*
* Computer language of this generated language. This must be
* spelled out as it spoken in English, with no capitalization or
* separators (e.g. "csharp", "nodejs").
*
*
* string language = 3;
* @return The bytes for language.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROTO_PACKAGE_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object protoPackage_ = "";
/**
*
* The proto package containing the API definition for which this
* GAPIC library was generated.
*
*
* string proto_package = 4;
* @return The protoPackage.
*/
@java.lang.Override
public java.lang.String getProtoPackage() {
java.lang.Object ref = protoPackage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
protoPackage_ = s;
return s;
}
}
/**
*
* The proto package containing the API definition for which this
* GAPIC library was generated.
*
*
* string proto_package = 4;
* @return The bytes for protoPackage.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProtoPackageBytes() {
java.lang.Object ref = protoPackage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protoPackage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LIBRARY_PACKAGE_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object libraryPackage_ = "";
/**
*
* The language-specific library package for this GAPIC library.
*
*
* string library_package = 5;
* @return The libraryPackage.
*/
@java.lang.Override
public java.lang.String getLibraryPackage() {
java.lang.Object ref = libraryPackage_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
libraryPackage_ = s;
return s;
}
}
/**
*
* The language-specific library package for this GAPIC library.
*
*
* string library_package = 5;
* @return The bytes for libraryPackage.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLibraryPackageBytes() {
java.lang.Object ref = libraryPackage_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
libraryPackage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERVICES_FIELD_NUMBER = 6;
private static final class ServicesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, com.google.gapic.metadata.GapicMetadata.ServiceForTransport> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_ServicesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
com.google.gapic.metadata.GapicMetadata.ServiceForTransport.getDefaultInstance());
}
@SuppressWarnings("serial")
private com.google.protobuf.MapField<
java.lang.String, com.google.gapic.metadata.GapicMetadata.ServiceForTransport> services_;
private com.google.protobuf.MapField
internalGetServices() {
if (services_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ServicesDefaultEntryHolder.defaultEntry);
}
return services_;
}
public int getServicesCount() {
return internalGetServices().getMap().size();
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
@java.lang.Override
public boolean containsServices(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetServices().getMap().containsKey(key);
}
/**
* Use {@link #getServicesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getServices() {
return getServicesMap();
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
@java.lang.Override
public java.util.Map getServicesMap() {
return internalGetServices().getMap();
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
@java.lang.Override
public /* nullable */
com.google.gapic.metadata.GapicMetadata.ServiceForTransport getServicesOrDefault(
java.lang.String key,
/* nullable */
com.google.gapic.metadata.GapicMetadata.ServiceForTransport defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetServices().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceForTransport getServicesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map =
internalGetServices().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schema_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, schema_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(comment_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, comment_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(language_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, language_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(protoPackage_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, protoPackage_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(libraryPackage_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, libraryPackage_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetServices(),
ServicesDefaultEntryHolder.defaultEntry,
6);
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schema_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, schema_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(comment_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, comment_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(language_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, language_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(protoPackage_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, protoPackage_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(libraryPackage_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, libraryPackage_);
}
for (java.util.Map.Entry entry
: internalGetServices().getMap().entrySet()) {
com.google.protobuf.MapEntry
services__ = ServicesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, services__);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.gapic.metadata.GapicMetadata)) {
return super.equals(obj);
}
com.google.gapic.metadata.GapicMetadata other = (com.google.gapic.metadata.GapicMetadata) obj;
if (!getSchema()
.equals(other.getSchema())) return false;
if (!getComment()
.equals(other.getComment())) return false;
if (!getLanguage()
.equals(other.getLanguage())) return false;
if (!getProtoPackage()
.equals(other.getProtoPackage())) return false;
if (!getLibraryPackage()
.equals(other.getLibraryPackage())) return false;
if (!internalGetServices().equals(
other.internalGetServices())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SCHEMA_FIELD_NUMBER;
hash = (53 * hash) + getSchema().hashCode();
hash = (37 * hash) + COMMENT_FIELD_NUMBER;
hash = (53 * hash) + getComment().hashCode();
hash = (37 * hash) + LANGUAGE_FIELD_NUMBER;
hash = (53 * hash) + getLanguage().hashCode();
hash = (37 * hash) + PROTO_PACKAGE_FIELD_NUMBER;
hash = (53 * hash) + getProtoPackage().hashCode();
hash = (37 * hash) + LIBRARY_PACKAGE_FIELD_NUMBER;
hash = (53 * hash) + getLibraryPackage().hashCode();
if (!internalGetServices().getMap().isEmpty()) {
hash = (37 * hash) + SERVICES_FIELD_NUMBER;
hash = (53 * hash) + internalGetServices().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.gapic.metadata.GapicMetadata parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.gapic.metadata.GapicMetadata parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.gapic.metadata.GapicMetadata parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.gapic.metadata.GapicMetadata parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.gapic.metadata.GapicMetadata prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Metadata about a GAPIC library for a specific combination of API, version, and
* computer language.
*
*
* Protobuf type {@code google.gapic.metadata.GapicMetadata}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:google.gapic.metadata.GapicMetadata)
com.google.gapic.metadata.GapicMetadataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMapFieldReflection(
int number) {
switch (number) {
case 6:
return internalGetServices();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapFieldReflectionAccessor internalGetMutableMapFieldReflection(
int number) {
switch (number) {
case 6:
return internalGetMutableServices();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.gapic.metadata.GapicMetadata.class, com.google.gapic.metadata.GapicMetadata.Builder.class);
}
// Construct using com.google.gapic.metadata.GapicMetadata.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
schema_ = "";
comment_ = "";
language_ = "";
protoPackage_ = "";
libraryPackage_ = "";
internalGetMutableServices().clear();
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.gapic.metadata.GapicMetadataProto.internal_static_google_gapic_metadata_GapicMetadata_descriptor;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata getDefaultInstanceForType() {
return com.google.gapic.metadata.GapicMetadata.getDefaultInstance();
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata build() {
com.google.gapic.metadata.GapicMetadata result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata buildPartial() {
com.google.gapic.metadata.GapicMetadata result = new com.google.gapic.metadata.GapicMetadata(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(com.google.gapic.metadata.GapicMetadata result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.schema_ = schema_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.comment_ = comment_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.language_ = language_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.protoPackage_ = protoPackage_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.libraryPackage_ = libraryPackage_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.services_ = internalGetServices().build(ServicesDefaultEntryHolder.defaultEntry);
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.gapic.metadata.GapicMetadata) {
return mergeFrom((com.google.gapic.metadata.GapicMetadata)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.gapic.metadata.GapicMetadata other) {
if (other == com.google.gapic.metadata.GapicMetadata.getDefaultInstance()) return this;
if (!other.getSchema().isEmpty()) {
schema_ = other.schema_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getComment().isEmpty()) {
comment_ = other.comment_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.getLanguage().isEmpty()) {
language_ = other.language_;
bitField0_ |= 0x00000004;
onChanged();
}
if (!other.getProtoPackage().isEmpty()) {
protoPackage_ = other.protoPackage_;
bitField0_ |= 0x00000008;
onChanged();
}
if (!other.getLibraryPackage().isEmpty()) {
libraryPackage_ = other.libraryPackage_;
bitField0_ |= 0x00000010;
onChanged();
}
internalGetMutableServices().mergeFrom(
other.internalGetServices());
bitField0_ |= 0x00000020;
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
schema_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
comment_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
language_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
protoPackage_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
libraryPackage_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
com.google.protobuf.MapEntry
services__ = input.readMessage(
ServicesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
internalGetMutableServices().ensureBuilderMap().put(
services__.getKey(), services__.getValue());
bitField0_ |= 0x00000020;
break;
} // case 50
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object schema_ = "";
/**
*
* Schema version of this proto. Current value: 1.0
*
*
* string schema = 1;
* @return The schema.
*/
public java.lang.String getSchema() {
java.lang.Object ref = schema_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schema_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Schema version of this proto. Current value: 1.0
*
*
* string schema = 1;
* @return The bytes for schema.
*/
public com.google.protobuf.ByteString
getSchemaBytes() {
java.lang.Object ref = schema_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schema_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Schema version of this proto. Current value: 1.0
*
*
* string schema = 1;
* @param value The schema to set.
* @return This builder for chaining.
*/
public Builder setSchema(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
schema_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Schema version of this proto. Current value: 1.0
*
*
* string schema = 1;
* @return This builder for chaining.
*/
public Builder clearSchema() {
schema_ = getDefaultInstance().getSchema();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Schema version of this proto. Current value: 1.0
*
*
* string schema = 1;
* @param value The bytes for schema to set.
* @return This builder for chaining.
*/
public Builder setSchemaBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
schema_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object comment_ = "";
/**
*
* Any human-readable comments to be included in this file.
*
*
* string comment = 2;
* @return The comment.
*/
public java.lang.String getComment() {
java.lang.Object ref = comment_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
comment_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Any human-readable comments to be included in this file.
*
*
* string comment = 2;
* @return The bytes for comment.
*/
public com.google.protobuf.ByteString
getCommentBytes() {
java.lang.Object ref = comment_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
comment_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Any human-readable comments to be included in this file.
*
*
* string comment = 2;
* @param value The comment to set.
* @return This builder for chaining.
*/
public Builder setComment(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
comment_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Any human-readable comments to be included in this file.
*
*
* string comment = 2;
* @return This builder for chaining.
*/
public Builder clearComment() {
comment_ = getDefaultInstance().getComment();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* Any human-readable comments to be included in this file.
*
*
* string comment = 2;
* @param value The bytes for comment to set.
* @return This builder for chaining.
*/
public Builder setCommentBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
comment_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object language_ = "";
/**
*
* Computer language of this generated language. This must be
* spelled out as it spoken in English, with no capitalization or
* separators (e.g. "csharp", "nodejs").
*
*
* string language = 3;
* @return The language.
*/
public java.lang.String getLanguage() {
java.lang.Object ref = language_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
language_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Computer language of this generated language. This must be
* spelled out as it spoken in English, with no capitalization or
* separators (e.g. "csharp", "nodejs").
*
*
* string language = 3;
* @return The bytes for language.
*/
public com.google.protobuf.ByteString
getLanguageBytes() {
java.lang.Object ref = language_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
language_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Computer language of this generated language. This must be
* spelled out as it spoken in English, with no capitalization or
* separators (e.g. "csharp", "nodejs").
*
*
* string language = 3;
* @param value The language to set.
* @return This builder for chaining.
*/
public Builder setLanguage(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
language_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Computer language of this generated language. This must be
* spelled out as it spoken in English, with no capitalization or
* separators (e.g. "csharp", "nodejs").
*
*
* string language = 3;
* @return This builder for chaining.
*/
public Builder clearLanguage() {
language_ = getDefaultInstance().getLanguage();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* Computer language of this generated language. This must be
* spelled out as it spoken in English, with no capitalization or
* separators (e.g. "csharp", "nodejs").
*
*
* string language = 3;
* @param value The bytes for language to set.
* @return This builder for chaining.
*/
public Builder setLanguageBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
language_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object protoPackage_ = "";
/**
*
* The proto package containing the API definition for which this
* GAPIC library was generated.
*
*
* string proto_package = 4;
* @return The protoPackage.
*/
public java.lang.String getProtoPackage() {
java.lang.Object ref = protoPackage_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
protoPackage_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The proto package containing the API definition for which this
* GAPIC library was generated.
*
*
* string proto_package = 4;
* @return The bytes for protoPackage.
*/
public com.google.protobuf.ByteString
getProtoPackageBytes() {
java.lang.Object ref = protoPackage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protoPackage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The proto package containing the API definition for which this
* GAPIC library was generated.
*
*
* string proto_package = 4;
* @param value The protoPackage to set.
* @return This builder for chaining.
*/
public Builder setProtoPackage(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
protoPackage_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The proto package containing the API definition for which this
* GAPIC library was generated.
*
*
* string proto_package = 4;
* @return This builder for chaining.
*/
public Builder clearProtoPackage() {
protoPackage_ = getDefaultInstance().getProtoPackage();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
*
* The proto package containing the API definition for which this
* GAPIC library was generated.
*
*
* string proto_package = 4;
* @param value The bytes for protoPackage to set.
* @return This builder for chaining.
*/
public Builder setProtoPackageBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
protoPackage_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private java.lang.Object libraryPackage_ = "";
/**
*
* The language-specific library package for this GAPIC library.
*
*
* string library_package = 5;
* @return The libraryPackage.
*/
public java.lang.String getLibraryPackage() {
java.lang.Object ref = libraryPackage_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
libraryPackage_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The language-specific library package for this GAPIC library.
*
*
* string library_package = 5;
* @return The bytes for libraryPackage.
*/
public com.google.protobuf.ByteString
getLibraryPackageBytes() {
java.lang.Object ref = libraryPackage_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
libraryPackage_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The language-specific library package for this GAPIC library.
*
*
* string library_package = 5;
* @param value The libraryPackage to set.
* @return This builder for chaining.
*/
public Builder setLibraryPackage(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
libraryPackage_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The language-specific library package for this GAPIC library.
*
*
* string library_package = 5;
* @return This builder for chaining.
*/
public Builder clearLibraryPackage() {
libraryPackage_ = getDefaultInstance().getLibraryPackage();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
* The language-specific library package for this GAPIC library.
*
*
* string library_package = 5;
* @param value The bytes for libraryPackage to set.
* @return This builder for chaining.
*/
public Builder setLibraryPackageBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
libraryPackage_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private static final class ServicesConverter implements com.google.protobuf.MapFieldBuilder.Converter {
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceForTransport build(com.google.gapic.metadata.GapicMetadata.ServiceForTransportOrBuilder val) {
if (val instanceof com.google.gapic.metadata.GapicMetadata.ServiceForTransport) { return (com.google.gapic.metadata.GapicMetadata.ServiceForTransport) val; }
return ((com.google.gapic.metadata.GapicMetadata.ServiceForTransport.Builder) val).build();
}
@java.lang.Override
public com.google.protobuf.MapEntry defaultEntry() {
return ServicesDefaultEntryHolder.defaultEntry;
}
};
private static final ServicesConverter servicesConverter = new ServicesConverter();
private com.google.protobuf.MapFieldBuilder<
java.lang.String, com.google.gapic.metadata.GapicMetadata.ServiceForTransportOrBuilder, com.google.gapic.metadata.GapicMetadata.ServiceForTransport, com.google.gapic.metadata.GapicMetadata.ServiceForTransport.Builder> services_;
private com.google.protobuf.MapFieldBuilder
internalGetServices() {
if (services_ == null) {
return new com.google.protobuf.MapFieldBuilder<>(servicesConverter);
}
return services_;
}
private com.google.protobuf.MapFieldBuilder
internalGetMutableServices() {
if (services_ == null) {
services_ = new com.google.protobuf.MapFieldBuilder<>(servicesConverter);
}
bitField0_ |= 0x00000020;
onChanged();
return services_;
}
public int getServicesCount() {
return internalGetServices().ensureBuilderMap().size();
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
@java.lang.Override
public boolean containsServices(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
return internalGetServices().ensureBuilderMap().containsKey(key);
}
/**
* Use {@link #getServicesMap()} instead.
*/
@java.lang.Override
@java.lang.Deprecated
public java.util.Map getServices() {
return getServicesMap();
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
@java.lang.Override
public java.util.Map getServicesMap() {
return internalGetServices().getImmutableMap();
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
@java.lang.Override
public /* nullable */
com.google.gapic.metadata.GapicMetadata.ServiceForTransport getServicesOrDefault(
java.lang.String key,
/* nullable */
com.google.gapic.metadata.GapicMetadata.ServiceForTransport defaultValue) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map = internalGetMutableServices().ensureBuilderMap();
return map.containsKey(key) ? servicesConverter.build(map.get(key)) : defaultValue;
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata.ServiceForTransport getServicesOrThrow(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
java.util.Map map = internalGetMutableServices().ensureBuilderMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return servicesConverter.build(map.get(key));
}
public Builder clearServices() {
bitField0_ = (bitField0_ & ~0x00000020);
internalGetMutableServices().clear();
return this;
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
public Builder removeServices(
java.lang.String key) {
if (key == null) { throw new NullPointerException("map key"); }
internalGetMutableServices().ensureBuilderMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableServices() {
bitField0_ |= 0x00000020;
return internalGetMutableServices().ensureMessageMap();
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
public Builder putServices(
java.lang.String key,
com.google.gapic.metadata.GapicMetadata.ServiceForTransport value) {
if (key == null) { throw new NullPointerException("map key"); }
if (value == null) { throw new NullPointerException("map value"); }
internalGetMutableServices().ensureBuilderMap()
.put(key, value);
bitField0_ |= 0x00000020;
return this;
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
public Builder putAllServices(
java.util.Map values) {
for (java.util.Map.Entry e : values.entrySet()) {
if (e.getKey() == null || e.getValue() == null) {
throw new NullPointerException();
}
}
internalGetMutableServices().ensureBuilderMap()
.putAll(values);
bitField0_ |= 0x00000020;
return this;
}
/**
*
* A map from each proto-defined service to ServiceForTransports,
* which allows listing information about transport-specific
* implementations of the service.
*
* The key is the name of the service as it appears in the .proto
* file.
*
*
* map<string, .google.gapic.metadata.GapicMetadata.ServiceForTransport> services = 6;
*/
public com.google.gapic.metadata.GapicMetadata.ServiceForTransport.Builder putServicesBuilderIfAbsent(
java.lang.String key) {
java.util.Map builderMap = internalGetMutableServices().ensureBuilderMap();
com.google.gapic.metadata.GapicMetadata.ServiceForTransportOrBuilder entry = builderMap.get(key);
if (entry == null) {
entry = com.google.gapic.metadata.GapicMetadata.ServiceForTransport.newBuilder();
builderMap.put(key, entry);
}
if (entry instanceof com.google.gapic.metadata.GapicMetadata.ServiceForTransport) {
entry = ((com.google.gapic.metadata.GapicMetadata.ServiceForTransport) entry).toBuilder();
builderMap.put(key, entry);
}
return (com.google.gapic.metadata.GapicMetadata.ServiceForTransport.Builder) entry;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:google.gapic.metadata.GapicMetadata)
}
// @@protoc_insertion_point(class_scope:google.gapic.metadata.GapicMetadata)
private static final com.google.gapic.metadata.GapicMetadata DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.gapic.metadata.GapicMetadata();
}
public static com.google.gapic.metadata.GapicMetadata getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GapicMetadata parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.gapic.metadata.GapicMetadata getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy