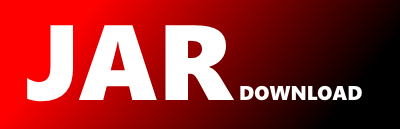
io.grpc.serviceconfig.MethodConfig Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: service_config.proto
// Protobuf Java Version: 3.25.4
package io.grpc.serviceconfig;
/**
*
* Configuration for a method.
*
*
* Protobuf type {@code grpc.service_config.MethodConfig}
*/
public final class MethodConfig extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:grpc.service_config.MethodConfig)
MethodConfigOrBuilder {
private static final long serialVersionUID = 0L;
// Use MethodConfig.newBuilder() to construct.
private MethodConfig(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MethodConfig() {
name_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MethodConfig();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.serviceconfig.MethodConfig.class, io.grpc.serviceconfig.MethodConfig.Builder.class);
}
public interface NameOrBuilder extends
// @@protoc_insertion_point(interface_extends:grpc.service_config.MethodConfig.Name)
com.google.protobuf.MessageOrBuilder {
/**
*
* Required. Includes proto package name.
*
*
* string service = 1;
* @return The service.
*/
java.lang.String getService();
/**
*
* Required. Includes proto package name.
*
*
* string service = 1;
* @return The bytes for service.
*/
com.google.protobuf.ByteString
getServiceBytes();
/**
* string method = 2;
* @return The method.
*/
java.lang.String getMethod();
/**
* string method = 2;
* @return The bytes for method.
*/
com.google.protobuf.ByteString
getMethodBytes();
}
/**
*
* The names of the methods to which this configuration applies.
* - MethodConfig without names (empty list) will be skipped.
* - Each name entry must be unique across the entire ServiceConfig.
* - If the 'method' field is empty, this MethodConfig specifies the defaults
* for all methods for the specified service.
* - If the 'service' field is empty, the 'method' field must be empty, and
* this MethodConfig specifies the default for all methods (it's the default
* config).
*
* When determining which MethodConfig to use for a given RPC, the most
* specific match wins. For example, let's say that the service config
* contains the following MethodConfig entries:
*
* method_config { name { } ... }
* method_config { name { service: "MyService" } ... }
* method_config { name { service: "MyService" method: "Foo" } ... }
*
* MyService/Foo will use the third entry, because it exactly matches the
* service and method name. MyService/Bar will use the second entry, because
* it provides the default for all methods of MyService. AnotherService/Baz
* will use the first entry, because it doesn't match the other two.
*
* In JSON representation, value "", value `null`, and not present are the
* same. The following are the same Name:
* - { "service": "s" }
* - { "service": "s", "method": null }
* - { "service": "s", "method": "" }
*
*
* Protobuf type {@code grpc.service_config.MethodConfig.Name}
*/
public static final class Name extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:grpc.service_config.MethodConfig.Name)
NameOrBuilder {
private static final long serialVersionUID = 0L;
// Use Name.newBuilder() to construct.
private Name(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Name() {
service_ = "";
method_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Name();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_Name_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_Name_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.serviceconfig.MethodConfig.Name.class, io.grpc.serviceconfig.MethodConfig.Name.Builder.class);
}
public static final int SERVICE_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object service_ = "";
/**
*
* Required. Includes proto package name.
*
*
* string service = 1;
* @return The service.
*/
@java.lang.Override
public java.lang.String getService() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
service_ = s;
return s;
}
}
/**
*
* Required. Includes proto package name.
*
*
* string service = 1;
* @return The bytes for service.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int METHOD_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object method_ = "";
/**
* string method = 2;
* @return The method.
*/
@java.lang.Override
public java.lang.String getMethod() {
java.lang.Object ref = method_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
method_ = s;
return s;
}
}
/**
* string method = 2;
* @return The bytes for method.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMethodBytes() {
java.lang.Object ref = method_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
method_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(service_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, service_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(method_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, method_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(service_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, service_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(method_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, method_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.grpc.serviceconfig.MethodConfig.Name)) {
return super.equals(obj);
}
io.grpc.serviceconfig.MethodConfig.Name other = (io.grpc.serviceconfig.MethodConfig.Name) obj;
if (!getService()
.equals(other.getService())) return false;
if (!getMethod()
.equals(other.getMethod())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SERVICE_FIELD_NUMBER;
hash = (53 * hash) + getService().hashCode();
hash = (37 * hash) + METHOD_FIELD_NUMBER;
hash = (53 * hash) + getMethod().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.grpc.serviceconfig.MethodConfig.Name parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig.Name parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.Name parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig.Name parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.Name parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig.Name parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.Name parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig.Name parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.Name parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig.Name parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.Name parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig.Name parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.grpc.serviceconfig.MethodConfig.Name prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The names of the methods to which this configuration applies.
* - MethodConfig without names (empty list) will be skipped.
* - Each name entry must be unique across the entire ServiceConfig.
* - If the 'method' field is empty, this MethodConfig specifies the defaults
* for all methods for the specified service.
* - If the 'service' field is empty, the 'method' field must be empty, and
* this MethodConfig specifies the default for all methods (it's the default
* config).
*
* When determining which MethodConfig to use for a given RPC, the most
* specific match wins. For example, let's say that the service config
* contains the following MethodConfig entries:
*
* method_config { name { } ... }
* method_config { name { service: "MyService" } ... }
* method_config { name { service: "MyService" method: "Foo" } ... }
*
* MyService/Foo will use the third entry, because it exactly matches the
* service and method name. MyService/Bar will use the second entry, because
* it provides the default for all methods of MyService. AnotherService/Baz
* will use the first entry, because it doesn't match the other two.
*
* In JSON representation, value "", value `null`, and not present are the
* same. The following are the same Name:
* - { "service": "s" }
* - { "service": "s", "method": null }
* - { "service": "s", "method": "" }
*
*
* Protobuf type {@code grpc.service_config.MethodConfig.Name}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:grpc.service_config.MethodConfig.Name)
io.grpc.serviceconfig.MethodConfig.NameOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_Name_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_Name_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.serviceconfig.MethodConfig.Name.class, io.grpc.serviceconfig.MethodConfig.Name.Builder.class);
}
// Construct using io.grpc.serviceconfig.MethodConfig.Name.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
service_ = "";
method_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_Name_descriptor;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.Name getDefaultInstanceForType() {
return io.grpc.serviceconfig.MethodConfig.Name.getDefaultInstance();
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.Name build() {
io.grpc.serviceconfig.MethodConfig.Name result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.Name buildPartial() {
io.grpc.serviceconfig.MethodConfig.Name result = new io.grpc.serviceconfig.MethodConfig.Name(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(io.grpc.serviceconfig.MethodConfig.Name result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.service_ = service_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.method_ = method_;
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.grpc.serviceconfig.MethodConfig.Name) {
return mergeFrom((io.grpc.serviceconfig.MethodConfig.Name)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.grpc.serviceconfig.MethodConfig.Name other) {
if (other == io.grpc.serviceconfig.MethodConfig.Name.getDefaultInstance()) return this;
if (!other.getService().isEmpty()) {
service_ = other.service_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getMethod().isEmpty()) {
method_ = other.method_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
service_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
method_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object service_ = "";
/**
*
* Required. Includes proto package name.
*
*
* string service = 1;
* @return The service.
*/
public java.lang.String getService() {
java.lang.Object ref = service_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
service_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Required. Includes proto package name.
*
*
* string service = 1;
* @return The bytes for service.
*/
public com.google.protobuf.ByteString
getServiceBytes() {
java.lang.Object ref = service_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
service_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Required. Includes proto package name.
*
*
* string service = 1;
* @param value The service to set.
* @return This builder for chaining.
*/
public Builder setService(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
service_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Required. Includes proto package name.
*
*
* string service = 1;
* @return This builder for chaining.
*/
public Builder clearService() {
service_ = getDefaultInstance().getService();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Required. Includes proto package name.
*
*
* string service = 1;
* @param value The bytes for service to set.
* @return This builder for chaining.
*/
public Builder setServiceBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
service_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object method_ = "";
/**
* string method = 2;
* @return The method.
*/
public java.lang.String getMethod() {
java.lang.Object ref = method_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
method_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string method = 2;
* @return The bytes for method.
*/
public com.google.protobuf.ByteString
getMethodBytes() {
java.lang.Object ref = method_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
method_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string method = 2;
* @param value The method to set.
* @return This builder for chaining.
*/
public Builder setMethod(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
method_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string method = 2;
* @return This builder for chaining.
*/
public Builder clearMethod() {
method_ = getDefaultInstance().getMethod();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string method = 2;
* @param value The bytes for method to set.
* @return This builder for chaining.
*/
public Builder setMethodBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
method_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:grpc.service_config.MethodConfig.Name)
}
// @@protoc_insertion_point(class_scope:grpc.service_config.MethodConfig.Name)
private static final io.grpc.serviceconfig.MethodConfig.Name DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.grpc.serviceconfig.MethodConfig.Name();
}
public static io.grpc.serviceconfig.MethodConfig.Name getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Name parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.Name getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface RetryPolicyOrBuilder extends
// @@protoc_insertion_point(interface_extends:grpc.service_config.MethodConfig.RetryPolicy)
com.google.protobuf.MessageOrBuilder {
/**
*
* The maximum number of RPC attempts, including the original attempt.
*
* This field is required and must be greater than 1.
* Any value greater than 5 will be treated as if it were 5.
*
*
* uint32 max_attempts = 1;
* @return The maxAttempts.
*/
int getMaxAttempts();
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
* @return Whether the initialBackoff field is set.
*/
boolean hasInitialBackoff();
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
* @return The initialBackoff.
*/
com.google.protobuf.Duration getInitialBackoff();
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
*/
com.google.protobuf.DurationOrBuilder getInitialBackoffOrBuilder();
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
* @return Whether the maxBackoff field is set.
*/
boolean hasMaxBackoff();
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
* @return The maxBackoff.
*/
com.google.protobuf.Duration getMaxBackoff();
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
*/
com.google.protobuf.DurationOrBuilder getMaxBackoffOrBuilder();
/**
*
* Required. Must be greater than zero.
*
*
* float backoff_multiplier = 4;
* @return The backoffMultiplier.
*/
float getBackoffMultiplier();
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @return A list containing the retryableStatusCodes.
*/
java.util.List getRetryableStatusCodesList();
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @return The count of retryableStatusCodes.
*/
int getRetryableStatusCodesCount();
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param index The index of the element to return.
* @return The retryableStatusCodes at the given index.
*/
com.google.rpc.Code getRetryableStatusCodes(int index);
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @return A list containing the enum numeric values on the wire for retryableStatusCodes.
*/
java.util.List
getRetryableStatusCodesValueList();
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of retryableStatusCodes at the given index.
*/
int getRetryableStatusCodesValue(int index);
}
/**
*
* The retry policy for outgoing RPCs.
*
*
* Protobuf type {@code grpc.service_config.MethodConfig.RetryPolicy}
*/
public static final class RetryPolicy extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:grpc.service_config.MethodConfig.RetryPolicy)
RetryPolicyOrBuilder {
private static final long serialVersionUID = 0L;
// Use RetryPolicy.newBuilder() to construct.
private RetryPolicy(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RetryPolicy() {
retryableStatusCodes_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RetryPolicy();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_RetryPolicy_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_RetryPolicy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.serviceconfig.MethodConfig.RetryPolicy.class, io.grpc.serviceconfig.MethodConfig.RetryPolicy.Builder.class);
}
private int bitField0_;
public static final int MAX_ATTEMPTS_FIELD_NUMBER = 1;
private int maxAttempts_ = 0;
/**
*
* The maximum number of RPC attempts, including the original attempt.
*
* This field is required and must be greater than 1.
* Any value greater than 5 will be treated as if it were 5.
*
*
* uint32 max_attempts = 1;
* @return The maxAttempts.
*/
@java.lang.Override
public int getMaxAttempts() {
return maxAttempts_;
}
public static final int INITIAL_BACKOFF_FIELD_NUMBER = 2;
private com.google.protobuf.Duration initialBackoff_;
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
* @return Whether the initialBackoff field is set.
*/
@java.lang.Override
public boolean hasInitialBackoff() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
* @return The initialBackoff.
*/
@java.lang.Override
public com.google.protobuf.Duration getInitialBackoff() {
return initialBackoff_ == null ? com.google.protobuf.Duration.getDefaultInstance() : initialBackoff_;
}
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getInitialBackoffOrBuilder() {
return initialBackoff_ == null ? com.google.protobuf.Duration.getDefaultInstance() : initialBackoff_;
}
public static final int MAX_BACKOFF_FIELD_NUMBER = 3;
private com.google.protobuf.Duration maxBackoff_;
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
* @return Whether the maxBackoff field is set.
*/
@java.lang.Override
public boolean hasMaxBackoff() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
* @return The maxBackoff.
*/
@java.lang.Override
public com.google.protobuf.Duration getMaxBackoff() {
return maxBackoff_ == null ? com.google.protobuf.Duration.getDefaultInstance() : maxBackoff_;
}
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getMaxBackoffOrBuilder() {
return maxBackoff_ == null ? com.google.protobuf.Duration.getDefaultInstance() : maxBackoff_;
}
public static final int BACKOFF_MULTIPLIER_FIELD_NUMBER = 4;
private float backoffMultiplier_ = 0F;
/**
*
* Required. Must be greater than zero.
*
*
* float backoff_multiplier = 4;
* @return The backoffMultiplier.
*/
@java.lang.Override
public float getBackoffMultiplier() {
return backoffMultiplier_;
}
public static final int RETRYABLE_STATUS_CODES_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private java.util.List retryableStatusCodes_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.rpc.Code> retryableStatusCodes_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.rpc.Code>() {
public com.google.rpc.Code convert(java.lang.Integer from) {
com.google.rpc.Code result = com.google.rpc.Code.forNumber(from);
return result == null ? com.google.rpc.Code.UNRECOGNIZED : result;
}
};
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @return A list containing the retryableStatusCodes.
*/
@java.lang.Override
public java.util.List getRetryableStatusCodesList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.rpc.Code>(retryableStatusCodes_, retryableStatusCodes_converter_);
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @return The count of retryableStatusCodes.
*/
@java.lang.Override
public int getRetryableStatusCodesCount() {
return retryableStatusCodes_.size();
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param index The index of the element to return.
* @return The retryableStatusCodes at the given index.
*/
@java.lang.Override
public com.google.rpc.Code getRetryableStatusCodes(int index) {
return retryableStatusCodes_converter_.convert(retryableStatusCodes_.get(index));
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @return A list containing the enum numeric values on the wire for retryableStatusCodes.
*/
@java.lang.Override
public java.util.List
getRetryableStatusCodesValueList() {
return retryableStatusCodes_;
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of retryableStatusCodes at the given index.
*/
@java.lang.Override
public int getRetryableStatusCodesValue(int index) {
return retryableStatusCodes_.get(index);
}
private int retryableStatusCodesMemoizedSerializedSize;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (maxAttempts_ != 0) {
output.writeUInt32(1, maxAttempts_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(2, getInitialBackoff());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(3, getMaxBackoff());
}
if (java.lang.Float.floatToRawIntBits(backoffMultiplier_) != 0) {
output.writeFloat(4, backoffMultiplier_);
}
if (getRetryableStatusCodesList().size() > 0) {
output.writeUInt32NoTag(42);
output.writeUInt32NoTag(retryableStatusCodesMemoizedSerializedSize);
}
for (int i = 0; i < retryableStatusCodes_.size(); i++) {
output.writeEnumNoTag(retryableStatusCodes_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (maxAttempts_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, maxAttempts_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getInitialBackoff());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getMaxBackoff());
}
if (java.lang.Float.floatToRawIntBits(backoffMultiplier_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(4, backoffMultiplier_);
}
{
int dataSize = 0;
for (int i = 0; i < retryableStatusCodes_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(retryableStatusCodes_.get(i));
}
size += dataSize;
if (!getRetryableStatusCodesList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}retryableStatusCodesMemoizedSerializedSize = dataSize;
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.grpc.serviceconfig.MethodConfig.RetryPolicy)) {
return super.equals(obj);
}
io.grpc.serviceconfig.MethodConfig.RetryPolicy other = (io.grpc.serviceconfig.MethodConfig.RetryPolicy) obj;
if (getMaxAttempts()
!= other.getMaxAttempts()) return false;
if (hasInitialBackoff() != other.hasInitialBackoff()) return false;
if (hasInitialBackoff()) {
if (!getInitialBackoff()
.equals(other.getInitialBackoff())) return false;
}
if (hasMaxBackoff() != other.hasMaxBackoff()) return false;
if (hasMaxBackoff()) {
if (!getMaxBackoff()
.equals(other.getMaxBackoff())) return false;
}
if (java.lang.Float.floatToIntBits(getBackoffMultiplier())
!= java.lang.Float.floatToIntBits(
other.getBackoffMultiplier())) return false;
if (!retryableStatusCodes_.equals(other.retryableStatusCodes_)) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MAX_ATTEMPTS_FIELD_NUMBER;
hash = (53 * hash) + getMaxAttempts();
if (hasInitialBackoff()) {
hash = (37 * hash) + INITIAL_BACKOFF_FIELD_NUMBER;
hash = (53 * hash) + getInitialBackoff().hashCode();
}
if (hasMaxBackoff()) {
hash = (37 * hash) + MAX_BACKOFF_FIELD_NUMBER;
hash = (53 * hash) + getMaxBackoff().hashCode();
}
hash = (37 * hash) + BACKOFF_MULTIPLIER_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getBackoffMultiplier());
if (getRetryableStatusCodesCount() > 0) {
hash = (37 * hash) + RETRYABLE_STATUS_CODES_FIELD_NUMBER;
hash = (53 * hash) + retryableStatusCodes_.hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.grpc.serviceconfig.MethodConfig.RetryPolicy prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The retry policy for outgoing RPCs.
*
*
* Protobuf type {@code grpc.service_config.MethodConfig.RetryPolicy}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:grpc.service_config.MethodConfig.RetryPolicy)
io.grpc.serviceconfig.MethodConfig.RetryPolicyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_RetryPolicy_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_RetryPolicy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.serviceconfig.MethodConfig.RetryPolicy.class, io.grpc.serviceconfig.MethodConfig.RetryPolicy.Builder.class);
}
// Construct using io.grpc.serviceconfig.MethodConfig.RetryPolicy.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getInitialBackoffFieldBuilder();
getMaxBackoffFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
maxAttempts_ = 0;
initialBackoff_ = null;
if (initialBackoffBuilder_ != null) {
initialBackoffBuilder_.dispose();
initialBackoffBuilder_ = null;
}
maxBackoff_ = null;
if (maxBackoffBuilder_ != null) {
maxBackoffBuilder_.dispose();
maxBackoffBuilder_ = null;
}
backoffMultiplier_ = 0F;
retryableStatusCodes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_RetryPolicy_descriptor;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.RetryPolicy getDefaultInstanceForType() {
return io.grpc.serviceconfig.MethodConfig.RetryPolicy.getDefaultInstance();
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.RetryPolicy build() {
io.grpc.serviceconfig.MethodConfig.RetryPolicy result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.RetryPolicy buildPartial() {
io.grpc.serviceconfig.MethodConfig.RetryPolicy result = new io.grpc.serviceconfig.MethodConfig.RetryPolicy(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(io.grpc.serviceconfig.MethodConfig.RetryPolicy result) {
if (((bitField0_ & 0x00000010) != 0)) {
retryableStatusCodes_ = java.util.Collections.unmodifiableList(retryableStatusCodes_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.retryableStatusCodes_ = retryableStatusCodes_;
}
private void buildPartial0(io.grpc.serviceconfig.MethodConfig.RetryPolicy result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.maxAttempts_ = maxAttempts_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.initialBackoff_ = initialBackoffBuilder_ == null
? initialBackoff_
: initialBackoffBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.maxBackoff_ = maxBackoffBuilder_ == null
? maxBackoff_
: maxBackoffBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.backoffMultiplier_ = backoffMultiplier_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.grpc.serviceconfig.MethodConfig.RetryPolicy) {
return mergeFrom((io.grpc.serviceconfig.MethodConfig.RetryPolicy)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.grpc.serviceconfig.MethodConfig.RetryPolicy other) {
if (other == io.grpc.serviceconfig.MethodConfig.RetryPolicy.getDefaultInstance()) return this;
if (other.getMaxAttempts() != 0) {
setMaxAttempts(other.getMaxAttempts());
}
if (other.hasInitialBackoff()) {
mergeInitialBackoff(other.getInitialBackoff());
}
if (other.hasMaxBackoff()) {
mergeMaxBackoff(other.getMaxBackoff());
}
if (other.getBackoffMultiplier() != 0F) {
setBackoffMultiplier(other.getBackoffMultiplier());
}
if (!other.retryableStatusCodes_.isEmpty()) {
if (retryableStatusCodes_.isEmpty()) {
retryableStatusCodes_ = other.retryableStatusCodes_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureRetryableStatusCodesIsMutable();
retryableStatusCodes_.addAll(other.retryableStatusCodes_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
maxAttempts_ = input.readUInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
input.readMessage(
getInitialBackoffFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
input.readMessage(
getMaxBackoffFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 37: {
backoffMultiplier_ = input.readFloat();
bitField0_ |= 0x00000008;
break;
} // case 37
case 40: {
int tmpRaw = input.readEnum();
ensureRetryableStatusCodesIsMutable();
retryableStatusCodes_.add(tmpRaw);
break;
} // case 40
case 42: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int tmpRaw = input.readEnum();
ensureRetryableStatusCodesIsMutable();
retryableStatusCodes_.add(tmpRaw);
}
input.popLimit(oldLimit);
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int maxAttempts_ ;
/**
*
* The maximum number of RPC attempts, including the original attempt.
*
* This field is required and must be greater than 1.
* Any value greater than 5 will be treated as if it were 5.
*
*
* uint32 max_attempts = 1;
* @return The maxAttempts.
*/
@java.lang.Override
public int getMaxAttempts() {
return maxAttempts_;
}
/**
*
* The maximum number of RPC attempts, including the original attempt.
*
* This field is required and must be greater than 1.
* Any value greater than 5 will be treated as if it were 5.
*
*
* uint32 max_attempts = 1;
* @param value The maxAttempts to set.
* @return This builder for chaining.
*/
public Builder setMaxAttempts(int value) {
maxAttempts_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The maximum number of RPC attempts, including the original attempt.
*
* This field is required and must be greater than 1.
* Any value greater than 5 will be treated as if it were 5.
*
*
* uint32 max_attempts = 1;
* @return This builder for chaining.
*/
public Builder clearMaxAttempts() {
bitField0_ = (bitField0_ & ~0x00000001);
maxAttempts_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Duration initialBackoff_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> initialBackoffBuilder_;
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
* @return Whether the initialBackoff field is set.
*/
public boolean hasInitialBackoff() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
* @return The initialBackoff.
*/
public com.google.protobuf.Duration getInitialBackoff() {
if (initialBackoffBuilder_ == null) {
return initialBackoff_ == null ? com.google.protobuf.Duration.getDefaultInstance() : initialBackoff_;
} else {
return initialBackoffBuilder_.getMessage();
}
}
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
*/
public Builder setInitialBackoff(com.google.protobuf.Duration value) {
if (initialBackoffBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
initialBackoff_ = value;
} else {
initialBackoffBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
*/
public Builder setInitialBackoff(
com.google.protobuf.Duration.Builder builderForValue) {
if (initialBackoffBuilder_ == null) {
initialBackoff_ = builderForValue.build();
} else {
initialBackoffBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
*/
public Builder mergeInitialBackoff(com.google.protobuf.Duration value) {
if (initialBackoffBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
initialBackoff_ != null &&
initialBackoff_ != com.google.protobuf.Duration.getDefaultInstance()) {
getInitialBackoffBuilder().mergeFrom(value);
} else {
initialBackoff_ = value;
}
} else {
initialBackoffBuilder_.mergeFrom(value);
}
if (initialBackoff_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
*/
public Builder clearInitialBackoff() {
bitField0_ = (bitField0_ & ~0x00000002);
initialBackoff_ = null;
if (initialBackoffBuilder_ != null) {
initialBackoffBuilder_.dispose();
initialBackoffBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
*/
public com.google.protobuf.Duration.Builder getInitialBackoffBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getInitialBackoffFieldBuilder().getBuilder();
}
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
*/
public com.google.protobuf.DurationOrBuilder getInitialBackoffOrBuilder() {
if (initialBackoffBuilder_ != null) {
return initialBackoffBuilder_.getMessageOrBuilder();
} else {
return initialBackoff_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : initialBackoff_;
}
}
/**
*
* Exponential backoff parameters. The initial retry attempt will occur at
* random(0, initial_backoff). In general, the nth attempt will occur at
* random(0,
* min(initial_backoff*backoff_multiplier**(n-1), max_backoff)).
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration initial_backoff = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getInitialBackoffFieldBuilder() {
if (initialBackoffBuilder_ == null) {
initialBackoffBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getInitialBackoff(),
getParentForChildren(),
isClean());
initialBackoff_ = null;
}
return initialBackoffBuilder_;
}
private com.google.protobuf.Duration maxBackoff_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> maxBackoffBuilder_;
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
* @return Whether the maxBackoff field is set.
*/
public boolean hasMaxBackoff() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
* @return The maxBackoff.
*/
public com.google.protobuf.Duration getMaxBackoff() {
if (maxBackoffBuilder_ == null) {
return maxBackoff_ == null ? com.google.protobuf.Duration.getDefaultInstance() : maxBackoff_;
} else {
return maxBackoffBuilder_.getMessage();
}
}
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
*/
public Builder setMaxBackoff(com.google.protobuf.Duration value) {
if (maxBackoffBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maxBackoff_ = value;
} else {
maxBackoffBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
*/
public Builder setMaxBackoff(
com.google.protobuf.Duration.Builder builderForValue) {
if (maxBackoffBuilder_ == null) {
maxBackoff_ = builderForValue.build();
} else {
maxBackoffBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
*/
public Builder mergeMaxBackoff(com.google.protobuf.Duration value) {
if (maxBackoffBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
maxBackoff_ != null &&
maxBackoff_ != com.google.protobuf.Duration.getDefaultInstance()) {
getMaxBackoffBuilder().mergeFrom(value);
} else {
maxBackoff_ = value;
}
} else {
maxBackoffBuilder_.mergeFrom(value);
}
if (maxBackoff_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
*/
public Builder clearMaxBackoff() {
bitField0_ = (bitField0_ & ~0x00000004);
maxBackoff_ = null;
if (maxBackoffBuilder_ != null) {
maxBackoffBuilder_.dispose();
maxBackoffBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
*/
public com.google.protobuf.Duration.Builder getMaxBackoffBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getMaxBackoffFieldBuilder().getBuilder();
}
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
*/
public com.google.protobuf.DurationOrBuilder getMaxBackoffOrBuilder() {
if (maxBackoffBuilder_ != null) {
return maxBackoffBuilder_.getMessageOrBuilder();
} else {
return maxBackoff_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : maxBackoff_;
}
}
/**
*
* Required. Must be greater than zero.
*
*
* .google.protobuf.Duration max_backoff = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getMaxBackoffFieldBuilder() {
if (maxBackoffBuilder_ == null) {
maxBackoffBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getMaxBackoff(),
getParentForChildren(),
isClean());
maxBackoff_ = null;
}
return maxBackoffBuilder_;
}
private float backoffMultiplier_ ;
/**
*
* Required. Must be greater than zero.
*
*
* float backoff_multiplier = 4;
* @return The backoffMultiplier.
*/
@java.lang.Override
public float getBackoffMultiplier() {
return backoffMultiplier_;
}
/**
*
* Required. Must be greater than zero.
*
*
* float backoff_multiplier = 4;
* @param value The backoffMultiplier to set.
* @return This builder for chaining.
*/
public Builder setBackoffMultiplier(float value) {
backoffMultiplier_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Required. Must be greater than zero.
*
*
* float backoff_multiplier = 4;
* @return This builder for chaining.
*/
public Builder clearBackoffMultiplier() {
bitField0_ = (bitField0_ & ~0x00000008);
backoffMultiplier_ = 0F;
onChanged();
return this;
}
private java.util.List retryableStatusCodes_ =
java.util.Collections.emptyList();
private void ensureRetryableStatusCodesIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
retryableStatusCodes_ = new java.util.ArrayList(retryableStatusCodes_);
bitField0_ |= 0x00000010;
}
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @return A list containing the retryableStatusCodes.
*/
public java.util.List getRetryableStatusCodesList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.rpc.Code>(retryableStatusCodes_, retryableStatusCodes_converter_);
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @return The count of retryableStatusCodes.
*/
public int getRetryableStatusCodesCount() {
return retryableStatusCodes_.size();
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param index The index of the element to return.
* @return The retryableStatusCodes at the given index.
*/
public com.google.rpc.Code getRetryableStatusCodes(int index) {
return retryableStatusCodes_converter_.convert(retryableStatusCodes_.get(index));
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param index The index to set the value at.
* @param value The retryableStatusCodes to set.
* @return This builder for chaining.
*/
public Builder setRetryableStatusCodes(
int index, com.google.rpc.Code value) {
if (value == null) {
throw new NullPointerException();
}
ensureRetryableStatusCodesIsMutable();
retryableStatusCodes_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param value The retryableStatusCodes to add.
* @return This builder for chaining.
*/
public Builder addRetryableStatusCodes(com.google.rpc.Code value) {
if (value == null) {
throw new NullPointerException();
}
ensureRetryableStatusCodesIsMutable();
retryableStatusCodes_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param values The retryableStatusCodes to add.
* @return This builder for chaining.
*/
public Builder addAllRetryableStatusCodes(
java.lang.Iterable extends com.google.rpc.Code> values) {
ensureRetryableStatusCodesIsMutable();
for (com.google.rpc.Code value : values) {
retryableStatusCodes_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @return This builder for chaining.
*/
public Builder clearRetryableStatusCodes() {
retryableStatusCodes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @return A list containing the enum numeric values on the wire for retryableStatusCodes.
*/
public java.util.List
getRetryableStatusCodesValueList() {
return java.util.Collections.unmodifiableList(retryableStatusCodes_);
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of retryableStatusCodes at the given index.
*/
public int getRetryableStatusCodesValue(int index) {
return retryableStatusCodes_.get(index);
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param index The index to set the value at.
* @param value The enum numeric value on the wire for retryableStatusCodes to set.
* @return This builder for chaining.
*/
public Builder setRetryableStatusCodesValue(
int index, int value) {
ensureRetryableStatusCodesIsMutable();
retryableStatusCodes_.set(index, value);
onChanged();
return this;
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param value The enum numeric value on the wire for retryableStatusCodes to add.
* @return This builder for chaining.
*/
public Builder addRetryableStatusCodesValue(int value) {
ensureRetryableStatusCodesIsMutable();
retryableStatusCodes_.add(value);
onChanged();
return this;
}
/**
*
* The set of status codes which may be retried.
*
* This field is required and must be non-empty.
*
*
* repeated .google.rpc.Code retryable_status_codes = 5;
* @param values The enum numeric values on the wire for retryableStatusCodes to add.
* @return This builder for chaining.
*/
public Builder addAllRetryableStatusCodesValue(
java.lang.Iterable values) {
ensureRetryableStatusCodesIsMutable();
for (int value : values) {
retryableStatusCodes_.add(value);
}
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:grpc.service_config.MethodConfig.RetryPolicy)
}
// @@protoc_insertion_point(class_scope:grpc.service_config.MethodConfig.RetryPolicy)
private static final io.grpc.serviceconfig.MethodConfig.RetryPolicy DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.grpc.serviceconfig.MethodConfig.RetryPolicy();
}
public static io.grpc.serviceconfig.MethodConfig.RetryPolicy getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RetryPolicy parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.RetryPolicy getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface HedgingPolicyOrBuilder extends
// @@protoc_insertion_point(interface_extends:grpc.service_config.MethodConfig.HedgingPolicy)
com.google.protobuf.MessageOrBuilder {
/**
*
* The hedging policy will send up to max_requests RPCs.
* This number represents the total number of all attempts, including
* the original attempt.
*
* This field is required and must be greater than 1.
* Any value greater than 5 will be treated as if it were 5.
*
*
* uint32 max_attempts = 1;
* @return The maxAttempts.
*/
int getMaxAttempts();
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
* @return Whether the hedgingDelay field is set.
*/
boolean hasHedgingDelay();
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
* @return The hedgingDelay.
*/
com.google.protobuf.Duration getHedgingDelay();
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
*/
com.google.protobuf.DurationOrBuilder getHedgingDelayOrBuilder();
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @return A list containing the nonFatalStatusCodes.
*/
java.util.List getNonFatalStatusCodesList();
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @return The count of nonFatalStatusCodes.
*/
int getNonFatalStatusCodesCount();
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param index The index of the element to return.
* @return The nonFatalStatusCodes at the given index.
*/
com.google.rpc.Code getNonFatalStatusCodes(int index);
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @return A list containing the enum numeric values on the wire for nonFatalStatusCodes.
*/
java.util.List
getNonFatalStatusCodesValueList();
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of nonFatalStatusCodes at the given index.
*/
int getNonFatalStatusCodesValue(int index);
}
/**
*
* The hedging policy for outgoing RPCs. Hedged RPCs may execute more than
* once on the server, so only idempotent methods should specify a hedging
* policy.
*
*
* Protobuf type {@code grpc.service_config.MethodConfig.HedgingPolicy}
*/
public static final class HedgingPolicy extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:grpc.service_config.MethodConfig.HedgingPolicy)
HedgingPolicyOrBuilder {
private static final long serialVersionUID = 0L;
// Use HedgingPolicy.newBuilder() to construct.
private HedgingPolicy(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private HedgingPolicy() {
nonFatalStatusCodes_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new HedgingPolicy();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_HedgingPolicy_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_HedgingPolicy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.serviceconfig.MethodConfig.HedgingPolicy.class, io.grpc.serviceconfig.MethodConfig.HedgingPolicy.Builder.class);
}
private int bitField0_;
public static final int MAX_ATTEMPTS_FIELD_NUMBER = 1;
private int maxAttempts_ = 0;
/**
*
* The hedging policy will send up to max_requests RPCs.
* This number represents the total number of all attempts, including
* the original attempt.
*
* This field is required and must be greater than 1.
* Any value greater than 5 will be treated as if it were 5.
*
*
* uint32 max_attempts = 1;
* @return The maxAttempts.
*/
@java.lang.Override
public int getMaxAttempts() {
return maxAttempts_;
}
public static final int HEDGING_DELAY_FIELD_NUMBER = 2;
private com.google.protobuf.Duration hedgingDelay_;
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
* @return Whether the hedgingDelay field is set.
*/
@java.lang.Override
public boolean hasHedgingDelay() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
* @return The hedgingDelay.
*/
@java.lang.Override
public com.google.protobuf.Duration getHedgingDelay() {
return hedgingDelay_ == null ? com.google.protobuf.Duration.getDefaultInstance() : hedgingDelay_;
}
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getHedgingDelayOrBuilder() {
return hedgingDelay_ == null ? com.google.protobuf.Duration.getDefaultInstance() : hedgingDelay_;
}
public static final int NON_FATAL_STATUS_CODES_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private java.util.List nonFatalStatusCodes_;
private static final com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.rpc.Code> nonFatalStatusCodes_converter_ =
new com.google.protobuf.Internal.ListAdapter.Converter<
java.lang.Integer, com.google.rpc.Code>() {
public com.google.rpc.Code convert(java.lang.Integer from) {
com.google.rpc.Code result = com.google.rpc.Code.forNumber(from);
return result == null ? com.google.rpc.Code.UNRECOGNIZED : result;
}
};
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @return A list containing the nonFatalStatusCodes.
*/
@java.lang.Override
public java.util.List getNonFatalStatusCodesList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.rpc.Code>(nonFatalStatusCodes_, nonFatalStatusCodes_converter_);
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @return The count of nonFatalStatusCodes.
*/
@java.lang.Override
public int getNonFatalStatusCodesCount() {
return nonFatalStatusCodes_.size();
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param index The index of the element to return.
* @return The nonFatalStatusCodes at the given index.
*/
@java.lang.Override
public com.google.rpc.Code getNonFatalStatusCodes(int index) {
return nonFatalStatusCodes_converter_.convert(nonFatalStatusCodes_.get(index));
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @return A list containing the enum numeric values on the wire for nonFatalStatusCodes.
*/
@java.lang.Override
public java.util.List
getNonFatalStatusCodesValueList() {
return nonFatalStatusCodes_;
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of nonFatalStatusCodes at the given index.
*/
@java.lang.Override
public int getNonFatalStatusCodesValue(int index) {
return nonFatalStatusCodes_.get(index);
}
private int nonFatalStatusCodesMemoizedSerializedSize;
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (maxAttempts_ != 0) {
output.writeUInt32(1, maxAttempts_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(2, getHedgingDelay());
}
if (getNonFatalStatusCodesList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(nonFatalStatusCodesMemoizedSerializedSize);
}
for (int i = 0; i < nonFatalStatusCodes_.size(); i++) {
output.writeEnumNoTag(nonFatalStatusCodes_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (maxAttempts_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, maxAttempts_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getHedgingDelay());
}
{
int dataSize = 0;
for (int i = 0; i < nonFatalStatusCodes_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(nonFatalStatusCodes_.get(i));
}
size += dataSize;
if (!getNonFatalStatusCodesList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}nonFatalStatusCodesMemoizedSerializedSize = dataSize;
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.grpc.serviceconfig.MethodConfig.HedgingPolicy)) {
return super.equals(obj);
}
io.grpc.serviceconfig.MethodConfig.HedgingPolicy other = (io.grpc.serviceconfig.MethodConfig.HedgingPolicy) obj;
if (getMaxAttempts()
!= other.getMaxAttempts()) return false;
if (hasHedgingDelay() != other.hasHedgingDelay()) return false;
if (hasHedgingDelay()) {
if (!getHedgingDelay()
.equals(other.getHedgingDelay())) return false;
}
if (!nonFatalStatusCodes_.equals(other.nonFatalStatusCodes_)) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MAX_ATTEMPTS_FIELD_NUMBER;
hash = (53 * hash) + getMaxAttempts();
if (hasHedgingDelay()) {
hash = (37 * hash) + HEDGING_DELAY_FIELD_NUMBER;
hash = (53 * hash) + getHedgingDelay().hashCode();
}
if (getNonFatalStatusCodesCount() > 0) {
hash = (37 * hash) + NON_FATAL_STATUS_CODES_FIELD_NUMBER;
hash = (53 * hash) + nonFatalStatusCodes_.hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.grpc.serviceconfig.MethodConfig.HedgingPolicy prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The hedging policy for outgoing RPCs. Hedged RPCs may execute more than
* once on the server, so only idempotent methods should specify a hedging
* policy.
*
*
* Protobuf type {@code grpc.service_config.MethodConfig.HedgingPolicy}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:grpc.service_config.MethodConfig.HedgingPolicy)
io.grpc.serviceconfig.MethodConfig.HedgingPolicyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_HedgingPolicy_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_HedgingPolicy_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.serviceconfig.MethodConfig.HedgingPolicy.class, io.grpc.serviceconfig.MethodConfig.HedgingPolicy.Builder.class);
}
// Construct using io.grpc.serviceconfig.MethodConfig.HedgingPolicy.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getHedgingDelayFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
maxAttempts_ = 0;
hedgingDelay_ = null;
if (hedgingDelayBuilder_ != null) {
hedgingDelayBuilder_.dispose();
hedgingDelayBuilder_ = null;
}
nonFatalStatusCodes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_HedgingPolicy_descriptor;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.HedgingPolicy getDefaultInstanceForType() {
return io.grpc.serviceconfig.MethodConfig.HedgingPolicy.getDefaultInstance();
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.HedgingPolicy build() {
io.grpc.serviceconfig.MethodConfig.HedgingPolicy result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.HedgingPolicy buildPartial() {
io.grpc.serviceconfig.MethodConfig.HedgingPolicy result = new io.grpc.serviceconfig.MethodConfig.HedgingPolicy(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(io.grpc.serviceconfig.MethodConfig.HedgingPolicy result) {
if (((bitField0_ & 0x00000004) != 0)) {
nonFatalStatusCodes_ = java.util.Collections.unmodifiableList(nonFatalStatusCodes_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.nonFatalStatusCodes_ = nonFatalStatusCodes_;
}
private void buildPartial0(io.grpc.serviceconfig.MethodConfig.HedgingPolicy result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.maxAttempts_ = maxAttempts_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.hedgingDelay_ = hedgingDelayBuilder_ == null
? hedgingDelay_
: hedgingDelayBuilder_.build();
to_bitField0_ |= 0x00000001;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.grpc.serviceconfig.MethodConfig.HedgingPolicy) {
return mergeFrom((io.grpc.serviceconfig.MethodConfig.HedgingPolicy)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.grpc.serviceconfig.MethodConfig.HedgingPolicy other) {
if (other == io.grpc.serviceconfig.MethodConfig.HedgingPolicy.getDefaultInstance()) return this;
if (other.getMaxAttempts() != 0) {
setMaxAttempts(other.getMaxAttempts());
}
if (other.hasHedgingDelay()) {
mergeHedgingDelay(other.getHedgingDelay());
}
if (!other.nonFatalStatusCodes_.isEmpty()) {
if (nonFatalStatusCodes_.isEmpty()) {
nonFatalStatusCodes_ = other.nonFatalStatusCodes_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureNonFatalStatusCodesIsMutable();
nonFatalStatusCodes_.addAll(other.nonFatalStatusCodes_);
}
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
maxAttempts_ = input.readUInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
input.readMessage(
getHedgingDelayFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
int tmpRaw = input.readEnum();
ensureNonFatalStatusCodesIsMutable();
nonFatalStatusCodes_.add(tmpRaw);
break;
} // case 24
case 26: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int tmpRaw = input.readEnum();
ensureNonFatalStatusCodesIsMutable();
nonFatalStatusCodes_.add(tmpRaw);
}
input.popLimit(oldLimit);
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int maxAttempts_ ;
/**
*
* The hedging policy will send up to max_requests RPCs.
* This number represents the total number of all attempts, including
* the original attempt.
*
* This field is required and must be greater than 1.
* Any value greater than 5 will be treated as if it were 5.
*
*
* uint32 max_attempts = 1;
* @return The maxAttempts.
*/
@java.lang.Override
public int getMaxAttempts() {
return maxAttempts_;
}
/**
*
* The hedging policy will send up to max_requests RPCs.
* This number represents the total number of all attempts, including
* the original attempt.
*
* This field is required and must be greater than 1.
* Any value greater than 5 will be treated as if it were 5.
*
*
* uint32 max_attempts = 1;
* @param value The maxAttempts to set.
* @return This builder for chaining.
*/
public Builder setMaxAttempts(int value) {
maxAttempts_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The hedging policy will send up to max_requests RPCs.
* This number represents the total number of all attempts, including
* the original attempt.
*
* This field is required and must be greater than 1.
* Any value greater than 5 will be treated as if it were 5.
*
*
* uint32 max_attempts = 1;
* @return This builder for chaining.
*/
public Builder clearMaxAttempts() {
bitField0_ = (bitField0_ & ~0x00000001);
maxAttempts_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Duration hedgingDelay_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> hedgingDelayBuilder_;
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
* @return Whether the hedgingDelay field is set.
*/
public boolean hasHedgingDelay() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
* @return The hedgingDelay.
*/
public com.google.protobuf.Duration getHedgingDelay() {
if (hedgingDelayBuilder_ == null) {
return hedgingDelay_ == null ? com.google.protobuf.Duration.getDefaultInstance() : hedgingDelay_;
} else {
return hedgingDelayBuilder_.getMessage();
}
}
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
*/
public Builder setHedgingDelay(com.google.protobuf.Duration value) {
if (hedgingDelayBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
hedgingDelay_ = value;
} else {
hedgingDelayBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
*/
public Builder setHedgingDelay(
com.google.protobuf.Duration.Builder builderForValue) {
if (hedgingDelayBuilder_ == null) {
hedgingDelay_ = builderForValue.build();
} else {
hedgingDelayBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
*/
public Builder mergeHedgingDelay(com.google.protobuf.Duration value) {
if (hedgingDelayBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
hedgingDelay_ != null &&
hedgingDelay_ != com.google.protobuf.Duration.getDefaultInstance()) {
getHedgingDelayBuilder().mergeFrom(value);
} else {
hedgingDelay_ = value;
}
} else {
hedgingDelayBuilder_.mergeFrom(value);
}
if (hedgingDelay_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
*/
public Builder clearHedgingDelay() {
bitField0_ = (bitField0_ & ~0x00000002);
hedgingDelay_ = null;
if (hedgingDelayBuilder_ != null) {
hedgingDelayBuilder_.dispose();
hedgingDelayBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
*/
public com.google.protobuf.Duration.Builder getHedgingDelayBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getHedgingDelayFieldBuilder().getBuilder();
}
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
*/
public com.google.protobuf.DurationOrBuilder getHedgingDelayOrBuilder() {
if (hedgingDelayBuilder_ != null) {
return hedgingDelayBuilder_.getMessageOrBuilder();
} else {
return hedgingDelay_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : hedgingDelay_;
}
}
/**
*
* The first RPC will be sent immediately, but the max_requests-1 subsequent
* hedged RPCs will be sent at intervals of every hedging_delay. Set this
* to 0 to immediately send all max_requests RPCs.
*
*
* .google.protobuf.Duration hedging_delay = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getHedgingDelayFieldBuilder() {
if (hedgingDelayBuilder_ == null) {
hedgingDelayBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getHedgingDelay(),
getParentForChildren(),
isClean());
hedgingDelay_ = null;
}
return hedgingDelayBuilder_;
}
private java.util.List nonFatalStatusCodes_ =
java.util.Collections.emptyList();
private void ensureNonFatalStatusCodesIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
nonFatalStatusCodes_ = new java.util.ArrayList(nonFatalStatusCodes_);
bitField0_ |= 0x00000004;
}
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @return A list containing the nonFatalStatusCodes.
*/
public java.util.List getNonFatalStatusCodesList() {
return new com.google.protobuf.Internal.ListAdapter<
java.lang.Integer, com.google.rpc.Code>(nonFatalStatusCodes_, nonFatalStatusCodes_converter_);
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @return The count of nonFatalStatusCodes.
*/
public int getNonFatalStatusCodesCount() {
return nonFatalStatusCodes_.size();
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param index The index of the element to return.
* @return The nonFatalStatusCodes at the given index.
*/
public com.google.rpc.Code getNonFatalStatusCodes(int index) {
return nonFatalStatusCodes_converter_.convert(nonFatalStatusCodes_.get(index));
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param index The index to set the value at.
* @param value The nonFatalStatusCodes to set.
* @return This builder for chaining.
*/
public Builder setNonFatalStatusCodes(
int index, com.google.rpc.Code value) {
if (value == null) {
throw new NullPointerException();
}
ensureNonFatalStatusCodesIsMutable();
nonFatalStatusCodes_.set(index, value.getNumber());
onChanged();
return this;
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param value The nonFatalStatusCodes to add.
* @return This builder for chaining.
*/
public Builder addNonFatalStatusCodes(com.google.rpc.Code value) {
if (value == null) {
throw new NullPointerException();
}
ensureNonFatalStatusCodesIsMutable();
nonFatalStatusCodes_.add(value.getNumber());
onChanged();
return this;
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param values The nonFatalStatusCodes to add.
* @return This builder for chaining.
*/
public Builder addAllNonFatalStatusCodes(
java.lang.Iterable extends com.google.rpc.Code> values) {
ensureNonFatalStatusCodesIsMutable();
for (com.google.rpc.Code value : values) {
nonFatalStatusCodes_.add(value.getNumber());
}
onChanged();
return this;
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @return This builder for chaining.
*/
public Builder clearNonFatalStatusCodes() {
nonFatalStatusCodes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @return A list containing the enum numeric values on the wire for nonFatalStatusCodes.
*/
public java.util.List
getNonFatalStatusCodesValueList() {
return java.util.Collections.unmodifiableList(nonFatalStatusCodes_);
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of nonFatalStatusCodes at the given index.
*/
public int getNonFatalStatusCodesValue(int index) {
return nonFatalStatusCodes_.get(index);
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param index The index to set the value at.
* @param value The enum numeric value on the wire for nonFatalStatusCodes to set.
* @return This builder for chaining.
*/
public Builder setNonFatalStatusCodesValue(
int index, int value) {
ensureNonFatalStatusCodesIsMutable();
nonFatalStatusCodes_.set(index, value);
onChanged();
return this;
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param value The enum numeric value on the wire for nonFatalStatusCodes to add.
* @return This builder for chaining.
*/
public Builder addNonFatalStatusCodesValue(int value) {
ensureNonFatalStatusCodesIsMutable();
nonFatalStatusCodes_.add(value);
onChanged();
return this;
}
/**
*
* The set of status codes which indicate other hedged RPCs may still
* succeed. If a non-fatal status code is returned by the server, hedged
* RPCs will continue. Otherwise, outstanding requests will be canceled and
* the error returned to the client application layer.
*
* This field is optional.
*
*
* repeated .google.rpc.Code non_fatal_status_codes = 3;
* @param values The enum numeric values on the wire for nonFatalStatusCodes to add.
* @return This builder for chaining.
*/
public Builder addAllNonFatalStatusCodesValue(
java.lang.Iterable values) {
ensureNonFatalStatusCodesIsMutable();
for (int value : values) {
nonFatalStatusCodes_.add(value);
}
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:grpc.service_config.MethodConfig.HedgingPolicy)
}
// @@protoc_insertion_point(class_scope:grpc.service_config.MethodConfig.HedgingPolicy)
private static final io.grpc.serviceconfig.MethodConfig.HedgingPolicy DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.grpc.serviceconfig.MethodConfig.HedgingPolicy();
}
public static io.grpc.serviceconfig.MethodConfig.HedgingPolicy getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public HedgingPolicy parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.HedgingPolicy getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
private int retryOrHedgingPolicyCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object retryOrHedgingPolicy_;
public enum RetryOrHedgingPolicyCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
RETRY_POLICY(6),
HEDGING_POLICY(7),
RETRYORHEDGINGPOLICY_NOT_SET(0);
private final int value;
private RetryOrHedgingPolicyCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static RetryOrHedgingPolicyCase valueOf(int value) {
return forNumber(value);
}
public static RetryOrHedgingPolicyCase forNumber(int value) {
switch (value) {
case 6: return RETRY_POLICY;
case 7: return HEDGING_POLICY;
case 0: return RETRYORHEDGINGPOLICY_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public RetryOrHedgingPolicyCase
getRetryOrHedgingPolicyCase() {
return RetryOrHedgingPolicyCase.forNumber(
retryOrHedgingPolicyCase_);
}
public static final int NAME_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List name_;
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
@java.lang.Override
public java.util.List getNameList() {
return name_;
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
@java.lang.Override
public java.util.List extends io.grpc.serviceconfig.MethodConfig.NameOrBuilder>
getNameOrBuilderList() {
return name_;
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
@java.lang.Override
public int getNameCount() {
return name_.size();
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.Name getName(int index) {
return name_.get(index);
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.NameOrBuilder getNameOrBuilder(
int index) {
return name_.get(index);
}
public static final int WAIT_FOR_READY_FIELD_NUMBER = 2;
private com.google.protobuf.BoolValue waitForReady_;
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
* @return Whether the waitForReady field is set.
*/
@java.lang.Override
public boolean hasWaitForReady() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
* @return The waitForReady.
*/
@java.lang.Override
public com.google.protobuf.BoolValue getWaitForReady() {
return waitForReady_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : waitForReady_;
}
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
*/
@java.lang.Override
public com.google.protobuf.BoolValueOrBuilder getWaitForReadyOrBuilder() {
return waitForReady_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : waitForReady_;
}
public static final int TIMEOUT_FIELD_NUMBER = 3;
private com.google.protobuf.Duration timeout_;
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
* @return Whether the timeout field is set.
*/
@java.lang.Override
public boolean hasTimeout() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
* @return The timeout.
*/
@java.lang.Override
public com.google.protobuf.Duration getTimeout() {
return timeout_ == null ? com.google.protobuf.Duration.getDefaultInstance() : timeout_;
}
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getTimeoutOrBuilder() {
return timeout_ == null ? com.google.protobuf.Duration.getDefaultInstance() : timeout_;
}
public static final int MAX_REQUEST_MESSAGE_BYTES_FIELD_NUMBER = 4;
private com.google.protobuf.UInt32Value maxRequestMessageBytes_;
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
* @return Whether the maxRequestMessageBytes field is set.
*/
@java.lang.Override
public boolean hasMaxRequestMessageBytes() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
* @return The maxRequestMessageBytes.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getMaxRequestMessageBytes() {
return maxRequestMessageBytes_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : maxRequestMessageBytes_;
}
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getMaxRequestMessageBytesOrBuilder() {
return maxRequestMessageBytes_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : maxRequestMessageBytes_;
}
public static final int MAX_RESPONSE_MESSAGE_BYTES_FIELD_NUMBER = 5;
private com.google.protobuf.UInt32Value maxResponseMessageBytes_;
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
* @return Whether the maxResponseMessageBytes field is set.
*/
@java.lang.Override
public boolean hasMaxResponseMessageBytes() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
* @return The maxResponseMessageBytes.
*/
@java.lang.Override
public com.google.protobuf.UInt32Value getMaxResponseMessageBytes() {
return maxResponseMessageBytes_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : maxResponseMessageBytes_;
}
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
*/
@java.lang.Override
public com.google.protobuf.UInt32ValueOrBuilder getMaxResponseMessageBytesOrBuilder() {
return maxResponseMessageBytes_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : maxResponseMessageBytes_;
}
public static final int RETRY_POLICY_FIELD_NUMBER = 6;
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
* @return Whether the retryPolicy field is set.
*/
@java.lang.Override
public boolean hasRetryPolicy() {
return retryOrHedgingPolicyCase_ == 6;
}
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
* @return The retryPolicy.
*/
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.RetryPolicy getRetryPolicy() {
if (retryOrHedgingPolicyCase_ == 6) {
return (io.grpc.serviceconfig.MethodConfig.RetryPolicy) retryOrHedgingPolicy_;
}
return io.grpc.serviceconfig.MethodConfig.RetryPolicy.getDefaultInstance();
}
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
*/
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.RetryPolicyOrBuilder getRetryPolicyOrBuilder() {
if (retryOrHedgingPolicyCase_ == 6) {
return (io.grpc.serviceconfig.MethodConfig.RetryPolicy) retryOrHedgingPolicy_;
}
return io.grpc.serviceconfig.MethodConfig.RetryPolicy.getDefaultInstance();
}
public static final int HEDGING_POLICY_FIELD_NUMBER = 7;
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
* @return Whether the hedgingPolicy field is set.
*/
@java.lang.Override
public boolean hasHedgingPolicy() {
return retryOrHedgingPolicyCase_ == 7;
}
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
* @return The hedgingPolicy.
*/
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.HedgingPolicy getHedgingPolicy() {
if (retryOrHedgingPolicyCase_ == 7) {
return (io.grpc.serviceconfig.MethodConfig.HedgingPolicy) retryOrHedgingPolicy_;
}
return io.grpc.serviceconfig.MethodConfig.HedgingPolicy.getDefaultInstance();
}
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
*/
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.HedgingPolicyOrBuilder getHedgingPolicyOrBuilder() {
if (retryOrHedgingPolicyCase_ == 7) {
return (io.grpc.serviceconfig.MethodConfig.HedgingPolicy) retryOrHedgingPolicy_;
}
return io.grpc.serviceconfig.MethodConfig.HedgingPolicy.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < name_.size(); i++) {
output.writeMessage(1, name_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(2, getWaitForReady());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(3, getTimeout());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(4, getMaxRequestMessageBytes());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(5, getMaxResponseMessageBytes());
}
if (retryOrHedgingPolicyCase_ == 6) {
output.writeMessage(6, (io.grpc.serviceconfig.MethodConfig.RetryPolicy) retryOrHedgingPolicy_);
}
if (retryOrHedgingPolicyCase_ == 7) {
output.writeMessage(7, (io.grpc.serviceconfig.MethodConfig.HedgingPolicy) retryOrHedgingPolicy_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < name_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, name_.get(i));
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getWaitForReady());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getTimeout());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getMaxRequestMessageBytes());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getMaxResponseMessageBytes());
}
if (retryOrHedgingPolicyCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, (io.grpc.serviceconfig.MethodConfig.RetryPolicy) retryOrHedgingPolicy_);
}
if (retryOrHedgingPolicyCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, (io.grpc.serviceconfig.MethodConfig.HedgingPolicy) retryOrHedgingPolicy_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof io.grpc.serviceconfig.MethodConfig)) {
return super.equals(obj);
}
io.grpc.serviceconfig.MethodConfig other = (io.grpc.serviceconfig.MethodConfig) obj;
if (!getNameList()
.equals(other.getNameList())) return false;
if (hasWaitForReady() != other.hasWaitForReady()) return false;
if (hasWaitForReady()) {
if (!getWaitForReady()
.equals(other.getWaitForReady())) return false;
}
if (hasTimeout() != other.hasTimeout()) return false;
if (hasTimeout()) {
if (!getTimeout()
.equals(other.getTimeout())) return false;
}
if (hasMaxRequestMessageBytes() != other.hasMaxRequestMessageBytes()) return false;
if (hasMaxRequestMessageBytes()) {
if (!getMaxRequestMessageBytes()
.equals(other.getMaxRequestMessageBytes())) return false;
}
if (hasMaxResponseMessageBytes() != other.hasMaxResponseMessageBytes()) return false;
if (hasMaxResponseMessageBytes()) {
if (!getMaxResponseMessageBytes()
.equals(other.getMaxResponseMessageBytes())) return false;
}
if (!getRetryOrHedgingPolicyCase().equals(other.getRetryOrHedgingPolicyCase())) return false;
switch (retryOrHedgingPolicyCase_) {
case 6:
if (!getRetryPolicy()
.equals(other.getRetryPolicy())) return false;
break;
case 7:
if (!getHedgingPolicy()
.equals(other.getHedgingPolicy())) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getNameCount() > 0) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getNameList().hashCode();
}
if (hasWaitForReady()) {
hash = (37 * hash) + WAIT_FOR_READY_FIELD_NUMBER;
hash = (53 * hash) + getWaitForReady().hashCode();
}
if (hasTimeout()) {
hash = (37 * hash) + TIMEOUT_FIELD_NUMBER;
hash = (53 * hash) + getTimeout().hashCode();
}
if (hasMaxRequestMessageBytes()) {
hash = (37 * hash) + MAX_REQUEST_MESSAGE_BYTES_FIELD_NUMBER;
hash = (53 * hash) + getMaxRequestMessageBytes().hashCode();
}
if (hasMaxResponseMessageBytes()) {
hash = (37 * hash) + MAX_RESPONSE_MESSAGE_BYTES_FIELD_NUMBER;
hash = (53 * hash) + getMaxResponseMessageBytes().hashCode();
}
switch (retryOrHedgingPolicyCase_) {
case 6:
hash = (37 * hash) + RETRY_POLICY_FIELD_NUMBER;
hash = (53 * hash) + getRetryPolicy().hashCode();
break;
case 7:
hash = (37 * hash) + HEDGING_POLICY_FIELD_NUMBER;
hash = (53 * hash) + getHedgingPolicy().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static io.grpc.serviceconfig.MethodConfig parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static io.grpc.serviceconfig.MethodConfig parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static io.grpc.serviceconfig.MethodConfig parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static io.grpc.serviceconfig.MethodConfig parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(io.grpc.serviceconfig.MethodConfig prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Configuration for a method.
*
*
* Protobuf type {@code grpc.service_config.MethodConfig}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:grpc.service_config.MethodConfig)
io.grpc.serviceconfig.MethodConfigOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_fieldAccessorTable
.ensureFieldAccessorsInitialized(
io.grpc.serviceconfig.MethodConfig.class, io.grpc.serviceconfig.MethodConfig.Builder.class);
}
// Construct using io.grpc.serviceconfig.MethodConfig.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getNameFieldBuilder();
getWaitForReadyFieldBuilder();
getTimeoutFieldBuilder();
getMaxRequestMessageBytesFieldBuilder();
getMaxResponseMessageBytesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (nameBuilder_ == null) {
name_ = java.util.Collections.emptyList();
} else {
name_ = null;
nameBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
waitForReady_ = null;
if (waitForReadyBuilder_ != null) {
waitForReadyBuilder_.dispose();
waitForReadyBuilder_ = null;
}
timeout_ = null;
if (timeoutBuilder_ != null) {
timeoutBuilder_.dispose();
timeoutBuilder_ = null;
}
maxRequestMessageBytes_ = null;
if (maxRequestMessageBytesBuilder_ != null) {
maxRequestMessageBytesBuilder_.dispose();
maxRequestMessageBytesBuilder_ = null;
}
maxResponseMessageBytes_ = null;
if (maxResponseMessageBytesBuilder_ != null) {
maxResponseMessageBytesBuilder_.dispose();
maxResponseMessageBytesBuilder_ = null;
}
if (retryPolicyBuilder_ != null) {
retryPolicyBuilder_.clear();
}
if (hedgingPolicyBuilder_ != null) {
hedgingPolicyBuilder_.clear();
}
retryOrHedgingPolicyCase_ = 0;
retryOrHedgingPolicy_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return io.grpc.serviceconfig.ServiceConfigProto.internal_static_grpc_service_config_MethodConfig_descriptor;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig getDefaultInstanceForType() {
return io.grpc.serviceconfig.MethodConfig.getDefaultInstance();
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig build() {
io.grpc.serviceconfig.MethodConfig result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig buildPartial() {
io.grpc.serviceconfig.MethodConfig result = new io.grpc.serviceconfig.MethodConfig(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartialRepeatedFields(io.grpc.serviceconfig.MethodConfig result) {
if (nameBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
name_ = java.util.Collections.unmodifiableList(name_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.name_ = name_;
} else {
result.name_ = nameBuilder_.build();
}
}
private void buildPartial0(io.grpc.serviceconfig.MethodConfig result) {
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000002) != 0)) {
result.waitForReady_ = waitForReadyBuilder_ == null
? waitForReady_
: waitForReadyBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.timeout_ = timeoutBuilder_ == null
? timeout_
: timeoutBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.maxRequestMessageBytes_ = maxRequestMessageBytesBuilder_ == null
? maxRequestMessageBytes_
: maxRequestMessageBytesBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.maxResponseMessageBytes_ = maxResponseMessageBytesBuilder_ == null
? maxResponseMessageBytes_
: maxResponseMessageBytesBuilder_.build();
to_bitField0_ |= 0x00000008;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartialOneofs(io.grpc.serviceconfig.MethodConfig result) {
result.retryOrHedgingPolicyCase_ = retryOrHedgingPolicyCase_;
result.retryOrHedgingPolicy_ = this.retryOrHedgingPolicy_;
if (retryOrHedgingPolicyCase_ == 6 &&
retryPolicyBuilder_ != null) {
result.retryOrHedgingPolicy_ = retryPolicyBuilder_.build();
}
if (retryOrHedgingPolicyCase_ == 7 &&
hedgingPolicyBuilder_ != null) {
result.retryOrHedgingPolicy_ = hedgingPolicyBuilder_.build();
}
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof io.grpc.serviceconfig.MethodConfig) {
return mergeFrom((io.grpc.serviceconfig.MethodConfig)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(io.grpc.serviceconfig.MethodConfig other) {
if (other == io.grpc.serviceconfig.MethodConfig.getDefaultInstance()) return this;
if (nameBuilder_ == null) {
if (!other.name_.isEmpty()) {
if (name_.isEmpty()) {
name_ = other.name_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureNameIsMutable();
name_.addAll(other.name_);
}
onChanged();
}
} else {
if (!other.name_.isEmpty()) {
if (nameBuilder_.isEmpty()) {
nameBuilder_.dispose();
nameBuilder_ = null;
name_ = other.name_;
bitField0_ = (bitField0_ & ~0x00000001);
nameBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNameFieldBuilder() : null;
} else {
nameBuilder_.addAllMessages(other.name_);
}
}
}
if (other.hasWaitForReady()) {
mergeWaitForReady(other.getWaitForReady());
}
if (other.hasTimeout()) {
mergeTimeout(other.getTimeout());
}
if (other.hasMaxRequestMessageBytes()) {
mergeMaxRequestMessageBytes(other.getMaxRequestMessageBytes());
}
if (other.hasMaxResponseMessageBytes()) {
mergeMaxResponseMessageBytes(other.getMaxResponseMessageBytes());
}
switch (other.getRetryOrHedgingPolicyCase()) {
case RETRY_POLICY: {
mergeRetryPolicy(other.getRetryPolicy());
break;
}
case HEDGING_POLICY: {
mergeHedgingPolicy(other.getHedgingPolicy());
break;
}
case RETRYORHEDGINGPOLICY_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
io.grpc.serviceconfig.MethodConfig.Name m =
input.readMessage(
io.grpc.serviceconfig.MethodConfig.Name.parser(),
extensionRegistry);
if (nameBuilder_ == null) {
ensureNameIsMutable();
name_.add(m);
} else {
nameBuilder_.addMessage(m);
}
break;
} // case 10
case 18: {
input.readMessage(
getWaitForReadyFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
input.readMessage(
getTimeoutFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
input.readMessage(
getMaxRequestMessageBytesFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000008;
break;
} // case 34
case 42: {
input.readMessage(
getMaxResponseMessageBytesFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
input.readMessage(
getRetryPolicyFieldBuilder().getBuilder(),
extensionRegistry);
retryOrHedgingPolicyCase_ = 6;
break;
} // case 50
case 58: {
input.readMessage(
getHedgingPolicyFieldBuilder().getBuilder(),
extensionRegistry);
retryOrHedgingPolicyCase_ = 7;
break;
} // case 58
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int retryOrHedgingPolicyCase_ = 0;
private java.lang.Object retryOrHedgingPolicy_;
public RetryOrHedgingPolicyCase
getRetryOrHedgingPolicyCase() {
return RetryOrHedgingPolicyCase.forNumber(
retryOrHedgingPolicyCase_);
}
public Builder clearRetryOrHedgingPolicy() {
retryOrHedgingPolicyCase_ = 0;
retryOrHedgingPolicy_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.util.List name_ =
java.util.Collections.emptyList();
private void ensureNameIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
name_ = new java.util.ArrayList(name_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.serviceconfig.MethodConfig.Name, io.grpc.serviceconfig.MethodConfig.Name.Builder, io.grpc.serviceconfig.MethodConfig.NameOrBuilder> nameBuilder_;
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public java.util.List getNameList() {
if (nameBuilder_ == null) {
return java.util.Collections.unmodifiableList(name_);
} else {
return nameBuilder_.getMessageList();
}
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public int getNameCount() {
if (nameBuilder_ == null) {
return name_.size();
} else {
return nameBuilder_.getCount();
}
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public io.grpc.serviceconfig.MethodConfig.Name getName(int index) {
if (nameBuilder_ == null) {
return name_.get(index);
} else {
return nameBuilder_.getMessage(index);
}
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public Builder setName(
int index, io.grpc.serviceconfig.MethodConfig.Name value) {
if (nameBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameIsMutable();
name_.set(index, value);
onChanged();
} else {
nameBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public Builder setName(
int index, io.grpc.serviceconfig.MethodConfig.Name.Builder builderForValue) {
if (nameBuilder_ == null) {
ensureNameIsMutable();
name_.set(index, builderForValue.build());
onChanged();
} else {
nameBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public Builder addName(io.grpc.serviceconfig.MethodConfig.Name value) {
if (nameBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameIsMutable();
name_.add(value);
onChanged();
} else {
nameBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public Builder addName(
int index, io.grpc.serviceconfig.MethodConfig.Name value) {
if (nameBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNameIsMutable();
name_.add(index, value);
onChanged();
} else {
nameBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public Builder addName(
io.grpc.serviceconfig.MethodConfig.Name.Builder builderForValue) {
if (nameBuilder_ == null) {
ensureNameIsMutable();
name_.add(builderForValue.build());
onChanged();
} else {
nameBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public Builder addName(
int index, io.grpc.serviceconfig.MethodConfig.Name.Builder builderForValue) {
if (nameBuilder_ == null) {
ensureNameIsMutable();
name_.add(index, builderForValue.build());
onChanged();
} else {
nameBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public Builder addAllName(
java.lang.Iterable extends io.grpc.serviceconfig.MethodConfig.Name> values) {
if (nameBuilder_ == null) {
ensureNameIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, name_);
onChanged();
} else {
nameBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public Builder clearName() {
if (nameBuilder_ == null) {
name_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
nameBuilder_.clear();
}
return this;
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public Builder removeName(int index) {
if (nameBuilder_ == null) {
ensureNameIsMutable();
name_.remove(index);
onChanged();
} else {
nameBuilder_.remove(index);
}
return this;
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public io.grpc.serviceconfig.MethodConfig.Name.Builder getNameBuilder(
int index) {
return getNameFieldBuilder().getBuilder(index);
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public io.grpc.serviceconfig.MethodConfig.NameOrBuilder getNameOrBuilder(
int index) {
if (nameBuilder_ == null) {
return name_.get(index); } else {
return nameBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public java.util.List extends io.grpc.serviceconfig.MethodConfig.NameOrBuilder>
getNameOrBuilderList() {
if (nameBuilder_ != null) {
return nameBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(name_);
}
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public io.grpc.serviceconfig.MethodConfig.Name.Builder addNameBuilder() {
return getNameFieldBuilder().addBuilder(
io.grpc.serviceconfig.MethodConfig.Name.getDefaultInstance());
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public io.grpc.serviceconfig.MethodConfig.Name.Builder addNameBuilder(
int index) {
return getNameFieldBuilder().addBuilder(
index, io.grpc.serviceconfig.MethodConfig.Name.getDefaultInstance());
}
/**
* repeated .grpc.service_config.MethodConfig.Name name = 1;
*/
public java.util.List
getNameBuilderList() {
return getNameFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.serviceconfig.MethodConfig.Name, io.grpc.serviceconfig.MethodConfig.Name.Builder, io.grpc.serviceconfig.MethodConfig.NameOrBuilder>
getNameFieldBuilder() {
if (nameBuilder_ == null) {
nameBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
io.grpc.serviceconfig.MethodConfig.Name, io.grpc.serviceconfig.MethodConfig.Name.Builder, io.grpc.serviceconfig.MethodConfig.NameOrBuilder>(
name_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
name_ = null;
}
return nameBuilder_;
}
private com.google.protobuf.BoolValue waitForReady_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder> waitForReadyBuilder_;
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
* @return Whether the waitForReady field is set.
*/
public boolean hasWaitForReady() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
* @return The waitForReady.
*/
public com.google.protobuf.BoolValue getWaitForReady() {
if (waitForReadyBuilder_ == null) {
return waitForReady_ == null ? com.google.protobuf.BoolValue.getDefaultInstance() : waitForReady_;
} else {
return waitForReadyBuilder_.getMessage();
}
}
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
*/
public Builder setWaitForReady(com.google.protobuf.BoolValue value) {
if (waitForReadyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
waitForReady_ = value;
} else {
waitForReadyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
*/
public Builder setWaitForReady(
com.google.protobuf.BoolValue.Builder builderForValue) {
if (waitForReadyBuilder_ == null) {
waitForReady_ = builderForValue.build();
} else {
waitForReadyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
*/
public Builder mergeWaitForReady(com.google.protobuf.BoolValue value) {
if (waitForReadyBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
waitForReady_ != null &&
waitForReady_ != com.google.protobuf.BoolValue.getDefaultInstance()) {
getWaitForReadyBuilder().mergeFrom(value);
} else {
waitForReady_ = value;
}
} else {
waitForReadyBuilder_.mergeFrom(value);
}
if (waitForReady_ != null) {
bitField0_ |= 0x00000002;
onChanged();
}
return this;
}
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
*/
public Builder clearWaitForReady() {
bitField0_ = (bitField0_ & ~0x00000002);
waitForReady_ = null;
if (waitForReadyBuilder_ != null) {
waitForReadyBuilder_.dispose();
waitForReadyBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
*/
public com.google.protobuf.BoolValue.Builder getWaitForReadyBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getWaitForReadyFieldBuilder().getBuilder();
}
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
*/
public com.google.protobuf.BoolValueOrBuilder getWaitForReadyOrBuilder() {
if (waitForReadyBuilder_ != null) {
return waitForReadyBuilder_.getMessageOrBuilder();
} else {
return waitForReady_ == null ?
com.google.protobuf.BoolValue.getDefaultInstance() : waitForReady_;
}
}
/**
*
* Whether RPCs sent to this method should wait until the connection is
* ready by default. If false, the RPC will abort immediately if there is
* a transient failure connecting to the server. Otherwise, gRPC will
* attempt to connect until the deadline is exceeded.
*
* The value specified via the gRPC client API will override the value
* set here. However, note that setting the value in the client API will
* also affect transient errors encountered during name resolution, which
* cannot be caught by the value here, since the service config is
* obtained by the gRPC client via name resolution.
*
*
* .google.protobuf.BoolValue wait_for_ready = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>
getWaitForReadyFieldBuilder() {
if (waitForReadyBuilder_ == null) {
waitForReadyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.BoolValue, com.google.protobuf.BoolValue.Builder, com.google.protobuf.BoolValueOrBuilder>(
getWaitForReady(),
getParentForChildren(),
isClean());
waitForReady_ = null;
}
return waitForReadyBuilder_;
}
private com.google.protobuf.Duration timeout_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> timeoutBuilder_;
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
* @return Whether the timeout field is set.
*/
public boolean hasTimeout() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
* @return The timeout.
*/
public com.google.protobuf.Duration getTimeout() {
if (timeoutBuilder_ == null) {
return timeout_ == null ? com.google.protobuf.Duration.getDefaultInstance() : timeout_;
} else {
return timeoutBuilder_.getMessage();
}
}
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
*/
public Builder setTimeout(com.google.protobuf.Duration value) {
if (timeoutBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
timeout_ = value;
} else {
timeoutBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
*/
public Builder setTimeout(
com.google.protobuf.Duration.Builder builderForValue) {
if (timeoutBuilder_ == null) {
timeout_ = builderForValue.build();
} else {
timeoutBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
*/
public Builder mergeTimeout(com.google.protobuf.Duration value) {
if (timeoutBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
timeout_ != null &&
timeout_ != com.google.protobuf.Duration.getDefaultInstance()) {
getTimeoutBuilder().mergeFrom(value);
} else {
timeout_ = value;
}
} else {
timeoutBuilder_.mergeFrom(value);
}
if (timeout_ != null) {
bitField0_ |= 0x00000004;
onChanged();
}
return this;
}
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
*/
public Builder clearTimeout() {
bitField0_ = (bitField0_ & ~0x00000004);
timeout_ = null;
if (timeoutBuilder_ != null) {
timeoutBuilder_.dispose();
timeoutBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
*/
public com.google.protobuf.Duration.Builder getTimeoutBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getTimeoutFieldBuilder().getBuilder();
}
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
*/
public com.google.protobuf.DurationOrBuilder getTimeoutOrBuilder() {
if (timeoutBuilder_ != null) {
return timeoutBuilder_.getMessageOrBuilder();
} else {
return timeout_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : timeout_;
}
}
/**
*
* The default timeout in seconds for RPCs sent to this method. This can be
* overridden in code. If no reply is received in the specified amount of
* time, the request is aborted and a DEADLINE_EXCEEDED error status
* is returned to the caller.
*
* The actual deadline used will be the minimum of the value specified here
* and the value set by the application via the gRPC client API. If either
* one is not set, then the other will be used. If neither is set, then the
* request has no deadline.
*
*
* .google.protobuf.Duration timeout = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getTimeoutFieldBuilder() {
if (timeoutBuilder_ == null) {
timeoutBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getTimeout(),
getParentForChildren(),
isClean());
timeout_ = null;
}
return timeoutBuilder_;
}
private com.google.protobuf.UInt32Value maxRequestMessageBytes_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> maxRequestMessageBytesBuilder_;
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
* @return Whether the maxRequestMessageBytes field is set.
*/
public boolean hasMaxRequestMessageBytes() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
* @return The maxRequestMessageBytes.
*/
public com.google.protobuf.UInt32Value getMaxRequestMessageBytes() {
if (maxRequestMessageBytesBuilder_ == null) {
return maxRequestMessageBytes_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : maxRequestMessageBytes_;
} else {
return maxRequestMessageBytesBuilder_.getMessage();
}
}
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
*/
public Builder setMaxRequestMessageBytes(com.google.protobuf.UInt32Value value) {
if (maxRequestMessageBytesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maxRequestMessageBytes_ = value;
} else {
maxRequestMessageBytesBuilder_.setMessage(value);
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
*/
public Builder setMaxRequestMessageBytes(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (maxRequestMessageBytesBuilder_ == null) {
maxRequestMessageBytes_ = builderForValue.build();
} else {
maxRequestMessageBytesBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
*/
public Builder mergeMaxRequestMessageBytes(com.google.protobuf.UInt32Value value) {
if (maxRequestMessageBytesBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0) &&
maxRequestMessageBytes_ != null &&
maxRequestMessageBytes_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getMaxRequestMessageBytesBuilder().mergeFrom(value);
} else {
maxRequestMessageBytes_ = value;
}
} else {
maxRequestMessageBytesBuilder_.mergeFrom(value);
}
if (maxRequestMessageBytes_ != null) {
bitField0_ |= 0x00000008;
onChanged();
}
return this;
}
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
*/
public Builder clearMaxRequestMessageBytes() {
bitField0_ = (bitField0_ & ~0x00000008);
maxRequestMessageBytes_ = null;
if (maxRequestMessageBytesBuilder_ != null) {
maxRequestMessageBytesBuilder_.dispose();
maxRequestMessageBytesBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
*/
public com.google.protobuf.UInt32Value.Builder getMaxRequestMessageBytesBuilder() {
bitField0_ |= 0x00000008;
onChanged();
return getMaxRequestMessageBytesFieldBuilder().getBuilder();
}
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
*/
public com.google.protobuf.UInt32ValueOrBuilder getMaxRequestMessageBytesOrBuilder() {
if (maxRequestMessageBytesBuilder_ != null) {
return maxRequestMessageBytesBuilder_.getMessageOrBuilder();
} else {
return maxRequestMessageBytes_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : maxRequestMessageBytes_;
}
}
/**
*
* The maximum allowed payload size for an individual request or object in a
* stream (client->server) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a client attempts to send an object larger than this value, it will not
* be sent and the client will see a ClientError.
* Note that 0 is a valid value, meaning that the request message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_request_message_bytes = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getMaxRequestMessageBytesFieldBuilder() {
if (maxRequestMessageBytesBuilder_ == null) {
maxRequestMessageBytesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getMaxRequestMessageBytes(),
getParentForChildren(),
isClean());
maxRequestMessageBytes_ = null;
}
return maxRequestMessageBytesBuilder_;
}
private com.google.protobuf.UInt32Value maxResponseMessageBytes_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder> maxResponseMessageBytesBuilder_;
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
* @return Whether the maxResponseMessageBytes field is set.
*/
public boolean hasMaxResponseMessageBytes() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
* @return The maxResponseMessageBytes.
*/
public com.google.protobuf.UInt32Value getMaxResponseMessageBytes() {
if (maxResponseMessageBytesBuilder_ == null) {
return maxResponseMessageBytes_ == null ? com.google.protobuf.UInt32Value.getDefaultInstance() : maxResponseMessageBytes_;
} else {
return maxResponseMessageBytesBuilder_.getMessage();
}
}
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
*/
public Builder setMaxResponseMessageBytes(com.google.protobuf.UInt32Value value) {
if (maxResponseMessageBytesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
maxResponseMessageBytes_ = value;
} else {
maxResponseMessageBytesBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
*/
public Builder setMaxResponseMessageBytes(
com.google.protobuf.UInt32Value.Builder builderForValue) {
if (maxResponseMessageBytesBuilder_ == null) {
maxResponseMessageBytes_ = builderForValue.build();
} else {
maxResponseMessageBytesBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
*/
public Builder mergeMaxResponseMessageBytes(com.google.protobuf.UInt32Value value) {
if (maxResponseMessageBytesBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0) &&
maxResponseMessageBytes_ != null &&
maxResponseMessageBytes_ != com.google.protobuf.UInt32Value.getDefaultInstance()) {
getMaxResponseMessageBytesBuilder().mergeFrom(value);
} else {
maxResponseMessageBytes_ = value;
}
} else {
maxResponseMessageBytesBuilder_.mergeFrom(value);
}
if (maxResponseMessageBytes_ != null) {
bitField0_ |= 0x00000010;
onChanged();
}
return this;
}
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
*/
public Builder clearMaxResponseMessageBytes() {
bitField0_ = (bitField0_ & ~0x00000010);
maxResponseMessageBytes_ = null;
if (maxResponseMessageBytesBuilder_ != null) {
maxResponseMessageBytesBuilder_.dispose();
maxResponseMessageBytesBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
*/
public com.google.protobuf.UInt32Value.Builder getMaxResponseMessageBytesBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getMaxResponseMessageBytesFieldBuilder().getBuilder();
}
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
*/
public com.google.protobuf.UInt32ValueOrBuilder getMaxResponseMessageBytesOrBuilder() {
if (maxResponseMessageBytesBuilder_ != null) {
return maxResponseMessageBytesBuilder_.getMessageOrBuilder();
} else {
return maxResponseMessageBytes_ == null ?
com.google.protobuf.UInt32Value.getDefaultInstance() : maxResponseMessageBytes_;
}
}
/**
*
* The maximum allowed payload size for an individual response or object in a
* stream (server->client) in bytes. The size which is measured is the
* serialized payload after per-message compression (but before stream
* compression) in bytes. This applies both to streaming and non-streaming
* requests.
*
* The actual value used is the minimum of the value specified here and the
* value set by the application via the gRPC client API. If either one is
* not set, then the other will be used. If neither is set, then the
* built-in default is used.
*
* If a server attempts to send an object larger than this value, it will not
* be sent, and a ServerError will be sent to the client instead.
* Note that 0 is a valid value, meaning that the response message
* must be empty.
*
*
* .google.protobuf.UInt32Value max_response_message_bytes = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>
getMaxResponseMessageBytesFieldBuilder() {
if (maxResponseMessageBytesBuilder_ == null) {
maxResponseMessageBytesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.UInt32Value, com.google.protobuf.UInt32Value.Builder, com.google.protobuf.UInt32ValueOrBuilder>(
getMaxResponseMessageBytes(),
getParentForChildren(),
isClean());
maxResponseMessageBytes_ = null;
}
return maxResponseMessageBytesBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grpc.serviceconfig.MethodConfig.RetryPolicy, io.grpc.serviceconfig.MethodConfig.RetryPolicy.Builder, io.grpc.serviceconfig.MethodConfig.RetryPolicyOrBuilder> retryPolicyBuilder_;
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
* @return Whether the retryPolicy field is set.
*/
@java.lang.Override
public boolean hasRetryPolicy() {
return retryOrHedgingPolicyCase_ == 6;
}
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
* @return The retryPolicy.
*/
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.RetryPolicy getRetryPolicy() {
if (retryPolicyBuilder_ == null) {
if (retryOrHedgingPolicyCase_ == 6) {
return (io.grpc.serviceconfig.MethodConfig.RetryPolicy) retryOrHedgingPolicy_;
}
return io.grpc.serviceconfig.MethodConfig.RetryPolicy.getDefaultInstance();
} else {
if (retryOrHedgingPolicyCase_ == 6) {
return retryPolicyBuilder_.getMessage();
}
return io.grpc.serviceconfig.MethodConfig.RetryPolicy.getDefaultInstance();
}
}
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
*/
public Builder setRetryPolicy(io.grpc.serviceconfig.MethodConfig.RetryPolicy value) {
if (retryPolicyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
retryOrHedgingPolicy_ = value;
onChanged();
} else {
retryPolicyBuilder_.setMessage(value);
}
retryOrHedgingPolicyCase_ = 6;
return this;
}
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
*/
public Builder setRetryPolicy(
io.grpc.serviceconfig.MethodConfig.RetryPolicy.Builder builderForValue) {
if (retryPolicyBuilder_ == null) {
retryOrHedgingPolicy_ = builderForValue.build();
onChanged();
} else {
retryPolicyBuilder_.setMessage(builderForValue.build());
}
retryOrHedgingPolicyCase_ = 6;
return this;
}
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
*/
public Builder mergeRetryPolicy(io.grpc.serviceconfig.MethodConfig.RetryPolicy value) {
if (retryPolicyBuilder_ == null) {
if (retryOrHedgingPolicyCase_ == 6 &&
retryOrHedgingPolicy_ != io.grpc.serviceconfig.MethodConfig.RetryPolicy.getDefaultInstance()) {
retryOrHedgingPolicy_ = io.grpc.serviceconfig.MethodConfig.RetryPolicy.newBuilder((io.grpc.serviceconfig.MethodConfig.RetryPolicy) retryOrHedgingPolicy_)
.mergeFrom(value).buildPartial();
} else {
retryOrHedgingPolicy_ = value;
}
onChanged();
} else {
if (retryOrHedgingPolicyCase_ == 6) {
retryPolicyBuilder_.mergeFrom(value);
} else {
retryPolicyBuilder_.setMessage(value);
}
}
retryOrHedgingPolicyCase_ = 6;
return this;
}
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
*/
public Builder clearRetryPolicy() {
if (retryPolicyBuilder_ == null) {
if (retryOrHedgingPolicyCase_ == 6) {
retryOrHedgingPolicyCase_ = 0;
retryOrHedgingPolicy_ = null;
onChanged();
}
} else {
if (retryOrHedgingPolicyCase_ == 6) {
retryOrHedgingPolicyCase_ = 0;
retryOrHedgingPolicy_ = null;
}
retryPolicyBuilder_.clear();
}
return this;
}
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
*/
public io.grpc.serviceconfig.MethodConfig.RetryPolicy.Builder getRetryPolicyBuilder() {
return getRetryPolicyFieldBuilder().getBuilder();
}
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
*/
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.RetryPolicyOrBuilder getRetryPolicyOrBuilder() {
if ((retryOrHedgingPolicyCase_ == 6) && (retryPolicyBuilder_ != null)) {
return retryPolicyBuilder_.getMessageOrBuilder();
} else {
if (retryOrHedgingPolicyCase_ == 6) {
return (io.grpc.serviceconfig.MethodConfig.RetryPolicy) retryOrHedgingPolicy_;
}
return io.grpc.serviceconfig.MethodConfig.RetryPolicy.getDefaultInstance();
}
}
/**
* .grpc.service_config.MethodConfig.RetryPolicy retry_policy = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grpc.serviceconfig.MethodConfig.RetryPolicy, io.grpc.serviceconfig.MethodConfig.RetryPolicy.Builder, io.grpc.serviceconfig.MethodConfig.RetryPolicyOrBuilder>
getRetryPolicyFieldBuilder() {
if (retryPolicyBuilder_ == null) {
if (!(retryOrHedgingPolicyCase_ == 6)) {
retryOrHedgingPolicy_ = io.grpc.serviceconfig.MethodConfig.RetryPolicy.getDefaultInstance();
}
retryPolicyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.grpc.serviceconfig.MethodConfig.RetryPolicy, io.grpc.serviceconfig.MethodConfig.RetryPolicy.Builder, io.grpc.serviceconfig.MethodConfig.RetryPolicyOrBuilder>(
(io.grpc.serviceconfig.MethodConfig.RetryPolicy) retryOrHedgingPolicy_,
getParentForChildren(),
isClean());
retryOrHedgingPolicy_ = null;
}
retryOrHedgingPolicyCase_ = 6;
onChanged();
return retryPolicyBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
io.grpc.serviceconfig.MethodConfig.HedgingPolicy, io.grpc.serviceconfig.MethodConfig.HedgingPolicy.Builder, io.grpc.serviceconfig.MethodConfig.HedgingPolicyOrBuilder> hedgingPolicyBuilder_;
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
* @return Whether the hedgingPolicy field is set.
*/
@java.lang.Override
public boolean hasHedgingPolicy() {
return retryOrHedgingPolicyCase_ == 7;
}
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
* @return The hedgingPolicy.
*/
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.HedgingPolicy getHedgingPolicy() {
if (hedgingPolicyBuilder_ == null) {
if (retryOrHedgingPolicyCase_ == 7) {
return (io.grpc.serviceconfig.MethodConfig.HedgingPolicy) retryOrHedgingPolicy_;
}
return io.grpc.serviceconfig.MethodConfig.HedgingPolicy.getDefaultInstance();
} else {
if (retryOrHedgingPolicyCase_ == 7) {
return hedgingPolicyBuilder_.getMessage();
}
return io.grpc.serviceconfig.MethodConfig.HedgingPolicy.getDefaultInstance();
}
}
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
*/
public Builder setHedgingPolicy(io.grpc.serviceconfig.MethodConfig.HedgingPolicy value) {
if (hedgingPolicyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
retryOrHedgingPolicy_ = value;
onChanged();
} else {
hedgingPolicyBuilder_.setMessage(value);
}
retryOrHedgingPolicyCase_ = 7;
return this;
}
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
*/
public Builder setHedgingPolicy(
io.grpc.serviceconfig.MethodConfig.HedgingPolicy.Builder builderForValue) {
if (hedgingPolicyBuilder_ == null) {
retryOrHedgingPolicy_ = builderForValue.build();
onChanged();
} else {
hedgingPolicyBuilder_.setMessage(builderForValue.build());
}
retryOrHedgingPolicyCase_ = 7;
return this;
}
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
*/
public Builder mergeHedgingPolicy(io.grpc.serviceconfig.MethodConfig.HedgingPolicy value) {
if (hedgingPolicyBuilder_ == null) {
if (retryOrHedgingPolicyCase_ == 7 &&
retryOrHedgingPolicy_ != io.grpc.serviceconfig.MethodConfig.HedgingPolicy.getDefaultInstance()) {
retryOrHedgingPolicy_ = io.grpc.serviceconfig.MethodConfig.HedgingPolicy.newBuilder((io.grpc.serviceconfig.MethodConfig.HedgingPolicy) retryOrHedgingPolicy_)
.mergeFrom(value).buildPartial();
} else {
retryOrHedgingPolicy_ = value;
}
onChanged();
} else {
if (retryOrHedgingPolicyCase_ == 7) {
hedgingPolicyBuilder_.mergeFrom(value);
} else {
hedgingPolicyBuilder_.setMessage(value);
}
}
retryOrHedgingPolicyCase_ = 7;
return this;
}
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
*/
public Builder clearHedgingPolicy() {
if (hedgingPolicyBuilder_ == null) {
if (retryOrHedgingPolicyCase_ == 7) {
retryOrHedgingPolicyCase_ = 0;
retryOrHedgingPolicy_ = null;
onChanged();
}
} else {
if (retryOrHedgingPolicyCase_ == 7) {
retryOrHedgingPolicyCase_ = 0;
retryOrHedgingPolicy_ = null;
}
hedgingPolicyBuilder_.clear();
}
return this;
}
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
*/
public io.grpc.serviceconfig.MethodConfig.HedgingPolicy.Builder getHedgingPolicyBuilder() {
return getHedgingPolicyFieldBuilder().getBuilder();
}
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
*/
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig.HedgingPolicyOrBuilder getHedgingPolicyOrBuilder() {
if ((retryOrHedgingPolicyCase_ == 7) && (hedgingPolicyBuilder_ != null)) {
return hedgingPolicyBuilder_.getMessageOrBuilder();
} else {
if (retryOrHedgingPolicyCase_ == 7) {
return (io.grpc.serviceconfig.MethodConfig.HedgingPolicy) retryOrHedgingPolicy_;
}
return io.grpc.serviceconfig.MethodConfig.HedgingPolicy.getDefaultInstance();
}
}
/**
* .grpc.service_config.MethodConfig.HedgingPolicy hedging_policy = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
io.grpc.serviceconfig.MethodConfig.HedgingPolicy, io.grpc.serviceconfig.MethodConfig.HedgingPolicy.Builder, io.grpc.serviceconfig.MethodConfig.HedgingPolicyOrBuilder>
getHedgingPolicyFieldBuilder() {
if (hedgingPolicyBuilder_ == null) {
if (!(retryOrHedgingPolicyCase_ == 7)) {
retryOrHedgingPolicy_ = io.grpc.serviceconfig.MethodConfig.HedgingPolicy.getDefaultInstance();
}
hedgingPolicyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
io.grpc.serviceconfig.MethodConfig.HedgingPolicy, io.grpc.serviceconfig.MethodConfig.HedgingPolicy.Builder, io.grpc.serviceconfig.MethodConfig.HedgingPolicyOrBuilder>(
(io.grpc.serviceconfig.MethodConfig.HedgingPolicy) retryOrHedgingPolicy_,
getParentForChildren(),
isClean());
retryOrHedgingPolicy_ = null;
}
retryOrHedgingPolicyCase_ = 7;
onChanged();
return hedgingPolicyBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:grpc.service_config.MethodConfig)
}
// @@protoc_insertion_point(class_scope:grpc.service_config.MethodConfig)
private static final io.grpc.serviceconfig.MethodConfig DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new io.grpc.serviceconfig.MethodConfig();
}
public static io.grpc.serviceconfig.MethodConfig getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MethodConfig parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public io.grpc.serviceconfig.MethodConfig getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy