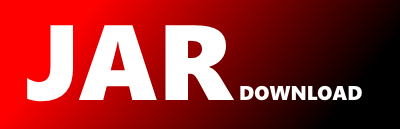
com.google.api.gax.httpjson.AutoValue_ApiMethodDescriptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gax-httpjson Show documentation
Show all versions of gax-httpjson Show documentation
Google Api eXtensions for Java
package com.google.api.gax.httpjson;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ApiMethodDescriptor extends ApiMethodDescriptor {
private final String fullMethodName;
private final HttpRequestFormatter requestFormatter;
private final HttpResponseParser responseParser;
@Nullable
private final String httpMethod;
@Nullable
private final OperationSnapshotFactory operationSnapshotFactory;
@Nullable
private final PollingRequestFactory pollingRequestFactory;
private final ApiMethodDescriptor.MethodType type;
private AutoValue_ApiMethodDescriptor(
String fullMethodName,
HttpRequestFormatter requestFormatter,
HttpResponseParser responseParser,
@Nullable String httpMethod,
@Nullable OperationSnapshotFactory operationSnapshotFactory,
@Nullable PollingRequestFactory pollingRequestFactory,
ApiMethodDescriptor.MethodType type) {
this.fullMethodName = fullMethodName;
this.requestFormatter = requestFormatter;
this.responseParser = responseParser;
this.httpMethod = httpMethod;
this.operationSnapshotFactory = operationSnapshotFactory;
this.pollingRequestFactory = pollingRequestFactory;
this.type = type;
}
@Override
public String getFullMethodName() {
return fullMethodName;
}
@Override
public HttpRequestFormatter getRequestFormatter() {
return requestFormatter;
}
@Override
public HttpResponseParser getResponseParser() {
return responseParser;
}
@Nullable
@Override
public String getHttpMethod() {
return httpMethod;
}
@Nullable
@Override
public OperationSnapshotFactory getOperationSnapshotFactory() {
return operationSnapshotFactory;
}
@Nullable
@Override
public PollingRequestFactory getPollingRequestFactory() {
return pollingRequestFactory;
}
@Override
public ApiMethodDescriptor.MethodType getType() {
return type;
}
@Override
public String toString() {
return "ApiMethodDescriptor{"
+ "fullMethodName=" + fullMethodName + ", "
+ "requestFormatter=" + requestFormatter + ", "
+ "responseParser=" + responseParser + ", "
+ "httpMethod=" + httpMethod + ", "
+ "operationSnapshotFactory=" + operationSnapshotFactory + ", "
+ "pollingRequestFactory=" + pollingRequestFactory + ", "
+ "type=" + type
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ApiMethodDescriptor) {
ApiMethodDescriptor, ?> that = (ApiMethodDescriptor, ?>) o;
return this.fullMethodName.equals(that.getFullMethodName())
&& this.requestFormatter.equals(that.getRequestFormatter())
&& this.responseParser.equals(that.getResponseParser())
&& (this.httpMethod == null ? that.getHttpMethod() == null : this.httpMethod.equals(that.getHttpMethod()))
&& (this.operationSnapshotFactory == null ? that.getOperationSnapshotFactory() == null : this.operationSnapshotFactory.equals(that.getOperationSnapshotFactory()))
&& (this.pollingRequestFactory == null ? that.getPollingRequestFactory() == null : this.pollingRequestFactory.equals(that.getPollingRequestFactory()))
&& this.type.equals(that.getType());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= fullMethodName.hashCode();
h$ *= 1000003;
h$ ^= requestFormatter.hashCode();
h$ *= 1000003;
h$ ^= responseParser.hashCode();
h$ *= 1000003;
h$ ^= (httpMethod == null) ? 0 : httpMethod.hashCode();
h$ *= 1000003;
h$ ^= (operationSnapshotFactory == null) ? 0 : operationSnapshotFactory.hashCode();
h$ *= 1000003;
h$ ^= (pollingRequestFactory == null) ? 0 : pollingRequestFactory.hashCode();
h$ *= 1000003;
h$ ^= type.hashCode();
return h$;
}
@Override
public ApiMethodDescriptor.Builder toBuilder() {
return new AutoValue_ApiMethodDescriptor.Builder(this);
}
static final class Builder extends ApiMethodDescriptor.Builder {
private String fullMethodName;
private HttpRequestFormatter requestFormatter;
private HttpResponseParser responseParser;
private String httpMethod;
private OperationSnapshotFactory operationSnapshotFactory;
private PollingRequestFactory pollingRequestFactory;
private ApiMethodDescriptor.MethodType type;
Builder() {
}
Builder(ApiMethodDescriptor source) {
this.fullMethodName = source.getFullMethodName();
this.requestFormatter = source.getRequestFormatter();
this.responseParser = source.getResponseParser();
this.httpMethod = source.getHttpMethod();
this.operationSnapshotFactory = source.getOperationSnapshotFactory();
this.pollingRequestFactory = source.getPollingRequestFactory();
this.type = source.getType();
}
@Override
public ApiMethodDescriptor.Builder setFullMethodName(String fullMethodName) {
if (fullMethodName == null) {
throw new NullPointerException("Null fullMethodName");
}
this.fullMethodName = fullMethodName;
return this;
}
@Override
public ApiMethodDescriptor.Builder setRequestFormatter(HttpRequestFormatter requestFormatter) {
if (requestFormatter == null) {
throw new NullPointerException("Null requestFormatter");
}
this.requestFormatter = requestFormatter;
return this;
}
@Override
public HttpRequestFormatter getRequestFormatter() {
if (this.requestFormatter == null) {
throw new IllegalStateException("Property \"requestFormatter\" has not been set");
}
return requestFormatter;
}
@Override
public ApiMethodDescriptor.Builder setResponseParser(HttpResponseParser responseParser) {
if (responseParser == null) {
throw new NullPointerException("Null responseParser");
}
this.responseParser = responseParser;
return this;
}
@Override
public ApiMethodDescriptor.Builder setHttpMethod(String httpMethod) {
this.httpMethod = httpMethod;
return this;
}
@Override
public ApiMethodDescriptor.Builder setOperationSnapshotFactory(OperationSnapshotFactory operationSnapshotFactory) {
this.operationSnapshotFactory = operationSnapshotFactory;
return this;
}
@Override
public ApiMethodDescriptor.Builder setPollingRequestFactory(PollingRequestFactory pollingRequestFactory) {
this.pollingRequestFactory = pollingRequestFactory;
return this;
}
@Override
public ApiMethodDescriptor.Builder setType(ApiMethodDescriptor.MethodType type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public ApiMethodDescriptor build() {
if (this.fullMethodName == null
|| this.requestFormatter == null
|| this.responseParser == null
|| this.type == null) {
StringBuilder missing = new StringBuilder();
if (this.fullMethodName == null) {
missing.append(" fullMethodName");
}
if (this.requestFormatter == null) {
missing.append(" requestFormatter");
}
if (this.responseParser == null) {
missing.append(" responseParser");
}
if (this.type == null) {
missing.append(" type");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ApiMethodDescriptor(
this.fullMethodName,
this.requestFormatter,
this.responseParser,
this.httpMethod,
this.operationSnapshotFactory,
this.pollingRequestFactory,
this.type);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy