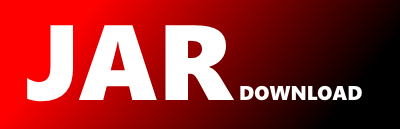
com.google.api.gax.httpjson.AutoValue_HttpJsonCallOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gax-httpjson Show documentation
Show all versions of gax-httpjson Show documentation
Google Api eXtensions for Java
package com.google.api.gax.httpjson;
import com.google.auth.Credentials;
import com.google.protobuf.TypeRegistry;
import java.time.Duration;
import java.time.Instant;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_HttpJsonCallOptions extends HttpJsonCallOptions {
@Nullable
private final Duration timeoutDuration;
@Nullable
private final Instant deadlineInstant;
@Nullable
private final Credentials credentials;
@Nullable
private final TypeRegistry typeRegistry;
private AutoValue_HttpJsonCallOptions(
@Nullable Duration timeoutDuration,
@Nullable Instant deadlineInstant,
@Nullable Credentials credentials,
@Nullable TypeRegistry typeRegistry) {
this.timeoutDuration = timeoutDuration;
this.deadlineInstant = deadlineInstant;
this.credentials = credentials;
this.typeRegistry = typeRegistry;
}
@Nullable
@Override
public Duration getTimeoutDuration() {
return timeoutDuration;
}
@Nullable
@Override
public Instant getDeadlineInstant() {
return deadlineInstant;
}
@Nullable
@Override
public Credentials getCredentials() {
return credentials;
}
@Nullable
@Override
public TypeRegistry getTypeRegistry() {
return typeRegistry;
}
@Override
public String toString() {
return "HttpJsonCallOptions{"
+ "timeoutDuration=" + timeoutDuration + ", "
+ "deadlineInstant=" + deadlineInstant + ", "
+ "credentials=" + credentials + ", "
+ "typeRegistry=" + typeRegistry
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof HttpJsonCallOptions) {
HttpJsonCallOptions that = (HttpJsonCallOptions) o;
return (this.timeoutDuration == null ? that.getTimeoutDuration() == null : this.timeoutDuration.equals(that.getTimeoutDuration()))
&& (this.deadlineInstant == null ? that.getDeadlineInstant() == null : this.deadlineInstant.equals(that.getDeadlineInstant()))
&& (this.credentials == null ? that.getCredentials() == null : this.credentials.equals(that.getCredentials()))
&& (this.typeRegistry == null ? that.getTypeRegistry() == null : this.typeRegistry.equals(that.getTypeRegistry()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (timeoutDuration == null) ? 0 : timeoutDuration.hashCode();
h$ *= 1000003;
h$ ^= (deadlineInstant == null) ? 0 : deadlineInstant.hashCode();
h$ *= 1000003;
h$ ^= (credentials == null) ? 0 : credentials.hashCode();
h$ *= 1000003;
h$ ^= (typeRegistry == null) ? 0 : typeRegistry.hashCode();
return h$;
}
@Override
public HttpJsonCallOptions.Builder toBuilder() {
return new AutoValue_HttpJsonCallOptions.Builder(this);
}
static final class Builder extends HttpJsonCallOptions.Builder {
private Duration timeoutDuration;
private Instant deadlineInstant;
private Credentials credentials;
private TypeRegistry typeRegistry;
Builder() {
}
Builder(HttpJsonCallOptions source) {
this.timeoutDuration = source.getTimeoutDuration();
this.deadlineInstant = source.getDeadlineInstant();
this.credentials = source.getCredentials();
this.typeRegistry = source.getTypeRegistry();
}
@Override
public HttpJsonCallOptions.Builder setTimeoutDuration(Duration timeoutDuration) {
this.timeoutDuration = timeoutDuration;
return this;
}
@Override
public HttpJsonCallOptions.Builder setDeadlineInstant(Instant deadlineInstant) {
this.deadlineInstant = deadlineInstant;
return this;
}
@Override
public HttpJsonCallOptions.Builder setCredentials(Credentials credentials) {
this.credentials = credentials;
return this;
}
@Override
public HttpJsonCallOptions.Builder setTypeRegistry(TypeRegistry typeRegistry) {
this.typeRegistry = typeRegistry;
return this;
}
@Override
public HttpJsonCallOptions build() {
return new AutoValue_HttpJsonCallOptions(
this.timeoutDuration,
this.deadlineInstant,
this.credentials,
this.typeRegistry);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy