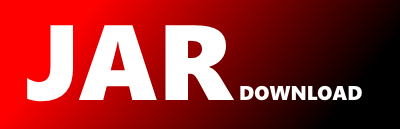
com.google.api.services.accessapproval.v1.AccessApproval Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.accessapproval.v1;
/**
* Service definition for AccessApproval (v1).
*
*
* An API for controlling access to data by Google personnel.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link AccessApprovalRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class AccessApproval extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Access Approval API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://accessapproval.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://accessapproval.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public AccessApproval(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
AccessApproval(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Folders collection.
*
* The typical use is:
*
* {@code AccessApproval accessapproval = new AccessApproval(...);}
* {@code AccessApproval.Folders.List request = accessapproval.folders().list(parameters ...)}
*
*
* @return the resource collection
*/
public Folders folders() {
return new Folders();
}
/**
* The "folders" collection of methods.
*/
public class Folders {
/**
* Deletes the settings associated with a project, folder, or organization. This will have the
* effect of disabling Access Approval for the project, folder, or organization, but only if all
* ancestors also have Access Approval disabled. If Access Approval is enabled at a higher level of
* the hierarchy, then Access Approval will still be enabled at this level as the settings are
* inherited.
*
* Create a request for the method "folders.deleteAccessApprovalSettings".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link DeleteAccessApprovalSettings#execute()} method to invoke the
* remote operation.
*
* @param name Name of the AccessApprovalSettings to delete.
* @return the request
*/
public DeleteAccessApprovalSettings deleteAccessApprovalSettings(java.lang.String name) throws java.io.IOException {
DeleteAccessApprovalSettings result = new DeleteAccessApprovalSettings(name);
initialize(result);
return result;
}
public class DeleteAccessApprovalSettings extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/accessApprovalSettings$");
/**
* Deletes the settings associated with a project, folder, or organization. This will have the
* effect of disabling Access Approval for the project, folder, or organization, but only if all
* ancestors also have Access Approval disabled. If Access Approval is enabled at a higher level
* of the hierarchy, then Access Approval will still be enabled at this level as the settings are
* inherited.
*
* Create a request for the method "folders.deleteAccessApprovalSettings".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link DeleteAccessApprovalSettings#execute()} method to invoke
* the remote operation. {@link DeleteAccessApprovalSettings#initialize(com.google.api.client.
* googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param name Name of the AccessApprovalSettings to delete.
* @since 1.13
*/
protected DeleteAccessApprovalSettings(java.lang.String name) {
super(AccessApproval.this, "DELETE", REST_PATH, null, com.google.api.services.accessapproval.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/accessApprovalSettings$");
}
}
@Override
public DeleteAccessApprovalSettings set$Xgafv(java.lang.String $Xgafv) {
return (DeleteAccessApprovalSettings) super.set$Xgafv($Xgafv);
}
@Override
public DeleteAccessApprovalSettings setAccessToken(java.lang.String accessToken) {
return (DeleteAccessApprovalSettings) super.setAccessToken(accessToken);
}
@Override
public DeleteAccessApprovalSettings setAlt(java.lang.String alt) {
return (DeleteAccessApprovalSettings) super.setAlt(alt);
}
@Override
public DeleteAccessApprovalSettings setCallback(java.lang.String callback) {
return (DeleteAccessApprovalSettings) super.setCallback(callback);
}
@Override
public DeleteAccessApprovalSettings setFields(java.lang.String fields) {
return (DeleteAccessApprovalSettings) super.setFields(fields);
}
@Override
public DeleteAccessApprovalSettings setKey(java.lang.String key) {
return (DeleteAccessApprovalSettings) super.setKey(key);
}
@Override
public DeleteAccessApprovalSettings setOauthToken(java.lang.String oauthToken) {
return (DeleteAccessApprovalSettings) super.setOauthToken(oauthToken);
}
@Override
public DeleteAccessApprovalSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DeleteAccessApprovalSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public DeleteAccessApprovalSettings setQuotaUser(java.lang.String quotaUser) {
return (DeleteAccessApprovalSettings) super.setQuotaUser(quotaUser);
}
@Override
public DeleteAccessApprovalSettings setUploadType(java.lang.String uploadType) {
return (DeleteAccessApprovalSettings) super.setUploadType(uploadType);
}
@Override
public DeleteAccessApprovalSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (DeleteAccessApprovalSettings) super.setUploadProtocol(uploadProtocol);
}
/** Name of the AccessApprovalSettings to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the AccessApprovalSettings to delete.
*/
public java.lang.String getName() {
return name;
}
/** Name of the AccessApprovalSettings to delete. */
public DeleteAccessApprovalSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/accessApprovalSettings$");
}
this.name = name;
return this;
}
@Override
public DeleteAccessApprovalSettings set(String parameterName, Object value) {
return (DeleteAccessApprovalSettings) super.set(parameterName, value);
}
}
/**
* Gets the settings associated with a project, folder, or organization.
*
* Create a request for the method "folders.getAccessApprovalSettings".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link GetAccessApprovalSettings#execute()} method to invoke the
* remote operation.
*
* @param name The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
* @return the request
*/
public GetAccessApprovalSettings getAccessApprovalSettings(java.lang.String name) throws java.io.IOException {
GetAccessApprovalSettings result = new GetAccessApprovalSettings(name);
initialize(result);
return result;
}
public class GetAccessApprovalSettings extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/accessApprovalSettings$");
/**
* Gets the settings associated with a project, folder, or organization.
*
* Create a request for the method "folders.getAccessApprovalSettings".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link GetAccessApprovalSettings#execute()} method to invoke the
* remote operation. {@link GetAccessApprovalSettings#initialize(com.google.api.client.googlea
* pis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param name The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
* @since 1.13
*/
protected GetAccessApprovalSettings(java.lang.String name) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/accessApprovalSettings$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetAccessApprovalSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetAccessApprovalSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetAccessApprovalSettings setAccessToken(java.lang.String accessToken) {
return (GetAccessApprovalSettings) super.setAccessToken(accessToken);
}
@Override
public GetAccessApprovalSettings setAlt(java.lang.String alt) {
return (GetAccessApprovalSettings) super.setAlt(alt);
}
@Override
public GetAccessApprovalSettings setCallback(java.lang.String callback) {
return (GetAccessApprovalSettings) super.setCallback(callback);
}
@Override
public GetAccessApprovalSettings setFields(java.lang.String fields) {
return (GetAccessApprovalSettings) super.setFields(fields);
}
@Override
public GetAccessApprovalSettings setKey(java.lang.String key) {
return (GetAccessApprovalSettings) super.setKey(key);
}
@Override
public GetAccessApprovalSettings setOauthToken(java.lang.String oauthToken) {
return (GetAccessApprovalSettings) super.setOauthToken(oauthToken);
}
@Override
public GetAccessApprovalSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetAccessApprovalSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetAccessApprovalSettings setQuotaUser(java.lang.String quotaUser) {
return (GetAccessApprovalSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetAccessApprovalSettings setUploadType(java.lang.String uploadType) {
return (GetAccessApprovalSettings) super.setUploadType(uploadType);
}
@Override
public GetAccessApprovalSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetAccessApprovalSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the AccessApprovalSettings to retrieve. Format:
"{projects|folders|organizations}/{id}/accessApprovalSettings"
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
*/
public GetAccessApprovalSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/accessApprovalSettings$");
}
this.name = name;
return this;
}
@Override
public GetAccessApprovalSettings set(String parameterName, Object value) {
return (GetAccessApprovalSettings) super.set(parameterName, value);
}
}
/**
* Retrieves the service account that is used by Access Approval to access KMS keys for signing
* approved approval requests.
*
* Create a request for the method "folders.getServiceAccount".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link GetServiceAccount#execute()} method to invoke the remote
* operation.
*
* @param name Name of the AccessApprovalServiceAccount to retrieve.
* @return the request
*/
public GetServiceAccount getServiceAccount(java.lang.String name) throws java.io.IOException {
GetServiceAccount result = new GetServiceAccount(name);
initialize(result);
return result;
}
public class GetServiceAccount extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/serviceAccount$");
/**
* Retrieves the service account that is used by Access Approval to access KMS keys for signing
* approved approval requests.
*
* Create a request for the method "folders.getServiceAccount".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link GetServiceAccount#execute()} method to invoke the remote
* operation. {@link GetServiceAccount#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Name of the AccessApprovalServiceAccount to retrieve.
* @since 1.13
*/
protected GetServiceAccount(java.lang.String name) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.AccessApprovalServiceAccount.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/serviceAccount$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetServiceAccount set$Xgafv(java.lang.String $Xgafv) {
return (GetServiceAccount) super.set$Xgafv($Xgafv);
}
@Override
public GetServiceAccount setAccessToken(java.lang.String accessToken) {
return (GetServiceAccount) super.setAccessToken(accessToken);
}
@Override
public GetServiceAccount setAlt(java.lang.String alt) {
return (GetServiceAccount) super.setAlt(alt);
}
@Override
public GetServiceAccount setCallback(java.lang.String callback) {
return (GetServiceAccount) super.setCallback(callback);
}
@Override
public GetServiceAccount setFields(java.lang.String fields) {
return (GetServiceAccount) super.setFields(fields);
}
@Override
public GetServiceAccount setKey(java.lang.String key) {
return (GetServiceAccount) super.setKey(key);
}
@Override
public GetServiceAccount setOauthToken(java.lang.String oauthToken) {
return (GetServiceAccount) super.setOauthToken(oauthToken);
}
@Override
public GetServiceAccount setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetServiceAccount) super.setPrettyPrint(prettyPrint);
}
@Override
public GetServiceAccount setQuotaUser(java.lang.String quotaUser) {
return (GetServiceAccount) super.setQuotaUser(quotaUser);
}
@Override
public GetServiceAccount setUploadType(java.lang.String uploadType) {
return (GetServiceAccount) super.setUploadType(uploadType);
}
@Override
public GetServiceAccount setUploadProtocol(java.lang.String uploadProtocol) {
return (GetServiceAccount) super.setUploadProtocol(uploadProtocol);
}
/** Name of the AccessApprovalServiceAccount to retrieve. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the AccessApprovalServiceAccount to retrieve.
*/
public java.lang.String getName() {
return name;
}
/** Name of the AccessApprovalServiceAccount to retrieve. */
public GetServiceAccount setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/serviceAccount$");
}
this.name = name;
return this;
}
@Override
public GetServiceAccount set(String parameterName, Object value) {
return (GetServiceAccount) super.set(parameterName, value);
}
}
/**
* Updates the settings associated with a project, folder, or organization. Settings to update are
* determined by the value of field_mask.
*
* Create a request for the method "folders.updateAccessApprovalSettings".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link UpdateAccessApprovalSettings#execute()} method to invoke the
* remote operation.
*
* @param name The resource name of the settings. Format is one of: * "projects/{project}/accessApprovalSettings" *
* "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
* @param content the {@link com.google.api.services.accessapproval.v1.model.AccessApprovalSettings}
* @return the request
*/
public UpdateAccessApprovalSettings updateAccessApprovalSettings(java.lang.String name, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings content) throws java.io.IOException {
UpdateAccessApprovalSettings result = new UpdateAccessApprovalSettings(name, content);
initialize(result);
return result;
}
public class UpdateAccessApprovalSettings extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/accessApprovalSettings$");
/**
* Updates the settings associated with a project, folder, or organization. Settings to update are
* determined by the value of field_mask.
*
* Create a request for the method "folders.updateAccessApprovalSettings".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link UpdateAccessApprovalSettings#execute()} method to invoke
* the remote operation. {@link UpdateAccessApprovalSettings#initialize(com.google.api.client.
* googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param name The resource name of the settings. Format is one of: * "projects/{project}/accessApprovalSettings" *
* "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
* @param content the {@link com.google.api.services.accessapproval.v1.model.AccessApprovalSettings}
* @since 1.13
*/
protected UpdateAccessApprovalSettings(java.lang.String name, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings content) {
super(AccessApproval.this, "PATCH", REST_PATH, content, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/accessApprovalSettings$");
}
}
@Override
public UpdateAccessApprovalSettings set$Xgafv(java.lang.String $Xgafv) {
return (UpdateAccessApprovalSettings) super.set$Xgafv($Xgafv);
}
@Override
public UpdateAccessApprovalSettings setAccessToken(java.lang.String accessToken) {
return (UpdateAccessApprovalSettings) super.setAccessToken(accessToken);
}
@Override
public UpdateAccessApprovalSettings setAlt(java.lang.String alt) {
return (UpdateAccessApprovalSettings) super.setAlt(alt);
}
@Override
public UpdateAccessApprovalSettings setCallback(java.lang.String callback) {
return (UpdateAccessApprovalSettings) super.setCallback(callback);
}
@Override
public UpdateAccessApprovalSettings setFields(java.lang.String fields) {
return (UpdateAccessApprovalSettings) super.setFields(fields);
}
@Override
public UpdateAccessApprovalSettings setKey(java.lang.String key) {
return (UpdateAccessApprovalSettings) super.setKey(key);
}
@Override
public UpdateAccessApprovalSettings setOauthToken(java.lang.String oauthToken) {
return (UpdateAccessApprovalSettings) super.setOauthToken(oauthToken);
}
@Override
public UpdateAccessApprovalSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateAccessApprovalSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateAccessApprovalSettings setQuotaUser(java.lang.String quotaUser) {
return (UpdateAccessApprovalSettings) super.setQuotaUser(quotaUser);
}
@Override
public UpdateAccessApprovalSettings setUploadType(java.lang.String uploadType) {
return (UpdateAccessApprovalSettings) super.setUploadType(uploadType);
}
@Override
public UpdateAccessApprovalSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateAccessApprovalSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* The resource name of the settings. Format is one of: *
* "projects/{project}/accessApprovalSettings" * "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource name of the settings. Format is one of: * "projects/{project}/accessApprovalSettings"
* "folders/{folder}/accessApprovalSettings" * "organizations/{organization}/accessApprovalSettings"
*/
public java.lang.String getName() {
return name;
}
/**
* The resource name of the settings. Format is one of: *
* "projects/{project}/accessApprovalSettings" * "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
*/
public UpdateAccessApprovalSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/accessApprovalSettings$");
}
this.name = name;
return this;
}
/**
* The update mask applies to the settings. Only the top level fields of
* AccessApprovalSettings (notification_emails & enrolled_services) are supported. For each
* field, if it is included, the currently stored value will be entirely overwritten with the
* value of the field passed in this request. For the `FieldMask` definition, see
* https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#fieldmask If
* this field is left unset, only the notification_emails field will be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The update mask applies to the settings. Only the top level fields of AccessApprovalSettings
(notification_emails & enrolled_services) are supported. For each field, if it is included, the
currently stored value will be entirely overwritten with the value of the field passed in this
request. For the `FieldMask` definition, see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#fieldmask If this field is left unset, only the
notification_emails field will be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The update mask applies to the settings. Only the top level fields of
* AccessApprovalSettings (notification_emails & enrolled_services) are supported. For each
* field, if it is included, the currently stored value will be entirely overwritten with the
* value of the field passed in this request. For the `FieldMask` definition, see
* https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#fieldmask If
* this field is left unset, only the notification_emails field will be updated.
*/
public UpdateAccessApprovalSettings setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateAccessApprovalSettings set(String parameterName, Object value) {
return (UpdateAccessApprovalSettings) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the ApprovalRequests collection.
*
* The typical use is:
*
* {@code AccessApproval accessapproval = new AccessApproval(...);}
* {@code AccessApproval.ApprovalRequests.List request = accessapproval.approvalRequests().list(parameters ...)}
*
*
* @return the resource collection
*/
public ApprovalRequests approvalRequests() {
return new ApprovalRequests();
}
/**
* The "approvalRequests" collection of methods.
*/
public class ApprovalRequests {
/**
* Approves a request and returns the updated ApprovalRequest. Returns NOT_FOUND if the request does
* not exist. Returns FAILED_PRECONDITION if the request exists but is not in a pending state.
*
* Create a request for the method "approvalRequests.approve".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Approve#execute()} method to invoke the remote operation.
*
* @param name Name of the approval request to approve.
* @param content the {@link com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage}
* @return the request
*/
public Approve approve(java.lang.String name, com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage content) throws java.io.IOException {
Approve result = new Approve(name, content);
initialize(result);
return result;
}
public class Approve extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}:approve";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/approvalRequests/[^/]+$");
/**
* Approves a request and returns the updated ApprovalRequest. Returns NOT_FOUND if the request
* does not exist. Returns FAILED_PRECONDITION if the request exists but is not in a pending
* state.
*
* Create a request for the method "approvalRequests.approve".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Approve#execute()} method to invoke the remote operation.
* {@link
* Approve#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the approval request to approve.
* @param content the {@link com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage}
* @since 1.13
*/
protected Approve(java.lang.String name, com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage content) {
super(AccessApproval.this, "POST", REST_PATH, content, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public Approve set$Xgafv(java.lang.String $Xgafv) {
return (Approve) super.set$Xgafv($Xgafv);
}
@Override
public Approve setAccessToken(java.lang.String accessToken) {
return (Approve) super.setAccessToken(accessToken);
}
@Override
public Approve setAlt(java.lang.String alt) {
return (Approve) super.setAlt(alt);
}
@Override
public Approve setCallback(java.lang.String callback) {
return (Approve) super.setCallback(callback);
}
@Override
public Approve setFields(java.lang.String fields) {
return (Approve) super.setFields(fields);
}
@Override
public Approve setKey(java.lang.String key) {
return (Approve) super.setKey(key);
}
@Override
public Approve setOauthToken(java.lang.String oauthToken) {
return (Approve) super.setOauthToken(oauthToken);
}
@Override
public Approve setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Approve) super.setPrettyPrint(prettyPrint);
}
@Override
public Approve setQuotaUser(java.lang.String quotaUser) {
return (Approve) super.setQuotaUser(quotaUser);
}
@Override
public Approve setUploadType(java.lang.String uploadType) {
return (Approve) super.setUploadType(uploadType);
}
@Override
public Approve setUploadProtocol(java.lang.String uploadProtocol) {
return (Approve) super.setUploadProtocol(uploadProtocol);
}
/** Name of the approval request to approve. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the approval request to approve.
*/
public java.lang.String getName() {
return name;
}
/** Name of the approval request to approve. */
public Approve setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Approve set(String parameterName, Object value) {
return (Approve) super.set(parameterName, value);
}
}
/**
* Dismisses a request. Returns the updated ApprovalRequest. NOTE: This does not deny access to the
* resource if another request has been made and approved. It is equivalent in effect to ignoring
* the request altogether. Returns NOT_FOUND if the request does not exist. Returns
* FAILED_PRECONDITION if the request exists but is not in a pending state.
*
* Create a request for the method "approvalRequests.dismiss".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
*
* @param name Name of the ApprovalRequest to dismiss.
* @param content the {@link com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage}
* @return the request
*/
public Dismiss dismiss(java.lang.String name, com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage content) throws java.io.IOException {
Dismiss result = new Dismiss(name, content);
initialize(result);
return result;
}
public class Dismiss extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}:dismiss";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/approvalRequests/[^/]+$");
/**
* Dismisses a request. Returns the updated ApprovalRequest. NOTE: This does not deny access to
* the resource if another request has been made and approved. It is equivalent in effect to
* ignoring the request altogether. Returns NOT_FOUND if the request does not exist. Returns
* FAILED_PRECONDITION if the request exists but is not in a pending state.
*
* Create a request for the method "approvalRequests.dismiss".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
* {@link
* Dismiss#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the ApprovalRequest to dismiss.
* @param content the {@link com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage}
* @since 1.13
*/
protected Dismiss(java.lang.String name, com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage content) {
super(AccessApproval.this, "POST", REST_PATH, content, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public Dismiss set$Xgafv(java.lang.String $Xgafv) {
return (Dismiss) super.set$Xgafv($Xgafv);
}
@Override
public Dismiss setAccessToken(java.lang.String accessToken) {
return (Dismiss) super.setAccessToken(accessToken);
}
@Override
public Dismiss setAlt(java.lang.String alt) {
return (Dismiss) super.setAlt(alt);
}
@Override
public Dismiss setCallback(java.lang.String callback) {
return (Dismiss) super.setCallback(callback);
}
@Override
public Dismiss setFields(java.lang.String fields) {
return (Dismiss) super.setFields(fields);
}
@Override
public Dismiss setKey(java.lang.String key) {
return (Dismiss) super.setKey(key);
}
@Override
public Dismiss setOauthToken(java.lang.String oauthToken) {
return (Dismiss) super.setOauthToken(oauthToken);
}
@Override
public Dismiss setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Dismiss) super.setPrettyPrint(prettyPrint);
}
@Override
public Dismiss setQuotaUser(java.lang.String quotaUser) {
return (Dismiss) super.setQuotaUser(quotaUser);
}
@Override
public Dismiss setUploadType(java.lang.String uploadType) {
return (Dismiss) super.setUploadType(uploadType);
}
@Override
public Dismiss setUploadProtocol(java.lang.String uploadProtocol) {
return (Dismiss) super.setUploadProtocol(uploadProtocol);
}
/** Name of the ApprovalRequest to dismiss. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the ApprovalRequest to dismiss.
*/
public java.lang.String getName() {
return name;
}
/** Name of the ApprovalRequest to dismiss. */
public Dismiss setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Dismiss set(String parameterName, Object value) {
return (Dismiss) super.set(parameterName, value);
}
}
/**
* Gets an approval request. Returns NOT_FOUND if the request does not exist.
*
* Create a request for the method "approvalRequests.get".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/approvalRequests/[^/]+$");
/**
* Gets an approval request. Returns NOT_FOUND if the request does not exist.
*
* Create a request for the method "approvalRequests.get".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the approval request to retrieve. Format:
"{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Invalidates an existing ApprovalRequest. Returns the updated ApprovalRequest. NOTE: This does not
* deny access to the resource if another request has been made and approved. It only invalidates a
* single approval. Returns FAILED_PRECONDITION if the request exists but is not in an approved
* state.
*
* Create a request for the method "approvalRequests.invalidate".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Invalidate#execute()} method to invoke the remote operation.
*
* @param name Name of the ApprovalRequest to invalidate.
* @param content the {@link com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage}
* @return the request
*/
public Invalidate invalidate(java.lang.String name, com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage content) throws java.io.IOException {
Invalidate result = new Invalidate(name, content);
initialize(result);
return result;
}
public class Invalidate extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}:invalidate";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+/approvalRequests/[^/]+$");
/**
* Invalidates an existing ApprovalRequest. Returns the updated ApprovalRequest. NOTE: This does
* not deny access to the resource if another request has been made and approved. It only
* invalidates a single approval. Returns FAILED_PRECONDITION if the request exists but is not in
* an approved state.
*
* Create a request for the method "approvalRequests.invalidate".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Invalidate#execute()} method to invoke the remote
* operation. {@link
* Invalidate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the ApprovalRequest to invalidate.
* @param content the {@link com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage}
* @since 1.13
*/
protected Invalidate(java.lang.String name, com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage content) {
super(AccessApproval.this, "POST", REST_PATH, content, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public Invalidate set$Xgafv(java.lang.String $Xgafv) {
return (Invalidate) super.set$Xgafv($Xgafv);
}
@Override
public Invalidate setAccessToken(java.lang.String accessToken) {
return (Invalidate) super.setAccessToken(accessToken);
}
@Override
public Invalidate setAlt(java.lang.String alt) {
return (Invalidate) super.setAlt(alt);
}
@Override
public Invalidate setCallback(java.lang.String callback) {
return (Invalidate) super.setCallback(callback);
}
@Override
public Invalidate setFields(java.lang.String fields) {
return (Invalidate) super.setFields(fields);
}
@Override
public Invalidate setKey(java.lang.String key) {
return (Invalidate) super.setKey(key);
}
@Override
public Invalidate setOauthToken(java.lang.String oauthToken) {
return (Invalidate) super.setOauthToken(oauthToken);
}
@Override
public Invalidate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Invalidate) super.setPrettyPrint(prettyPrint);
}
@Override
public Invalidate setQuotaUser(java.lang.String quotaUser) {
return (Invalidate) super.setQuotaUser(quotaUser);
}
@Override
public Invalidate setUploadType(java.lang.String uploadType) {
return (Invalidate) super.setUploadType(uploadType);
}
@Override
public Invalidate setUploadProtocol(java.lang.String uploadProtocol) {
return (Invalidate) super.setUploadProtocol(uploadProtocol);
}
/** Name of the ApprovalRequest to invalidate. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the ApprovalRequest to invalidate.
*/
public java.lang.String getName() {
return name;
}
/** Name of the ApprovalRequest to invalidate. */
public Invalidate setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^folders/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Invalidate set(String parameterName, Object value) {
return (Invalidate) super.set(parameterName, value);
}
}
/**
* Lists approval requests associated with a project, folder, or organization. Approval requests can
* be filtered by state (pending, active, dismissed). The order is reverse chronological.
*
* Create a request for the method "approvalRequests.list".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+parent}/approvalRequests";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^folders/[^/]+$");
/**
* Lists approval requests associated with a project, folder, or organization. Approval requests
* can be filtered by state (pending, active, dismissed). The order is reverse chronological.
*
* Create a request for the method "approvalRequests.list".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.ListApprovalRequestsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The parent resource. This may be "projects/{project}", "folders/{folder}", or
"organizations/{organization}".
*/
public java.lang.String getParent() {
return parent;
}
/**
* The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^folders/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter on the type of approval requests to retrieve. Must be one of the following
* values: * [not set]: Requests that are pending or have active approvals. * ALL: All
* requests. * PENDING: Only pending requests. * ACTIVE: Only active (i.e. currently
* approved) requests. * DISMISSED: Only requests that have been dismissed, or requests that
* are not approved and past expiration. * EXPIRED: Only requests that have been approved,
* and the approval has expired. * HISTORY: Active, dismissed and expired requests.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter on the type of approval requests to retrieve. Must be one of the following values: * [not
set]: Requests that are pending or have active approvals. * ALL: All requests. * PENDING: Only
pending requests. * ACTIVE: Only active (i.e. currently approved) requests. * DISMISSED: Only
requests that have been dismissed, or requests that are not approved and past expiration. *
EXPIRED: Only requests that have been approved, and the approval has expired. * HISTORY: Active,
dismissed and expired requests.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter on the type of approval requests to retrieve. Must be one of the following
* values: * [not set]: Requests that are pending or have active approvals. * ALL: All
* requests. * PENDING: Only pending requests. * ACTIVE: Only active (i.e. currently
* approved) requests. * DISMISSED: Only requests that have been dismissed, or requests that
* are not approved and past expiration. * EXPIRED: Only requests that have been approved,
* and the approval has expired. * HISTORY: Active, dismissed and expired requests.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Requested page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Requested page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token identifying the page of results to return. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying the page of results to return.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token identifying the page of results to return. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Organizations collection.
*
* The typical use is:
*
* {@code AccessApproval accessapproval = new AccessApproval(...);}
* {@code AccessApproval.Organizations.List request = accessapproval.organizations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Organizations organizations() {
return new Organizations();
}
/**
* The "organizations" collection of methods.
*/
public class Organizations {
/**
* Deletes the settings associated with a project, folder, or organization. This will have the
* effect of disabling Access Approval for the project, folder, or organization, but only if all
* ancestors also have Access Approval disabled. If Access Approval is enabled at a higher level of
* the hierarchy, then Access Approval will still be enabled at this level as the settings are
* inherited.
*
* Create a request for the method "organizations.deleteAccessApprovalSettings".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link DeleteAccessApprovalSettings#execute()} method to invoke the
* remote operation.
*
* @param name Name of the AccessApprovalSettings to delete.
* @return the request
*/
public DeleteAccessApprovalSettings deleteAccessApprovalSettings(java.lang.String name) throws java.io.IOException {
DeleteAccessApprovalSettings result = new DeleteAccessApprovalSettings(name);
initialize(result);
return result;
}
public class DeleteAccessApprovalSettings extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/accessApprovalSettings$");
/**
* Deletes the settings associated with a project, folder, or organization. This will have the
* effect of disabling Access Approval for the project, folder, or organization, but only if all
* ancestors also have Access Approval disabled. If Access Approval is enabled at a higher level
* of the hierarchy, then Access Approval will still be enabled at this level as the settings are
* inherited.
*
* Create a request for the method "organizations.deleteAccessApprovalSettings".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link DeleteAccessApprovalSettings#execute()} method to invoke
* the remote operation. {@link DeleteAccessApprovalSettings#initialize(com.google.api.client.
* googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param name Name of the AccessApprovalSettings to delete.
* @since 1.13
*/
protected DeleteAccessApprovalSettings(java.lang.String name) {
super(AccessApproval.this, "DELETE", REST_PATH, null, com.google.api.services.accessapproval.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/accessApprovalSettings$");
}
}
@Override
public DeleteAccessApprovalSettings set$Xgafv(java.lang.String $Xgafv) {
return (DeleteAccessApprovalSettings) super.set$Xgafv($Xgafv);
}
@Override
public DeleteAccessApprovalSettings setAccessToken(java.lang.String accessToken) {
return (DeleteAccessApprovalSettings) super.setAccessToken(accessToken);
}
@Override
public DeleteAccessApprovalSettings setAlt(java.lang.String alt) {
return (DeleteAccessApprovalSettings) super.setAlt(alt);
}
@Override
public DeleteAccessApprovalSettings setCallback(java.lang.String callback) {
return (DeleteAccessApprovalSettings) super.setCallback(callback);
}
@Override
public DeleteAccessApprovalSettings setFields(java.lang.String fields) {
return (DeleteAccessApprovalSettings) super.setFields(fields);
}
@Override
public DeleteAccessApprovalSettings setKey(java.lang.String key) {
return (DeleteAccessApprovalSettings) super.setKey(key);
}
@Override
public DeleteAccessApprovalSettings setOauthToken(java.lang.String oauthToken) {
return (DeleteAccessApprovalSettings) super.setOauthToken(oauthToken);
}
@Override
public DeleteAccessApprovalSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DeleteAccessApprovalSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public DeleteAccessApprovalSettings setQuotaUser(java.lang.String quotaUser) {
return (DeleteAccessApprovalSettings) super.setQuotaUser(quotaUser);
}
@Override
public DeleteAccessApprovalSettings setUploadType(java.lang.String uploadType) {
return (DeleteAccessApprovalSettings) super.setUploadType(uploadType);
}
@Override
public DeleteAccessApprovalSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (DeleteAccessApprovalSettings) super.setUploadProtocol(uploadProtocol);
}
/** Name of the AccessApprovalSettings to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the AccessApprovalSettings to delete.
*/
public java.lang.String getName() {
return name;
}
/** Name of the AccessApprovalSettings to delete. */
public DeleteAccessApprovalSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/accessApprovalSettings$");
}
this.name = name;
return this;
}
@Override
public DeleteAccessApprovalSettings set(String parameterName, Object value) {
return (DeleteAccessApprovalSettings) super.set(parameterName, value);
}
}
/**
* Gets the settings associated with a project, folder, or organization.
*
* Create a request for the method "organizations.getAccessApprovalSettings".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link GetAccessApprovalSettings#execute()} method to invoke the
* remote operation.
*
* @param name The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
* @return the request
*/
public GetAccessApprovalSettings getAccessApprovalSettings(java.lang.String name) throws java.io.IOException {
GetAccessApprovalSettings result = new GetAccessApprovalSettings(name);
initialize(result);
return result;
}
public class GetAccessApprovalSettings extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/accessApprovalSettings$");
/**
* Gets the settings associated with a project, folder, or organization.
*
* Create a request for the method "organizations.getAccessApprovalSettings".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link GetAccessApprovalSettings#execute()} method to invoke the
* remote operation. {@link GetAccessApprovalSettings#initialize(com.google.api.client.googlea
* pis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param name The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
* @since 1.13
*/
protected GetAccessApprovalSettings(java.lang.String name) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/accessApprovalSettings$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetAccessApprovalSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetAccessApprovalSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetAccessApprovalSettings setAccessToken(java.lang.String accessToken) {
return (GetAccessApprovalSettings) super.setAccessToken(accessToken);
}
@Override
public GetAccessApprovalSettings setAlt(java.lang.String alt) {
return (GetAccessApprovalSettings) super.setAlt(alt);
}
@Override
public GetAccessApprovalSettings setCallback(java.lang.String callback) {
return (GetAccessApprovalSettings) super.setCallback(callback);
}
@Override
public GetAccessApprovalSettings setFields(java.lang.String fields) {
return (GetAccessApprovalSettings) super.setFields(fields);
}
@Override
public GetAccessApprovalSettings setKey(java.lang.String key) {
return (GetAccessApprovalSettings) super.setKey(key);
}
@Override
public GetAccessApprovalSettings setOauthToken(java.lang.String oauthToken) {
return (GetAccessApprovalSettings) super.setOauthToken(oauthToken);
}
@Override
public GetAccessApprovalSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetAccessApprovalSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetAccessApprovalSettings setQuotaUser(java.lang.String quotaUser) {
return (GetAccessApprovalSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetAccessApprovalSettings setUploadType(java.lang.String uploadType) {
return (GetAccessApprovalSettings) super.setUploadType(uploadType);
}
@Override
public GetAccessApprovalSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetAccessApprovalSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the AccessApprovalSettings to retrieve. Format:
"{projects|folders|organizations}/{id}/accessApprovalSettings"
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
*/
public GetAccessApprovalSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/accessApprovalSettings$");
}
this.name = name;
return this;
}
@Override
public GetAccessApprovalSettings set(String parameterName, Object value) {
return (GetAccessApprovalSettings) super.set(parameterName, value);
}
}
/**
* Retrieves the service account that is used by Access Approval to access KMS keys for signing
* approved approval requests.
*
* Create a request for the method "organizations.getServiceAccount".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link GetServiceAccount#execute()} method to invoke the remote
* operation.
*
* @param name Name of the AccessApprovalServiceAccount to retrieve.
* @return the request
*/
public GetServiceAccount getServiceAccount(java.lang.String name) throws java.io.IOException {
GetServiceAccount result = new GetServiceAccount(name);
initialize(result);
return result;
}
public class GetServiceAccount extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/serviceAccount$");
/**
* Retrieves the service account that is used by Access Approval to access KMS keys for signing
* approved approval requests.
*
* Create a request for the method "organizations.getServiceAccount".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link GetServiceAccount#execute()} method to invoke the remote
* operation. {@link GetServiceAccount#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Name of the AccessApprovalServiceAccount to retrieve.
* @since 1.13
*/
protected GetServiceAccount(java.lang.String name) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.AccessApprovalServiceAccount.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/serviceAccount$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetServiceAccount set$Xgafv(java.lang.String $Xgafv) {
return (GetServiceAccount) super.set$Xgafv($Xgafv);
}
@Override
public GetServiceAccount setAccessToken(java.lang.String accessToken) {
return (GetServiceAccount) super.setAccessToken(accessToken);
}
@Override
public GetServiceAccount setAlt(java.lang.String alt) {
return (GetServiceAccount) super.setAlt(alt);
}
@Override
public GetServiceAccount setCallback(java.lang.String callback) {
return (GetServiceAccount) super.setCallback(callback);
}
@Override
public GetServiceAccount setFields(java.lang.String fields) {
return (GetServiceAccount) super.setFields(fields);
}
@Override
public GetServiceAccount setKey(java.lang.String key) {
return (GetServiceAccount) super.setKey(key);
}
@Override
public GetServiceAccount setOauthToken(java.lang.String oauthToken) {
return (GetServiceAccount) super.setOauthToken(oauthToken);
}
@Override
public GetServiceAccount setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetServiceAccount) super.setPrettyPrint(prettyPrint);
}
@Override
public GetServiceAccount setQuotaUser(java.lang.String quotaUser) {
return (GetServiceAccount) super.setQuotaUser(quotaUser);
}
@Override
public GetServiceAccount setUploadType(java.lang.String uploadType) {
return (GetServiceAccount) super.setUploadType(uploadType);
}
@Override
public GetServiceAccount setUploadProtocol(java.lang.String uploadProtocol) {
return (GetServiceAccount) super.setUploadProtocol(uploadProtocol);
}
/** Name of the AccessApprovalServiceAccount to retrieve. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the AccessApprovalServiceAccount to retrieve.
*/
public java.lang.String getName() {
return name;
}
/** Name of the AccessApprovalServiceAccount to retrieve. */
public GetServiceAccount setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/serviceAccount$");
}
this.name = name;
return this;
}
@Override
public GetServiceAccount set(String parameterName, Object value) {
return (GetServiceAccount) super.set(parameterName, value);
}
}
/**
* Updates the settings associated with a project, folder, or organization. Settings to update are
* determined by the value of field_mask.
*
* Create a request for the method "organizations.updateAccessApprovalSettings".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link UpdateAccessApprovalSettings#execute()} method to invoke the
* remote operation.
*
* @param name The resource name of the settings. Format is one of: * "projects/{project}/accessApprovalSettings" *
* "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
* @param content the {@link com.google.api.services.accessapproval.v1.model.AccessApprovalSettings}
* @return the request
*/
public UpdateAccessApprovalSettings updateAccessApprovalSettings(java.lang.String name, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings content) throws java.io.IOException {
UpdateAccessApprovalSettings result = new UpdateAccessApprovalSettings(name, content);
initialize(result);
return result;
}
public class UpdateAccessApprovalSettings extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/accessApprovalSettings$");
/**
* Updates the settings associated with a project, folder, or organization. Settings to update are
* determined by the value of field_mask.
*
* Create a request for the method "organizations.updateAccessApprovalSettings".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link UpdateAccessApprovalSettings#execute()} method to invoke
* the remote operation. {@link UpdateAccessApprovalSettings#initialize(com.google.api.client.
* googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param name The resource name of the settings. Format is one of: * "projects/{project}/accessApprovalSettings" *
* "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
* @param content the {@link com.google.api.services.accessapproval.v1.model.AccessApprovalSettings}
* @since 1.13
*/
protected UpdateAccessApprovalSettings(java.lang.String name, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings content) {
super(AccessApproval.this, "PATCH", REST_PATH, content, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/accessApprovalSettings$");
}
}
@Override
public UpdateAccessApprovalSettings set$Xgafv(java.lang.String $Xgafv) {
return (UpdateAccessApprovalSettings) super.set$Xgafv($Xgafv);
}
@Override
public UpdateAccessApprovalSettings setAccessToken(java.lang.String accessToken) {
return (UpdateAccessApprovalSettings) super.setAccessToken(accessToken);
}
@Override
public UpdateAccessApprovalSettings setAlt(java.lang.String alt) {
return (UpdateAccessApprovalSettings) super.setAlt(alt);
}
@Override
public UpdateAccessApprovalSettings setCallback(java.lang.String callback) {
return (UpdateAccessApprovalSettings) super.setCallback(callback);
}
@Override
public UpdateAccessApprovalSettings setFields(java.lang.String fields) {
return (UpdateAccessApprovalSettings) super.setFields(fields);
}
@Override
public UpdateAccessApprovalSettings setKey(java.lang.String key) {
return (UpdateAccessApprovalSettings) super.setKey(key);
}
@Override
public UpdateAccessApprovalSettings setOauthToken(java.lang.String oauthToken) {
return (UpdateAccessApprovalSettings) super.setOauthToken(oauthToken);
}
@Override
public UpdateAccessApprovalSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateAccessApprovalSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateAccessApprovalSettings setQuotaUser(java.lang.String quotaUser) {
return (UpdateAccessApprovalSettings) super.setQuotaUser(quotaUser);
}
@Override
public UpdateAccessApprovalSettings setUploadType(java.lang.String uploadType) {
return (UpdateAccessApprovalSettings) super.setUploadType(uploadType);
}
@Override
public UpdateAccessApprovalSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateAccessApprovalSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* The resource name of the settings. Format is one of: *
* "projects/{project}/accessApprovalSettings" * "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource name of the settings. Format is one of: * "projects/{project}/accessApprovalSettings"
* "folders/{folder}/accessApprovalSettings" * "organizations/{organization}/accessApprovalSettings"
*/
public java.lang.String getName() {
return name;
}
/**
* The resource name of the settings. Format is one of: *
* "projects/{project}/accessApprovalSettings" * "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
*/
public UpdateAccessApprovalSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/accessApprovalSettings$");
}
this.name = name;
return this;
}
/**
* The update mask applies to the settings. Only the top level fields of
* AccessApprovalSettings (notification_emails & enrolled_services) are supported. For each
* field, if it is included, the currently stored value will be entirely overwritten with the
* value of the field passed in this request. For the `FieldMask` definition, see
* https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#fieldmask If
* this field is left unset, only the notification_emails field will be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The update mask applies to the settings. Only the top level fields of AccessApprovalSettings
(notification_emails & enrolled_services) are supported. For each field, if it is included, the
currently stored value will be entirely overwritten with the value of the field passed in this
request. For the `FieldMask` definition, see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#fieldmask If this field is left unset, only the
notification_emails field will be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The update mask applies to the settings. Only the top level fields of
* AccessApprovalSettings (notification_emails & enrolled_services) are supported. For each
* field, if it is included, the currently stored value will be entirely overwritten with the
* value of the field passed in this request. For the `FieldMask` definition, see
* https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#fieldmask If
* this field is left unset, only the notification_emails field will be updated.
*/
public UpdateAccessApprovalSettings setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateAccessApprovalSettings set(String parameterName, Object value) {
return (UpdateAccessApprovalSettings) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the ApprovalRequests collection.
*
* The typical use is:
*
* {@code AccessApproval accessapproval = new AccessApproval(...);}
* {@code AccessApproval.ApprovalRequests.List request = accessapproval.approvalRequests().list(parameters ...)}
*
*
* @return the resource collection
*/
public ApprovalRequests approvalRequests() {
return new ApprovalRequests();
}
/**
* The "approvalRequests" collection of methods.
*/
public class ApprovalRequests {
/**
* Approves a request and returns the updated ApprovalRequest. Returns NOT_FOUND if the request does
* not exist. Returns FAILED_PRECONDITION if the request exists but is not in a pending state.
*
* Create a request for the method "approvalRequests.approve".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Approve#execute()} method to invoke the remote operation.
*
* @param name Name of the approval request to approve.
* @param content the {@link com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage}
* @return the request
*/
public Approve approve(java.lang.String name, com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage content) throws java.io.IOException {
Approve result = new Approve(name, content);
initialize(result);
return result;
}
public class Approve extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}:approve";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/approvalRequests/[^/]+$");
/**
* Approves a request and returns the updated ApprovalRequest. Returns NOT_FOUND if the request
* does not exist. Returns FAILED_PRECONDITION if the request exists but is not in a pending
* state.
*
* Create a request for the method "approvalRequests.approve".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Approve#execute()} method to invoke the remote operation.
* {@link
* Approve#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the approval request to approve.
* @param content the {@link com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage}
* @since 1.13
*/
protected Approve(java.lang.String name, com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage content) {
super(AccessApproval.this, "POST", REST_PATH, content, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public Approve set$Xgafv(java.lang.String $Xgafv) {
return (Approve) super.set$Xgafv($Xgafv);
}
@Override
public Approve setAccessToken(java.lang.String accessToken) {
return (Approve) super.setAccessToken(accessToken);
}
@Override
public Approve setAlt(java.lang.String alt) {
return (Approve) super.setAlt(alt);
}
@Override
public Approve setCallback(java.lang.String callback) {
return (Approve) super.setCallback(callback);
}
@Override
public Approve setFields(java.lang.String fields) {
return (Approve) super.setFields(fields);
}
@Override
public Approve setKey(java.lang.String key) {
return (Approve) super.setKey(key);
}
@Override
public Approve setOauthToken(java.lang.String oauthToken) {
return (Approve) super.setOauthToken(oauthToken);
}
@Override
public Approve setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Approve) super.setPrettyPrint(prettyPrint);
}
@Override
public Approve setQuotaUser(java.lang.String quotaUser) {
return (Approve) super.setQuotaUser(quotaUser);
}
@Override
public Approve setUploadType(java.lang.String uploadType) {
return (Approve) super.setUploadType(uploadType);
}
@Override
public Approve setUploadProtocol(java.lang.String uploadProtocol) {
return (Approve) super.setUploadProtocol(uploadProtocol);
}
/** Name of the approval request to approve. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the approval request to approve.
*/
public java.lang.String getName() {
return name;
}
/** Name of the approval request to approve. */
public Approve setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Approve set(String parameterName, Object value) {
return (Approve) super.set(parameterName, value);
}
}
/**
* Dismisses a request. Returns the updated ApprovalRequest. NOTE: This does not deny access to the
* resource if another request has been made and approved. It is equivalent in effect to ignoring
* the request altogether. Returns NOT_FOUND if the request does not exist. Returns
* FAILED_PRECONDITION if the request exists but is not in a pending state.
*
* Create a request for the method "approvalRequests.dismiss".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
*
* @param name Name of the ApprovalRequest to dismiss.
* @param content the {@link com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage}
* @return the request
*/
public Dismiss dismiss(java.lang.String name, com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage content) throws java.io.IOException {
Dismiss result = new Dismiss(name, content);
initialize(result);
return result;
}
public class Dismiss extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}:dismiss";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/approvalRequests/[^/]+$");
/**
* Dismisses a request. Returns the updated ApprovalRequest. NOTE: This does not deny access to
* the resource if another request has been made and approved. It is equivalent in effect to
* ignoring the request altogether. Returns NOT_FOUND if the request does not exist. Returns
* FAILED_PRECONDITION if the request exists but is not in a pending state.
*
* Create a request for the method "approvalRequests.dismiss".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
* {@link
* Dismiss#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the ApprovalRequest to dismiss.
* @param content the {@link com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage}
* @since 1.13
*/
protected Dismiss(java.lang.String name, com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage content) {
super(AccessApproval.this, "POST", REST_PATH, content, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public Dismiss set$Xgafv(java.lang.String $Xgafv) {
return (Dismiss) super.set$Xgafv($Xgafv);
}
@Override
public Dismiss setAccessToken(java.lang.String accessToken) {
return (Dismiss) super.setAccessToken(accessToken);
}
@Override
public Dismiss setAlt(java.lang.String alt) {
return (Dismiss) super.setAlt(alt);
}
@Override
public Dismiss setCallback(java.lang.String callback) {
return (Dismiss) super.setCallback(callback);
}
@Override
public Dismiss setFields(java.lang.String fields) {
return (Dismiss) super.setFields(fields);
}
@Override
public Dismiss setKey(java.lang.String key) {
return (Dismiss) super.setKey(key);
}
@Override
public Dismiss setOauthToken(java.lang.String oauthToken) {
return (Dismiss) super.setOauthToken(oauthToken);
}
@Override
public Dismiss setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Dismiss) super.setPrettyPrint(prettyPrint);
}
@Override
public Dismiss setQuotaUser(java.lang.String quotaUser) {
return (Dismiss) super.setQuotaUser(quotaUser);
}
@Override
public Dismiss setUploadType(java.lang.String uploadType) {
return (Dismiss) super.setUploadType(uploadType);
}
@Override
public Dismiss setUploadProtocol(java.lang.String uploadProtocol) {
return (Dismiss) super.setUploadProtocol(uploadProtocol);
}
/** Name of the ApprovalRequest to dismiss. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the ApprovalRequest to dismiss.
*/
public java.lang.String getName() {
return name;
}
/** Name of the ApprovalRequest to dismiss. */
public Dismiss setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Dismiss set(String parameterName, Object value) {
return (Dismiss) super.set(parameterName, value);
}
}
/**
* Gets an approval request. Returns NOT_FOUND if the request does not exist.
*
* Create a request for the method "approvalRequests.get".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/approvalRequests/[^/]+$");
/**
* Gets an approval request. Returns NOT_FOUND if the request does not exist.
*
* Create a request for the method "approvalRequests.get".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the approval request to retrieve. Format:
"{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Invalidates an existing ApprovalRequest. Returns the updated ApprovalRequest. NOTE: This does not
* deny access to the resource if another request has been made and approved. It only invalidates a
* single approval. Returns FAILED_PRECONDITION if the request exists but is not in an approved
* state.
*
* Create a request for the method "approvalRequests.invalidate".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Invalidate#execute()} method to invoke the remote operation.
*
* @param name Name of the ApprovalRequest to invalidate.
* @param content the {@link com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage}
* @return the request
*/
public Invalidate invalidate(java.lang.String name, com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage content) throws java.io.IOException {
Invalidate result = new Invalidate(name, content);
initialize(result);
return result;
}
public class Invalidate extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}:invalidate";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/approvalRequests/[^/]+$");
/**
* Invalidates an existing ApprovalRequest. Returns the updated ApprovalRequest. NOTE: This does
* not deny access to the resource if another request has been made and approved. It only
* invalidates a single approval. Returns FAILED_PRECONDITION if the request exists but is not in
* an approved state.
*
* Create a request for the method "approvalRequests.invalidate".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Invalidate#execute()} method to invoke the remote
* operation. {@link
* Invalidate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the ApprovalRequest to invalidate.
* @param content the {@link com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage}
* @since 1.13
*/
protected Invalidate(java.lang.String name, com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage content) {
super(AccessApproval.this, "POST", REST_PATH, content, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public Invalidate set$Xgafv(java.lang.String $Xgafv) {
return (Invalidate) super.set$Xgafv($Xgafv);
}
@Override
public Invalidate setAccessToken(java.lang.String accessToken) {
return (Invalidate) super.setAccessToken(accessToken);
}
@Override
public Invalidate setAlt(java.lang.String alt) {
return (Invalidate) super.setAlt(alt);
}
@Override
public Invalidate setCallback(java.lang.String callback) {
return (Invalidate) super.setCallback(callback);
}
@Override
public Invalidate setFields(java.lang.String fields) {
return (Invalidate) super.setFields(fields);
}
@Override
public Invalidate setKey(java.lang.String key) {
return (Invalidate) super.setKey(key);
}
@Override
public Invalidate setOauthToken(java.lang.String oauthToken) {
return (Invalidate) super.setOauthToken(oauthToken);
}
@Override
public Invalidate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Invalidate) super.setPrettyPrint(prettyPrint);
}
@Override
public Invalidate setQuotaUser(java.lang.String quotaUser) {
return (Invalidate) super.setQuotaUser(quotaUser);
}
@Override
public Invalidate setUploadType(java.lang.String uploadType) {
return (Invalidate) super.setUploadType(uploadType);
}
@Override
public Invalidate setUploadProtocol(java.lang.String uploadProtocol) {
return (Invalidate) super.setUploadProtocol(uploadProtocol);
}
/** Name of the ApprovalRequest to invalidate. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the ApprovalRequest to invalidate.
*/
public java.lang.String getName() {
return name;
}
/** Name of the ApprovalRequest to invalidate. */
public Invalidate setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Invalidate set(String parameterName, Object value) {
return (Invalidate) super.set(parameterName, value);
}
}
/**
* Lists approval requests associated with a project, folder, or organization. Approval requests can
* be filtered by state (pending, active, dismissed). The order is reverse chronological.
*
* Create a request for the method "approvalRequests.list".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+parent}/approvalRequests";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Lists approval requests associated with a project, folder, or organization. Approval requests
* can be filtered by state (pending, active, dismissed). The order is reverse chronological.
*
* Create a request for the method "approvalRequests.list".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.ListApprovalRequestsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The parent resource. This may be "projects/{project}", "folders/{folder}", or
"organizations/{organization}".
*/
public java.lang.String getParent() {
return parent;
}
/**
* The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter on the type of approval requests to retrieve. Must be one of the following
* values: * [not set]: Requests that are pending or have active approvals. * ALL: All
* requests. * PENDING: Only pending requests. * ACTIVE: Only active (i.e. currently
* approved) requests. * DISMISSED: Only requests that have been dismissed, or requests that
* are not approved and past expiration. * EXPIRED: Only requests that have been approved,
* and the approval has expired. * HISTORY: Active, dismissed and expired requests.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter on the type of approval requests to retrieve. Must be one of the following values: * [not
set]: Requests that are pending or have active approvals. * ALL: All requests. * PENDING: Only
pending requests. * ACTIVE: Only active (i.e. currently approved) requests. * DISMISSED: Only
requests that have been dismissed, or requests that are not approved and past expiration. *
EXPIRED: Only requests that have been approved, and the approval has expired. * HISTORY: Active,
dismissed and expired requests.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter on the type of approval requests to retrieve. Must be one of the following
* values: * [not set]: Requests that are pending or have active approvals. * ALL: All
* requests. * PENDING: Only pending requests. * ACTIVE: Only active (i.e. currently
* approved) requests. * DISMISSED: Only requests that have been dismissed, or requests that
* are not approved and past expiration. * EXPIRED: Only requests that have been approved,
* and the approval has expired. * HISTORY: Active, dismissed and expired requests.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Requested page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Requested page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token identifying the page of results to return. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying the page of results to return.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token identifying the page of results to return. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code AccessApproval accessapproval = new AccessApproval(...);}
* {@code AccessApproval.Projects.List request = accessapproval.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* Deletes the settings associated with a project, folder, or organization. This will have the
* effect of disabling Access Approval for the project, folder, or organization, but only if all
* ancestors also have Access Approval disabled. If Access Approval is enabled at a higher level of
* the hierarchy, then Access Approval will still be enabled at this level as the settings are
* inherited.
*
* Create a request for the method "projects.deleteAccessApprovalSettings".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link DeleteAccessApprovalSettings#execute()} method to invoke the
* remote operation.
*
* @param name Name of the AccessApprovalSettings to delete.
* @return the request
*/
public DeleteAccessApprovalSettings deleteAccessApprovalSettings(java.lang.String name) throws java.io.IOException {
DeleteAccessApprovalSettings result = new DeleteAccessApprovalSettings(name);
initialize(result);
return result;
}
public class DeleteAccessApprovalSettings extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/accessApprovalSettings$");
/**
* Deletes the settings associated with a project, folder, or organization. This will have the
* effect of disabling Access Approval for the project, folder, or organization, but only if all
* ancestors also have Access Approval disabled. If Access Approval is enabled at a higher level
* of the hierarchy, then Access Approval will still be enabled at this level as the settings are
* inherited.
*
* Create a request for the method "projects.deleteAccessApprovalSettings".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link DeleteAccessApprovalSettings#execute()} method to invoke
* the remote operation. {@link DeleteAccessApprovalSettings#initialize(com.google.api.client.
* googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param name Name of the AccessApprovalSettings to delete.
* @since 1.13
*/
protected DeleteAccessApprovalSettings(java.lang.String name) {
super(AccessApproval.this, "DELETE", REST_PATH, null, com.google.api.services.accessapproval.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/accessApprovalSettings$");
}
}
@Override
public DeleteAccessApprovalSettings set$Xgafv(java.lang.String $Xgafv) {
return (DeleteAccessApprovalSettings) super.set$Xgafv($Xgafv);
}
@Override
public DeleteAccessApprovalSettings setAccessToken(java.lang.String accessToken) {
return (DeleteAccessApprovalSettings) super.setAccessToken(accessToken);
}
@Override
public DeleteAccessApprovalSettings setAlt(java.lang.String alt) {
return (DeleteAccessApprovalSettings) super.setAlt(alt);
}
@Override
public DeleteAccessApprovalSettings setCallback(java.lang.String callback) {
return (DeleteAccessApprovalSettings) super.setCallback(callback);
}
@Override
public DeleteAccessApprovalSettings setFields(java.lang.String fields) {
return (DeleteAccessApprovalSettings) super.setFields(fields);
}
@Override
public DeleteAccessApprovalSettings setKey(java.lang.String key) {
return (DeleteAccessApprovalSettings) super.setKey(key);
}
@Override
public DeleteAccessApprovalSettings setOauthToken(java.lang.String oauthToken) {
return (DeleteAccessApprovalSettings) super.setOauthToken(oauthToken);
}
@Override
public DeleteAccessApprovalSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DeleteAccessApprovalSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public DeleteAccessApprovalSettings setQuotaUser(java.lang.String quotaUser) {
return (DeleteAccessApprovalSettings) super.setQuotaUser(quotaUser);
}
@Override
public DeleteAccessApprovalSettings setUploadType(java.lang.String uploadType) {
return (DeleteAccessApprovalSettings) super.setUploadType(uploadType);
}
@Override
public DeleteAccessApprovalSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (DeleteAccessApprovalSettings) super.setUploadProtocol(uploadProtocol);
}
/** Name of the AccessApprovalSettings to delete. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the AccessApprovalSettings to delete.
*/
public java.lang.String getName() {
return name;
}
/** Name of the AccessApprovalSettings to delete. */
public DeleteAccessApprovalSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/accessApprovalSettings$");
}
this.name = name;
return this;
}
@Override
public DeleteAccessApprovalSettings set(String parameterName, Object value) {
return (DeleteAccessApprovalSettings) super.set(parameterName, value);
}
}
/**
* Gets the settings associated with a project, folder, or organization.
*
* Create a request for the method "projects.getAccessApprovalSettings".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link GetAccessApprovalSettings#execute()} method to invoke the
* remote operation.
*
* @param name The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
* @return the request
*/
public GetAccessApprovalSettings getAccessApprovalSettings(java.lang.String name) throws java.io.IOException {
GetAccessApprovalSettings result = new GetAccessApprovalSettings(name);
initialize(result);
return result;
}
public class GetAccessApprovalSettings extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/accessApprovalSettings$");
/**
* Gets the settings associated with a project, folder, or organization.
*
* Create a request for the method "projects.getAccessApprovalSettings".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link GetAccessApprovalSettings#execute()} method to invoke the
* remote operation. {@link GetAccessApprovalSettings#initialize(com.google.api.client.googlea
* pis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param name The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
* @since 1.13
*/
protected GetAccessApprovalSettings(java.lang.String name) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/accessApprovalSettings$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetAccessApprovalSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetAccessApprovalSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetAccessApprovalSettings setAccessToken(java.lang.String accessToken) {
return (GetAccessApprovalSettings) super.setAccessToken(accessToken);
}
@Override
public GetAccessApprovalSettings setAlt(java.lang.String alt) {
return (GetAccessApprovalSettings) super.setAlt(alt);
}
@Override
public GetAccessApprovalSettings setCallback(java.lang.String callback) {
return (GetAccessApprovalSettings) super.setCallback(callback);
}
@Override
public GetAccessApprovalSettings setFields(java.lang.String fields) {
return (GetAccessApprovalSettings) super.setFields(fields);
}
@Override
public GetAccessApprovalSettings setKey(java.lang.String key) {
return (GetAccessApprovalSettings) super.setKey(key);
}
@Override
public GetAccessApprovalSettings setOauthToken(java.lang.String oauthToken) {
return (GetAccessApprovalSettings) super.setOauthToken(oauthToken);
}
@Override
public GetAccessApprovalSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetAccessApprovalSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetAccessApprovalSettings setQuotaUser(java.lang.String quotaUser) {
return (GetAccessApprovalSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetAccessApprovalSettings setUploadType(java.lang.String uploadType) {
return (GetAccessApprovalSettings) super.setUploadType(uploadType);
}
@Override
public GetAccessApprovalSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetAccessApprovalSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the AccessApprovalSettings to retrieve. Format:
"{projects|folders|organizations}/{id}/accessApprovalSettings"
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the AccessApprovalSettings to retrieve. Format:
* "{projects|folders|organizations}/{id}/accessApprovalSettings"
*/
public GetAccessApprovalSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/accessApprovalSettings$");
}
this.name = name;
return this;
}
@Override
public GetAccessApprovalSettings set(String parameterName, Object value) {
return (GetAccessApprovalSettings) super.set(parameterName, value);
}
}
/**
* Retrieves the service account that is used by Access Approval to access KMS keys for signing
* approved approval requests.
*
* Create a request for the method "projects.getServiceAccount".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link GetServiceAccount#execute()} method to invoke the remote
* operation.
*
* @param name Name of the AccessApprovalServiceAccount to retrieve.
* @return the request
*/
public GetServiceAccount getServiceAccount(java.lang.String name) throws java.io.IOException {
GetServiceAccount result = new GetServiceAccount(name);
initialize(result);
return result;
}
public class GetServiceAccount extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/serviceAccount$");
/**
* Retrieves the service account that is used by Access Approval to access KMS keys for signing
* approved approval requests.
*
* Create a request for the method "projects.getServiceAccount".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link GetServiceAccount#execute()} method to invoke the remote
* operation. {@link GetServiceAccount#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Name of the AccessApprovalServiceAccount to retrieve.
* @since 1.13
*/
protected GetServiceAccount(java.lang.String name) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.AccessApprovalServiceAccount.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/serviceAccount$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetServiceAccount set$Xgafv(java.lang.String $Xgafv) {
return (GetServiceAccount) super.set$Xgafv($Xgafv);
}
@Override
public GetServiceAccount setAccessToken(java.lang.String accessToken) {
return (GetServiceAccount) super.setAccessToken(accessToken);
}
@Override
public GetServiceAccount setAlt(java.lang.String alt) {
return (GetServiceAccount) super.setAlt(alt);
}
@Override
public GetServiceAccount setCallback(java.lang.String callback) {
return (GetServiceAccount) super.setCallback(callback);
}
@Override
public GetServiceAccount setFields(java.lang.String fields) {
return (GetServiceAccount) super.setFields(fields);
}
@Override
public GetServiceAccount setKey(java.lang.String key) {
return (GetServiceAccount) super.setKey(key);
}
@Override
public GetServiceAccount setOauthToken(java.lang.String oauthToken) {
return (GetServiceAccount) super.setOauthToken(oauthToken);
}
@Override
public GetServiceAccount setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetServiceAccount) super.setPrettyPrint(prettyPrint);
}
@Override
public GetServiceAccount setQuotaUser(java.lang.String quotaUser) {
return (GetServiceAccount) super.setQuotaUser(quotaUser);
}
@Override
public GetServiceAccount setUploadType(java.lang.String uploadType) {
return (GetServiceAccount) super.setUploadType(uploadType);
}
@Override
public GetServiceAccount setUploadProtocol(java.lang.String uploadProtocol) {
return (GetServiceAccount) super.setUploadProtocol(uploadProtocol);
}
/** Name of the AccessApprovalServiceAccount to retrieve. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the AccessApprovalServiceAccount to retrieve.
*/
public java.lang.String getName() {
return name;
}
/** Name of the AccessApprovalServiceAccount to retrieve. */
public GetServiceAccount setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/serviceAccount$");
}
this.name = name;
return this;
}
@Override
public GetServiceAccount set(String parameterName, Object value) {
return (GetServiceAccount) super.set(parameterName, value);
}
}
/**
* Updates the settings associated with a project, folder, or organization. Settings to update are
* determined by the value of field_mask.
*
* Create a request for the method "projects.updateAccessApprovalSettings".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link UpdateAccessApprovalSettings#execute()} method to invoke the
* remote operation.
*
* @param name The resource name of the settings. Format is one of: * "projects/{project}/accessApprovalSettings" *
* "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
* @param content the {@link com.google.api.services.accessapproval.v1.model.AccessApprovalSettings}
* @return the request
*/
public UpdateAccessApprovalSettings updateAccessApprovalSettings(java.lang.String name, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings content) throws java.io.IOException {
UpdateAccessApprovalSettings result = new UpdateAccessApprovalSettings(name, content);
initialize(result);
return result;
}
public class UpdateAccessApprovalSettings extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/accessApprovalSettings$");
/**
* Updates the settings associated with a project, folder, or organization. Settings to update are
* determined by the value of field_mask.
*
* Create a request for the method "projects.updateAccessApprovalSettings".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link UpdateAccessApprovalSettings#execute()} method to invoke
* the remote operation. {@link UpdateAccessApprovalSettings#initialize(com.google.api.client.
* googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param name The resource name of the settings. Format is one of: * "projects/{project}/accessApprovalSettings" *
* "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
* @param content the {@link com.google.api.services.accessapproval.v1.model.AccessApprovalSettings}
* @since 1.13
*/
protected UpdateAccessApprovalSettings(java.lang.String name, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings content) {
super(AccessApproval.this, "PATCH", REST_PATH, content, com.google.api.services.accessapproval.v1.model.AccessApprovalSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/accessApprovalSettings$");
}
}
@Override
public UpdateAccessApprovalSettings set$Xgafv(java.lang.String $Xgafv) {
return (UpdateAccessApprovalSettings) super.set$Xgafv($Xgafv);
}
@Override
public UpdateAccessApprovalSettings setAccessToken(java.lang.String accessToken) {
return (UpdateAccessApprovalSettings) super.setAccessToken(accessToken);
}
@Override
public UpdateAccessApprovalSettings setAlt(java.lang.String alt) {
return (UpdateAccessApprovalSettings) super.setAlt(alt);
}
@Override
public UpdateAccessApprovalSettings setCallback(java.lang.String callback) {
return (UpdateAccessApprovalSettings) super.setCallback(callback);
}
@Override
public UpdateAccessApprovalSettings setFields(java.lang.String fields) {
return (UpdateAccessApprovalSettings) super.setFields(fields);
}
@Override
public UpdateAccessApprovalSettings setKey(java.lang.String key) {
return (UpdateAccessApprovalSettings) super.setKey(key);
}
@Override
public UpdateAccessApprovalSettings setOauthToken(java.lang.String oauthToken) {
return (UpdateAccessApprovalSettings) super.setOauthToken(oauthToken);
}
@Override
public UpdateAccessApprovalSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateAccessApprovalSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateAccessApprovalSettings setQuotaUser(java.lang.String quotaUser) {
return (UpdateAccessApprovalSettings) super.setQuotaUser(quotaUser);
}
@Override
public UpdateAccessApprovalSettings setUploadType(java.lang.String uploadType) {
return (UpdateAccessApprovalSettings) super.setUploadType(uploadType);
}
@Override
public UpdateAccessApprovalSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateAccessApprovalSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* The resource name of the settings. Format is one of: *
* "projects/{project}/accessApprovalSettings" * "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource name of the settings. Format is one of: * "projects/{project}/accessApprovalSettings"
* "folders/{folder}/accessApprovalSettings" * "organizations/{organization}/accessApprovalSettings"
*/
public java.lang.String getName() {
return name;
}
/**
* The resource name of the settings. Format is one of: *
* "projects/{project}/accessApprovalSettings" * "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
*/
public UpdateAccessApprovalSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/accessApprovalSettings$");
}
this.name = name;
return this;
}
/**
* The update mask applies to the settings. Only the top level fields of
* AccessApprovalSettings (notification_emails & enrolled_services) are supported. For each
* field, if it is included, the currently stored value will be entirely overwritten with the
* value of the field passed in this request. For the `FieldMask` definition, see
* https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#fieldmask If
* this field is left unset, only the notification_emails field will be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The update mask applies to the settings. Only the top level fields of AccessApprovalSettings
(notification_emails & enrolled_services) are supported. For each field, if it is included, the
currently stored value will be entirely overwritten with the value of the field passed in this
request. For the `FieldMask` definition, see https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#fieldmask If this field is left unset, only the
notification_emails field will be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The update mask applies to the settings. Only the top level fields of
* AccessApprovalSettings (notification_emails & enrolled_services) are supported. For each
* field, if it is included, the currently stored value will be entirely overwritten with the
* value of the field passed in this request. For the `FieldMask` definition, see
* https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#fieldmask If
* this field is left unset, only the notification_emails field will be updated.
*/
public UpdateAccessApprovalSettings setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateAccessApprovalSettings set(String parameterName, Object value) {
return (UpdateAccessApprovalSettings) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the ApprovalRequests collection.
*
* The typical use is:
*
* {@code AccessApproval accessapproval = new AccessApproval(...);}
* {@code AccessApproval.ApprovalRequests.List request = accessapproval.approvalRequests().list(parameters ...)}
*
*
* @return the resource collection
*/
public ApprovalRequests approvalRequests() {
return new ApprovalRequests();
}
/**
* The "approvalRequests" collection of methods.
*/
public class ApprovalRequests {
/**
* Approves a request and returns the updated ApprovalRequest. Returns NOT_FOUND if the request does
* not exist. Returns FAILED_PRECONDITION if the request exists but is not in a pending state.
*
* Create a request for the method "approvalRequests.approve".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Approve#execute()} method to invoke the remote operation.
*
* @param name Name of the approval request to approve.
* @param content the {@link com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage}
* @return the request
*/
public Approve approve(java.lang.String name, com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage content) throws java.io.IOException {
Approve result = new Approve(name, content);
initialize(result);
return result;
}
public class Approve extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}:approve";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/approvalRequests/[^/]+$");
/**
* Approves a request and returns the updated ApprovalRequest. Returns NOT_FOUND if the request
* does not exist. Returns FAILED_PRECONDITION if the request exists but is not in a pending
* state.
*
* Create a request for the method "approvalRequests.approve".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Approve#execute()} method to invoke the remote operation.
* {@link
* Approve#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the approval request to approve.
* @param content the {@link com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage}
* @since 1.13
*/
protected Approve(java.lang.String name, com.google.api.services.accessapproval.v1.model.ApproveApprovalRequestMessage content) {
super(AccessApproval.this, "POST", REST_PATH, content, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public Approve set$Xgafv(java.lang.String $Xgafv) {
return (Approve) super.set$Xgafv($Xgafv);
}
@Override
public Approve setAccessToken(java.lang.String accessToken) {
return (Approve) super.setAccessToken(accessToken);
}
@Override
public Approve setAlt(java.lang.String alt) {
return (Approve) super.setAlt(alt);
}
@Override
public Approve setCallback(java.lang.String callback) {
return (Approve) super.setCallback(callback);
}
@Override
public Approve setFields(java.lang.String fields) {
return (Approve) super.setFields(fields);
}
@Override
public Approve setKey(java.lang.String key) {
return (Approve) super.setKey(key);
}
@Override
public Approve setOauthToken(java.lang.String oauthToken) {
return (Approve) super.setOauthToken(oauthToken);
}
@Override
public Approve setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Approve) super.setPrettyPrint(prettyPrint);
}
@Override
public Approve setQuotaUser(java.lang.String quotaUser) {
return (Approve) super.setQuotaUser(quotaUser);
}
@Override
public Approve setUploadType(java.lang.String uploadType) {
return (Approve) super.setUploadType(uploadType);
}
@Override
public Approve setUploadProtocol(java.lang.String uploadProtocol) {
return (Approve) super.setUploadProtocol(uploadProtocol);
}
/** Name of the approval request to approve. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the approval request to approve.
*/
public java.lang.String getName() {
return name;
}
/** Name of the approval request to approve. */
public Approve setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Approve set(String parameterName, Object value) {
return (Approve) super.set(parameterName, value);
}
}
/**
* Dismisses a request. Returns the updated ApprovalRequest. NOTE: This does not deny access to the
* resource if another request has been made and approved. It is equivalent in effect to ignoring
* the request altogether. Returns NOT_FOUND if the request does not exist. Returns
* FAILED_PRECONDITION if the request exists but is not in a pending state.
*
* Create a request for the method "approvalRequests.dismiss".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
*
* @param name Name of the ApprovalRequest to dismiss.
* @param content the {@link com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage}
* @return the request
*/
public Dismiss dismiss(java.lang.String name, com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage content) throws java.io.IOException {
Dismiss result = new Dismiss(name, content);
initialize(result);
return result;
}
public class Dismiss extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}:dismiss";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/approvalRequests/[^/]+$");
/**
* Dismisses a request. Returns the updated ApprovalRequest. NOTE: This does not deny access to
* the resource if another request has been made and approved. It is equivalent in effect to
* ignoring the request altogether. Returns NOT_FOUND if the request does not exist. Returns
* FAILED_PRECONDITION if the request exists but is not in a pending state.
*
* Create a request for the method "approvalRequests.dismiss".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
* {@link
* Dismiss#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the ApprovalRequest to dismiss.
* @param content the {@link com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage}
* @since 1.13
*/
protected Dismiss(java.lang.String name, com.google.api.services.accessapproval.v1.model.DismissApprovalRequestMessage content) {
super(AccessApproval.this, "POST", REST_PATH, content, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public Dismiss set$Xgafv(java.lang.String $Xgafv) {
return (Dismiss) super.set$Xgafv($Xgafv);
}
@Override
public Dismiss setAccessToken(java.lang.String accessToken) {
return (Dismiss) super.setAccessToken(accessToken);
}
@Override
public Dismiss setAlt(java.lang.String alt) {
return (Dismiss) super.setAlt(alt);
}
@Override
public Dismiss setCallback(java.lang.String callback) {
return (Dismiss) super.setCallback(callback);
}
@Override
public Dismiss setFields(java.lang.String fields) {
return (Dismiss) super.setFields(fields);
}
@Override
public Dismiss setKey(java.lang.String key) {
return (Dismiss) super.setKey(key);
}
@Override
public Dismiss setOauthToken(java.lang.String oauthToken) {
return (Dismiss) super.setOauthToken(oauthToken);
}
@Override
public Dismiss setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Dismiss) super.setPrettyPrint(prettyPrint);
}
@Override
public Dismiss setQuotaUser(java.lang.String quotaUser) {
return (Dismiss) super.setQuotaUser(quotaUser);
}
@Override
public Dismiss setUploadType(java.lang.String uploadType) {
return (Dismiss) super.setUploadType(uploadType);
}
@Override
public Dismiss setUploadProtocol(java.lang.String uploadProtocol) {
return (Dismiss) super.setUploadProtocol(uploadProtocol);
}
/** Name of the ApprovalRequest to dismiss. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the ApprovalRequest to dismiss.
*/
public java.lang.String getName() {
return name;
}
/** Name of the ApprovalRequest to dismiss. */
public Dismiss setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Dismiss set(String parameterName, Object value) {
return (Dismiss) super.set(parameterName, value);
}
}
/**
* Gets an approval request. Returns NOT_FOUND if the request does not exist.
*
* Create a request for the method "approvalRequests.get".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/approvalRequests/[^/]+$");
/**
* Gets an approval request. Returns NOT_FOUND if the request does not exist.
*
* Create a request for the method "approvalRequests.get".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the approval request to retrieve. Format:
"{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the approval request to retrieve. Format:
* "{projects|folders|organizations}/{id}/approvalRequests/{approval_request}"
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Invalidates an existing ApprovalRequest. Returns the updated ApprovalRequest. NOTE: This does not
* deny access to the resource if another request has been made and approved. It only invalidates a
* single approval. Returns FAILED_PRECONDITION if the request exists but is not in an approved
* state.
*
* Create a request for the method "approvalRequests.invalidate".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link Invalidate#execute()} method to invoke the remote operation.
*
* @param name Name of the ApprovalRequest to invalidate.
* @param content the {@link com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage}
* @return the request
*/
public Invalidate invalidate(java.lang.String name, com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage content) throws java.io.IOException {
Invalidate result = new Invalidate(name, content);
initialize(result);
return result;
}
public class Invalidate extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+name}:invalidate";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/approvalRequests/[^/]+$");
/**
* Invalidates an existing ApprovalRequest. Returns the updated ApprovalRequest. NOTE: This does
* not deny access to the resource if another request has been made and approved. It only
* invalidates a single approval. Returns FAILED_PRECONDITION if the request exists but is not in
* an approved state.
*
* Create a request for the method "approvalRequests.invalidate".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link Invalidate#execute()} method to invoke the remote
* operation. {@link
* Invalidate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the ApprovalRequest to invalidate.
* @param content the {@link com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage}
* @since 1.13
*/
protected Invalidate(java.lang.String name, com.google.api.services.accessapproval.v1.model.InvalidateApprovalRequestMessage content) {
super(AccessApproval.this, "POST", REST_PATH, content, com.google.api.services.accessapproval.v1.model.ApprovalRequest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/approvalRequests/[^/]+$");
}
}
@Override
public Invalidate set$Xgafv(java.lang.String $Xgafv) {
return (Invalidate) super.set$Xgafv($Xgafv);
}
@Override
public Invalidate setAccessToken(java.lang.String accessToken) {
return (Invalidate) super.setAccessToken(accessToken);
}
@Override
public Invalidate setAlt(java.lang.String alt) {
return (Invalidate) super.setAlt(alt);
}
@Override
public Invalidate setCallback(java.lang.String callback) {
return (Invalidate) super.setCallback(callback);
}
@Override
public Invalidate setFields(java.lang.String fields) {
return (Invalidate) super.setFields(fields);
}
@Override
public Invalidate setKey(java.lang.String key) {
return (Invalidate) super.setKey(key);
}
@Override
public Invalidate setOauthToken(java.lang.String oauthToken) {
return (Invalidate) super.setOauthToken(oauthToken);
}
@Override
public Invalidate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Invalidate) super.setPrettyPrint(prettyPrint);
}
@Override
public Invalidate setQuotaUser(java.lang.String quotaUser) {
return (Invalidate) super.setQuotaUser(quotaUser);
}
@Override
public Invalidate setUploadType(java.lang.String uploadType) {
return (Invalidate) super.setUploadType(uploadType);
}
@Override
public Invalidate setUploadProtocol(java.lang.String uploadProtocol) {
return (Invalidate) super.setUploadProtocol(uploadProtocol);
}
/** Name of the ApprovalRequest to invalidate. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the ApprovalRequest to invalidate.
*/
public java.lang.String getName() {
return name;
}
/** Name of the ApprovalRequest to invalidate. */
public Invalidate setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/approvalRequests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Invalidate set(String parameterName, Object value) {
return (Invalidate) super.set(parameterName, value);
}
}
/**
* Lists approval requests associated with a project, folder, or organization. Approval requests can
* be filtered by state (pending, active, dismissed). The order is reverse chronological.
*
* Create a request for the method "approvalRequests.list".
*
* This request holds the parameters needed by the accessapproval server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AccessApprovalRequest {
private static final String REST_PATH = "v1/{+parent}/approvalRequests";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists approval requests associated with a project, folder, or organization. Approval requests
* can be filtered by state (pending, active, dismissed). The order is reverse chronological.
*
* Create a request for the method "approvalRequests.list".
*
* This request holds the parameters needed by the the accessapproval server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AccessApproval.this, "GET", REST_PATH, null, com.google.api.services.accessapproval.v1.model.ListApprovalRequestsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** The parent resource. This may be "projects/{project}", "folders/{folder}", or
"organizations/{organization}".
*/
public java.lang.String getParent() {
return parent;
}
/**
* The parent resource. This may be "projects/{project}", "folders/{folder}", or
* "organizations/{organization}".
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* A filter on the type of approval requests to retrieve. Must be one of the following
* values: * [not set]: Requests that are pending or have active approvals. * ALL: All
* requests. * PENDING: Only pending requests. * ACTIVE: Only active (i.e. currently
* approved) requests. * DISMISSED: Only requests that have been dismissed, or requests that
* are not approved and past expiration. * EXPIRED: Only requests that have been approved,
* and the approval has expired. * HISTORY: Active, dismissed and expired requests.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter on the type of approval requests to retrieve. Must be one of the following values: * [not
set]: Requests that are pending or have active approvals. * ALL: All requests. * PENDING: Only
pending requests. * ACTIVE: Only active (i.e. currently approved) requests. * DISMISSED: Only
requests that have been dismissed, or requests that are not approved and past expiration. *
EXPIRED: Only requests that have been approved, and the approval has expired. * HISTORY: Active,
dismissed and expired requests.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter on the type of approval requests to retrieve. Must be one of the following
* values: * [not set]: Requests that are pending or have active approvals. * ALL: All
* requests. * PENDING: Only pending requests. * ACTIVE: Only active (i.e. currently
* approved) requests. * DISMISSED: Only requests that have been dismissed, or requests that
* are not approved and past expiration. * EXPIRED: Only requests that have been approved,
* and the approval has expired. * HISTORY: Active, dismissed and expired requests.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Requested page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Requested page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token identifying the page of results to return. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying the page of results to return.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token identifying the page of results to return. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link AccessApproval}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link AccessApproval}. */
@Override
public AccessApproval build() {
return new AccessApproval(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link AccessApprovalRequestInitializer}.
*
* @since 1.12
*/
public Builder setAccessApprovalRequestInitializer(
AccessApprovalRequestInitializer accessapprovalRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(accessapprovalRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}