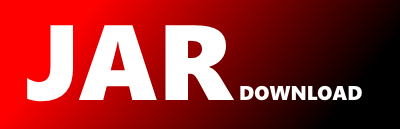
com.google.api.services.accessapproval.v1.model.AccessApprovalSettings Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.accessapproval.v1.model;
/**
* Settings on a Project/Folder/Organization related to Access Approval.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Access Approval API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AccessApprovalSettings extends com.google.api.client.json.GenericJson {
/**
* The asymmetric crypto key version to use for signing approval requests. Empty
* active_key_version indicates that a Google-managed key should be used for signing. This
* property will be ignored if set by an ancestor of this resource, and new non-empty values may
* not be set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String activeKeyVersion;
/**
* Output only. This field is read only (not settable via UpdateAccessApprovalSettings method). If
* the field is true, that indicates that an ancestor of this Project or Folder has set
* active_key_version (this field will always be unset for the organization since organizations do
* not have ancestors).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean ancestorHasActiveKeyVersion;
/**
* Output only. This field is read only (not settable via UpdateAccessApprovalSettings method). If
* the field is true, that indicates that at least one service is enrolled for Access Approval in
* one or more ancestors of the Project or Folder (this field will always be unset for the
* organization since organizations do not have ancestors).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enrolledAncestor;
/**
* A list of Google Cloud Services for which the given resource has Access Approval enrolled.
* Access requests for the resource given by name against any of these services contained here
* will be required to have explicit approval. If name refers to an organization, enrollment can
* be done for individual services. If name refers to a folder or project, enrollment can only be
* done on an all or nothing basis. If a cloud_product is repeated in this list, the first entry
* will be honored and all following entries will be discarded. A maximum of 10 enrolled services
* will be enforced, to be expanded as the set of supported services is expanded.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List enrolledServices;
/**
* Output only. This field is read only (not settable via UpdateAccessApprovalSettings method). If
* the field is true, that indicates that there is some configuration issue with the
* active_key_version configured at this level in the resource hierarchy (e.g. it doesn't exist or
* the Access Approval service account doesn't have the correct permissions on it, etc.) This key
* version is not necessarily the effective key version at this level, as key versions are
* inherited top-down.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean invalidKeyVersion;
/**
* The resource name of the settings. Format is one of: *
* "projects/{project}/accessApprovalSettings" * "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* A list of email addresses to which notifications relating to approval requests should be sent.
* Notifications relating to a resource will be sent to all emails in the settings of ancestor
* resources of that resource. A maximum of 50 email addresses are allowed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List notificationEmails;
/**
* Optional. A pubsub topic to which notifications relating to approval requests should be sent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String notificationPubsubTopic;
/**
* This preference is communicated to Google personnel when sending an approval request but can be
* overridden if necessary.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean preferNoBroadApprovalRequests;
/**
* This preference is shared with Google personnel, but can be overridden if said personnel deems
* necessary. The approver ultimately can set the expiration at approval time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer preferredRequestExpirationDays;
/**
* Optional. A setting to require approval request justifications to be customer visible.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean requireCustomerVisibleJustification;
/**
* The asymmetric crypto key version to use for signing approval requests. Empty
* active_key_version indicates that a Google-managed key should be used for signing. This
* property will be ignored if set by an ancestor of this resource, and new non-empty values may
* not be set.
* @return value or {@code null} for none
*/
public java.lang.String getActiveKeyVersion() {
return activeKeyVersion;
}
/**
* The asymmetric crypto key version to use for signing approval requests. Empty
* active_key_version indicates that a Google-managed key should be used for signing. This
* property will be ignored if set by an ancestor of this resource, and new non-empty values may
* not be set.
* @param activeKeyVersion activeKeyVersion or {@code null} for none
*/
public AccessApprovalSettings setActiveKeyVersion(java.lang.String activeKeyVersion) {
this.activeKeyVersion = activeKeyVersion;
return this;
}
/**
* Output only. This field is read only (not settable via UpdateAccessApprovalSettings method). If
* the field is true, that indicates that an ancestor of this Project or Folder has set
* active_key_version (this field will always be unset for the organization since organizations do
* not have ancestors).
* @return value or {@code null} for none
*/
public java.lang.Boolean getAncestorHasActiveKeyVersion() {
return ancestorHasActiveKeyVersion;
}
/**
* Output only. This field is read only (not settable via UpdateAccessApprovalSettings method). If
* the field is true, that indicates that an ancestor of this Project or Folder has set
* active_key_version (this field will always be unset for the organization since organizations do
* not have ancestors).
* @param ancestorHasActiveKeyVersion ancestorHasActiveKeyVersion or {@code null} for none
*/
public AccessApprovalSettings setAncestorHasActiveKeyVersion(java.lang.Boolean ancestorHasActiveKeyVersion) {
this.ancestorHasActiveKeyVersion = ancestorHasActiveKeyVersion;
return this;
}
/**
* Output only. This field is read only (not settable via UpdateAccessApprovalSettings method). If
* the field is true, that indicates that at least one service is enrolled for Access Approval in
* one or more ancestors of the Project or Folder (this field will always be unset for the
* organization since organizations do not have ancestors).
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnrolledAncestor() {
return enrolledAncestor;
}
/**
* Output only. This field is read only (not settable via UpdateAccessApprovalSettings method). If
* the field is true, that indicates that at least one service is enrolled for Access Approval in
* one or more ancestors of the Project or Folder (this field will always be unset for the
* organization since organizations do not have ancestors).
* @param enrolledAncestor enrolledAncestor or {@code null} for none
*/
public AccessApprovalSettings setEnrolledAncestor(java.lang.Boolean enrolledAncestor) {
this.enrolledAncestor = enrolledAncestor;
return this;
}
/**
* A list of Google Cloud Services for which the given resource has Access Approval enrolled.
* Access requests for the resource given by name against any of these services contained here
* will be required to have explicit approval. If name refers to an organization, enrollment can
* be done for individual services. If name refers to a folder or project, enrollment can only be
* done on an all or nothing basis. If a cloud_product is repeated in this list, the first entry
* will be honored and all following entries will be discarded. A maximum of 10 enrolled services
* will be enforced, to be expanded as the set of supported services is expanded.
* @return value or {@code null} for none
*/
public java.util.List getEnrolledServices() {
return enrolledServices;
}
/**
* A list of Google Cloud Services for which the given resource has Access Approval enrolled.
* Access requests for the resource given by name against any of these services contained here
* will be required to have explicit approval. If name refers to an organization, enrollment can
* be done for individual services. If name refers to a folder or project, enrollment can only be
* done on an all or nothing basis. If a cloud_product is repeated in this list, the first entry
* will be honored and all following entries will be discarded. A maximum of 10 enrolled services
* will be enforced, to be expanded as the set of supported services is expanded.
* @param enrolledServices enrolledServices or {@code null} for none
*/
public AccessApprovalSettings setEnrolledServices(java.util.List enrolledServices) {
this.enrolledServices = enrolledServices;
return this;
}
/**
* Output only. This field is read only (not settable via UpdateAccessApprovalSettings method). If
* the field is true, that indicates that there is some configuration issue with the
* active_key_version configured at this level in the resource hierarchy (e.g. it doesn't exist or
* the Access Approval service account doesn't have the correct permissions on it, etc.) This key
* version is not necessarily the effective key version at this level, as key versions are
* inherited top-down.
* @return value or {@code null} for none
*/
public java.lang.Boolean getInvalidKeyVersion() {
return invalidKeyVersion;
}
/**
* Output only. This field is read only (not settable via UpdateAccessApprovalSettings method). If
* the field is true, that indicates that there is some configuration issue with the
* active_key_version configured at this level in the resource hierarchy (e.g. it doesn't exist or
* the Access Approval service account doesn't have the correct permissions on it, etc.) This key
* version is not necessarily the effective key version at this level, as key versions are
* inherited top-down.
* @param invalidKeyVersion invalidKeyVersion or {@code null} for none
*/
public AccessApprovalSettings setInvalidKeyVersion(java.lang.Boolean invalidKeyVersion) {
this.invalidKeyVersion = invalidKeyVersion;
return this;
}
/**
* The resource name of the settings. Format is one of: *
* "projects/{project}/accessApprovalSettings" * "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The resource name of the settings. Format is one of: *
* "projects/{project}/accessApprovalSettings" * "folders/{folder}/accessApprovalSettings" *
* "organizations/{organization}/accessApprovalSettings"
* @param name name or {@code null} for none
*/
public AccessApprovalSettings setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* A list of email addresses to which notifications relating to approval requests should be sent.
* Notifications relating to a resource will be sent to all emails in the settings of ancestor
* resources of that resource. A maximum of 50 email addresses are allowed.
* @return value or {@code null} for none
*/
public java.util.List getNotificationEmails() {
return notificationEmails;
}
/**
* A list of email addresses to which notifications relating to approval requests should be sent.
* Notifications relating to a resource will be sent to all emails in the settings of ancestor
* resources of that resource. A maximum of 50 email addresses are allowed.
* @param notificationEmails notificationEmails or {@code null} for none
*/
public AccessApprovalSettings setNotificationEmails(java.util.List notificationEmails) {
this.notificationEmails = notificationEmails;
return this;
}
/**
* Optional. A pubsub topic to which notifications relating to approval requests should be sent.
* @return value or {@code null} for none
*/
public java.lang.String getNotificationPubsubTopic() {
return notificationPubsubTopic;
}
/**
* Optional. A pubsub topic to which notifications relating to approval requests should be sent.
* @param notificationPubsubTopic notificationPubsubTopic or {@code null} for none
*/
public AccessApprovalSettings setNotificationPubsubTopic(java.lang.String notificationPubsubTopic) {
this.notificationPubsubTopic = notificationPubsubTopic;
return this;
}
/**
* This preference is communicated to Google personnel when sending an approval request but can be
* overridden if necessary.
* @return value or {@code null} for none
*/
public java.lang.Boolean getPreferNoBroadApprovalRequests() {
return preferNoBroadApprovalRequests;
}
/**
* This preference is communicated to Google personnel when sending an approval request but can be
* overridden if necessary.
* @param preferNoBroadApprovalRequests preferNoBroadApprovalRequests or {@code null} for none
*/
public AccessApprovalSettings setPreferNoBroadApprovalRequests(java.lang.Boolean preferNoBroadApprovalRequests) {
this.preferNoBroadApprovalRequests = preferNoBroadApprovalRequests;
return this;
}
/**
* This preference is shared with Google personnel, but can be overridden if said personnel deems
* necessary. The approver ultimately can set the expiration at approval time.
* @return value or {@code null} for none
*/
public java.lang.Integer getPreferredRequestExpirationDays() {
return preferredRequestExpirationDays;
}
/**
* This preference is shared with Google personnel, but can be overridden if said personnel deems
* necessary. The approver ultimately can set the expiration at approval time.
* @param preferredRequestExpirationDays preferredRequestExpirationDays or {@code null} for none
*/
public AccessApprovalSettings setPreferredRequestExpirationDays(java.lang.Integer preferredRequestExpirationDays) {
this.preferredRequestExpirationDays = preferredRequestExpirationDays;
return this;
}
/**
* Optional. A setting to require approval request justifications to be customer visible.
* @return value or {@code null} for none
*/
public java.lang.Boolean getRequireCustomerVisibleJustification() {
return requireCustomerVisibleJustification;
}
/**
* Optional. A setting to require approval request justifications to be customer visible.
* @param requireCustomerVisibleJustification requireCustomerVisibleJustification or {@code null} for none
*/
public AccessApprovalSettings setRequireCustomerVisibleJustification(java.lang.Boolean requireCustomerVisibleJustification) {
this.requireCustomerVisibleJustification = requireCustomerVisibleJustification;
return this;
}
@Override
public AccessApprovalSettings set(String fieldName, Object value) {
return (AccessApprovalSettings) super.set(fieldName, value);
}
@Override
public AccessApprovalSettings clone() {
return (AccessApprovalSettings) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy