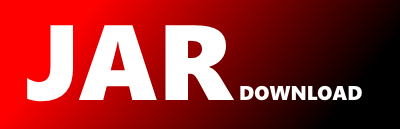
com.google.api.services.accessapproval.v1.model.EnrolledService Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.accessapproval.v1.model;
/**
* Represents the enrollment of a cloud resource into a specific service.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Access Approval API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class EnrolledService extends com.google.api.client.json.GenericJson {
/**
* The product for which Access Approval will be enrolled. Allowed values are listed below (case-
* sensitive): * all * GA * App Engine * Artifact Registry * BigQuery * Certificate Authority
* Service * Cloud Bigtable * Cloud Key Management Service * Compute Engine * Cloud Composer *
* Cloud Dataflow * Cloud Dataproc * Cloud DLP * Cloud EKM * Cloud Firestore * Cloud HSM * Cloud
* Identity and Access Management * Cloud Logging * Cloud NAT * Cloud Pub/Sub * Cloud Spanner *
* Cloud SQL * Cloud Storage * Eventarc * Google Kubernetes Engine * Organization Policy Serivice
* * Persistent Disk * Resource Manager * Secret Manager * Speaker ID Note: These values are
* supported as input for legacy purposes, but will not be returned from the API. * all * ga-only
* * appengine.googleapis.com * artifactregistry.googleapis.com * bigquery.googleapis.com *
* bigtable.googleapis.com * container.googleapis.com * cloudkms.googleapis.com *
* cloudresourcemanager.googleapis.com * cloudsql.googleapis.com * compute.googleapis.com *
* dataflow.googleapis.com * dataproc.googleapis.com * dlp.googleapis.com * iam.googleapis.com *
* logging.googleapis.com * orgpolicy.googleapis.com * pubsub.googleapis.com *
* spanner.googleapis.com * secretmanager.googleapis.com * speakerid.googleapis.com *
* storage.googleapis.com Calls to UpdateAccessApprovalSettings using 'all' or any of the
* XXX.googleapis.com will be translated to the associated product name ('all', 'App Engine',
* etc.). Note: 'all' will enroll the resource in all products supported at both 'GA' and
* 'Preview' levels. More information about levels of support is available at
* https://cloud.google.com/access-approval/docs/supported-services
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cloudProduct;
/**
* The enrollment level of the service.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String enrollmentLevel;
/**
* The product for which Access Approval will be enrolled. Allowed values are listed below (case-
* sensitive): * all * GA * App Engine * Artifact Registry * BigQuery * Certificate Authority
* Service * Cloud Bigtable * Cloud Key Management Service * Compute Engine * Cloud Composer *
* Cloud Dataflow * Cloud Dataproc * Cloud DLP * Cloud EKM * Cloud Firestore * Cloud HSM * Cloud
* Identity and Access Management * Cloud Logging * Cloud NAT * Cloud Pub/Sub * Cloud Spanner *
* Cloud SQL * Cloud Storage * Eventarc * Google Kubernetes Engine * Organization Policy Serivice
* * Persistent Disk * Resource Manager * Secret Manager * Speaker ID Note: These values are
* supported as input for legacy purposes, but will not be returned from the API. * all * ga-only
* * appengine.googleapis.com * artifactregistry.googleapis.com * bigquery.googleapis.com *
* bigtable.googleapis.com * container.googleapis.com * cloudkms.googleapis.com *
* cloudresourcemanager.googleapis.com * cloudsql.googleapis.com * compute.googleapis.com *
* dataflow.googleapis.com * dataproc.googleapis.com * dlp.googleapis.com * iam.googleapis.com *
* logging.googleapis.com * orgpolicy.googleapis.com * pubsub.googleapis.com *
* spanner.googleapis.com * secretmanager.googleapis.com * speakerid.googleapis.com *
* storage.googleapis.com Calls to UpdateAccessApprovalSettings using 'all' or any of the
* XXX.googleapis.com will be translated to the associated product name ('all', 'App Engine',
* etc.). Note: 'all' will enroll the resource in all products supported at both 'GA' and
* 'Preview' levels. More information about levels of support is available at
* https://cloud.google.com/access-approval/docs/supported-services
* @return value or {@code null} for none
*/
public java.lang.String getCloudProduct() {
return cloudProduct;
}
/**
* The product for which Access Approval will be enrolled. Allowed values are listed below (case-
* sensitive): * all * GA * App Engine * Artifact Registry * BigQuery * Certificate Authority
* Service * Cloud Bigtable * Cloud Key Management Service * Compute Engine * Cloud Composer *
* Cloud Dataflow * Cloud Dataproc * Cloud DLP * Cloud EKM * Cloud Firestore * Cloud HSM * Cloud
* Identity and Access Management * Cloud Logging * Cloud NAT * Cloud Pub/Sub * Cloud Spanner *
* Cloud SQL * Cloud Storage * Eventarc * Google Kubernetes Engine * Organization Policy Serivice
* * Persistent Disk * Resource Manager * Secret Manager * Speaker ID Note: These values are
* supported as input for legacy purposes, but will not be returned from the API. * all * ga-only
* * appengine.googleapis.com * artifactregistry.googleapis.com * bigquery.googleapis.com *
* bigtable.googleapis.com * container.googleapis.com * cloudkms.googleapis.com *
* cloudresourcemanager.googleapis.com * cloudsql.googleapis.com * compute.googleapis.com *
* dataflow.googleapis.com * dataproc.googleapis.com * dlp.googleapis.com * iam.googleapis.com *
* logging.googleapis.com * orgpolicy.googleapis.com * pubsub.googleapis.com *
* spanner.googleapis.com * secretmanager.googleapis.com * speakerid.googleapis.com *
* storage.googleapis.com Calls to UpdateAccessApprovalSettings using 'all' or any of the
* XXX.googleapis.com will be translated to the associated product name ('all', 'App Engine',
* etc.). Note: 'all' will enroll the resource in all products supported at both 'GA' and
* 'Preview' levels. More information about levels of support is available at
* https://cloud.google.com/access-approval/docs/supported-services
* @param cloudProduct cloudProduct or {@code null} for none
*/
public EnrolledService setCloudProduct(java.lang.String cloudProduct) {
this.cloudProduct = cloudProduct;
return this;
}
/**
* The enrollment level of the service.
* @return value or {@code null} for none
*/
public java.lang.String getEnrollmentLevel() {
return enrollmentLevel;
}
/**
* The enrollment level of the service.
* @param enrollmentLevel enrollmentLevel or {@code null} for none
*/
public EnrolledService setEnrollmentLevel(java.lang.String enrollmentLevel) {
this.enrollmentLevel = enrollmentLevel;
return this;
}
@Override
public EnrolledService set(String fieldName, Object value) {
return (EnrolledService) super.set(fieldName, value);
}
@Override
public EnrolledService clone() {
return (EnrolledService) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy