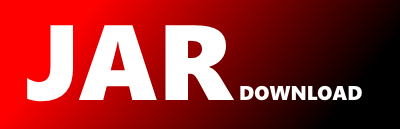
com.google.api.services.accesscontextmanager.v1.model.ScopedAccessSettings Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.accesscontextmanager.v1.model;
/**
* A relationship between access settings and its scope.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Access Context Manager API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ScopedAccessSettings extends com.google.api.client.json.GenericJson {
/**
* Optional. Access settings for this scoped access settings. This field may be empty if
* dry_run_settings is set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AccessSettings activeSettings;
/**
* Optional. Dry-run access settings for this scoped access settings. This field may be empty if
* active_settings is set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AccessSettings dryRunSettings;
/**
* Optional. Application, etc. to which the access settings will be applied to. Implicitly, this
* is the scoped access settings key; as such, it must be unique and non-empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AccessScope scope;
/**
* Optional. Access settings for this scoped access settings. This field may be empty if
* dry_run_settings is set.
* @return value or {@code null} for none
*/
public AccessSettings getActiveSettings() {
return activeSettings;
}
/**
* Optional. Access settings for this scoped access settings. This field may be empty if
* dry_run_settings is set.
* @param activeSettings activeSettings or {@code null} for none
*/
public ScopedAccessSettings setActiveSettings(AccessSettings activeSettings) {
this.activeSettings = activeSettings;
return this;
}
/**
* Optional. Dry-run access settings for this scoped access settings. This field may be empty if
* active_settings is set.
* @return value or {@code null} for none
*/
public AccessSettings getDryRunSettings() {
return dryRunSettings;
}
/**
* Optional. Dry-run access settings for this scoped access settings. This field may be empty if
* active_settings is set.
* @param dryRunSettings dryRunSettings or {@code null} for none
*/
public ScopedAccessSettings setDryRunSettings(AccessSettings dryRunSettings) {
this.dryRunSettings = dryRunSettings;
return this;
}
/**
* Optional. Application, etc. to which the access settings will be applied to. Implicitly, this
* is the scoped access settings key; as such, it must be unique and non-empty.
* @return value or {@code null} for none
*/
public AccessScope getScope() {
return scope;
}
/**
* Optional. Application, etc. to which the access settings will be applied to. Implicitly, this
* is the scoped access settings key; as such, it must be unique and non-empty.
* @param scope scope or {@code null} for none
*/
public ScopedAccessSettings setScope(AccessScope scope) {
this.scope = scope;
return this;
}
@Override
public ScopedAccessSettings set(String fieldName, Object value) {
return (ScopedAccessSettings) super.set(fieldName, value);
}
@Override
public ScopedAccessSettings clone() {
return (ScopedAccessSettings) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy