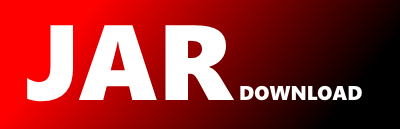
com.google.api.services.accesscontextmanager.v1.model.ServicePerimeter Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.accesscontextmanager.v1.model;
/**
* `ServicePerimeter` describes a set of Google Cloud resources which can freely import and export
* data amongst themselves, but not export outside of the `ServicePerimeter`. If a request with a
* source within this `ServicePerimeter` has a target outside of the `ServicePerimeter`, the request
* will be blocked. Otherwise the request is allowed. There are two types of Service Perimeter -
* Regular and Bridge. Regular Service Perimeters cannot overlap, a single Google Cloud project or
* VPC network can only belong to a single regular Service Perimeter. Service Perimeter Bridges can
* contain only Google Cloud projects as members, a single Google Cloud project may belong to
* multiple Service Perimeter Bridges.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Access Context Manager API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ServicePerimeter extends com.google.api.client.json.GenericJson {
/**
* Description of the `ServicePerimeter` and its use. Does not affect behavior.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Identifier. Resource name for the `ServicePerimeter`. Format:
* `accessPolicies/{access_policy}/servicePerimeters/{service_perimeter}`. The `service_perimeter`
* component must begin with a letter, followed by alphanumeric characters or `_`. After you
* create a `ServicePerimeter`, you cannot change its `name`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Perimeter type indicator. A single project or VPC network is allowed to be a member of single
* regular perimeter, but multiple service perimeter bridges. A project cannot be a included in a
* perimeter bridge without being included in regular perimeter. For perimeter bridges, the
* restricted service list as well as access level lists must be empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String perimeterType;
/**
* Proposed (or dry run) ServicePerimeter configuration. This configuration allows to specify and
* test ServicePerimeter configuration without enforcing actual access restrictions. Only allowed
* to be set when the "use_explicit_dry_run_spec" flag is set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ServicePerimeterConfig spec;
/**
* Current ServicePerimeter configuration. Specifies sets of resources, restricted services and
* access levels that determine perimeter content and boundaries.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ServicePerimeterConfig status;
/**
* Human readable title. Must be unique within the Policy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* Use explicit dry run spec flag. Ordinarily, a dry-run spec implicitly exists for all Service
* Perimeters, and that spec is identical to the status for those Service Perimeters. When this
* flag is set, it inhibits the generation of the implicit spec, thereby allowing the user to
* explicitly provide a configuration ("spec") to use in a dry-run version of the Service
* Perimeter. This allows the user to test changes to the enforced config ("status") without
* actually enforcing them. This testing is done through analyzing the differences between
* currently enforced and suggested restrictions. use_explicit_dry_run_spec must bet set to True
* if any of the fields in the spec are set to non-default values.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useExplicitDryRunSpec;
/**
* Description of the `ServicePerimeter` and its use. Does not affect behavior.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Description of the `ServicePerimeter` and its use. Does not affect behavior.
* @param description description or {@code null} for none
*/
public ServicePerimeter setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Identifier. Resource name for the `ServicePerimeter`. Format:
* `accessPolicies/{access_policy}/servicePerimeters/{service_perimeter}`. The `service_perimeter`
* component must begin with a letter, followed by alphanumeric characters or `_`. After you
* create a `ServicePerimeter`, you cannot change its `name`.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. Resource name for the `ServicePerimeter`. Format:
* `accessPolicies/{access_policy}/servicePerimeters/{service_perimeter}`. The `service_perimeter`
* component must begin with a letter, followed by alphanumeric characters or `_`. After you
* create a `ServicePerimeter`, you cannot change its `name`.
* @param name name or {@code null} for none
*/
public ServicePerimeter setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Perimeter type indicator. A single project or VPC network is allowed to be a member of single
* regular perimeter, but multiple service perimeter bridges. A project cannot be a included in a
* perimeter bridge without being included in regular perimeter. For perimeter bridges, the
* restricted service list as well as access level lists must be empty.
* @return value or {@code null} for none
*/
public java.lang.String getPerimeterType() {
return perimeterType;
}
/**
* Perimeter type indicator. A single project or VPC network is allowed to be a member of single
* regular perimeter, but multiple service perimeter bridges. A project cannot be a included in a
* perimeter bridge without being included in regular perimeter. For perimeter bridges, the
* restricted service list as well as access level lists must be empty.
* @param perimeterType perimeterType or {@code null} for none
*/
public ServicePerimeter setPerimeterType(java.lang.String perimeterType) {
this.perimeterType = perimeterType;
return this;
}
/**
* Proposed (or dry run) ServicePerimeter configuration. This configuration allows to specify and
* test ServicePerimeter configuration without enforcing actual access restrictions. Only allowed
* to be set when the "use_explicit_dry_run_spec" flag is set.
* @return value or {@code null} for none
*/
public ServicePerimeterConfig getSpec() {
return spec;
}
/**
* Proposed (or dry run) ServicePerimeter configuration. This configuration allows to specify and
* test ServicePerimeter configuration without enforcing actual access restrictions. Only allowed
* to be set when the "use_explicit_dry_run_spec" flag is set.
* @param spec spec or {@code null} for none
*/
public ServicePerimeter setSpec(ServicePerimeterConfig spec) {
this.spec = spec;
return this;
}
/**
* Current ServicePerimeter configuration. Specifies sets of resources, restricted services and
* access levels that determine perimeter content and boundaries.
* @return value or {@code null} for none
*/
public ServicePerimeterConfig getStatus() {
return status;
}
/**
* Current ServicePerimeter configuration. Specifies sets of resources, restricted services and
* access levels that determine perimeter content and boundaries.
* @param status status or {@code null} for none
*/
public ServicePerimeter setStatus(ServicePerimeterConfig status) {
this.status = status;
return this;
}
/**
* Human readable title. Must be unique within the Policy.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* Human readable title. Must be unique within the Policy.
* @param title title or {@code null} for none
*/
public ServicePerimeter setTitle(java.lang.String title) {
this.title = title;
return this;
}
/**
* Use explicit dry run spec flag. Ordinarily, a dry-run spec implicitly exists for all Service
* Perimeters, and that spec is identical to the status for those Service Perimeters. When this
* flag is set, it inhibits the generation of the implicit spec, thereby allowing the user to
* explicitly provide a configuration ("spec") to use in a dry-run version of the Service
* Perimeter. This allows the user to test changes to the enforced config ("status") without
* actually enforcing them. This testing is done through analyzing the differences between
* currently enforced and suggested restrictions. use_explicit_dry_run_spec must bet set to True
* if any of the fields in the spec are set to non-default values.
* @return value or {@code null} for none
*/
public java.lang.Boolean getUseExplicitDryRunSpec() {
return useExplicitDryRunSpec;
}
/**
* Use explicit dry run spec flag. Ordinarily, a dry-run spec implicitly exists for all Service
* Perimeters, and that spec is identical to the status for those Service Perimeters. When this
* flag is set, it inhibits the generation of the implicit spec, thereby allowing the user to
* explicitly provide a configuration ("spec") to use in a dry-run version of the Service
* Perimeter. This allows the user to test changes to the enforced config ("status") without
* actually enforcing them. This testing is done through analyzing the differences between
* currently enforced and suggested restrictions. use_explicit_dry_run_spec must bet set to True
* if any of the fields in the spec are set to non-default values.
* @param useExplicitDryRunSpec useExplicitDryRunSpec or {@code null} for none
*/
public ServicePerimeter setUseExplicitDryRunSpec(java.lang.Boolean useExplicitDryRunSpec) {
this.useExplicitDryRunSpec = useExplicitDryRunSpec;
return this;
}
@Override
public ServicePerimeter set(String fieldName, Object value) {
return (ServicePerimeter) super.set(fieldName, value);
}
@Override
public ServicePerimeter clone() {
return (ServicePerimeter) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy