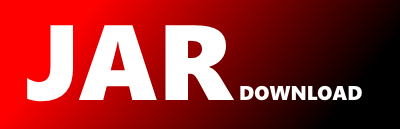
com.google.api.services.accesscontextmanager.v1.model.SupportedService Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.accesscontextmanager.v1.model;
/**
* `SupportedService` specifies the VPC Service Controls and its properties.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Access Context Manager API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class SupportedService extends com.google.api.client.json.GenericJson {
/**
* True if the service is available on the restricted VIP. Services on the restricted VIP
* typically either support VPC Service Controls or are core infrastructure services required for
* the functioning of Google Cloud.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean availableOnRestrictedVip;
/**
* True if the service is supported with some limitations. Check
* [documentation](https://cloud.google.com/vpc-service-controls/docs/supported-products) for
* details.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean knownLimitations;
/**
* The service name or address of the supported service, such as `service.googleapis.com`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The support stage of the service.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serviceSupportStage;
/**
* The support stage of the service.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String supportStage;
/**
* The list of the supported methods. This field exists only in response to GetSupportedService
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List supportedMethods;
static {
// hack to force ProGuard to consider MethodSelector used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(MethodSelector.class);
}
/**
* The name of the supported product, such as 'Cloud Product API'.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* True if the service is available on the restricted VIP. Services on the restricted VIP
* typically either support VPC Service Controls or are core infrastructure services required for
* the functioning of Google Cloud.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAvailableOnRestrictedVip() {
return availableOnRestrictedVip;
}
/**
* True if the service is available on the restricted VIP. Services on the restricted VIP
* typically either support VPC Service Controls or are core infrastructure services required for
* the functioning of Google Cloud.
* @param availableOnRestrictedVip availableOnRestrictedVip or {@code null} for none
*/
public SupportedService setAvailableOnRestrictedVip(java.lang.Boolean availableOnRestrictedVip) {
this.availableOnRestrictedVip = availableOnRestrictedVip;
return this;
}
/**
* True if the service is supported with some limitations. Check
* [documentation](https://cloud.google.com/vpc-service-controls/docs/supported-products) for
* details.
* @return value or {@code null} for none
*/
public java.lang.Boolean getKnownLimitations() {
return knownLimitations;
}
/**
* True if the service is supported with some limitations. Check
* [documentation](https://cloud.google.com/vpc-service-controls/docs/supported-products) for
* details.
* @param knownLimitations knownLimitations or {@code null} for none
*/
public SupportedService setKnownLimitations(java.lang.Boolean knownLimitations) {
this.knownLimitations = knownLimitations;
return this;
}
/**
* The service name or address of the supported service, such as `service.googleapis.com`.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The service name or address of the supported service, such as `service.googleapis.com`.
* @param name name or {@code null} for none
*/
public SupportedService setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* The support stage of the service.
* @return value or {@code null} for none
*/
public java.lang.String getServiceSupportStage() {
return serviceSupportStage;
}
/**
* The support stage of the service.
* @param serviceSupportStage serviceSupportStage or {@code null} for none
*/
public SupportedService setServiceSupportStage(java.lang.String serviceSupportStage) {
this.serviceSupportStage = serviceSupportStage;
return this;
}
/**
* The support stage of the service.
* @return value or {@code null} for none
*/
public java.lang.String getSupportStage() {
return supportStage;
}
/**
* The support stage of the service.
* @param supportStage supportStage or {@code null} for none
*/
public SupportedService setSupportStage(java.lang.String supportStage) {
this.supportStage = supportStage;
return this;
}
/**
* The list of the supported methods. This field exists only in response to GetSupportedService
* @return value or {@code null} for none
*/
public java.util.List getSupportedMethods() {
return supportedMethods;
}
/**
* The list of the supported methods. This field exists only in response to GetSupportedService
* @param supportedMethods supportedMethods or {@code null} for none
*/
public SupportedService setSupportedMethods(java.util.List supportedMethods) {
this.supportedMethods = supportedMethods;
return this;
}
/**
* The name of the supported product, such as 'Cloud Product API'.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* The name of the supported product, such as 'Cloud Product API'.
* @param title title or {@code null} for none
*/
public SupportedService setTitle(java.lang.String title) {
this.title = title;
return this;
}
@Override
public SupportedService set(String fieldName, Object value) {
return (SupportedService) super.set(fieldName, value);
}
@Override
public SupportedService clone() {
return (SupportedService) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy