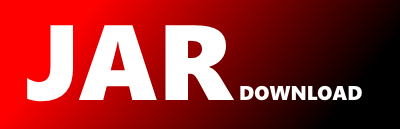
com.google.api.services.accesscontextmanager.v1.AccessContextManager Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.accesscontextmanager.v1;
/**
* Service definition for AccessContextManager (v1).
*
*
* An API for setting attribute based access control to requests to Google Cloud services. *Warning:* Do not mix *v1alpha* and *v1* API usage in the same access policy. The v1alpha API supports new Access Context Manager features, which may have different attributes or behaviors that are not supported by v1. The practice of mixed API usage within a policy may result in the inability to update that policy, including any access levels or service perimeters belonging to it. It is not recommended to use both v1 and v1alpha for modifying policies with critical service perimeters. Modifications using v1alpha should be limited to policies with non-production/non-critical service perimeters.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link AccessContextManagerRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class AccessContextManager extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Access Context Manager API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://accesscontextmanager.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://accesscontextmanager.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public AccessContextManager(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
AccessContextManager(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the AccessPolicies collection.
*
* The typical use is:
*
* {@code AccessContextManager accesscontextmanager = new AccessContextManager(...);}
* {@code AccessContextManager.AccessPolicies.List request = accesscontextmanager.accessPolicies().list(parameters ...)}
*
*
* @return the resource collection
*/
public AccessPolicies accessPolicies() {
return new AccessPolicies();
}
/**
* The "accessPolicies" collection of methods.
*/
public class AccessPolicies {
/**
* Creates an access policy. This method fails if the organization already has an access policy. The
* long-running operation has a successful status after the access policy propagates to long-lasting
* storage. Syntactic and basic semantic errors are returned in `metadata` as a BadRequest proto.
*
* Create a request for the method "accessPolicies.create".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AccessPolicy}
* @return the request
*/
public Create create(com.google.api.services.accesscontextmanager.v1.model.AccessPolicy content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/accessPolicies";
/**
* Creates an access policy. This method fails if the organization already has an access policy.
* The long-running operation has a successful status after the access policy propagates to long-
* lasting storage. Syntactic and basic semantic errors are returned in `metadata` as a BadRequest
* proto.
*
* Create a request for the method "accessPolicies.create".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AccessPolicy}
* @since 1.13
*/
protected Create(com.google.api.services.accesscontextmanager.v1.model.AccessPolicy content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an access policy based on the resource name. The long-running operation has a successful
* status after the access policy is removed from long-lasting storage.
*
* Create a request for the method "accessPolicies.delete".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name for the access policy to delete. Format `accessPolicies/{policy_id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Deletes an access policy based on the resource name. The long-running operation has a
* successful status after the access policy is removed from long-lasting storage.
*
* Create a request for the method "accessPolicies.delete".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name for the access policy to delete. Format `accessPolicies/{policy_id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AccessContextManager.this, "DELETE", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the access policy to delete. Format
* `accessPolicies/{policy_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name for the access policy to delete. Format `accessPolicies/{policy_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name for the access policy to delete. Format
* `accessPolicies/{policy_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns an access policy based on the name.
*
* Create a request for the method "accessPolicies.get".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name for the access policy to get. Format `accessPolicies/{policy_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Returns an access policy based on the name.
*
* Create a request for the method "accessPolicies.get".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name for the access policy to get. Format `accessPolicies/{policy_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.AccessPolicy.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the access policy to get. Format `accessPolicies/{policy_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name for the access policy to get. Format `accessPolicies/{policy_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name for the access policy to get. Format `accessPolicies/{policy_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the IAM policy for the specified Access Context Manager access policy.
*
* Create a request for the method "accessPolicies.getIamPolicy".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.accesscontextmanager.v1.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Gets the IAM policy for the specified Access Context Manager access policy.
*
* Create a request for the method "accessPolicies.getIamPolicy".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.accesscontextmanager.v1.model.GetIamPolicyRequest content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists all access policies in an organization.
*
* Create a request for the method "accessPolicies.list".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/accessPolicies";
/**
* Lists all access policies in an organization.
*
* Create a request for the method "accessPolicies.list".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.ListAccessPoliciesResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Number of AccessPolicy instances to include in the list. Default 100. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of AccessPolicy instances to include in the list. Default 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Number of AccessPolicy instances to include in the list. Default 100. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Next page token for the next batch of AccessPolicy instances. Defaults to the first page of
* results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Next page token for the next batch of AccessPolicy instances. Defaults to the first page of
results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Next page token for the next batch of AccessPolicy instances. Defaults to the first page of
* results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Required. Resource name for the container to list AccessPolicy instances from. Format:
* `organizations/{org_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name for the container to list AccessPolicy instances from. Format:
`organizations/{org_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name for the container to list AccessPolicy instances from. Format:
* `organizations/{org_id}`
*/
public List setParent(java.lang.String parent) {
this.parent = parent;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an access policy. The long-running operation from this RPC has a successful status after
* the changes to the access policy propagate to long-lasting storage.
*
* Create a request for the method "accessPolicies.patch".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Identifier. Resource name of the `AccessPolicy`. Format:
* `accessPolicies/{access_policy}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AccessPolicy}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.AccessPolicy content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Updates an access policy. The long-running operation from this RPC has a successful status
* after the changes to the access policy propagate to long-lasting storage.
*
* Create a request for the method "accessPolicies.patch".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Identifier. Resource name of the `AccessPolicy`. Format:
* `accessPolicies/{access_policy}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AccessPolicy}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.AccessPolicy content) {
super(AccessContextManager.this, "PATCH", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Identifier. Resource name of the `AccessPolicy`. Format:
* `accessPolicies/{access_policy}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Identifier. Resource name of the `AccessPolicy`. Format:
`accessPolicies/{access_policy}`
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Identifier. Resource name of the `AccessPolicy`. Format:
* `accessPolicies/{access_policy}`
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.name = name;
return this;
}
/** Required. Mask to control which fields get updated. Must be non-empty. */
@com.google.api.client.util.Key
private String updateMask;
/** Required. Mask to control which fields get updated. Must be non-empty.
*/
public String getUpdateMask() {
return updateMask;
}
/** Required. Mask to control which fields get updated. Must be non-empty. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the IAM policy for the specified Access Context Manager access policy. This method replaces
* the existing IAM policy on the access policy. The IAM policy controls the set of users who can
* perform specific operations on the Access Context Manager access policy.
*
* Create a request for the method "accessPolicies.setIamPolicy".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.accesscontextmanager.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Sets the IAM policy for the specified Access Context Manager access policy. This method
* replaces the existing IAM policy on the access policy. The IAM policy controls the set of users
* who can perform specific operations on the Access Context Manager access policy.
*
* Create a request for the method "accessPolicies.setIamPolicy".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.accesscontextmanager.v1.model.SetIamPolicyRequest content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns the IAM permissions that the caller has on the specified Access Context Manager resource.
* The resource can be an AccessPolicy, AccessLevel, or ServicePerimeter. This method does not
* support other resources.
*
* Create a request for the method "accessPolicies.testIamPermissions".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Returns the IAM permissions that the caller has on the specified Access Context Manager
* resource. The resource can be an AccessPolicy, AccessLevel, or ServicePerimeter. This method
* does not support other resources.
*
* Create a request for the method "accessPolicies.testIamPermissions".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the
* remote operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.ser
* vices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the AccessLevels collection.
*
* The typical use is:
*
* {@code AccessContextManager accesscontextmanager = new AccessContextManager(...);}
* {@code AccessContextManager.AccessLevels.List request = accesscontextmanager.accessLevels().list(parameters ...)}
*
*
* @return the resource collection
*/
public AccessLevels accessLevels() {
return new AccessLevels();
}
/**
* The "accessLevels" collection of methods.
*/
public class AccessLevels {
/**
* Creates an access level. The long-running operation from this RPC has a successful status after
* the access level propagates to long-lasting storage. If access levels contain errors, an error
* response is returned for the first error encountered.
*
* Create a request for the method "accessLevels.create".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name for the access policy which owns this Access Level. Format:
* `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AccessLevel}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.AccessLevel content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+parent}/accessLevels";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Creates an access level. The long-running operation from this RPC has a successful status after
* the access level propagates to long-lasting storage. If access levels contain errors, an error
* response is returned for the first error encountered.
*
* Create a request for the method "accessLevels.create".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name for the access policy which owns this Access Level. Format:
* `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AccessLevel}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.AccessLevel content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the access policy which owns this Access Level. Format:
* `accessPolicies/{policy_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name for the access policy which owns this Access Level. Format:
`accessPolicies/{policy_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name for the access policy which owns this Access Level. Format:
* `accessPolicies/{policy_id}`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an access level based on the resource name. The long-running operation from this RPC has
* a successful status after the access level has been removed from long-lasting storage.
*
* Create a request for the method "accessLevels.delete".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name for the Access Level. Format:
* `accessPolicies/{policy_id}/accessLevels/{access_level_id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+/accessLevels/[^/]+$");
/**
* Deletes an access level based on the resource name. The long-running operation from this RPC
* has a successful status after the access level has been removed from long-lasting storage.
*
* Create a request for the method "accessLevels.delete".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name for the Access Level. Format:
* `accessPolicies/{policy_id}/accessLevels/{access_level_id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AccessContextManager.this, "DELETE", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/accessLevels/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the Access Level. Format:
* `accessPolicies/{policy_id}/accessLevels/{access_level_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name for the Access Level. Format:
`accessPolicies/{policy_id}/accessLevels/{access_level_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name for the Access Level. Format:
* `accessPolicies/{policy_id}/accessLevels/{access_level_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/accessLevels/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an access level based on the resource name.
*
* Create a request for the method "accessLevels.get".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name for the Access Level. Format:
* `accessPolicies/{policy_id}/accessLevels/{access_level_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+/accessLevels/[^/]+$");
/**
* Gets an access level based on the resource name.
*
* Create a request for the method "accessLevels.get".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name for the Access Level. Format:
* `accessPolicies/{policy_id}/accessLevels/{access_level_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.AccessLevel.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/accessLevels/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the Access Level. Format:
* `accessPolicies/{policy_id}/accessLevels/{access_level_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name for the Access Level. Format:
`accessPolicies/{policy_id}/accessLevels/{access_level_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name for the Access Level. Format:
* `accessPolicies/{policy_id}/accessLevels/{access_level_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/accessLevels/[^/]+$");
}
this.name = name;
return this;
}
/**
* Whether to return `BasicLevels` in the Cloud Common Expression Language rather than as
* `BasicLevels`. Defaults to AS_DEFINED, where Access Levels are returned as `BasicLevels`
* or `CustomLevels` based on how they were created. If set to CEL, all Access Levels are
* returned as `CustomLevels`. In the CEL case, `BasicLevels` are translated to equivalent
* `CustomLevels`.
*/
@com.google.api.client.util.Key
private java.lang.String accessLevelFormat;
/** Whether to return `BasicLevels` in the Cloud Common Expression Language rather than as
`BasicLevels`. Defaults to AS_DEFINED, where Access Levels are returned as `BasicLevels` or
`CustomLevels` based on how they were created. If set to CEL, all Access Levels are returned as
`CustomLevels`. In the CEL case, `BasicLevels` are translated to equivalent `CustomLevels`.
*/
public java.lang.String getAccessLevelFormat() {
return accessLevelFormat;
}
/**
* Whether to return `BasicLevels` in the Cloud Common Expression Language rather than as
* `BasicLevels`. Defaults to AS_DEFINED, where Access Levels are returned as `BasicLevels`
* or `CustomLevels` based on how they were created. If set to CEL, all Access Levels are
* returned as `CustomLevels`. In the CEL case, `BasicLevels` are translated to equivalent
* `CustomLevels`.
*/
public Get setAccessLevelFormat(java.lang.String accessLevelFormat) {
this.accessLevelFormat = accessLevelFormat;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all access levels for an access policy.
*
* Create a request for the method "accessLevels.list".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name for the access policy to list Access Levels from. Format:
* `accessPolicies/{policy_id}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+parent}/accessLevels";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Lists all access levels for an access policy.
*
* Create a request for the method "accessLevels.list".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name for the access policy to list Access Levels from. Format:
* `accessPolicies/{policy_id}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.ListAccessLevelsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the access policy to list Access Levels from. Format:
* `accessPolicies/{policy_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name for the access policy to list Access Levels from. Format:
`accessPolicies/{policy_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name for the access policy to list Access Levels from. Format:
* `accessPolicies/{policy_id}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Whether to return `BasicLevels` in the Cloud Common Expression language, as
* `CustomLevels`, rather than as `BasicLevels`. Defaults to returning `AccessLevels` in the
* format they were defined.
*/
@com.google.api.client.util.Key
private java.lang.String accessLevelFormat;
/** Whether to return `BasicLevels` in the Cloud Common Expression language, as `CustomLevels`, rather
than as `BasicLevels`. Defaults to returning `AccessLevels` in the format they were defined.
*/
public java.lang.String getAccessLevelFormat() {
return accessLevelFormat;
}
/**
* Whether to return `BasicLevels` in the Cloud Common Expression language, as
* `CustomLevels`, rather than as `BasicLevels`. Defaults to returning `AccessLevels` in the
* format they were defined.
*/
public List setAccessLevelFormat(java.lang.String accessLevelFormat) {
this.accessLevelFormat = accessLevelFormat;
return this;
}
/** Number of Access Levels to include in the list. Default 100. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of Access Levels to include in the list. Default 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Number of Access Levels to include in the list. Default 100. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Next page token for the next batch of Access Level instances. Defaults to the first page
* of results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Next page token for the next batch of Access Level instances. Defaults to the first page of
results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Next page token for the next batch of Access Level instances. Defaults to the first page
* of results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an access level. The long-running operation from this RPC has a successful status after
* the changes to the access level propagate to long-lasting storage. If access levels contain
* errors, an error response is returned for the first error encountered.
*
* Create a request for the method "accessLevels.patch".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Identifier. Resource name for the `AccessLevel`. Format:
* `accessPolicies/{access_policy}/accessLevels/{access_level}`. The `access_level` component
* must begin with a letter, followed by alphanumeric characters or `_`. Its maximum length
* is 50 characters. After you create an `AccessLevel`, you cannot change its `name`.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AccessLevel}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.AccessLevel content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+/accessLevels/[^/]+$");
/**
* Updates an access level. The long-running operation from this RPC has a successful status after
* the changes to the access level propagate to long-lasting storage. If access levels contain
* errors, an error response is returned for the first error encountered.
*
* Create a request for the method "accessLevels.patch".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Identifier. Resource name for the `AccessLevel`. Format:
* `accessPolicies/{access_policy}/accessLevels/{access_level}`. The `access_level` component
* must begin with a letter, followed by alphanumeric characters or `_`. Its maximum length
* is 50 characters. After you create an `AccessLevel`, you cannot change its `name`.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AccessLevel}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.AccessLevel content) {
super(AccessContextManager.this, "PATCH", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/accessLevels/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Identifier. Resource name for the `AccessLevel`. Format:
* `accessPolicies/{access_policy}/accessLevels/{access_level}`. The `access_level`
* component must begin with a letter, followed by alphanumeric characters or `_`. Its
* maximum length is 50 characters. After you create an `AccessLevel`, you cannot change its
* `name`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Identifier. Resource name for the `AccessLevel`. Format:
`accessPolicies/{access_policy}/accessLevels/{access_level}`. The `access_level` component must
begin with a letter, followed by alphanumeric characters or `_`. Its maximum length is 50
characters. After you create an `AccessLevel`, you cannot change its `name`.
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. Resource name for the `AccessLevel`. Format:
* `accessPolicies/{access_policy}/accessLevels/{access_level}`. The `access_level`
* component must begin with a letter, followed by alphanumeric characters or `_`. Its
* maximum length is 50 characters. After you create an `AccessLevel`, you cannot change its
* `name`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/accessLevels/[^/]+$");
}
this.name = name;
return this;
}
/** Required. Mask to control which fields get updated. Must be non-empty. */
@com.google.api.client.util.Key
private String updateMask;
/** Required. Mask to control which fields get updated. Must be non-empty.
*/
public String getUpdateMask() {
return updateMask;
}
/** Required. Mask to control which fields get updated. Must be non-empty. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Replaces all existing access levels in an access policy with the access levels provided. This is
* done atomically. The long-running operation from this RPC has a successful status after all
* replacements propagate to long-lasting storage. If the replacement contains errors, an error
* response is returned for the first error encountered. Upon error, the replacement is cancelled,
* and existing access levels are not affected. The Operation.response field contains
* ReplaceAccessLevelsResponse. Removing access levels contained in existing service perimeters
* result in an error.
*
* Create a request for the method "accessLevels.replaceAll".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link ReplaceAll#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name for the access policy which owns these Access Levels. Format:
* `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.ReplaceAccessLevelsRequest}
* @return the request
*/
public ReplaceAll replaceAll(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.ReplaceAccessLevelsRequest content) throws java.io.IOException {
ReplaceAll result = new ReplaceAll(parent, content);
initialize(result);
return result;
}
public class ReplaceAll extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+parent}/accessLevels:replaceAll";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Replaces all existing access levels in an access policy with the access levels provided. This
* is done atomically. The long-running operation from this RPC has a successful status after all
* replacements propagate to long-lasting storage. If the replacement contains errors, an error
* response is returned for the first error encountered. Upon error, the replacement is cancelled,
* and existing access levels are not affected. The Operation.response field contains
* ReplaceAccessLevelsResponse. Removing access levels contained in existing service perimeters
* result in an error.
*
* Create a request for the method "accessLevels.replaceAll".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link ReplaceAll#execute()} method to invoke the remote
* operation. {@link
* ReplaceAll#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name for the access policy which owns these Access Levels. Format:
* `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.ReplaceAccessLevelsRequest}
* @since 1.13
*/
protected ReplaceAll(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.ReplaceAccessLevelsRequest content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public ReplaceAll set$Xgafv(java.lang.String $Xgafv) {
return (ReplaceAll) super.set$Xgafv($Xgafv);
}
@Override
public ReplaceAll setAccessToken(java.lang.String accessToken) {
return (ReplaceAll) super.setAccessToken(accessToken);
}
@Override
public ReplaceAll setAlt(java.lang.String alt) {
return (ReplaceAll) super.setAlt(alt);
}
@Override
public ReplaceAll setCallback(java.lang.String callback) {
return (ReplaceAll) super.setCallback(callback);
}
@Override
public ReplaceAll setFields(java.lang.String fields) {
return (ReplaceAll) super.setFields(fields);
}
@Override
public ReplaceAll setKey(java.lang.String key) {
return (ReplaceAll) super.setKey(key);
}
@Override
public ReplaceAll setOauthToken(java.lang.String oauthToken) {
return (ReplaceAll) super.setOauthToken(oauthToken);
}
@Override
public ReplaceAll setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReplaceAll) super.setPrettyPrint(prettyPrint);
}
@Override
public ReplaceAll setQuotaUser(java.lang.String quotaUser) {
return (ReplaceAll) super.setQuotaUser(quotaUser);
}
@Override
public ReplaceAll setUploadType(java.lang.String uploadType) {
return (ReplaceAll) super.setUploadType(uploadType);
}
@Override
public ReplaceAll setUploadProtocol(java.lang.String uploadProtocol) {
return (ReplaceAll) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the access policy which owns these Access Levels. Format:
* `accessPolicies/{policy_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name for the access policy which owns these Access Levels. Format:
`accessPolicies/{policy_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name for the access policy which owns these Access Levels. Format:
* `accessPolicies/{policy_id}`
*/
public ReplaceAll setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public ReplaceAll set(String parameterName, Object value) {
return (ReplaceAll) super.set(parameterName, value);
}
}
/**
* Returns the IAM permissions that the caller has on the specified Access Context Manager resource.
* The resource can be an AccessPolicy, AccessLevel, or ServicePerimeter. This method does not
* support other resources.
*
* Create a request for the method "accessLevels.testIamPermissions".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+/accessLevels/[^/]+$");
/**
* Returns the IAM permissions that the caller has on the specified Access Context Manager
* resource. The resource can be an AccessPolicy, AccessLevel, or ServicePerimeter. This method
* does not support other resources.
*
* Create a request for the method "accessLevels.testIamPermissions".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the
* remote operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.ser
* vices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^accessPolicies/[^/]+/accessLevels/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^accessPolicies/[^/]+/accessLevels/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the AuthorizedOrgsDescs collection.
*
* The typical use is:
*
* {@code AccessContextManager accesscontextmanager = new AccessContextManager(...);}
* {@code AccessContextManager.AuthorizedOrgsDescs.List request = accesscontextmanager.authorizedOrgsDescs().list(parameters ...)}
*
*
* @return the resource collection
*/
public AuthorizedOrgsDescs authorizedOrgsDescs() {
return new AuthorizedOrgsDescs();
}
/**
* The "authorizedOrgsDescs" collection of methods.
*/
public class AuthorizedOrgsDescs {
/**
* Creates an authorized orgs desc. The long-running operation from this RPC has a successful status
* after the authorized orgs desc propagates to long-lasting storage. If a authorized orgs desc
* contains errors, an error response is returned for the first error encountered. The name of this
* `AuthorizedOrgsDesc` will be assigned during creation.
*
* Create a request for the method "authorizedOrgsDescs.create".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name for the access policy which owns this Authorized Orgs Desc. Format:
* `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AuthorizedOrgsDesc}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.AuthorizedOrgsDesc content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+parent}/authorizedOrgsDescs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Creates an authorized orgs desc. The long-running operation from this RPC has a successful
* status after the authorized orgs desc propagates to long-lasting storage. If a authorized orgs
* desc contains errors, an error response is returned for the first error encountered. The name
* of this `AuthorizedOrgsDesc` will be assigned during creation.
*
* Create a request for the method "authorizedOrgsDescs.create".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name for the access policy which owns this Authorized Orgs Desc. Format:
* `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AuthorizedOrgsDesc}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.AuthorizedOrgsDesc content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the access policy which owns this Authorized Orgs Desc.
* Format: `accessPolicies/{policy_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name for the access policy which owns this Authorized Orgs Desc. Format:
`accessPolicies/{policy_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name for the access policy which owns this Authorized Orgs Desc.
* Format: `accessPolicies/{policy_id}`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an authorized orgs desc based on the resource name. The long-running operation from this
* RPC has a successful status after the authorized orgs desc is removed from long-lasting storage.
*
* Create a request for the method "authorizedOrgsDescs.delete".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name for the Authorized Orgs Desc. Format:
* `accessPolicies/{policy_id}/authorizedOrgsDesc/{authorized_orgs_desc_id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+/authorizedOrgsDescs/[^/]+$");
/**
* Deletes an authorized orgs desc based on the resource name. The long-running operation from
* this RPC has a successful status after the authorized orgs desc is removed from long-lasting
* storage.
*
* Create a request for the method "authorizedOrgsDescs.delete".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name for the Authorized Orgs Desc. Format:
* `accessPolicies/{policy_id}/authorizedOrgsDesc/{authorized_orgs_desc_id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AccessContextManager.this, "DELETE", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/authorizedOrgsDescs/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the Authorized Orgs Desc. Format:
* `accessPolicies/{policy_id}/authorizedOrgsDesc/{authorized_orgs_desc_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name for the Authorized Orgs Desc. Format:
`accessPolicies/{policy_id}/authorizedOrgsDesc/{authorized_orgs_desc_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name for the Authorized Orgs Desc. Format:
* `accessPolicies/{policy_id}/authorizedOrgsDesc/{authorized_orgs_desc_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/authorizedOrgsDescs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an authorized orgs desc based on the resource name.
*
* Create a request for the method "authorizedOrgsDescs.get".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name for the Authorized Orgs Desc. Format:
* `accessPolicies/{policy_id}/authorizedOrgsDescs/{authorized_orgs_descs_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+/authorizedOrgsDescs/[^/]+$");
/**
* Gets an authorized orgs desc based on the resource name.
*
* Create a request for the method "authorizedOrgsDescs.get".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name for the Authorized Orgs Desc. Format:
* `accessPolicies/{policy_id}/authorizedOrgsDescs/{authorized_orgs_descs_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.AuthorizedOrgsDesc.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/authorizedOrgsDescs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the Authorized Orgs Desc. Format:
* `accessPolicies/{policy_id}/authorizedOrgsDescs/{authorized_orgs_descs_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name for the Authorized Orgs Desc. Format:
`accessPolicies/{policy_id}/authorizedOrgsDescs/{authorized_orgs_descs_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name for the Authorized Orgs Desc. Format:
* `accessPolicies/{policy_id}/authorizedOrgsDescs/{authorized_orgs_descs_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/authorizedOrgsDescs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all authorized orgs descs for an access policy.
*
* Create a request for the method "authorizedOrgsDescs.list".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name for the access policy to list Authorized Orgs Desc from. Format:
* `accessPolicies/{policy_id}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+parent}/authorizedOrgsDescs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Lists all authorized orgs descs for an access policy.
*
* Create a request for the method "authorizedOrgsDescs.list".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name for the access policy to list Authorized Orgs Desc from. Format:
* `accessPolicies/{policy_id}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.ListAuthorizedOrgsDescsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the access policy to list Authorized Orgs Desc from. Format:
* `accessPolicies/{policy_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name for the access policy to list Authorized Orgs Desc from. Format:
`accessPolicies/{policy_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name for the access policy to list Authorized Orgs Desc from. Format:
* `accessPolicies/{policy_id}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.parent = parent;
return this;
}
/** Number of Authorized Orgs Descs to include in the list. Default 100. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of Authorized Orgs Descs to include in the list. Default 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Number of Authorized Orgs Descs to include in the list. Default 100. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Next page token for the next batch of Authorized Orgs Desc instances. Defaults to the
* first page of results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Next page token for the next batch of Authorized Orgs Desc instances. Defaults to the first page of
results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Next page token for the next batch of Authorized Orgs Desc instances. Defaults to the
* first page of results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an authorized orgs desc. The long-running operation from this RPC has a successful status
* after the authorized orgs desc propagates to long-lasting storage. If a authorized orgs desc
* contains errors, an error response is returned for the first error encountered. Only the
* organization list in `AuthorizedOrgsDesc` can be updated. The name, authorization_type,
* asset_type and authorization_direction cannot be updated.
*
* Create a request for the method "authorizedOrgsDescs.patch".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Identifier. Resource name for the `AuthorizedOrgsDesc`. Format:
* `accessPolicies/{access_policy}/authorizedOrgsDescs/{authorized_orgs_desc}`. The
* `authorized_orgs_desc` component must begin with a letter, followed by alphanumeric
* characters or `_`. After you create an `AuthorizedOrgsDesc`, you cannot change its `name`.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AuthorizedOrgsDesc}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.AuthorizedOrgsDesc content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+/authorizedOrgsDescs/[^/]+$");
/**
* Updates an authorized orgs desc. The long-running operation from this RPC has a successful
* status after the authorized orgs desc propagates to long-lasting storage. If a authorized orgs
* desc contains errors, an error response is returned for the first error encountered. Only the
* organization list in `AuthorizedOrgsDesc` can be updated. The name, authorization_type,
* asset_type and authorization_direction cannot be updated.
*
* Create a request for the method "authorizedOrgsDescs.patch".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Identifier. Resource name for the `AuthorizedOrgsDesc`. Format:
* `accessPolicies/{access_policy}/authorizedOrgsDescs/{authorized_orgs_desc}`. The
* `authorized_orgs_desc` component must begin with a letter, followed by alphanumeric
* characters or `_`. After you create an `AuthorizedOrgsDesc`, you cannot change its `name`.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.AuthorizedOrgsDesc}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.AuthorizedOrgsDesc content) {
super(AccessContextManager.this, "PATCH", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/authorizedOrgsDescs/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Identifier. Resource name for the `AuthorizedOrgsDesc`. Format:
* `accessPolicies/{access_policy}/authorizedOrgsDescs/{authorized_orgs_desc}`. The
* `authorized_orgs_desc` component must begin with a letter, followed by alphanumeric
* characters or `_`. After you create an `AuthorizedOrgsDesc`, you cannot change its
* `name`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Identifier. Resource name for the `AuthorizedOrgsDesc`. Format:
`accessPolicies/{access_policy}/authorizedOrgsDescs/{authorized_orgs_desc}`. The
`authorized_orgs_desc` component must begin with a letter, followed by alphanumeric characters or
`_`. After you create an `AuthorizedOrgsDesc`, you cannot change its `name`.
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. Resource name for the `AuthorizedOrgsDesc`. Format:
* `accessPolicies/{access_policy}/authorizedOrgsDescs/{authorized_orgs_desc}`. The
* `authorized_orgs_desc` component must begin with a letter, followed by alphanumeric
* characters or `_`. After you create an `AuthorizedOrgsDesc`, you cannot change its
* `name`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/authorizedOrgsDescs/[^/]+$");
}
this.name = name;
return this;
}
/** Required. Mask to control which fields get updated. Must be non-empty. */
@com.google.api.client.util.Key
private String updateMask;
/** Required. Mask to control which fields get updated. Must be non-empty.
*/
public String getUpdateMask() {
return updateMask;
}
/** Required. Mask to control which fields get updated. Must be non-empty. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the ServicePerimeters collection.
*
* The typical use is:
*
* {@code AccessContextManager accesscontextmanager = new AccessContextManager(...);}
* {@code AccessContextManager.ServicePerimeters.List request = accesscontextmanager.servicePerimeters().list(parameters ...)}
*
*
* @return the resource collection
*/
public ServicePerimeters servicePerimeters() {
return new ServicePerimeters();
}
/**
* The "servicePerimeters" collection of methods.
*/
public class ServicePerimeters {
/**
* Commits the dry-run specification for all the service perimeters in an access policy. A commit
* operation on a service perimeter involves copying its `spec` field to the `status` field of the
* service perimeter. Only service perimeters with `use_explicit_dry_run_spec` field set to true are
* affected by a commit operation. The long-running operation from this RPC has a successful status
* after the dry-run specifications for all the service perimeters have been committed. If a commit
* fails, it causes the long-running operation to return an error response and the entire commit
* operation is cancelled. When successful, the Operation.response field contains
* CommitServicePerimetersResponse. The `dry_run` and the `spec` fields are cleared after a
* successful commit operation.
*
* Create a request for the method "servicePerimeters.commit".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Commit#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name for the parent Access Policy which owns all Service Perimeters in scope for
* the commit operation. Format: `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.CommitServicePerimetersRequest}
* @return the request
*/
public Commit commit(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.CommitServicePerimetersRequest content) throws java.io.IOException {
Commit result = new Commit(parent, content);
initialize(result);
return result;
}
public class Commit extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+parent}/servicePerimeters:commit";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Commits the dry-run specification for all the service perimeters in an access policy. A commit
* operation on a service perimeter involves copying its `spec` field to the `status` field of the
* service perimeter. Only service perimeters with `use_explicit_dry_run_spec` field set to true
* are affected by a commit operation. The long-running operation from this RPC has a successful
* status after the dry-run specifications for all the service perimeters have been committed. If
* a commit fails, it causes the long-running operation to return an error response and the entire
* commit operation is cancelled. When successful, the Operation.response field contains
* CommitServicePerimetersResponse. The `dry_run` and the `spec` fields are cleared after a
* successful commit operation.
*
* Create a request for the method "servicePerimeters.commit".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Commit#execute()} method to invoke the remote
* operation. {@link
* Commit#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name for the parent Access Policy which owns all Service Perimeters in scope for
* the commit operation. Format: `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.CommitServicePerimetersRequest}
* @since 1.13
*/
protected Commit(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.CommitServicePerimetersRequest content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public Commit set$Xgafv(java.lang.String $Xgafv) {
return (Commit) super.set$Xgafv($Xgafv);
}
@Override
public Commit setAccessToken(java.lang.String accessToken) {
return (Commit) super.setAccessToken(accessToken);
}
@Override
public Commit setAlt(java.lang.String alt) {
return (Commit) super.setAlt(alt);
}
@Override
public Commit setCallback(java.lang.String callback) {
return (Commit) super.setCallback(callback);
}
@Override
public Commit setFields(java.lang.String fields) {
return (Commit) super.setFields(fields);
}
@Override
public Commit setKey(java.lang.String key) {
return (Commit) super.setKey(key);
}
@Override
public Commit setOauthToken(java.lang.String oauthToken) {
return (Commit) super.setOauthToken(oauthToken);
}
@Override
public Commit setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Commit) super.setPrettyPrint(prettyPrint);
}
@Override
public Commit setQuotaUser(java.lang.String quotaUser) {
return (Commit) super.setQuotaUser(quotaUser);
}
@Override
public Commit setUploadType(java.lang.String uploadType) {
return (Commit) super.setUploadType(uploadType);
}
@Override
public Commit setUploadProtocol(java.lang.String uploadProtocol) {
return (Commit) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the parent Access Policy which owns all Service Perimeters in
* scope for the commit operation. Format: `accessPolicies/{policy_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name for the parent Access Policy which owns all Service Perimeters in scope for
the commit operation. Format: `accessPolicies/{policy_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name for the parent Access Policy which owns all Service Perimeters in
* scope for the commit operation. Format: `accessPolicies/{policy_id}`
*/
public Commit setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Commit set(String parameterName, Object value) {
return (Commit) super.set(parameterName, value);
}
}
/**
* Creates a service perimeter. The long-running operation from this RPC has a successful status
* after the service perimeter propagates to long-lasting storage. If a service perimeter contains
* errors, an error response is returned for the first error encountered.
*
* Create a request for the method "servicePerimeters.create".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name for the access policy which owns this Service Perimeter. Format:
* `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.ServicePerimeter}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.ServicePerimeter content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+parent}/servicePerimeters";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Creates a service perimeter. The long-running operation from this RPC has a successful status
* after the service perimeter propagates to long-lasting storage. If a service perimeter contains
* errors, an error response is returned for the first error encountered.
*
* Create a request for the method "servicePerimeters.create".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name for the access policy which owns this Service Perimeter. Format:
* `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.ServicePerimeter}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.ServicePerimeter content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the access policy which owns this Service Perimeter. Format:
* `accessPolicies/{policy_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name for the access policy which owns this Service Perimeter. Format:
`accessPolicies/{policy_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name for the access policy which owns this Service Perimeter. Format:
* `accessPolicies/{policy_id}`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a service perimeter based on the resource name. The long-running operation from this RPC
* has a successful status after the service perimeter is removed from long-lasting storage.
*
* Create a request for the method "servicePerimeters.delete".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name for the Service Perimeter. Format:
* `accessPolicies/{policy_id}/servicePerimeters/{service_perimeter_id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
/**
* Deletes a service perimeter based on the resource name. The long-running operation from this
* RPC has a successful status after the service perimeter is removed from long-lasting storage.
*
* Create a request for the method "servicePerimeters.delete".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name for the Service Perimeter. Format:
* `accessPolicies/{policy_id}/servicePerimeters/{service_perimeter_id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AccessContextManager.this, "DELETE", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the Service Perimeter. Format:
* `accessPolicies/{policy_id}/servicePerimeters/{service_perimeter_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name for the Service Perimeter. Format:
`accessPolicies/{policy_id}/servicePerimeters/{service_perimeter_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name for the Service Perimeter. Format:
* `accessPolicies/{policy_id}/servicePerimeters/{service_perimeter_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a service perimeter based on the resource name.
*
* Create a request for the method "servicePerimeters.get".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name for the Service Perimeter. Format:
* `accessPolicies/{policy_id}/servicePerimeters/{service_perimeters_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
/**
* Gets a service perimeter based on the resource name.
*
* Create a request for the method "servicePerimeters.get".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name for the Service Perimeter. Format:
* `accessPolicies/{policy_id}/servicePerimeters/{service_perimeters_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.ServicePerimeter.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the Service Perimeter. Format:
* `accessPolicies/{policy_id}/servicePerimeters/{service_perimeters_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name for the Service Perimeter. Format:
`accessPolicies/{policy_id}/servicePerimeters/{service_perimeters_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name for the Service Perimeter. Format:
* `accessPolicies/{policy_id}/servicePerimeters/{service_perimeters_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all service perimeters for an access policy.
*
* Create a request for the method "servicePerimeters.list".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name for the access policy to list Service Perimeters from. Format:
* `accessPolicies/{policy_id}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+parent}/servicePerimeters";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Lists all service perimeters for an access policy.
*
* Create a request for the method "servicePerimeters.list".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name for the access policy to list Service Perimeters from. Format:
* `accessPolicies/{policy_id}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.ListServicePerimetersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the access policy to list Service Perimeters from. Format:
* `accessPolicies/{policy_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name for the access policy to list Service Perimeters from. Format:
`accessPolicies/{policy_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name for the access policy to list Service Perimeters from. Format:
* `accessPolicies/{policy_id}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.parent = parent;
return this;
}
/** Number of Service Perimeters to include in the list. Default 100. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Number of Service Perimeters to include in the list. Default 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Number of Service Perimeters to include in the list. Default 100. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Next page token for the next batch of Service Perimeter instances. Defaults to the first
* page of results.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Next page token for the next batch of Service Perimeter instances. Defaults to the first page of
results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Next page token for the next batch of Service Perimeter instances. Defaults to the first
* page of results.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a service perimeter. The long-running operation from this RPC has a successful status
* after the service perimeter propagates to long-lasting storage. If a service perimeter contains
* errors, an error response is returned for the first error encountered.
*
* Create a request for the method "servicePerimeters.patch".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Identifier. Resource name for the `ServicePerimeter`. Format:
* `accessPolicies/{access_policy}/servicePerimeters/{service_perimeter}`. The
* `service_perimeter` component must begin with a letter, followed by alphanumeric
* characters or `_`. After you create a `ServicePerimeter`, you cannot change its `name`.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.ServicePerimeter}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.ServicePerimeter content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
/**
* Updates a service perimeter. The long-running operation from this RPC has a successful status
* after the service perimeter propagates to long-lasting storage. If a service perimeter contains
* errors, an error response is returned for the first error encountered.
*
* Create a request for the method "servicePerimeters.patch".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Identifier. Resource name for the `ServicePerimeter`. Format:
* `accessPolicies/{access_policy}/servicePerimeters/{service_perimeter}`. The
* `service_perimeter` component must begin with a letter, followed by alphanumeric
* characters or `_`. After you create a `ServicePerimeter`, you cannot change its `name`.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.ServicePerimeter}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.ServicePerimeter content) {
super(AccessContextManager.this, "PATCH", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Identifier. Resource name for the `ServicePerimeter`. Format:
* `accessPolicies/{access_policy}/servicePerimeters/{service_perimeter}`. The
* `service_perimeter` component must begin with a letter, followed by alphanumeric
* characters or `_`. After you create a `ServicePerimeter`, you cannot change its `name`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Identifier. Resource name for the `ServicePerimeter`. Format:
`accessPolicies/{access_policy}/servicePerimeters/{service_perimeter}`. The `service_perimeter`
component must begin with a letter, followed by alphanumeric characters or `_`. After you create a
`ServicePerimeter`, you cannot change its `name`.
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. Resource name for the `ServicePerimeter`. Format:
* `accessPolicies/{access_policy}/servicePerimeters/{service_perimeter}`. The
* `service_perimeter` component must begin with a letter, followed by alphanumeric
* characters or `_`. After you create a `ServicePerimeter`, you cannot change its `name`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
}
this.name = name;
return this;
}
/** Required. Mask to control which fields get updated. Must be non-empty. */
@com.google.api.client.util.Key
private String updateMask;
/** Required. Mask to control which fields get updated. Must be non-empty.
*/
public String getUpdateMask() {
return updateMask;
}
/** Required. Mask to control which fields get updated. Must be non-empty. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Replace all existing service perimeters in an access policy with the service perimeters provided.
* This is done atomically. The long-running operation from this RPC has a successful status after
* all replacements propagate to long-lasting storage. Replacements containing errors result in an
* error response for the first error encountered. Upon an error, replacement are cancelled and
* existing service perimeters are not affected. The Operation.response field contains
* ReplaceServicePerimetersResponse.
*
* Create a request for the method "servicePerimeters.replaceAll".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link ReplaceAll#execute()} method to invoke the remote operation.
*
* @param parent Required. Resource name for the access policy which owns these Service Perimeters. Format:
* `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.ReplaceServicePerimetersRequest}
* @return the request
*/
public ReplaceAll replaceAll(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.ReplaceServicePerimetersRequest content) throws java.io.IOException {
ReplaceAll result = new ReplaceAll(parent, content);
initialize(result);
return result;
}
public class ReplaceAll extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+parent}/servicePerimeters:replaceAll";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+$");
/**
* Replace all existing service perimeters in an access policy with the service perimeters
* provided. This is done atomically. The long-running operation from this RPC has a successful
* status after all replacements propagate to long-lasting storage. Replacements containing errors
* result in an error response for the first error encountered. Upon an error, replacement are
* cancelled and existing service perimeters are not affected. The Operation.response field
* contains ReplaceServicePerimetersResponse.
*
* Create a request for the method "servicePerimeters.replaceAll".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link ReplaceAll#execute()} method to invoke the remote
* operation. {@link
* ReplaceAll#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Resource name for the access policy which owns these Service Perimeters. Format:
* `accessPolicies/{policy_id}`
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.ReplaceServicePerimetersRequest}
* @since 1.13
*/
protected ReplaceAll(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.ReplaceServicePerimetersRequest content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
}
@Override
public ReplaceAll set$Xgafv(java.lang.String $Xgafv) {
return (ReplaceAll) super.set$Xgafv($Xgafv);
}
@Override
public ReplaceAll setAccessToken(java.lang.String accessToken) {
return (ReplaceAll) super.setAccessToken(accessToken);
}
@Override
public ReplaceAll setAlt(java.lang.String alt) {
return (ReplaceAll) super.setAlt(alt);
}
@Override
public ReplaceAll setCallback(java.lang.String callback) {
return (ReplaceAll) super.setCallback(callback);
}
@Override
public ReplaceAll setFields(java.lang.String fields) {
return (ReplaceAll) super.setFields(fields);
}
@Override
public ReplaceAll setKey(java.lang.String key) {
return (ReplaceAll) super.setKey(key);
}
@Override
public ReplaceAll setOauthToken(java.lang.String oauthToken) {
return (ReplaceAll) super.setOauthToken(oauthToken);
}
@Override
public ReplaceAll setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReplaceAll) super.setPrettyPrint(prettyPrint);
}
@Override
public ReplaceAll setQuotaUser(java.lang.String quotaUser) {
return (ReplaceAll) super.setQuotaUser(quotaUser);
}
@Override
public ReplaceAll setUploadType(java.lang.String uploadType) {
return (ReplaceAll) super.setUploadType(uploadType);
}
@Override
public ReplaceAll setUploadProtocol(java.lang.String uploadProtocol) {
return (ReplaceAll) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name for the access policy which owns these Service Perimeters.
* Format: `accessPolicies/{policy_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Resource name for the access policy which owns these Service Perimeters. Format:
`accessPolicies/{policy_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Resource name for the access policy which owns these Service Perimeters.
* Format: `accessPolicies/{policy_id}`
*/
public ReplaceAll setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accessPolicies/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public ReplaceAll set(String parameterName, Object value) {
return (ReplaceAll) super.set(parameterName, value);
}
}
/**
* Returns the IAM permissions that the caller has on the specified Access Context Manager resource.
* The resource can be an AccessPolicy, AccessLevel, or ServicePerimeter. This method does not
* support other resources.
*
* Create a request for the method "servicePerimeters.testIamPermissions".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
/**
* Returns the IAM permissions that the caller has on the specified Access Context Manager
* resource. The resource can be an AccessPolicy, AccessLevel, or ServicePerimeter. This method
* does not support other resources.
*
* Create a request for the method "servicePerimeters.testIamPermissions".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the
* remote operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.ser
* vices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsRequest content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^accessPolicies/[^/]+/servicePerimeters/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code AccessContextManager accesscontextmanager = new AccessContextManager(...);}
* {@code AccessContextManager.Operations.List request = accesscontextmanager.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of `1`, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.CancelOperationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.CancelOperationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations/.*$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of `1`, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Cancel#execute()} method to invoke the remote
* operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.CancelOperationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.CancelOperationRequest content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations/.*$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AccessContextManager.this, "DELETE", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations/.*$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Organizations collection.
*
* The typical use is:
*
* {@code AccessContextManager accesscontextmanager = new AccessContextManager(...);}
* {@code AccessContextManager.Organizations.List request = accesscontextmanager.organizations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Organizations organizations() {
return new Organizations();
}
/**
* The "organizations" collection of methods.
*/
public class Organizations {
/**
* An accessor for creating requests from the GcpUserAccessBindings collection.
*
* The typical use is:
*
* {@code AccessContextManager accesscontextmanager = new AccessContextManager(...);}
* {@code AccessContextManager.GcpUserAccessBindings.List request = accesscontextmanager.gcpUserAccessBindings().list(parameters ...)}
*
*
* @return the resource collection
*/
public GcpUserAccessBindings gcpUserAccessBindings() {
return new GcpUserAccessBindings();
}
/**
* The "gcpUserAccessBindings" collection of methods.
*/
public class GcpUserAccessBindings {
/**
* Creates a GcpUserAccessBinding. If the client specifies a name, the server ignores it. Fails if a
* resource already exists with the same group_key. Completion of this long-running operation does
* not necessarily signify that the new binding is deployed onto all affected users, which may take
* more time.
*
* Create a request for the method "gcpUserAccessBindings.create".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Example: "organizations/256"
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.GcpUserAccessBinding}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.GcpUserAccessBinding content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+parent}/gcpUserAccessBindings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Creates a GcpUserAccessBinding. If the client specifies a name, the server ignores it. Fails if
* a resource already exists with the same group_key. Completion of this long-running operation
* does not necessarily signify that the new binding is deployed onto all affected users, which
* may take more time.
*
* Create a request for the method "gcpUserAccessBindings.create".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Example: "organizations/256"
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.GcpUserAccessBinding}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.accesscontextmanager.v1.model.GcpUserAccessBinding content) {
super(AccessContextManager.this, "POST", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. Example: "organizations/256" */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Example: "organizations/256"
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Example: "organizations/256" */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a GcpUserAccessBinding. Completion of this long-running operation does not necessarily
* signify that the binding deletion is deployed onto all affected users, which may take more time.
*
* Create a request for the method "gcpUserAccessBindings.delete".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N"
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/gcpUserAccessBindings/[^/]+$");
/**
* Deletes a GcpUserAccessBinding. Completion of this long-running operation does not necessarily
* signify that the binding deletion is deployed onto all affected users, which may take more
* time.
*
* Create a request for the method "gcpUserAccessBindings.delete".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N"
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AccessContextManager.this, "DELETE", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/gcpUserAccessBindings/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N" */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N"
*/
public java.lang.String getName() {
return name;
}
/** Required. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N" */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/gcpUserAccessBindings/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the GcpUserAccessBinding with the given name.
*
* Create a request for the method "gcpUserAccessBindings.get".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N"
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/gcpUserAccessBindings/[^/]+$");
/**
* Gets the GcpUserAccessBinding with the given name.
*
* Create a request for the method "gcpUserAccessBindings.get".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N"
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.GcpUserAccessBinding.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/gcpUserAccessBindings/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N" */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N"
*/
public java.lang.String getName() {
return name;
}
/** Required. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N" */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/gcpUserAccessBindings/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all GcpUserAccessBindings for a Google Cloud organization.
*
* Create a request for the method "gcpUserAccessBindings.list".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Example: "organizations/256"
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+parent}/gcpUserAccessBindings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+$");
/**
* Lists all GcpUserAccessBindings for a Google Cloud organization.
*
* Create a request for the method "gcpUserAccessBindings.list".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Example: "organizations/256"
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.ListGcpUserAccessBindingsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. Example: "organizations/256" */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Example: "organizations/256"
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Example: "organizations/256" */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^organizations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. Maximum number of items to return. The server may return fewer items. If left
* blank, the server may return any number of items.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Maximum number of items to return. The server may return fewer items. If left blank, the
server may return any number of items.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Maximum number of items to return. The server may return fewer items. If left
* blank, the server may return any number of items.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. If left blank, returns the first page. To enumerate all items, use the
* next_page_token from your previous list operation.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. If left blank, returns the first page. To enumerate all items, use the next_page_token
from your previous list operation.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. If left blank, returns the first page. To enumerate all items, use the
* next_page_token from your previous list operation.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a GcpUserAccessBinding. Completion of this long-running operation does not necessarily
* signify that the changed binding is deployed onto all affected users, which may take more time.
*
* Create a request for the method "gcpUserAccessBindings.patch".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Immutable. Assigned by the server during creation. The last segment has an arbitrary length and has
* only URI unreserved characters (as defined by [RFC 3986 Section
* 2.3](https://tools.ietf.org/html/rfc3986#section-2.3)). Should not be specified by the
* client during creation. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N"
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.GcpUserAccessBinding}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.GcpUserAccessBinding content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^organizations/[^/]+/gcpUserAccessBindings/[^/]+$");
/**
* Updates a GcpUserAccessBinding. Completion of this long-running operation does not necessarily
* signify that the changed binding is deployed onto all affected users, which may take more time.
*
* Create a request for the method "gcpUserAccessBindings.patch".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Immutable. Assigned by the server during creation. The last segment has an arbitrary length and has
* only URI unreserved characters (as defined by [RFC 3986 Section
* 2.3](https://tools.ietf.org/html/rfc3986#section-2.3)). Should not be specified by the
* client during creation. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N"
* @param content the {@link com.google.api.services.accesscontextmanager.v1.model.GcpUserAccessBinding}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.accesscontextmanager.v1.model.GcpUserAccessBinding content) {
super(AccessContextManager.this, "PATCH", REST_PATH, content, com.google.api.services.accesscontextmanager.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/gcpUserAccessBindings/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Immutable. Assigned by the server during creation. The last segment has an arbitrary
* length and has only URI unreserved characters (as defined by [RFC 3986 Section
* 2.3](https://tools.ietf.org/html/rfc3986#section-2.3)). Should not be specified by the
* client during creation. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N"
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Immutable. Assigned by the server during creation. The last segment has an arbitrary length and has
only URI unreserved characters (as defined by [RFC 3986 Section
2.3](https://tools.ietf.org/html/rfc3986#section-2.3)). Should not be specified by the client
during creation. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N"
*/
public java.lang.String getName() {
return name;
}
/**
* Immutable. Assigned by the server during creation. The last segment has an arbitrary
* length and has only URI unreserved characters (as defined by [RFC 3986 Section
* 2.3](https://tools.ietf.org/html/rfc3986#section-2.3)). Should not be specified by the
* client during creation. Example: "organizations/256/gcpUserAccessBindings/b3-BhcX_Ud5N"
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^organizations/[^/]+/gcpUserAccessBindings/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. This field controls whether or not certain repeated settings in the update
* request overwrite or append to existing settings on the binding. If true, then append.
* Otherwise overwrite. So far, only scoped_access_settings with reauth_settings supports
* appending. Global access_levels, access_levels in scoped_access_settings,
* dry_run_access_levels, reauth_settings, and session_settings are not compatible with
* append functionality, and the request will return an error if append=true when these
* settings are in the update_mask. The request will also return an error if append=true
* when "scoped_access_settings" is not set in the update_mask.
*/
@com.google.api.client.util.Key
private java.lang.Boolean append;
/** Optional. This field controls whether or not certain repeated settings in the update request
overwrite or append to existing settings on the binding. If true, then append. Otherwise overwrite.
So far, only scoped_access_settings with reauth_settings supports appending. Global access_levels,
access_levels in scoped_access_settings, dry_run_access_levels, reauth_settings, and
session_settings are not compatible with append functionality, and the request will return an error
if append=true when these settings are in the update_mask. The request will also return an error if
append=true when "scoped_access_settings" is not set in the update_mask.
*/
public java.lang.Boolean getAppend() {
return append;
}
/**
* Optional. This field controls whether or not certain repeated settings in the update
* request overwrite or append to existing settings on the binding. If true, then append.
* Otherwise overwrite. So far, only scoped_access_settings with reauth_settings supports
* appending. Global access_levels, access_levels in scoped_access_settings,
* dry_run_access_levels, reauth_settings, and session_settings are not compatible with
* append functionality, and the request will return an error if append=true when these
* settings are in the update_mask. The request will also return an error if append=true
* when "scoped_access_settings" is not set in the update_mask.
*/
public Patch setAppend(java.lang.Boolean append) {
this.append = append;
return this;
}
/**
* Required. Only the fields specified in this mask are updated. Because name and group_key
* cannot be changed, update_mask is required and may only contain the following fields:
* `access_levels`, `dry_run_access_levels`, `reauth_settings` `session_settings`,
* `scoped_access_settings`. update_mask { paths: "access_levels" }
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Only the fields specified in this mask are updated. Because name and group_key cannot be
changed, update_mask is required and may only contain the following fields: `access_levels`,
`dry_run_access_levels`, `reauth_settings` `session_settings`, `scoped_access_settings`.
update_mask { paths: "access_levels" }
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Only the fields specified in this mask are updated. Because name and group_key
* cannot be changed, update_mask is required and may only contain the following fields:
* `access_levels`, `dry_run_access_levels`, `reauth_settings` `session_settings`,
* `scoped_access_settings`. update_mask { paths: "access_levels" }
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Services collection.
*
* The typical use is:
*
* {@code AccessContextManager accesscontextmanager = new AccessContextManager(...);}
* {@code AccessContextManager.Services.List request = accesscontextmanager.services().list(parameters ...)}
*
*
* @return the resource collection
*/
public Services services() {
return new Services();
}
/**
* The "services" collection of methods.
*/
public class Services {
/**
* Returns a VPC-SC supported service based on the service name.
*
* Create a request for the method "services.get".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the service to get information about. The names must be in the same format as used in
* defining a service perimeter, for example, `storage.googleapis.com`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/services/{name}";
/**
* Returns a VPC-SC supported service based on the service name.
*
* Create a request for the method "services.get".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the service to get information about. The names must be in the same format as used in
* defining a service perimeter, for example, `storage.googleapis.com`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.SupportedService.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the service to get information about. The names must be in the same format as
* used in defining a service perimeter, for example, `storage.googleapis.com`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the service to get information about. The names must be in the same format as used in
defining a service perimeter, for example, `storage.googleapis.com`.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the service to get information about. The names must be in the same format as
* used in defining a service perimeter, for example, `storage.googleapis.com`.
*/
public Get setName(java.lang.String name) {
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all VPC-SC supported services.
*
* Create a request for the method "services.list".
*
* This request holds the parameters needed by the accesscontextmanager server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends AccessContextManagerRequest {
private static final String REST_PATH = "v1/services";
/**
* Lists all VPC-SC supported services.
*
* Create a request for the method "services.list".
*
* This request holds the parameters needed by the the accesscontextmanager server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(AccessContextManager.this, "GET", REST_PATH, null, com.google.api.services.accesscontextmanager.v1.model.ListSupportedServicesResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** This flag specifies the maximum number of services to return per page. Default is 100. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** This flag specifies the maximum number of services to return per page. Default is 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** This flag specifies the maximum number of services to return per page. Default is 100. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Token to start on a later page. Default is the first page. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Token to start on a later page. Default is the first page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Token to start on a later page. Default is the first page. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link AccessContextManager}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link AccessContextManager}. */
@Override
public AccessContextManager build() {
return new AccessContextManager(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link AccessContextManagerRequestInitializer}.
*
* @since 1.12
*/
public Builder setAccessContextManagerRequestInitializer(
AccessContextManagerRequestInitializer accesscontextmanagerRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(accesscontextmanagerRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}