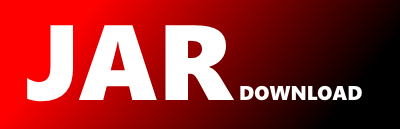
com.google.api.services.adexchangebuyer2.v2beta1.AdExchangeBuyerII Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.adexchangebuyer2.v2beta1;
/**
* Service definition for AdExchangeBuyerII (v2beta1).
*
*
* Accesses the latest features for managing Authorized Buyers accounts, Real-Time Bidding configurations and auction metrics, and Marketplace programmatic deals.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link AdExchangeBuyerIIRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class AdExchangeBuyerII extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Ad Exchange Buyer API II library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://adexchangebuyer.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://adexchangebuyer.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public AdExchangeBuyerII(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
AdExchangeBuyerII(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Accounts collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Accounts.List request = adexchangebuyer2.accounts().list(parameters ...)}
*
*
* @return the resource collection
*/
public Accounts accounts() {
return new Accounts();
}
/**
* The "accounts" collection of methods.
*/
public class Accounts {
/**
* An accessor for creating requests from the Clients collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Clients.List request = adexchangebuyer2.clients().list(parameters ...)}
*
*
* @return the resource collection
*/
public Clients clients() {
return new Clients();
}
/**
* The "clients" collection of methods.
*/
public class Clients {
/**
* Creates a new client buyer.
*
* Create a request for the method "clients.create".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param accountId Unique numerical account ID for the buyer of which the client buyer is a customer; the sponsor buyer
* to create a client for. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Client}
* @return the request
*/
public Create create(java.lang.Long accountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Client content) throws java.io.IOException {
Create result = new Create(accountId, content);
initialize(result);
return result;
}
public class Create extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients";
/**
* Creates a new client buyer.
*
* Create a request for the method "clients.create".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Unique numerical account ID for the buyer of which the client buyer is a customer; the sponsor buyer
* to create a client for. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Client}
* @since 1.13
*/
protected Create(java.lang.Long accountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Client content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Client.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique numerical account ID for the buyer of which the client buyer is a customer; the
* sponsor buyer to create a client for. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Unique numerical account ID for the buyer of which the client buyer is a customer; the sponsor
buyer to create a client for. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/**
* Unique numerical account ID for the buyer of which the client buyer is a customer; the
* sponsor buyer to create a client for. (required)
*/
public Create setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets a client buyer with a given client account ID.
*
* Create a request for the method "clients.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer to retrieve. (required)
* @return the request
*/
public Get get(java.lang.Long accountId, java.lang.Long clientAccountId) throws java.io.IOException {
Get result = new Get(accountId, clientAccountId);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}";
/**
* Gets a client buyer with a given client account ID.
*
* Create a request for the method "clients.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer to retrieve. (required)
* @since 1.13
*/
protected Get(java.lang.Long accountId, java.lang.Long clientAccountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.Client.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public Get setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/** Numerical account ID of the client buyer to retrieve. (required) */
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Numerical account ID of the client buyer to retrieve. (required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/** Numerical account ID of the client buyer to retrieve. (required) */
public Get setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the clients for the current sponsor buyer.
*
* Create a request for the method "clients.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId Unique numerical account ID of the sponsor buyer to list the clients for.
* @return the request
*/
public List list(java.lang.Long accountId) throws java.io.IOException {
List result = new List(accountId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients";
/**
* Lists all the clients for the current sponsor buyer.
*
* Create a request for the method "clients.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Unique numerical account ID of the sponsor buyer to list the clients for.
* @since 1.13
*/
protected List(java.lang.Long accountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListClientsResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Unique numerical account ID of the sponsor buyer to list the clients for. */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Unique numerical account ID of the sponsor buyer to list the clients for.
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Unique numerical account ID of the sponsor buyer to list the clients for. */
public List setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Requested page size. The server may return fewer clients than requested. If unspecified,
* the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer clients than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer clients than requested. If unspecified,
* the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientsResponse.nextPageToken returned from the previous call to the
* accounts.clients.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListClientsResponse.nextPageToken returned from the previous call to the accounts.clients.list
method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientsResponse.nextPageToken returned from the previous call to the
* accounts.clients.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Optional unique identifier (from the standpoint of an Ad Exchange sponsor buyer partner)
* of the client to return. If specified, at most one client will be returned in the
* response.
*/
@com.google.api.client.util.Key
private java.lang.String partnerClientId;
/** Optional unique identifier (from the standpoint of an Ad Exchange sponsor buyer partner) of the
client to return. If specified, at most one client will be returned in the response.
*/
public java.lang.String getPartnerClientId() {
return partnerClientId;
}
/**
* Optional unique identifier (from the standpoint of an Ad Exchange sponsor buyer partner)
* of the client to return. If specified, at most one client will be returned in the
* response.
*/
public List setPartnerClientId(java.lang.String partnerClientId) {
this.partnerClientId = partnerClientId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing client buyer.
*
* Create a request for the method "clients.update".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param accountId Unique numerical account ID for the buyer of which the client buyer is a customer; the sponsor buyer
* to update a client for. (required)
* @param clientAccountId Unique numerical account ID of the client to update. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Client}
* @return the request
*/
public Update update(java.lang.Long accountId, java.lang.Long clientAccountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Client content) throws java.io.IOException {
Update result = new Update(accountId, clientAccountId, content);
initialize(result);
return result;
}
public class Update extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}";
/**
* Updates an existing client buyer.
*
* Create a request for the method "clients.update".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Unique numerical account ID for the buyer of which the client buyer is a customer; the sponsor buyer
* to update a client for. (required)
* @param clientAccountId Unique numerical account ID of the client to update. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Client}
* @since 1.13
*/
protected Update(java.lang.Long accountId, java.lang.Long clientAccountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Client content) {
super(AdExchangeBuyerII.this, "PUT", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Client.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique numerical account ID for the buyer of which the client buyer is a customer; the
* sponsor buyer to update a client for. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Unique numerical account ID for the buyer of which the client buyer is a customer; the sponsor
buyer to update a client for. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/**
* Unique numerical account ID for the buyer of which the client buyer is a customer; the
* sponsor buyer to update a client for. (required)
*/
public Update setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/** Unique numerical account ID of the client to update. (required) */
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Unique numerical account ID of the client to update. (required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/** Unique numerical account ID of the client to update. (required) */
public Update setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Invitations collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Invitations.List request = adexchangebuyer2.invitations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Invitations invitations() {
return new Invitations();
}
/**
* The "invitations" collection of methods.
*/
public class Invitations {
/**
* Creates and sends out an email invitation to access an Ad Exchange client buyer account.
*
* Create a request for the method "invitations.create".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user should be associated with. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation}
* @return the request
*/
public Create create(java.lang.Long accountId, java.lang.Long clientAccountId, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation content) throws java.io.IOException {
Create result = new Create(accountId, clientAccountId, content);
initialize(result);
return result;
}
public class Create extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/invitations";
/**
* Creates and sends out an email invitation to access an Ad Exchange client buyer account.
*
* Create a request for the method "invitations.create".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user should be associated with. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation}
* @since 1.13
*/
protected Create(java.lang.Long accountId, java.lang.Long clientAccountId, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public Create setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Numerical account ID of the client buyer that the user should be associated with.
* (required)
*/
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Numerical account ID of the client buyer that the user should be associated with. (required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/**
* Numerical account ID of the client buyer that the user should be associated with.
* (required)
*/
public Create setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Retrieves an existing client user invitation.
*
* Create a request for the method "invitations.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user invitation to be retrieved is associated
* with. (required)
* @param invitationId Numerical identifier of the user invitation to retrieve. (required)
* @return the request
*/
public Get get(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long invitationId) throws java.io.IOException {
Get result = new Get(accountId, clientAccountId, invitationId);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/invitations/{invitationId}";
/**
* Retrieves an existing client user invitation.
*
* Create a request for the method "invitations.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user invitation to be retrieved is associated
* with. (required)
* @param invitationId Numerical identifier of the user invitation to retrieve. (required)
* @since 1.13
*/
protected Get(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long invitationId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
this.invitationId = com.google.api.client.util.Preconditions.checkNotNull(invitationId, "Required parameter invitationId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public Get setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Numerical account ID of the client buyer that the user invitation to be retrieved is
* associated with. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Numerical account ID of the client buyer that the user invitation to be retrieved is associated
with. (required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/**
* Numerical account ID of the client buyer that the user invitation to be retrieved is
* associated with. (required)
*/
public Get setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
/** Numerical identifier of the user invitation to retrieve. (required) */
@com.google.api.client.util.Key
private java.lang.Long invitationId;
/** Numerical identifier of the user invitation to retrieve. (required)
*/
public java.lang.Long getInvitationId() {
return invitationId;
}
/** Numerical identifier of the user invitation to retrieve. (required) */
public Get setInvitationId(java.lang.Long invitationId) {
this.invitationId = invitationId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the client users invitations for a client with a given account ID.
*
* Create a request for the method "invitations.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer to list invitations for. (required) You must either specify
* a string representation of a numerical account identifier or the `-` character to list all
* the invitations for all the clients of a given sponsor buyer.
* @return the request
*/
public List list(java.lang.Long accountId, java.lang.String clientAccountId) throws java.io.IOException {
List result = new List(accountId, clientAccountId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/invitations";
/**
* Lists all the client users invitations for a client with a given account ID.
*
* Create a request for the method "invitations.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer to list invitations for. (required) You must either specify
* a string representation of a numerical account identifier or the `-` character to list all
* the invitations for all the clients of a given sponsor buyer.
* @since 1.13
*/
protected List(java.lang.Long accountId, java.lang.String clientAccountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListClientUserInvitationsResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public List setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Numerical account ID of the client buyer to list invitations for. (required) You must
* either specify a string representation of a numerical account identifier or the `-`
* character to list all the invitations for all the clients of a given sponsor buyer.
*/
@com.google.api.client.util.Key
private java.lang.String clientAccountId;
/** Numerical account ID of the client buyer to list invitations for. (required) You must either
specify a string representation of a numerical account identifier or the `-` character to list all
the invitations for all the clients of a given sponsor buyer.
*/
public java.lang.String getClientAccountId() {
return clientAccountId;
}
/**
* Numerical account ID of the client buyer to list invitations for. (required) You must
* either specify a string representation of a numerical account identifier or the `-`
* character to list all the invitations for all the clients of a given sponsor buyer.
*/
public List setClientAccountId(java.lang.String clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
/**
* Requested page size. Server may return fewer clients than requested. If unspecified,
* server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. Server may return fewer clients than requested. If unspecified, server will
pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. Server may return fewer clients than requested. If unspecified,
* server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientUserInvitationsResponse.nextPageToken returned from the previous
* call to the clients.invitations.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListClientUserInvitationsResponse.nextPageToken returned from the previous call to the
clients.invitations.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientUserInvitationsResponse.nextPageToken returned from the previous
* call to the clients.invitations.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Users collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Users.List request = adexchangebuyer2.users().list(parameters ...)}
*
*
* @return the resource collection
*/
public Users users() {
return new Users();
}
/**
* The "users" collection of methods.
*/
public class Users {
/**
* Retrieves an existing client user.
*
* Create a request for the method "users.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user to be retrieved is associated with.
* (required)
* @param userId Numerical identifier of the user to retrieve. (required)
* @return the request
*/
public Get get(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long userId) throws java.io.IOException {
Get result = new Get(accountId, clientAccountId, userId);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/users/{userId}";
/**
* Retrieves an existing client user.
*
* Create a request for the method "users.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user to be retrieved is associated with.
* (required)
* @param userId Numerical identifier of the user to retrieve. (required)
* @since 1.13
*/
protected Get(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long userId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public Get setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Numerical account ID of the client buyer that the user to be retrieved is associated
* with. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Numerical account ID of the client buyer that the user to be retrieved is associated with.
(required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/**
* Numerical account ID of the client buyer that the user to be retrieved is associated
* with. (required)
*/
public Get setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
/** Numerical identifier of the user to retrieve. (required) */
@com.google.api.client.util.Key
private java.lang.Long userId;
/** Numerical identifier of the user to retrieve. (required)
*/
public java.lang.Long getUserId() {
return userId;
}
/** Numerical identifier of the user to retrieve. (required) */
public Get setUserId(java.lang.Long userId) {
this.userId = userId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the known client users for a specified sponsor buyer account ID.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the sponsor buyer of the client to list users for. (required)
* @param clientAccountId The account ID of the client buyer to list users for. (required) You must specify either a string
* representation of a numerical account identifier or the `-` character to list all the
* client users for all the clients of a given sponsor buyer.
* @return the request
*/
public List list(java.lang.Long accountId, java.lang.String clientAccountId) throws java.io.IOException {
List result = new List(accountId, clientAccountId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/users";
/**
* Lists all the known client users for a specified sponsor buyer account ID.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the sponsor buyer of the client to list users for. (required)
* @param clientAccountId The account ID of the client buyer to list users for. (required) You must specify either a string
* representation of a numerical account identifier or the `-` character to list all the
* client users for all the clients of a given sponsor buyer.
* @since 1.13
*/
protected List(java.lang.Long accountId, java.lang.String clientAccountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListClientUsersResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Numerical account ID of the sponsor buyer of the client to list users for. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the sponsor buyer of the client to list users for. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/**
* Numerical account ID of the sponsor buyer of the client to list users for. (required)
*/
public List setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* The account ID of the client buyer to list users for. (required) You must specify
* either a string representation of a numerical account identifier or the `-` character
* to list all the client users for all the clients of a given sponsor buyer.
*/
@com.google.api.client.util.Key
private java.lang.String clientAccountId;
/** The account ID of the client buyer to list users for. (required) You must specify either a string
representation of a numerical account identifier or the `-` character to list all the client users
for all the clients of a given sponsor buyer.
*/
public java.lang.String getClientAccountId() {
return clientAccountId;
}
/**
* The account ID of the client buyer to list users for. (required) You must specify
* either a string representation of a numerical account identifier or the `-` character
* to list all the client users for all the clients of a given sponsor buyer.
*/
public List setClientAccountId(java.lang.String clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
/**
* Requested page size. The server may return fewer clients than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer clients than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer clients than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientUsersResponse.nextPageToken returned from the previous call to the
* accounts.clients.users.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListClientUsersResponse.nextPageToken returned from the previous call to the
accounts.clients.users.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientUsersResponse.nextPageToken returned from the previous call to the
* accounts.clients.users.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing client user. Only the user status can be changed on update.
*
* Create a request for the method "users.update".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user to be retrieved is associated with.
* (required)
* @param userId Numerical identifier of the user to retrieve. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser}
* @return the request
*/
public Update update(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long userId, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser content) throws java.io.IOException {
Update result = new Update(accountId, clientAccountId, userId, content);
initialize(result);
return result;
}
public class Update extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/users/{userId}";
/**
* Updates an existing client user. Only the user status can be changed on update.
*
* Create a request for the method "users.update".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user to be retrieved is associated with.
* (required)
* @param userId Numerical identifier of the user to retrieve. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser}
* @since 1.13
*/
protected Update(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long userId, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser content) {
super(AdExchangeBuyerII.this, "PUT", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public Update setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Numerical account ID of the client buyer that the user to be retrieved is associated
* with. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Numerical account ID of the client buyer that the user to be retrieved is associated with.
(required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/**
* Numerical account ID of the client buyer that the user to be retrieved is associated
* with. (required)
*/
public Update setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
/** Numerical identifier of the user to retrieve. (required) */
@com.google.api.client.util.Key
private java.lang.Long userId;
/** Numerical identifier of the user to retrieve. (required)
*/
public java.lang.Long getUserId() {
return userId;
}
/** Numerical identifier of the user to retrieve. (required) */
public Update setUserId(java.lang.Long userId) {
this.userId = userId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Creatives collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Creatives.List request = adexchangebuyer2.creatives().list(parameters ...)}
*
*
* @return the resource collection
*/
public Creatives creatives() {
return new Creatives();
}
/**
* The "creatives" collection of methods.
*/
public class Creatives {
/**
* Creates a creative.
*
* Create a request for the method "creatives.create".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param accountId The account that this creative belongs to. Can be used to filter the response of the creatives.list
* method.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Creative}
* @return the request
*/
public Create create(java.lang.String accountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Creative content) throws java.io.IOException {
Create result = new Create(accountId, content);
initialize(result);
return result;
}
public class Create extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/creatives";
/**
* Creates a creative.
*
* Create a request for the method "creatives.create".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId The account that this creative belongs to. Can be used to filter the response of the creatives.list
* method.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Creative}
* @since 1.13
*/
protected Create(java.lang.String accountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Creative content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Creative.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* The account that this creative belongs to. Can be used to filter the response of the
* creatives.list method.
*/
@com.google.api.client.util.Key
private java.lang.String accountId;
/** The account that this creative belongs to. Can be used to filter the response of the creatives.list
method.
*/
public java.lang.String getAccountId() {
return accountId;
}
/**
* The account that this creative belongs to. Can be used to filter the response of the
* creatives.list method.
*/
public Create setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/**
* Indicates if multiple creatives can share an ID or not. Default is NO_DUPLICATES (one ID
* per creative).
*/
@com.google.api.client.util.Key
private java.lang.String duplicateIdMode;
/** Indicates if multiple creatives can share an ID or not. Default is NO_DUPLICATES (one ID per
creative).
*/
public java.lang.String getDuplicateIdMode() {
return duplicateIdMode;
}
/**
* Indicates if multiple creatives can share an ID or not. Default is NO_DUPLICATES (one ID
* per creative).
*/
public Create setDuplicateIdMode(java.lang.String duplicateIdMode) {
this.duplicateIdMode = duplicateIdMode;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets a creative.
*
* Create a request for the method "creatives.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param accountId The account the creative belongs to.
* @param creativeId The ID of the creative to retrieve.
* @return the request
*/
public Get get(java.lang.String accountId, java.lang.String creativeId) throws java.io.IOException {
Get result = new Get(accountId, creativeId);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/creatives/{creativeId}";
/**
* Gets a creative.
*
* Create a request for the method "creatives.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId The account the creative belongs to.
* @param creativeId The ID of the creative to retrieve.
* @since 1.13
*/
protected Get(java.lang.String accountId, java.lang.String creativeId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.Creative.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.creativeId = com.google.api.client.util.Preconditions.checkNotNull(creativeId, "Required parameter creativeId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The account the creative belongs to. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** The account the creative belongs to.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** The account the creative belongs to. */
public Get setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The ID of the creative to retrieve. */
@com.google.api.client.util.Key
private java.lang.String creativeId;
/** The ID of the creative to retrieve.
*/
public java.lang.String getCreativeId() {
return creativeId;
}
/** The ID of the creative to retrieve. */
public Get setCreativeId(java.lang.String creativeId) {
this.creativeId = creativeId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists creatives.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId The account to list the creatives from. Specify "-" to list all creatives the current user has
* access to.
* @return the request
*/
public List list(java.lang.String accountId) throws java.io.IOException {
List result = new List(accountId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/creatives";
/**
* Lists creatives.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId The account to list the creatives from. Specify "-" to list all creatives the current user has
* access to.
* @since 1.13
*/
protected List(java.lang.String accountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListCreativesResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The account to list the creatives from. Specify "-" to list all creatives the current
* user has access to.
*/
@com.google.api.client.util.Key
private java.lang.String accountId;
/** The account to list the creatives from. Specify "-" to list all creatives the current user has
access to.
*/
public java.lang.String getAccountId() {
return accountId;
}
/**
* The account to list the creatives from. Specify "-" to list all creatives the current
* user has access to.
*/
public List setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/**
* Requested page size. The server may return fewer creatives than requested (due to timeout
* constraint) even if more are available through another call. If unspecified, server will
* pick an appropriate default. Acceptable values are 1 to 1000, inclusive.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer creatives than requested (due to timeout
constraint) even if more are available through another call. If unspecified, server will pick an
appropriate default. Acceptable values are 1 to 1000, inclusive.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer creatives than requested (due to timeout
* constraint) even if more are available through another call. If unspecified, server will
* pick an appropriate default. Acceptable values are 1 to 1000, inclusive.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListCreativesResponse.next_page_token returned from the previous call to
* 'ListCreatives' method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListCreativesResponse.next_page_token returned from the previous call to 'ListCreatives' method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListCreativesResponse.next_page_token returned from the previous call to
* 'ListCreatives' method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* An optional query string to filter creatives. If no filter is specified, all active
* creatives will be returned. Supported queries are: - accountId=*account_id_string* -
* creativeId=*creative_id_string* - dealsStatus: {approved, conditionally_approved,
* disapproved, not_checked} - openAuctionStatus: {approved, conditionally_approved,
* disapproved, not_checked} - attribute: {a numeric attribute from the list of attributes}
* - disapprovalReason: {a reason from DisapprovalReason} Example: 'accountId=12345 AND
* (dealsStatus:disapproved AND disapprovalReason:unacceptable_content) OR attribute:47'
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** An optional query string to filter creatives. If no filter is specified, all active creatives will
be returned. Supported queries are: - accountId=*account_id_string* -
creativeId=*creative_id_string* - dealsStatus: {approved, conditionally_approved, disapproved,
not_checked} - openAuctionStatus: {approved, conditionally_approved, disapproved, not_checked} -
attribute: {a numeric attribute from the list of attributes} - disapprovalReason: {a reason from
DisapprovalReason} Example: 'accountId=12345 AND (dealsStatus:disapproved AND
disapprovalReason:unacceptable_content) OR attribute:47'
*/
public java.lang.String getQuery() {
return query;
}
/**
* An optional query string to filter creatives. If no filter is specified, all active
* creatives will be returned. Supported queries are: - accountId=*account_id_string* -
* creativeId=*creative_id_string* - dealsStatus: {approved, conditionally_approved,
* disapproved, not_checked} - openAuctionStatus: {approved, conditionally_approved,
* disapproved, not_checked} - attribute: {a numeric attribute from the list of attributes}
* - disapprovalReason: {a reason from DisapprovalReason} Example: 'accountId=12345 AND
* (dealsStatus:disapproved AND disapprovalReason:unacceptable_content) OR attribute:47'
*/
public List setQuery(java.lang.String query) {
this.query = query;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Stops watching a creative. Will stop push notifications being sent to the topics when the
* creative changes status.
*
* Create a request for the method "creatives.stopWatching".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link StopWatching#execute()} method to invoke the remote
* operation.
*
* @param accountId The account of the creative to stop notifications for.
* @param creativeId The creative ID of the creative to stop notifications for. Specify "-" to specify stopping account
* level notifications.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.StopWatchingCreativeRequest}
* @return the request
*/
public StopWatching stopWatching(java.lang.String accountId, java.lang.String creativeId, com.google.api.services.adexchangebuyer2.v2beta1.model.StopWatchingCreativeRequest content) throws java.io.IOException {
StopWatching result = new StopWatching(accountId, creativeId, content);
initialize(result);
return result;
}
public class StopWatching extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/creatives/{creativeId}:stopWatching";
/**
* Stops watching a creative. Will stop push notifications being sent to the topics when the
* creative changes status.
*
* Create a request for the method "creatives.stopWatching".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link StopWatching#execute()} method to invoke the remote
* operation. {@link
* StopWatching#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId The account of the creative to stop notifications for.
* @param creativeId The creative ID of the creative to stop notifications for. Specify "-" to specify stopping account
* level notifications.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.StopWatchingCreativeRequest}
* @since 1.13
*/
protected StopWatching(java.lang.String accountId, java.lang.String creativeId, com.google.api.services.adexchangebuyer2.v2beta1.model.StopWatchingCreativeRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Empty.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.creativeId = com.google.api.client.util.Preconditions.checkNotNull(creativeId, "Required parameter creativeId must be specified.");
}
@Override
public StopWatching set$Xgafv(java.lang.String $Xgafv) {
return (StopWatching) super.set$Xgafv($Xgafv);
}
@Override
public StopWatching setAccessToken(java.lang.String accessToken) {
return (StopWatching) super.setAccessToken(accessToken);
}
@Override
public StopWatching setAlt(java.lang.String alt) {
return (StopWatching) super.setAlt(alt);
}
@Override
public StopWatching setCallback(java.lang.String callback) {
return (StopWatching) super.setCallback(callback);
}
@Override
public StopWatching setFields(java.lang.String fields) {
return (StopWatching) super.setFields(fields);
}
@Override
public StopWatching setKey(java.lang.String key) {
return (StopWatching) super.setKey(key);
}
@Override
public StopWatching setOauthToken(java.lang.String oauthToken) {
return (StopWatching) super.setOauthToken(oauthToken);
}
@Override
public StopWatching setPrettyPrint(java.lang.Boolean prettyPrint) {
return (StopWatching) super.setPrettyPrint(prettyPrint);
}
@Override
public StopWatching setQuotaUser(java.lang.String quotaUser) {
return (StopWatching) super.setQuotaUser(quotaUser);
}
@Override
public StopWatching setUploadType(java.lang.String uploadType) {
return (StopWatching) super.setUploadType(uploadType);
}
@Override
public StopWatching setUploadProtocol(java.lang.String uploadProtocol) {
return (StopWatching) super.setUploadProtocol(uploadProtocol);
}
/** The account of the creative to stop notifications for. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** The account of the creative to stop notifications for.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** The account of the creative to stop notifications for. */
public StopWatching setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/**
* The creative ID of the creative to stop notifications for. Specify "-" to specify
* stopping account level notifications.
*/
@com.google.api.client.util.Key
private java.lang.String creativeId;
/** The creative ID of the creative to stop notifications for. Specify "-" to specify stopping account
level notifications.
*/
public java.lang.String getCreativeId() {
return creativeId;
}
/**
* The creative ID of the creative to stop notifications for. Specify "-" to specify
* stopping account level notifications.
*/
public StopWatching setCreativeId(java.lang.String creativeId) {
this.creativeId = creativeId;
return this;
}
@Override
public StopWatching set(String parameterName, Object value) {
return (StopWatching) super.set(parameterName, value);
}
}
/**
* Updates a creative.
*
* Create a request for the method "creatives.update".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param accountId The account that this creative belongs to. Can be used to filter the response of the creatives.list
* method.
* @param creativeId The buyer-defined creative ID of this creative. Can be used to filter the response of the
* creatives.list method.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Creative}
* @return the request
*/
public Update update(java.lang.String accountId, java.lang.String creativeId, com.google.api.services.adexchangebuyer2.v2beta1.model.Creative content) throws java.io.IOException {
Update result = new Update(accountId, creativeId, content);
initialize(result);
return result;
}
public class Update extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/creatives/{creativeId}";
/**
* Updates a creative.
*
* Create a request for the method "creatives.update".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId The account that this creative belongs to. Can be used to filter the response of the creatives.list
* method.
* @param creativeId The buyer-defined creative ID of this creative. Can be used to filter the response of the
* creatives.list method.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Creative}
* @since 1.13
*/
protected Update(java.lang.String accountId, java.lang.String creativeId, com.google.api.services.adexchangebuyer2.v2beta1.model.Creative content) {
super(AdExchangeBuyerII.this, "PUT", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Creative.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.creativeId = com.google.api.client.util.Preconditions.checkNotNull(creativeId, "Required parameter creativeId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* The account that this creative belongs to. Can be used to filter the response of the
* creatives.list method.
*/
@com.google.api.client.util.Key
private java.lang.String accountId;
/** The account that this creative belongs to. Can be used to filter the response of the creatives.list
method.
*/
public java.lang.String getAccountId() {
return accountId;
}
/**
* The account that this creative belongs to. Can be used to filter the response of the
* creatives.list method.
*/
public Update setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/**
* The buyer-defined creative ID of this creative. Can be used to filter the response of the
* creatives.list method.
*/
@com.google.api.client.util.Key
private java.lang.String creativeId;
/** The buyer-defined creative ID of this creative. Can be used to filter the response of the
creatives.list method.
*/
public java.lang.String getCreativeId() {
return creativeId;
}
/**
* The buyer-defined creative ID of this creative. Can be used to filter the response of the
* creatives.list method.
*/
public Update setCreativeId(java.lang.String creativeId) {
this.creativeId = creativeId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
/**
* Watches a creative. Will result in push notifications being sent to the topic when the creative
* changes status.
*
* Create a request for the method "creatives.watch".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Watch#execute()} method to invoke the remote operation.
*
* @param accountId The account of the creative to watch.
* @param creativeId The creative ID to watch for status changes. Specify "-" to watch all creatives under the above
* account. If both creative-level and account-level notifications are sent, only a single
* notification will be sent to the creative-level notification topic.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.WatchCreativeRequest}
* @return the request
*/
public Watch watch(java.lang.String accountId, java.lang.String creativeId, com.google.api.services.adexchangebuyer2.v2beta1.model.WatchCreativeRequest content) throws java.io.IOException {
Watch result = new Watch(accountId, creativeId, content);
initialize(result);
return result;
}
public class Watch extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/creatives/{creativeId}:watch";
/**
* Watches a creative. Will result in push notifications being sent to the topic when the creative
* changes status.
*
* Create a request for the method "creatives.watch".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Watch#execute()} method to invoke the remote operation.
* {@link
* Watch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId The account of the creative to watch.
* @param creativeId The creative ID to watch for status changes. Specify "-" to watch all creatives under the above
* account. If both creative-level and account-level notifications are sent, only a single
* notification will be sent to the creative-level notification topic.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.WatchCreativeRequest}
* @since 1.13
*/
protected Watch(java.lang.String accountId, java.lang.String creativeId, com.google.api.services.adexchangebuyer2.v2beta1.model.WatchCreativeRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Empty.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.creativeId = com.google.api.client.util.Preconditions.checkNotNull(creativeId, "Required parameter creativeId must be specified.");
}
@Override
public Watch set$Xgafv(java.lang.String $Xgafv) {
return (Watch) super.set$Xgafv($Xgafv);
}
@Override
public Watch setAccessToken(java.lang.String accessToken) {
return (Watch) super.setAccessToken(accessToken);
}
@Override
public Watch setAlt(java.lang.String alt) {
return (Watch) super.setAlt(alt);
}
@Override
public Watch setCallback(java.lang.String callback) {
return (Watch) super.setCallback(callback);
}
@Override
public Watch setFields(java.lang.String fields) {
return (Watch) super.setFields(fields);
}
@Override
public Watch setKey(java.lang.String key) {
return (Watch) super.setKey(key);
}
@Override
public Watch setOauthToken(java.lang.String oauthToken) {
return (Watch) super.setOauthToken(oauthToken);
}
@Override
public Watch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Watch) super.setPrettyPrint(prettyPrint);
}
@Override
public Watch setQuotaUser(java.lang.String quotaUser) {
return (Watch) super.setQuotaUser(quotaUser);
}
@Override
public Watch setUploadType(java.lang.String uploadType) {
return (Watch) super.setUploadType(uploadType);
}
@Override
public Watch setUploadProtocol(java.lang.String uploadProtocol) {
return (Watch) super.setUploadProtocol(uploadProtocol);
}
/** The account of the creative to watch. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** The account of the creative to watch.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** The account of the creative to watch. */
public Watch setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/**
* The creative ID to watch for status changes. Specify "-" to watch all creatives under the
* above account. If both creative-level and account-level notifications are sent, only a
* single notification will be sent to the creative-level notification topic.
*/
@com.google.api.client.util.Key
private java.lang.String creativeId;
/** The creative ID to watch for status changes. Specify "-" to watch all creatives under the above
account. If both creative-level and account-level notifications are sent, only a single
notification will be sent to the creative-level notification topic.
*/
public java.lang.String getCreativeId() {
return creativeId;
}
/**
* The creative ID to watch for status changes. Specify "-" to watch all creatives under the
* above account. If both creative-level and account-level notifications are sent, only a
* single notification will be sent to the creative-level notification topic.
*/
public Watch setCreativeId(java.lang.String creativeId) {
this.creativeId = creativeId;
return this;
}
@Override
public Watch set(String parameterName, Object value) {
return (Watch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the DealAssociations collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.DealAssociations.List request = adexchangebuyer2.dealAssociations().list(parameters ...)}
*
*
* @return the resource collection
*/
public DealAssociations dealAssociations() {
return new DealAssociations();
}
/**
* The "dealAssociations" collection of methods.
*/
public class DealAssociations {
/**
* Associate an existing deal with a creative.
*
* Create a request for the method "dealAssociations.add".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Add#execute()} method to invoke the remote operation.
*
* @param accountId The account the creative belongs to.
* @param creativeId The ID of the creative associated with the deal.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.AddDealAssociationRequest}
* @return the request
*/
public Add add(java.lang.String accountId, java.lang.String creativeId, com.google.api.services.adexchangebuyer2.v2beta1.model.AddDealAssociationRequest content) throws java.io.IOException {
Add result = new Add(accountId, creativeId, content);
initialize(result);
return result;
}
public class Add extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/creatives/{creativeId}/dealAssociations:add";
/**
* Associate an existing deal with a creative.
*
* Create a request for the method "dealAssociations.add".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Add#execute()} method to invoke the remote operation.
* {@link Add#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId The account the creative belongs to.
* @param creativeId The ID of the creative associated with the deal.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.AddDealAssociationRequest}
* @since 1.13
*/
protected Add(java.lang.String accountId, java.lang.String creativeId, com.google.api.services.adexchangebuyer2.v2beta1.model.AddDealAssociationRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Empty.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.creativeId = com.google.api.client.util.Preconditions.checkNotNull(creativeId, "Required parameter creativeId must be specified.");
}
@Override
public Add set$Xgafv(java.lang.String $Xgafv) {
return (Add) super.set$Xgafv($Xgafv);
}
@Override
public Add setAccessToken(java.lang.String accessToken) {
return (Add) super.setAccessToken(accessToken);
}
@Override
public Add setAlt(java.lang.String alt) {
return (Add) super.setAlt(alt);
}
@Override
public Add setCallback(java.lang.String callback) {
return (Add) super.setCallback(callback);
}
@Override
public Add setFields(java.lang.String fields) {
return (Add) super.setFields(fields);
}
@Override
public Add setKey(java.lang.String key) {
return (Add) super.setKey(key);
}
@Override
public Add setOauthToken(java.lang.String oauthToken) {
return (Add) super.setOauthToken(oauthToken);
}
@Override
public Add setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Add) super.setPrettyPrint(prettyPrint);
}
@Override
public Add setQuotaUser(java.lang.String quotaUser) {
return (Add) super.setQuotaUser(quotaUser);
}
@Override
public Add setUploadType(java.lang.String uploadType) {
return (Add) super.setUploadType(uploadType);
}
@Override
public Add setUploadProtocol(java.lang.String uploadProtocol) {
return (Add) super.setUploadProtocol(uploadProtocol);
}
/** The account the creative belongs to. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** The account the creative belongs to.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** The account the creative belongs to. */
public Add setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The ID of the creative associated with the deal. */
@com.google.api.client.util.Key
private java.lang.String creativeId;
/** The ID of the creative associated with the deal.
*/
public java.lang.String getCreativeId() {
return creativeId;
}
/** The ID of the creative associated with the deal. */
public Add setCreativeId(java.lang.String creativeId) {
this.creativeId = creativeId;
return this;
}
@Override
public Add set(String parameterName, Object value) {
return (Add) super.set(parameterName, value);
}
}
/**
* List all creative-deal associations.
*
* Create a request for the method "dealAssociations.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId The account to list the associations from. Specify "-" to list all creatives the current user has
* access to.
* @param creativeId The creative ID to list the associations from. Specify "-" to list all creatives under the above
* account.
* @return the request
*/
public List list(java.lang.String accountId, java.lang.String creativeId) throws java.io.IOException {
List result = new List(accountId, creativeId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/creatives/{creativeId}/dealAssociations";
/**
* List all creative-deal associations.
*
* Create a request for the method "dealAssociations.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId The account to list the associations from. Specify "-" to list all creatives the current user has
* access to.
* @param creativeId The creative ID to list the associations from. Specify "-" to list all creatives under the above
* account.
* @since 1.13
*/
protected List(java.lang.String accountId, java.lang.String creativeId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListDealAssociationsResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.creativeId = com.google.api.client.util.Preconditions.checkNotNull(creativeId, "Required parameter creativeId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The account to list the associations from. Specify "-" to list all creatives the
* current user has access to.
*/
@com.google.api.client.util.Key
private java.lang.String accountId;
/** The account to list the associations from. Specify "-" to list all creatives the current user has
access to.
*/
public java.lang.String getAccountId() {
return accountId;
}
/**
* The account to list the associations from. Specify "-" to list all creatives the
* current user has access to.
*/
public List setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/**
* The creative ID to list the associations from. Specify "-" to list all creatives under
* the above account.
*/
@com.google.api.client.util.Key
private java.lang.String creativeId;
/** The creative ID to list the associations from. Specify "-" to list all creatives under the above
account.
*/
public java.lang.String getCreativeId() {
return creativeId;
}
/**
* The creative ID to list the associations from. Specify "-" to list all creatives under
* the above account.
*/
public List setCreativeId(java.lang.String creativeId) {
this.creativeId = creativeId;
return this;
}
/**
* Requested page size. Server may return fewer associations than requested. If
* unspecified, server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. Server may return fewer associations than requested. If unspecified, server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. Server may return fewer associations than requested. If
* unspecified, server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListDealAssociationsResponse.next_page_token returned from the previous call
* to 'ListDealAssociations' method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListDealAssociationsResponse.next_page_token returned from the previous call to
'ListDealAssociations' method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListDealAssociationsResponse.next_page_token returned from the previous call
* to 'ListDealAssociations' method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* An optional query string to filter deal associations. If no filter is specified, all
* associations will be returned. Supported queries are: - accountId=*account_id_string* -
* creativeId=*creative_id_string* - dealsId=*deals_id_string* - dealsStatus:{approved,
* conditionally_approved, disapproved, not_checked} - openAuctionStatus:{approved,
* conditionally_approved, disapproved, not_checked} Example: 'dealsId=12345 AND
* dealsStatus:disapproved'
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** An optional query string to filter deal associations. If no filter is specified, all associations
will be returned. Supported queries are: - accountId=*account_id_string* -
creativeId=*creative_id_string* - dealsId=*deals_id_string* - dealsStatus:{approved,
conditionally_approved, disapproved, not_checked} - openAuctionStatus:{approved,
conditionally_approved, disapproved, not_checked} Example: 'dealsId=12345 AND
dealsStatus:disapproved'
*/
public java.lang.String getQuery() {
return query;
}
/**
* An optional query string to filter deal associations. If no filter is specified, all
* associations will be returned. Supported queries are: - accountId=*account_id_string* -
* creativeId=*creative_id_string* - dealsId=*deals_id_string* - dealsStatus:{approved,
* conditionally_approved, disapproved, not_checked} - openAuctionStatus:{approved,
* conditionally_approved, disapproved, not_checked} Example: 'dealsId=12345 AND
* dealsStatus:disapproved'
*/
public List setQuery(java.lang.String query) {
this.query = query;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Remove the association between a deal and a creative.
*
* Create a request for the method "dealAssociations.remove".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Remove#execute()} method to invoke the remote operation.
*
* @param accountId The account the creative belongs to.
* @param creativeId The ID of the creative associated with the deal.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.RemoveDealAssociationRequest}
* @return the request
*/
public Remove remove(java.lang.String accountId, java.lang.String creativeId, com.google.api.services.adexchangebuyer2.v2beta1.model.RemoveDealAssociationRequest content) throws java.io.IOException {
Remove result = new Remove(accountId, creativeId, content);
initialize(result);
return result;
}
public class Remove extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/creatives/{creativeId}/dealAssociations:remove";
/**
* Remove the association between a deal and a creative.
*
* Create a request for the method "dealAssociations.remove".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Remove#execute()} method to invoke the remote operation.
* {@link
* Remove#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId The account the creative belongs to.
* @param creativeId The ID of the creative associated with the deal.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.RemoveDealAssociationRequest}
* @since 1.13
*/
protected Remove(java.lang.String accountId, java.lang.String creativeId, com.google.api.services.adexchangebuyer2.v2beta1.model.RemoveDealAssociationRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Empty.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.creativeId = com.google.api.client.util.Preconditions.checkNotNull(creativeId, "Required parameter creativeId must be specified.");
}
@Override
public Remove set$Xgafv(java.lang.String $Xgafv) {
return (Remove) super.set$Xgafv($Xgafv);
}
@Override
public Remove setAccessToken(java.lang.String accessToken) {
return (Remove) super.setAccessToken(accessToken);
}
@Override
public Remove setAlt(java.lang.String alt) {
return (Remove) super.setAlt(alt);
}
@Override
public Remove setCallback(java.lang.String callback) {
return (Remove) super.setCallback(callback);
}
@Override
public Remove setFields(java.lang.String fields) {
return (Remove) super.setFields(fields);
}
@Override
public Remove setKey(java.lang.String key) {
return (Remove) super.setKey(key);
}
@Override
public Remove setOauthToken(java.lang.String oauthToken) {
return (Remove) super.setOauthToken(oauthToken);
}
@Override
public Remove setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Remove) super.setPrettyPrint(prettyPrint);
}
@Override
public Remove setQuotaUser(java.lang.String quotaUser) {
return (Remove) super.setQuotaUser(quotaUser);
}
@Override
public Remove setUploadType(java.lang.String uploadType) {
return (Remove) super.setUploadType(uploadType);
}
@Override
public Remove setUploadProtocol(java.lang.String uploadProtocol) {
return (Remove) super.setUploadProtocol(uploadProtocol);
}
/** The account the creative belongs to. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** The account the creative belongs to.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** The account the creative belongs to. */
public Remove setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The ID of the creative associated with the deal. */
@com.google.api.client.util.Key
private java.lang.String creativeId;
/** The ID of the creative associated with the deal.
*/
public java.lang.String getCreativeId() {
return creativeId;
}
/** The ID of the creative associated with the deal. */
public Remove setCreativeId(java.lang.String creativeId) {
this.creativeId = creativeId;
return this;
}
@Override
public Remove set(String parameterName, Object value) {
return (Remove) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the FinalizedProposals collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.FinalizedProposals.List request = adexchangebuyer2.finalizedProposals().list(parameters ...)}
*
*
* @return the resource collection
*/
public FinalizedProposals finalizedProposals() {
return new FinalizedProposals();
}
/**
* The "finalizedProposals" collection of methods.
*/
public class FinalizedProposals {
/**
* List finalized proposals, regardless if a proposal is being renegotiated. A filter expression
* (PQL query) may be specified to filter the results. The notes will not be returned.
*
* Create a request for the method "finalizedProposals.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @return the request
*/
public List list(java.lang.String accountId) throws java.io.IOException {
List result = new List(accountId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/finalizedProposals";
/**
* List finalized proposals, regardless if a proposal is being renegotiated. A filter expression
* (PQL query) may be specified to filter the results. The notes will not be returned.
*
* Create a request for the method "finalizedProposals.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @since 1.13
*/
protected List(java.lang.String accountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListProposalsResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public List setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/**
* An optional PQL filter query used to query for proposals. Nested repeated fields, such as
* proposal.deals.targetingCriterion, cannot be filtered.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** An optional PQL filter query used to query for proposals. Nested repeated fields, such as
proposal.deals.targetingCriterion, cannot be filtered.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* An optional PQL filter query used to query for proposals. Nested repeated fields, such as
* proposal.deals.targetingCriterion, cannot be filtered.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Syntax the filter is written in. Current implementation defaults to PQL but in the future
* it will be LIST_FILTER.
*/
@com.google.api.client.util.Key
private java.lang.String filterSyntax;
/** Syntax the filter is written in. Current implementation defaults to PQL but in the future it will
be LIST_FILTER.
*/
public java.lang.String getFilterSyntax() {
return filterSyntax;
}
/**
* Syntax the filter is written in. Current implementation defaults to PQL but in the future
* it will be LIST_FILTER.
*/
public List setFilterSyntax(java.lang.String filterSyntax) {
this.filterSyntax = filterSyntax;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If unspecified,
* the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If unspecified,
* the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The page token as returned from ListProposalsResponse. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The page token as returned from ListProposalsResponse.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The page token as returned from ListProposalsResponse. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Update given deals to pause serving. This method will set the
* `DealServingMetadata.DealPauseStatus.has_buyer_paused` bit to true for all listed deals in the
* request. Currently, this method only applies to PG and PD deals. For PA deals, call
* accounts.proposals.pause endpoint. It is a no-op to pause already-paused deals. It is an error to
* call PauseProposalDeals for deals which are not part of the proposal of proposal_id or which are
* not finalized or renegotiating.
*
* Create a request for the method "finalizedProposals.pause".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Pause#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @param proposalId The proposal_id of the proposal containing the deals.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.PauseProposalDealsRequest}
* @return the request
*/
public Pause pause(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.PauseProposalDealsRequest content) throws java.io.IOException {
Pause result = new Pause(accountId, proposalId, content);
initialize(result);
return result;
}
public class Pause extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/finalizedProposals/{proposalId}:pause";
/**
* Update given deals to pause serving. This method will set the
* `DealServingMetadata.DealPauseStatus.has_buyer_paused` bit to true for all listed deals in the
* request. Currently, this method only applies to PG and PD deals. For PA deals, call
* accounts.proposals.pause endpoint. It is a no-op to pause already-paused deals. It is an error
* to call PauseProposalDeals for deals which are not part of the proposal of proposal_id or which
* are not finalized or renegotiating.
*
* Create a request for the method "finalizedProposals.pause".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Pause#execute()} method to invoke the remote operation.
* {@link
* Pause#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param proposalId The proposal_id of the proposal containing the deals.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.PauseProposalDealsRequest}
* @since 1.13
*/
protected Pause(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.PauseProposalDealsRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.proposalId = com.google.api.client.util.Preconditions.checkNotNull(proposalId, "Required parameter proposalId must be specified.");
}
@Override
public Pause set$Xgafv(java.lang.String $Xgafv) {
return (Pause) super.set$Xgafv($Xgafv);
}
@Override
public Pause setAccessToken(java.lang.String accessToken) {
return (Pause) super.setAccessToken(accessToken);
}
@Override
public Pause setAlt(java.lang.String alt) {
return (Pause) super.setAlt(alt);
}
@Override
public Pause setCallback(java.lang.String callback) {
return (Pause) super.setCallback(callback);
}
@Override
public Pause setFields(java.lang.String fields) {
return (Pause) super.setFields(fields);
}
@Override
public Pause setKey(java.lang.String key) {
return (Pause) super.setKey(key);
}
@Override
public Pause setOauthToken(java.lang.String oauthToken) {
return (Pause) super.setOauthToken(oauthToken);
}
@Override
public Pause setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Pause) super.setPrettyPrint(prettyPrint);
}
@Override
public Pause setQuotaUser(java.lang.String quotaUser) {
return (Pause) super.setQuotaUser(quotaUser);
}
@Override
public Pause setUploadType(java.lang.String uploadType) {
return (Pause) super.setUploadType(uploadType);
}
@Override
public Pause setUploadProtocol(java.lang.String uploadProtocol) {
return (Pause) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public Pause setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The proposal_id of the proposal containing the deals. */
@com.google.api.client.util.Key
private java.lang.String proposalId;
/** The proposal_id of the proposal containing the deals.
*/
public java.lang.String getProposalId() {
return proposalId;
}
/** The proposal_id of the proposal containing the deals. */
public Pause setProposalId(java.lang.String proposalId) {
this.proposalId = proposalId;
return this;
}
@Override
public Pause set(String parameterName, Object value) {
return (Pause) super.set(parameterName, value);
}
}
/**
* Update given deals to resume serving. This method will set the
* `DealServingMetadata.DealPauseStatus.has_buyer_paused` bit to false for all listed deals in the
* request. Currently, this method only applies to PG and PD deals. For PA deals, call
* accounts.proposals.resume endpoint. It is a no-op to resume running deals or deals paused by the
* other party. It is an error to call ResumeProposalDeals for deals which are not part of the
* proposal of proposal_id or which are not finalized or renegotiating.
*
* Create a request for the method "finalizedProposals.resume".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Resume#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @param proposalId The proposal_id of the proposal containing the deals.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ResumeProposalDealsRequest}
* @return the request
*/
public Resume resume(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.ResumeProposalDealsRequest content) throws java.io.IOException {
Resume result = new Resume(accountId, proposalId, content);
initialize(result);
return result;
}
public class Resume extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/finalizedProposals/{proposalId}:resume";
/**
* Update given deals to resume serving. This method will set the
* `DealServingMetadata.DealPauseStatus.has_buyer_paused` bit to false for all listed deals in the
* request. Currently, this method only applies to PG and PD deals. For PA deals, call
* accounts.proposals.resume endpoint. It is a no-op to resume running deals or deals paused by
* the other party. It is an error to call ResumeProposalDeals for deals which are not part of the
* proposal of proposal_id or which are not finalized or renegotiating.
*
* Create a request for the method "finalizedProposals.resume".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Resume#execute()} method to invoke the remote operation.
* {@link
* Resume#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param proposalId The proposal_id of the proposal containing the deals.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ResumeProposalDealsRequest}
* @since 1.13
*/
protected Resume(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.ResumeProposalDealsRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.proposalId = com.google.api.client.util.Preconditions.checkNotNull(proposalId, "Required parameter proposalId must be specified.");
}
@Override
public Resume set$Xgafv(java.lang.String $Xgafv) {
return (Resume) super.set$Xgafv($Xgafv);
}
@Override
public Resume setAccessToken(java.lang.String accessToken) {
return (Resume) super.setAccessToken(accessToken);
}
@Override
public Resume setAlt(java.lang.String alt) {
return (Resume) super.setAlt(alt);
}
@Override
public Resume setCallback(java.lang.String callback) {
return (Resume) super.setCallback(callback);
}
@Override
public Resume setFields(java.lang.String fields) {
return (Resume) super.setFields(fields);
}
@Override
public Resume setKey(java.lang.String key) {
return (Resume) super.setKey(key);
}
@Override
public Resume setOauthToken(java.lang.String oauthToken) {
return (Resume) super.setOauthToken(oauthToken);
}
@Override
public Resume setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Resume) super.setPrettyPrint(prettyPrint);
}
@Override
public Resume setQuotaUser(java.lang.String quotaUser) {
return (Resume) super.setQuotaUser(quotaUser);
}
@Override
public Resume setUploadType(java.lang.String uploadType) {
return (Resume) super.setUploadType(uploadType);
}
@Override
public Resume setUploadProtocol(java.lang.String uploadProtocol) {
return (Resume) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public Resume setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The proposal_id of the proposal containing the deals. */
@com.google.api.client.util.Key
private java.lang.String proposalId;
/** The proposal_id of the proposal containing the deals.
*/
public java.lang.String getProposalId() {
return proposalId;
}
/** The proposal_id of the proposal containing the deals. */
public Resume setProposalId(java.lang.String proposalId) {
this.proposalId = proposalId;
return this;
}
@Override
public Resume set(String parameterName, Object value) {
return (Resume) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Products collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Products.List request = adexchangebuyer2.products().list(parameters ...)}
*
*
* @return the resource collection
*/
public Products products() {
return new Products();
}
/**
* The "products" collection of methods.
*/
public class Products {
/**
* Gets the requested product by ID.
*
* Create a request for the method "products.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @param productId The ID for the product to get the head revision for.
* @return the request
*/
public Get get(java.lang.String accountId, java.lang.String productId) throws java.io.IOException {
Get result = new Get(accountId, productId);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/products/{productId}";
/**
* Gets the requested product by ID.
*
* Create a request for the method "products.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param productId The ID for the product to get the head revision for.
* @since 1.13
*/
protected Get(java.lang.String accountId, java.lang.String productId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.Product.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public Get setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The ID for the product to get the head revision for. */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The ID for the product to get the head revision for.
*/
public java.lang.String getProductId() {
return productId;
}
/** The ID for the product to get the head revision for. */
public Get setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List all products visible to the buyer (optionally filtered by the specified PQL query).
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @return the request
*/
public List list(java.lang.String accountId) throws java.io.IOException {
List result = new List(accountId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/products";
/**
* List all products visible to the buyer (optionally filtered by the specified PQL query).
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @since 1.13
*/
protected List(java.lang.String accountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListProductsResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public List setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/**
* An optional PQL query used to query for products. See https://developers.google.com/ad-
* manager/docs/pqlreference for documentation about PQL and examples. Nested repeated
* fields, such as product.targetingCriterion.inclusions, cannot be filtered.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** An optional PQL query used to query for products. See https://developers.google.com/ad-
manager/docs/pqlreference for documentation about PQL and examples. Nested repeated fields, such as
product.targetingCriterion.inclusions, cannot be filtered.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* An optional PQL query used to query for products. See https://developers.google.com/ad-
* manager/docs/pqlreference for documentation about PQL and examples. Nested repeated
* fields, such as product.targetingCriterion.inclusions, cannot be filtered.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If unspecified,
* the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If unspecified,
* the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The page token as returned from ListProductsResponse. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The page token as returned from ListProductsResponse.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The page token as returned from ListProductsResponse. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Proposals collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Proposals.List request = adexchangebuyer2.proposals().list(parameters ...)}
*
*
* @return the resource collection
*/
public Proposals proposals() {
return new Proposals();
}
/**
* The "proposals" collection of methods.
*/
public class Proposals {
/**
* Mark the proposal as accepted at the given revision number. If the number does not match the
* server's revision number an `ABORTED` error message will be returned. This call updates the
* proposal_state from `PROPOSED` to `BUYER_ACCEPTED`, or from `SELLER_ACCEPTED` to `FINALIZED`.
* Upon calling this endpoint, the buyer implicitly agrees to the terms and conditions optionally
* set within the proposal by the publisher.
*
* Create a request for the method "proposals.accept".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Accept#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to accept.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.AcceptProposalRequest}
* @return the request
*/
public Accept accept(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.AcceptProposalRequest content) throws java.io.IOException {
Accept result = new Accept(accountId, proposalId, content);
initialize(result);
return result;
}
public class Accept extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/proposals/{proposalId}:accept";
/**
* Mark the proposal as accepted at the given revision number. If the number does not match the
* server's revision number an `ABORTED` error message will be returned. This call updates the
* proposal_state from `PROPOSED` to `BUYER_ACCEPTED`, or from `SELLER_ACCEPTED` to `FINALIZED`.
* Upon calling this endpoint, the buyer implicitly agrees to the terms and conditions optionally
* set within the proposal by the publisher.
*
* Create a request for the method "proposals.accept".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Accept#execute()} method to invoke the remote operation.
* {@link
* Accept#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to accept.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.AcceptProposalRequest}
* @since 1.13
*/
protected Accept(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.AcceptProposalRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.proposalId = com.google.api.client.util.Preconditions.checkNotNull(proposalId, "Required parameter proposalId must be specified.");
}
@Override
public Accept set$Xgafv(java.lang.String $Xgafv) {
return (Accept) super.set$Xgafv($Xgafv);
}
@Override
public Accept setAccessToken(java.lang.String accessToken) {
return (Accept) super.setAccessToken(accessToken);
}
@Override
public Accept setAlt(java.lang.String alt) {
return (Accept) super.setAlt(alt);
}
@Override
public Accept setCallback(java.lang.String callback) {
return (Accept) super.setCallback(callback);
}
@Override
public Accept setFields(java.lang.String fields) {
return (Accept) super.setFields(fields);
}
@Override
public Accept setKey(java.lang.String key) {
return (Accept) super.setKey(key);
}
@Override
public Accept setOauthToken(java.lang.String oauthToken) {
return (Accept) super.setOauthToken(oauthToken);
}
@Override
public Accept setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Accept) super.setPrettyPrint(prettyPrint);
}
@Override
public Accept setQuotaUser(java.lang.String quotaUser) {
return (Accept) super.setQuotaUser(quotaUser);
}
@Override
public Accept setUploadType(java.lang.String uploadType) {
return (Accept) super.setUploadType(uploadType);
}
@Override
public Accept setUploadProtocol(java.lang.String uploadProtocol) {
return (Accept) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public Accept setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The ID of the proposal to accept. */
@com.google.api.client.util.Key
private java.lang.String proposalId;
/** The ID of the proposal to accept.
*/
public java.lang.String getProposalId() {
return proposalId;
}
/** The ID of the proposal to accept. */
public Accept setProposalId(java.lang.String proposalId) {
this.proposalId = proposalId;
return this;
}
@Override
public Accept set(String parameterName, Object value) {
return (Accept) super.set(parameterName, value);
}
}
/**
* Create a new note and attach it to the proposal. The note is assigned a unique ID by the server.
* The proposal revision number will not increase when associated with a new note.
*
* Create a request for the method "proposals.addNote".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link AddNote#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to attach the note to.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.AddNoteRequest}
* @return the request
*/
public AddNote addNote(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.AddNoteRequest content) throws java.io.IOException {
AddNote result = new AddNote(accountId, proposalId, content);
initialize(result);
return result;
}
public class AddNote extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/proposals/{proposalId}:addNote";
/**
* Create a new note and attach it to the proposal. The note is assigned a unique ID by the
* server. The proposal revision number will not increase when associated with a new note.
*
* Create a request for the method "proposals.addNote".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link AddNote#execute()} method to invoke the remote operation.
* {@link
* AddNote#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to attach the note to.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.AddNoteRequest}
* @since 1.13
*/
protected AddNote(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.AddNoteRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Note.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.proposalId = com.google.api.client.util.Preconditions.checkNotNull(proposalId, "Required parameter proposalId must be specified.");
}
@Override
public AddNote set$Xgafv(java.lang.String $Xgafv) {
return (AddNote) super.set$Xgafv($Xgafv);
}
@Override
public AddNote setAccessToken(java.lang.String accessToken) {
return (AddNote) super.setAccessToken(accessToken);
}
@Override
public AddNote setAlt(java.lang.String alt) {
return (AddNote) super.setAlt(alt);
}
@Override
public AddNote setCallback(java.lang.String callback) {
return (AddNote) super.setCallback(callback);
}
@Override
public AddNote setFields(java.lang.String fields) {
return (AddNote) super.setFields(fields);
}
@Override
public AddNote setKey(java.lang.String key) {
return (AddNote) super.setKey(key);
}
@Override
public AddNote setOauthToken(java.lang.String oauthToken) {
return (AddNote) super.setOauthToken(oauthToken);
}
@Override
public AddNote setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AddNote) super.setPrettyPrint(prettyPrint);
}
@Override
public AddNote setQuotaUser(java.lang.String quotaUser) {
return (AddNote) super.setQuotaUser(quotaUser);
}
@Override
public AddNote setUploadType(java.lang.String uploadType) {
return (AddNote) super.setUploadType(uploadType);
}
@Override
public AddNote setUploadProtocol(java.lang.String uploadProtocol) {
return (AddNote) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public AddNote setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The ID of the proposal to attach the note to. */
@com.google.api.client.util.Key
private java.lang.String proposalId;
/** The ID of the proposal to attach the note to.
*/
public java.lang.String getProposalId() {
return proposalId;
}
/** The ID of the proposal to attach the note to. */
public AddNote setProposalId(java.lang.String proposalId) {
this.proposalId = proposalId;
return this;
}
@Override
public AddNote set(String parameterName, Object value) {
return (AddNote) super.set(parameterName, value);
}
}
/**
* Cancel an ongoing negotiation on a proposal. This does not cancel or end serving for the deals if
* the proposal has been finalized, but only cancels a negotiation unilaterally.
*
* Create a request for the method "proposals.cancelNegotiation".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link CancelNegotiation#execute()} method to invoke the remote
* operation.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to cancel negotiation for.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.CancelNegotiationRequest}
* @return the request
*/
public CancelNegotiation cancelNegotiation(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.CancelNegotiationRequest content) throws java.io.IOException {
CancelNegotiation result = new CancelNegotiation(accountId, proposalId, content);
initialize(result);
return result;
}
public class CancelNegotiation extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/proposals/{proposalId}:cancelNegotiation";
/**
* Cancel an ongoing negotiation on a proposal. This does not cancel or end serving for the deals
* if the proposal has been finalized, but only cancels a negotiation unilaterally.
*
* Create a request for the method "proposals.cancelNegotiation".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link CancelNegotiation#execute()} method to invoke the remote
* operation. {@link CancelNegotiation#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to cancel negotiation for.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.CancelNegotiationRequest}
* @since 1.13
*/
protected CancelNegotiation(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.CancelNegotiationRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.proposalId = com.google.api.client.util.Preconditions.checkNotNull(proposalId, "Required parameter proposalId must be specified.");
}
@Override
public CancelNegotiation set$Xgafv(java.lang.String $Xgafv) {
return (CancelNegotiation) super.set$Xgafv($Xgafv);
}
@Override
public CancelNegotiation setAccessToken(java.lang.String accessToken) {
return (CancelNegotiation) super.setAccessToken(accessToken);
}
@Override
public CancelNegotiation setAlt(java.lang.String alt) {
return (CancelNegotiation) super.setAlt(alt);
}
@Override
public CancelNegotiation setCallback(java.lang.String callback) {
return (CancelNegotiation) super.setCallback(callback);
}
@Override
public CancelNegotiation setFields(java.lang.String fields) {
return (CancelNegotiation) super.setFields(fields);
}
@Override
public CancelNegotiation setKey(java.lang.String key) {
return (CancelNegotiation) super.setKey(key);
}
@Override
public CancelNegotiation setOauthToken(java.lang.String oauthToken) {
return (CancelNegotiation) super.setOauthToken(oauthToken);
}
@Override
public CancelNegotiation setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CancelNegotiation) super.setPrettyPrint(prettyPrint);
}
@Override
public CancelNegotiation setQuotaUser(java.lang.String quotaUser) {
return (CancelNegotiation) super.setQuotaUser(quotaUser);
}
@Override
public CancelNegotiation setUploadType(java.lang.String uploadType) {
return (CancelNegotiation) super.setUploadType(uploadType);
}
@Override
public CancelNegotiation setUploadProtocol(java.lang.String uploadProtocol) {
return (CancelNegotiation) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public CancelNegotiation setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The ID of the proposal to cancel negotiation for. */
@com.google.api.client.util.Key
private java.lang.String proposalId;
/** The ID of the proposal to cancel negotiation for.
*/
public java.lang.String getProposalId() {
return proposalId;
}
/** The ID of the proposal to cancel negotiation for. */
public CancelNegotiation setProposalId(java.lang.String proposalId) {
this.proposalId = proposalId;
return this;
}
@Override
public CancelNegotiation set(String parameterName, Object value) {
return (CancelNegotiation) super.set(parameterName, value);
}
}
/**
* You can opt-in to manually update proposals to indicate that setup is complete. By default,
* proposal setup is automatically completed after their deals are finalized. Contact your Technical
* Account Manager to opt in. Buyers can call this method when the proposal has been finalized, and
* all the required creatives have been uploaded using the Creatives API. This call updates the
* `is_setup_completed` field on the deals in the proposal, and notifies the seller. The server then
* advances the revision number of the most recent proposal. To mark an individual deal as ready to
* serve, call `buyers.finalizedDeals.setReadyToServe` in the Marketplace API.
*
* Create a request for the method "proposals.completeSetup".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link CompleteSetup#execute()} method to invoke the remote
* operation.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to mark as setup completed.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.CompleteSetupRequest}
* @return the request
*/
public CompleteSetup completeSetup(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.CompleteSetupRequest content) throws java.io.IOException {
CompleteSetup result = new CompleteSetup(accountId, proposalId, content);
initialize(result);
return result;
}
public class CompleteSetup extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/proposals/{proposalId}:completeSetup";
/**
* You can opt-in to manually update proposals to indicate that setup is complete. By default,
* proposal setup is automatically completed after their deals are finalized. Contact your
* Technical Account Manager to opt in. Buyers can call this method when the proposal has been
* finalized, and all the required creatives have been uploaded using the Creatives API. This call
* updates the `is_setup_completed` field on the deals in the proposal, and notifies the seller.
* The server then advances the revision number of the most recent proposal. To mark an individual
* deal as ready to serve, call `buyers.finalizedDeals.setReadyToServe` in the Marketplace API.
*
* Create a request for the method "proposals.completeSetup".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link CompleteSetup#execute()} method to invoke the remote
* operation. {@link CompleteSetup#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to mark as setup completed.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.CompleteSetupRequest}
* @since 1.13
*/
protected CompleteSetup(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.CompleteSetupRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.proposalId = com.google.api.client.util.Preconditions.checkNotNull(proposalId, "Required parameter proposalId must be specified.");
}
@Override
public CompleteSetup set$Xgafv(java.lang.String $Xgafv) {
return (CompleteSetup) super.set$Xgafv($Xgafv);
}
@Override
public CompleteSetup setAccessToken(java.lang.String accessToken) {
return (CompleteSetup) super.setAccessToken(accessToken);
}
@Override
public CompleteSetup setAlt(java.lang.String alt) {
return (CompleteSetup) super.setAlt(alt);
}
@Override
public CompleteSetup setCallback(java.lang.String callback) {
return (CompleteSetup) super.setCallback(callback);
}
@Override
public CompleteSetup setFields(java.lang.String fields) {
return (CompleteSetup) super.setFields(fields);
}
@Override
public CompleteSetup setKey(java.lang.String key) {
return (CompleteSetup) super.setKey(key);
}
@Override
public CompleteSetup setOauthToken(java.lang.String oauthToken) {
return (CompleteSetup) super.setOauthToken(oauthToken);
}
@Override
public CompleteSetup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CompleteSetup) super.setPrettyPrint(prettyPrint);
}
@Override
public CompleteSetup setQuotaUser(java.lang.String quotaUser) {
return (CompleteSetup) super.setQuotaUser(quotaUser);
}
@Override
public CompleteSetup setUploadType(java.lang.String uploadType) {
return (CompleteSetup) super.setUploadType(uploadType);
}
@Override
public CompleteSetup setUploadProtocol(java.lang.String uploadProtocol) {
return (CompleteSetup) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public CompleteSetup setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The ID of the proposal to mark as setup completed. */
@com.google.api.client.util.Key
private java.lang.String proposalId;
/** The ID of the proposal to mark as setup completed.
*/
public java.lang.String getProposalId() {
return proposalId;
}
/** The ID of the proposal to mark as setup completed. */
public CompleteSetup setProposalId(java.lang.String proposalId) {
this.proposalId = proposalId;
return this;
}
@Override
public CompleteSetup set(String parameterName, Object value) {
return (CompleteSetup) super.set(parameterName, value);
}
}
/**
* Create the given proposal. Each created proposal and any deals it contains are assigned a unique
* ID by the server.
*
* Create a request for the method "proposals.create".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal}
* @return the request
*/
public Create create(java.lang.String accountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal content) throws java.io.IOException {
Create result = new Create(accountId, content);
initialize(result);
return result;
}
public class Create extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/proposals";
/**
* Create the given proposal. Each created proposal and any deals it contains are assigned a
* unique ID by the server.
*
* Create a request for the method "proposals.create".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal}
* @since 1.13
*/
protected Create(java.lang.String accountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public Create setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets a proposal given its ID. The proposal is returned at its head revision.
*
* Create a request for the method "proposals.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @param proposalId The unique ID of the proposal
* @return the request
*/
public Get get(java.lang.String accountId, java.lang.String proposalId) throws java.io.IOException {
Get result = new Get(accountId, proposalId);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/proposals/{proposalId}";
/**
* Gets a proposal given its ID. The proposal is returned at its head revision.
*
* Create a request for the method "proposals.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param proposalId The unique ID of the proposal
* @since 1.13
*/
protected Get(java.lang.String accountId, java.lang.String proposalId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.proposalId = com.google.api.client.util.Preconditions.checkNotNull(proposalId, "Required parameter proposalId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public Get setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The unique ID of the proposal */
@com.google.api.client.util.Key
private java.lang.String proposalId;
/** The unique ID of the proposal
*/
public java.lang.String getProposalId() {
return proposalId;
}
/** The unique ID of the proposal */
public Get setProposalId(java.lang.String proposalId) {
this.proposalId = proposalId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List proposals. A filter expression (PQL query) may be specified to filter the results. To
* retrieve all finalized proposals, regardless if a proposal is being renegotiated, see the
* FinalizedProposals resource. Note that Bidder/ChildSeat relationships differ from the usual
* behavior. A Bidder account can only see its child seats' proposals by specifying the ChildSeat's
* accountId in the request path.
*
* Create a request for the method "proposals.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @return the request
*/
public List list(java.lang.String accountId) throws java.io.IOException {
List result = new List(accountId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/proposals";
/**
* List proposals. A filter expression (PQL query) may be specified to filter the results. To
* retrieve all finalized proposals, regardless if a proposal is being renegotiated, see the
* FinalizedProposals resource. Note that Bidder/ChildSeat relationships differ from the usual
* behavior. A Bidder account can only see its child seats' proposals by specifying the
* ChildSeat's accountId in the request path.
*
* Create a request for the method "proposals.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @since 1.13
*/
protected List(java.lang.String accountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListProposalsResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public List setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/**
* An optional PQL filter query used to query for proposals. Nested repeated fields, such as
* proposal.deals.targetingCriterion, cannot be filtered.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** An optional PQL filter query used to query for proposals. Nested repeated fields, such as
proposal.deals.targetingCriterion, cannot be filtered.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* An optional PQL filter query used to query for proposals. Nested repeated fields, such as
* proposal.deals.targetingCriterion, cannot be filtered.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Syntax the filter is written in. Current implementation defaults to PQL but in the future
* it will be LIST_FILTER.
*/
@com.google.api.client.util.Key
private java.lang.String filterSyntax;
/** Syntax the filter is written in. Current implementation defaults to PQL but in the future it will
be LIST_FILTER.
*/
public java.lang.String getFilterSyntax() {
return filterSyntax;
}
/**
* Syntax the filter is written in. Current implementation defaults to PQL but in the future
* it will be LIST_FILTER.
*/
public List setFilterSyntax(java.lang.String filterSyntax) {
this.filterSyntax = filterSyntax;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If unspecified,
* the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If unspecified,
* the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The page token as returned from ListProposalsResponse. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The page token as returned from ListProposalsResponse.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The page token as returned from ListProposalsResponse. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Update the given proposal to pause serving. This method will set the
* `DealServingMetadata.DealPauseStatus.has_buyer_paused` bit to true for all deals in the proposal.
* It is a no-op to pause an already-paused proposal. It is an error to call PauseProposal for a
* proposal that is not finalized or renegotiating.
*
* Create a request for the method "proposals.pause".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Pause#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to pause.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.PauseProposalRequest}
* @return the request
*/
public Pause pause(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.PauseProposalRequest content) throws java.io.IOException {
Pause result = new Pause(accountId, proposalId, content);
initialize(result);
return result;
}
public class Pause extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/proposals/{proposalId}:pause";
/**
* Update the given proposal to pause serving. This method will set the
* `DealServingMetadata.DealPauseStatus.has_buyer_paused` bit to true for all deals in the
* proposal. It is a no-op to pause an already-paused proposal. It is an error to call
* PauseProposal for a proposal that is not finalized or renegotiating.
*
* Create a request for the method "proposals.pause".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Pause#execute()} method to invoke the remote operation.
* {@link
* Pause#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to pause.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.PauseProposalRequest}
* @since 1.13
*/
protected Pause(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.PauseProposalRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.proposalId = com.google.api.client.util.Preconditions.checkNotNull(proposalId, "Required parameter proposalId must be specified.");
}
@Override
public Pause set$Xgafv(java.lang.String $Xgafv) {
return (Pause) super.set$Xgafv($Xgafv);
}
@Override
public Pause setAccessToken(java.lang.String accessToken) {
return (Pause) super.setAccessToken(accessToken);
}
@Override
public Pause setAlt(java.lang.String alt) {
return (Pause) super.setAlt(alt);
}
@Override
public Pause setCallback(java.lang.String callback) {
return (Pause) super.setCallback(callback);
}
@Override
public Pause setFields(java.lang.String fields) {
return (Pause) super.setFields(fields);
}
@Override
public Pause setKey(java.lang.String key) {
return (Pause) super.setKey(key);
}
@Override
public Pause setOauthToken(java.lang.String oauthToken) {
return (Pause) super.setOauthToken(oauthToken);
}
@Override
public Pause setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Pause) super.setPrettyPrint(prettyPrint);
}
@Override
public Pause setQuotaUser(java.lang.String quotaUser) {
return (Pause) super.setQuotaUser(quotaUser);
}
@Override
public Pause setUploadType(java.lang.String uploadType) {
return (Pause) super.setUploadType(uploadType);
}
@Override
public Pause setUploadProtocol(java.lang.String uploadProtocol) {
return (Pause) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public Pause setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The ID of the proposal to pause. */
@com.google.api.client.util.Key
private java.lang.String proposalId;
/** The ID of the proposal to pause.
*/
public java.lang.String getProposalId() {
return proposalId;
}
/** The ID of the proposal to pause. */
public Pause setProposalId(java.lang.String proposalId) {
this.proposalId = proposalId;
return this;
}
@Override
public Pause set(String parameterName, Object value) {
return (Pause) super.set(parameterName, value);
}
}
/**
* Update the given proposal to resume serving. This method will set the
* `DealServingMetadata.DealPauseStatus.has_buyer_paused` bit to false for all deals in the
* proposal. Note that if the `has_seller_paused` bit is also set, serving will not resume until the
* seller also resumes. It is a no-op to resume an already-running proposal. It is an error to call
* ResumeProposal for a proposal that is not finalized or renegotiating.
*
* Create a request for the method "proposals.resume".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Resume#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to resume.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ResumeProposalRequest}
* @return the request
*/
public Resume resume(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.ResumeProposalRequest content) throws java.io.IOException {
Resume result = new Resume(accountId, proposalId, content);
initialize(result);
return result;
}
public class Resume extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/proposals/{proposalId}:resume";
/**
* Update the given proposal to resume serving. This method will set the
* `DealServingMetadata.DealPauseStatus.has_buyer_paused` bit to false for all deals in the
* proposal. Note that if the `has_seller_paused` bit is also set, serving will not resume until
* the seller also resumes. It is a no-op to resume an already-running proposal. It is an error to
* call ResumeProposal for a proposal that is not finalized or renegotiating.
*
* Create a request for the method "proposals.resume".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Resume#execute()} method to invoke the remote operation.
* {@link
* Resume#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param proposalId The ID of the proposal to resume.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ResumeProposalRequest}
* @since 1.13
*/
protected Resume(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.ResumeProposalRequest content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.proposalId = com.google.api.client.util.Preconditions.checkNotNull(proposalId, "Required parameter proposalId must be specified.");
}
@Override
public Resume set$Xgafv(java.lang.String $Xgafv) {
return (Resume) super.set$Xgafv($Xgafv);
}
@Override
public Resume setAccessToken(java.lang.String accessToken) {
return (Resume) super.setAccessToken(accessToken);
}
@Override
public Resume setAlt(java.lang.String alt) {
return (Resume) super.setAlt(alt);
}
@Override
public Resume setCallback(java.lang.String callback) {
return (Resume) super.setCallback(callback);
}
@Override
public Resume setFields(java.lang.String fields) {
return (Resume) super.setFields(fields);
}
@Override
public Resume setKey(java.lang.String key) {
return (Resume) super.setKey(key);
}
@Override
public Resume setOauthToken(java.lang.String oauthToken) {
return (Resume) super.setOauthToken(oauthToken);
}
@Override
public Resume setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Resume) super.setPrettyPrint(prettyPrint);
}
@Override
public Resume setQuotaUser(java.lang.String quotaUser) {
return (Resume) super.setQuotaUser(quotaUser);
}
@Override
public Resume setUploadType(java.lang.String uploadType) {
return (Resume) super.setUploadType(uploadType);
}
@Override
public Resume setUploadProtocol(java.lang.String uploadProtocol) {
return (Resume) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public Resume setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The ID of the proposal to resume. */
@com.google.api.client.util.Key
private java.lang.String proposalId;
/** The ID of the proposal to resume.
*/
public java.lang.String getProposalId() {
return proposalId;
}
/** The ID of the proposal to resume. */
public Resume setProposalId(java.lang.String proposalId) {
this.proposalId = proposalId;
return this;
}
@Override
public Resume set(String parameterName, Object value) {
return (Resume) super.set(parameterName, value);
}
}
/**
* Update the given proposal at the client known revision number. If the server revision has
* advanced since the passed-in `proposal.proposal_revision`, an `ABORTED` error message will be
* returned. Only the buyer-modifiable fields of the proposal will be updated. Note that the deals
* in the proposal will be updated to match the passed-in copy. If a passed-in deal does not have a
* `deal_id`, the server will assign a new unique ID and create the deal. If passed-in deal has a
* `deal_id`, it will be updated to match the passed-in copy. Any existing deals not present in the
* passed-in proposal will be deleted. It is an error to pass in a deal with a `deal_id` not present
* at head.
*
* Create a request for the method "proposals.update".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @param proposalId The unique ID of the proposal.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal}
* @return the request
*/
public Update update(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal content) throws java.io.IOException {
Update result = new Update(accountId, proposalId, content);
initialize(result);
return result;
}
public class Update extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/proposals/{proposalId}";
/**
* Update the given proposal at the client known revision number. If the server revision has
* advanced since the passed-in `proposal.proposal_revision`, an `ABORTED` error message will be
* returned. Only the buyer-modifiable fields of the proposal will be updated. Note that the deals
* in the proposal will be updated to match the passed-in copy. If a passed-in deal does not have
* a `deal_id`, the server will assign a new unique ID and create the deal. If passed-in deal has
* a `deal_id`, it will be updated to match the passed-in copy. Any existing deals not present in
* the passed-in proposal will be deleted. It is an error to pass in a deal with a `deal_id` not
* present at head.
*
* Create a request for the method "proposals.update".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param proposalId The unique ID of the proposal.
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal}
* @since 1.13
*/
protected Update(java.lang.String accountId, java.lang.String proposalId, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal content) {
super(AdExchangeBuyerII.this, "PUT", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Proposal.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.proposalId = com.google.api.client.util.Preconditions.checkNotNull(proposalId, "Required parameter proposalId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public Update setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The unique ID of the proposal. */
@com.google.api.client.util.Key
private java.lang.String proposalId;
/** The unique ID of the proposal.
*/
public java.lang.String getProposalId() {
return proposalId;
}
/** The unique ID of the proposal. */
public Update setProposalId(java.lang.String proposalId) {
this.proposalId = proposalId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the PublisherProfiles collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.PublisherProfiles.List request = adexchangebuyer2.publisherProfiles().list(parameters ...)}
*
*
* @return the resource collection
*/
public PublisherProfiles publisherProfiles() {
return new PublisherProfiles();
}
/**
* The "publisherProfiles" collection of methods.
*/
public class PublisherProfiles {
/**
* Gets the requested publisher profile by id.
*
* Create a request for the method "publisherProfiles.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @param publisherProfileId The id for the publisher profile to get.
* @return the request
*/
public Get get(java.lang.String accountId, java.lang.String publisherProfileId) throws java.io.IOException {
Get result = new Get(accountId, publisherProfileId);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/publisherProfiles/{publisherProfileId}";
/**
* Gets the requested publisher profile by id.
*
* Create a request for the method "publisherProfiles.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @param publisherProfileId The id for the publisher profile to get.
* @since 1.13
*/
protected Get(java.lang.String accountId, java.lang.String publisherProfileId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.PublisherProfile.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.publisherProfileId = com.google.api.client.util.Preconditions.checkNotNull(publisherProfileId, "Required parameter publisherProfileId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public Get setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** The id for the publisher profile to get. */
@com.google.api.client.util.Key
private java.lang.String publisherProfileId;
/** The id for the publisher profile to get.
*/
public java.lang.String getPublisherProfileId() {
return publisherProfileId;
}
/** The id for the publisher profile to get. */
public Get setPublisherProfileId(java.lang.String publisherProfileId) {
this.publisherProfileId = publisherProfileId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List all publisher profiles visible to the buyer
*
* Create a request for the method "publisherProfiles.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId Account ID of the buyer.
* @return the request
*/
public List list(java.lang.String accountId) throws java.io.IOException {
List result = new List(accountId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/publisherProfiles";
/**
* List all publisher profiles visible to the buyer
*
* Create a request for the method "publisherProfiles.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Account ID of the buyer.
* @since 1.13
*/
protected List(java.lang.String accountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListPublisherProfilesResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Account ID of the buyer. */
@com.google.api.client.util.Key
private java.lang.String accountId;
/** Account ID of the buyer.
*/
public java.lang.String getAccountId() {
return accountId;
}
/** Account ID of the buyer. */
public List setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/** Specify the number of results to include per page. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Specify the number of results to include per page.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Specify the number of results to include per page. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The page token as return from ListPublisherProfilesResponse. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The page token as return from ListPublisherProfilesResponse.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The page token as return from ListPublisherProfilesResponse. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Bidders collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Bidders.List request = adexchangebuyer2.bidders().list(parameters ...)}
*
*
* @return the resource collection
*/
public Bidders bidders() {
return new Bidders();
}
/**
* The "bidders" collection of methods.
*/
public class Bidders {
/**
* An accessor for creating requests from the Accounts collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Accounts.List request = adexchangebuyer2.accounts().list(parameters ...)}
*
*
* @return the resource collection
*/
public Accounts accounts() {
return new Accounts();
}
/**
* The "accounts" collection of methods.
*/
public class Accounts {
/**
* An accessor for creating requests from the FilterSets collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.FilterSets.List request = adexchangebuyer2.filterSets().list(parameters ...)}
*
*
* @return the resource collection
*/
public FilterSets filterSets() {
return new FilterSets();
}
/**
* The "filterSets" collection of methods.
*/
public class FilterSets {
/**
* Creates the specified filter set for the account with the given account ID.
*
* Create a request for the method "filterSets.create".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param ownerName Name of the owner (bidder or account) of the filter set to be created. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet}
* @return the request
*/
public Create create(java.lang.String ownerName, com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet content) throws java.io.IOException {
Create result = new Create(ownerName, content);
initialize(result);
return result;
}
public class Create extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+ownerName}/filterSets";
private final java.util.regex.Pattern OWNER_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+$");
/**
* Creates the specified filter set for the account with the given account ID.
*
* Create a request for the method "filterSets.create".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param ownerName Name of the owner (bidder or account) of the filter set to be created. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet}
* @since 1.13
*/
protected Create(java.lang.String ownerName, com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet.class);
this.ownerName = com.google.api.client.util.Preconditions.checkNotNull(ownerName, "Required parameter ownerName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OWNER_NAME_PATTERN.matcher(ownerName).matches(),
"Parameter ownerName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the owner (bidder or account) of the filter set to be created. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123` - For an account-level
* filter set for the buyer account representing bidder 123: `bidders/123/accounts/123` -
* For an account-level filter set for the child seat buyer account 456 whose bidder is
* 123: `bidders/123/accounts/456`
*/
@com.google.api.client.util.Key
private java.lang.String ownerName;
/** Name of the owner (bidder or account) of the filter set to be created. For example: - For a bidder-
level filter set for bidder 123: `bidders/123` - For an account-level filter set for the buyer
account representing bidder 123: `bidders/123/accounts/123` - For an account-level filter set for
the child seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456`
*/
public java.lang.String getOwnerName() {
return ownerName;
}
/**
* Name of the owner (bidder or account) of the filter set to be created. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123` - For an account-level
* filter set for the buyer account representing bidder 123: `bidders/123/accounts/123` -
* For an account-level filter set for the child seat buyer account 456 whose bidder is
* 123: `bidders/123/accounts/456`
*/
public Create setOwnerName(java.lang.String ownerName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OWNER_NAME_PATTERN.matcher(ownerName).matches(),
"Parameter ownerName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+$");
}
this.ownerName = ownerName;
return this;
}
/**
* Whether the filter set is transient, or should be persisted indefinitely. By default,
* filter sets are not transient. If transient, it will be available for at least 1 hour
* after creation.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isTransient;
/** Whether the filter set is transient, or should be persisted indefinitely. By default, filter sets
are not transient. If transient, it will be available for at least 1 hour after creation.
*/
public java.lang.Boolean getIsTransient() {
return isTransient;
}
/**
* Whether the filter set is transient, or should be persisted indefinitely. By default,
* filter sets are not transient. If transient, it will be available for at least 1 hour
* after creation.
*/
public Create setIsTransient(java.lang.Boolean isTransient) {
this.isTransient = isTransient;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the requested filter set from the account with the given account ID.
*
* Create a request for the method "filterSets.delete".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Full name of the resource to delete. For example: - For a bidder-level filter set for bidder 123:
* `bidders/123/filterSets/abc` - For an account-level filter set for the buyer account
* representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* Deletes the requested filter set from the account with the given account ID.
*
* Create a request for the method "filterSets.delete".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Full name of the resource to delete. For example: - For a bidder-level filter set for bidder 123:
* `bidders/123/filterSets/abc` - For an account-level filter set for the buyer account
* representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AdExchangeBuyerII.this, "DELETE", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Full name of the resource to delete. For example: - For a bidder-level filter set for
* bidder 123: `bidders/123/filterSets/abc` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
* an account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Full name of the resource to delete. For example: - For a bidder-level filter set for bidder 123:
`bidders/123/filterSets/abc` - For an account-level filter set for the buyer account representing
bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
child seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getName() {
return name;
}
/**
* Full name of the resource to delete. For example: - For a bidder-level filter set for
* bidder 123: `bidders/123/filterSets/abc` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
* an account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the requested filter set for the account with the given account ID.
*
* Create a request for the method "filterSets.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Full name of the resource being requested. For example: - For a bidder-level filter set for bidder
* 123: `bidders/123/filterSets/abc` - For an account-level filter set for the buyer account
* representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* Retrieves the requested filter set for the account with the given account ID.
*
* Create a request for the method "filterSets.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Full name of the resource being requested. For example: - For a bidder-level filter set for bidder
* 123: `bidders/123/filterSets/abc` - For an account-level filter set for the buyer account
* representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Full name of the resource being requested. For example: - For a bidder-level filter set
* for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
* an account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Full name of the resource being requested. For example: - For a bidder-level filter set for bidder
123: `bidders/123/filterSets/abc` - For an account-level filter set for the buyer account
representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level filter
set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getName() {
return name;
}
/**
* Full name of the resource being requested. For example: - For a bidder-level filter set
* for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
* an account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all filter sets for the account with the given account ID.
*
* Create a request for the method "filterSets.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param ownerName Name of the owner (bidder or account) of the filter sets to be listed. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
* @return the request
*/
public List list(java.lang.String ownerName) throws java.io.IOException {
List result = new List(ownerName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+ownerName}/filterSets";
private final java.util.regex.Pattern OWNER_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+$");
/**
* Lists all filter sets for the account with the given account ID.
*
* Create a request for the method "filterSets.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param ownerName Name of the owner (bidder or account) of the filter sets to be listed. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
* @since 1.13
*/
protected List(java.lang.String ownerName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListFilterSetsResponse.class);
this.ownerName = com.google.api.client.util.Preconditions.checkNotNull(ownerName, "Required parameter ownerName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OWNER_NAME_PATTERN.matcher(ownerName).matches(),
"Parameter ownerName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the owner (bidder or account) of the filter sets to be listed. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123` - For an account-level
* filter set for the buyer account representing bidder 123: `bidders/123/accounts/123` -
* For an account-level filter set for the child seat buyer account 456 whose bidder is
* 123: `bidders/123/accounts/456`
*/
@com.google.api.client.util.Key
private java.lang.String ownerName;
/** Name of the owner (bidder or account) of the filter sets to be listed. For example: - For a bidder-
level filter set for bidder 123: `bidders/123` - For an account-level filter set for the buyer
account representing bidder 123: `bidders/123/accounts/123` - For an account-level filter set for
the child seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456`
*/
public java.lang.String getOwnerName() {
return ownerName;
}
/**
* Name of the owner (bidder or account) of the filter sets to be listed. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123` - For an account-level
* filter set for the buyer account representing bidder 123: `bidders/123/accounts/123` -
* For an account-level filter set for the child seat buyer account 456 whose bidder is
* 123: `bidders/123/accounts/456`
*/
public List setOwnerName(java.lang.String ownerName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OWNER_NAME_PATTERN.matcher(ownerName).matches(),
"Parameter ownerName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+$");
}
this.ownerName = ownerName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListFilterSetsResponse.nextPageToken returned from the previous call to the
* accounts.filterSets.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListFilterSetsResponse.nextPageToken returned from the previous call to the
accounts.filterSets.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListFilterSetsResponse.nextPageToken returned from the previous call to the
* accounts.filterSets.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the BidMetrics collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.BidMetrics.List request = adexchangebuyer2.bidMetrics().list(parameters ...)}
*
*
* @return the resource collection
*/
public BidMetrics bidMetrics() {
return new BidMetrics();
}
/**
* The "bidMetrics" collection of methods.
*/
public class BidMetrics {
/**
* Lists all metrics that are measured in terms of number of bids.
*
* Create a request for the method "bidMetrics.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/bidMetrics";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* Lists all metrics that are measured in terms of number of bids.
*
* Create a request for the method "bidMetrics.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListBidMetricsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListBidMetricsResponse.nextPageToken returned from the previous call to
* the bidMetrics.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListBidMetricsResponse.nextPageToken returned from the previous call to the bidMetrics.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListBidMetricsResponse.nextPageToken returned from the previous call to
* the bidMetrics.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the BidResponseErrors collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.BidResponseErrors.List request = adexchangebuyer2.bidResponseErrors().list(parameters ...)}
*
*
* @return the resource collection
*/
public BidResponseErrors bidResponseErrors() {
return new BidResponseErrors();
}
/**
* The "bidResponseErrors" collection of methods.
*/
public class BidResponseErrors {
/**
* List all errors that occurred in bid responses, with the number of bid responses affected for
* each reason.
*
* Create a request for the method "bidResponseErrors.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/bidResponseErrors";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* List all errors that occurred in bid responses, with the number of bid responses affected for
* each reason.
*
* Create a request for the method "bidResponseErrors.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListBidResponseErrorsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListBidResponseErrorsResponse.nextPageToken returned from the previous
* call to the bidResponseErrors.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListBidResponseErrorsResponse.nextPageToken returned from the previous call to the
bidResponseErrors.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListBidResponseErrorsResponse.nextPageToken returned from the previous
* call to the bidResponseErrors.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the BidResponsesWithoutBids collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.BidResponsesWithoutBids.List request = adexchangebuyer2.bidResponsesWithoutBids().list(parameters ...)}
*
*
* @return the resource collection
*/
public BidResponsesWithoutBids bidResponsesWithoutBids() {
return new BidResponsesWithoutBids();
}
/**
* The "bidResponsesWithoutBids" collection of methods.
*/
public class BidResponsesWithoutBids {
/**
* List all reasons for which bid responses were considered to have no applicable bids, with the
* number of bid responses affected for each reason.
*
* Create a request for the method "bidResponsesWithoutBids.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/bidResponsesWithoutBids";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* List all reasons for which bid responses were considered to have no applicable bids, with the
* number of bid responses affected for each reason.
*
* Create a request for the method "bidResponsesWithoutBids.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListBidResponsesWithoutBidsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListBidResponsesWithoutBidsResponse.nextPageToken returned from the
* previous call to the bidResponsesWithoutBids.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListBidResponsesWithoutBidsResponse.nextPageToken returned from the previous call to the
bidResponsesWithoutBids.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListBidResponsesWithoutBidsResponse.nextPageToken returned from the
* previous call to the bidResponsesWithoutBids.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the FilteredBidRequests collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.FilteredBidRequests.List request = adexchangebuyer2.filteredBidRequests().list(parameters ...)}
*
*
* @return the resource collection
*/
public FilteredBidRequests filteredBidRequests() {
return new FilteredBidRequests();
}
/**
* The "filteredBidRequests" collection of methods.
*/
public class FilteredBidRequests {
/**
* List all reasons that caused a bid request not to be sent for an impression, with the number of
* bid requests not sent for each reason.
*
* Create a request for the method "filteredBidRequests.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/filteredBidRequests";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* List all reasons that caused a bid request not to be sent for an impression, with the number of
* bid requests not sent for each reason.
*
* Create a request for the method "filteredBidRequests.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListFilteredBidRequestsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListFilteredBidRequestsResponse.nextPageToken returned from the previous
* call to the filteredBidRequests.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListFilteredBidRequestsResponse.nextPageToken returned from the previous call to the
filteredBidRequests.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListFilteredBidRequestsResponse.nextPageToken returned from the previous
* call to the filteredBidRequests.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the FilteredBids collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.FilteredBids.List request = adexchangebuyer2.filteredBids().list(parameters ...)}
*
*
* @return the resource collection
*/
public FilteredBids filteredBids() {
return new FilteredBids();
}
/**
* The "filteredBids" collection of methods.
*/
public class FilteredBids {
/**
* List all reasons for which bids were filtered, with the number of bids filtered for each reason.
*
* Create a request for the method "filteredBids.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/filteredBids";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* List all reasons for which bids were filtered, with the number of bids filtered for each
* reason.
*
* Create a request for the method "filteredBids.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListFilteredBidsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListFilteredBidsResponse.nextPageToken returned from the previous call
* to the filteredBids.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListFilteredBidsResponse.nextPageToken returned from the previous call to the filteredBids.list
method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListFilteredBidsResponse.nextPageToken returned from the previous call
* to the filteredBids.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Creatives collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Creatives.List request = adexchangebuyer2.creatives().list(parameters ...)}
*
*
* @return the resource collection
*/
public Creatives creatives() {
return new Creatives();
}
/**
* The "creatives" collection of methods.
*/
public class Creatives {
/**
* List all creatives associated with a specific reason for which bids were filtered, with the
* number of bids filtered for each creative.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @param creativeStatusId The ID of the creative status for which to retrieve a breakdown by creative. See [creative-status-
* codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-
* codes).
* @return the request
*/
public List list(java.lang.String filterSetName, java.lang.Integer creativeStatusId) throws java.io.IOException {
List result = new List(filterSetName, creativeStatusId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/filteredBids/{creativeStatusId}/creatives";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* List all creatives associated with a specific reason for which bids were filtered, with the
* number of bids filtered for each creative.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @param creativeStatusId The ID of the creative status for which to retrieve a breakdown by creative. See [creative-status-
* codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-
* codes).
* @since 1.13
*/
protected List(java.lang.String filterSetName, java.lang.Integer creativeStatusId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListCreativeStatusBreakdownByCreativeResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.creativeStatusId = com.google.api.client.util.Preconditions.checkNotNull(creativeStatusId, "Required parameter creativeStatusId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For
* example: - For a bidder-level filter set for bidder 123:
* `bidders/123/filterSets/abc` - For an account-level filter set for the buyer
* account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For
* example: - For a bidder-level filter set for bidder 123:
* `bidders/123/filterSets/abc` - For an account-level filter set for the buyer
* account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* The ID of the creative status for which to retrieve a breakdown by creative. See
* [creative-status-codes](https://developers.google.com/authorized-
* buyers/rtb/downloads/creative-status-codes).
*/
@com.google.api.client.util.Key
private java.lang.Integer creativeStatusId;
/** The ID of the creative status for which to retrieve a breakdown by creative. See [creative-status-
codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-codes).
*/
public java.lang.Integer getCreativeStatusId() {
return creativeStatusId;
}
/**
* The ID of the creative status for which to retrieve a breakdown by creative. See
* [creative-status-codes](https://developers.google.com/authorized-
* buyers/rtb/downloads/creative-status-codes).
*/
public List setCreativeStatusId(java.lang.Integer creativeStatusId) {
this.creativeStatusId = creativeStatusId;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListCreativeStatusBreakdownByCreativeResponse.nextPageToken returned
* from the previous call to the filteredBids.creatives.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListCreativeStatusBreakdownByCreativeResponse.nextPageToken returned from the previous call to the
filteredBids.creatives.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListCreativeStatusBreakdownByCreativeResponse.nextPageToken returned
* from the previous call to the filteredBids.creatives.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Details collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Details.List request = adexchangebuyer2.details().list(parameters ...)}
*
*
* @return the resource collection
*/
public Details details() {
return new Details();
}
/**
* The "details" collection of methods.
*/
public class Details {
/**
* List all details associated with a specific reason for which bids were filtered, with the number
* of bids filtered for each detail.
*
* Create a request for the method "details.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @param creativeStatusId The ID of the creative status for which to retrieve a breakdown by detail. See [creative-status-
* codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-
* codes). Details are only available for statuses 10, 14, 15, 17, 18, 19, 86, and 87.
* @return the request
*/
public List list(java.lang.String filterSetName, java.lang.Integer creativeStatusId) throws java.io.IOException {
List result = new List(filterSetName, creativeStatusId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/filteredBids/{creativeStatusId}/details";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* List all details associated with a specific reason for which bids were filtered, with the
* number of bids filtered for each detail.
*
* Create a request for the method "details.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @param creativeStatusId The ID of the creative status for which to retrieve a breakdown by detail. See [creative-status-
* codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-
* codes). Details are only available for statuses 10, 14, 15, 17, 18, 19, 86, and 87.
* @since 1.13
*/
protected List(java.lang.String filterSetName, java.lang.Integer creativeStatusId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListCreativeStatusBreakdownByDetailResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.creativeStatusId = com.google.api.client.util.Preconditions.checkNotNull(creativeStatusId, "Required parameter creativeStatusId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For
* example: - For a bidder-level filter set for bidder 123:
* `bidders/123/filterSets/abc` - For an account-level filter set for the buyer
* account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For
* example: - For a bidder-level filter set for bidder 123:
* `bidders/123/filterSets/abc` - For an account-level filter set for the buyer
* account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* The ID of the creative status for which to retrieve a breakdown by detail. See
* [creative-status-codes](https://developers.google.com/authorized-
* buyers/rtb/downloads/creative-status-codes). Details are only available for
* statuses 10, 14, 15, 17, 18, 19, 86, and 87.
*/
@com.google.api.client.util.Key
private java.lang.Integer creativeStatusId;
/** The ID of the creative status for which to retrieve a breakdown by detail. See [creative-status-
codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-codes).
Details are only available for statuses 10, 14, 15, 17, 18, 19, 86, and 87.
*/
public java.lang.Integer getCreativeStatusId() {
return creativeStatusId;
}
/**
* The ID of the creative status for which to retrieve a breakdown by detail. See
* [creative-status-codes](https://developers.google.com/authorized-
* buyers/rtb/downloads/creative-status-codes). Details are only available for
* statuses 10, 14, 15, 17, 18, 19, 86, and 87.
*/
public List setCreativeStatusId(java.lang.Integer creativeStatusId) {
this.creativeStatusId = creativeStatusId;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListCreativeStatusBreakdownByDetailResponse.nextPageToken returned
* from the previous call to the filteredBids.details.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListCreativeStatusBreakdownByDetailResponse.nextPageToken returned from the previous call to the
filteredBids.details.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListCreativeStatusBreakdownByDetailResponse.nextPageToken returned
* from the previous call to the filteredBids.details.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the ImpressionMetrics collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.ImpressionMetrics.List request = adexchangebuyer2.impressionMetrics().list(parameters ...)}
*
*
* @return the resource collection
*/
public ImpressionMetrics impressionMetrics() {
return new ImpressionMetrics();
}
/**
* The "impressionMetrics" collection of methods.
*/
public class ImpressionMetrics {
/**
* Lists all metrics that are measured in terms of number of impressions.
*
* Create a request for the method "impressionMetrics.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/impressionMetrics";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* Lists all metrics that are measured in terms of number of impressions.
*
* Create a request for the method "impressionMetrics.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListImpressionMetricsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListImpressionMetricsResponse.nextPageToken returned from the previous
* call to the impressionMetrics.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListImpressionMetricsResponse.nextPageToken returned from the previous call to the
impressionMetrics.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListImpressionMetricsResponse.nextPageToken returned from the previous
* call to the impressionMetrics.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the LosingBids collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.LosingBids.List request = adexchangebuyer2.losingBids().list(parameters ...)}
*
*
* @return the resource collection
*/
public LosingBids losingBids() {
return new LosingBids();
}
/**
* The "losingBids" collection of methods.
*/
public class LosingBids {
/**
* List all reasons for which bids lost in the auction, with the number of bids that lost for each
* reason.
*
* Create a request for the method "losingBids.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/losingBids";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* List all reasons for which bids lost in the auction, with the number of bids that lost for each
* reason.
*
* Create a request for the method "losingBids.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListLosingBidsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListLosingBidsResponse.nextPageToken returned from the previous call to
* the losingBids.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListLosingBidsResponse.nextPageToken returned from the previous call to the losingBids.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListLosingBidsResponse.nextPageToken returned from the previous call to
* the losingBids.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the NonBillableWinningBids collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.NonBillableWinningBids.List request = adexchangebuyer2.nonBillableWinningBids().list(parameters ...)}
*
*
* @return the resource collection
*/
public NonBillableWinningBids nonBillableWinningBids() {
return new NonBillableWinningBids();
}
/**
* The "nonBillableWinningBids" collection of methods.
*/
public class NonBillableWinningBids {
/**
* List all reasons for which winning bids were not billable, with the number of bids not billed for
* each reason.
*
* Create a request for the method "nonBillableWinningBids.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/nonBillableWinningBids";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
/**
* List all reasons for which winning bids were not billable, with the number of bids not billed
* for each reason.
*
* Create a request for the method "nonBillableWinningBids.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListNonBillableWinningBidsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/accounts/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListNonBillableWinningBidsResponse.nextPageToken returned from the
* previous call to the nonBillableWinningBids.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListNonBillableWinningBidsResponse.nextPageToken returned from the previous call to the
nonBillableWinningBids.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListNonBillableWinningBidsResponse.nextPageToken returned from the
* previous call to the nonBillableWinningBids.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the FilterSets collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.FilterSets.List request = adexchangebuyer2.filterSets().list(parameters ...)}
*
*
* @return the resource collection
*/
public FilterSets filterSets() {
return new FilterSets();
}
/**
* The "filterSets" collection of methods.
*/
public class FilterSets {
/**
* Creates the specified filter set for the account with the given account ID.
*
* Create a request for the method "filterSets.create".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param ownerName Name of the owner (bidder or account) of the filter set to be created. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet}
* @return the request
*/
public Create create(java.lang.String ownerName, com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet content) throws java.io.IOException {
Create result = new Create(ownerName, content);
initialize(result);
return result;
}
public class Create extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+ownerName}/filterSets";
private final java.util.regex.Pattern OWNER_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+$");
/**
* Creates the specified filter set for the account with the given account ID.
*
* Create a request for the method "filterSets.create".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param ownerName Name of the owner (bidder or account) of the filter set to be created. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet}
* @since 1.13
*/
protected Create(java.lang.String ownerName, com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet.class);
this.ownerName = com.google.api.client.util.Preconditions.checkNotNull(ownerName, "Required parameter ownerName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OWNER_NAME_PATTERN.matcher(ownerName).matches(),
"Parameter ownerName must conform to the pattern " +
"^bidders/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the owner (bidder or account) of the filter set to be created. For example: - For
* a bidder-level filter set for bidder 123: `bidders/123` - For an account-level filter set
* for the buyer account representing bidder 123: `bidders/123/accounts/123` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
*/
@com.google.api.client.util.Key
private java.lang.String ownerName;
/** Name of the owner (bidder or account) of the filter set to be created. For example: - For a bidder-
level filter set for bidder 123: `bidders/123` - For an account-level filter set for the buyer
account representing bidder 123: `bidders/123/accounts/123` - For an account-level filter set for
the child seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456`
*/
public java.lang.String getOwnerName() {
return ownerName;
}
/**
* Name of the owner (bidder or account) of the filter set to be created. For example: - For
* a bidder-level filter set for bidder 123: `bidders/123` - For an account-level filter set
* for the buyer account representing bidder 123: `bidders/123/accounts/123` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
*/
public Create setOwnerName(java.lang.String ownerName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OWNER_NAME_PATTERN.matcher(ownerName).matches(),
"Parameter ownerName must conform to the pattern " +
"^bidders/[^/]+$");
}
this.ownerName = ownerName;
return this;
}
/**
* Whether the filter set is transient, or should be persisted indefinitely. By default,
* filter sets are not transient. If transient, it will be available for at least 1 hour
* after creation.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isTransient;
/** Whether the filter set is transient, or should be persisted indefinitely. By default, filter sets
are not transient. If transient, it will be available for at least 1 hour after creation.
*/
public java.lang.Boolean getIsTransient() {
return isTransient;
}
/**
* Whether the filter set is transient, or should be persisted indefinitely. By default,
* filter sets are not transient. If transient, it will be available for at least 1 hour
* after creation.
*/
public Create setIsTransient(java.lang.Boolean isTransient) {
this.isTransient = isTransient;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the requested filter set from the account with the given account ID.
*
* Create a request for the method "filterSets.delete".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Full name of the resource to delete. For example: - For a bidder-level filter set for bidder 123:
* `bidders/123/filterSets/abc` - For an account-level filter set for the buyer account
* representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* Deletes the requested filter set from the account with the given account ID.
*
* Create a request for the method "filterSets.delete".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Full name of the resource to delete. For example: - For a bidder-level filter set for bidder 123:
* `bidders/123/filterSets/abc` - For an account-level filter set for the buyer account
* representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AdExchangeBuyerII.this, "DELETE", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Full name of the resource to delete. For example: - For a bidder-level filter set for
* bidder 123: `bidders/123/filterSets/abc` - For an account-level filter set for the buyer
* account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Full name of the resource to delete. For example: - For a bidder-level filter set for bidder 123:
`bidders/123/filterSets/abc` - For an account-level filter set for the buyer account representing
bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
child seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getName() {
return name;
}
/**
* Full name of the resource to delete. For example: - For a bidder-level filter set for
* bidder 123: `bidders/123/filterSets/abc` - For an account-level filter set for the buyer
* account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the requested filter set for the account with the given account ID.
*
* Create a request for the method "filterSets.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Full name of the resource being requested. For example: - For a bidder-level filter set for bidder
* 123: `bidders/123/filterSets/abc` - For an account-level filter set for the buyer account
* representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* Retrieves the requested filter set for the account with the given account ID.
*
* Create a request for the method "filterSets.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Full name of the resource being requested. For example: - For a bidder-level filter set for bidder
* 123: `bidders/123/filterSets/abc` - For an account-level filter set for the buyer account
* representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.FilterSet.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Full name of the resource being requested. For example: - For a bidder-level filter set
* for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Full name of the resource being requested. For example: - For a bidder-level filter set for bidder
123: `bidders/123/filterSets/abc` - For an account-level filter set for the buyer account
representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an account-level filter
set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getName() {
return name;
}
/**
* Full name of the resource being requested. For example: - For a bidder-level filter set
* for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all filter sets for the account with the given account ID.
*
* Create a request for the method "filterSets.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param ownerName Name of the owner (bidder or account) of the filter sets to be listed. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
* @return the request
*/
public List list(java.lang.String ownerName) throws java.io.IOException {
List result = new List(ownerName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+ownerName}/filterSets";
private final java.util.regex.Pattern OWNER_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+$");
/**
* Lists all filter sets for the account with the given account ID.
*
* Create a request for the method "filterSets.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param ownerName Name of the owner (bidder or account) of the filter sets to be listed. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123` - For an account-level filter set for the
* buyer account representing bidder 123: `bidders/123/accounts/123` - For an account-level
* filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
* @since 1.13
*/
protected List(java.lang.String ownerName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListFilterSetsResponse.class);
this.ownerName = com.google.api.client.util.Preconditions.checkNotNull(ownerName, "Required parameter ownerName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OWNER_NAME_PATTERN.matcher(ownerName).matches(),
"Parameter ownerName must conform to the pattern " +
"^bidders/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the owner (bidder or account) of the filter sets to be listed. For example: - For
* a bidder-level filter set for bidder 123: `bidders/123` - For an account-level filter set
* for the buyer account representing bidder 123: `bidders/123/accounts/123` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
*/
@com.google.api.client.util.Key
private java.lang.String ownerName;
/** Name of the owner (bidder or account) of the filter sets to be listed. For example: - For a bidder-
level filter set for bidder 123: `bidders/123` - For an account-level filter set for the buyer
account representing bidder 123: `bidders/123/accounts/123` - For an account-level filter set for
the child seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456`
*/
public java.lang.String getOwnerName() {
return ownerName;
}
/**
* Name of the owner (bidder or account) of the filter sets to be listed. For example: - For
* a bidder-level filter set for bidder 123: `bidders/123` - For an account-level filter set
* for the buyer account representing bidder 123: `bidders/123/accounts/123` - For an
* account-level filter set for the child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456`
*/
public List setOwnerName(java.lang.String ownerName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(OWNER_NAME_PATTERN.matcher(ownerName).matches(),
"Parameter ownerName must conform to the pattern " +
"^bidders/[^/]+$");
}
this.ownerName = ownerName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If unspecified,
* the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If unspecified,
* the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListFilterSetsResponse.nextPageToken returned from the previous call to the
* accounts.filterSets.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListFilterSetsResponse.nextPageToken returned from the previous call to the
accounts.filterSets.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListFilterSetsResponse.nextPageToken returned from the previous call to the
* accounts.filterSets.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the BidMetrics collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.BidMetrics.List request = adexchangebuyer2.bidMetrics().list(parameters ...)}
*
*
* @return the resource collection
*/
public BidMetrics bidMetrics() {
return new BidMetrics();
}
/**
* The "bidMetrics" collection of methods.
*/
public class BidMetrics {
/**
* Lists all metrics that are measured in terms of number of bids.
*
* Create a request for the method "bidMetrics.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/bidMetrics";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* Lists all metrics that are measured in terms of number of bids.
*
* Create a request for the method "bidMetrics.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListBidMetricsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListBidMetricsResponse.nextPageToken returned from the previous call to the
* bidMetrics.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListBidMetricsResponse.nextPageToken returned from the previous call to the bidMetrics.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListBidMetricsResponse.nextPageToken returned from the previous call to the
* bidMetrics.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the BidResponseErrors collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.BidResponseErrors.List request = adexchangebuyer2.bidResponseErrors().list(parameters ...)}
*
*
* @return the resource collection
*/
public BidResponseErrors bidResponseErrors() {
return new BidResponseErrors();
}
/**
* The "bidResponseErrors" collection of methods.
*/
public class BidResponseErrors {
/**
* List all errors that occurred in bid responses, with the number of bid responses affected for
* each reason.
*
* Create a request for the method "bidResponseErrors.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/bidResponseErrors";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* List all errors that occurred in bid responses, with the number of bid responses affected for
* each reason.
*
* Create a request for the method "bidResponseErrors.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListBidResponseErrorsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListBidResponseErrorsResponse.nextPageToken returned from the previous call to
* the bidResponseErrors.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListBidResponseErrorsResponse.nextPageToken returned from the previous call to the
bidResponseErrors.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListBidResponseErrorsResponse.nextPageToken returned from the previous call to
* the bidResponseErrors.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the BidResponsesWithoutBids collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.BidResponsesWithoutBids.List request = adexchangebuyer2.bidResponsesWithoutBids().list(parameters ...)}
*
*
* @return the resource collection
*/
public BidResponsesWithoutBids bidResponsesWithoutBids() {
return new BidResponsesWithoutBids();
}
/**
* The "bidResponsesWithoutBids" collection of methods.
*/
public class BidResponsesWithoutBids {
/**
* List all reasons for which bid responses were considered to have no applicable bids, with the
* number of bid responses affected for each reason.
*
* Create a request for the method "bidResponsesWithoutBids.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/bidResponsesWithoutBids";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* List all reasons for which bid responses were considered to have no applicable bids, with the
* number of bid responses affected for each reason.
*
* Create a request for the method "bidResponsesWithoutBids.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListBidResponsesWithoutBidsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListBidResponsesWithoutBidsResponse.nextPageToken returned from the previous
* call to the bidResponsesWithoutBids.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListBidResponsesWithoutBidsResponse.nextPageToken returned from the previous call to the
bidResponsesWithoutBids.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListBidResponsesWithoutBidsResponse.nextPageToken returned from the previous
* call to the bidResponsesWithoutBids.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the FilteredBidRequests collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.FilteredBidRequests.List request = adexchangebuyer2.filteredBidRequests().list(parameters ...)}
*
*
* @return the resource collection
*/
public FilteredBidRequests filteredBidRequests() {
return new FilteredBidRequests();
}
/**
* The "filteredBidRequests" collection of methods.
*/
public class FilteredBidRequests {
/**
* List all reasons that caused a bid request not to be sent for an impression, with the number of
* bid requests not sent for each reason.
*
* Create a request for the method "filteredBidRequests.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/filteredBidRequests";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* List all reasons that caused a bid request not to be sent for an impression, with the number of
* bid requests not sent for each reason.
*
* Create a request for the method "filteredBidRequests.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListFilteredBidRequestsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListFilteredBidRequestsResponse.nextPageToken returned from the previous call
* to the filteredBidRequests.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListFilteredBidRequestsResponse.nextPageToken returned from the previous call to the
filteredBidRequests.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListFilteredBidRequestsResponse.nextPageToken returned from the previous call
* to the filteredBidRequests.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the FilteredBids collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.FilteredBids.List request = adexchangebuyer2.filteredBids().list(parameters ...)}
*
*
* @return the resource collection
*/
public FilteredBids filteredBids() {
return new FilteredBids();
}
/**
* The "filteredBids" collection of methods.
*/
public class FilteredBids {
/**
* List all reasons for which bids were filtered, with the number of bids filtered for each reason.
*
* Create a request for the method "filteredBids.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/filteredBids";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* List all reasons for which bids were filtered, with the number of bids filtered for each
* reason.
*
* Create a request for the method "filteredBids.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListFilteredBidsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListFilteredBidsResponse.nextPageToken returned from the previous call to the
* filteredBids.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListFilteredBidsResponse.nextPageToken returned from the previous call to the filteredBids.list
method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListFilteredBidsResponse.nextPageToken returned from the previous call to the
* filteredBids.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Creatives collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Creatives.List request = adexchangebuyer2.creatives().list(parameters ...)}
*
*
* @return the resource collection
*/
public Creatives creatives() {
return new Creatives();
}
/**
* The "creatives" collection of methods.
*/
public class Creatives {
/**
* List all creatives associated with a specific reason for which bids were filtered, with the
* number of bids filtered for each creative.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @param creativeStatusId The ID of the creative status for which to retrieve a breakdown by creative. See [creative-status-
* codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-
* codes).
* @return the request
*/
public List list(java.lang.String filterSetName, java.lang.Integer creativeStatusId) throws java.io.IOException {
List result = new List(filterSetName, creativeStatusId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/filteredBids/{creativeStatusId}/creatives";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* List all creatives associated with a specific reason for which bids were filtered, with the
* number of bids filtered for each creative.
*
* Create a request for the method "creatives.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @param creativeStatusId The ID of the creative status for which to retrieve a breakdown by creative. See [creative-status-
* codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-
* codes).
* @since 1.13
*/
protected List(java.lang.String filterSetName, java.lang.Integer creativeStatusId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListCreativeStatusBreakdownByCreativeResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.creativeStatusId = com.google.api.client.util.Preconditions.checkNotNull(creativeStatusId, "Required parameter creativeStatusId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* The ID of the creative status for which to retrieve a breakdown by creative. See
* [creative-status-codes](https://developers.google.com/authorized-buyers/rtb/downloads
* /creative-status-codes).
*/
@com.google.api.client.util.Key
private java.lang.Integer creativeStatusId;
/** The ID of the creative status for which to retrieve a breakdown by creative. See [creative-status-
codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-codes).
*/
public java.lang.Integer getCreativeStatusId() {
return creativeStatusId;
}
/**
* The ID of the creative status for which to retrieve a breakdown by creative. See
* [creative-status-codes](https://developers.google.com/authorized-buyers/rtb/downloads
* /creative-status-codes).
*/
public List setCreativeStatusId(java.lang.Integer creativeStatusId) {
this.creativeStatusId = creativeStatusId;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListCreativeStatusBreakdownByCreativeResponse.nextPageToken returned
* from the previous call to the filteredBids.creatives.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListCreativeStatusBreakdownByCreativeResponse.nextPageToken returned from the previous call to the
filteredBids.creatives.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListCreativeStatusBreakdownByCreativeResponse.nextPageToken returned
* from the previous call to the filteredBids.creatives.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Details collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Details.List request = adexchangebuyer2.details().list(parameters ...)}
*
*
* @return the resource collection
*/
public Details details() {
return new Details();
}
/**
* The "details" collection of methods.
*/
public class Details {
/**
* List all details associated with a specific reason for which bids were filtered, with the number
* of bids filtered for each detail.
*
* Create a request for the method "details.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @param creativeStatusId The ID of the creative status for which to retrieve a breakdown by detail. See [creative-status-
* codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-
* codes). Details are only available for statuses 10, 14, 15, 17, 18, 19, 86, and 87.
* @return the request
*/
public List list(java.lang.String filterSetName, java.lang.Integer creativeStatusId) throws java.io.IOException {
List result = new List(filterSetName, creativeStatusId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/filteredBids/{creativeStatusId}/details";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* List all details associated with a specific reason for which bids were filtered, with the
* number of bids filtered for each detail.
*
* Create a request for the method "details.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @param creativeStatusId The ID of the creative status for which to retrieve a breakdown by detail. See [creative-status-
* codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-
* codes). Details are only available for statuses 10, 14, 15, 17, 18, 19, 86, and 87.
* @since 1.13
*/
protected List(java.lang.String filterSetName, java.lang.Integer creativeStatusId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListCreativeStatusBreakdownByDetailResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.creativeStatusId = com.google.api.client.util.Preconditions.checkNotNull(creativeStatusId, "Required parameter creativeStatusId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example:
* - For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* The ID of the creative status for which to retrieve a breakdown by detail. See
* [creative-status-codes](https://developers.google.com/authorized-buyers/rtb/downloads
* /creative-status-codes). Details are only available for statuses 10, 14, 15, 17, 18,
* 19, 86, and 87.
*/
@com.google.api.client.util.Key
private java.lang.Integer creativeStatusId;
/** The ID of the creative status for which to retrieve a breakdown by detail. See [creative-status-
codes](https://developers.google.com/authorized-buyers/rtb/downloads/creative-status-codes).
Details are only available for statuses 10, 14, 15, 17, 18, 19, 86, and 87.
*/
public java.lang.Integer getCreativeStatusId() {
return creativeStatusId;
}
/**
* The ID of the creative status for which to retrieve a breakdown by detail. See
* [creative-status-codes](https://developers.google.com/authorized-buyers/rtb/downloads
* /creative-status-codes). Details are only available for statuses 10, 14, 15, 17, 18,
* 19, 86, and 87.
*/
public List setCreativeStatusId(java.lang.Integer creativeStatusId) {
this.creativeStatusId = creativeStatusId;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListCreativeStatusBreakdownByDetailResponse.nextPageToken returned from
* the previous call to the filteredBids.details.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListCreativeStatusBreakdownByDetailResponse.nextPageToken returned from the previous call to the
filteredBids.details.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is
* the value of ListCreativeStatusBreakdownByDetailResponse.nextPageToken returned from
* the previous call to the filteredBids.details.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the ImpressionMetrics collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.ImpressionMetrics.List request = adexchangebuyer2.impressionMetrics().list(parameters ...)}
*
*
* @return the resource collection
*/
public ImpressionMetrics impressionMetrics() {
return new ImpressionMetrics();
}
/**
* The "impressionMetrics" collection of methods.
*/
public class ImpressionMetrics {
/**
* Lists all metrics that are measured in terms of number of impressions.
*
* Create a request for the method "impressionMetrics.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/impressionMetrics";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* Lists all metrics that are measured in terms of number of impressions.
*
* Create a request for the method "impressionMetrics.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListImpressionMetricsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListImpressionMetricsResponse.nextPageToken returned from the previous call to
* the impressionMetrics.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListImpressionMetricsResponse.nextPageToken returned from the previous call to the
impressionMetrics.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListImpressionMetricsResponse.nextPageToken returned from the previous call to
* the impressionMetrics.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the LosingBids collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.LosingBids.List request = adexchangebuyer2.losingBids().list(parameters ...)}
*
*
* @return the resource collection
*/
public LosingBids losingBids() {
return new LosingBids();
}
/**
* The "losingBids" collection of methods.
*/
public class LosingBids {
/**
* List all reasons for which bids lost in the auction, with the number of bids that lost for each
* reason.
*
* Create a request for the method "losingBids.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/losingBids";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* List all reasons for which bids lost in the auction, with the number of bids that lost for each
* reason.
*
* Create a request for the method "losingBids.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListLosingBidsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListLosingBidsResponse.nextPageToken returned from the previous call to the
* losingBids.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListLosingBidsResponse.nextPageToken returned from the previous call to the losingBids.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListLosingBidsResponse.nextPageToken returned from the previous call to the
* losingBids.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the NonBillableWinningBids collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.NonBillableWinningBids.List request = adexchangebuyer2.nonBillableWinningBids().list(parameters ...)}
*
*
* @return the resource collection
*/
public NonBillableWinningBids nonBillableWinningBids() {
return new NonBillableWinningBids();
}
/**
* The "nonBillableWinningBids" collection of methods.
*/
public class NonBillableWinningBids {
/**
* List all reasons for which winning bids were not billable, with the number of bids not billed for
* each reason.
*
* Create a request for the method "nonBillableWinningBids.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @return the request
*/
public List list(java.lang.String filterSetName) throws java.io.IOException {
List result = new List(filterSetName);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/{+filterSetName}/nonBillableWinningBids";
private final java.util.regex.Pattern FILTER_SET_NAME_PATTERN =
java.util.regex.Pattern.compile("^bidders/[^/]+/filterSets/[^/]+$");
/**
* List all reasons for which winning bids were not billable, with the number of bids not billed
* for each reason.
*
* Create a request for the method "nonBillableWinningBids.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param filterSetName Name of the filter set that should be applied to the requested metrics. For example: - For a bidder-
* level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level
* filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the child
* seat buyer account 456 whose bidder is 123: `bidders/123/accounts/456/filterSets/abc`
* @since 1.13
*/
protected List(java.lang.String filterSetName) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListNonBillableWinningBidsResponse.class);
this.filterSetName = com.google.api.client.util.Preconditions.checkNotNull(filterSetName, "Required parameter filterSetName must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
@com.google.api.client.util.Key
private java.lang.String filterSetName;
/** Name of the filter set that should be applied to the requested metrics. For example: - For a
bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an account-level filter
set for the buyer account representing bidder 123: `bidders/123/accounts/123/filterSets/abc` - For
an account-level filter set for the child seat buyer account 456 whose bidder is 123:
`bidders/123/accounts/456/filterSets/abc`
*/
public java.lang.String getFilterSetName() {
return filterSetName;
}
/**
* Name of the filter set that should be applied to the requested metrics. For example: -
* For a bidder-level filter set for bidder 123: `bidders/123/filterSets/abc` - For an
* account-level filter set for the buyer account representing bidder 123:
* `bidders/123/accounts/123/filterSets/abc` - For an account-level filter set for the
* child seat buyer account 456 whose bidder is 123:
* `bidders/123/accounts/456/filterSets/abc`
*/
public List setFilterSetName(java.lang.String filterSetName) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(FILTER_SET_NAME_PATTERN.matcher(filterSetName).matches(),
"Parameter filterSetName must conform to the pattern " +
"^bidders/[^/]+/filterSets/[^/]+$");
}
this.filterSetName = filterSetName;
return this;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer results than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer results than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListNonBillableWinningBidsResponse.nextPageToken returned from the previous
* call to the nonBillableWinningBids.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListNonBillableWinningBidsResponse.nextPageToken returned from the previous call to the
nonBillableWinningBids.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListNonBillableWinningBidsResponse.nextPageToken returned from the previous
* call to the nonBillableWinningBids.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* Builder for {@link AdExchangeBuyerII}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link AdExchangeBuyerII}. */
@Override
public AdExchangeBuyerII build() {
return new AdExchangeBuyerII(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link AdExchangeBuyerIIRequestInitializer}.
*
* @since 1.12
*/
public Builder setAdExchangeBuyerIIRequestInitializer(
AdExchangeBuyerIIRequestInitializer adexchangebuyeriiRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(adexchangebuyeriiRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}