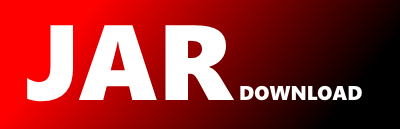
com.google.api.services.adexchangebuyer2.v2beta1.model.Creative Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.adexchangebuyer2.v2beta1.model;
/**
* A creative and its classification data.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Ad Exchange Buyer API II. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Creative extends com.google.api.client.json.GenericJson {
/**
* The account that this creative belongs to. Can be used to filter the response of the
* creatives.list method.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String accountId;
/**
* The link to AdChoices destination page.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String adChoicesDestinationUrl;
/**
* Output only. The detected ad technology providers.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AdTechnologyProviders adTechnologyProviders;
/**
* The name of the company being advertised in the creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String advertiserName;
/**
* The agency ID for this creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long agencyId;
/**
* Output only. The last update timestamp of the creative through the API.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String apiUpdateTime;
/**
* All attributes for the ads that may be shown from this creative. Can be used to filter the
* response of the creatives.list method.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List attributes;
/**
* The set of destination URLs for the creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List clickThroughUrls;
/**
* Output only. Shows any corrections that were applied to this creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List corrections;
static {
// hack to force ProGuard to consider Correction used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Correction.class);
}
/**
* The buyer-defined creative ID of this creative. Can be used to filter the response of the
* creatives.list method.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creativeId;
/**
* Output only. The top-level deals status of this creative. If disapproved, an entry for
* 'auctionType=DIRECT_DEALS' (or 'ALL') in serving_restrictions will also exist. Note that this
* may be nuanced with other contextual restrictions, in which case, it may be preferable to read
* from serving_restrictions directly. Can be used to filter the response of the creatives.list
* method.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dealsStatus;
/**
* The set of declared destination URLs for the creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List declaredClickThroughUrls;
/**
* Output only. Detected advertiser IDs, if any.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.util.List detectedAdvertiserIds;
/**
* Output only. The detected domains for this creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedDomains;
/**
* Output only. The detected languages for this creative. The order is arbitrary. The codes are 2
* or 5 characters and are documented at
* https://developers.google.com/adwords/api/docs/appendix/languagecodes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedLanguages;
/**
* Output only. Detected product categories, if any. See the ad-product-categories.txt file in the
* technical documentation for a list of IDs.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedProductCategories;
/**
* Output only. Detected sensitive categories, if any. See the ad-sensitive-categories.txt file in
* the technical documentation for a list of IDs. You should use these IDs along with the
* excluded-sensitive-category field in the bid request to filter your bids.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List detectedSensitiveCategories;
/**
* An HTML creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private HtmlContent html;
/**
* The set of URLs to be called to record an impression.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List impressionTrackingUrls;
/**
* A native creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key("native")
private NativeContent native__;
/**
* Output only. The top-level open auction status of this creative. If disapproved, an entry for
* 'auctionType = OPEN_AUCTION' (or 'ALL') in serving_restrictions will also exist. Note that this
* may be nuanced with other contextual restrictions, in which case, it may be preferable to read
* from serving_restrictions directly. Can be used to filter the response of the creatives.list
* method.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String openAuctionStatus;
/**
* All restricted categories for the ads that may be shown from this creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List restrictedCategories;
/**
* Output only. The granular status of this ad in specific contexts. A context here relates to
* where something ultimately serves (for example, a physical location, a platform, an HTTPS
* versus HTTP request, or the type of auction).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List servingRestrictions;
/**
* All vendor IDs for the ads that may be shown from this creative. See
* https://storage.googleapis.com/adx-rtb-dictionaries/vendors.txt for possible values.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List vendorIds;
/**
* Output only. The version of this creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer version;
/**
* A video creative.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private VideoContent video;
/**
* The account that this creative belongs to. Can be used to filter the response of the
* creatives.list method.
* @return value or {@code null} for none
*/
public java.lang.String getAccountId() {
return accountId;
}
/**
* The account that this creative belongs to. Can be used to filter the response of the
* creatives.list method.
* @param accountId accountId or {@code null} for none
*/
public Creative setAccountId(java.lang.String accountId) {
this.accountId = accountId;
return this;
}
/**
* The link to AdChoices destination page.
* @return value or {@code null} for none
*/
public java.lang.String getAdChoicesDestinationUrl() {
return adChoicesDestinationUrl;
}
/**
* The link to AdChoices destination page.
* @param adChoicesDestinationUrl adChoicesDestinationUrl or {@code null} for none
*/
public Creative setAdChoicesDestinationUrl(java.lang.String adChoicesDestinationUrl) {
this.adChoicesDestinationUrl = adChoicesDestinationUrl;
return this;
}
/**
* Output only. The detected ad technology providers.
* @return value or {@code null} for none
*/
public AdTechnologyProviders getAdTechnologyProviders() {
return adTechnologyProviders;
}
/**
* Output only. The detected ad technology providers.
* @param adTechnologyProviders adTechnologyProviders or {@code null} for none
*/
public Creative setAdTechnologyProviders(AdTechnologyProviders adTechnologyProviders) {
this.adTechnologyProviders = adTechnologyProviders;
return this;
}
/**
* The name of the company being advertised in the creative.
* @return value or {@code null} for none
*/
public java.lang.String getAdvertiserName() {
return advertiserName;
}
/**
* The name of the company being advertised in the creative.
* @param advertiserName advertiserName or {@code null} for none
*/
public Creative setAdvertiserName(java.lang.String advertiserName) {
this.advertiserName = advertiserName;
return this;
}
/**
* The agency ID for this creative.
* @return value or {@code null} for none
*/
public java.lang.Long getAgencyId() {
return agencyId;
}
/**
* The agency ID for this creative.
* @param agencyId agencyId or {@code null} for none
*/
public Creative setAgencyId(java.lang.Long agencyId) {
this.agencyId = agencyId;
return this;
}
/**
* Output only. The last update timestamp of the creative through the API.
* @return value or {@code null} for none
*/
public String getApiUpdateTime() {
return apiUpdateTime;
}
/**
* Output only. The last update timestamp of the creative through the API.
* @param apiUpdateTime apiUpdateTime or {@code null} for none
*/
public Creative setApiUpdateTime(String apiUpdateTime) {
this.apiUpdateTime = apiUpdateTime;
return this;
}
/**
* All attributes for the ads that may be shown from this creative. Can be used to filter the
* response of the creatives.list method.
* @return value or {@code null} for none
*/
public java.util.List getAttributes() {
return attributes;
}
/**
* All attributes for the ads that may be shown from this creative. Can be used to filter the
* response of the creatives.list method.
* @param attributes attributes or {@code null} for none
*/
public Creative setAttributes(java.util.List attributes) {
this.attributes = attributes;
return this;
}
/**
* The set of destination URLs for the creative.
* @return value or {@code null} for none
*/
public java.util.List getClickThroughUrls() {
return clickThroughUrls;
}
/**
* The set of destination URLs for the creative.
* @param clickThroughUrls clickThroughUrls or {@code null} for none
*/
public Creative setClickThroughUrls(java.util.List clickThroughUrls) {
this.clickThroughUrls = clickThroughUrls;
return this;
}
/**
* Output only. Shows any corrections that were applied to this creative.
* @return value or {@code null} for none
*/
public java.util.List getCorrections() {
return corrections;
}
/**
* Output only. Shows any corrections that were applied to this creative.
* @param corrections corrections or {@code null} for none
*/
public Creative setCorrections(java.util.List corrections) {
this.corrections = corrections;
return this;
}
/**
* The buyer-defined creative ID of this creative. Can be used to filter the response of the
* creatives.list method.
* @return value or {@code null} for none
*/
public java.lang.String getCreativeId() {
return creativeId;
}
/**
* The buyer-defined creative ID of this creative. Can be used to filter the response of the
* creatives.list method.
* @param creativeId creativeId or {@code null} for none
*/
public Creative setCreativeId(java.lang.String creativeId) {
this.creativeId = creativeId;
return this;
}
/**
* Output only. The top-level deals status of this creative. If disapproved, an entry for
* 'auctionType=DIRECT_DEALS' (or 'ALL') in serving_restrictions will also exist. Note that this
* may be nuanced with other contextual restrictions, in which case, it may be preferable to read
* from serving_restrictions directly. Can be used to filter the response of the creatives.list
* method.
* @return value or {@code null} for none
*/
public java.lang.String getDealsStatus() {
return dealsStatus;
}
/**
* Output only. The top-level deals status of this creative. If disapproved, an entry for
* 'auctionType=DIRECT_DEALS' (or 'ALL') in serving_restrictions will also exist. Note that this
* may be nuanced with other contextual restrictions, in which case, it may be preferable to read
* from serving_restrictions directly. Can be used to filter the response of the creatives.list
* method.
* @param dealsStatus dealsStatus or {@code null} for none
*/
public Creative setDealsStatus(java.lang.String dealsStatus) {
this.dealsStatus = dealsStatus;
return this;
}
/**
* The set of declared destination URLs for the creative.
* @return value or {@code null} for none
*/
public java.util.List getDeclaredClickThroughUrls() {
return declaredClickThroughUrls;
}
/**
* The set of declared destination URLs for the creative.
* @param declaredClickThroughUrls declaredClickThroughUrls or {@code null} for none
*/
public Creative setDeclaredClickThroughUrls(java.util.List declaredClickThroughUrls) {
this.declaredClickThroughUrls = declaredClickThroughUrls;
return this;
}
/**
* Output only. Detected advertiser IDs, if any.
* @return value or {@code null} for none
*/
public java.util.List getDetectedAdvertiserIds() {
return detectedAdvertiserIds;
}
/**
* Output only. Detected advertiser IDs, if any.
* @param detectedAdvertiserIds detectedAdvertiserIds or {@code null} for none
*/
public Creative setDetectedAdvertiserIds(java.util.List detectedAdvertiserIds) {
this.detectedAdvertiserIds = detectedAdvertiserIds;
return this;
}
/**
* Output only. The detected domains for this creative.
* @return value or {@code null} for none
*/
public java.util.List getDetectedDomains() {
return detectedDomains;
}
/**
* Output only. The detected domains for this creative.
* @param detectedDomains detectedDomains or {@code null} for none
*/
public Creative setDetectedDomains(java.util.List detectedDomains) {
this.detectedDomains = detectedDomains;
return this;
}
/**
* Output only. The detected languages for this creative. The order is arbitrary. The codes are 2
* or 5 characters and are documented at
* https://developers.google.com/adwords/api/docs/appendix/languagecodes.
* @return value or {@code null} for none
*/
public java.util.List getDetectedLanguages() {
return detectedLanguages;
}
/**
* Output only. The detected languages for this creative. The order is arbitrary. The codes are 2
* or 5 characters and are documented at
* https://developers.google.com/adwords/api/docs/appendix/languagecodes.
* @param detectedLanguages detectedLanguages or {@code null} for none
*/
public Creative setDetectedLanguages(java.util.List detectedLanguages) {
this.detectedLanguages = detectedLanguages;
return this;
}
/**
* Output only. Detected product categories, if any. See the ad-product-categories.txt file in the
* technical documentation for a list of IDs.
* @return value or {@code null} for none
*/
public java.util.List getDetectedProductCategories() {
return detectedProductCategories;
}
/**
* Output only. Detected product categories, if any. See the ad-product-categories.txt file in the
* technical documentation for a list of IDs.
* @param detectedProductCategories detectedProductCategories or {@code null} for none
*/
public Creative setDetectedProductCategories(java.util.List detectedProductCategories) {
this.detectedProductCategories = detectedProductCategories;
return this;
}
/**
* Output only. Detected sensitive categories, if any. See the ad-sensitive-categories.txt file in
* the technical documentation for a list of IDs. You should use these IDs along with the
* excluded-sensitive-category field in the bid request to filter your bids.
* @return value or {@code null} for none
*/
public java.util.List getDetectedSensitiveCategories() {
return detectedSensitiveCategories;
}
/**
* Output only. Detected sensitive categories, if any. See the ad-sensitive-categories.txt file in
* the technical documentation for a list of IDs. You should use these IDs along with the
* excluded-sensitive-category field in the bid request to filter your bids.
* @param detectedSensitiveCategories detectedSensitiveCategories or {@code null} for none
*/
public Creative setDetectedSensitiveCategories(java.util.List detectedSensitiveCategories) {
this.detectedSensitiveCategories = detectedSensitiveCategories;
return this;
}
/**
* An HTML creative.
* @return value or {@code null} for none
*/
public HtmlContent getHtml() {
return html;
}
/**
* An HTML creative.
* @param html html or {@code null} for none
*/
public Creative setHtml(HtmlContent html) {
this.html = html;
return this;
}
/**
* The set of URLs to be called to record an impression.
* @return value or {@code null} for none
*/
public java.util.List getImpressionTrackingUrls() {
return impressionTrackingUrls;
}
/**
* The set of URLs to be called to record an impression.
* @param impressionTrackingUrls impressionTrackingUrls or {@code null} for none
*/
public Creative setImpressionTrackingUrls(java.util.List impressionTrackingUrls) {
this.impressionTrackingUrls = impressionTrackingUrls;
return this;
}
/**
* A native creative.
* @return value or {@code null} for none
*/
public NativeContent getNative() {
return native__;
}
/**
* A native creative.
* @param native__ native__ or {@code null} for none
*/
public Creative setNative(NativeContent native__) {
this.native__ = native__;
return this;
}
/**
* Output only. The top-level open auction status of this creative. If disapproved, an entry for
* 'auctionType = OPEN_AUCTION' (or 'ALL') in serving_restrictions will also exist. Note that this
* may be nuanced with other contextual restrictions, in which case, it may be preferable to read
* from serving_restrictions directly. Can be used to filter the response of the creatives.list
* method.
* @return value or {@code null} for none
*/
public java.lang.String getOpenAuctionStatus() {
return openAuctionStatus;
}
/**
* Output only. The top-level open auction status of this creative. If disapproved, an entry for
* 'auctionType = OPEN_AUCTION' (or 'ALL') in serving_restrictions will also exist. Note that this
* may be nuanced with other contextual restrictions, in which case, it may be preferable to read
* from serving_restrictions directly. Can be used to filter the response of the creatives.list
* method.
* @param openAuctionStatus openAuctionStatus or {@code null} for none
*/
public Creative setOpenAuctionStatus(java.lang.String openAuctionStatus) {
this.openAuctionStatus = openAuctionStatus;
return this;
}
/**
* All restricted categories for the ads that may be shown from this creative.
* @return value or {@code null} for none
*/
public java.util.List getRestrictedCategories() {
return restrictedCategories;
}
/**
* All restricted categories for the ads that may be shown from this creative.
* @param restrictedCategories restrictedCategories or {@code null} for none
*/
public Creative setRestrictedCategories(java.util.List restrictedCategories) {
this.restrictedCategories = restrictedCategories;
return this;
}
/**
* Output only. The granular status of this ad in specific contexts. A context here relates to
* where something ultimately serves (for example, a physical location, a platform, an HTTPS
* versus HTTP request, or the type of auction).
* @return value or {@code null} for none
*/
public java.util.List getServingRestrictions() {
return servingRestrictions;
}
/**
* Output only. The granular status of this ad in specific contexts. A context here relates to
* where something ultimately serves (for example, a physical location, a platform, an HTTPS
* versus HTTP request, or the type of auction).
* @param servingRestrictions servingRestrictions or {@code null} for none
*/
public Creative setServingRestrictions(java.util.List servingRestrictions) {
this.servingRestrictions = servingRestrictions;
return this;
}
/**
* All vendor IDs for the ads that may be shown from this creative. See
* https://storage.googleapis.com/adx-rtb-dictionaries/vendors.txt for possible values.
* @return value or {@code null} for none
*/
public java.util.List getVendorIds() {
return vendorIds;
}
/**
* All vendor IDs for the ads that may be shown from this creative. See
* https://storage.googleapis.com/adx-rtb-dictionaries/vendors.txt for possible values.
* @param vendorIds vendorIds or {@code null} for none
*/
public Creative setVendorIds(java.util.List vendorIds) {
this.vendorIds = vendorIds;
return this;
}
/**
* Output only. The version of this creative.
* @return value or {@code null} for none
*/
public java.lang.Integer getVersion() {
return version;
}
/**
* Output only. The version of this creative.
* @param version version or {@code null} for none
*/
public Creative setVersion(java.lang.Integer version) {
this.version = version;
return this;
}
/**
* A video creative.
* @return value or {@code null} for none
*/
public VideoContent getVideo() {
return video;
}
/**
* A video creative.
* @param video video or {@code null} for none
*/
public Creative setVideo(VideoContent video) {
this.video = video;
return this;
}
@Override
public Creative set(String fieldName, Object value) {
return (Creative) super.set(fieldName, value);
}
@Override
public Creative clone() {
return (Creative) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy