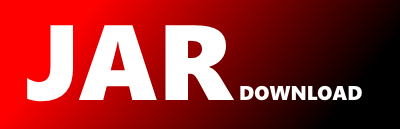
com.google.api.services.adexchangebuyer2.v2beta1.model.Deal Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.adexchangebuyer2.v2beta1.model;
/**
* A deal represents a segment of inventory for displaying ads on. A proposal can contain multiple
* deals. A deal contains the terms and targeting information that is used for serving.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Ad Exchange Buyer API II. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Deal extends com.google.api.client.json.GenericJson {
/**
* Proposed flight end time of the deal. This will generally be stored in a granularity of a
* second. A value is not required for Private Auction deals or Preferred Deals.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String availableEndTime;
/**
* Optional. Proposed flight start time of the deal. This will generally be stored in the
* granularity of one second since deal serving starts at seconds boundary. Any time specified
* with more granularity (for example, in milliseconds) will be truncated towards the start of
* time in seconds.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String availableStartTime;
/**
* Buyer private data (hidden from seller).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PrivateData buyerPrivateData;
/**
* The product ID from which this deal was created. Note: This field may be set only when creating
* the resource. Modifying this field while updating the resource will result in an error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String createProductId;
/**
* Optional. Revision number of the product that the deal was created from. If present on create,
* and the server `product_revision` has advanced since the passed-in `create_product_revision`,
* an `ABORTED` error will be returned. Note: This field may be set only when creating the
* resource. Modifying this field while updating the resource will result in an error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long createProductRevision;
/**
* Output only. The time of the deal creation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Output only. Specifies the creative pre-approval policy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creativePreApprovalPolicy;
/**
* Output only. Restricitions about the creatives associated with the deal (for example, size)
* This is available for Programmatic Guaranteed/Preferred Deals in Ad Manager.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CreativeRestrictions creativeRestrictions;
/**
* Output only. Specifies whether the creative is safeFrame compatible.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creativeSafeFrameCompatibility;
/**
* Output only. A unique deal ID for the deal (server-assigned).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dealId;
/**
* Output only. Metadata about the serving status of this deal.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DealServingMetadata dealServingMetadata;
/**
* The negotiable terms of the deal.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DealTerms dealTerms;
/**
* The set of fields around delivery control that are interesting for a buyer to see but are non-
* negotiable. These are set by the publisher.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeliveryControl deliveryControl;
/**
* Description for the deal terms.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The name of the deal.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* Output only. The external deal ID assigned to this deal once the deal is finalized. This is the
* deal ID that shows up in serving/reporting etc.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String externalDealId;
/**
* Output only. True, if the buyside inventory setup is complete for this deal.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isSetupComplete;
/**
* Output only. Specifies the creative source for programmatic deals. PUBLISHER means creative is
* provided by seller and ADVERTISER means creative is provided by buyer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String programmaticCreativeSource;
/**
* Output only. ID of the proposal that this deal is part of.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String proposalId;
/**
* Output only. Seller contact information for the deal.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List sellerContacts;
static {
// hack to force ProGuard to consider ContactInformation used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(ContactInformation.class);
}
/**
* The syndication product associated with the deal. Note: This field may be set only when
* creating the resource. Modifying this field while updating the resource will result in an
* error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String syndicationProduct;
/**
* Output only. Specifies the subset of inventory targeted by the deal.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MarketplaceTargeting targeting;
/**
* The shared targeting visible to buyers and sellers. Each shared targeting entity is AND'd
* together.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List targetingCriterion;
/**
* Output only. The time when the deal was last updated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* The web property code for the seller copied over from the product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String webPropertyCode;
/**
* Proposed flight end time of the deal. This will generally be stored in a granularity of a
* second. A value is not required for Private Auction deals or Preferred Deals.
* @return value or {@code null} for none
*/
public String getAvailableEndTime() {
return availableEndTime;
}
/**
* Proposed flight end time of the deal. This will generally be stored in a granularity of a
* second. A value is not required for Private Auction deals or Preferred Deals.
* @param availableEndTime availableEndTime or {@code null} for none
*/
public Deal setAvailableEndTime(String availableEndTime) {
this.availableEndTime = availableEndTime;
return this;
}
/**
* Optional. Proposed flight start time of the deal. This will generally be stored in the
* granularity of one second since deal serving starts at seconds boundary. Any time specified
* with more granularity (for example, in milliseconds) will be truncated towards the start of
* time in seconds.
* @return value or {@code null} for none
*/
public String getAvailableStartTime() {
return availableStartTime;
}
/**
* Optional. Proposed flight start time of the deal. This will generally be stored in the
* granularity of one second since deal serving starts at seconds boundary. Any time specified
* with more granularity (for example, in milliseconds) will be truncated towards the start of
* time in seconds.
* @param availableStartTime availableStartTime or {@code null} for none
*/
public Deal setAvailableStartTime(String availableStartTime) {
this.availableStartTime = availableStartTime;
return this;
}
/**
* Buyer private data (hidden from seller).
* @return value or {@code null} for none
*/
public PrivateData getBuyerPrivateData() {
return buyerPrivateData;
}
/**
* Buyer private data (hidden from seller).
* @param buyerPrivateData buyerPrivateData or {@code null} for none
*/
public Deal setBuyerPrivateData(PrivateData buyerPrivateData) {
this.buyerPrivateData = buyerPrivateData;
return this;
}
/**
* The product ID from which this deal was created. Note: This field may be set only when creating
* the resource. Modifying this field while updating the resource will result in an error.
* @return value or {@code null} for none
*/
public java.lang.String getCreateProductId() {
return createProductId;
}
/**
* The product ID from which this deal was created. Note: This field may be set only when creating
* the resource. Modifying this field while updating the resource will result in an error.
* @param createProductId createProductId or {@code null} for none
*/
public Deal setCreateProductId(java.lang.String createProductId) {
this.createProductId = createProductId;
return this;
}
/**
* Optional. Revision number of the product that the deal was created from. If present on create,
* and the server `product_revision` has advanced since the passed-in `create_product_revision`,
* an `ABORTED` error will be returned. Note: This field may be set only when creating the
* resource. Modifying this field while updating the resource will result in an error.
* @return value or {@code null} for none
*/
public java.lang.Long getCreateProductRevision() {
return createProductRevision;
}
/**
* Optional. Revision number of the product that the deal was created from. If present on create,
* and the server `product_revision` has advanced since the passed-in `create_product_revision`,
* an `ABORTED` error will be returned. Note: This field may be set only when creating the
* resource. Modifying this field while updating the resource will result in an error.
* @param createProductRevision createProductRevision or {@code null} for none
*/
public Deal setCreateProductRevision(java.lang.Long createProductRevision) {
this.createProductRevision = createProductRevision;
return this;
}
/**
* Output only. The time of the deal creation.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. The time of the deal creation.
* @param createTime createTime or {@code null} for none
*/
public Deal setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Output only. Specifies the creative pre-approval policy.
* @return value or {@code null} for none
*/
public java.lang.String getCreativePreApprovalPolicy() {
return creativePreApprovalPolicy;
}
/**
* Output only. Specifies the creative pre-approval policy.
* @param creativePreApprovalPolicy creativePreApprovalPolicy or {@code null} for none
*/
public Deal setCreativePreApprovalPolicy(java.lang.String creativePreApprovalPolicy) {
this.creativePreApprovalPolicy = creativePreApprovalPolicy;
return this;
}
/**
* Output only. Restricitions about the creatives associated with the deal (for example, size)
* This is available for Programmatic Guaranteed/Preferred Deals in Ad Manager.
* @return value or {@code null} for none
*/
public CreativeRestrictions getCreativeRestrictions() {
return creativeRestrictions;
}
/**
* Output only. Restricitions about the creatives associated with the deal (for example, size)
* This is available for Programmatic Guaranteed/Preferred Deals in Ad Manager.
* @param creativeRestrictions creativeRestrictions or {@code null} for none
*/
public Deal setCreativeRestrictions(CreativeRestrictions creativeRestrictions) {
this.creativeRestrictions = creativeRestrictions;
return this;
}
/**
* Output only. Specifies whether the creative is safeFrame compatible.
* @return value or {@code null} for none
*/
public java.lang.String getCreativeSafeFrameCompatibility() {
return creativeSafeFrameCompatibility;
}
/**
* Output only. Specifies whether the creative is safeFrame compatible.
* @param creativeSafeFrameCompatibility creativeSafeFrameCompatibility or {@code null} for none
*/
public Deal setCreativeSafeFrameCompatibility(java.lang.String creativeSafeFrameCompatibility) {
this.creativeSafeFrameCompatibility = creativeSafeFrameCompatibility;
return this;
}
/**
* Output only. A unique deal ID for the deal (server-assigned).
* @return value or {@code null} for none
*/
public java.lang.String getDealId() {
return dealId;
}
/**
* Output only. A unique deal ID for the deal (server-assigned).
* @param dealId dealId or {@code null} for none
*/
public Deal setDealId(java.lang.String dealId) {
this.dealId = dealId;
return this;
}
/**
* Output only. Metadata about the serving status of this deal.
* @return value or {@code null} for none
*/
public DealServingMetadata getDealServingMetadata() {
return dealServingMetadata;
}
/**
* Output only. Metadata about the serving status of this deal.
* @param dealServingMetadata dealServingMetadata or {@code null} for none
*/
public Deal setDealServingMetadata(DealServingMetadata dealServingMetadata) {
this.dealServingMetadata = dealServingMetadata;
return this;
}
/**
* The negotiable terms of the deal.
* @return value or {@code null} for none
*/
public DealTerms getDealTerms() {
return dealTerms;
}
/**
* The negotiable terms of the deal.
* @param dealTerms dealTerms or {@code null} for none
*/
public Deal setDealTerms(DealTerms dealTerms) {
this.dealTerms = dealTerms;
return this;
}
/**
* The set of fields around delivery control that are interesting for a buyer to see but are non-
* negotiable. These are set by the publisher.
* @return value or {@code null} for none
*/
public DeliveryControl getDeliveryControl() {
return deliveryControl;
}
/**
* The set of fields around delivery control that are interesting for a buyer to see but are non-
* negotiable. These are set by the publisher.
* @param deliveryControl deliveryControl or {@code null} for none
*/
public Deal setDeliveryControl(DeliveryControl deliveryControl) {
this.deliveryControl = deliveryControl;
return this;
}
/**
* Description for the deal terms.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Description for the deal terms.
* @param description description or {@code null} for none
*/
public Deal setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The name of the deal.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The name of the deal.
* @param displayName displayName or {@code null} for none
*/
public Deal setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* Output only. The external deal ID assigned to this deal once the deal is finalized. This is the
* deal ID that shows up in serving/reporting etc.
* @return value or {@code null} for none
*/
public java.lang.String getExternalDealId() {
return externalDealId;
}
/**
* Output only. The external deal ID assigned to this deal once the deal is finalized. This is the
* deal ID that shows up in serving/reporting etc.
* @param externalDealId externalDealId or {@code null} for none
*/
public Deal setExternalDealId(java.lang.String externalDealId) {
this.externalDealId = externalDealId;
return this;
}
/**
* Output only. True, if the buyside inventory setup is complete for this deal.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsSetupComplete() {
return isSetupComplete;
}
/**
* Output only. True, if the buyside inventory setup is complete for this deal.
* @param isSetupComplete isSetupComplete or {@code null} for none
*/
public Deal setIsSetupComplete(java.lang.Boolean isSetupComplete) {
this.isSetupComplete = isSetupComplete;
return this;
}
/**
* Output only. Specifies the creative source for programmatic deals. PUBLISHER means creative is
* provided by seller and ADVERTISER means creative is provided by buyer.
* @return value or {@code null} for none
*/
public java.lang.String getProgrammaticCreativeSource() {
return programmaticCreativeSource;
}
/**
* Output only. Specifies the creative source for programmatic deals. PUBLISHER means creative is
* provided by seller and ADVERTISER means creative is provided by buyer.
* @param programmaticCreativeSource programmaticCreativeSource or {@code null} for none
*/
public Deal setProgrammaticCreativeSource(java.lang.String programmaticCreativeSource) {
this.programmaticCreativeSource = programmaticCreativeSource;
return this;
}
/**
* Output only. ID of the proposal that this deal is part of.
* @return value or {@code null} for none
*/
public java.lang.String getProposalId() {
return proposalId;
}
/**
* Output only. ID of the proposal that this deal is part of.
* @param proposalId proposalId or {@code null} for none
*/
public Deal setProposalId(java.lang.String proposalId) {
this.proposalId = proposalId;
return this;
}
/**
* Output only. Seller contact information for the deal.
* @return value or {@code null} for none
*/
public java.util.List getSellerContacts() {
return sellerContacts;
}
/**
* Output only. Seller contact information for the deal.
* @param sellerContacts sellerContacts or {@code null} for none
*/
public Deal setSellerContacts(java.util.List sellerContacts) {
this.sellerContacts = sellerContacts;
return this;
}
/**
* The syndication product associated with the deal. Note: This field may be set only when
* creating the resource. Modifying this field while updating the resource will result in an
* error.
* @return value or {@code null} for none
*/
public java.lang.String getSyndicationProduct() {
return syndicationProduct;
}
/**
* The syndication product associated with the deal. Note: This field may be set only when
* creating the resource. Modifying this field while updating the resource will result in an
* error.
* @param syndicationProduct syndicationProduct or {@code null} for none
*/
public Deal setSyndicationProduct(java.lang.String syndicationProduct) {
this.syndicationProduct = syndicationProduct;
return this;
}
/**
* Output only. Specifies the subset of inventory targeted by the deal.
* @return value or {@code null} for none
*/
public MarketplaceTargeting getTargeting() {
return targeting;
}
/**
* Output only. Specifies the subset of inventory targeted by the deal.
* @param targeting targeting or {@code null} for none
*/
public Deal setTargeting(MarketplaceTargeting targeting) {
this.targeting = targeting;
return this;
}
/**
* The shared targeting visible to buyers and sellers. Each shared targeting entity is AND'd
* together.
* @return value or {@code null} for none
*/
public java.util.List getTargetingCriterion() {
return targetingCriterion;
}
/**
* The shared targeting visible to buyers and sellers. Each shared targeting entity is AND'd
* together.
* @param targetingCriterion targetingCriterion or {@code null} for none
*/
public Deal setTargetingCriterion(java.util.List targetingCriterion) {
this.targetingCriterion = targetingCriterion;
return this;
}
/**
* Output only. The time when the deal was last updated.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. The time when the deal was last updated.
* @param updateTime updateTime or {@code null} for none
*/
public Deal setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
/**
* The web property code for the seller copied over from the product.
* @return value or {@code null} for none
*/
public java.lang.String getWebPropertyCode() {
return webPropertyCode;
}
/**
* The web property code for the seller copied over from the product.
* @param webPropertyCode webPropertyCode or {@code null} for none
*/
public Deal setWebPropertyCode(java.lang.String webPropertyCode) {
this.webPropertyCode = webPropertyCode;
return this;
}
@Override
public Deal set(String fieldName, Object value) {
return (Deal) super.set(fieldName, value);
}
@Override
public Deal clone() {
return (Deal) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy