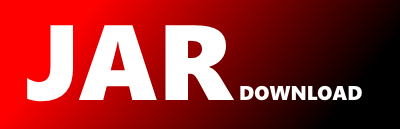
com.google.api.services.adexchangebuyer2.v2beta1.model.DealTerms Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.adexchangebuyer2.v2beta1.model;
/**
* The deal terms specify the details of a Product/deal. They specify things like price per buyer,
* the type of pricing model (for example, fixed price, auction) and expected impressions from the
* publisher.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Ad Exchange Buyer API II. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DealTerms extends com.google.api.client.json.GenericJson {
/**
* Visibility of the URL in bid requests. (default: BRANDED)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String brandingType;
/**
* Publisher provided description for the terms.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Non-binding estimate of the estimated gross spend for this deal. Can be set by buyer or seller.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Price estimatedGrossSpend;
/**
* Non-binding estimate of the impressions served per day. Can be set by buyer or seller.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long estimatedImpressionsPerDay;
/**
* The terms for guaranteed fixed price deals.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GuaranteedFixedPriceTerms guaranteedFixedPriceTerms;
/**
* The terms for non-guaranteed auction deals.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NonGuaranteedAuctionTerms nonGuaranteedAuctionTerms;
/**
* The terms for non-guaranteed fixed price deals.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NonGuaranteedFixedPriceTerms nonGuaranteedFixedPriceTerms;
/**
* The time zone name. For deals with Cost Per Day billing, defines the time zone used to mark the
* boundaries of a day. It should be an IANA TZ name, such as "America/Los_Angeles". For more
* information, see https://en.wikipedia.org/wiki/List_of_tz_database_time_zones.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sellerTimeZone;
/**
* Visibility of the URL in bid requests. (default: BRANDED)
* @return value or {@code null} for none
*/
public java.lang.String getBrandingType() {
return brandingType;
}
/**
* Visibility of the URL in bid requests. (default: BRANDED)
* @param brandingType brandingType or {@code null} for none
*/
public DealTerms setBrandingType(java.lang.String brandingType) {
this.brandingType = brandingType;
return this;
}
/**
* Publisher provided description for the terms.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Publisher provided description for the terms.
* @param description description or {@code null} for none
*/
public DealTerms setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Non-binding estimate of the estimated gross spend for this deal. Can be set by buyer or seller.
* @return value or {@code null} for none
*/
public Price getEstimatedGrossSpend() {
return estimatedGrossSpend;
}
/**
* Non-binding estimate of the estimated gross spend for this deal. Can be set by buyer or seller.
* @param estimatedGrossSpend estimatedGrossSpend or {@code null} for none
*/
public DealTerms setEstimatedGrossSpend(Price estimatedGrossSpend) {
this.estimatedGrossSpend = estimatedGrossSpend;
return this;
}
/**
* Non-binding estimate of the impressions served per day. Can be set by buyer or seller.
* @return value or {@code null} for none
*/
public java.lang.Long getEstimatedImpressionsPerDay() {
return estimatedImpressionsPerDay;
}
/**
* Non-binding estimate of the impressions served per day. Can be set by buyer or seller.
* @param estimatedImpressionsPerDay estimatedImpressionsPerDay or {@code null} for none
*/
public DealTerms setEstimatedImpressionsPerDay(java.lang.Long estimatedImpressionsPerDay) {
this.estimatedImpressionsPerDay = estimatedImpressionsPerDay;
return this;
}
/**
* The terms for guaranteed fixed price deals.
* @return value or {@code null} for none
*/
public GuaranteedFixedPriceTerms getGuaranteedFixedPriceTerms() {
return guaranteedFixedPriceTerms;
}
/**
* The terms for guaranteed fixed price deals.
* @param guaranteedFixedPriceTerms guaranteedFixedPriceTerms or {@code null} for none
*/
public DealTerms setGuaranteedFixedPriceTerms(GuaranteedFixedPriceTerms guaranteedFixedPriceTerms) {
this.guaranteedFixedPriceTerms = guaranteedFixedPriceTerms;
return this;
}
/**
* The terms for non-guaranteed auction deals.
* @return value or {@code null} for none
*/
public NonGuaranteedAuctionTerms getNonGuaranteedAuctionTerms() {
return nonGuaranteedAuctionTerms;
}
/**
* The terms for non-guaranteed auction deals.
* @param nonGuaranteedAuctionTerms nonGuaranteedAuctionTerms or {@code null} for none
*/
public DealTerms setNonGuaranteedAuctionTerms(NonGuaranteedAuctionTerms nonGuaranteedAuctionTerms) {
this.nonGuaranteedAuctionTerms = nonGuaranteedAuctionTerms;
return this;
}
/**
* The terms for non-guaranteed fixed price deals.
* @return value or {@code null} for none
*/
public NonGuaranteedFixedPriceTerms getNonGuaranteedFixedPriceTerms() {
return nonGuaranteedFixedPriceTerms;
}
/**
* The terms for non-guaranteed fixed price deals.
* @param nonGuaranteedFixedPriceTerms nonGuaranteedFixedPriceTerms or {@code null} for none
*/
public DealTerms setNonGuaranteedFixedPriceTerms(NonGuaranteedFixedPriceTerms nonGuaranteedFixedPriceTerms) {
this.nonGuaranteedFixedPriceTerms = nonGuaranteedFixedPriceTerms;
return this;
}
/**
* The time zone name. For deals with Cost Per Day billing, defines the time zone used to mark the
* boundaries of a day. It should be an IANA TZ name, such as "America/Los_Angeles". For more
* information, see https://en.wikipedia.org/wiki/List_of_tz_database_time_zones.
* @return value or {@code null} for none
*/
public java.lang.String getSellerTimeZone() {
return sellerTimeZone;
}
/**
* The time zone name. For deals with Cost Per Day billing, defines the time zone used to mark the
* boundaries of a day. It should be an IANA TZ name, such as "America/Los_Angeles". For more
* information, see https://en.wikipedia.org/wiki/List_of_tz_database_time_zones.
* @param sellerTimeZone sellerTimeZone or {@code null} for none
*/
public DealTerms setSellerTimeZone(java.lang.String sellerTimeZone) {
this.sellerTimeZone = sellerTimeZone;
return this;
}
@Override
public DealTerms set(String fieldName, Object value) {
return (DealTerms) super.set(fieldName, value);
}
@Override
public DealTerms clone() {
return (DealTerms) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy