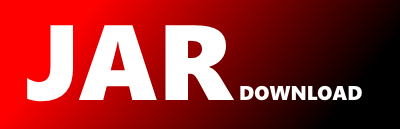
com.google.api.services.adexchangebuyer2.v2beta1.AdExchangeBuyerII Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-27 16:00:31 UTC)
* on 2016-06-13 at 16:00:08 UTC
* Modify at your own risk.
*/
package com.google.api.services.adexchangebuyer2.v2beta1;
/**
* Service definition for AdExchangeBuyerII (v2beta1).
*
*
* Accesses the latest features for managing Ad Exchange accounts and Real-Time Bidding configurations.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link AdExchangeBuyerIIRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class AdExchangeBuyerII extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.22.0 of the Ad Exchange Buyer API II library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://adexchangebuyer.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public AdExchangeBuyerII(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
AdExchangeBuyerII(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Accounts collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Accounts.List request = adexchangebuyer2.accounts().list(parameters ...)}
*
*
* @return the resource collection
*/
public Accounts accounts() {
return new Accounts();
}
/**
* The "accounts" collection of methods.
*/
public class Accounts {
/**
* An accessor for creating requests from the Clients collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Clients.List request = adexchangebuyer2.clients().list(parameters ...)}
*
*
* @return the resource collection
*/
public Clients clients() {
return new Clients();
}
/**
* The "clients" collection of methods.
*/
public class Clients {
/**
* Creates a new client buyer.
*
* Create a request for the method "clients.create".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param accountId Unique numerical account ID for the buyer of which the client buyer
is a customer; the sponsor buyer
* to create a client for. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Client}
* @return the request
*/
public Create create(java.lang.Long accountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Client content) throws java.io.IOException {
Create result = new Create(accountId, content);
initialize(result);
return result;
}
public class Create extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients";
/**
* Creates a new client buyer.
*
* Create a request for the method "clients.create".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Unique numerical account ID for the buyer of which the client buyer
is a customer; the sponsor buyer
* to create a client for. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Client}
* @since 1.13
*/
protected Create(java.lang.Long accountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Client content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Client.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setBearerToken(java.lang.String bearerToken) {
return (Create) super.setBearerToken(bearerToken);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPp(java.lang.Boolean pp) {
return (Create) super.setPp(pp);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique numerical account ID for the buyer of which the client buyer is a customer; the
* sponsor buyer to create a client for. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Unique numerical account ID for the buyer of which the client buyer is a customer; the sponsor
buyer to create a client for. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/**
* Unique numerical account ID for the buyer of which the client buyer is a customer; the
* sponsor buyer to create a client for. (required)
*/
public Create setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets a client buyer with a given client account ID.
*
* Create a request for the method "clients.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer to retrieve. (required)
* @return the request
*/
public Get get(java.lang.Long accountId, java.lang.Long clientAccountId) throws java.io.IOException {
Get result = new Get(accountId, clientAccountId);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}";
/**
* Gets a client buyer with a given client account ID.
*
* Create a request for the method "clients.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer to retrieve. (required)
* @since 1.13
*/
protected Get(java.lang.Long accountId, java.lang.Long clientAccountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.Client.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public Get setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/** Numerical account ID of the client buyer to retrieve. (required) */
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Numerical account ID of the client buyer to retrieve. (required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/** Numerical account ID of the client buyer to retrieve. (required) */
public Get setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the clients for the current sponsor buyer.
*
* Create a request for the method "clients.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId Unique numerical account ID of the sponsor buyer to list the clients for.
* @return the request
*/
public List list(java.lang.Long accountId) throws java.io.IOException {
List result = new List(accountId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients";
/**
* Lists all the clients for the current sponsor buyer.
*
* Create a request for the method "clients.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Unique numerical account ID of the sponsor buyer to list the clients for.
* @since 1.13
*/
protected List(java.lang.Long accountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListClientsResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Unique numerical account ID of the sponsor buyer to list the clients for. */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Unique numerical account ID of the sponsor buyer to list the clients for.
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Unique numerical account ID of the sponsor buyer to list the clients for. */
public List setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Requested page size. The server may return fewer clients than requested. If unspecified,
* the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer clients than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer clients than requested. If unspecified,
* the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientsResponse.nextPageToken returned from the previous call to the
* accounts.clients.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListClientsResponse.nextPageToken returned from the previous call to the accounts.clients.list
method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientsResponse.nextPageToken returned from the previous call to the
* accounts.clients.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing client buyer.
*
* Create a request for the method "clients.update".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param accountId Unique numerical account ID for the buyer of which the client buyer
is a customer; the sponsor buyer
* to update a client for. (required)
* @param clientAccountId Unique numerical account ID of the client to update. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Client}
* @return the request
*/
public Update update(java.lang.Long accountId, java.lang.Long clientAccountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Client content) throws java.io.IOException {
Update result = new Update(accountId, clientAccountId, content);
initialize(result);
return result;
}
public class Update extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}";
/**
* Updates an existing client buyer.
*
* Create a request for the method "clients.update".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Unique numerical account ID for the buyer of which the client buyer
is a customer; the sponsor buyer
* to update a client for. (required)
* @param clientAccountId Unique numerical account ID of the client to update. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.Client}
* @since 1.13
*/
protected Update(java.lang.Long accountId, java.lang.Long clientAccountId, com.google.api.services.adexchangebuyer2.v2beta1.model.Client content) {
super(AdExchangeBuyerII.this, "PUT", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.Client.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setBearerToken(java.lang.String bearerToken) {
return (Update) super.setBearerToken(bearerToken);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPp(java.lang.Boolean pp) {
return (Update) super.setPp(pp);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique numerical account ID for the buyer of which the client buyer is a customer; the
* sponsor buyer to update a client for. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Unique numerical account ID for the buyer of which the client buyer is a customer; the sponsor
buyer to update a client for. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/**
* Unique numerical account ID for the buyer of which the client buyer is a customer; the
* sponsor buyer to update a client for. (required)
*/
public Update setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/** Unique numerical account ID of the client to update. (required) */
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Unique numerical account ID of the client to update. (required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/** Unique numerical account ID of the client to update. (required) */
public Update setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Invitations collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Invitations.List request = adexchangebuyer2.invitations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Invitations invitations() {
return new Invitations();
}
/**
* The "invitations" collection of methods.
*/
public class Invitations {
/**
* Creates and sends out an email invitation to access an Ad Exchange client buyer account.
*
* Create a request for the method "invitations.create".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user
should be associated with. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation}
* @return the request
*/
public Create create(java.lang.Long accountId, java.lang.Long clientAccountId, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation content) throws java.io.IOException {
Create result = new Create(accountId, clientAccountId, content);
initialize(result);
return result;
}
public class Create extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/invitations";
/**
* Creates and sends out an email invitation to access an Ad Exchange client buyer account.
*
* Create a request for the method "invitations.create".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user
should be associated with. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation}
* @since 1.13
*/
protected Create(java.lang.Long accountId, java.lang.Long clientAccountId, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation content) {
super(AdExchangeBuyerII.this, "POST", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setBearerToken(java.lang.String bearerToken) {
return (Create) super.setBearerToken(bearerToken);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPp(java.lang.Boolean pp) {
return (Create) super.setPp(pp);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public Create setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Numerical account ID of the client buyer that the user should be associated with.
* (required)
*/
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Numerical account ID of the client buyer that the user should be associated with. (required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/**
* Numerical account ID of the client buyer that the user should be associated with.
* (required)
*/
public Create setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Retrieves an existing client user invitation.
*
* Create a request for the method "invitations.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user invitation
to be retrieved is associated
* with. (required)
* @param invitationId Numerical identifier of the user invitation to retrieve. (required)
* @return the request
*/
public Get get(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long invitationId) throws java.io.IOException {
Get result = new Get(accountId, clientAccountId, invitationId);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/invitations/{invitationId}";
/**
* Retrieves an existing client user invitation.
*
* Create a request for the method "invitations.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user invitation
to be retrieved is associated
* with. (required)
* @param invitationId Numerical identifier of the user invitation to retrieve. (required)
* @since 1.13
*/
protected Get(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long invitationId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUserInvitation.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
this.invitationId = com.google.api.client.util.Preconditions.checkNotNull(invitationId, "Required parameter invitationId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public Get setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Numerical account ID of the client buyer that the user invitation to be retrieved is
* associated with. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Numerical account ID of the client buyer that the user invitation to be retrieved is associated
with. (required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/**
* Numerical account ID of the client buyer that the user invitation to be retrieved is
* associated with. (required)
*/
public Get setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
/** Numerical identifier of the user invitation to retrieve. (required) */
@com.google.api.client.util.Key
private java.lang.Long invitationId;
/** Numerical identifier of the user invitation to retrieve. (required)
*/
public java.lang.Long getInvitationId() {
return invitationId;
}
/** Numerical identifier of the user invitation to retrieve. (required) */
public Get setInvitationId(java.lang.Long invitationId) {
this.invitationId = invitationId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the client users invitations for a client with a given account ID.
*
* Create a request for the method "invitations.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer to list invitations for.
(required)
You must either specify
* a string representation of a
numerical account identifier or the `-` character
to list all
* the invitations for all the clients
of a given sponsor buyer.
* @return the request
*/
public List list(java.lang.Long accountId, java.lang.String clientAccountId) throws java.io.IOException {
List result = new List(accountId, clientAccountId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/invitations";
/**
* Lists all the client users invitations for a client with a given account ID.
*
* Create a request for the method "invitations.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer to list invitations for.
(required)
You must either specify
* a string representation of a
numerical account identifier or the `-` character
to list all
* the invitations for all the clients
of a given sponsor buyer.
* @since 1.13
*/
protected List(java.lang.Long accountId, java.lang.String clientAccountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListClientUserInvitationsResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public List setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Numerical account ID of the client buyer to list invitations for. (required) You must
* either specify a string representation of a numerical account identifier or the `-`
* character to list all the invitations for all the clients of a given sponsor buyer.
*/
@com.google.api.client.util.Key
private java.lang.String clientAccountId;
/** Numerical account ID of the client buyer to list invitations for. (required) You must either
specify a string representation of a numerical account identifier or the `-` character to list all
the invitations for all the clients of a given sponsor buyer.
*/
public java.lang.String getClientAccountId() {
return clientAccountId;
}
/**
* Numerical account ID of the client buyer to list invitations for. (required) You must
* either specify a string representation of a numerical account identifier or the `-`
* character to list all the invitations for all the clients of a given sponsor buyer.
*/
public List setClientAccountId(java.lang.String clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
/**
* Requested page size. Server may return fewer clients than requested. If unspecified,
* server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. Server may return fewer clients than requested. If unspecified, server will
pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. Server may return fewer clients than requested. If unspecified,
* server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientUserInvitationsResponse.nextPageToken returned from the previous
* call to the clients.invitations.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListClientUserInvitationsResponse.nextPageToken returned from the previous call to the
clients.invitations.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientUserInvitationsResponse.nextPageToken returned from the previous
* call to the clients.invitations.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Users collection.
*
* The typical use is:
*
* {@code AdExchangeBuyerII adexchangebuyer2 = new AdExchangeBuyerII(...);}
* {@code AdExchangeBuyerII.Users.List request = adexchangebuyer2.users().list(parameters ...)}
*
*
* @return the resource collection
*/
public Users users() {
return new Users();
}
/**
* The "users" collection of methods.
*/
public class Users {
/**
* Retrieves an existing client user.
*
* Create a request for the method "users.get".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer
that the user to be retrieved is associated with.
* (required)
* @param userId Numerical identifier of the user to retrieve. (required)
* @return the request
*/
public Get get(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long userId) throws java.io.IOException {
Get result = new Get(accountId, clientAccountId, userId);
initialize(result);
return result;
}
public class Get extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/users/{userId}";
/**
* Retrieves an existing client user.
*
* Create a request for the method "users.get".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer
that the user to be retrieved is associated with.
* (required)
* @param userId Numerical identifier of the user to retrieve. (required)
* @since 1.13
*/
protected Get(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long userId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public Get setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Numerical account ID of the client buyer that the user to be retrieved is associated
* with. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Numerical account ID of the client buyer that the user to be retrieved is associated with.
(required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/**
* Numerical account ID of the client buyer that the user to be retrieved is associated
* with. (required)
*/
public Get setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
/** Numerical identifier of the user to retrieve. (required) */
@com.google.api.client.util.Key
private java.lang.Long userId;
/** Numerical identifier of the user to retrieve. (required)
*/
public java.lang.Long getUserId() {
return userId;
}
/** Numerical identifier of the user to retrieve. (required) */
public Get setUserId(java.lang.Long userId) {
this.userId = userId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the known client users for a specified sponsor buyer account ID.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the sponsor buyer of the client to list users for.
(required)
* @param clientAccountId The account ID of the client buyer to list users for. (required)
You must specify either a string
* representation of a
numerical account identifier or the `-` character
to list all the
* client users for all the clients
of a given sponsor buyer.
* @return the request
*/
public List list(java.lang.Long accountId, java.lang.String clientAccountId) throws java.io.IOException {
List result = new List(accountId, clientAccountId);
initialize(result);
return result;
}
public class List extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/users";
/**
* Lists all the known client users for a specified sponsor buyer account ID.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the sponsor buyer of the client to list users for.
(required)
* @param clientAccountId The account ID of the client buyer to list users for. (required)
You must specify either a string
* representation of a
numerical account identifier or the `-` character
to list all the
* client users for all the clients
of a given sponsor buyer.
* @since 1.13
*/
protected List(java.lang.Long accountId, java.lang.String clientAccountId) {
super(AdExchangeBuyerII.this, "GET", REST_PATH, null, com.google.api.services.adexchangebuyer2.v2beta1.model.ListClientUsersResponse.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Numerical account ID of the sponsor buyer of the client to list users for. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the sponsor buyer of the client to list users for. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/**
* Numerical account ID of the sponsor buyer of the client to list users for. (required)
*/
public List setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* The account ID of the client buyer to list users for. (required) You must specify
* either a string representation of a numerical account identifier or the `-` character
* to list all the client users for all the clients of a given sponsor buyer.
*/
@com.google.api.client.util.Key
private java.lang.String clientAccountId;
/** The account ID of the client buyer to list users for. (required) You must specify either a string
representation of a numerical account identifier or the `-` character to list all the client users
for all the clients of a given sponsor buyer.
*/
public java.lang.String getClientAccountId() {
return clientAccountId;
}
/**
* The account ID of the client buyer to list users for. (required) You must specify
* either a string representation of a numerical account identifier or the `-` character
* to list all the client users for all the clients of a given sponsor buyer.
*/
public List setClientAccountId(java.lang.String clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
/**
* Requested page size. The server may return fewer clients than requested. If
* unspecified, the server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Requested page size. The server may return fewer clients than requested. If unspecified, the server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Requested page size. The server may return fewer clients than requested. If
* unspecified, the server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientUsersResponse.nextPageToken returned from the previous call to the
* accounts.clients.users.list method.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results the server should return. Typically, this is the value of
ListClientUsersResponse.nextPageToken returned from the previous call to the
accounts.clients.users.list method.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token identifying a page of results the server should return. Typically, this is the
* value of ListClientUsersResponse.nextPageToken returned from the previous call to the
* accounts.clients.users.list method.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing client user. Only the user status can be changed on update.
*
* Create a request for the method "users.update".
*
* This request holds the parameters needed by the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user to be retrieved
is associated with.
* (required)
* @param userId Numerical identifier of the user to retrieve. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser}
* @return the request
*/
public Update update(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long userId, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser content) throws java.io.IOException {
Update result = new Update(accountId, clientAccountId, userId, content);
initialize(result);
return result;
}
public class Update extends AdExchangeBuyerIIRequest {
private static final String REST_PATH = "v2beta1/accounts/{accountId}/clients/{clientAccountId}/users/{userId}";
/**
* Updates an existing client user. Only the user status can be changed on update.
*
* Create a request for the method "users.update".
*
* This request holds the parameters needed by the the adexchangebuyer2 server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param accountId Numerical account ID of the client's sponsor buyer. (required)
* @param clientAccountId Numerical account ID of the client buyer that the user to be retrieved
is associated with.
* (required)
* @param userId Numerical identifier of the user to retrieve. (required)
* @param content the {@link com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser}
* @since 1.13
*/
protected Update(java.lang.Long accountId, java.lang.Long clientAccountId, java.lang.Long userId, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser content) {
super(AdExchangeBuyerII.this, "PUT", REST_PATH, content, com.google.api.services.adexchangebuyer2.v2beta1.model.ClientUser.class);
this.accountId = com.google.api.client.util.Preconditions.checkNotNull(accountId, "Required parameter accountId must be specified.");
this.clientAccountId = com.google.api.client.util.Preconditions.checkNotNull(clientAccountId, "Required parameter clientAccountId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setBearerToken(java.lang.String bearerToken) {
return (Update) super.setBearerToken(bearerToken);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPp(java.lang.Boolean pp) {
return (Update) super.setPp(pp);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** Numerical account ID of the client's sponsor buyer. (required) */
@com.google.api.client.util.Key
private java.lang.Long accountId;
/** Numerical account ID of the client's sponsor buyer. (required)
*/
public java.lang.Long getAccountId() {
return accountId;
}
/** Numerical account ID of the client's sponsor buyer. (required) */
public Update setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Numerical account ID of the client buyer that the user to be retrieved is associated
* with. (required)
*/
@com.google.api.client.util.Key
private java.lang.Long clientAccountId;
/** Numerical account ID of the client buyer that the user to be retrieved is associated with.
(required)
*/
public java.lang.Long getClientAccountId() {
return clientAccountId;
}
/**
* Numerical account ID of the client buyer that the user to be retrieved is associated
* with. (required)
*/
public Update setClientAccountId(java.lang.Long clientAccountId) {
this.clientAccountId = clientAccountId;
return this;
}
/** Numerical identifier of the user to retrieve. (required) */
@com.google.api.client.util.Key
private java.lang.Long userId;
/** Numerical identifier of the user to retrieve. (required)
*/
public java.lang.Long getUserId() {
return userId;
}
/** Numerical identifier of the user to retrieve. (required) */
public Update setUserId(java.lang.Long userId) {
this.userId = userId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
}
}
/**
* Builder for {@link AdExchangeBuyerII}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link AdExchangeBuyerII}. */
@Override
public AdExchangeBuyerII build() {
return new AdExchangeBuyerII(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link AdExchangeBuyerIIRequestInitializer}.
*
* @since 1.12
*/
public Builder setAdExchangeBuyerIIRequestInitializer(
AdExchangeBuyerIIRequestInitializer adexchangebuyeriiRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(adexchangebuyeriiRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}