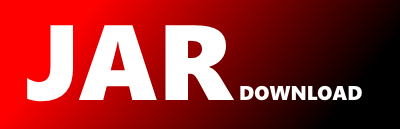
com.google.api.services.adsense.v2.Adsense Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.adsense.v2;
/**
* Service definition for Adsense (v2).
*
*
* The AdSense Management API allows publishers to access their inventory and run earnings and performance reports.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link AdsenseRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Adsense extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the AdSense Management API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://adsense.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://adsense.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Adsense(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Adsense(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Accounts collection.
*
* The typical use is:
*
* {@code Adsense adsense = new Adsense(...);}
* {@code Adsense.Accounts.List request = adsense.accounts().list(parameters ...)}
*
*
* @return the resource collection
*/
public Accounts accounts() {
return new Accounts();
}
/**
* The "accounts" collection of methods.
*/
public class Accounts {
/**
* Gets information about the selected AdSense account.
*
* Create a request for the method "accounts.get".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Account to get information about. Format: accounts/{account}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Gets information about the selected AdSense account.
*
* Create a request for the method "accounts.get".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Account to get information about. Format: accounts/{account}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.Account.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. Account to get information about. Format: accounts/{account} */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Account to get information about. Format: accounts/{account}
*/
public java.lang.String getName() {
return name;
}
/** Required. Account to get information about. Format: accounts/{account} */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the ad blocking recovery tag of an account.
*
* Create a request for the method "accounts.getAdBlockingRecoveryTag".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link GetAdBlockingRecoveryTag#execute()} method to invoke the remote
* operation.
*
* @param name Required. The name of the account to get the tag for. Format: accounts/{account}
* @return the request
*/
public GetAdBlockingRecoveryTag getAdBlockingRecoveryTag(java.lang.String name) throws java.io.IOException {
GetAdBlockingRecoveryTag result = new GetAdBlockingRecoveryTag(name);
initialize(result);
return result;
}
public class GetAdBlockingRecoveryTag extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}/adBlockingRecoveryTag";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Gets the ad blocking recovery tag of an account.
*
* Create a request for the method "accounts.getAdBlockingRecoveryTag".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link GetAdBlockingRecoveryTag#execute()} method to invoke the remote
* operation. {@link GetAdBlockingRecoveryTag#initialize(com.google.api.client.googleapis.serv
* ices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The name of the account to get the tag for. Format: accounts/{account}
* @since 1.13
*/
protected GetAdBlockingRecoveryTag(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.AdBlockingRecoveryTag.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetAdBlockingRecoveryTag set$Xgafv(java.lang.String $Xgafv) {
return (GetAdBlockingRecoveryTag) super.set$Xgafv($Xgafv);
}
@Override
public GetAdBlockingRecoveryTag setAccessToken(java.lang.String accessToken) {
return (GetAdBlockingRecoveryTag) super.setAccessToken(accessToken);
}
@Override
public GetAdBlockingRecoveryTag setAlt(java.lang.String alt) {
return (GetAdBlockingRecoveryTag) super.setAlt(alt);
}
@Override
public GetAdBlockingRecoveryTag setCallback(java.lang.String callback) {
return (GetAdBlockingRecoveryTag) super.setCallback(callback);
}
@Override
public GetAdBlockingRecoveryTag setFields(java.lang.String fields) {
return (GetAdBlockingRecoveryTag) super.setFields(fields);
}
@Override
public GetAdBlockingRecoveryTag setKey(java.lang.String key) {
return (GetAdBlockingRecoveryTag) super.setKey(key);
}
@Override
public GetAdBlockingRecoveryTag setOauthToken(java.lang.String oauthToken) {
return (GetAdBlockingRecoveryTag) super.setOauthToken(oauthToken);
}
@Override
public GetAdBlockingRecoveryTag setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetAdBlockingRecoveryTag) super.setPrettyPrint(prettyPrint);
}
@Override
public GetAdBlockingRecoveryTag setQuotaUser(java.lang.String quotaUser) {
return (GetAdBlockingRecoveryTag) super.setQuotaUser(quotaUser);
}
@Override
public GetAdBlockingRecoveryTag setUploadType(java.lang.String uploadType) {
return (GetAdBlockingRecoveryTag) super.setUploadType(uploadType);
}
@Override
public GetAdBlockingRecoveryTag setUploadProtocol(java.lang.String uploadProtocol) {
return (GetAdBlockingRecoveryTag) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the account to get the tag for. Format: accounts/{account} */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the account to get the tag for. Format: accounts/{account}
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the account to get the tag for. Format: accounts/{account} */
public GetAdBlockingRecoveryTag setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetAdBlockingRecoveryTag set(String parameterName, Object value) {
return (GetAdBlockingRecoveryTag) super.set(parameterName, value);
}
}
/**
* Lists all accounts available to this user.
*
* Create a request for the method "accounts.list".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends AdsenseRequest {
private static final String REST_PATH = "v2/accounts";
/**
* Lists all accounts available to this user.
*
* Create a request for the method "accounts.list".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListAccountsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* The maximum number of accounts to include in the response, used for paging. If unspecified,
* at most 10000 accounts will be returned. The maximum value is 10000; values above 10000
* will be coerced to 10000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of accounts to include in the response, used for paging. If unspecified, at most
10000 accounts will be returned. The maximum value is 10000; values above 10000 will be coerced to
10000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of accounts to include in the response, used for paging. If unspecified,
* at most 10000 accounts will be returned. The maximum value is 10000; values above 10000
* will be coerced to 10000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListAccounts` call. Provide this to retrieve the
* subsequent page. When paginating, all other parameters provided to `ListAccounts` must
* match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListAccounts` call. Provide this to retrieve the subsequent
page. When paginating, all other parameters provided to `ListAccounts` must match the call that
provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListAccounts` call. Provide this to retrieve the
* subsequent page. When paginating, all other parameters provided to `ListAccounts` must
* match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Lists all accounts directly managed by the given AdSense account.
*
* Create a request for the method "accounts.listChildAccounts".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link ListChildAccounts#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent account, which owns the child accounts. Format: accounts/{account}
* @return the request
*/
public ListChildAccounts listChildAccounts(java.lang.String parent) throws java.io.IOException {
ListChildAccounts result = new ListChildAccounts(parent);
initialize(result);
return result;
}
public class ListChildAccounts extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}:listChildAccounts";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Lists all accounts directly managed by the given AdSense account.
*
* Create a request for the method "accounts.listChildAccounts".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link ListChildAccounts#execute()} method to invoke the remote operation.
* {@link ListChildAccounts#initialize(com.google.api.client.googleapis.services.AbstractGoogl
* eClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param parent Required. The parent account, which owns the child accounts. Format: accounts/{account}
* @since 1.13
*/
protected ListChildAccounts(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListChildAccountsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListChildAccounts set$Xgafv(java.lang.String $Xgafv) {
return (ListChildAccounts) super.set$Xgafv($Xgafv);
}
@Override
public ListChildAccounts setAccessToken(java.lang.String accessToken) {
return (ListChildAccounts) super.setAccessToken(accessToken);
}
@Override
public ListChildAccounts setAlt(java.lang.String alt) {
return (ListChildAccounts) super.setAlt(alt);
}
@Override
public ListChildAccounts setCallback(java.lang.String callback) {
return (ListChildAccounts) super.setCallback(callback);
}
@Override
public ListChildAccounts setFields(java.lang.String fields) {
return (ListChildAccounts) super.setFields(fields);
}
@Override
public ListChildAccounts setKey(java.lang.String key) {
return (ListChildAccounts) super.setKey(key);
}
@Override
public ListChildAccounts setOauthToken(java.lang.String oauthToken) {
return (ListChildAccounts) super.setOauthToken(oauthToken);
}
@Override
public ListChildAccounts setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListChildAccounts) super.setPrettyPrint(prettyPrint);
}
@Override
public ListChildAccounts setQuotaUser(java.lang.String quotaUser) {
return (ListChildAccounts) super.setQuotaUser(quotaUser);
}
@Override
public ListChildAccounts setUploadType(java.lang.String uploadType) {
return (ListChildAccounts) super.setUploadType(uploadType);
}
@Override
public ListChildAccounts setUploadProtocol(java.lang.String uploadProtocol) {
return (ListChildAccounts) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent account, which owns the child accounts. Format: accounts/{account}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent account, which owns the child accounts. Format: accounts/{account}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent account, which owns the child accounts. Format: accounts/{account}
*/
public ListChildAccounts setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of accounts to include in the response, used for paging. If unspecified,
* at most 10000 accounts will be returned. The maximum value is 10000; values above 10000
* will be coerced to 10000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of accounts to include in the response, used for paging. If unspecified, at most
10000 accounts will be returned. The maximum value is 10000; values above 10000 will be coerced to
10000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of accounts to include in the response, used for paging. If unspecified,
* at most 10000 accounts will be returned. The maximum value is 10000; values above 10000
* will be coerced to 10000.
*/
public ListChildAccounts setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListChildAccounts` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to `ListChildAccounts`
* must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListChildAccounts` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListChildAccounts` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListChildAccounts` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to `ListChildAccounts`
* must match the call that provided the page token.
*/
public ListChildAccounts setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListChildAccounts set(String parameterName, Object value) {
return (ListChildAccounts) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Adclients collection.
*
* The typical use is:
*
* {@code Adsense adsense = new Adsense(...);}
* {@code Adsense.Adclients.List request = adsense.adclients().list(parameters ...)}
*
*
* @return the resource collection
*/
public Adclients adclients() {
return new Adclients();
}
/**
* The "adclients" collection of methods.
*/
public class Adclients {
/**
* Gets the ad client from the given resource name.
*
* Create a request for the method "adclients.get".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the ad client to retrieve. Format: accounts/{account}/adclients/{adclient}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+$");
/**
* Gets the ad client from the given resource name.
*
* Create a request for the method "adclients.get".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the ad client to retrieve. Format: accounts/{account}/adclients/{adclient}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.AdClient.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the ad client to retrieve. Format:
* accounts/{account}/adclients/{adclient}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the ad client to retrieve. Format: accounts/{account}/adclients/{adclient}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the ad client to retrieve. Format:
* accounts/{account}/adclients/{adclient}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the AdSense code for a given ad client. This returns what was previously known as the 'auto
* ad code'. This is only supported for ad clients with a product_code of AFC. For more information,
* see [About the AdSense code](https://support.google.com/adsense/answer/9274634).
*
* Create a request for the method "adclients.getAdcode".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link GetAdcode#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the ad client for which to get the adcode. Format:
* accounts/{account}/adclients/{adclient}
* @return the request
*/
public GetAdcode getAdcode(java.lang.String name) throws java.io.IOException {
GetAdcode result = new GetAdcode(name);
initialize(result);
return result;
}
public class GetAdcode extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}/adcode";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+$");
/**
* Gets the AdSense code for a given ad client. This returns what was previously known as the
* 'auto ad code'. This is only supported for ad clients with a product_code of AFC. For more
* information, see [About the AdSense code](https://support.google.com/adsense/answer/9274634).
*
* Create a request for the method "adclients.getAdcode".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link GetAdcode#execute()} method to invoke the remote operation.
* {@link
* GetAdcode#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the ad client for which to get the adcode. Format:
* accounts/{account}/adclients/{adclient}
* @since 1.13
*/
protected GetAdcode(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.AdClientAdCode.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetAdcode set$Xgafv(java.lang.String $Xgafv) {
return (GetAdcode) super.set$Xgafv($Xgafv);
}
@Override
public GetAdcode setAccessToken(java.lang.String accessToken) {
return (GetAdcode) super.setAccessToken(accessToken);
}
@Override
public GetAdcode setAlt(java.lang.String alt) {
return (GetAdcode) super.setAlt(alt);
}
@Override
public GetAdcode setCallback(java.lang.String callback) {
return (GetAdcode) super.setCallback(callback);
}
@Override
public GetAdcode setFields(java.lang.String fields) {
return (GetAdcode) super.setFields(fields);
}
@Override
public GetAdcode setKey(java.lang.String key) {
return (GetAdcode) super.setKey(key);
}
@Override
public GetAdcode setOauthToken(java.lang.String oauthToken) {
return (GetAdcode) super.setOauthToken(oauthToken);
}
@Override
public GetAdcode setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetAdcode) super.setPrettyPrint(prettyPrint);
}
@Override
public GetAdcode setQuotaUser(java.lang.String quotaUser) {
return (GetAdcode) super.setQuotaUser(quotaUser);
}
@Override
public GetAdcode setUploadType(java.lang.String uploadType) {
return (GetAdcode) super.setUploadType(uploadType);
}
@Override
public GetAdcode setUploadProtocol(java.lang.String uploadProtocol) {
return (GetAdcode) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the ad client for which to get the adcode. Format:
* accounts/{account}/adclients/{adclient}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the ad client for which to get the adcode. Format:
accounts/{account}/adclients/{adclient}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the ad client for which to get the adcode. Format:
* accounts/{account}/adclients/{adclient}
*/
public GetAdcode setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetAdcode set(String parameterName, Object value) {
return (GetAdcode) super.set(parameterName, value);
}
}
/**
* Lists all the ad clients available in an account.
*
* Create a request for the method "adclients.list".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The account which owns the collection of ad clients. Format: accounts/{account}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}/adclients";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Lists all the ad clients available in an account.
*
* Create a request for the method "adclients.list".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The account which owns the collection of ad clients. Format: accounts/{account}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListAdClientsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The account which owns the collection of ad clients. Format: accounts/{account}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The account which owns the collection of ad clients. Format: accounts/{account}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The account which owns the collection of ad clients. Format: accounts/{account}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of ad clients to include in the response, used for paging. If
* unspecified, at most 10000 ad clients will be returned. The maximum value is 10000;
* values above 10000 will be coerced to 10000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of ad clients to include in the response, used for paging. If unspecified, at
most 10000 ad clients will be returned. The maximum value is 10000; values above 10000 will be
coerced to 10000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of ad clients to include in the response, used for paging. If
* unspecified, at most 10000 ad clients will be returned. The maximum value is 10000;
* values above 10000 will be coerced to 10000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListAdClients` call. Provide this to retrieve the
* subsequent page. When paginating, all other parameters provided to `ListAdClients` must
* match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListAdClients` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListAdClients` must match the
call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListAdClients` call. Provide this to retrieve the
* subsequent page. When paginating, all other parameters provided to `ListAdClients` must
* match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Adunits collection.
*
* The typical use is:
*
* {@code Adsense adsense = new Adsense(...);}
* {@code Adsense.Adunits.List request = adsense.adunits().list(parameters ...)}
*
*
* @return the resource collection
*/
public Adunits adunits() {
return new Adunits();
}
/**
* The "adunits" collection of methods.
*/
public class Adunits {
/**
* Creates an ad unit. This method can be called only by a restricted set of projects, which are
* usually owned by [AdSense for Platforms](https://developers.google.com/adsense/platforms/)
* publishers. Contact your account manager if you need to use this method. Note that ad units can
* only be created for ad clients with an "AFC" product code. For more info see the [AdClient
* resource](/adsense/management/reference/rest/v2/accounts.adclients). For now, this method can
* only be used to create `DISPLAY` ad units. See: https://support.google.com/adsense/answer/9183566
*
* Create a request for the method "adunits.create".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Ad client to create an ad unit under. Format: accounts/{account}/adclients/{adclient}
* @param content the {@link com.google.api.services.adsense.v2.model.AdUnit}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.adsense.v2.model.AdUnit content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}/adunits";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+$");
/**
* Creates an ad unit. This method can be called only by a restricted set of projects, which are
* usually owned by [AdSense for Platforms](https://developers.google.com/adsense/platforms/)
* publishers. Contact your account manager if you need to use this method. Note that ad units can
* only be created for ad clients with an "AFC" product code. For more info see the [AdClient
* resource](/adsense/management/reference/rest/v2/accounts.adclients). For now, this method can
* only be used to create `DISPLAY` ad units. See:
* https://support.google.com/adsense/answer/9183566
*
* Create a request for the method "adunits.create".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Ad client to create an ad unit under. Format: accounts/{account}/adclients/{adclient}
* @param content the {@link com.google.api.services.adsense.v2.model.AdUnit}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.adsense.v2.model.AdUnit content) {
super(Adsense.this, "POST", REST_PATH, content, com.google.api.services.adsense.v2.model.AdUnit.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Ad client to create an ad unit under. Format:
* accounts/{account}/adclients/{adclient}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Ad client to create an ad unit under. Format: accounts/{account}/adclients/{adclient}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Ad client to create an ad unit under. Format:
* accounts/{account}/adclients/{adclient}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets an ad unit from a specified account and ad client.
*
* Create a request for the method "adunits.get".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. AdUnit to get information about. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
/**
* Gets an ad unit from a specified account and ad client.
*
* Create a request for the method "adunits.get".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. AdUnit to get information about. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.AdUnit.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. AdUnit to get information about. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. AdUnit to get information about. Format:
accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. AdUnit to get information about. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the ad unit code for a given ad unit. For more information, see [About the AdSense
* code](https://support.google.com/adsense/answer/9274634) and [Where to place the ad code in your
* HTML](https://support.google.com/adsense/answer/9190028).
*
* Create a request for the method "adunits.getAdcode".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link GetAdcode#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the adunit for which to get the adcode. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
* @return the request
*/
public GetAdcode getAdcode(java.lang.String name) throws java.io.IOException {
GetAdcode result = new GetAdcode(name);
initialize(result);
return result;
}
public class GetAdcode extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}/adcode";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
/**
* Gets the ad unit code for a given ad unit. For more information, see [About the AdSense
* code](https://support.google.com/adsense/answer/9274634) and [Where to place the ad code in
* your HTML](https://support.google.com/adsense/answer/9190028).
*
* Create a request for the method "adunits.getAdcode".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link GetAdcode#execute()} method to invoke the remote operation.
* {@link
* GetAdcode#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the adunit for which to get the adcode. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
* @since 1.13
*/
protected GetAdcode(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.AdUnitAdCode.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetAdcode set$Xgafv(java.lang.String $Xgafv) {
return (GetAdcode) super.set$Xgafv($Xgafv);
}
@Override
public GetAdcode setAccessToken(java.lang.String accessToken) {
return (GetAdcode) super.setAccessToken(accessToken);
}
@Override
public GetAdcode setAlt(java.lang.String alt) {
return (GetAdcode) super.setAlt(alt);
}
@Override
public GetAdcode setCallback(java.lang.String callback) {
return (GetAdcode) super.setCallback(callback);
}
@Override
public GetAdcode setFields(java.lang.String fields) {
return (GetAdcode) super.setFields(fields);
}
@Override
public GetAdcode setKey(java.lang.String key) {
return (GetAdcode) super.setKey(key);
}
@Override
public GetAdcode setOauthToken(java.lang.String oauthToken) {
return (GetAdcode) super.setOauthToken(oauthToken);
}
@Override
public GetAdcode setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetAdcode) super.setPrettyPrint(prettyPrint);
}
@Override
public GetAdcode setQuotaUser(java.lang.String quotaUser) {
return (GetAdcode) super.setQuotaUser(quotaUser);
}
@Override
public GetAdcode setUploadType(java.lang.String uploadType) {
return (GetAdcode) super.setUploadType(uploadType);
}
@Override
public GetAdcode setUploadProtocol(java.lang.String uploadProtocol) {
return (GetAdcode) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the adunit for which to get the adcode. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the adunit for which to get the adcode. Format:
accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the adunit for which to get the adcode. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
public GetAdcode setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetAdcode set(String parameterName, Object value) {
return (GetAdcode) super.set(parameterName, value);
}
}
/**
* Lists all ad units under a specified account and ad client.
*
* Create a request for the method "adunits.list".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The ad client which owns the collection of ad units. Format:
* accounts/{account}/adclients/{adclient}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}/adunits";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+$");
/**
* Lists all ad units under a specified account and ad client.
*
* Create a request for the method "adunits.list".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The ad client which owns the collection of ad units. Format:
* accounts/{account}/adclients/{adclient}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListAdUnitsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The ad client which owns the collection of ad units. Format:
* accounts/{account}/adclients/{adclient}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The ad client which owns the collection of ad units. Format:
accounts/{account}/adclients/{adclient}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The ad client which owns the collection of ad units. Format:
* accounts/{account}/adclients/{adclient}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of ad units to include in the response, used for paging. If
* unspecified, at most 10000 ad units will be returned. The maximum value is 10000;
* values above 10000 will be coerced to 10000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of ad units to include in the response, used for paging. If unspecified, at most
10000 ad units will be returned. The maximum value is 10000; values above 10000 will be coerced to
10000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of ad units to include in the response, used for paging. If
* unspecified, at most 10000 ad units will be returned. The maximum value is 10000;
* values above 10000 will be coerced to 10000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListAdUnits` call. Provide this to retrieve the
* subsequent page. When paginating, all other parameters provided to `ListAdUnits` must
* match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListAdUnits` call. Provide this to retrieve the subsequent
page. When paginating, all other parameters provided to `ListAdUnits` must match the call that
provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListAdUnits` call. Provide this to retrieve the
* subsequent page. When paginating, all other parameters provided to `ListAdUnits` must
* match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Lists all the custom channels available for an ad unit.
*
* Create a request for the method "adunits.listLinkedCustomChannels".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link ListLinkedCustomChannels#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The ad unit which owns the collection of custom channels. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
* @return the request
*/
public ListLinkedCustomChannels listLinkedCustomChannels(java.lang.String parent) throws java.io.IOException {
ListLinkedCustomChannels result = new ListLinkedCustomChannels(parent);
initialize(result);
return result;
}
public class ListLinkedCustomChannels extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}:listLinkedCustomChannels";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
/**
* Lists all the custom channels available for an ad unit.
*
* Create a request for the method "adunits.listLinkedCustomChannels".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link ListLinkedCustomChannels#execute()} method to invoke the remote
* operation. {@link ListLinkedCustomChannels#initialize(com.google.api.client.googleapis.serv
* ices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. The ad unit which owns the collection of custom channels. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
* @since 1.13
*/
protected ListLinkedCustomChannels(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListLinkedCustomChannelsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListLinkedCustomChannels set$Xgafv(java.lang.String $Xgafv) {
return (ListLinkedCustomChannels) super.set$Xgafv($Xgafv);
}
@Override
public ListLinkedCustomChannels setAccessToken(java.lang.String accessToken) {
return (ListLinkedCustomChannels) super.setAccessToken(accessToken);
}
@Override
public ListLinkedCustomChannels setAlt(java.lang.String alt) {
return (ListLinkedCustomChannels) super.setAlt(alt);
}
@Override
public ListLinkedCustomChannels setCallback(java.lang.String callback) {
return (ListLinkedCustomChannels) super.setCallback(callback);
}
@Override
public ListLinkedCustomChannels setFields(java.lang.String fields) {
return (ListLinkedCustomChannels) super.setFields(fields);
}
@Override
public ListLinkedCustomChannels setKey(java.lang.String key) {
return (ListLinkedCustomChannels) super.setKey(key);
}
@Override
public ListLinkedCustomChannels setOauthToken(java.lang.String oauthToken) {
return (ListLinkedCustomChannels) super.setOauthToken(oauthToken);
}
@Override
public ListLinkedCustomChannels setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListLinkedCustomChannels) super.setPrettyPrint(prettyPrint);
}
@Override
public ListLinkedCustomChannels setQuotaUser(java.lang.String quotaUser) {
return (ListLinkedCustomChannels) super.setQuotaUser(quotaUser);
}
@Override
public ListLinkedCustomChannels setUploadType(java.lang.String uploadType) {
return (ListLinkedCustomChannels) super.setUploadType(uploadType);
}
@Override
public ListLinkedCustomChannels setUploadProtocol(java.lang.String uploadProtocol) {
return (ListLinkedCustomChannels) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The ad unit which owns the collection of custom channels. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The ad unit which owns the collection of custom channels. Format:
accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The ad unit which owns the collection of custom channels. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
public ListLinkedCustomChannels setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of custom channels to include in the response, used for paging. If
* unspecified, at most 10000 custom channels will be returned. The maximum value is
* 10000; values above 10000 will be coerced to 10000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of custom channels to include in the response, used for paging. If unspecified,
at most 10000 custom channels will be returned. The maximum value is 10000; values above 10000 will
be coerced to 10000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of custom channels to include in the response, used for paging. If
* unspecified, at most 10000 custom channels will be returned. The maximum value is
* 10000; values above 10000 will be coerced to 10000.
*/
public ListLinkedCustomChannels setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListLinkedCustomChannels` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListLinkedCustomChannels` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListLinkedCustomChannels` call. Provide this to retrieve
the subsequent page. When paginating, all other parameters provided to `ListLinkedCustomChannels`
must match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListLinkedCustomChannels` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListLinkedCustomChannels` must match the call that provided the page token.
*/
public ListLinkedCustomChannels setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListLinkedCustomChannels set(String parameterName, Object value) {
return (ListLinkedCustomChannels) super.set(parameterName, value);
}
}
/**
* Updates an ad unit. This method can be called only by a restricted set of projects, which are
* usually owned by [AdSense for Platforms](https://developers.google.com/adsense/platforms/)
* publishers. Contact your account manager if you need to use this method. For now, this method can
* only be used to update `DISPLAY` ad units. See: https://support.google.com/adsense/answer/9183566
*
* Create a request for the method "adunits.patch".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Resource name of the ad unit. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
* @param content the {@link com.google.api.services.adsense.v2.model.AdUnit}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.adsense.v2.model.AdUnit content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
/**
* Updates an ad unit. This method can be called only by a restricted set of projects, which are
* usually owned by [AdSense for Platforms](https://developers.google.com/adsense/platforms/)
* publishers. Contact your account manager if you need to use this method. For now, this method
* can only be used to update `DISPLAY` ad units. See:
* https://support.google.com/adsense/answer/9183566
*
* Create a request for the method "adunits.patch".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Resource name of the ad unit. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
* @param content the {@link com.google.api.services.adsense.v2.model.AdUnit}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.adsense.v2.model.AdUnit content) {
super(Adsense.this, "PATCH", REST_PATH, content, com.google.api.services.adsense.v2.model.AdUnit.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Resource name of the ad unit. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Resource name of the ad unit. Format:
accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Resource name of the ad unit. Format:
* accounts/{account}/adclients/{adclient}/adunits/{adunit}
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/adunits/[^/]+$");
}
this.name = name;
return this;
}
/** The list of fields to update. If empty, a full update is performed. */
@com.google.api.client.util.Key
private String updateMask;
/** The list of fields to update. If empty, a full update is performed.
*/
public String getUpdateMask() {
return updateMask;
}
/** The list of fields to update. If empty, a full update is performed. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Customchannels collection.
*
* The typical use is:
*
* {@code Adsense adsense = new Adsense(...);}
* {@code Adsense.Customchannels.List request = adsense.customchannels().list(parameters ...)}
*
*
* @return the resource collection
*/
public Customchannels customchannels() {
return new Customchannels();
}
/**
* The "customchannels" collection of methods.
*/
public class Customchannels {
/**
* Creates a custom channel. This method can be called only by a restricted set of projects, which
* are usually owned by [AdSense for Platforms](https://developers.google.com/adsense/platforms/)
* publishers. Contact your account manager if you need to use this method.
*
* Create a request for the method "customchannels.create".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The ad client to create a custom channel under. Format:
* accounts/{account}/adclients/{adclient}
* @param content the {@link com.google.api.services.adsense.v2.model.CustomChannel}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.adsense.v2.model.CustomChannel content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}/customchannels";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+$");
/**
* Creates a custom channel. This method can be called only by a restricted set of projects, which
* are usually owned by [AdSense for Platforms](https://developers.google.com/adsense/platforms/)
* publishers. Contact your account manager if you need to use this method.
*
* Create a request for the method "customchannels.create".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The ad client to create a custom channel under. Format:
* accounts/{account}/adclients/{adclient}
* @param content the {@link com.google.api.services.adsense.v2.model.CustomChannel}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.adsense.v2.model.CustomChannel content) {
super(Adsense.this, "POST", REST_PATH, content, com.google.api.services.adsense.v2.model.CustomChannel.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The ad client to create a custom channel under. Format:
* accounts/{account}/adclients/{adclient}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The ad client to create a custom channel under. Format:
accounts/{account}/adclients/{adclient}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The ad client to create a custom channel under. Format:
* accounts/{account}/adclients/{adclient}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a custom channel. This method can be called only by a restricted set of projects, which
* are usually owned by [AdSense for Platforms](https://developers.google.com/adsense/platforms/)
* publishers. Contact your account manager if you need to use this method.
*
* Create a request for the method "customchannels.delete".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the custom channel to delete. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
/**
* Deletes a custom channel. This method can be called only by a restricted set of projects, which
* are usually owned by [AdSense for Platforms](https://developers.google.com/adsense/platforms/)
* publishers. Contact your account manager if you need to use this method.
*
* Create a request for the method "customchannels.delete".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the custom channel to delete. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Adsense.this, "DELETE", REST_PATH, null, com.google.api.services.adsense.v2.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the custom channel to delete. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the custom channel to delete. Format:
accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the custom channel to delete. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets information about the selected custom channel.
*
* Create a request for the method "customchannels.get".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the custom channel. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
/**
* Gets information about the selected custom channel.
*
* Create a request for the method "customchannels.get".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the custom channel. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.CustomChannel.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the custom channel. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the custom channel. Format:
accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the custom channel. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the custom channels available in an ad client.
*
* Create a request for the method "customchannels.list".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The ad client which owns the collection of custom channels. Format:
* accounts/{account}/adclients/{adclient}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}/customchannels";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+$");
/**
* Lists all the custom channels available in an ad client.
*
* Create a request for the method "customchannels.list".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The ad client which owns the collection of custom channels. Format:
* accounts/{account}/adclients/{adclient}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListCustomChannelsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The ad client which owns the collection of custom channels. Format:
* accounts/{account}/adclients/{adclient}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The ad client which owns the collection of custom channels. Format:
accounts/{account}/adclients/{adclient}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The ad client which owns the collection of custom channels. Format:
* accounts/{account}/adclients/{adclient}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of custom channels to include in the response, used for paging. If
* unspecified, at most 10000 custom channels will be returned. The maximum value is
* 10000; values above 10000 will be coerced to 10000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of custom channels to include in the response, used for paging. If unspecified,
at most 10000 custom channels will be returned. The maximum value is 10000; values above 10000 will
be coerced to 10000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of custom channels to include in the response, used for paging. If
* unspecified, at most 10000 custom channels will be returned. The maximum value is
* 10000; values above 10000 will be coerced to 10000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListCustomChannels` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListCustomChannels` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListCustomChannels` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListCustomChannels` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListCustomChannels` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListCustomChannels` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Lists all the ad units available for a custom channel.
*
* Create a request for the method "customchannels.listLinkedAdUnits".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link ListLinkedAdUnits#execute()} method to invoke the remote operation.
*
* @param parent Required. The custom channel which owns the collection of ad units. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
* @return the request
*/
public ListLinkedAdUnits listLinkedAdUnits(java.lang.String parent) throws java.io.IOException {
ListLinkedAdUnits result = new ListLinkedAdUnits(parent);
initialize(result);
return result;
}
public class ListLinkedAdUnits extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}:listLinkedAdUnits";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
/**
* Lists all the ad units available for a custom channel.
*
* Create a request for the method "customchannels.listLinkedAdUnits".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link ListLinkedAdUnits#execute()} method to invoke the remote operation.
* {@link ListLinkedAdUnits#initialize(com.google.api.client.googleapis.services.AbstractGoogl
* eClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param parent Required. The custom channel which owns the collection of ad units. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
* @since 1.13
*/
protected ListLinkedAdUnits(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListLinkedAdUnitsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListLinkedAdUnits set$Xgafv(java.lang.String $Xgafv) {
return (ListLinkedAdUnits) super.set$Xgafv($Xgafv);
}
@Override
public ListLinkedAdUnits setAccessToken(java.lang.String accessToken) {
return (ListLinkedAdUnits) super.setAccessToken(accessToken);
}
@Override
public ListLinkedAdUnits setAlt(java.lang.String alt) {
return (ListLinkedAdUnits) super.setAlt(alt);
}
@Override
public ListLinkedAdUnits setCallback(java.lang.String callback) {
return (ListLinkedAdUnits) super.setCallback(callback);
}
@Override
public ListLinkedAdUnits setFields(java.lang.String fields) {
return (ListLinkedAdUnits) super.setFields(fields);
}
@Override
public ListLinkedAdUnits setKey(java.lang.String key) {
return (ListLinkedAdUnits) super.setKey(key);
}
@Override
public ListLinkedAdUnits setOauthToken(java.lang.String oauthToken) {
return (ListLinkedAdUnits) super.setOauthToken(oauthToken);
}
@Override
public ListLinkedAdUnits setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListLinkedAdUnits) super.setPrettyPrint(prettyPrint);
}
@Override
public ListLinkedAdUnits setQuotaUser(java.lang.String quotaUser) {
return (ListLinkedAdUnits) super.setQuotaUser(quotaUser);
}
@Override
public ListLinkedAdUnits setUploadType(java.lang.String uploadType) {
return (ListLinkedAdUnits) super.setUploadType(uploadType);
}
@Override
public ListLinkedAdUnits setUploadProtocol(java.lang.String uploadProtocol) {
return (ListLinkedAdUnits) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The custom channel which owns the collection of ad units. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The custom channel which owns the collection of ad units. Format:
accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The custom channel which owns the collection of ad units. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
public ListLinkedAdUnits setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of ad units to include in the response, used for paging. If
* unspecified, at most 10000 ad units will be returned. The maximum value is 10000;
* values above 10000 will be coerced to 10000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of ad units to include in the response, used for paging. If unspecified, at most
10000 ad units will be returned. The maximum value is 10000; values above 10000 will be coerced to
10000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of ad units to include in the response, used for paging. If
* unspecified, at most 10000 ad units will be returned. The maximum value is 10000;
* values above 10000 will be coerced to 10000.
*/
public ListLinkedAdUnits setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListLinkedAdUnits` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListLinkedAdUnits` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListLinkedAdUnits` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListLinkedAdUnits` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListLinkedAdUnits` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListLinkedAdUnits` must match the call that provided the page token.
*/
public ListLinkedAdUnits setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListLinkedAdUnits set(String parameterName, Object value) {
return (ListLinkedAdUnits) super.set(parameterName, value);
}
}
/**
* Updates a custom channel. This method can be called only by a restricted set of projects, which
* are usually owned by [AdSense for Platforms](https://developers.google.com/adsense/platforms/)
* publishers. Contact your account manager if you need to use this method.
*
* Create a request for the method "customchannels.patch".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Resource name of the custom channel. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
* @param content the {@link com.google.api.services.adsense.v2.model.CustomChannel}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.adsense.v2.model.CustomChannel content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
/**
* Updates a custom channel. This method can be called only by a restricted set of projects, which
* are usually owned by [AdSense for Platforms](https://developers.google.com/adsense/platforms/)
* publishers. Contact your account manager if you need to use this method.
*
* Create a request for the method "customchannels.patch".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Resource name of the custom channel. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
* @param content the {@link com.google.api.services.adsense.v2.model.CustomChannel}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.adsense.v2.model.CustomChannel content) {
super(Adsense.this, "PATCH", REST_PATH, content, com.google.api.services.adsense.v2.model.CustomChannel.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. Resource name of the custom channel. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Resource name of the custom channel. Format:
accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Resource name of the custom channel. Format:
* accounts/{account}/adclients/{adclient}/customchannels/{customchannel}
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/customchannels/[^/]+$");
}
this.name = name;
return this;
}
/** The list of fields to update. If empty, a full update is performed. */
@com.google.api.client.util.Key
private String updateMask;
/** The list of fields to update. If empty, a full update is performed.
*/
public String getUpdateMask() {
return updateMask;
}
/** The list of fields to update. If empty, a full update is performed. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Urlchannels collection.
*
* The typical use is:
*
* {@code Adsense adsense = new Adsense(...);}
* {@code Adsense.Urlchannels.List request = adsense.urlchannels().list(parameters ...)}
*
*
* @return the resource collection
*/
public Urlchannels urlchannels() {
return new Urlchannels();
}
/**
* The "urlchannels" collection of methods.
*/
public class Urlchannels {
/**
* Gets information about the selected url channel.
*
* Create a request for the method "urlchannels.get".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the url channel to retrieve. Format:
* accounts/{account}/adclients/{adclient}/urlchannels/{urlchannel}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+/urlchannels/[^/]+$");
/**
* Gets information about the selected url channel.
*
* Create a request for the method "urlchannels.get".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the url channel to retrieve. Format:
* accounts/{account}/adclients/{adclient}/urlchannels/{urlchannel}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.UrlChannel.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/urlchannels/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the url channel to retrieve. Format:
* accounts/{account}/adclients/{adclient}/urlchannels/{urlchannel}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the url channel to retrieve. Format:
accounts/{account}/adclients/{adclient}/urlchannels/{urlchannel}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the url channel to retrieve. Format:
* accounts/{account}/adclients/{adclient}/urlchannels/{urlchannel}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+/urlchannels/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists active url channels.
*
* Create a request for the method "urlchannels.list".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The ad client which owns the collection of url channels. Format:
* accounts/{account}/adclients/{adclient}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}/urlchannels";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/adclients/[^/]+$");
/**
* Lists active url channels.
*
* Create a request for the method "urlchannels.list".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The ad client which owns the collection of url channels. Format:
* accounts/{account}/adclients/{adclient}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListUrlChannelsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The ad client which owns the collection of url channels. Format:
* accounts/{account}/adclients/{adclient}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The ad client which owns the collection of url channels. Format:
accounts/{account}/adclients/{adclient}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The ad client which owns the collection of url channels. Format:
* accounts/{account}/adclients/{adclient}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+/adclients/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of url channels to include in the response, used for paging. If
* unspecified, at most 10000 url channels will be returned. The maximum value is 10000;
* values above 10000 will be coerced to 10000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of url channels to include in the response, used for paging. If unspecified, at
most 10000 url channels will be returned. The maximum value is 10000; values above 10000 will be
coerced to 10000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of url channels to include in the response, used for paging. If
* unspecified, at most 10000 url channels will be returned. The maximum value is 10000;
* values above 10000 will be coerced to 10000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListUrlChannels` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to
* `ListUrlChannels` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListUrlChannels` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListUrlChannels` must match the
call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListUrlChannels` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to
* `ListUrlChannels` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Alerts collection.
*
* The typical use is:
*
* {@code Adsense adsense = new Adsense(...);}
* {@code Adsense.Alerts.List request = adsense.alerts().list(parameters ...)}
*
*
* @return the resource collection
*/
public Alerts alerts() {
return new Alerts();
}
/**
* The "alerts" collection of methods.
*/
public class Alerts {
/**
* Lists all the alerts available in an account.
*
* Create a request for the method "alerts.list".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The account which owns the collection of alerts. Format: accounts/{account}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}/alerts";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Lists all the alerts available in an account.
*
* Create a request for the method "alerts.list".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The account which owns the collection of alerts. Format: accounts/{account}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListAlertsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The account which owns the collection of alerts. Format: accounts/{account}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The account which owns the collection of alerts. Format: accounts/{account}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The account which owns the collection of alerts. Format: accounts/{account}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language to use for translating alert messages. If unspecified, this defaults to the
* user's display language. If the given language is not supported, alerts will be returned
* in English. The language is specified as an [IETF BCP-47 language
* code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to use for translating alert messages. If unspecified, this defaults to the user's
display language. If the given language is not supported, alerts will be returned in English. The
language is specified as an [IETF BCP-47 language
code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to use for translating alert messages. If unspecified, this defaults to the
* user's display language. If the given language is not supported, alerts will be returned
* in English. The language is specified as an [IETF BCP-47 language
* code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Payments collection.
*
* The typical use is:
*
* {@code Adsense adsense = new Adsense(...);}
* {@code Adsense.Payments.List request = adsense.payments().list(parameters ...)}
*
*
* @return the resource collection
*/
public Payments payments() {
return new Payments();
}
/**
* The "payments" collection of methods.
*/
public class Payments {
/**
* Lists all the payments available for an account.
*
* Create a request for the method "payments.list".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The account which owns the collection of payments. Format: accounts/{account}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}/payments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Lists all the payments available for an account.
*
* Create a request for the method "payments.list".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The account which owns the collection of payments. Format: accounts/{account}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListPaymentsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The account which owns the collection of payments. Format: accounts/{account}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The account which owns the collection of payments. Format: accounts/{account}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The account which owns the collection of payments. Format: accounts/{account}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the PolicyIssues collection.
*
* The typical use is:
*
* {@code Adsense adsense = new Adsense(...);}
* {@code Adsense.PolicyIssues.List request = adsense.policyIssues().list(parameters ...)}
*
*
* @return the resource collection
*/
public PolicyIssues policyIssues() {
return new PolicyIssues();
}
/**
* The "policyIssues" collection of methods.
*/
public class PolicyIssues {
/**
* Gets information about the selected policy issue.
*
* Create a request for the method "policyIssues.get".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the policy issue. Format: accounts/{account}/policyIssues/{policy_issue}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/policyIssues/[^/]+$");
/**
* Gets information about the selected policy issue.
*
* Create a request for the method "policyIssues.get".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the policy issue. Format: accounts/{account}/policyIssues/{policy_issue}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.PolicyIssue.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/policyIssues/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the policy issue. Format:
* accounts/{account}/policyIssues/{policy_issue}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the policy issue. Format: accounts/{account}/policyIssues/{policy_issue}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the policy issue. Format:
* accounts/{account}/policyIssues/{policy_issue}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/policyIssues/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the policy issues where the specified account is involved, both directly and through
* any AFP child accounts.
*
* Create a request for the method "policyIssues.list".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The account for which policy issues are being retrieved. Format: accounts/{account}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}/policyIssues";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Lists all the policy issues where the specified account is involved, both directly and through
* any AFP child accounts.
*
* Create a request for the method "policyIssues.list".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The account for which policy issues are being retrieved. Format: accounts/{account}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListPolicyIssuesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The account for which policy issues are being retrieved. Format:
* accounts/{account}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The account for which policy issues are being retrieved. Format: accounts/{account}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The account for which policy issues are being retrieved. Format:
* accounts/{account}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of policy issues to include in the response, used for paging. If
* unspecified, at most 10000 policy issues will be returned. The maximum value is 10000;
* values above 10000 will be coerced to 10000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of policy issues to include in the response, used for paging. If unspecified, at
most 10000 policy issues will be returned. The maximum value is 10000; values above 10000 will be
coerced to 10000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of policy issues to include in the response, used for paging. If
* unspecified, at most 10000 policy issues will be returned. The maximum value is 10000;
* values above 10000 will be coerced to 10000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListPolicyIssues` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to `ListPolicyIssues`
* must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListPolicyIssues` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListPolicyIssues` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListPolicyIssues` call. Provide this to retrieve
* the subsequent page. When paginating, all other parameters provided to `ListPolicyIssues`
* must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Reports collection.
*
* The typical use is:
*
* {@code Adsense adsense = new Adsense(...);}
* {@code Adsense.Reports.List request = adsense.reports().list(parameters ...)}
*
*
* @return the resource collection
*/
public Reports reports() {
return new Reports();
}
/**
* The "reports" collection of methods.
*/
public class Reports {
/**
* Generates an ad hoc report.
*
* Create a request for the method "reports.generate".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Generate#execute()} method to invoke the remote operation.
*
* @param account Required. The account which owns the collection of reports. Format: accounts/{account}
* @return the request
*/
public Generate generate(java.lang.String account) throws java.io.IOException {
Generate result = new Generate(account);
initialize(result);
return result;
}
public class Generate extends AdsenseRequest {
private static final String REST_PATH = "v2/{+account}/reports:generate";
private final java.util.regex.Pattern ACCOUNT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Generates an ad hoc report.
*
* Create a request for the method "reports.generate".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Generate#execute()} method to invoke the remote operation.
* {@link
* Generate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param account Required. The account which owns the collection of reports. Format: accounts/{account}
* @since 1.13
*/
protected Generate(java.lang.String account) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ReportResult.class);
this.account = com.google.api.client.util.Preconditions.checkNotNull(account, "Required parameter account must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ACCOUNT_PATTERN.matcher(account).matches(),
"Parameter account must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Generate set$Xgafv(java.lang.String $Xgafv) {
return (Generate) super.set$Xgafv($Xgafv);
}
@Override
public Generate setAccessToken(java.lang.String accessToken) {
return (Generate) super.setAccessToken(accessToken);
}
@Override
public Generate setAlt(java.lang.String alt) {
return (Generate) super.setAlt(alt);
}
@Override
public Generate setCallback(java.lang.String callback) {
return (Generate) super.setCallback(callback);
}
@Override
public Generate setFields(java.lang.String fields) {
return (Generate) super.setFields(fields);
}
@Override
public Generate setKey(java.lang.String key) {
return (Generate) super.setKey(key);
}
@Override
public Generate setOauthToken(java.lang.String oauthToken) {
return (Generate) super.setOauthToken(oauthToken);
}
@Override
public Generate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Generate) super.setPrettyPrint(prettyPrint);
}
@Override
public Generate setQuotaUser(java.lang.String quotaUser) {
return (Generate) super.setQuotaUser(quotaUser);
}
@Override
public Generate setUploadType(java.lang.String uploadType) {
return (Generate) super.setUploadType(uploadType);
}
@Override
public Generate setUploadProtocol(java.lang.String uploadProtocol) {
return (Generate) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The account which owns the collection of reports. Format: accounts/{account}
*/
@com.google.api.client.util.Key
private java.lang.String account;
/** Required. The account which owns the collection of reports. Format: accounts/{account}
*/
public java.lang.String getAccount() {
return account;
}
/**
* Required. The account which owns the collection of reports. Format: accounts/{account}
*/
public Generate setAccount(java.lang.String account) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ACCOUNT_PATTERN.matcher(account).matches(),
"Parameter account must conform to the pattern " +
"^accounts/[^/]+$");
}
this.account = account;
return this;
}
/**
* The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when
* reporting on monetary metrics. Defaults to the account's currency if not set.
*/
@com.google.api.client.util.Key
private java.lang.String currencyCode;
/** The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when reporting on
monetary metrics. Defaults to the account's currency if not set.
*/
public java.lang.String getCurrencyCode() {
return currencyCode;
}
/**
* The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when
* reporting on monetary metrics. Defaults to the account's currency if not set.
*/
public Generate setCurrencyCode(java.lang.String currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/** Date range of the report, if unset the range will be considered CUSTOM. */
@com.google.api.client.util.Key
private java.lang.String dateRange;
/** Date range of the report, if unset the range will be considered CUSTOM.
*/
public java.lang.String getDateRange() {
return dateRange;
}
/** Date range of the report, if unset the range will be considered CUSTOM. */
public Generate setDateRange(java.lang.String dateRange) {
this.dateRange = dateRange;
return this;
}
/** Dimensions to base the report on. */
@com.google.api.client.util.Key
private java.util.List dimensions;
/** Dimensions to base the report on.
*/
public java.util.List getDimensions() {
return dimensions;
}
/** Dimensions to base the report on. */
public Generate setDimensions(java.util.List dimensions) {
this.dimensions = dimensions;
return this;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a
* year by itself or a year and month where the day isn't significant.
*/
@com.google.api.client.util.Key("endDate.day")
private java.lang.Integer endDateDay;
/** Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a year by
itself or a year and month where the day isn't significant.
*/
public java.lang.Integer getEndDateDay() {
return endDateDay;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a
* year by itself or a year and month where the day isn't significant.
*/
public Generate setEndDateDay(java.lang.Integer endDateDay) {
this.endDateDay = endDateDay;
return this;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
@com.google.api.client.util.Key("endDate.month")
private java.lang.Integer endDateMonth;
/** Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public java.lang.Integer getEndDateMonth() {
return endDateMonth;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public Generate setEndDateMonth(java.lang.Integer endDateMonth) {
this.endDateMonth = endDateMonth;
return this;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
@com.google.api.client.util.Key("endDate.year")
private java.lang.Integer endDateYear;
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year.
*/
public java.lang.Integer getEndDateYear() {
return endDateYear;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
public Generate setEndDateYear(java.lang.Integer endDateYear) {
this.endDateYear = endDateYear;
return this;
}
/**
* A list of [filters](/adsense/management/reporting/filtering) to apply to the report. All
* provided filters must match in order for the data to be included in the report.
*/
@com.google.api.client.util.Key
private java.util.List filters;
/** A list of [filters](/adsense/management/reporting/filtering) to apply to the report. All provided
filters must match in order for the data to be included in the report.
*/
public java.util.List getFilters() {
return filters;
}
/**
* A list of [filters](/adsense/management/reporting/filtering) to apply to the report. All
* provided filters must match in order for the data to be included in the report.
*/
public Generate setFilters(java.util.List filters) {
this.filters = filters;
return this;
}
/**
* The language to use for translating report output. If unspecified, this defaults to
* English ("en"). If the given language is not supported, report output will be returned in
* English. The language is specified as an [IETF BCP-47 language
* code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to use for translating report output. If unspecified, this defaults to English ("en").
If the given language is not supported, report output will be returned in English. The language is
specified as an [IETF BCP-47 language code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to use for translating report output. If unspecified, this defaults to
* English ("en"). If the given language is not supported, report output will be returned in
* English. The language is specified as an [IETF BCP-47 language
* code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
public Generate setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The maximum number of rows of report data to return. Reports producing more rows than the
* requested limit will be truncated. If unset, this defaults to 100,000 rows for
* `Reports.GenerateReport` and 1,000,000 rows for `Reports.GenerateCsvReport`, which are
* also the maximum values permitted here. Report truncation can be identified (for
* `Reports.GenerateReport` only) by comparing the number of rows returned to the value
* returned in `total_matched_rows`.
*/
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of rows of report data to return. Reports producing more rows than the requested
limit will be truncated. If unset, this defaults to 100,000 rows for `Reports.GenerateReport` and
1,000,000 rows for `Reports.GenerateCsvReport`, which are also the maximum values permitted here.
Report truncation can be identified (for `Reports.GenerateReport` only) by comparing the number of
rows returned to the value returned in `total_matched_rows`.
*/
public java.lang.Integer getLimit() {
return limit;
}
/**
* The maximum number of rows of report data to return. Reports producing more rows than the
* requested limit will be truncated. If unset, this defaults to 100,000 rows for
* `Reports.GenerateReport` and 1,000,000 rows for `Reports.GenerateCsvReport`, which are
* also the maximum values permitted here. Report truncation can be identified (for
* `Reports.GenerateReport` only) by comparing the number of rows returned to the value
* returned in `total_matched_rows`.
*/
public Generate setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/** Required. Reporting metrics. */
@com.google.api.client.util.Key
private java.util.List metrics;
/** Required. Reporting metrics.
*/
public java.util.List getMetrics() {
return metrics;
}
/** Required. Reporting metrics. */
public Generate setMetrics(java.util.List metrics) {
this.metrics = metrics;
return this;
}
/**
* The name of a dimension or metric to sort the resulting report on, can be prefixed with
* "+" to sort ascending or "-" to sort descending. If no prefix is specified, the column is
* sorted ascending.
*/
@com.google.api.client.util.Key
private java.util.List orderBy;
/** The name of a dimension or metric to sort the resulting report on, can be prefixed with "+" to sort
ascending or "-" to sort descending. If no prefix is specified, the column is sorted ascending.
*/
public java.util.List getOrderBy() {
return orderBy;
}
/**
* The name of a dimension or metric to sort the resulting report on, can be prefixed with
* "+" to sort ascending or "-" to sort descending. If no prefix is specified, the column is
* sorted ascending.
*/
public Generate setOrderBy(java.util.List orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Timezone in which to generate the report. If unspecified, this defaults to the account
* timezone. For more information, see [changing the time zone of your
* reports](https://support.google.com/adsense/answer/9830725).
*/
@com.google.api.client.util.Key
private java.lang.String reportingTimeZone;
/** Timezone in which to generate the report. If unspecified, this defaults to the account timezone.
For more information, see [changing the time zone of your
reports](https://support.google.com/adsense/answer/9830725).
*/
public java.lang.String getReportingTimeZone() {
return reportingTimeZone;
}
/**
* Timezone in which to generate the report. If unspecified, this defaults to the account
* timezone. For more information, see [changing the time zone of your
* reports](https://support.google.com/adsense/answer/9830725).
*/
public Generate setReportingTimeZone(java.lang.String reportingTimeZone) {
this.reportingTimeZone = reportingTimeZone;
return this;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a
* year by itself or a year and month where the day isn't significant.
*/
@com.google.api.client.util.Key("startDate.day")
private java.lang.Integer startDateDay;
/** Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a year by
itself or a year and month where the day isn't significant.
*/
public java.lang.Integer getStartDateDay() {
return startDateDay;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a
* year by itself or a year and month where the day isn't significant.
*/
public Generate setStartDateDay(java.lang.Integer startDateDay) {
this.startDateDay = startDateDay;
return this;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
@com.google.api.client.util.Key("startDate.month")
private java.lang.Integer startDateMonth;
/** Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public java.lang.Integer getStartDateMonth() {
return startDateMonth;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public Generate setStartDateMonth(java.lang.Integer startDateMonth) {
this.startDateMonth = startDateMonth;
return this;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
@com.google.api.client.util.Key("startDate.year")
private java.lang.Integer startDateYear;
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year.
*/
public java.lang.Integer getStartDateYear() {
return startDateYear;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
public Generate setStartDateYear(java.lang.Integer startDateYear) {
this.startDateYear = startDateYear;
return this;
}
@Override
public Generate set(String parameterName, Object value) {
return (Generate) super.set(parameterName, value);
}
}
/**
* Generates a csv formatted ad hoc report.
*
* Create a request for the method "reports.generateCsv".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link GenerateCsv#execute()} method to invoke the remote operation.
*
* @param account Required. The account which owns the collection of reports. Format: accounts/{account}
* @return the request
*/
public GenerateCsv generateCsv(java.lang.String account) throws java.io.IOException {
GenerateCsv result = new GenerateCsv(account);
initialize(result);
return result;
}
public class GenerateCsv extends AdsenseRequest {
private static final String REST_PATH = "v2/{+account}/reports:generateCsv";
private final java.util.regex.Pattern ACCOUNT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Generates a csv formatted ad hoc report.
*
* Create a request for the method "reports.generateCsv".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link GenerateCsv#execute()} method to invoke the remote operation.
* {@link
* GenerateCsv#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param account Required. The account which owns the collection of reports. Format: accounts/{account}
* @since 1.13
*/
protected GenerateCsv(java.lang.String account) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.HttpBody.class);
this.account = com.google.api.client.util.Preconditions.checkNotNull(account, "Required parameter account must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ACCOUNT_PATTERN.matcher(account).matches(),
"Parameter account must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GenerateCsv set$Xgafv(java.lang.String $Xgafv) {
return (GenerateCsv) super.set$Xgafv($Xgafv);
}
@Override
public GenerateCsv setAccessToken(java.lang.String accessToken) {
return (GenerateCsv) super.setAccessToken(accessToken);
}
@Override
public GenerateCsv setAlt(java.lang.String alt) {
return (GenerateCsv) super.setAlt(alt);
}
@Override
public GenerateCsv setCallback(java.lang.String callback) {
return (GenerateCsv) super.setCallback(callback);
}
@Override
public GenerateCsv setFields(java.lang.String fields) {
return (GenerateCsv) super.setFields(fields);
}
@Override
public GenerateCsv setKey(java.lang.String key) {
return (GenerateCsv) super.setKey(key);
}
@Override
public GenerateCsv setOauthToken(java.lang.String oauthToken) {
return (GenerateCsv) super.setOauthToken(oauthToken);
}
@Override
public GenerateCsv setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GenerateCsv) super.setPrettyPrint(prettyPrint);
}
@Override
public GenerateCsv setQuotaUser(java.lang.String quotaUser) {
return (GenerateCsv) super.setQuotaUser(quotaUser);
}
@Override
public GenerateCsv setUploadType(java.lang.String uploadType) {
return (GenerateCsv) super.setUploadType(uploadType);
}
@Override
public GenerateCsv setUploadProtocol(java.lang.String uploadProtocol) {
return (GenerateCsv) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The account which owns the collection of reports. Format: accounts/{account}
*/
@com.google.api.client.util.Key
private java.lang.String account;
/** Required. The account which owns the collection of reports. Format: accounts/{account}
*/
public java.lang.String getAccount() {
return account;
}
/**
* Required. The account which owns the collection of reports. Format: accounts/{account}
*/
public GenerateCsv setAccount(java.lang.String account) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ACCOUNT_PATTERN.matcher(account).matches(),
"Parameter account must conform to the pattern " +
"^accounts/[^/]+$");
}
this.account = account;
return this;
}
/**
* The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when
* reporting on monetary metrics. Defaults to the account's currency if not set.
*/
@com.google.api.client.util.Key
private java.lang.String currencyCode;
/** The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when reporting on
monetary metrics. Defaults to the account's currency if not set.
*/
public java.lang.String getCurrencyCode() {
return currencyCode;
}
/**
* The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when
* reporting on monetary metrics. Defaults to the account's currency if not set.
*/
public GenerateCsv setCurrencyCode(java.lang.String currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/** Date range of the report, if unset the range will be considered CUSTOM. */
@com.google.api.client.util.Key
private java.lang.String dateRange;
/** Date range of the report, if unset the range will be considered CUSTOM.
*/
public java.lang.String getDateRange() {
return dateRange;
}
/** Date range of the report, if unset the range will be considered CUSTOM. */
public GenerateCsv setDateRange(java.lang.String dateRange) {
this.dateRange = dateRange;
return this;
}
/** Dimensions to base the report on. */
@com.google.api.client.util.Key
private java.util.List dimensions;
/** Dimensions to base the report on.
*/
public java.util.List getDimensions() {
return dimensions;
}
/** Dimensions to base the report on. */
public GenerateCsv setDimensions(java.util.List dimensions) {
this.dimensions = dimensions;
return this;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a
* year by itself or a year and month where the day isn't significant.
*/
@com.google.api.client.util.Key("endDate.day")
private java.lang.Integer endDateDay;
/** Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a year by
itself or a year and month where the day isn't significant.
*/
public java.lang.Integer getEndDateDay() {
return endDateDay;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a
* year by itself or a year and month where the day isn't significant.
*/
public GenerateCsv setEndDateDay(java.lang.Integer endDateDay) {
this.endDateDay = endDateDay;
return this;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
@com.google.api.client.util.Key("endDate.month")
private java.lang.Integer endDateMonth;
/** Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public java.lang.Integer getEndDateMonth() {
return endDateMonth;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public GenerateCsv setEndDateMonth(java.lang.Integer endDateMonth) {
this.endDateMonth = endDateMonth;
return this;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
@com.google.api.client.util.Key("endDate.year")
private java.lang.Integer endDateYear;
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year.
*/
public java.lang.Integer getEndDateYear() {
return endDateYear;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
public GenerateCsv setEndDateYear(java.lang.Integer endDateYear) {
this.endDateYear = endDateYear;
return this;
}
/**
* A list of [filters](/adsense/management/reporting/filtering) to apply to the report. All
* provided filters must match in order for the data to be included in the report.
*/
@com.google.api.client.util.Key
private java.util.List filters;
/** A list of [filters](/adsense/management/reporting/filtering) to apply to the report. All provided
filters must match in order for the data to be included in the report.
*/
public java.util.List getFilters() {
return filters;
}
/**
* A list of [filters](/adsense/management/reporting/filtering) to apply to the report. All
* provided filters must match in order for the data to be included in the report.
*/
public GenerateCsv setFilters(java.util.List filters) {
this.filters = filters;
return this;
}
/**
* The language to use for translating report output. If unspecified, this defaults to
* English ("en"). If the given language is not supported, report output will be returned in
* English. The language is specified as an [IETF BCP-47 language
* code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to use for translating report output. If unspecified, this defaults to English ("en").
If the given language is not supported, report output will be returned in English. The language is
specified as an [IETF BCP-47 language code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to use for translating report output. If unspecified, this defaults to
* English ("en"). If the given language is not supported, report output will be returned in
* English. The language is specified as an [IETF BCP-47 language
* code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
public GenerateCsv setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The maximum number of rows of report data to return. Reports producing more rows than the
* requested limit will be truncated. If unset, this defaults to 100,000 rows for
* `Reports.GenerateReport` and 1,000,000 rows for `Reports.GenerateCsvReport`, which are
* also the maximum values permitted here. Report truncation can be identified (for
* `Reports.GenerateReport` only) by comparing the number of rows returned to the value
* returned in `total_matched_rows`.
*/
@com.google.api.client.util.Key
private java.lang.Integer limit;
/** The maximum number of rows of report data to return. Reports producing more rows than the requested
limit will be truncated. If unset, this defaults to 100,000 rows for `Reports.GenerateReport` and
1,000,000 rows for `Reports.GenerateCsvReport`, which are also the maximum values permitted here.
Report truncation can be identified (for `Reports.GenerateReport` only) by comparing the number of
rows returned to the value returned in `total_matched_rows`.
*/
public java.lang.Integer getLimit() {
return limit;
}
/**
* The maximum number of rows of report data to return. Reports producing more rows than the
* requested limit will be truncated. If unset, this defaults to 100,000 rows for
* `Reports.GenerateReport` and 1,000,000 rows for `Reports.GenerateCsvReport`, which are
* also the maximum values permitted here. Report truncation can be identified (for
* `Reports.GenerateReport` only) by comparing the number of rows returned to the value
* returned in `total_matched_rows`.
*/
public GenerateCsv setLimit(java.lang.Integer limit) {
this.limit = limit;
return this;
}
/** Required. Reporting metrics. */
@com.google.api.client.util.Key
private java.util.List metrics;
/** Required. Reporting metrics.
*/
public java.util.List getMetrics() {
return metrics;
}
/** Required. Reporting metrics. */
public GenerateCsv setMetrics(java.util.List metrics) {
this.metrics = metrics;
return this;
}
/**
* The name of a dimension or metric to sort the resulting report on, can be prefixed with
* "+" to sort ascending or "-" to sort descending. If no prefix is specified, the column is
* sorted ascending.
*/
@com.google.api.client.util.Key
private java.util.List orderBy;
/** The name of a dimension or metric to sort the resulting report on, can be prefixed with "+" to sort
ascending or "-" to sort descending. If no prefix is specified, the column is sorted ascending.
*/
public java.util.List getOrderBy() {
return orderBy;
}
/**
* The name of a dimension or metric to sort the resulting report on, can be prefixed with
* "+" to sort ascending or "-" to sort descending. If no prefix is specified, the column is
* sorted ascending.
*/
public GenerateCsv setOrderBy(java.util.List orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Timezone in which to generate the report. If unspecified, this defaults to the account
* timezone. For more information, see [changing the time zone of your
* reports](https://support.google.com/adsense/answer/9830725).
*/
@com.google.api.client.util.Key
private java.lang.String reportingTimeZone;
/** Timezone in which to generate the report. If unspecified, this defaults to the account timezone.
For more information, see [changing the time zone of your
reports](https://support.google.com/adsense/answer/9830725).
*/
public java.lang.String getReportingTimeZone() {
return reportingTimeZone;
}
/**
* Timezone in which to generate the report. If unspecified, this defaults to the account
* timezone. For more information, see [changing the time zone of your
* reports](https://support.google.com/adsense/answer/9830725).
*/
public GenerateCsv setReportingTimeZone(java.lang.String reportingTimeZone) {
this.reportingTimeZone = reportingTimeZone;
return this;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a
* year by itself or a year and month where the day isn't significant.
*/
@com.google.api.client.util.Key("startDate.day")
private java.lang.Integer startDateDay;
/** Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a year by
itself or a year and month where the day isn't significant.
*/
public java.lang.Integer getStartDateDay() {
return startDateDay;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a
* year by itself or a year and month where the day isn't significant.
*/
public GenerateCsv setStartDateDay(java.lang.Integer startDateDay) {
this.startDateDay = startDateDay;
return this;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
@com.google.api.client.util.Key("startDate.month")
private java.lang.Integer startDateMonth;
/** Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public java.lang.Integer getStartDateMonth() {
return startDateMonth;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public GenerateCsv setStartDateMonth(java.lang.Integer startDateMonth) {
this.startDateMonth = startDateMonth;
return this;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
@com.google.api.client.util.Key("startDate.year")
private java.lang.Integer startDateYear;
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year.
*/
public java.lang.Integer getStartDateYear() {
return startDateYear;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
public GenerateCsv setStartDateYear(java.lang.Integer startDateYear) {
this.startDateYear = startDateYear;
return this;
}
@Override
public GenerateCsv set(String parameterName, Object value) {
return (GenerateCsv) super.set(parameterName, value);
}
}
/**
* Gets the saved report from the given resource name.
*
* Create a request for the method "reports.getSaved".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link GetSaved#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the saved report to retrieve. Format: accounts/{account}/reports/{report}
* @return the request
*/
public GetSaved getSaved(java.lang.String name) throws java.io.IOException {
GetSaved result = new GetSaved(name);
initialize(result);
return result;
}
public class GetSaved extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}/saved";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/reports/[^/]+$");
/**
* Gets the saved report from the given resource name.
*
* Create a request for the method "reports.getSaved".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link GetSaved#execute()} method to invoke the remote operation.
* {@link
* GetSaved#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the saved report to retrieve. Format: accounts/{account}/reports/{report}
* @since 1.13
*/
protected GetSaved(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.SavedReport.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/reports/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetSaved set$Xgafv(java.lang.String $Xgafv) {
return (GetSaved) super.set$Xgafv($Xgafv);
}
@Override
public GetSaved setAccessToken(java.lang.String accessToken) {
return (GetSaved) super.setAccessToken(accessToken);
}
@Override
public GetSaved setAlt(java.lang.String alt) {
return (GetSaved) super.setAlt(alt);
}
@Override
public GetSaved setCallback(java.lang.String callback) {
return (GetSaved) super.setCallback(callback);
}
@Override
public GetSaved setFields(java.lang.String fields) {
return (GetSaved) super.setFields(fields);
}
@Override
public GetSaved setKey(java.lang.String key) {
return (GetSaved) super.setKey(key);
}
@Override
public GetSaved setOauthToken(java.lang.String oauthToken) {
return (GetSaved) super.setOauthToken(oauthToken);
}
@Override
public GetSaved setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetSaved) super.setPrettyPrint(prettyPrint);
}
@Override
public GetSaved setQuotaUser(java.lang.String quotaUser) {
return (GetSaved) super.setQuotaUser(quotaUser);
}
@Override
public GetSaved setUploadType(java.lang.String uploadType) {
return (GetSaved) super.setUploadType(uploadType);
}
@Override
public GetSaved setUploadProtocol(java.lang.String uploadProtocol) {
return (GetSaved) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the saved report to retrieve. Format:
* accounts/{account}/reports/{report}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the saved report to retrieve. Format: accounts/{account}/reports/{report}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the saved report to retrieve. Format:
* accounts/{account}/reports/{report}
*/
public GetSaved setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/reports/[^/]+$");
}
this.name = name;
return this;
}
@Override
public GetSaved set(String parameterName, Object value) {
return (GetSaved) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Saved collection.
*
* The typical use is:
*
* {@code Adsense adsense = new Adsense(...);}
* {@code Adsense.Saved.List request = adsense.saved().list(parameters ...)}
*
*
* @return the resource collection
*/
public Saved saved() {
return new Saved();
}
/**
* The "saved" collection of methods.
*/
public class Saved {
/**
* Generates a saved report.
*
* Create a request for the method "saved.generate".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Generate#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the saved report. Format: accounts/{account}/reports/{report}
* @return the request
*/
public Generate generate(java.lang.String name) throws java.io.IOException {
Generate result = new Generate(name);
initialize(result);
return result;
}
public class Generate extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}/saved:generate";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/reports/[^/]+$");
/**
* Generates a saved report.
*
* Create a request for the method "saved.generate".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Generate#execute()} method to invoke the remote operation.
* {@link
* Generate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the saved report. Format: accounts/{account}/reports/{report}
* @since 1.13
*/
protected Generate(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ReportResult.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/reports/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Generate set$Xgafv(java.lang.String $Xgafv) {
return (Generate) super.set$Xgafv($Xgafv);
}
@Override
public Generate setAccessToken(java.lang.String accessToken) {
return (Generate) super.setAccessToken(accessToken);
}
@Override
public Generate setAlt(java.lang.String alt) {
return (Generate) super.setAlt(alt);
}
@Override
public Generate setCallback(java.lang.String callback) {
return (Generate) super.setCallback(callback);
}
@Override
public Generate setFields(java.lang.String fields) {
return (Generate) super.setFields(fields);
}
@Override
public Generate setKey(java.lang.String key) {
return (Generate) super.setKey(key);
}
@Override
public Generate setOauthToken(java.lang.String oauthToken) {
return (Generate) super.setOauthToken(oauthToken);
}
@Override
public Generate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Generate) super.setPrettyPrint(prettyPrint);
}
@Override
public Generate setQuotaUser(java.lang.String quotaUser) {
return (Generate) super.setQuotaUser(quotaUser);
}
@Override
public Generate setUploadType(java.lang.String uploadType) {
return (Generate) super.setUploadType(uploadType);
}
@Override
public Generate setUploadProtocol(java.lang.String uploadProtocol) {
return (Generate) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the saved report. Format: accounts/{account}/reports/{report} */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the saved report. Format: accounts/{account}/reports/{report}
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the saved report. Format: accounts/{account}/reports/{report} */
public Generate setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/reports/[^/]+$");
}
this.name = name;
return this;
}
/**
* The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when
* reporting on monetary metrics. Defaults to the account's currency if not set.
*/
@com.google.api.client.util.Key
private java.lang.String currencyCode;
/** The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when reporting on
monetary metrics. Defaults to the account's currency if not set.
*/
public java.lang.String getCurrencyCode() {
return currencyCode;
}
/**
* The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when
* reporting on monetary metrics. Defaults to the account's currency if not set.
*/
public Generate setCurrencyCode(java.lang.String currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/** Date range of the report, if unset the range will be considered CUSTOM. */
@com.google.api.client.util.Key
private java.lang.String dateRange;
/** Date range of the report, if unset the range will be considered CUSTOM.
*/
public java.lang.String getDateRange() {
return dateRange;
}
/** Date range of the report, if unset the range will be considered CUSTOM. */
public Generate setDateRange(java.lang.String dateRange) {
this.dateRange = dateRange;
return this;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify
* a year by itself or a year and month where the day isn't significant.
*/
@com.google.api.client.util.Key("endDate.day")
private java.lang.Integer endDateDay;
/** Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a year by
itself or a year and month where the day isn't significant.
*/
public java.lang.Integer getEndDateDay() {
return endDateDay;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify
* a year by itself or a year and month where the day isn't significant.
*/
public Generate setEndDateDay(java.lang.Integer endDateDay) {
this.endDateDay = endDateDay;
return this;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
@com.google.api.client.util.Key("endDate.month")
private java.lang.Integer endDateMonth;
/** Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public java.lang.Integer getEndDateMonth() {
return endDateMonth;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public Generate setEndDateMonth(java.lang.Integer endDateMonth) {
this.endDateMonth = endDateMonth;
return this;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
@com.google.api.client.util.Key("endDate.year")
private java.lang.Integer endDateYear;
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year.
*/
public java.lang.Integer getEndDateYear() {
return endDateYear;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
public Generate setEndDateYear(java.lang.Integer endDateYear) {
this.endDateYear = endDateYear;
return this;
}
/**
* The language to use for translating report output. If unspecified, this defaults to
* English ("en"). If the given language is not supported, report output will be returned
* in English. The language is specified as an [IETF BCP-47 language
* code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to use for translating report output. If unspecified, this defaults to English ("en").
If the given language is not supported, report output will be returned in English. The language is
specified as an [IETF BCP-47 language code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to use for translating report output. If unspecified, this defaults to
* English ("en"). If the given language is not supported, report output will be returned
* in English. The language is specified as an [IETF BCP-47 language
* code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
public Generate setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Timezone in which to generate the report. If unspecified, this defaults to the account
* timezone. For more information, see [changing the time zone of your
* reports](https://support.google.com/adsense/answer/9830725).
*/
@com.google.api.client.util.Key
private java.lang.String reportingTimeZone;
/** Timezone in which to generate the report. If unspecified, this defaults to the account timezone.
For more information, see [changing the time zone of your
reports](https://support.google.com/adsense/answer/9830725).
*/
public java.lang.String getReportingTimeZone() {
return reportingTimeZone;
}
/**
* Timezone in which to generate the report. If unspecified, this defaults to the account
* timezone. For more information, see [changing the time zone of your
* reports](https://support.google.com/adsense/answer/9830725).
*/
public Generate setReportingTimeZone(java.lang.String reportingTimeZone) {
this.reportingTimeZone = reportingTimeZone;
return this;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify
* a year by itself or a year and month where the day isn't significant.
*/
@com.google.api.client.util.Key("startDate.day")
private java.lang.Integer startDateDay;
/** Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a year by
itself or a year and month where the day isn't significant.
*/
public java.lang.Integer getStartDateDay() {
return startDateDay;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify
* a year by itself or a year and month where the day isn't significant.
*/
public Generate setStartDateDay(java.lang.Integer startDateDay) {
this.startDateDay = startDateDay;
return this;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
@com.google.api.client.util.Key("startDate.month")
private java.lang.Integer startDateMonth;
/** Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public java.lang.Integer getStartDateMonth() {
return startDateMonth;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public Generate setStartDateMonth(java.lang.Integer startDateMonth) {
this.startDateMonth = startDateMonth;
return this;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
@com.google.api.client.util.Key("startDate.year")
private java.lang.Integer startDateYear;
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year.
*/
public java.lang.Integer getStartDateYear() {
return startDateYear;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
public Generate setStartDateYear(java.lang.Integer startDateYear) {
this.startDateYear = startDateYear;
return this;
}
@Override
public Generate set(String parameterName, Object value) {
return (Generate) super.set(parameterName, value);
}
}
/**
* Generates a csv formatted saved report.
*
* Create a request for the method "saved.generateCsv".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link GenerateCsv#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the saved report. Format: accounts/{account}/reports/{report}
* @return the request
*/
public GenerateCsv generateCsv(java.lang.String name) throws java.io.IOException {
GenerateCsv result = new GenerateCsv(name);
initialize(result);
return result;
}
public class GenerateCsv extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}/saved:generateCsv";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/reports/[^/]+$");
/**
* Generates a csv formatted saved report.
*
* Create a request for the method "saved.generateCsv".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link GenerateCsv#execute()} method to invoke the remote operation.
* {@link
* GenerateCsv#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the saved report. Format: accounts/{account}/reports/{report}
* @since 1.13
*/
protected GenerateCsv(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.HttpBody.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/reports/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GenerateCsv set$Xgafv(java.lang.String $Xgafv) {
return (GenerateCsv) super.set$Xgafv($Xgafv);
}
@Override
public GenerateCsv setAccessToken(java.lang.String accessToken) {
return (GenerateCsv) super.setAccessToken(accessToken);
}
@Override
public GenerateCsv setAlt(java.lang.String alt) {
return (GenerateCsv) super.setAlt(alt);
}
@Override
public GenerateCsv setCallback(java.lang.String callback) {
return (GenerateCsv) super.setCallback(callback);
}
@Override
public GenerateCsv setFields(java.lang.String fields) {
return (GenerateCsv) super.setFields(fields);
}
@Override
public GenerateCsv setKey(java.lang.String key) {
return (GenerateCsv) super.setKey(key);
}
@Override
public GenerateCsv setOauthToken(java.lang.String oauthToken) {
return (GenerateCsv) super.setOauthToken(oauthToken);
}
@Override
public GenerateCsv setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GenerateCsv) super.setPrettyPrint(prettyPrint);
}
@Override
public GenerateCsv setQuotaUser(java.lang.String quotaUser) {
return (GenerateCsv) super.setQuotaUser(quotaUser);
}
@Override
public GenerateCsv setUploadType(java.lang.String uploadType) {
return (GenerateCsv) super.setUploadType(uploadType);
}
@Override
public GenerateCsv setUploadProtocol(java.lang.String uploadProtocol) {
return (GenerateCsv) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the saved report. Format: accounts/{account}/reports/{report} */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the saved report. Format: accounts/{account}/reports/{report}
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the saved report. Format: accounts/{account}/reports/{report} */
public GenerateCsv setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/reports/[^/]+$");
}
this.name = name;
return this;
}
/**
* The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when
* reporting on monetary metrics. Defaults to the account's currency if not set.
*/
@com.google.api.client.util.Key
private java.lang.String currencyCode;
/** The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when reporting on
monetary metrics. Defaults to the account's currency if not set.
*/
public java.lang.String getCurrencyCode() {
return currencyCode;
}
/**
* The [ISO-4217 currency code](https://en.wikipedia.org/wiki/ISO_4217) to use when
* reporting on monetary metrics. Defaults to the account's currency if not set.
*/
public GenerateCsv setCurrencyCode(java.lang.String currencyCode) {
this.currencyCode = currencyCode;
return this;
}
/** Date range of the report, if unset the range will be considered CUSTOM. */
@com.google.api.client.util.Key
private java.lang.String dateRange;
/** Date range of the report, if unset the range will be considered CUSTOM.
*/
public java.lang.String getDateRange() {
return dateRange;
}
/** Date range of the report, if unset the range will be considered CUSTOM. */
public GenerateCsv setDateRange(java.lang.String dateRange) {
this.dateRange = dateRange;
return this;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify
* a year by itself or a year and month where the day isn't significant.
*/
@com.google.api.client.util.Key("endDate.day")
private java.lang.Integer endDateDay;
/** Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a year by
itself or a year and month where the day isn't significant.
*/
public java.lang.Integer getEndDateDay() {
return endDateDay;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify
* a year by itself or a year and month where the day isn't significant.
*/
public GenerateCsv setEndDateDay(java.lang.Integer endDateDay) {
this.endDateDay = endDateDay;
return this;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
@com.google.api.client.util.Key("endDate.month")
private java.lang.Integer endDateMonth;
/** Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public java.lang.Integer getEndDateMonth() {
return endDateMonth;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public GenerateCsv setEndDateMonth(java.lang.Integer endDateMonth) {
this.endDateMonth = endDateMonth;
return this;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
@com.google.api.client.util.Key("endDate.year")
private java.lang.Integer endDateYear;
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year.
*/
public java.lang.Integer getEndDateYear() {
return endDateYear;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
public GenerateCsv setEndDateYear(java.lang.Integer endDateYear) {
this.endDateYear = endDateYear;
return this;
}
/**
* The language to use for translating report output. If unspecified, this defaults to
* English ("en"). If the given language is not supported, report output will be returned
* in English. The language is specified as an [IETF BCP-47 language
* code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to use for translating report output. If unspecified, this defaults to English ("en").
If the given language is not supported, report output will be returned in English. The language is
specified as an [IETF BCP-47 language code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to use for translating report output. If unspecified, this defaults to
* English ("en"). If the given language is not supported, report output will be returned
* in English. The language is specified as an [IETF BCP-47 language
* code](https://en.wikipedia.org/wiki/IETF_language_tag).
*/
public GenerateCsv setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Timezone in which to generate the report. If unspecified, this defaults to the account
* timezone. For more information, see [changing the time zone of your
* reports](https://support.google.com/adsense/answer/9830725).
*/
@com.google.api.client.util.Key
private java.lang.String reportingTimeZone;
/** Timezone in which to generate the report. If unspecified, this defaults to the account timezone.
For more information, see [changing the time zone of your
reports](https://support.google.com/adsense/answer/9830725).
*/
public java.lang.String getReportingTimeZone() {
return reportingTimeZone;
}
/**
* Timezone in which to generate the report. If unspecified, this defaults to the account
* timezone. For more information, see [changing the time zone of your
* reports](https://support.google.com/adsense/answer/9830725).
*/
public GenerateCsv setReportingTimeZone(java.lang.String reportingTimeZone) {
this.reportingTimeZone = reportingTimeZone;
return this;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify
* a year by itself or a year and month where the day isn't significant.
*/
@com.google.api.client.util.Key("startDate.day")
private java.lang.Integer startDateDay;
/** Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify a year by
itself or a year and month where the day isn't significant.
*/
public java.lang.Integer getStartDateDay() {
return startDateDay;
}
/**
* Day of a month. Must be from 1 to 31 and valid for the year and month, or 0 to specify
* a year by itself or a year and month where the day isn't significant.
*/
public GenerateCsv setStartDateDay(java.lang.Integer startDateDay) {
this.startDateDay = startDateDay;
return this;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
@com.google.api.client.util.Key("startDate.month")
private java.lang.Integer startDateMonth;
/** Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public java.lang.Integer getStartDateMonth() {
return startDateMonth;
}
/**
* Month of a year. Must be from 1 to 12, or 0 to specify a year without a month and day.
*/
public GenerateCsv setStartDateMonth(java.lang.Integer startDateMonth) {
this.startDateMonth = startDateMonth;
return this;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
@com.google.api.client.util.Key("startDate.year")
private java.lang.Integer startDateYear;
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year.
*/
public java.lang.Integer getStartDateYear() {
return startDateYear;
}
/** Year of the date. Must be from 1 to 9999, or 0 to specify a date without a year. */
public GenerateCsv setStartDateYear(java.lang.Integer startDateYear) {
this.startDateYear = startDateYear;
return this;
}
@Override
public GenerateCsv set(String parameterName, Object value) {
return (GenerateCsv) super.set(parameterName, value);
}
}
/**
* Lists saved reports.
*
* Create a request for the method "saved.list".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The account which owns the collection of reports. Format: accounts/{account}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}/reports/saved";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Lists saved reports.
*
* Create a request for the method "saved.list".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The account which owns the collection of reports. Format: accounts/{account}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListSavedReportsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The account which owns the collection of reports. Format: accounts/{account}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The account which owns the collection of reports. Format: accounts/{account}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The account which owns the collection of reports. Format: accounts/{account}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of reports to include in the response, used for paging. If
* unspecified, at most 10000 reports will be returned. The maximum value is 10000; values
* above 10000 will be coerced to 10000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of reports to include in the response, used for paging. If unspecified, at most
10000 reports will be returned. The maximum value is 10000; values above 10000 will be coerced to
10000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of reports to include in the response, used for paging. If
* unspecified, at most 10000 reports will be returned. The maximum value is 10000; values
* above 10000 will be coerced to 10000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListSavedReports` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListSavedReports` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListSavedReports` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListSavedReports` must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListSavedReports` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListSavedReports` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Sites collection.
*
* The typical use is:
*
* {@code Adsense adsense = new Adsense(...);}
* {@code Adsense.Sites.List request = adsense.sites().list(parameters ...)}
*
*
* @return the resource collection
*/
public Sites sites() {
return new Sites();
}
/**
* The "sites" collection of methods.
*/
public class Sites {
/**
* Gets information about the selected site.
*
* Create a request for the method "sites.get".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the site. Format: accounts/{account}/sites/{site}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AdsenseRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+/sites/[^/]+$");
/**
* Gets information about the selected site.
*
* Create a request for the method "sites.get".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the site. Format: accounts/{account}/sites/{site}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.Site.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/sites/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the site. Format: accounts/{account}/sites/{site} */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the site. Format: accounts/{account}/sites/{site}
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the site. Format: accounts/{account}/sites/{site} */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^accounts/[^/]+/sites/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the sites available in an account.
*
* Create a request for the method "sites.list".
*
* This request holds the parameters needed by the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The account which owns the collection of sites. Format: accounts/{account}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AdsenseRequest {
private static final String REST_PATH = "v2/{+parent}/sites";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^accounts/[^/]+$");
/**
* Lists all the sites available in an account.
*
* Create a request for the method "sites.list".
*
* This request holds the parameters needed by the the adsense server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The account which owns the collection of sites. Format: accounts/{account}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Adsense.this, "GET", REST_PATH, null, com.google.api.services.adsense.v2.model.ListSitesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The account which owns the collection of sites. Format: accounts/{account} */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The account which owns the collection of sites. Format: accounts/{account}
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The account which owns the collection of sites. Format: accounts/{account} */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^accounts/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of sites to include in the response, used for paging. If unspecified,
* at most 10000 sites will be returned. The maximum value is 10000; values above 10000 will
* be coerced to 10000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of sites to include in the response, used for paging. If unspecified, at most
10000 sites will be returned. The maximum value is 10000; values above 10000 will be coerced to
10000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of sites to include in the response, used for paging. If unspecified,
* at most 10000 sites will be returned. The maximum value is 10000; values above 10000 will
* be coerced to 10000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListSites` call. Provide this to retrieve the
* subsequent page. When paginating, all other parameters provided to `ListSites` must match
* the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListSites` call. Provide this to retrieve the subsequent
page. When paginating, all other parameters provided to `ListSites` must match the call that
provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListSites` call. Provide this to retrieve the
* subsequent page. When paginating, all other parameters provided to `ListSites` must match
* the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link Adsense}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link Adsense}. */
@Override
public Adsense build() {
return new Adsense(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link AdsenseRequestInitializer}.
*
* @since 1.12
*/
public Builder setAdsenseRequestInitializer(
AdsenseRequestInitializer adsenseRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(adsenseRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}