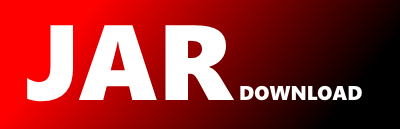
com.google.api.services.adsense.v2.model.PolicyIssue Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.adsense.v2.model;
/**
* Representation of a policy issue for a single entity (site, site-section, or page). All issues
* for a single entity are represented by a single PolicyIssue resource, though that PolicyIssue can
* have multiple causes (or "topics") that can change over time. Policy issues are removed if there
* are no issues detected recently or if there's a recent successful appeal for the entity.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the AdSense Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class PolicyIssue extends com.google.api.client.json.GenericJson {
/**
* Required. The most severe action taken on the entity over the past seven days.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String action;
/**
* Optional. List of ad clients associated with the policy issue (either as the primary ad client
* or an associated host/secondary ad client). In the latter case, this will be an ad client that
* is not owned by the current account.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List adClients;
/**
* Required. Total number of ad requests affected by the policy violations over the past seven
* days.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long adRequestCount;
/**
* Required. Type of the entity indicating if the entity is a site, site-section, or page.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String entityType;
/**
* Required. The date (in the America/Los_Angeles timezone) when policy violations were first
* detected on the entity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Date firstDetectedDate;
/**
* Required. The date (in the America/Los_Angeles timezone) when policy violations were last
* detected on the entity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Date lastDetectedDate;
/**
* Required. Resource name of the entity with policy issues. Format:
* accounts/{account}/policyIssues/{policy_issue}
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Required. Unordered list. The policy topics that this entity was found to violate over the past
* seven days.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List policyTopics;
/**
* Required. Hostname/domain of the entity (for example "foo.com" or "www.foo.com"). This _should_
* be a bare domain/host name without any protocol. This will be present for all policy issues.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String site;
/**
* Optional. Prefix of the site-section having policy issues (For example "foo.com/bar-section").
* This will be present if the `entity_type` is `SITE_SECTION` and will be absent for other entity
* types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String siteSection;
/**
* Optional. URI of the page having policy violations (for example "foo.com/bar" or
* "www.foo.com/bar"). This will be present if the `entity_type` is `PAGE` and will be absent for
* other entity types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uri;
/**
* Optional. The date (in the America/Los_Angeles timezone) when the entity will have ad serving
* demand restricted or ad serving disabled. This is present only for issues with a `WARNED`
* enforcement action. See https://support.google.com/adsense/answer/11066888.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Date warningEscalationDate;
/**
* Required. The most severe action taken on the entity over the past seven days.
* @return value or {@code null} for none
*/
public java.lang.String getAction() {
return action;
}
/**
* Required. The most severe action taken on the entity over the past seven days.
* @param action action or {@code null} for none
*/
public PolicyIssue setAction(java.lang.String action) {
this.action = action;
return this;
}
/**
* Optional. List of ad clients associated with the policy issue (either as the primary ad client
* or an associated host/secondary ad client). In the latter case, this will be an ad client that
* is not owned by the current account.
* @return value or {@code null} for none
*/
public java.util.List getAdClients() {
return adClients;
}
/**
* Optional. List of ad clients associated with the policy issue (either as the primary ad client
* or an associated host/secondary ad client). In the latter case, this will be an ad client that
* is not owned by the current account.
* @param adClients adClients or {@code null} for none
*/
public PolicyIssue setAdClients(java.util.List adClients) {
this.adClients = adClients;
return this;
}
/**
* Required. Total number of ad requests affected by the policy violations over the past seven
* days.
* @return value or {@code null} for none
*/
public java.lang.Long getAdRequestCount() {
return adRequestCount;
}
/**
* Required. Total number of ad requests affected by the policy violations over the past seven
* days.
* @param adRequestCount adRequestCount or {@code null} for none
*/
public PolicyIssue setAdRequestCount(java.lang.Long adRequestCount) {
this.adRequestCount = adRequestCount;
return this;
}
/**
* Required. Type of the entity indicating if the entity is a site, site-section, or page.
* @return value or {@code null} for none
*/
public java.lang.String getEntityType() {
return entityType;
}
/**
* Required. Type of the entity indicating if the entity is a site, site-section, or page.
* @param entityType entityType or {@code null} for none
*/
public PolicyIssue setEntityType(java.lang.String entityType) {
this.entityType = entityType;
return this;
}
/**
* Required. The date (in the America/Los_Angeles timezone) when policy violations were first
* detected on the entity.
* @return value or {@code null} for none
*/
public Date getFirstDetectedDate() {
return firstDetectedDate;
}
/**
* Required. The date (in the America/Los_Angeles timezone) when policy violations were first
* detected on the entity.
* @param firstDetectedDate firstDetectedDate or {@code null} for none
*/
public PolicyIssue setFirstDetectedDate(Date firstDetectedDate) {
this.firstDetectedDate = firstDetectedDate;
return this;
}
/**
* Required. The date (in the America/Los_Angeles timezone) when policy violations were last
* detected on the entity.
* @return value or {@code null} for none
*/
public Date getLastDetectedDate() {
return lastDetectedDate;
}
/**
* Required. The date (in the America/Los_Angeles timezone) when policy violations were last
* detected on the entity.
* @param lastDetectedDate lastDetectedDate or {@code null} for none
*/
public PolicyIssue setLastDetectedDate(Date lastDetectedDate) {
this.lastDetectedDate = lastDetectedDate;
return this;
}
/**
* Required. Resource name of the entity with policy issues. Format:
* accounts/{account}/policyIssues/{policy_issue}
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the entity with policy issues. Format:
* accounts/{account}/policyIssues/{policy_issue}
* @param name name or {@code null} for none
*/
public PolicyIssue setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Required. Unordered list. The policy topics that this entity was found to violate over the past
* seven days.
* @return value or {@code null} for none
*/
public java.util.List getPolicyTopics() {
return policyTopics;
}
/**
* Required. Unordered list. The policy topics that this entity was found to violate over the past
* seven days.
* @param policyTopics policyTopics or {@code null} for none
*/
public PolicyIssue setPolicyTopics(java.util.List policyTopics) {
this.policyTopics = policyTopics;
return this;
}
/**
* Required. Hostname/domain of the entity (for example "foo.com" or "www.foo.com"). This _should_
* be a bare domain/host name without any protocol. This will be present for all policy issues.
* @return value or {@code null} for none
*/
public java.lang.String getSite() {
return site;
}
/**
* Required. Hostname/domain of the entity (for example "foo.com" or "www.foo.com"). This _should_
* be a bare domain/host name without any protocol. This will be present for all policy issues.
* @param site site or {@code null} for none
*/
public PolicyIssue setSite(java.lang.String site) {
this.site = site;
return this;
}
/**
* Optional. Prefix of the site-section having policy issues (For example "foo.com/bar-section").
* This will be present if the `entity_type` is `SITE_SECTION` and will be absent for other entity
* types.
* @return value or {@code null} for none
*/
public java.lang.String getSiteSection() {
return siteSection;
}
/**
* Optional. Prefix of the site-section having policy issues (For example "foo.com/bar-section").
* This will be present if the `entity_type` is `SITE_SECTION` and will be absent for other entity
* types.
* @param siteSection siteSection or {@code null} for none
*/
public PolicyIssue setSiteSection(java.lang.String siteSection) {
this.siteSection = siteSection;
return this;
}
/**
* Optional. URI of the page having policy violations (for example "foo.com/bar" or
* "www.foo.com/bar"). This will be present if the `entity_type` is `PAGE` and will be absent for
* other entity types.
* @return value or {@code null} for none
*/
public java.lang.String getUri() {
return uri;
}
/**
* Optional. URI of the page having policy violations (for example "foo.com/bar" or
* "www.foo.com/bar"). This will be present if the `entity_type` is `PAGE` and will be absent for
* other entity types.
* @param uri uri or {@code null} for none
*/
public PolicyIssue setUri(java.lang.String uri) {
this.uri = uri;
return this;
}
/**
* Optional. The date (in the America/Los_Angeles timezone) when the entity will have ad serving
* demand restricted or ad serving disabled. This is present only for issues with a `WARNED`
* enforcement action. See https://support.google.com/adsense/answer/11066888.
* @return value or {@code null} for none
*/
public Date getWarningEscalationDate() {
return warningEscalationDate;
}
/**
* Optional. The date (in the America/Los_Angeles timezone) when the entity will have ad serving
* demand restricted or ad serving disabled. This is present only for issues with a `WARNED`
* enforcement action. See https://support.google.com/adsense/answer/11066888.
* @param warningEscalationDate warningEscalationDate or {@code null} for none
*/
public PolicyIssue setWarningEscalationDate(Date warningEscalationDate) {
this.warningEscalationDate = warningEscalationDate;
return this;
}
@Override
public PolicyIssue set(String fieldName, Object value) {
return (PolicyIssue) super.set(fieldName, value);
}
@Override
public PolicyIssue clone() {
return (PolicyIssue) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy