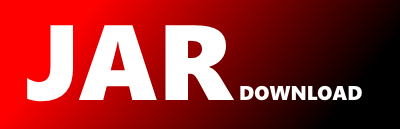
com.google.api.services.analyticshub.v1.model.DataExchange Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.analyticshub.v1.model;
/**
* A data exchange is a container that lets you share data. Along with the descriptive information
* about the data exchange, it contains listings that reference shared datasets.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Analytics Hub API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DataExchange extends com.google.api.client.json.GenericJson {
/**
* Optional. Description of the data exchange. The description must not contain Unicode non-
* characters as well as C0 and C1 control codes except tabs (HT), new lines (LF), carriage
* returns (CR), and page breaks (FF). Default value is an empty string. Max length: 2000 bytes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Optional. Type of discovery on the discovery page for all the listings under this exchange.
* Updating this field also updates (overwrites) the discovery_type field for all the listings
* under this exchange.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String discoveryType;
/**
* Required. Human-readable display name of the data exchange. The display name must contain only
* Unicode letters, numbers (0-9), underscores (_), dashes (-), spaces ( ), ampersands (&) and
* must not start or end with spaces. Default value is an empty string. Max length: 63 bytes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* Optional. Documentation describing the data exchange.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String documentation;
/**
* Optional. Base64 encoded image representing the data exchange. Max Size: 3.0MiB Expected image
* dimensions are 512x512 pixels, however the API only performs validation on size of the encoded
* data. Note: For byte fields, the content of the fields are base64-encoded (which increases the
* size of the data by 33-36%) when using JSON on the wire.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String icon;
/**
* Output only. Number of listings contained in the data exchange.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer listingCount;
/**
* Output only. The resource name of the data exchange. e.g.
* `projects/myproject/locations/US/dataExchanges/123`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Optional. Email or URL of the primary point of contact of the data exchange. Max Length: 1000
* bytes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String primaryContact;
/**
* Optional. Configurable data sharing environment option for a data exchange.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SharingEnvironmentConfig sharingEnvironmentConfig;
/**
* Optional. Description of the data exchange. The description must not contain Unicode non-
* characters as well as C0 and C1 control codes except tabs (HT), new lines (LF), carriage
* returns (CR), and page breaks (FF). Default value is an empty string. Max length: 2000 bytes.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Optional. Description of the data exchange. The description must not contain Unicode non-
* characters as well as C0 and C1 control codes except tabs (HT), new lines (LF), carriage
* returns (CR), and page breaks (FF). Default value is an empty string. Max length: 2000 bytes.
* @param description description or {@code null} for none
*/
public DataExchange setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Optional. Type of discovery on the discovery page for all the listings under this exchange.
* Updating this field also updates (overwrites) the discovery_type field for all the listings
* under this exchange.
* @return value or {@code null} for none
*/
public java.lang.String getDiscoveryType() {
return discoveryType;
}
/**
* Optional. Type of discovery on the discovery page for all the listings under this exchange.
* Updating this field also updates (overwrites) the discovery_type field for all the listings
* under this exchange.
* @param discoveryType discoveryType or {@code null} for none
*/
public DataExchange setDiscoveryType(java.lang.String discoveryType) {
this.discoveryType = discoveryType;
return this;
}
/**
* Required. Human-readable display name of the data exchange. The display name must contain only
* Unicode letters, numbers (0-9), underscores (_), dashes (-), spaces ( ), ampersands (&) and
* must not start or end with spaces. Default value is an empty string. Max length: 63 bytes.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* Required. Human-readable display name of the data exchange. The display name must contain only
* Unicode letters, numbers (0-9), underscores (_), dashes (-), spaces ( ), ampersands (&) and
* must not start or end with spaces. Default value is an empty string. Max length: 63 bytes.
* @param displayName displayName or {@code null} for none
*/
public DataExchange setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* Optional. Documentation describing the data exchange.
* @return value or {@code null} for none
*/
public java.lang.String getDocumentation() {
return documentation;
}
/**
* Optional. Documentation describing the data exchange.
* @param documentation documentation or {@code null} for none
*/
public DataExchange setDocumentation(java.lang.String documentation) {
this.documentation = documentation;
return this;
}
/**
* Optional. Base64 encoded image representing the data exchange. Max Size: 3.0MiB Expected image
* dimensions are 512x512 pixels, however the API only performs validation on size of the encoded
* data. Note: For byte fields, the content of the fields are base64-encoded (which increases the
* size of the data by 33-36%) when using JSON on the wire.
* @see #decodeIcon()
* @return value or {@code null} for none
*/
public java.lang.String getIcon() {
return icon;
}
/**
* Optional. Base64 encoded image representing the data exchange. Max Size: 3.0MiB Expected image
* dimensions are 512x512 pixels, however the API only performs validation on size of the encoded
* data. Note: For byte fields, the content of the fields are base64-encoded (which increases the
* size of the data by 33-36%) when using JSON on the wire.
* @see #getIcon()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeIcon() {
return com.google.api.client.util.Base64.decodeBase64(icon);
}
/**
* Optional. Base64 encoded image representing the data exchange. Max Size: 3.0MiB Expected image
* dimensions are 512x512 pixels, however the API only performs validation on size of the encoded
* data. Note: For byte fields, the content of the fields are base64-encoded (which increases the
* size of the data by 33-36%) when using JSON on the wire.
* @see #encodeIcon()
* @param icon icon or {@code null} for none
*/
public DataExchange setIcon(java.lang.String icon) {
this.icon = icon;
return this;
}
/**
* Optional. Base64 encoded image representing the data exchange. Max Size: 3.0MiB Expected image
* dimensions are 512x512 pixels, however the API only performs validation on size of the encoded
* data. Note: For byte fields, the content of the fields are base64-encoded (which increases the
* size of the data by 33-36%) when using JSON on the wire.
* @see #setIcon()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public DataExchange encodeIcon(byte[] icon) {
this.icon = com.google.api.client.util.Base64.encodeBase64URLSafeString(icon);
return this;
}
/**
* Output only. Number of listings contained in the data exchange.
* @return value or {@code null} for none
*/
public java.lang.Integer getListingCount() {
return listingCount;
}
/**
* Output only. Number of listings contained in the data exchange.
* @param listingCount listingCount or {@code null} for none
*/
public DataExchange setListingCount(java.lang.Integer listingCount) {
this.listingCount = listingCount;
return this;
}
/**
* Output only. The resource name of the data exchange. e.g.
* `projects/myproject/locations/US/dataExchanges/123`.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The resource name of the data exchange. e.g.
* `projects/myproject/locations/US/dataExchanges/123`.
* @param name name or {@code null} for none
*/
public DataExchange setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Optional. Email or URL of the primary point of contact of the data exchange. Max Length: 1000
* bytes.
* @return value or {@code null} for none
*/
public java.lang.String getPrimaryContact() {
return primaryContact;
}
/**
* Optional. Email or URL of the primary point of contact of the data exchange. Max Length: 1000
* bytes.
* @param primaryContact primaryContact or {@code null} for none
*/
public DataExchange setPrimaryContact(java.lang.String primaryContact) {
this.primaryContact = primaryContact;
return this;
}
/**
* Optional. Configurable data sharing environment option for a data exchange.
* @return value or {@code null} for none
*/
public SharingEnvironmentConfig getSharingEnvironmentConfig() {
return sharingEnvironmentConfig;
}
/**
* Optional. Configurable data sharing environment option for a data exchange.
* @param sharingEnvironmentConfig sharingEnvironmentConfig or {@code null} for none
*/
public DataExchange setSharingEnvironmentConfig(SharingEnvironmentConfig sharingEnvironmentConfig) {
this.sharingEnvironmentConfig = sharingEnvironmentConfig;
return this;
}
@Override
public DataExchange set(String fieldName, Object value) {
return (DataExchange) super.set(fieldName, value);
}
@Override
public DataExchange clone() {
return (DataExchange) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy