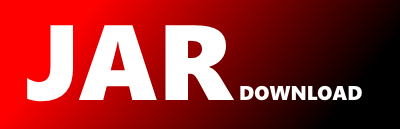
com.google.api.services.androiddeviceprovisioning.v1.AndroidProvisioningPartner Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androiddeviceprovisioning.v1;
/**
* Service definition for AndroidProvisioningPartner (v1).
*
*
* Automates Android zero-touch enrollment for device resellers, customers, and EMMs.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link AndroidProvisioningPartnerRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class AndroidProvisioningPartner extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Android Device Provisioning Partner API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://androiddeviceprovisioning.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://androiddeviceprovisioning.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public AndroidProvisioningPartner(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
AndroidProvisioningPartner(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Customers collection.
*
* The typical use is:
*
* {@code AndroidProvisioningPartner androiddeviceprovisioning = new AndroidProvisioningPartner(...);}
* {@code AndroidProvisioningPartner.Customers.List request = androiddeviceprovisioning.customers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Customers customers() {
return new Customers();
}
/**
* The "customers" collection of methods.
*/
public class Customers {
/**
* Lists the user's customer accounts.
*
* Create a request for the method "customers.list".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/customers";
/**
* Lists the user's customer accounts.
*
* Create a request for the method "customers.list".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(AndroidProvisioningPartner.this, "GET", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.CustomerListCustomersResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The maximum number of customers to show in a page of results. A number between 1
* and 100 (inclusive).
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Required. The maximum number of customers to show in a page of results. A number between 1 and 100
(inclusive).
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Required. The maximum number of customers to show in a page of results. A number between 1
* and 100 (inclusive).
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A token specifying which result page to return. This field has custom validations in
* ListCustomersRequestValidator
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token specifying which result page to return. This field has custom validations in
ListCustomersRequestValidator
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token specifying which result page to return. This field has custom validations in
* ListCustomersRequestValidator
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Configurations collection.
*
* The typical use is:
*
* {@code AndroidProvisioningPartner androiddeviceprovisioning = new AndroidProvisioningPartner(...);}
* {@code AndroidProvisioningPartner.Configurations.List request = androiddeviceprovisioning.configurations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Configurations configurations() {
return new Configurations();
}
/**
* The "configurations" collection of methods.
*/
public class Configurations {
/**
* Creates a new configuration. Once created, a customer can apply the configuration to devices.
*
* Create a request for the method "configurations.create".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The customer that manages the configuration. An API resource name in the format
* `customers/[CUSTOMER_ID]`. This field has custom validation in
* CreateConfigurationRequestValidator
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.Configuration}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.androiddeviceprovisioning.v1.model.Configuration content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+parent}/configurations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+$");
/**
* Creates a new configuration. Once created, a customer can apply the configuration to devices.
*
* Create a request for the method "configurations.create".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The customer that manages the configuration. An API resource name in the format
* `customers/[CUSTOMER_ID]`. This field has custom validation in
* CreateConfigurationRequestValidator
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.Configuration}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.androiddeviceprovisioning.v1.model.Configuration content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.Configuration.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The customer that manages the configuration. An API resource name in the format
* `customers/[CUSTOMER_ID]`. This field has custom validation in
* CreateConfigurationRequestValidator
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The customer that manages the configuration. An API resource name in the format
`customers/[CUSTOMER_ID]`. This field has custom validation in CreateConfigurationRequestValidator
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The customer that manages the configuration. An API resource name in the format
* `customers/[CUSTOMER_ID]`. This field has custom validation in
* CreateConfigurationRequestValidator
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an unused configuration. The API call fails if the customer has devices with the
* configuration applied.
*
* Create a request for the method "configurations.delete".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The configuration to delete. An API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. If the configuration is
* applied to any devices, the API call fails.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+/configurations/[^/]+$");
/**
* Deletes an unused configuration. The API call fails if the customer has devices with the
* configuration applied.
*
* Create a request for the method "configurations.delete".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The configuration to delete. An API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. If the configuration is
* applied to any devices, the API call fails.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AndroidProvisioningPartner.this, "DELETE", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/configurations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The configuration to delete. An API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. If the configuration is
* applied to any devices, the API call fails.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The configuration to delete. An API resource name in the format
`customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. If the configuration is applied to any
devices, the API call fails.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The configuration to delete. An API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. If the configuration is
* applied to any devices, the API call fails.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/configurations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the details of a configuration.
*
* Create a request for the method "configurations.get".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The configuration to get. An API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+/configurations/[^/]+$");
/**
* Gets the details of a configuration.
*
* Create a request for the method "configurations.get".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Get#execute()} method to invoke the remote
* operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The configuration to get. An API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidProvisioningPartner.this, "GET", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.Configuration.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/configurations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The configuration to get. An API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The configuration to get. An API resource name in the format
`customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The configuration to get. An API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/configurations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists a customer's configurations.
*
* Create a request for the method "configurations.list".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The customer that manages the listed configurations. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+parent}/configurations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+$");
/**
* Lists a customer's configurations.
*
* Create a request for the method "configurations.list".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The customer that manages the listed configurations. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AndroidProvisioningPartner.this, "GET", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.CustomerListConfigurationsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The customer that manages the listed configurations. An API resource name in
* the format `customers/[CUSTOMER_ID]`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The customer that manages the listed configurations. An API resource name in the format
`customers/[CUSTOMER_ID]`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The customer that manages the listed configurations. An API resource name in
* the format `customers/[CUSTOMER_ID]`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a configuration's field values.
*
* Create a request for the method "configurations.patch".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. The API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. Assigned by the server.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.Configuration}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.androiddeviceprovisioning.v1.model.Configuration content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+/configurations/[^/]+$");
/**
* Updates a configuration's field values.
*
* Create a request for the method "configurations.patch".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. The API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. Assigned by the server.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.Configuration}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.androiddeviceprovisioning.v1.model.Configuration content) {
super(AndroidProvisioningPartner.this, "PATCH", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.Configuration.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/configurations/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Output only. The API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. Assigned by the server.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. The API resource name in the format
`customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. Assigned by the server.
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. Assigned by the server.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/configurations/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. The field mask applied to the target `Configuration` before updating the
* fields. To learn more about using field masks, read [FieldMask](/protocol-
* buffers/docs/reference/google.protobuf#fieldmask) in the Protocol Buffers documentation.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The field mask applied to the target `Configuration` before updating the fields. To learn
more about using field masks, read [FieldMask](/protocol-
buffers/docs/reference/google.protobuf#fieldmask) in the Protocol Buffers documentation.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The field mask applied to the target `Configuration` before updating the
* fields. To learn more about using field masks, read [FieldMask](/protocol-
* buffers/docs/reference/google.protobuf#fieldmask) in the Protocol Buffers documentation.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Devices collection.
*
* The typical use is:
*
* {@code AndroidProvisioningPartner androiddeviceprovisioning = new AndroidProvisioningPartner(...);}
* {@code AndroidProvisioningPartner.Devices.List request = androiddeviceprovisioning.devices().list(parameters ...)}
*
*
* @return the resource collection
*/
public Devices devices() {
return new Devices();
}
/**
* The "devices" collection of methods.
*/
public class Devices {
/**
* Applies a Configuration to the device to register the device for zero-touch enrollment. After
* applying a configuration to a device, the device automatically provisions itself on first boot,
* or next factory reset.
*
* Create a request for the method "devices.applyConfiguration".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link ApplyConfiguration#execute()} method to invoke the
* remote operation.
*
* @param parent Required. The customer managing the device. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.CustomerApplyConfigurationRequest}
* @return the request
*/
public ApplyConfiguration applyConfiguration(java.lang.String parent, com.google.api.services.androiddeviceprovisioning.v1.model.CustomerApplyConfigurationRequest content) throws java.io.IOException {
ApplyConfiguration result = new ApplyConfiguration(parent, content);
initialize(result);
return result;
}
public class ApplyConfiguration extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+parent}/devices:applyConfiguration";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+$");
/**
* Applies a Configuration to the device to register the device for zero-touch enrollment. After
* applying a configuration to a device, the device automatically provisions itself on first boot,
* or next factory reset.
*
* Create a request for the method "devices.applyConfiguration".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link ApplyConfiguration#execute()} method to invoke
* the remote operation. {@link ApplyConfiguration#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param parent Required. The customer managing the device. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.CustomerApplyConfigurationRequest}
* @since 1.13
*/
protected ApplyConfiguration(java.lang.String parent, com.google.api.services.androiddeviceprovisioning.v1.model.CustomerApplyConfigurationRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.Empty.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
}
@Override
public ApplyConfiguration set$Xgafv(java.lang.String $Xgafv) {
return (ApplyConfiguration) super.set$Xgafv($Xgafv);
}
@Override
public ApplyConfiguration setAccessToken(java.lang.String accessToken) {
return (ApplyConfiguration) super.setAccessToken(accessToken);
}
@Override
public ApplyConfiguration setAlt(java.lang.String alt) {
return (ApplyConfiguration) super.setAlt(alt);
}
@Override
public ApplyConfiguration setCallback(java.lang.String callback) {
return (ApplyConfiguration) super.setCallback(callback);
}
@Override
public ApplyConfiguration setFields(java.lang.String fields) {
return (ApplyConfiguration) super.setFields(fields);
}
@Override
public ApplyConfiguration setKey(java.lang.String key) {
return (ApplyConfiguration) super.setKey(key);
}
@Override
public ApplyConfiguration setOauthToken(java.lang.String oauthToken) {
return (ApplyConfiguration) super.setOauthToken(oauthToken);
}
@Override
public ApplyConfiguration setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ApplyConfiguration) super.setPrettyPrint(prettyPrint);
}
@Override
public ApplyConfiguration setQuotaUser(java.lang.String quotaUser) {
return (ApplyConfiguration) super.setQuotaUser(quotaUser);
}
@Override
public ApplyConfiguration setUploadType(java.lang.String uploadType) {
return (ApplyConfiguration) super.setUploadType(uploadType);
}
@Override
public ApplyConfiguration setUploadProtocol(java.lang.String uploadProtocol) {
return (ApplyConfiguration) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The customer managing the device. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The customer managing the device. An API resource name in the format
`customers/[CUSTOMER_ID]`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The customer managing the device. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
*/
public ApplyConfiguration setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public ApplyConfiguration set(String parameterName, Object value) {
return (ApplyConfiguration) super.set(parameterName, value);
}
}
/**
* Gets the details of a device.
*
* Create a request for the method "devices.get".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The device to get. An API resource name in the format
* `customers/[CUSTOMER_ID]/devices/[DEVICE_ID]`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+/devices/[^/]+$");
/**
* Gets the details of a device.
*
* Create a request for the method "devices.get".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Get#execute()} method to invoke the remote
* operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The device to get. An API resource name in the format
* `customers/[CUSTOMER_ID]/devices/[DEVICE_ID]`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidProvisioningPartner.this, "GET", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.Device.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/devices/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The device to get. An API resource name in the format
* `customers/[CUSTOMER_ID]/devices/[DEVICE_ID]`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The device to get. An API resource name in the format
`customers/[CUSTOMER_ID]/devices/[DEVICE_ID]`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The device to get. An API resource name in the format
* `customers/[CUSTOMER_ID]/devices/[DEVICE_ID]`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^customers/[^/]+/devices/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists a customer's devices.
*
* Create a request for the method "devices.list".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The customer managing the devices. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+parent}/devices";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+$");
/**
* Lists a customer's devices.
*
* Create a request for the method "devices.list".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The customer managing the devices. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AndroidProvisioningPartner.this, "GET", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.CustomerListDevicesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The customer managing the devices. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The customer managing the devices. An API resource name in the format
`customers/[CUSTOMER_ID]`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The customer managing the devices. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The maximum number of devices to show in a page of results. Must be between 1
* and 100 inclusive.
*/
@com.google.api.client.util.Key
private java.lang.Long pageSize;
/** Required. The maximum number of devices to show in a page of results. Must be between 1 and 100
inclusive.
*/
public java.lang.Long getPageSize() {
return pageSize;
}
/**
* Required. The maximum number of devices to show in a page of results. Must be between 1
* and 100 inclusive.
*/
public List setPageSize(java.lang.Long pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token specifying which result page to return. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token specifying which result page to return.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token specifying which result page to return. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Removes a configuration from device.
*
* Create a request for the method "devices.removeConfiguration".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link RemoveConfiguration#execute()} method to invoke the
* remote operation.
*
* @param parent Required. The customer managing the device in the format `customers/[CUSTOMER_ID]`.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.CustomerRemoveConfigurationRequest}
* @return the request
*/
public RemoveConfiguration removeConfiguration(java.lang.String parent, com.google.api.services.androiddeviceprovisioning.v1.model.CustomerRemoveConfigurationRequest content) throws java.io.IOException {
RemoveConfiguration result = new RemoveConfiguration(parent, content);
initialize(result);
return result;
}
public class RemoveConfiguration extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+parent}/devices:removeConfiguration";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+$");
/**
* Removes a configuration from device.
*
* Create a request for the method "devices.removeConfiguration".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link RemoveConfiguration#execute()} method to
* invoke the remote operation. {@link RemoveConfiguration#initialize(com.google.api.client.go
* ogleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param parent Required. The customer managing the device in the format `customers/[CUSTOMER_ID]`.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.CustomerRemoveConfigurationRequest}
* @since 1.13
*/
protected RemoveConfiguration(java.lang.String parent, com.google.api.services.androiddeviceprovisioning.v1.model.CustomerRemoveConfigurationRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.Empty.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
}
@Override
public RemoveConfiguration set$Xgafv(java.lang.String $Xgafv) {
return (RemoveConfiguration) super.set$Xgafv($Xgafv);
}
@Override
public RemoveConfiguration setAccessToken(java.lang.String accessToken) {
return (RemoveConfiguration) super.setAccessToken(accessToken);
}
@Override
public RemoveConfiguration setAlt(java.lang.String alt) {
return (RemoveConfiguration) super.setAlt(alt);
}
@Override
public RemoveConfiguration setCallback(java.lang.String callback) {
return (RemoveConfiguration) super.setCallback(callback);
}
@Override
public RemoveConfiguration setFields(java.lang.String fields) {
return (RemoveConfiguration) super.setFields(fields);
}
@Override
public RemoveConfiguration setKey(java.lang.String key) {
return (RemoveConfiguration) super.setKey(key);
}
@Override
public RemoveConfiguration setOauthToken(java.lang.String oauthToken) {
return (RemoveConfiguration) super.setOauthToken(oauthToken);
}
@Override
public RemoveConfiguration setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RemoveConfiguration) super.setPrettyPrint(prettyPrint);
}
@Override
public RemoveConfiguration setQuotaUser(java.lang.String quotaUser) {
return (RemoveConfiguration) super.setQuotaUser(quotaUser);
}
@Override
public RemoveConfiguration setUploadType(java.lang.String uploadType) {
return (RemoveConfiguration) super.setUploadType(uploadType);
}
@Override
public RemoveConfiguration setUploadProtocol(java.lang.String uploadProtocol) {
return (RemoveConfiguration) super.setUploadProtocol(uploadProtocol);
}
/** Required. The customer managing the device in the format `customers/[CUSTOMER_ID]`. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The customer managing the device in the format `customers/[CUSTOMER_ID]`.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The customer managing the device in the format `customers/[CUSTOMER_ID]`. */
public RemoveConfiguration setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public RemoveConfiguration set(String parameterName, Object value) {
return (RemoveConfiguration) super.set(parameterName, value);
}
}
/**
* Unclaims a device from a customer and removes it from zero-touch enrollment. After removing a
* device, a customer must contact their reseller to register the device into zero-touch enrollment
* again.
*
* Create a request for the method "devices.unclaim".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Unclaim#execute()} method to invoke the remote
* operation.
*
* @param parent Required. The customer managing the device. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.CustomerUnclaimDeviceRequest}
* @return the request
*/
public Unclaim unclaim(java.lang.String parent, com.google.api.services.androiddeviceprovisioning.v1.model.CustomerUnclaimDeviceRequest content) throws java.io.IOException {
Unclaim result = new Unclaim(parent, content);
initialize(result);
return result;
}
public class Unclaim extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+parent}/devices:unclaim";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+$");
/**
* Unclaims a device from a customer and removes it from zero-touch enrollment. After removing a
* device, a customer must contact their reseller to register the device into zero-touch
* enrollment again.
*
* Create a request for the method "devices.unclaim".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Unclaim#execute()} method to invoke the remote
* operation. {@link
* Unclaim#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The customer managing the device. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.CustomerUnclaimDeviceRequest}
* @since 1.13
*/
protected Unclaim(java.lang.String parent, com.google.api.services.androiddeviceprovisioning.v1.model.CustomerUnclaimDeviceRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.Empty.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
}
@Override
public Unclaim set$Xgafv(java.lang.String $Xgafv) {
return (Unclaim) super.set$Xgafv($Xgafv);
}
@Override
public Unclaim setAccessToken(java.lang.String accessToken) {
return (Unclaim) super.setAccessToken(accessToken);
}
@Override
public Unclaim setAlt(java.lang.String alt) {
return (Unclaim) super.setAlt(alt);
}
@Override
public Unclaim setCallback(java.lang.String callback) {
return (Unclaim) super.setCallback(callback);
}
@Override
public Unclaim setFields(java.lang.String fields) {
return (Unclaim) super.setFields(fields);
}
@Override
public Unclaim setKey(java.lang.String key) {
return (Unclaim) super.setKey(key);
}
@Override
public Unclaim setOauthToken(java.lang.String oauthToken) {
return (Unclaim) super.setOauthToken(oauthToken);
}
@Override
public Unclaim setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Unclaim) super.setPrettyPrint(prettyPrint);
}
@Override
public Unclaim setQuotaUser(java.lang.String quotaUser) {
return (Unclaim) super.setQuotaUser(quotaUser);
}
@Override
public Unclaim setUploadType(java.lang.String uploadType) {
return (Unclaim) super.setUploadType(uploadType);
}
@Override
public Unclaim setUploadProtocol(java.lang.String uploadProtocol) {
return (Unclaim) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The customer managing the device. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The customer managing the device. An API resource name in the format
`customers/[CUSTOMER_ID]`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The customer managing the device. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
*/
public Unclaim setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Unclaim set(String parameterName, Object value) {
return (Unclaim) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Dpcs collection.
*
* The typical use is:
*
* {@code AndroidProvisioningPartner androiddeviceprovisioning = new AndroidProvisioningPartner(...);}
* {@code AndroidProvisioningPartner.Dpcs.List request = androiddeviceprovisioning.dpcs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Dpcs dpcs() {
return new Dpcs();
}
/**
* The "dpcs" collection of methods.
*/
public class Dpcs {
/**
* Lists the DPCs (device policy controllers) that support zero-touch enrollment.
*
* Create a request for the method "dpcs.list".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The customer that can use the DPCs in configurations. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+parent}/dpcs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^customers/[^/]+$");
/**
* Lists the DPCs (device policy controllers) that support zero-touch enrollment.
*
* Create a request for the method "dpcs.list".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The customer that can use the DPCs in configurations. An API resource name in the format
* `customers/[CUSTOMER_ID]`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AndroidProvisioningPartner.this, "GET", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.CustomerListDpcsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The customer that can use the DPCs in configurations. An API resource name in
* the format `customers/[CUSTOMER_ID]`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The customer that can use the DPCs in configurations. An API resource name in the format
`customers/[CUSTOMER_ID]`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The customer that can use the DPCs in configurations. An API resource name in
* the format `customers/[CUSTOMER_ID]`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^customers/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code AndroidProvisioningPartner androiddeviceprovisioning = new AndroidProvisioningPartner(...);}
* {@code AndroidProvisioningPartner.Operations.List request = androiddeviceprovisioning.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations/.*$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Get#execute()} method to invoke the remote
* operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidProvisioningPartner.this, "GET", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Partners collection.
*
* The typical use is:
*
* {@code AndroidProvisioningPartner androiddeviceprovisioning = new AndroidProvisioningPartner(...);}
* {@code AndroidProvisioningPartner.Partners.List request = androiddeviceprovisioning.partners().list(parameters ...)}
*
*
* @return the resource collection
*/
public Partners partners() {
return new Partners();
}
/**
* The "partners" collection of methods.
*/
public class Partners {
/**
* An accessor for creating requests from the Customers collection.
*
* The typical use is:
*
* {@code AndroidProvisioningPartner androiddeviceprovisioning = new AndroidProvisioningPartner(...);}
* {@code AndroidProvisioningPartner.Customers.List request = androiddeviceprovisioning.customers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Customers customers() {
return new Customers();
}
/**
* The "customers" collection of methods.
*/
public class Customers {
/**
* Creates a customer for zero-touch enrollment. After the method returns successfully, admin and
* owner roles can manage devices and EMM configs by calling API methods or using their zero-touch
* enrollment portal. The customer receives an email that welcomes them to zero-touch enrollment and
* explains how to sign into the portal.
*
* Create a request for the method "customers.create".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The parent resource ID in the format `partners/[PARTNER_ID]` that identifies the reseller.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.CreateCustomerRequest}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.androiddeviceprovisioning.v1.model.CreateCustomerRequest content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+parent}/customers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+$");
/**
* Creates a customer for zero-touch enrollment. After the method returns successfully, admin and
* owner roles can manage devices and EMM configs by calling API methods or using their zero-touch
* enrollment portal. The customer receives an email that welcomes them to zero-touch enrollment
* and explains how to sign into the portal.
*
* Create a request for the method "customers.create".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The parent resource ID in the format `partners/[PARTNER_ID]` that identifies the reseller.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.CreateCustomerRequest}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.androiddeviceprovisioning.v1.model.CreateCustomerRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.Company.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The parent resource ID in the format `partners/[PARTNER_ID]` that identifies
* the reseller.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The parent resource ID in the format `partners/[PARTNER_ID]` that identifies the
reseller.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The parent resource ID in the format `partners/[PARTNER_ID]` that identifies
* the reseller.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Lists the customers that are enrolled to the reseller identified by the `partnerId` argument.
* This list includes customers that the reseller created and customers that enrolled themselves
* using the portal.
*
* Create a request for the method "customers.list".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param partnerId Required. The ID of the reseller partner.
* @return the request
*/
public List list(java.lang.Long partnerId) throws java.io.IOException {
List result = new List(partnerId);
initialize(result);
return result;
}
public class List extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/partners/{+partnerId}/customers";
private final java.util.regex.Pattern PARTNER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Lists the customers that are enrolled to the reseller identified by the `partnerId` argument.
* This list includes customers that the reseller created and customers that enrolled themselves
* using the portal.
*
* Create a request for the method "customers.list".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param partnerId Required. The ID of the reseller partner.
* @since 1.13
*/
protected List(java.lang.Long partnerId) {
super(AndroidProvisioningPartner.this, "GET", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.ListCustomersResponse.class);
this.partnerId = com.google.api.client.util.Preconditions.checkNotNull(partnerId, "Required parameter partnerId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the reseller partner. */
@com.google.api.client.util.Key
private java.lang.Long partnerId;
/** Required. The ID of the reseller partner.
*/
public java.lang.Long getPartnerId() {
return partnerId;
}
/** Required. The ID of the reseller partner. */
public List setPartnerId(java.lang.Long partnerId) {
this.partnerId = partnerId;
return this;
}
/**
* The maximum number of results to be returned. If not specified or 0, all the records are
* returned.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to be returned. If not specified or 0, all the records are returned.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to be returned. If not specified or 0, all the records are
* returned.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token identifying a page of results returned by the server. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results returned by the server.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token identifying a page of results returned by the server. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Devices collection.
*
* The typical use is:
*
* {@code AndroidProvisioningPartner androiddeviceprovisioning = new AndroidProvisioningPartner(...);}
* {@code AndroidProvisioningPartner.Devices.List request = androiddeviceprovisioning.devices().list(parameters ...)}
*
*
* @return the resource collection
*/
public Devices devices() {
return new Devices();
}
/**
* The "devices" collection of methods.
*/
public class Devices {
/**
* Claims a device for a customer and adds it to zero-touch enrollment. If the device is already
* claimed by another customer, the call returns an error.
*
* Create a request for the method "devices.claim".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Claim#execute()} method to invoke the remote operation.
*
* @param partnerId Required. The ID of the reseller partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.ClaimDeviceRequest}
* @return the request
*/
public Claim claim(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.ClaimDeviceRequest content) throws java.io.IOException {
Claim result = new Claim(partnerId, content);
initialize(result);
return result;
}
public class Claim extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/partners/{+partnerId}/devices:claim";
private final java.util.regex.Pattern PARTNER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Claims a device for a customer and adds it to zero-touch enrollment. If the device is already
* claimed by another customer, the call returns an error.
*
* Create a request for the method "devices.claim".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Claim#execute()} method to invoke the remote
* operation. {@link
* Claim#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param partnerId Required. The ID of the reseller partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.ClaimDeviceRequest}
* @since 1.13
*/
protected Claim(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.ClaimDeviceRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.ClaimDeviceResponse.class);
this.partnerId = com.google.api.client.util.Preconditions.checkNotNull(partnerId, "Required parameter partnerId must be specified.");
}
@Override
public Claim set$Xgafv(java.lang.String $Xgafv) {
return (Claim) super.set$Xgafv($Xgafv);
}
@Override
public Claim setAccessToken(java.lang.String accessToken) {
return (Claim) super.setAccessToken(accessToken);
}
@Override
public Claim setAlt(java.lang.String alt) {
return (Claim) super.setAlt(alt);
}
@Override
public Claim setCallback(java.lang.String callback) {
return (Claim) super.setCallback(callback);
}
@Override
public Claim setFields(java.lang.String fields) {
return (Claim) super.setFields(fields);
}
@Override
public Claim setKey(java.lang.String key) {
return (Claim) super.setKey(key);
}
@Override
public Claim setOauthToken(java.lang.String oauthToken) {
return (Claim) super.setOauthToken(oauthToken);
}
@Override
public Claim setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Claim) super.setPrettyPrint(prettyPrint);
}
@Override
public Claim setQuotaUser(java.lang.String quotaUser) {
return (Claim) super.setQuotaUser(quotaUser);
}
@Override
public Claim setUploadType(java.lang.String uploadType) {
return (Claim) super.setUploadType(uploadType);
}
@Override
public Claim setUploadProtocol(java.lang.String uploadProtocol) {
return (Claim) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the reseller partner. */
@com.google.api.client.util.Key
private java.lang.Long partnerId;
/** Required. The ID of the reseller partner.
*/
public java.lang.Long getPartnerId() {
return partnerId;
}
/** Required. The ID of the reseller partner. */
public Claim setPartnerId(java.lang.Long partnerId) {
this.partnerId = partnerId;
return this;
}
@Override
public Claim set(String parameterName, Object value) {
return (Claim) super.set(parameterName, value);
}
}
/**
* Claims a batch of devices for a customer asynchronously. Adds the devices to zero-touch
* enrollment. To learn more, read [Long‑running batch operations](/zero-touch/guides/how-it-
* works#operations).
*
* Create a request for the method "devices.claimAsync".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link ClaimAsync#execute()} method to invoke the remote
* operation.
*
* @param partnerId Required. The ID of the reseller partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.ClaimDevicesRequest}
* @return the request
*/
public ClaimAsync claimAsync(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.ClaimDevicesRequest content) throws java.io.IOException {
ClaimAsync result = new ClaimAsync(partnerId, content);
initialize(result);
return result;
}
public class ClaimAsync extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/partners/{+partnerId}/devices:claimAsync";
private final java.util.regex.Pattern PARTNER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Claims a batch of devices for a customer asynchronously. Adds the devices to zero-touch
* enrollment. To learn more, read [Long‑running batch operations](/zero-touch/guides/how-it-
* works#operations).
*
* Create a request for the method "devices.claimAsync".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link ClaimAsync#execute()} method to invoke the
* remote operation. {@link
* ClaimAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param partnerId Required. The ID of the reseller partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.ClaimDevicesRequest}
* @since 1.13
*/
protected ClaimAsync(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.ClaimDevicesRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.Operation.class);
this.partnerId = com.google.api.client.util.Preconditions.checkNotNull(partnerId, "Required parameter partnerId must be specified.");
}
@Override
public ClaimAsync set$Xgafv(java.lang.String $Xgafv) {
return (ClaimAsync) super.set$Xgafv($Xgafv);
}
@Override
public ClaimAsync setAccessToken(java.lang.String accessToken) {
return (ClaimAsync) super.setAccessToken(accessToken);
}
@Override
public ClaimAsync setAlt(java.lang.String alt) {
return (ClaimAsync) super.setAlt(alt);
}
@Override
public ClaimAsync setCallback(java.lang.String callback) {
return (ClaimAsync) super.setCallback(callback);
}
@Override
public ClaimAsync setFields(java.lang.String fields) {
return (ClaimAsync) super.setFields(fields);
}
@Override
public ClaimAsync setKey(java.lang.String key) {
return (ClaimAsync) super.setKey(key);
}
@Override
public ClaimAsync setOauthToken(java.lang.String oauthToken) {
return (ClaimAsync) super.setOauthToken(oauthToken);
}
@Override
public ClaimAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ClaimAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public ClaimAsync setQuotaUser(java.lang.String quotaUser) {
return (ClaimAsync) super.setQuotaUser(quotaUser);
}
@Override
public ClaimAsync setUploadType(java.lang.String uploadType) {
return (ClaimAsync) super.setUploadType(uploadType);
}
@Override
public ClaimAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (ClaimAsync) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the reseller partner. */
@com.google.api.client.util.Key
private java.lang.Long partnerId;
/** Required. The ID of the reseller partner.
*/
public java.lang.Long getPartnerId() {
return partnerId;
}
/** Required. The ID of the reseller partner. */
public ClaimAsync setPartnerId(java.lang.Long partnerId) {
this.partnerId = partnerId;
return this;
}
@Override
public ClaimAsync set(String parameterName, Object value) {
return (ClaimAsync) super.set(parameterName, value);
}
}
/**
* Finds devices by hardware identifiers, such as IMEI.
*
* Create a request for the method "devices.findByIdentifier".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link FindByIdentifier#execute()} method to invoke the remote
* operation.
*
* @param partnerId Required. The ID of the reseller partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.FindDevicesByDeviceIdentifierRequest}
* @return the request
*/
public FindByIdentifier findByIdentifier(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.FindDevicesByDeviceIdentifierRequest content) throws java.io.IOException {
FindByIdentifier result = new FindByIdentifier(partnerId, content);
initialize(result);
return result;
}
public class FindByIdentifier extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/partners/{+partnerId}/devices:findByIdentifier";
private final java.util.regex.Pattern PARTNER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Finds devices by hardware identifiers, such as IMEI.
*
* Create a request for the method "devices.findByIdentifier".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link FindByIdentifier#execute()} method to invoke
* the remote operation. {@link FindByIdentifier#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param partnerId Required. The ID of the reseller partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.FindDevicesByDeviceIdentifierRequest}
* @since 1.13
*/
protected FindByIdentifier(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.FindDevicesByDeviceIdentifierRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.FindDevicesByDeviceIdentifierResponse.class);
this.partnerId = com.google.api.client.util.Preconditions.checkNotNull(partnerId, "Required parameter partnerId must be specified.");
}
@Override
public FindByIdentifier set$Xgafv(java.lang.String $Xgafv) {
return (FindByIdentifier) super.set$Xgafv($Xgafv);
}
@Override
public FindByIdentifier setAccessToken(java.lang.String accessToken) {
return (FindByIdentifier) super.setAccessToken(accessToken);
}
@Override
public FindByIdentifier setAlt(java.lang.String alt) {
return (FindByIdentifier) super.setAlt(alt);
}
@Override
public FindByIdentifier setCallback(java.lang.String callback) {
return (FindByIdentifier) super.setCallback(callback);
}
@Override
public FindByIdentifier setFields(java.lang.String fields) {
return (FindByIdentifier) super.setFields(fields);
}
@Override
public FindByIdentifier setKey(java.lang.String key) {
return (FindByIdentifier) super.setKey(key);
}
@Override
public FindByIdentifier setOauthToken(java.lang.String oauthToken) {
return (FindByIdentifier) super.setOauthToken(oauthToken);
}
@Override
public FindByIdentifier setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FindByIdentifier) super.setPrettyPrint(prettyPrint);
}
@Override
public FindByIdentifier setQuotaUser(java.lang.String quotaUser) {
return (FindByIdentifier) super.setQuotaUser(quotaUser);
}
@Override
public FindByIdentifier setUploadType(java.lang.String uploadType) {
return (FindByIdentifier) super.setUploadType(uploadType);
}
@Override
public FindByIdentifier setUploadProtocol(java.lang.String uploadProtocol) {
return (FindByIdentifier) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the reseller partner. */
@com.google.api.client.util.Key
private java.lang.Long partnerId;
/** Required. The ID of the reseller partner.
*/
public java.lang.Long getPartnerId() {
return partnerId;
}
/** Required. The ID of the reseller partner. */
public FindByIdentifier setPartnerId(java.lang.Long partnerId) {
this.partnerId = partnerId;
return this;
}
@Override
public FindByIdentifier set(String parameterName, Object value) {
return (FindByIdentifier) super.set(parameterName, value);
}
}
/**
* Finds devices claimed for customers. The results only contain devices registered to the reseller
* that's identified by the `partnerId` argument. The customer's devices purchased from other
* resellers don't appear in the results.
*
* Create a request for the method "devices.findByOwner".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link FindByOwner#execute()} method to invoke the remote
* operation.
*
* @param partnerId Required. The ID of the reseller partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.FindDevicesByOwnerRequest}
* @return the request
*/
public FindByOwner findByOwner(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.FindDevicesByOwnerRequest content) throws java.io.IOException {
FindByOwner result = new FindByOwner(partnerId, content);
initialize(result);
return result;
}
public class FindByOwner extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/partners/{+partnerId}/devices:findByOwner";
private final java.util.regex.Pattern PARTNER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Finds devices claimed for customers. The results only contain devices registered to the
* reseller that's identified by the `partnerId` argument. The customer's devices purchased from
* other resellers don't appear in the results.
*
* Create a request for the method "devices.findByOwner".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link FindByOwner#execute()} method to invoke the
* remote operation. {@link
* FindByOwner#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param partnerId Required. The ID of the reseller partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.FindDevicesByOwnerRequest}
* @since 1.13
*/
protected FindByOwner(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.FindDevicesByOwnerRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.FindDevicesByOwnerResponse.class);
this.partnerId = com.google.api.client.util.Preconditions.checkNotNull(partnerId, "Required parameter partnerId must be specified.");
}
@Override
public FindByOwner set$Xgafv(java.lang.String $Xgafv) {
return (FindByOwner) super.set$Xgafv($Xgafv);
}
@Override
public FindByOwner setAccessToken(java.lang.String accessToken) {
return (FindByOwner) super.setAccessToken(accessToken);
}
@Override
public FindByOwner setAlt(java.lang.String alt) {
return (FindByOwner) super.setAlt(alt);
}
@Override
public FindByOwner setCallback(java.lang.String callback) {
return (FindByOwner) super.setCallback(callback);
}
@Override
public FindByOwner setFields(java.lang.String fields) {
return (FindByOwner) super.setFields(fields);
}
@Override
public FindByOwner setKey(java.lang.String key) {
return (FindByOwner) super.setKey(key);
}
@Override
public FindByOwner setOauthToken(java.lang.String oauthToken) {
return (FindByOwner) super.setOauthToken(oauthToken);
}
@Override
public FindByOwner setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FindByOwner) super.setPrettyPrint(prettyPrint);
}
@Override
public FindByOwner setQuotaUser(java.lang.String quotaUser) {
return (FindByOwner) super.setQuotaUser(quotaUser);
}
@Override
public FindByOwner setUploadType(java.lang.String uploadType) {
return (FindByOwner) super.setUploadType(uploadType);
}
@Override
public FindByOwner setUploadProtocol(java.lang.String uploadProtocol) {
return (FindByOwner) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the reseller partner. */
@com.google.api.client.util.Key
private java.lang.Long partnerId;
/** Required. The ID of the reseller partner.
*/
public java.lang.Long getPartnerId() {
return partnerId;
}
/** Required. The ID of the reseller partner. */
public FindByOwner setPartnerId(java.lang.Long partnerId) {
this.partnerId = partnerId;
return this;
}
@Override
public FindByOwner set(String parameterName, Object value) {
return (FindByOwner) super.set(parameterName, value);
}
}
/**
* Gets a device.
*
* Create a request for the method "devices.get".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The device API resource name in the format `partners/[PARTNER_ID]/devices/[DEVICE_ID]`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+/devices/[^/]+$");
/**
* Gets a device.
*
* Create a request for the method "devices.get".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Get#execute()} method to invoke the remote
* operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The device API resource name in the format `partners/[PARTNER_ID]/devices/[DEVICE_ID]`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidProvisioningPartner.this, "GET", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.Device.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/devices/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The device API resource name in the format
* `partners/[PARTNER_ID]/devices/[DEVICE_ID]`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The device API resource name in the format `partners/[PARTNER_ID]/devices/[DEVICE_ID]`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The device API resource name in the format
* `partners/[PARTNER_ID]/devices/[DEVICE_ID]`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^partners/[^/]+/devices/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets a device's SIM lock state.
*
* Create a request for the method "devices.getSimLockState".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link GetSimLockState#execute()} method to invoke the remote
* operation.
*
* @param partnerId Required. The ID of the partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.GetDeviceSimLockStateRequest}
* @return the request
*/
public GetSimLockState getSimLockState(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.GetDeviceSimLockStateRequest content) throws java.io.IOException {
GetSimLockState result = new GetSimLockState(partnerId, content);
initialize(result);
return result;
}
public class GetSimLockState extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/partners/{+partnerId}/devices:getSimLockState";
private final java.util.regex.Pattern PARTNER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Gets a device's SIM lock state.
*
* Create a request for the method "devices.getSimLockState".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link GetSimLockState#execute()} method to invoke
* the remote operation. {@link GetSimLockState#initialize(com.google.api.client.googleapis.se
* rvices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param partnerId Required. The ID of the partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.GetDeviceSimLockStateRequest}
* @since 1.13
*/
protected GetSimLockState(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.GetDeviceSimLockStateRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.GetDeviceSimLockStateResponse.class);
this.partnerId = com.google.api.client.util.Preconditions.checkNotNull(partnerId, "Required parameter partnerId must be specified.");
}
@Override
public GetSimLockState set$Xgafv(java.lang.String $Xgafv) {
return (GetSimLockState) super.set$Xgafv($Xgafv);
}
@Override
public GetSimLockState setAccessToken(java.lang.String accessToken) {
return (GetSimLockState) super.setAccessToken(accessToken);
}
@Override
public GetSimLockState setAlt(java.lang.String alt) {
return (GetSimLockState) super.setAlt(alt);
}
@Override
public GetSimLockState setCallback(java.lang.String callback) {
return (GetSimLockState) super.setCallback(callback);
}
@Override
public GetSimLockState setFields(java.lang.String fields) {
return (GetSimLockState) super.setFields(fields);
}
@Override
public GetSimLockState setKey(java.lang.String key) {
return (GetSimLockState) super.setKey(key);
}
@Override
public GetSimLockState setOauthToken(java.lang.String oauthToken) {
return (GetSimLockState) super.setOauthToken(oauthToken);
}
@Override
public GetSimLockState setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetSimLockState) super.setPrettyPrint(prettyPrint);
}
@Override
public GetSimLockState setQuotaUser(java.lang.String quotaUser) {
return (GetSimLockState) super.setQuotaUser(quotaUser);
}
@Override
public GetSimLockState setUploadType(java.lang.String uploadType) {
return (GetSimLockState) super.setUploadType(uploadType);
}
@Override
public GetSimLockState setUploadProtocol(java.lang.String uploadProtocol) {
return (GetSimLockState) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the partner. */
@com.google.api.client.util.Key
private java.lang.Long partnerId;
/** Required. The ID of the partner.
*/
public java.lang.Long getPartnerId() {
return partnerId;
}
/** Required. The ID of the partner. */
public GetSimLockState setPartnerId(java.lang.Long partnerId) {
this.partnerId = partnerId;
return this;
}
@Override
public GetSimLockState set(String parameterName, Object value) {
return (GetSimLockState) super.set(parameterName, value);
}
}
/**
* Updates reseller metadata associated with the device. Android devices only.
*
* Create a request for the method "devices.metadata".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Metadata#execute()} method to invoke the remote
* operation.
*
* @param metadataOwnerId Required. The owner of the newly set metadata. Set this to the partner ID.
* @param deviceId Required. The ID of the device.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.UpdateDeviceMetadataRequest}
* @return the request
*/
public Metadata metadata(java.lang.Long metadataOwnerId, java.lang.Long deviceId, com.google.api.services.androiddeviceprovisioning.v1.model.UpdateDeviceMetadataRequest content) throws java.io.IOException {
Metadata result = new Metadata(metadataOwnerId, deviceId, content);
initialize(result);
return result;
}
public class Metadata extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/partners/{+metadataOwnerId}/devices/{+deviceId}/metadata";
private final java.util.regex.Pattern METADATA_OWNER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
private final java.util.regex.Pattern DEVICE_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates reseller metadata associated with the device. Android devices only.
*
* Create a request for the method "devices.metadata".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Metadata#execute()} method to invoke the
* remote operation. {@link
* Metadata#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param metadataOwnerId Required. The owner of the newly set metadata. Set this to the partner ID.
* @param deviceId Required. The ID of the device.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.UpdateDeviceMetadataRequest}
* @since 1.13
*/
protected Metadata(java.lang.Long metadataOwnerId, java.lang.Long deviceId, com.google.api.services.androiddeviceprovisioning.v1.model.UpdateDeviceMetadataRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.DeviceMetadata.class);
this.metadataOwnerId = com.google.api.client.util.Preconditions.checkNotNull(metadataOwnerId, "Required parameter metadataOwnerId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
}
@Override
public Metadata set$Xgafv(java.lang.String $Xgafv) {
return (Metadata) super.set$Xgafv($Xgafv);
}
@Override
public Metadata setAccessToken(java.lang.String accessToken) {
return (Metadata) super.setAccessToken(accessToken);
}
@Override
public Metadata setAlt(java.lang.String alt) {
return (Metadata) super.setAlt(alt);
}
@Override
public Metadata setCallback(java.lang.String callback) {
return (Metadata) super.setCallback(callback);
}
@Override
public Metadata setFields(java.lang.String fields) {
return (Metadata) super.setFields(fields);
}
@Override
public Metadata setKey(java.lang.String key) {
return (Metadata) super.setKey(key);
}
@Override
public Metadata setOauthToken(java.lang.String oauthToken) {
return (Metadata) super.setOauthToken(oauthToken);
}
@Override
public Metadata setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Metadata) super.setPrettyPrint(prettyPrint);
}
@Override
public Metadata setQuotaUser(java.lang.String quotaUser) {
return (Metadata) super.setQuotaUser(quotaUser);
}
@Override
public Metadata setUploadType(java.lang.String uploadType) {
return (Metadata) super.setUploadType(uploadType);
}
@Override
public Metadata setUploadProtocol(java.lang.String uploadProtocol) {
return (Metadata) super.setUploadProtocol(uploadProtocol);
}
/** Required. The owner of the newly set metadata. Set this to the partner ID. */
@com.google.api.client.util.Key
private java.lang.Long metadataOwnerId;
/** Required. The owner of the newly set metadata. Set this to the partner ID.
*/
public java.lang.Long getMetadataOwnerId() {
return metadataOwnerId;
}
/** Required. The owner of the newly set metadata. Set this to the partner ID. */
public Metadata setMetadataOwnerId(java.lang.Long metadataOwnerId) {
this.metadataOwnerId = metadataOwnerId;
return this;
}
/** Required. The ID of the device. */
@com.google.api.client.util.Key
private java.lang.Long deviceId;
/** Required. The ID of the device.
*/
public java.lang.Long getDeviceId() {
return deviceId;
}
/** Required. The ID of the device. */
public Metadata setDeviceId(java.lang.Long deviceId) {
this.deviceId = deviceId;
return this;
}
@Override
public Metadata set(String parameterName, Object value) {
return (Metadata) super.set(parameterName, value);
}
}
/**
* Unclaims a device from a customer and removes it from zero-touch enrollment.
*
* Create a request for the method "devices.unclaim".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link Unclaim#execute()} method to invoke the remote
* operation.
*
* @param partnerId Required. The ID of the reseller partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.UnclaimDeviceRequest}
* @return the request
*/
public Unclaim unclaim(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.UnclaimDeviceRequest content) throws java.io.IOException {
Unclaim result = new Unclaim(partnerId, content);
initialize(result);
return result;
}
public class Unclaim extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/partners/{+partnerId}/devices:unclaim";
private final java.util.regex.Pattern PARTNER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Unclaims a device from a customer and removes it from zero-touch enrollment.
*
* Create a request for the method "devices.unclaim".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link Unclaim#execute()} method to invoke the remote
* operation. {@link
* Unclaim#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param partnerId Required. The ID of the reseller partner.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.UnclaimDeviceRequest}
* @since 1.13
*/
protected Unclaim(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.UnclaimDeviceRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.Empty.class);
this.partnerId = com.google.api.client.util.Preconditions.checkNotNull(partnerId, "Required parameter partnerId must be specified.");
}
@Override
public Unclaim set$Xgafv(java.lang.String $Xgafv) {
return (Unclaim) super.set$Xgafv($Xgafv);
}
@Override
public Unclaim setAccessToken(java.lang.String accessToken) {
return (Unclaim) super.setAccessToken(accessToken);
}
@Override
public Unclaim setAlt(java.lang.String alt) {
return (Unclaim) super.setAlt(alt);
}
@Override
public Unclaim setCallback(java.lang.String callback) {
return (Unclaim) super.setCallback(callback);
}
@Override
public Unclaim setFields(java.lang.String fields) {
return (Unclaim) super.setFields(fields);
}
@Override
public Unclaim setKey(java.lang.String key) {
return (Unclaim) super.setKey(key);
}
@Override
public Unclaim setOauthToken(java.lang.String oauthToken) {
return (Unclaim) super.setOauthToken(oauthToken);
}
@Override
public Unclaim setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Unclaim) super.setPrettyPrint(prettyPrint);
}
@Override
public Unclaim setQuotaUser(java.lang.String quotaUser) {
return (Unclaim) super.setQuotaUser(quotaUser);
}
@Override
public Unclaim setUploadType(java.lang.String uploadType) {
return (Unclaim) super.setUploadType(uploadType);
}
@Override
public Unclaim setUploadProtocol(java.lang.String uploadProtocol) {
return (Unclaim) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the reseller partner. */
@com.google.api.client.util.Key
private java.lang.Long partnerId;
/** Required. The ID of the reseller partner.
*/
public java.lang.Long getPartnerId() {
return partnerId;
}
/** Required. The ID of the reseller partner. */
public Unclaim setPartnerId(java.lang.Long partnerId) {
this.partnerId = partnerId;
return this;
}
@Override
public Unclaim set(String parameterName, Object value) {
return (Unclaim) super.set(parameterName, value);
}
}
/**
* Unclaims a batch of devices for a customer asynchronously. Removes the devices from zero-touch
* enrollment. To learn more, read [Long‑running batch operations](/zero-touch/guides/how-it-
* works#operations).
*
* Create a request for the method "devices.unclaimAsync".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link UnclaimAsync#execute()} method to invoke the remote
* operation.
*
* @param partnerId Required. The reseller partner ID.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.UnclaimDevicesRequest}
* @return the request
*/
public UnclaimAsync unclaimAsync(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.UnclaimDevicesRequest content) throws java.io.IOException {
UnclaimAsync result = new UnclaimAsync(partnerId, content);
initialize(result);
return result;
}
public class UnclaimAsync extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/partners/{+partnerId}/devices:unclaimAsync";
private final java.util.regex.Pattern PARTNER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Unclaims a batch of devices for a customer asynchronously. Removes the devices from zero-touch
* enrollment. To learn more, read [Long‑running batch operations](/zero-touch/guides/how-it-
* works#operations).
*
* Create a request for the method "devices.unclaimAsync".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link UnclaimAsync#execute()} method to invoke the
* remote operation. {@link
* UnclaimAsync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param partnerId Required. The reseller partner ID.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.UnclaimDevicesRequest}
* @since 1.13
*/
protected UnclaimAsync(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.UnclaimDevicesRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.Operation.class);
this.partnerId = com.google.api.client.util.Preconditions.checkNotNull(partnerId, "Required parameter partnerId must be specified.");
}
@Override
public UnclaimAsync set$Xgafv(java.lang.String $Xgafv) {
return (UnclaimAsync) super.set$Xgafv($Xgafv);
}
@Override
public UnclaimAsync setAccessToken(java.lang.String accessToken) {
return (UnclaimAsync) super.setAccessToken(accessToken);
}
@Override
public UnclaimAsync setAlt(java.lang.String alt) {
return (UnclaimAsync) super.setAlt(alt);
}
@Override
public UnclaimAsync setCallback(java.lang.String callback) {
return (UnclaimAsync) super.setCallback(callback);
}
@Override
public UnclaimAsync setFields(java.lang.String fields) {
return (UnclaimAsync) super.setFields(fields);
}
@Override
public UnclaimAsync setKey(java.lang.String key) {
return (UnclaimAsync) super.setKey(key);
}
@Override
public UnclaimAsync setOauthToken(java.lang.String oauthToken) {
return (UnclaimAsync) super.setOauthToken(oauthToken);
}
@Override
public UnclaimAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UnclaimAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public UnclaimAsync setQuotaUser(java.lang.String quotaUser) {
return (UnclaimAsync) super.setQuotaUser(quotaUser);
}
@Override
public UnclaimAsync setUploadType(java.lang.String uploadType) {
return (UnclaimAsync) super.setUploadType(uploadType);
}
@Override
public UnclaimAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (UnclaimAsync) super.setUploadProtocol(uploadProtocol);
}
/** Required. The reseller partner ID. */
@com.google.api.client.util.Key
private java.lang.Long partnerId;
/** Required. The reseller partner ID.
*/
public java.lang.Long getPartnerId() {
return partnerId;
}
/** Required. The reseller partner ID. */
public UnclaimAsync setPartnerId(java.lang.Long partnerId) {
this.partnerId = partnerId;
return this;
}
@Override
public UnclaimAsync set(String parameterName, Object value) {
return (UnclaimAsync) super.set(parameterName, value);
}
}
/**
* Updates the reseller metadata attached to a batch of devices. This method updates devices
* asynchronously and returns an `Operation` that can be used to track progress. Read [Long‑running
* batch operations](/zero-touch/guides/how-it-works#operations). Android Devices only.
*
* Create a request for the method "devices.updateMetadataAsync".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link UpdateMetadataAsync#execute()} method to invoke the
* remote operation.
*
* @param partnerId Required. The reseller partner ID.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.UpdateDeviceMetadataInBatchRequest}
* @return the request
*/
public UpdateMetadataAsync updateMetadataAsync(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.UpdateDeviceMetadataInBatchRequest content) throws java.io.IOException {
UpdateMetadataAsync result = new UpdateMetadataAsync(partnerId, content);
initialize(result);
return result;
}
public class UpdateMetadataAsync extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/partners/{+partnerId}/devices:updateMetadataAsync";
private final java.util.regex.Pattern PARTNER_ID_PATTERN =
java.util.regex.Pattern.compile("^[^/]+$");
/**
* Updates the reseller metadata attached to a batch of devices. This method updates devices
* asynchronously and returns an `Operation` that can be used to track progress. Read
* [Long‑running batch operations](/zero-touch/guides/how-it-works#operations). Android Devices
* only.
*
* Create a request for the method "devices.updateMetadataAsync".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link UpdateMetadataAsync#execute()} method to
* invoke the remote operation. {@link UpdateMetadataAsync#initialize(com.google.api.client.go
* ogleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param partnerId Required. The reseller partner ID.
* @param content the {@link com.google.api.services.androiddeviceprovisioning.v1.model.UpdateDeviceMetadataInBatchRequest}
* @since 1.13
*/
protected UpdateMetadataAsync(java.lang.Long partnerId, com.google.api.services.androiddeviceprovisioning.v1.model.UpdateDeviceMetadataInBatchRequest content) {
super(AndroidProvisioningPartner.this, "POST", REST_PATH, content, com.google.api.services.androiddeviceprovisioning.v1.model.Operation.class);
this.partnerId = com.google.api.client.util.Preconditions.checkNotNull(partnerId, "Required parameter partnerId must be specified.");
}
@Override
public UpdateMetadataAsync set$Xgafv(java.lang.String $Xgafv) {
return (UpdateMetadataAsync) super.set$Xgafv($Xgafv);
}
@Override
public UpdateMetadataAsync setAccessToken(java.lang.String accessToken) {
return (UpdateMetadataAsync) super.setAccessToken(accessToken);
}
@Override
public UpdateMetadataAsync setAlt(java.lang.String alt) {
return (UpdateMetadataAsync) super.setAlt(alt);
}
@Override
public UpdateMetadataAsync setCallback(java.lang.String callback) {
return (UpdateMetadataAsync) super.setCallback(callback);
}
@Override
public UpdateMetadataAsync setFields(java.lang.String fields) {
return (UpdateMetadataAsync) super.setFields(fields);
}
@Override
public UpdateMetadataAsync setKey(java.lang.String key) {
return (UpdateMetadataAsync) super.setKey(key);
}
@Override
public UpdateMetadataAsync setOauthToken(java.lang.String oauthToken) {
return (UpdateMetadataAsync) super.setOauthToken(oauthToken);
}
@Override
public UpdateMetadataAsync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateMetadataAsync) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateMetadataAsync setQuotaUser(java.lang.String quotaUser) {
return (UpdateMetadataAsync) super.setQuotaUser(quotaUser);
}
@Override
public UpdateMetadataAsync setUploadType(java.lang.String uploadType) {
return (UpdateMetadataAsync) super.setUploadType(uploadType);
}
@Override
public UpdateMetadataAsync setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateMetadataAsync) super.setUploadProtocol(uploadProtocol);
}
/** Required. The reseller partner ID. */
@com.google.api.client.util.Key
private java.lang.Long partnerId;
/** Required. The reseller partner ID.
*/
public java.lang.Long getPartnerId() {
return partnerId;
}
/** Required. The reseller partner ID. */
public UpdateMetadataAsync setPartnerId(java.lang.Long partnerId) {
this.partnerId = partnerId;
return this;
}
@Override
public UpdateMetadataAsync set(String parameterName, Object value) {
return (UpdateMetadataAsync) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Vendors collection.
*
* The typical use is:
*
* {@code AndroidProvisioningPartner androiddeviceprovisioning = new AndroidProvisioningPartner(...);}
* {@code AndroidProvisioningPartner.Vendors.List request = androiddeviceprovisioning.vendors().list(parameters ...)}
*
*
* @return the resource collection
*/
public Vendors vendors() {
return new Vendors();
}
/**
* The "vendors" collection of methods.
*/
public class Vendors {
/**
* Lists the vendors of the partner.
*
* Create a request for the method "vendors.list".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name in the format `partners/[PARTNER_ID]`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+parent}/vendors";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+$");
/**
* Lists the vendors of the partner.
*
* Create a request for the method "vendors.list".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name in the format `partners/[PARTNER_ID]`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AndroidProvisioningPartner.this, "GET", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.ListVendorsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The resource name in the format `partners/[PARTNER_ID]`. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name in the format `partners/[PARTNER_ID]`.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The resource name in the format `partners/[PARTNER_ID]`. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+$");
}
this.parent = parent;
return this;
}
/** The maximum number of results to be returned. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to be returned.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to be returned. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token identifying a page of results returned by the server. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results returned by the server.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token identifying a page of results returned by the server. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Customers collection.
*
* The typical use is:
*
* {@code AndroidProvisioningPartner androiddeviceprovisioning = new AndroidProvisioningPartner(...);}
* {@code AndroidProvisioningPartner.Customers.List request = androiddeviceprovisioning.customers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Customers customers() {
return new Customers();
}
/**
* The "customers" collection of methods.
*/
public class Customers {
/**
* Lists the customers of the vendor.
*
* Create a request for the method "customers.list".
*
* This request holds the parameters needed by the androiddeviceprovisioning server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource name in the format `partners/[PARTNER_ID]/vendors/[VENDOR_ID]`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AndroidProvisioningPartnerRequest {
private static final String REST_PATH = "v1/{+parent}/customers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^partners/[^/]+/vendors/[^/]+$");
/**
* Lists the customers of the vendor.
*
* Create a request for the method "customers.list".
*
* This request holds the parameters needed by the the androiddeviceprovisioning server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource name in the format `partners/[PARTNER_ID]/vendors/[VENDOR_ID]`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AndroidProvisioningPartner.this, "GET", REST_PATH, null, com.google.api.services.androiddeviceprovisioning.v1.model.ListVendorCustomersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+/vendors/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name in the format `partners/[PARTNER_ID]/vendors/[VENDOR_ID]`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource name in the format `partners/[PARTNER_ID]/vendors/[VENDOR_ID]`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource name in the format `partners/[PARTNER_ID]/vendors/[VENDOR_ID]`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^partners/[^/]+/vendors/[^/]+$");
}
this.parent = parent;
return this;
}
/** The maximum number of results to be returned. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to be returned.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to be returned. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token identifying a page of results returned by the server. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results returned by the server.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token identifying a page of results returned by the server. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* Builder for {@link AndroidProvisioningPartner}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link AndroidProvisioningPartner}. */
@Override
public AndroidProvisioningPartner build() {
return new AndroidProvisioningPartner(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link AndroidProvisioningPartnerRequestInitializer}.
*
* @since 1.12
*/
public Builder setAndroidProvisioningPartnerRequestInitializer(
AndroidProvisioningPartnerRequestInitializer androidprovisioningpartnerRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(androidprovisioningpartnerRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}